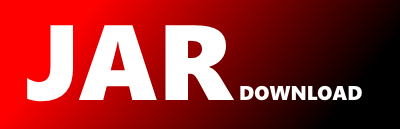
com.amazonaws.services.simplesystemsmanagement.model.Target Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An array of search criteria that targets managed nodes using a key-value pair that you specify.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task, targets
* are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more information
* about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* Supported formats include the following.
*
*
* For all Systems Manager capabilities:
*
*
* -
*
* Key=tag-key,Values=tag-value-1,tag-value-2
*
*
*
*
* For Automation and Change Manager:
*
*
* -
*
* Key=tag:tag-key,Values=tag-value
*
*
* -
*
* Key=ResourceGroup,Values=resource-group-name
*
*
* -
*
* Key=ParameterValues,Values=value-1,value-2,value-3
*
*
* -
*
* To target all instances in the Amazon Web Services Region:
*
*
* -
*
* Key=AWS::EC2::Instance,Values=*
*
*
* -
*
* Key=InstanceIds,Values=*
*
*
*
*
*
*
* For Run Command and Maintenance Windows:
*
*
* -
*
* Key=InstanceIds,Values=instance-id-1,instance-id-2,instance-id-3
*
*
* -
*
* Key=tag:tag-key,Values=tag-value-1,tag-value-2
*
*
* -
*
* Key=resource-groups:Name,Values=resource-group-name
*
*
* -
*
* Additionally, Maintenance Windows support targeting resource types:
*
*
* -
*
* Key=resource-groups:ResourceTypeFilters,Values=resource-type-1,resource-type-2
*
*
*
*
*
*
* For State Manager:
*
*
* -
*
* Key=InstanceIds,Values=instance-id-1,instance-id-2,instance-id-3
*
*
* -
*
* Key=tag:tag-key,Values=tag-value-1,tag-value-2
*
*
* -
*
* To target all instances in the Amazon Web Services Region:
*
*
* -
*
* Key=InstanceIds,Values=*
*
*
*
*
*
*
* For more information about how to send commands that target managed nodes using Key,Value
parameters,
* see Targeting multiple managed nodes in the Amazon Web Services Systems Manager User Guide.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Target implements Serializable, Cloneable, StructuredPojo {
/**
*
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
*
*/
private String key;
/**
*
* User-defined criteria that maps to Key
. For example, if you specified tag:ServerRole
,
* you could specify value:WebServer
to run a command on instances that include EC2 tags of
* ServerRole,WebServer
.
*
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global maximum of
* 50.
*
*/
private com.amazonaws.internal.SdkInternalList values;
/**
*
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
*
*
* @param key
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
*/
public void setKey(String key) {
this.key = key;
}
/**
*
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
*
*
* @return User-defined criteria for sending commands that target managed nodes that meet the criteria.
*/
public String getKey() {
return this.key;
}
/**
*
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
*
*
* @param key
* User-defined criteria for sending commands that target managed nodes that meet the criteria.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withKey(String key) {
setKey(key);
return this;
}
/**
*
* User-defined criteria that maps to Key
. For example, if you specified tag:ServerRole
,
* you could specify value:WebServer
to run a command on instances that include EC2 tags of
* ServerRole,WebServer
.
*
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global maximum of
* 50.
*
*
* @return User-defined criteria that maps to Key
. For example, if you specified
* tag:ServerRole
, you could specify value:WebServer
to run a command on instances
* that include EC2 tags of ServerRole,WebServer
.
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global
* maximum of 50.
*/
public java.util.List getValues() {
if (values == null) {
values = new com.amazonaws.internal.SdkInternalList();
}
return values;
}
/**
*
* User-defined criteria that maps to Key
. For example, if you specified tag:ServerRole
,
* you could specify value:WebServer
to run a command on instances that include EC2 tags of
* ServerRole,WebServer
.
*
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global maximum of
* 50.
*
*
* @param values
* User-defined criteria that maps to Key
. For example, if you specified
* tag:ServerRole
, you could specify value:WebServer
to run a command on instances
* that include EC2 tags of ServerRole,WebServer
.
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global
* maximum of 50.
*/
public void setValues(java.util.Collection values) {
if (values == null) {
this.values = null;
return;
}
this.values = new com.amazonaws.internal.SdkInternalList(values);
}
/**
*
* User-defined criteria that maps to Key
. For example, if you specified tag:ServerRole
,
* you could specify value:WebServer
to run a command on instances that include EC2 tags of
* ServerRole,WebServer
.
*
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global maximum of
* 50.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValues(java.util.Collection)} or {@link #withValues(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param values
* User-defined criteria that maps to Key
. For example, if you specified
* tag:ServerRole
, you could specify value:WebServer
to run a command on instances
* that include EC2 tags of ServerRole,WebServer
.
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global
* maximum of 50.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withValues(String... values) {
if (this.values == null) {
setValues(new com.amazonaws.internal.SdkInternalList(values.length));
}
for (String ele : values) {
this.values.add(ele);
}
return this;
}
/**
*
* User-defined criteria that maps to Key
. For example, if you specified tag:ServerRole
,
* you could specify value:WebServer
to run a command on instances that include EC2 tags of
* ServerRole,WebServer
.
*
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global maximum of
* 50.
*
*
* @param values
* User-defined criteria that maps to Key
. For example, if you specified
* tag:ServerRole
, you could specify value:WebServer
to run a command on instances
* that include EC2 tags of ServerRole,WebServer
.
*
* Depending on the type of target, the maximum number of values for a key might be lower than the global
* maximum of 50.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Target withValues(java.util.Collection values) {
setValues(values);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getKey() != null)
sb.append("Key: ").append(getKey()).append(",");
if (getValues() != null)
sb.append("Values: ").append(getValues());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Target == false)
return false;
Target other = (Target) obj;
if (other.getKey() == null ^ this.getKey() == null)
return false;
if (other.getKey() != null && other.getKey().equals(this.getKey()) == false)
return false;
if (other.getValues() == null ^ this.getValues() == null)
return false;
if (other.getValues() != null && other.getValues().equals(this.getValues()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getKey() == null) ? 0 : getKey().hashCode());
hashCode = prime * hashCode + ((getValues() == null) ? 0 : getValues().hashCode());
return hashCode;
}
@Override
public Target clone() {
try {
return (Target) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.simplesystemsmanagement.model.transform.TargetMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}