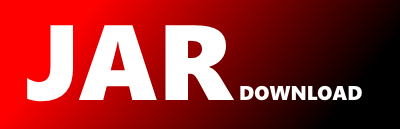
com.amazonaws.services.simplesystemsmanagement.model.UpdateMaintenanceWindowTargetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateMaintenanceWindowTargetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The maintenance window ID with which to modify the target.
*
*/
private String windowId;
/**
*
* The target ID to modify.
*
*/
private String windowTargetId;
/**
*
* The targets to add or replace.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running tasks for
* these targets in this maintenance window.
*
*/
private String ownerInformation;
/**
*
* A name for the update.
*
*/
private String name;
/**
*
* An optional description for the update.
*
*/
private String description;
/**
*
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*
*/
private Boolean replace;
/**
*
* The maintenance window ID with which to modify the target.
*
*
* @param windowId
* The maintenance window ID with which to modify the target.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The maintenance window ID with which to modify the target.
*
*
* @return The maintenance window ID with which to modify the target.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The maintenance window ID with which to modify the target.
*
*
* @param windowId
* The maintenance window ID with which to modify the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The target ID to modify.
*
*
* @param windowTargetId
* The target ID to modify.
*/
public void setWindowTargetId(String windowTargetId) {
this.windowTargetId = windowTargetId;
}
/**
*
* The target ID to modify.
*
*
* @return The target ID to modify.
*/
public String getWindowTargetId() {
return this.windowTargetId;
}
/**
*
* The target ID to modify.
*
*
* @param windowTargetId
* The target ID to modify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withWindowTargetId(String windowTargetId) {
setWindowTargetId(windowTargetId);
return this;
}
/**
*
* The targets to add or replace.
*
*
* @return The targets to add or replace.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets to add or replace.
*
*
* @param targets
* The targets to add or replace.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets to add or replace.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets to add or replace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets to add or replace.
*
*
* @param targets
* The targets to add or replace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running tasks for
* these targets in this maintenance window.
*
*
* @param ownerInformation
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running
* tasks for these targets in this maintenance window.
*/
public void setOwnerInformation(String ownerInformation) {
this.ownerInformation = ownerInformation;
}
/**
*
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running tasks for
* these targets in this maintenance window.
*
*
* @return User-provided value that will be included in any Amazon CloudWatch Events events raised while running
* tasks for these targets in this maintenance window.
*/
public String getOwnerInformation() {
return this.ownerInformation;
}
/**
*
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running tasks for
* these targets in this maintenance window.
*
*
* @param ownerInformation
* User-provided value that will be included in any Amazon CloudWatch Events events raised while running
* tasks for these targets in this maintenance window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withOwnerInformation(String ownerInformation) {
setOwnerInformation(ownerInformation);
return this;
}
/**
*
* A name for the update.
*
*
* @param name
* A name for the update.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A name for the update.
*
*
* @return A name for the update.
*/
public String getName() {
return this.name;
}
/**
*
* A name for the update.
*
*
* @param name
* A name for the update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withName(String name) {
setName(name);
return this;
}
/**
*
* An optional description for the update.
*
*
* @param description
* An optional description for the update.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* An optional description for the update.
*
*
* @return An optional description for the update.
*/
public String getDescription() {
return this.description;
}
/**
*
* An optional description for the update.
*
*
* @param description
* An optional description for the update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*/
public void setReplace(Boolean replace) {
this.replace = replace;
}
/**
*
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*
*
* @return If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean getReplace() {
return this.replace;
}
/**
*
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetRequest withReplace(Boolean replace) {
setReplace(replace);
return this;
}
/**
*
* If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*
*
* @return If True
, then all fields that are required by the RegisterTargetWithMaintenanceWindow
* operation are also required for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean isReplace() {
return this.replace;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTargetId() != null)
sb.append("WindowTargetId: ").append(getWindowTargetId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getOwnerInformation() != null)
sb.append("OwnerInformation: ").append("***Sensitive Data Redacted***").append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getReplace() != null)
sb.append("Replace: ").append(getReplace());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateMaintenanceWindowTargetRequest == false)
return false;
UpdateMaintenanceWindowTargetRequest other = (UpdateMaintenanceWindowTargetRequest) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTargetId() == null ^ this.getWindowTargetId() == null)
return false;
if (other.getWindowTargetId() != null && other.getWindowTargetId().equals(this.getWindowTargetId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getOwnerInformation() == null ^ this.getOwnerInformation() == null)
return false;
if (other.getOwnerInformation() != null && other.getOwnerInformation().equals(this.getOwnerInformation()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getReplace() == null ^ this.getReplace() == null)
return false;
if (other.getReplace() != null && other.getReplace().equals(this.getReplace()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTargetId() == null) ? 0 : getWindowTargetId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getOwnerInformation() == null) ? 0 : getOwnerInformation().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getReplace() == null) ? 0 : getReplace().hashCode());
return hashCode;
}
@Override
public UpdateMaintenanceWindowTargetRequest clone() {
return (UpdateMaintenanceWindowTargetRequest) super.clone();
}
}