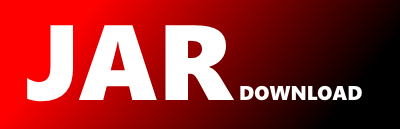
com.amazonaws.services.simplesystemsmanagement.model.UpdateMaintenanceWindowTargetResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateMaintenanceWindowTargetResult extends com.amazonaws.AmazonWebServiceResult implements Serializable,
Cloneable {
/**
*
* The maintenance window ID specified in the update request.
*
*/
private String windowId;
/**
*
* The target ID specified in the update request.
*
*/
private String windowTargetId;
/**
*
* The updated targets.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The updated owner.
*
*/
private String ownerInformation;
/**
*
* The updated name.
*
*/
private String name;
/**
*
* The updated description.
*
*/
private String description;
/**
*
* The maintenance window ID specified in the update request.
*
*
* @param windowId
* The maintenance window ID specified in the update request.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The maintenance window ID specified in the update request.
*
*
* @return The maintenance window ID specified in the update request.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The maintenance window ID specified in the update request.
*
*
* @param windowId
* The maintenance window ID specified in the update request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The target ID specified in the update request.
*
*
* @param windowTargetId
* The target ID specified in the update request.
*/
public void setWindowTargetId(String windowTargetId) {
this.windowTargetId = windowTargetId;
}
/**
*
* The target ID specified in the update request.
*
*
* @return The target ID specified in the update request.
*/
public String getWindowTargetId() {
return this.windowTargetId;
}
/**
*
* The target ID specified in the update request.
*
*
* @param windowTargetId
* The target ID specified in the update request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withWindowTargetId(String windowTargetId) {
setWindowTargetId(windowTargetId);
return this;
}
/**
*
* The updated targets.
*
*
* @return The updated targets.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The updated targets.
*
*
* @param targets
* The updated targets.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The updated targets.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The updated targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The updated targets.
*
*
* @param targets
* The updated targets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The updated owner.
*
*
* @param ownerInformation
* The updated owner.
*/
public void setOwnerInformation(String ownerInformation) {
this.ownerInformation = ownerInformation;
}
/**
*
* The updated owner.
*
*
* @return The updated owner.
*/
public String getOwnerInformation() {
return this.ownerInformation;
}
/**
*
* The updated owner.
*
*
* @param ownerInformation
* The updated owner.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withOwnerInformation(String ownerInformation) {
setOwnerInformation(ownerInformation);
return this;
}
/**
*
* The updated name.
*
*
* @param name
* The updated name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The updated name.
*
*
* @return The updated name.
*/
public String getName() {
return this.name;
}
/**
*
* The updated name.
*
*
* @param name
* The updated name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withName(String name) {
setName(name);
return this;
}
/**
*
* The updated description.
*
*
* @param description
* The updated description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The updated description.
*
*
* @return The updated description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The updated description.
*
*
* @param description
* The updated description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTargetResult withDescription(String description) {
setDescription(description);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTargetId() != null)
sb.append("WindowTargetId: ").append(getWindowTargetId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getOwnerInformation() != null)
sb.append("OwnerInformation: ").append("***Sensitive Data Redacted***").append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateMaintenanceWindowTargetResult == false)
return false;
UpdateMaintenanceWindowTargetResult other = (UpdateMaintenanceWindowTargetResult) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTargetId() == null ^ this.getWindowTargetId() == null)
return false;
if (other.getWindowTargetId() != null && other.getWindowTargetId().equals(this.getWindowTargetId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getOwnerInformation() == null ^ this.getOwnerInformation() == null)
return false;
if (other.getOwnerInformation() != null && other.getOwnerInformation().equals(this.getOwnerInformation()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTargetId() == null) ? 0 : getWindowTargetId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getOwnerInformation() == null) ? 0 : getOwnerInformation().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
return hashCode;
}
@Override
public UpdateMaintenanceWindowTargetResult clone() {
try {
return (UpdateMaintenanceWindowTargetResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}