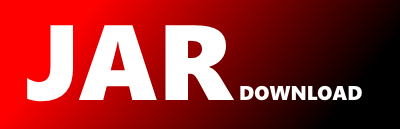
com.amazonaws.services.simplesystemsmanagement.model.UpdateMaintenanceWindowTaskRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateMaintenanceWindowTaskRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The maintenance window ID that contains the task to modify.
*
*/
private String windowId;
/**
*
* The task ID to modify.
*
*/
private String windowTaskId;
/**
*
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The task ARN to modify.
*
*/
private String taskArn;
/**
*
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume when
* running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in your
* account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom service role
* for running your maintenance window tasks. The policy can be crafted to provide only the permissions needed for
* your particular maintenance window tasks. For more information, see Setting
* up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*
*/
private String serviceRoleArn;
/**
*
* The parameters to modify.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*
*/
private java.util.Map taskParameters;
/**
*
* The parameters that the task should use during execution. Populate only the fields that match the task type. All
* other fields should be empty.
*
*
*
* When you update a maintenance window task that has options specified in TaskInvocationParameters
,
* you must provide again all the TaskInvocationParameters
values that you want to retain. The values
* you don't specify again are removed. For example, suppose that when you registered a Run Command task, you
* specified TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a different
* OutputS3BucketName
value, the values for Comment
and NotificationConfig
* are removed.
*
*
*/
private MaintenanceWindowTaskInvocationParameters taskInvocationParameters;
/**
*
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*/
private Integer priority;
/**
*
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number of
* targets that are allowed to run this task, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*/
private String maxConcurrency;
/**
*
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors that are
* allowed before the task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*/
private String maxErrors;
/**
*
* The new logging location in Amazon S3 to specify.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private LoggingInfo loggingInfo;
/**
*
* The new task name to specify.
*
*/
private String name;
/**
*
* The new task description to specify.
*
*/
private String description;
/**
*
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are also
* required for this API request. Optional fields that aren't specified are set to null.
*
*/
private Boolean replace;
/**
*
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows is
* reached.
*
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The default
* value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that are
* already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation that
* attempts to cancel the command associated with the task. However, there is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
*
*/
private String cutoffBehavior;
/**
*
* The CloudWatch alarm you want to apply to your maintenance window task.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The maintenance window ID that contains the task to modify.
*
*
* @param windowId
* The maintenance window ID that contains the task to modify.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The maintenance window ID that contains the task to modify.
*
*
* @return The maintenance window ID that contains the task to modify.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The maintenance window ID that contains the task to modify.
*
*
* @param windowId
* The maintenance window ID that contains the task to modify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The task ID to modify.
*
*
* @param windowTaskId
* The task ID to modify.
*/
public void setWindowTaskId(String windowTaskId) {
this.windowTaskId = windowTaskId;
}
/**
*
* The task ID to modify.
*
*
* @return The task ID to modify.
*/
public String getWindowTaskId() {
return this.windowTaskId;
}
/**
*
* The task ID to modify.
*
*
* @param windowTaskId
* The task ID to modify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withWindowTaskId(String windowTaskId) {
setWindowTaskId(windowTaskId);
return this;
}
/**
*
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* @return The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the
* task, targets are optional for other maintenance window task types (Automation, Lambda, and Step
* Functions). For more information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager
* User Guide.
*
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* @param targets
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the
* task, targets are optional for other maintenance window task types (Automation, Lambda, and Step
* Functions). For more information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager
* User Guide.
*
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the
* task, targets are optional for other maintenance window task types (Automation, Lambda, and Step
* Functions). For more information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager
* User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
*
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the task,
* targets are optional for other maintenance window task types (Automation, Lambda, and Step Functions). For more
* information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager User
* Guide.
*
*
*
* @param targets
* The targets (either managed nodes or tags) to modify. Managed nodes are specified using the format
* Key=instanceids,Values=instanceID_1,instanceID_2
. Tags are specified using the format
* Key=tag_name,Values=tag_value
.
*
* One or more targets must be specified for maintenance window Run Command-type tasks. Depending on the
* task, targets are optional for other maintenance window task types (Automation, Lambda, and Step
* Functions). For more information about running tasks that don't specify targets, see Registering maintenance window tasks without targets in the Amazon Web Services Systems Manager
* User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The task ARN to modify.
*
*
* @param taskArn
* The task ARN to modify.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The task ARN to modify.
*
*
* @return The task ARN to modify.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The task ARN to modify.
*
*
* @param taskArn
* The task ARN to modify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume when
* running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in your
* account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom service role
* for running your maintenance window tasks. The policy can be crafted to provide only the permissions needed for
* your particular maintenance window tasks. For more information, see Setting
* up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume
* when running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in
* your account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom
* service role for running your maintenance window tasks. The policy can be crafted to provide only the
* permissions needed for your particular maintenance window tasks. For more information, see Setting up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume when
* running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in your
* account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom service role
* for running your maintenance window tasks. The policy can be crafted to provide only the permissions needed for
* your particular maintenance window tasks. For more information, see Setting
* up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume
* when running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in
* your account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom
* service role for running your maintenance window tasks. The policy can be crafted to provide only the
* permissions needed for your particular maintenance window tasks. For more information, see Setting up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume when
* running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in your
* account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom service role
* for running your maintenance window tasks. The policy can be crafted to provide only the permissions needed for
* your particular maintenance window tasks. For more information, see Setting
* up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the IAM service role for Amazon Web Services Systems Manager to assume
* when running a maintenance window task. If you do not specify a service role ARN, Systems Manager uses a
* service-linked role in your account. If no appropriate service-linked role for Systems Manager exists in
* your account, it is created when you run RegisterTaskWithMaintenanceWindow
.
*
* However, for an improved security posture, we strongly recommend creating a custom policy and custom
* service role for running your maintenance window tasks. The policy can be crafted to provide only the
* permissions needed for your particular maintenance window tasks. For more information, see Setting up maintenance windows in the in the Amazon Web Services Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* The parameters to modify.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*
*
* @return The parameters to modify.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure.
* For information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*/
public java.util.Map getTaskParameters() {
return taskParameters;
}
/**
*
* The parameters to modify.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*
*
* @param taskParameters
* The parameters to modify.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*/
public void setTaskParameters(java.util.Map taskParameters) {
this.taskParameters = taskParameters;
}
/**
*
* The parameters to modify.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
*
*
* @param taskParameters
* The parameters to modify.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*
*
* The map has the following format:
*
*
* Key: string, between 1 and 255 characters
*
*
* Value: an array of strings, each string is between 1 and 255 characters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withTaskParameters(java.util.Map taskParameters) {
setTaskParameters(taskParameters);
return this;
}
/**
* Add a single TaskParameters entry
*
* @see UpdateMaintenanceWindowTaskRequest#withTaskParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest addTaskParametersEntry(String key, MaintenanceWindowTaskParameterValueExpression value) {
if (null == this.taskParameters) {
this.taskParameters = new java.util.HashMap();
}
if (this.taskParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.taskParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into TaskParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest clearTaskParametersEntries() {
this.taskParameters = null;
return this;
}
/**
*
* The parameters that the task should use during execution. Populate only the fields that match the task type. All
* other fields should be empty.
*
*
*
* When you update a maintenance window task that has options specified in TaskInvocationParameters
,
* you must provide again all the TaskInvocationParameters
values that you want to retain. The values
* you don't specify again are removed. For example, suppose that when you registered a Run Command task, you
* specified TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a different
* OutputS3BucketName
value, the values for Comment
and NotificationConfig
* are removed.
*
*
*
* @param taskInvocationParameters
* The parameters that the task should use during execution. Populate only the fields that match the task
* type. All other fields should be empty.
*
* When you update a maintenance window task that has options specified in
* TaskInvocationParameters
, you must provide again all the
* TaskInvocationParameters
values that you want to retain. The values you don't specify again
* are removed. For example, suppose that when you registered a Run Command task, you specified
* TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a
* different OutputS3BucketName
value, the values for Comment
and
* NotificationConfig
are removed.
*
*/
public void setTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
this.taskInvocationParameters = taskInvocationParameters;
}
/**
*
* The parameters that the task should use during execution. Populate only the fields that match the task type. All
* other fields should be empty.
*
*
*
* When you update a maintenance window task that has options specified in TaskInvocationParameters
,
* you must provide again all the TaskInvocationParameters
values that you want to retain. The values
* you don't specify again are removed. For example, suppose that when you registered a Run Command task, you
* specified TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a different
* OutputS3BucketName
value, the values for Comment
and NotificationConfig
* are removed.
*
*
*
* @return The parameters that the task should use during execution. Populate only the fields that match the task
* type. All other fields should be empty.
*
* When you update a maintenance window task that has options specified in
* TaskInvocationParameters
, you must provide again all the
* TaskInvocationParameters
values that you want to retain. The values you don't specify again
* are removed. For example, suppose that when you registered a Run Command task, you specified
* TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a
* different OutputS3BucketName
value, the values for Comment
and
* NotificationConfig
are removed.
*
*/
public MaintenanceWindowTaskInvocationParameters getTaskInvocationParameters() {
return this.taskInvocationParameters;
}
/**
*
* The parameters that the task should use during execution. Populate only the fields that match the task type. All
* other fields should be empty.
*
*
*
* When you update a maintenance window task that has options specified in TaskInvocationParameters
,
* you must provide again all the TaskInvocationParameters
values that you want to retain. The values
* you don't specify again are removed. For example, suppose that when you registered a Run Command task, you
* specified TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a different
* OutputS3BucketName
value, the values for Comment
and NotificationConfig
* are removed.
*
*
*
* @param taskInvocationParameters
* The parameters that the task should use during execution. Populate only the fields that match the task
* type. All other fields should be empty.
*
* When you update a maintenance window task that has options specified in
* TaskInvocationParameters
, you must provide again all the
* TaskInvocationParameters
values that you want to retain. The values you don't specify again
* are removed. For example, suppose that when you registered a Run Command task, you specified
* TaskInvocationParameters
values for Comment
, NotificationConfig
,
* and OutputS3BucketName
. If you update the maintenance window task and specify only a
* different OutputS3BucketName
value, the values for Comment
and
* NotificationConfig
are removed.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
setTaskInvocationParameters(taskInvocationParameters);
return this;
}
/**
*
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @param priority
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @return The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
*
*
* @param priority
* The new task priority to specify. The lower the number, the higher the priority. Tasks that have the same
* priority are scheduled in parallel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number of
* targets that are allowed to run this task, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxConcurrency
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number
* of targets that are allowed to run this task, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number of
* targets that are allowed to run this task, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @return The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number
* of targets that are allowed to run this task, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering
* or updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number of
* targets that are allowed to run this task, in parallel.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxConcurrency
* The new MaxConcurrency
value you want to specify. MaxConcurrency
is the number
* of targets that are allowed to run this task, in parallel.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors that are
* allowed before the task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxErrors
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors
* that are allowed before the task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors that are
* allowed before the task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @return The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors
* that are allowed before the task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering
* or updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors that are
* allowed before the task stops being scheduled.
*
*
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option. Instead, the
* system inserts a placeholder value of 1
. This value doesn't affect the running of your task.
*
*
*
* @param maxErrors
* The new MaxErrors
value to specify. MaxErrors
is the maximum number of errors
* that are allowed before the task stops being scheduled.
*
* Although this element is listed as "Required: No", a value can be omitted only when you are registering or
* updating a targetless task You must provide a value in all other cases.
*
*
* For maintenance window tasks without a target specified, you can't supply a value for this option.
* Instead, the system inserts a placeholder value of 1
. This value doesn't affect the running
* of your task.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The new logging location in Amazon S3 to specify.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The new logging location in Amazon S3 to specify.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*/
public void setLoggingInfo(LoggingInfo loggingInfo) {
this.loggingInfo = loggingInfo;
}
/**
*
* The new logging location in Amazon S3 to specify.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The new logging location in Amazon S3 to specify.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and
* OutputS3KeyPrefix
options in the TaskInvocationParameters
structure. For
* information about how Amazon Web Services Systems Manager handles these options for the supported
* maintenance window task types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public LoggingInfo getLoggingInfo() {
return this.loggingInfo;
}
/**
*
* The new logging location in Amazon S3 to specify.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The new logging location in Amazon S3 to specify.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withLoggingInfo(LoggingInfo loggingInfo) {
setLoggingInfo(loggingInfo);
return this;
}
/**
*
* The new task name to specify.
*
*
* @param name
* The new task name to specify.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The new task name to specify.
*
*
* @return The new task name to specify.
*/
public String getName() {
return this.name;
}
/**
*
* The new task name to specify.
*
*
* @param name
* The new task name to specify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withName(String name) {
setName(name);
return this;
}
/**
*
* The new task description to specify.
*
*
* @param description
* The new task description to specify.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The new task description to specify.
*
*
* @return The new task description to specify.
*/
public String getDescription() {
return this.description;
}
/**
*
* The new task description to specify.
*
*
* @param description
* The new task description to specify.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are also
* required for this API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are
* also required for this API request. Optional fields that aren't specified are set to null.
*/
public void setReplace(Boolean replace) {
this.replace = replace;
}
/**
*
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are also
* required for this API request. Optional fields that aren't specified are set to null.
*
*
* @return If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are
* also required for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean getReplace() {
return this.replace;
}
/**
*
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are also
* required for this API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are
* also required for this API request. Optional fields that aren't specified are set to null.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withReplace(Boolean replace) {
setReplace(replace);
return this;
}
/**
*
* If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are also
* required for this API request. Optional fields that aren't specified are set to null.
*
*
* @return If True, then all fields that are required by the RegisterTaskWithMaintenanceWindow operation are
* also required for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean isReplace() {
return this.replace;
}
/**
*
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows is
* reached.
*
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The default
* value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that are
* already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation that
* attempts to cancel the command associated with the task. However, there is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
*
*
* @param cutoffBehavior
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows
* is reached.
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The
* default value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that
* are already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation
* that attempts to cancel the command associated with the task. However, there is no guarantee that the
* command will be terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
* @see MaintenanceWindowTaskCutoffBehavior
*/
public void setCutoffBehavior(String cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior;
}
/**
*
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows is
* reached.
*
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The default
* value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that are
* already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation that
* attempts to cancel the command associated with the task. However, there is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
*
*
* @return Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows
* is reached.
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The
* default value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that
* are already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation
* that attempts to cancel the command associated with the task. However, there is no guarantee that the
* command will be terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
* @see MaintenanceWindowTaskCutoffBehavior
*/
public String getCutoffBehavior() {
return this.cutoffBehavior;
}
/**
*
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows is
* reached.
*
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The default
* value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that are
* already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation that
* attempts to cancel the command associated with the task. However, there is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
*
*
* @param cutoffBehavior
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows
* is reached.
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The
* default value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that
* are already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation
* that attempts to cancel the command associated with the task. However, there is no guarantee that the
* command will be terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public UpdateMaintenanceWindowTaskRequest withCutoffBehavior(String cutoffBehavior) {
setCutoffBehavior(cutoffBehavior);
return this;
}
/**
*
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows is
* reached.
*
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The default
* value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that are
* already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation that
* attempts to cancel the command associated with the task. However, there is no guarantee that the command will be
* terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
*
*
* @param cutoffBehavior
* Indicates whether tasks should continue to run after the cutoff time specified in the maintenance windows
* is reached.
*
* -
*
* CONTINUE_TASK
: When the cutoff time is reached, any tasks that are running continue. The
* default value.
*
*
* -
*
* CANCEL_TASK
:
*
*
* -
*
* For Automation, Lambda, Step Functions tasks: When the cutoff time is reached, any task invocations that
* are already running continue, but no new task invocations are started.
*
*
* -
*
* For Run Command tasks: When the cutoff time is reached, the system sends a CancelCommand operation
* that attempts to cancel the command associated with the task. However, there is no guarantee that the
* command will be terminated and the underlying process stopped.
*
*
*
*
* The status for tasks that are not completed is TIMED_OUT
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public UpdateMaintenanceWindowTaskRequest withCutoffBehavior(MaintenanceWindowTaskCutoffBehavior cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior.toString();
return this;
}
/**
*
* The CloudWatch alarm you want to apply to your maintenance window task.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your maintenance window task.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your maintenance window task.
*
*
* @return The CloudWatch alarm you want to apply to your maintenance window task.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The CloudWatch alarm you want to apply to your maintenance window task.
*
*
* @param alarmConfiguration
* The CloudWatch alarm you want to apply to your maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskRequest withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTaskId() != null)
sb.append("WindowTaskId: ").append(getWindowTaskId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getTaskParameters() != null)
sb.append("TaskParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getTaskInvocationParameters() != null)
sb.append("TaskInvocationParameters: ").append(getTaskInvocationParameters()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getLoggingInfo() != null)
sb.append("LoggingInfo: ").append(getLoggingInfo()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getReplace() != null)
sb.append("Replace: ").append(getReplace()).append(",");
if (getCutoffBehavior() != null)
sb.append("CutoffBehavior: ").append(getCutoffBehavior()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateMaintenanceWindowTaskRequest == false)
return false;
UpdateMaintenanceWindowTaskRequest other = (UpdateMaintenanceWindowTaskRequest) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTaskId() == null ^ this.getWindowTaskId() == null)
return false;
if (other.getWindowTaskId() != null && other.getWindowTaskId().equals(this.getWindowTaskId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getTaskParameters() == null ^ this.getTaskParameters() == null)
return false;
if (other.getTaskParameters() != null && other.getTaskParameters().equals(this.getTaskParameters()) == false)
return false;
if (other.getTaskInvocationParameters() == null ^ this.getTaskInvocationParameters() == null)
return false;
if (other.getTaskInvocationParameters() != null && other.getTaskInvocationParameters().equals(this.getTaskInvocationParameters()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getLoggingInfo() == null ^ this.getLoggingInfo() == null)
return false;
if (other.getLoggingInfo() != null && other.getLoggingInfo().equals(this.getLoggingInfo()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getReplace() == null ^ this.getReplace() == null)
return false;
if (other.getReplace() != null && other.getReplace().equals(this.getReplace()) == false)
return false;
if (other.getCutoffBehavior() == null ^ this.getCutoffBehavior() == null)
return false;
if (other.getCutoffBehavior() != null && other.getCutoffBehavior().equals(this.getCutoffBehavior()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTaskId() == null) ? 0 : getWindowTaskId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getTaskParameters() == null) ? 0 : getTaskParameters().hashCode());
hashCode = prime * hashCode + ((getTaskInvocationParameters() == null) ? 0 : getTaskInvocationParameters().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getLoggingInfo() == null) ? 0 : getLoggingInfo().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getReplace() == null) ? 0 : getReplace().hashCode());
hashCode = prime * hashCode + ((getCutoffBehavior() == null) ? 0 : getCutoffBehavior().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public UpdateMaintenanceWindowTaskRequest clone() {
return (UpdateMaintenanceWindowTaskRequest) super.clone();
}
}