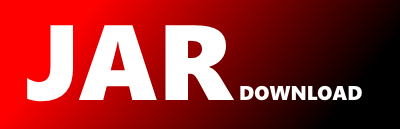
com.amazonaws.services.simplesystemsmanagement.model.UpdateMaintenanceWindowTaskResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateMaintenanceWindowTaskResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ID of the maintenance window that was updated.
*
*/
private String windowId;
/**
*
* The task ID of the maintenance window that was updated.
*
*/
private String windowTaskId;
/**
*
* The updated target values.
*
*/
private com.amazonaws.internal.SdkInternalList targets;
/**
*
* The updated task ARN value.
*
*/
private String taskArn;
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*/
private String serviceRoleArn;
/**
*
* The updated parameter values.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private java.util.Map taskParameters;
/**
*
* The updated parameter values.
*
*/
private MaintenanceWindowTaskInvocationParameters taskInvocationParameters;
/**
*
* The updated priority value.
*
*/
private Integer priority;
/**
*
* The updated MaxConcurrency
value.
*
*/
private String maxConcurrency;
/**
*
* The updated MaxErrors
value.
*
*/
private String maxErrors;
/**
*
* The updated logging information in Amazon S3.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*/
private LoggingInfo loggingInfo;
/**
*
* The updated task name.
*
*/
private String name;
/**
*
* The updated task description.
*
*/
private String description;
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*/
private String cutoffBehavior;
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*/
private AlarmConfiguration alarmConfiguration;
/**
*
* The ID of the maintenance window that was updated.
*
*
* @param windowId
* The ID of the maintenance window that was updated.
*/
public void setWindowId(String windowId) {
this.windowId = windowId;
}
/**
*
* The ID of the maintenance window that was updated.
*
*
* @return The ID of the maintenance window that was updated.
*/
public String getWindowId() {
return this.windowId;
}
/**
*
* The ID of the maintenance window that was updated.
*
*
* @param windowId
* The ID of the maintenance window that was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withWindowId(String windowId) {
setWindowId(windowId);
return this;
}
/**
*
* The task ID of the maintenance window that was updated.
*
*
* @param windowTaskId
* The task ID of the maintenance window that was updated.
*/
public void setWindowTaskId(String windowTaskId) {
this.windowTaskId = windowTaskId;
}
/**
*
* The task ID of the maintenance window that was updated.
*
*
* @return The task ID of the maintenance window that was updated.
*/
public String getWindowTaskId() {
return this.windowTaskId;
}
/**
*
* The task ID of the maintenance window that was updated.
*
*
* @param windowTaskId
* The task ID of the maintenance window that was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withWindowTaskId(String windowTaskId) {
setWindowTaskId(windowTaskId);
return this;
}
/**
*
* The updated target values.
*
*
* @return The updated target values.
*/
public java.util.List getTargets() {
if (targets == null) {
targets = new com.amazonaws.internal.SdkInternalList();
}
return targets;
}
/**
*
* The updated target values.
*
*
* @param targets
* The updated target values.
*/
public void setTargets(java.util.Collection targets) {
if (targets == null) {
this.targets = null;
return;
}
this.targets = new com.amazonaws.internal.SdkInternalList(targets);
}
/**
*
* The updated target values.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargets(java.util.Collection)} or {@link #withTargets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param targets
* The updated target values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withTargets(Target... targets) {
if (this.targets == null) {
setTargets(new com.amazonaws.internal.SdkInternalList(targets.length));
}
for (Target ele : targets) {
this.targets.add(ele);
}
return this;
}
/**
*
* The updated target values.
*
*
* @param targets
* The updated target values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withTargets(java.util.Collection targets) {
setTargets(targets);
return this;
}
/**
*
* The updated task ARN value.
*
*
* @param taskArn
* The updated task ARN value.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The updated task ARN value.
*
*
* @return The updated task ARN value.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The updated task ARN value.
*
*
* @param taskArn
* The updated task ARN value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public void setServiceRoleArn(String serviceRoleArn) {
this.serviceRoleArn = serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @return The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*/
public String getServiceRoleArn() {
return this.serviceRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish Amazon
* Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
*
*
* @param serviceRoleArn
* The Amazon Resource Name (ARN) of the Identity and Access Management (IAM) service role to use to publish
* Amazon Simple Notification Service (Amazon SNS) notifications for maintenance window Run Command tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withServiceRoleArn(String serviceRoleArn) {
setServiceRoleArn(serviceRoleArn);
return this;
}
/**
*
* The updated parameter values.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The updated parameter values.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure.
* For information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public java.util.Map getTaskParameters() {
return taskParameters;
}
/**
*
* The updated parameter values.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The updated parameter values.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public void setTaskParameters(java.util.Map taskParameters) {
this.taskParameters = taskParameters;
}
/**
*
* The updated parameter values.
*
*
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs, instead
* use the Parameters
option in the TaskInvocationParameters
structure. For information
* about how Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param taskParameters
* The updated parameter values.
*
* TaskParameters
has been deprecated. To specify parameters to pass to a task when it runs,
* instead use the Parameters
option in the TaskInvocationParameters
structure. For
* information about how Systems Manager handles these options for the supported maintenance window task
* types, see MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withTaskParameters(java.util.Map taskParameters) {
setTaskParameters(taskParameters);
return this;
}
/**
* Add a single TaskParameters entry
*
* @see UpdateMaintenanceWindowTaskResult#withTaskParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult addTaskParametersEntry(String key, MaintenanceWindowTaskParameterValueExpression value) {
if (null == this.taskParameters) {
this.taskParameters = new java.util.HashMap();
}
if (this.taskParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.taskParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into TaskParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult clearTaskParametersEntries() {
this.taskParameters = null;
return this;
}
/**
*
* The updated parameter values.
*
*
* @param taskInvocationParameters
* The updated parameter values.
*/
public void setTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
this.taskInvocationParameters = taskInvocationParameters;
}
/**
*
* The updated parameter values.
*
*
* @return The updated parameter values.
*/
public MaintenanceWindowTaskInvocationParameters getTaskInvocationParameters() {
return this.taskInvocationParameters;
}
/**
*
* The updated parameter values.
*
*
* @param taskInvocationParameters
* The updated parameter values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withTaskInvocationParameters(MaintenanceWindowTaskInvocationParameters taskInvocationParameters) {
setTaskInvocationParameters(taskInvocationParameters);
return this;
}
/**
*
* The updated priority value.
*
*
* @param priority
* The updated priority value.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The updated priority value.
*
*
* @return The updated priority value.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The updated priority value.
*
*
* @param priority
* The updated priority value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* The updated MaxConcurrency
value.
*
*
* @param maxConcurrency
* The updated MaxConcurrency
value.
*/
public void setMaxConcurrency(String maxConcurrency) {
this.maxConcurrency = maxConcurrency;
}
/**
*
* The updated MaxConcurrency
value.
*
*
* @return The updated MaxConcurrency
value.
*/
public String getMaxConcurrency() {
return this.maxConcurrency;
}
/**
*
* The updated MaxConcurrency
value.
*
*
* @param maxConcurrency
* The updated MaxConcurrency
value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withMaxConcurrency(String maxConcurrency) {
setMaxConcurrency(maxConcurrency);
return this;
}
/**
*
* The updated MaxErrors
value.
*
*
* @param maxErrors
* The updated MaxErrors
value.
*/
public void setMaxErrors(String maxErrors) {
this.maxErrors = maxErrors;
}
/**
*
* The updated MaxErrors
value.
*
*
* @return The updated MaxErrors
value.
*/
public String getMaxErrors() {
return this.maxErrors;
}
/**
*
* The updated MaxErrors
value.
*
*
* @param maxErrors
* The updated MaxErrors
value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withMaxErrors(String maxErrors) {
setMaxErrors(maxErrors);
return this;
}
/**
*
* The updated logging information in Amazon S3.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The updated logging information in Amazon S3.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*/
public void setLoggingInfo(LoggingInfo loggingInfo) {
this.loggingInfo = loggingInfo;
}
/**
*
* The updated logging information in Amazon S3.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @return The updated logging information in Amazon S3.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and
* OutputS3KeyPrefix
options in the TaskInvocationParameters
structure. For
* information about how Amazon Web Services Systems Manager handles these options for the supported
* maintenance window task types, see MaintenanceWindowTaskInvocationParameters.
*
*/
public LoggingInfo getLoggingInfo() {
return this.loggingInfo;
}
/**
*
* The updated logging information in Amazon S3.
*
*
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3) bucket to
* contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
options in the
* TaskInvocationParameters
structure. For information about how Amazon Web Services Systems Manager
* handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
*
*
* @param loggingInfo
* The updated logging information in Amazon S3.
*
* LoggingInfo
has been deprecated. To specify an Amazon Simple Storage Service (Amazon S3)
* bucket to contain logs, instead use the OutputS3BucketName
and OutputS3KeyPrefix
* options in the TaskInvocationParameters
structure. For information about how Amazon Web
* Services Systems Manager handles these options for the supported maintenance window task types, see
* MaintenanceWindowTaskInvocationParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withLoggingInfo(LoggingInfo loggingInfo) {
setLoggingInfo(loggingInfo);
return this;
}
/**
*
* The updated task name.
*
*
* @param name
* The updated task name.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The updated task name.
*
*
* @return The updated task name.
*/
public String getName() {
return this.name;
}
/**
*
* The updated task name.
*
*
* @param name
* The updated task name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withName(String name) {
setName(name);
return this;
}
/**
*
* The updated task description.
*
*
* @param description
* The updated task description.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The updated task description.
*
*
* @return The updated task description.
*/
public String getDescription() {
return this.description;
}
/**
*
* The updated task description.
*
*
* @param description
* The updated task description.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public void setCutoffBehavior(String cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @return The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public String getCutoffBehavior() {
return this.cutoffBehavior;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public UpdateMaintenanceWindowTaskResult withCutoffBehavior(String cutoffBehavior) {
setCutoffBehavior(cutoffBehavior);
return this;
}
/**
*
* The specification for whether tasks should continue to run after the cutoff time specified in the maintenance
* windows is reached.
*
*
* @param cutoffBehavior
* The specification for whether tasks should continue to run after the cutoff time specified in the
* maintenance windows is reached.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MaintenanceWindowTaskCutoffBehavior
*/
public UpdateMaintenanceWindowTaskResult withCutoffBehavior(MaintenanceWindowTaskCutoffBehavior cutoffBehavior) {
this.cutoffBehavior = cutoffBehavior.toString();
return this;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public void setAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
this.alarmConfiguration = alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @return The details for the CloudWatch alarm you applied to your maintenance window task.
*/
public AlarmConfiguration getAlarmConfiguration() {
return this.alarmConfiguration;
}
/**
*
* The details for the CloudWatch alarm you applied to your maintenance window task.
*
*
* @param alarmConfiguration
* The details for the CloudWatch alarm you applied to your maintenance window task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateMaintenanceWindowTaskResult withAlarmConfiguration(AlarmConfiguration alarmConfiguration) {
setAlarmConfiguration(alarmConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWindowId() != null)
sb.append("WindowId: ").append(getWindowId()).append(",");
if (getWindowTaskId() != null)
sb.append("WindowTaskId: ").append(getWindowTaskId()).append(",");
if (getTargets() != null)
sb.append("Targets: ").append(getTargets()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getServiceRoleArn() != null)
sb.append("ServiceRoleArn: ").append(getServiceRoleArn()).append(",");
if (getTaskParameters() != null)
sb.append("TaskParameters: ").append("***Sensitive Data Redacted***").append(",");
if (getTaskInvocationParameters() != null)
sb.append("TaskInvocationParameters: ").append(getTaskInvocationParameters()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getMaxConcurrency() != null)
sb.append("MaxConcurrency: ").append(getMaxConcurrency()).append(",");
if (getMaxErrors() != null)
sb.append("MaxErrors: ").append(getMaxErrors()).append(",");
if (getLoggingInfo() != null)
sb.append("LoggingInfo: ").append(getLoggingInfo()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append("***Sensitive Data Redacted***").append(",");
if (getCutoffBehavior() != null)
sb.append("CutoffBehavior: ").append(getCutoffBehavior()).append(",");
if (getAlarmConfiguration() != null)
sb.append("AlarmConfiguration: ").append(getAlarmConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateMaintenanceWindowTaskResult == false)
return false;
UpdateMaintenanceWindowTaskResult other = (UpdateMaintenanceWindowTaskResult) obj;
if (other.getWindowId() == null ^ this.getWindowId() == null)
return false;
if (other.getWindowId() != null && other.getWindowId().equals(this.getWindowId()) == false)
return false;
if (other.getWindowTaskId() == null ^ this.getWindowTaskId() == null)
return false;
if (other.getWindowTaskId() != null && other.getWindowTaskId().equals(this.getWindowTaskId()) == false)
return false;
if (other.getTargets() == null ^ this.getTargets() == null)
return false;
if (other.getTargets() != null && other.getTargets().equals(this.getTargets()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getServiceRoleArn() == null ^ this.getServiceRoleArn() == null)
return false;
if (other.getServiceRoleArn() != null && other.getServiceRoleArn().equals(this.getServiceRoleArn()) == false)
return false;
if (other.getTaskParameters() == null ^ this.getTaskParameters() == null)
return false;
if (other.getTaskParameters() != null && other.getTaskParameters().equals(this.getTaskParameters()) == false)
return false;
if (other.getTaskInvocationParameters() == null ^ this.getTaskInvocationParameters() == null)
return false;
if (other.getTaskInvocationParameters() != null && other.getTaskInvocationParameters().equals(this.getTaskInvocationParameters()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getMaxConcurrency() == null ^ this.getMaxConcurrency() == null)
return false;
if (other.getMaxConcurrency() != null && other.getMaxConcurrency().equals(this.getMaxConcurrency()) == false)
return false;
if (other.getMaxErrors() == null ^ this.getMaxErrors() == null)
return false;
if (other.getMaxErrors() != null && other.getMaxErrors().equals(this.getMaxErrors()) == false)
return false;
if (other.getLoggingInfo() == null ^ this.getLoggingInfo() == null)
return false;
if (other.getLoggingInfo() != null && other.getLoggingInfo().equals(this.getLoggingInfo()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCutoffBehavior() == null ^ this.getCutoffBehavior() == null)
return false;
if (other.getCutoffBehavior() != null && other.getCutoffBehavior().equals(this.getCutoffBehavior()) == false)
return false;
if (other.getAlarmConfiguration() == null ^ this.getAlarmConfiguration() == null)
return false;
if (other.getAlarmConfiguration() != null && other.getAlarmConfiguration().equals(this.getAlarmConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWindowId() == null) ? 0 : getWindowId().hashCode());
hashCode = prime * hashCode + ((getWindowTaskId() == null) ? 0 : getWindowTaskId().hashCode());
hashCode = prime * hashCode + ((getTargets() == null) ? 0 : getTargets().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getServiceRoleArn() == null) ? 0 : getServiceRoleArn().hashCode());
hashCode = prime * hashCode + ((getTaskParameters() == null) ? 0 : getTaskParameters().hashCode());
hashCode = prime * hashCode + ((getTaskInvocationParameters() == null) ? 0 : getTaskInvocationParameters().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrency() == null) ? 0 : getMaxConcurrency().hashCode());
hashCode = prime * hashCode + ((getMaxErrors() == null) ? 0 : getMaxErrors().hashCode());
hashCode = prime * hashCode + ((getLoggingInfo() == null) ? 0 : getLoggingInfo().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getCutoffBehavior() == null) ? 0 : getCutoffBehavior().hashCode());
hashCode = prime * hashCode + ((getAlarmConfiguration() == null) ? 0 : getAlarmConfiguration().hashCode());
return hashCode;
}
@Override
public UpdateMaintenanceWindowTaskResult clone() {
try {
return (UpdateMaintenanceWindowTaskResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}