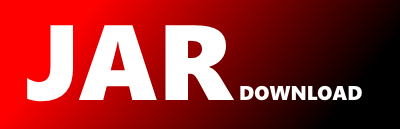
com.amazonaws.services.simplesystemsmanagement.model.UpdatePatchBaselineRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdatePatchBaselineRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ID of the patch baseline to update.
*
*/
private String baselineId;
/**
*
* The name of the patch baseline.
*
*/
private String name;
/**
*
* A set of global filters used to include patches in the baseline.
*
*/
private PatchFilterGroup globalFilters;
/**
*
* A set of rules used to include patches in the baseline.
*
*/
private PatchRuleGroup approvalRules;
/**
*
* A list of explicitly approved patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList approvedPatches;
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*/
private String approvedPatchesComplianceLevel;
/**
*
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*
*/
private Boolean approvedPatchesEnableNonSecurity;
/**
*
* A list of explicitly rejected patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList rejectedPatches;
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*/
private String rejectedPatchesAction;
/**
*
* A description of the patch baseline.
*
*/
private String description;
/**
*
* Information about the patches to use to update the managed nodes, including target operating systems and source
* repositories. Applies to Linux managed nodes only.
*
*/
private com.amazonaws.internal.SdkInternalList sources;
/**
*
* If True, then all fields that are required by the CreatePatchBaseline operation are also required for this
* API request. Optional fields that aren't specified are set to null.
*
*/
private Boolean replace;
/**
*
* The ID of the patch baseline to update.
*
*
* @param baselineId
* The ID of the patch baseline to update.
*/
public void setBaselineId(String baselineId) {
this.baselineId = baselineId;
}
/**
*
* The ID of the patch baseline to update.
*
*
* @return The ID of the patch baseline to update.
*/
public String getBaselineId() {
return this.baselineId;
}
/**
*
* The ID of the patch baseline to update.
*
*
* @param baselineId
* The ID of the patch baseline to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withBaselineId(String baselineId) {
setBaselineId(baselineId);
return this;
}
/**
*
* The name of the patch baseline.
*
*
* @param name
* The name of the patch baseline.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the patch baseline.
*
*
* @return The name of the patch baseline.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the patch baseline.
*
*
* @param name
* The name of the patch baseline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A set of global filters used to include patches in the baseline.
*
*
* @param globalFilters
* A set of global filters used to include patches in the baseline.
*/
public void setGlobalFilters(PatchFilterGroup globalFilters) {
this.globalFilters = globalFilters;
}
/**
*
* A set of global filters used to include patches in the baseline.
*
*
* @return A set of global filters used to include patches in the baseline.
*/
public PatchFilterGroup getGlobalFilters() {
return this.globalFilters;
}
/**
*
* A set of global filters used to include patches in the baseline.
*
*
* @param globalFilters
* A set of global filters used to include patches in the baseline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withGlobalFilters(PatchFilterGroup globalFilters) {
setGlobalFilters(globalFilters);
return this;
}
/**
*
* A set of rules used to include patches in the baseline.
*
*
* @param approvalRules
* A set of rules used to include patches in the baseline.
*/
public void setApprovalRules(PatchRuleGroup approvalRules) {
this.approvalRules = approvalRules;
}
/**
*
* A set of rules used to include patches in the baseline.
*
*
* @return A set of rules used to include patches in the baseline.
*/
public PatchRuleGroup getApprovalRules() {
return this.approvalRules;
}
/**
*
* A set of rules used to include patches in the baseline.
*
*
* @param approvalRules
* A set of rules used to include patches in the baseline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withApprovalRules(PatchRuleGroup approvalRules) {
setApprovalRules(approvalRules);
return this;
}
/**
*
* A list of explicitly approved patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @return A list of explicitly approved patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
*/
public java.util.List getApprovedPatches() {
if (approvedPatches == null) {
approvedPatches = new com.amazonaws.internal.SdkInternalList();
}
return approvedPatches;
}
/**
*
* A list of explicitly approved patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @param approvedPatches
* A list of explicitly approved patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
*/
public void setApprovedPatches(java.util.Collection approvedPatches) {
if (approvedPatches == null) {
this.approvedPatches = null;
return;
}
this.approvedPatches = new com.amazonaws.internal.SdkInternalList(approvedPatches);
}
/**
*
* A list of explicitly approved patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setApprovedPatches(java.util.Collection)} or {@link #withApprovedPatches(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param approvedPatches
* A list of explicitly approved patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withApprovedPatches(String... approvedPatches) {
if (this.approvedPatches == null) {
setApprovedPatches(new com.amazonaws.internal.SdkInternalList(approvedPatches.length));
}
for (String ele : approvedPatches) {
this.approvedPatches.add(ele);
}
return this;
}
/**
*
* A list of explicitly approved patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @param approvedPatches
* A list of explicitly approved patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withApprovedPatches(java.util.Collection approvedPatches) {
setApprovedPatches(approvedPatches);
return this;
}
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*
* @param approvedPatchesComplianceLevel
* Assigns a new compliance severity level to an existing patch baseline.
* @see PatchComplianceLevel
*/
public void setApprovedPatchesComplianceLevel(String approvedPatchesComplianceLevel) {
this.approvedPatchesComplianceLevel = approvedPatchesComplianceLevel;
}
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*
* @return Assigns a new compliance severity level to an existing patch baseline.
* @see PatchComplianceLevel
*/
public String getApprovedPatchesComplianceLevel() {
return this.approvedPatchesComplianceLevel;
}
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*
* @param approvedPatchesComplianceLevel
* Assigns a new compliance severity level to an existing patch baseline.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchComplianceLevel
*/
public UpdatePatchBaselineRequest withApprovedPatchesComplianceLevel(String approvedPatchesComplianceLevel) {
setApprovedPatchesComplianceLevel(approvedPatchesComplianceLevel);
return this;
}
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*
* @param approvedPatchesComplianceLevel
* Assigns a new compliance severity level to an existing patch baseline.
* @see PatchComplianceLevel
*/
public void setApprovedPatchesComplianceLevel(PatchComplianceLevel approvedPatchesComplianceLevel) {
withApprovedPatchesComplianceLevel(approvedPatchesComplianceLevel);
}
/**
*
* Assigns a new compliance severity level to an existing patch baseline.
*
*
* @param approvedPatchesComplianceLevel
* Assigns a new compliance severity level to an existing patch baseline.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchComplianceLevel
*/
public UpdatePatchBaselineRequest withApprovedPatchesComplianceLevel(PatchComplianceLevel approvedPatchesComplianceLevel) {
this.approvedPatchesComplianceLevel = approvedPatchesComplianceLevel.toString();
return this;
}
/**
*
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*
*
* @param approvedPatchesEnableNonSecurity
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*/
public void setApprovedPatchesEnableNonSecurity(Boolean approvedPatchesEnableNonSecurity) {
this.approvedPatchesEnableNonSecurity = approvedPatchesEnableNonSecurity;
}
/**
*
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*
*
* @return Indicates whether the list of approved patches includes non-security updates that should be applied to
* the managed nodes. The default value is false
. Applies to Linux managed nodes only.
*/
public Boolean getApprovedPatchesEnableNonSecurity() {
return this.approvedPatchesEnableNonSecurity;
}
/**
*
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*
*
* @param approvedPatchesEnableNonSecurity
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withApprovedPatchesEnableNonSecurity(Boolean approvedPatchesEnableNonSecurity) {
setApprovedPatchesEnableNonSecurity(approvedPatchesEnableNonSecurity);
return this;
}
/**
*
* Indicates whether the list of approved patches includes non-security updates that should be applied to the
* managed nodes. The default value is false
. Applies to Linux managed nodes only.
*
*
* @return Indicates whether the list of approved patches includes non-security updates that should be applied to
* the managed nodes. The default value is false
. Applies to Linux managed nodes only.
*/
public Boolean isApprovedPatchesEnableNonSecurity() {
return this.approvedPatchesEnableNonSecurity;
}
/**
*
* A list of explicitly rejected patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @return A list of explicitly rejected patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
*/
public java.util.List getRejectedPatches() {
if (rejectedPatches == null) {
rejectedPatches = new com.amazonaws.internal.SdkInternalList();
}
return rejectedPatches;
}
/**
*
* A list of explicitly rejected patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @param rejectedPatches
* A list of explicitly rejected patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
*/
public void setRejectedPatches(java.util.Collection rejectedPatches) {
if (rejectedPatches == null) {
this.rejectedPatches = null;
return;
}
this.rejectedPatches = new com.amazonaws.internal.SdkInternalList(rejectedPatches);
}
/**
*
* A list of explicitly rejected patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRejectedPatches(java.util.Collection)} or {@link #withRejectedPatches(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param rejectedPatches
* A list of explicitly rejected patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withRejectedPatches(String... rejectedPatches) {
if (this.rejectedPatches == null) {
setRejectedPatches(new com.amazonaws.internal.SdkInternalList(rejectedPatches.length));
}
for (String ele : rejectedPatches) {
this.rejectedPatches.add(ele);
}
return this;
}
/**
*
* A list of explicitly rejected patches for the baseline.
*
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services Systems
* Manager User Guide.
*
*
* @param rejectedPatches
* A list of explicitly rejected patches for the baseline.
*
* For information about accepted formats for lists of approved patches and rejected patches, see About package name formats for approved and rejected patch lists in the Amazon Web Services
* Systems Manager User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withRejectedPatches(java.util.Collection rejectedPatches) {
setRejectedPatches(rejectedPatches);
return this;
}
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*
* @param rejectedPatchesAction
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is
* installed only if it is a dependency of another package. It is considered compliant with the patch
* baseline, and its status is reported as InstalledOther
. This is the default action if no
* option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as
* dependencies, aren't installed by Patch Manager under any circumstances. If a package was installed before
* it was added to the Rejected patches list, or is installed outside of Patch Manager afterward, it's
* considered noncompliant with the patch baseline and its status is reported as InstalledRejected.
*
*
* @see PatchAction
*/
public void setRejectedPatchesAction(String rejectedPatchesAction) {
this.rejectedPatchesAction = rejectedPatchesAction;
}
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*
* @return The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is
* installed only if it is a dependency of another package. It is considered compliant with the patch
* baseline, and its status is reported as InstalledOther
. This is the default action if no
* option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as
* dependencies, aren't installed by Patch Manager under any circumstances. If a package was installed
* before it was added to the Rejected patches list, or is installed outside of Patch Manager
* afterward, it's considered noncompliant with the patch baseline and its status is reported as
* InstalledRejected.
*
*
* @see PatchAction
*/
public String getRejectedPatchesAction() {
return this.rejectedPatchesAction;
}
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*
* @param rejectedPatchesAction
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is
* installed only if it is a dependency of another package. It is considered compliant with the patch
* baseline, and its status is reported as InstalledOther
. This is the default action if no
* option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as
* dependencies, aren't installed by Patch Manager under any circumstances. If a package was installed before
* it was added to the Rejected patches list, or is installed outside of Patch Manager afterward, it's
* considered noncompliant with the patch baseline and its status is reported as InstalledRejected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchAction
*/
public UpdatePatchBaselineRequest withRejectedPatchesAction(String rejectedPatchesAction) {
setRejectedPatchesAction(rejectedPatchesAction);
return this;
}
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*
* @param rejectedPatchesAction
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is
* installed only if it is a dependency of another package. It is considered compliant with the patch
* baseline, and its status is reported as InstalledOther
. This is the default action if no
* option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as
* dependencies, aren't installed by Patch Manager under any circumstances. If a package was installed before
* it was added to the Rejected patches list, or is installed outside of Patch Manager afterward, it's
* considered noncompliant with the patch baseline and its status is reported as InstalledRejected.
*
*
* @see PatchAction
*/
public void setRejectedPatchesAction(PatchAction rejectedPatchesAction) {
withRejectedPatchesAction(rejectedPatchesAction);
}
/**
*
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is installed only
* if it is a dependency of another package. It is considered compliant with the patch baseline, and its status is
* reported as InstalledOther
. This is the default action if no option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as dependencies,
* aren't installed by Patch Manager under any circumstances. If a package was installed before it was added to the
* Rejected patches list, or is installed outside of Patch Manager afterward, it's considered noncompliant
* with the patch baseline and its status is reported as InstalledRejected.
*
*
*
*
* @param rejectedPatchesAction
* The action for Patch Manager to take on patches included in the RejectedPackages
list.
*
* -
*
* ALLOW_AS_DEPENDENCY
: A package in the Rejected
patches list is
* installed only if it is a dependency of another package. It is considered compliant with the patch
* baseline, and its status is reported as InstalledOther
. This is the default action if no
* option is specified.
*
*
* -
*
* BLOCK: Packages in the Rejected patches list, and packages that include them as
* dependencies, aren't installed by Patch Manager under any circumstances. If a package was installed before
* it was added to the Rejected patches list, or is installed outside of Patch Manager afterward, it's
* considered noncompliant with the patch baseline and its status is reported as InstalledRejected.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PatchAction
*/
public UpdatePatchBaselineRequest withRejectedPatchesAction(PatchAction rejectedPatchesAction) {
this.rejectedPatchesAction = rejectedPatchesAction.toString();
return this;
}
/**
*
* A description of the patch baseline.
*
*
* @param description
* A description of the patch baseline.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the patch baseline.
*
*
* @return A description of the patch baseline.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the patch baseline.
*
*
* @param description
* A description of the patch baseline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Information about the patches to use to update the managed nodes, including target operating systems and source
* repositories. Applies to Linux managed nodes only.
*
*
* @return Information about the patches to use to update the managed nodes, including target operating systems and
* source repositories. Applies to Linux managed nodes only.
*/
public java.util.List getSources() {
if (sources == null) {
sources = new com.amazonaws.internal.SdkInternalList();
}
return sources;
}
/**
*
* Information about the patches to use to update the managed nodes, including target operating systems and source
* repositories. Applies to Linux managed nodes only.
*
*
* @param sources
* Information about the patches to use to update the managed nodes, including target operating systems and
* source repositories. Applies to Linux managed nodes only.
*/
public void setSources(java.util.Collection sources) {
if (sources == null) {
this.sources = null;
return;
}
this.sources = new com.amazonaws.internal.SdkInternalList(sources);
}
/**
*
* Information about the patches to use to update the managed nodes, including target operating systems and source
* repositories. Applies to Linux managed nodes only.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSources(java.util.Collection)} or {@link #withSources(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param sources
* Information about the patches to use to update the managed nodes, including target operating systems and
* source repositories. Applies to Linux managed nodes only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withSources(PatchSource... sources) {
if (this.sources == null) {
setSources(new com.amazonaws.internal.SdkInternalList(sources.length));
}
for (PatchSource ele : sources) {
this.sources.add(ele);
}
return this;
}
/**
*
* Information about the patches to use to update the managed nodes, including target operating systems and source
* repositories. Applies to Linux managed nodes only.
*
*
* @param sources
* Information about the patches to use to update the managed nodes, including target operating systems and
* source repositories. Applies to Linux managed nodes only.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withSources(java.util.Collection sources) {
setSources(sources);
return this;
}
/**
*
* If True, then all fields that are required by the CreatePatchBaseline operation are also required for this
* API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True, then all fields that are required by the CreatePatchBaseline operation are also required
* for this API request. Optional fields that aren't specified are set to null.
*/
public void setReplace(Boolean replace) {
this.replace = replace;
}
/**
*
* If True, then all fields that are required by the CreatePatchBaseline operation are also required for this
* API request. Optional fields that aren't specified are set to null.
*
*
* @return If True, then all fields that are required by the CreatePatchBaseline operation are also required
* for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean getReplace() {
return this.replace;
}
/**
*
* If True, then all fields that are required by the CreatePatchBaseline operation are also required for this
* API request. Optional fields that aren't specified are set to null.
*
*
* @param replace
* If True, then all fields that are required by the CreatePatchBaseline operation are also required
* for this API request. Optional fields that aren't specified are set to null.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdatePatchBaselineRequest withReplace(Boolean replace) {
setReplace(replace);
return this;
}
/**
*
* If True, then all fields that are required by the CreatePatchBaseline operation are also required for this
* API request. Optional fields that aren't specified are set to null.
*
*
* @return If True, then all fields that are required by the CreatePatchBaseline operation are also required
* for this API request. Optional fields that aren't specified are set to null.
*/
public Boolean isReplace() {
return this.replace;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBaselineId() != null)
sb.append("BaselineId: ").append(getBaselineId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getGlobalFilters() != null)
sb.append("GlobalFilters: ").append(getGlobalFilters()).append(",");
if (getApprovalRules() != null)
sb.append("ApprovalRules: ").append(getApprovalRules()).append(",");
if (getApprovedPatches() != null)
sb.append("ApprovedPatches: ").append(getApprovedPatches()).append(",");
if (getApprovedPatchesComplianceLevel() != null)
sb.append("ApprovedPatchesComplianceLevel: ").append(getApprovedPatchesComplianceLevel()).append(",");
if (getApprovedPatchesEnableNonSecurity() != null)
sb.append("ApprovedPatchesEnableNonSecurity: ").append(getApprovedPatchesEnableNonSecurity()).append(",");
if (getRejectedPatches() != null)
sb.append("RejectedPatches: ").append(getRejectedPatches()).append(",");
if (getRejectedPatchesAction() != null)
sb.append("RejectedPatchesAction: ").append(getRejectedPatchesAction()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getSources() != null)
sb.append("Sources: ").append(getSources()).append(",");
if (getReplace() != null)
sb.append("Replace: ").append(getReplace());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdatePatchBaselineRequest == false)
return false;
UpdatePatchBaselineRequest other = (UpdatePatchBaselineRequest) obj;
if (other.getBaselineId() == null ^ this.getBaselineId() == null)
return false;
if (other.getBaselineId() != null && other.getBaselineId().equals(this.getBaselineId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getGlobalFilters() == null ^ this.getGlobalFilters() == null)
return false;
if (other.getGlobalFilters() != null && other.getGlobalFilters().equals(this.getGlobalFilters()) == false)
return false;
if (other.getApprovalRules() == null ^ this.getApprovalRules() == null)
return false;
if (other.getApprovalRules() != null && other.getApprovalRules().equals(this.getApprovalRules()) == false)
return false;
if (other.getApprovedPatches() == null ^ this.getApprovedPatches() == null)
return false;
if (other.getApprovedPatches() != null && other.getApprovedPatches().equals(this.getApprovedPatches()) == false)
return false;
if (other.getApprovedPatchesComplianceLevel() == null ^ this.getApprovedPatchesComplianceLevel() == null)
return false;
if (other.getApprovedPatchesComplianceLevel() != null
&& other.getApprovedPatchesComplianceLevel().equals(this.getApprovedPatchesComplianceLevel()) == false)
return false;
if (other.getApprovedPatchesEnableNonSecurity() == null ^ this.getApprovedPatchesEnableNonSecurity() == null)
return false;
if (other.getApprovedPatchesEnableNonSecurity() != null
&& other.getApprovedPatchesEnableNonSecurity().equals(this.getApprovedPatchesEnableNonSecurity()) == false)
return false;
if (other.getRejectedPatches() == null ^ this.getRejectedPatches() == null)
return false;
if (other.getRejectedPatches() != null && other.getRejectedPatches().equals(this.getRejectedPatches()) == false)
return false;
if (other.getRejectedPatchesAction() == null ^ this.getRejectedPatchesAction() == null)
return false;
if (other.getRejectedPatchesAction() != null && other.getRejectedPatchesAction().equals(this.getRejectedPatchesAction()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getSources() == null ^ this.getSources() == null)
return false;
if (other.getSources() != null && other.getSources().equals(this.getSources()) == false)
return false;
if (other.getReplace() == null ^ this.getReplace() == null)
return false;
if (other.getReplace() != null && other.getReplace().equals(this.getReplace()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBaselineId() == null) ? 0 : getBaselineId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getGlobalFilters() == null) ? 0 : getGlobalFilters().hashCode());
hashCode = prime * hashCode + ((getApprovalRules() == null) ? 0 : getApprovalRules().hashCode());
hashCode = prime * hashCode + ((getApprovedPatches() == null) ? 0 : getApprovedPatches().hashCode());
hashCode = prime * hashCode + ((getApprovedPatchesComplianceLevel() == null) ? 0 : getApprovedPatchesComplianceLevel().hashCode());
hashCode = prime * hashCode + ((getApprovedPatchesEnableNonSecurity() == null) ? 0 : getApprovedPatchesEnableNonSecurity().hashCode());
hashCode = prime * hashCode + ((getRejectedPatches() == null) ? 0 : getRejectedPatches().hashCode());
hashCode = prime * hashCode + ((getRejectedPatchesAction() == null) ? 0 : getRejectedPatchesAction().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getSources() == null) ? 0 : getSources().hashCode());
hashCode = prime * hashCode + ((getReplace() == null) ? 0 : getReplace().hashCode());
return hashCode;
}
@Override
public UpdatePatchBaselineRequest clone() {
return (UpdatePatchBaselineRequest) super.clone();
}
}