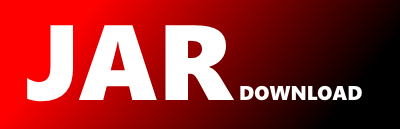
com.amazonaws.services.simplesystemsmanagement.model.transform.StepExecutionMarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-ssm Show documentation
Show all versions of aws-java-sdk-ssm Show documentation
The AWS Java SDK for AWS Simple Systems Management Service holds the client classes that are used for communicating with the AWS Simple Systems Management Service
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.simplesystemsmanagement.model.transform;
import java.util.Map;
import java.util.List;
import javax.annotation.Generated;
import com.amazonaws.SdkClientException;
import com.amazonaws.services.simplesystemsmanagement.model.*;
import com.amazonaws.protocol.*;
import com.amazonaws.annotation.SdkInternalApi;
/**
* StepExecutionMarshaller
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
@SdkInternalApi
public class StepExecutionMarshaller {
private static final MarshallingInfo STEPNAME_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("StepName").build();
private static final MarshallingInfo ACTION_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("Action").build();
private static final MarshallingInfo TIMEOUTSECONDS_BINDING = MarshallingInfo.builder(MarshallingType.LONG)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("TimeoutSeconds").build();
private static final MarshallingInfo ONFAILURE_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("OnFailure").build();
private static final MarshallingInfo MAXATTEMPTS_BINDING = MarshallingInfo.builder(MarshallingType.INTEGER)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("MaxAttempts").build();
private static final MarshallingInfo EXECUTIONSTARTTIME_BINDING = MarshallingInfo.builder(MarshallingType.DATE)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ExecutionStartTime").timestampFormat("unixTimestamp").build();
private static final MarshallingInfo EXECUTIONENDTIME_BINDING = MarshallingInfo.builder(MarshallingType.DATE)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ExecutionEndTime").timestampFormat("unixTimestamp").build();
private static final MarshallingInfo STEPSTATUS_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("StepStatus").build();
private static final MarshallingInfo RESPONSECODE_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ResponseCode").build();
private static final MarshallingInfo
© 2015 - 2025 Weber Informatics LLC | Privacy Policy