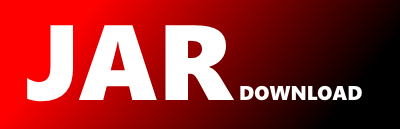
com.amazonaws.services.ssmsap.model.Component Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ssmsap.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The SAP component of your application.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Component implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the component.
*
*/
private String componentId;
/**
*
* The SAP System Identifier of the application component.
*
*/
private String sid;
/**
*
* The SAP system number of the application component.
*
*/
private String systemNumber;
/**
*
* The parent component of a highly available environment. For example, in a highly available SAP on AWS workload,
* the parent component consists of the entire setup, including the child components.
*
*/
private String parentComponent;
/**
*
* The child components of a highly available environment. For example, in a highly available SAP on AWS workload,
* the child component consists of the primary and secondar instances.
*
*/
private java.util.List childComponents;
/**
*
* The ID of the application.
*
*/
private String applicationId;
/**
*
* The type of the component.
*
*/
private String componentType;
/**
*
* The status of the component.
*
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call GetComponent
* to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered information.
* Verify your SAP application.
*
*
*
*/
private String status;
/**
*
* The hostname of the component.
*
*/
private String sapHostname;
/**
*
* The SAP feature of the component.
*
*/
private String sapFeature;
/**
*
* The kernel version of the component.
*
*/
private String sapKernelVersion;
/**
*
* The SAP HANA version of the component.
*
*/
private String hdbVersion;
/**
*
* Details of the SAP HANA system replication for the component.
*
*/
private Resilience resilience;
/**
*
* The associated host of the component.
*
*/
private AssociatedHost associatedHost;
/**
*
* The SAP HANA databases of the component.
*
*/
private java.util.List databases;
/**
*
* The hosts of the component.
*
*/
@Deprecated
private java.util.List hosts;
/**
*
* The primary host of the component.
*
*/
@Deprecated
private String primaryHost;
/**
*
* The connection specifications for the database of the component.
*
*/
private DatabaseConnection databaseConnection;
/**
*
* The time at which the component was last updated.
*
*/
private java.util.Date lastUpdated;
/**
*
* The Amazon Resource Name (ARN) of the component.
*
*/
private String arn;
/**
*
* The ID of the component.
*
*
* @param componentId
* The ID of the component.
*/
public void setComponentId(String componentId) {
this.componentId = componentId;
}
/**
*
* The ID of the component.
*
*
* @return The ID of the component.
*/
public String getComponentId() {
return this.componentId;
}
/**
*
* The ID of the component.
*
*
* @param componentId
* The ID of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withComponentId(String componentId) {
setComponentId(componentId);
return this;
}
/**
*
* The SAP System Identifier of the application component.
*
*
* @param sid
* The SAP System Identifier of the application component.
*/
public void setSid(String sid) {
this.sid = sid;
}
/**
*
* The SAP System Identifier of the application component.
*
*
* @return The SAP System Identifier of the application component.
*/
public String getSid() {
return this.sid;
}
/**
*
* The SAP System Identifier of the application component.
*
*
* @param sid
* The SAP System Identifier of the application component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withSid(String sid) {
setSid(sid);
return this;
}
/**
*
* The SAP system number of the application component.
*
*
* @param systemNumber
* The SAP system number of the application component.
*/
public void setSystemNumber(String systemNumber) {
this.systemNumber = systemNumber;
}
/**
*
* The SAP system number of the application component.
*
*
* @return The SAP system number of the application component.
*/
public String getSystemNumber() {
return this.systemNumber;
}
/**
*
* The SAP system number of the application component.
*
*
* @param systemNumber
* The SAP system number of the application component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withSystemNumber(String systemNumber) {
setSystemNumber(systemNumber);
return this;
}
/**
*
* The parent component of a highly available environment. For example, in a highly available SAP on AWS workload,
* the parent component consists of the entire setup, including the child components.
*
*
* @param parentComponent
* The parent component of a highly available environment. For example, in a highly available SAP on AWS
* workload, the parent component consists of the entire setup, including the child components.
*/
public void setParentComponent(String parentComponent) {
this.parentComponent = parentComponent;
}
/**
*
* The parent component of a highly available environment. For example, in a highly available SAP on AWS workload,
* the parent component consists of the entire setup, including the child components.
*
*
* @return The parent component of a highly available environment. For example, in a highly available SAP on AWS
* workload, the parent component consists of the entire setup, including the child components.
*/
public String getParentComponent() {
return this.parentComponent;
}
/**
*
* The parent component of a highly available environment. For example, in a highly available SAP on AWS workload,
* the parent component consists of the entire setup, including the child components.
*
*
* @param parentComponent
* The parent component of a highly available environment. For example, in a highly available SAP on AWS
* workload, the parent component consists of the entire setup, including the child components.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withParentComponent(String parentComponent) {
setParentComponent(parentComponent);
return this;
}
/**
*
* The child components of a highly available environment. For example, in a highly available SAP on AWS workload,
* the child component consists of the primary and secondar instances.
*
*
* @return The child components of a highly available environment. For example, in a highly available SAP on AWS
* workload, the child component consists of the primary and secondar instances.
*/
public java.util.List getChildComponents() {
return childComponents;
}
/**
*
* The child components of a highly available environment. For example, in a highly available SAP on AWS workload,
* the child component consists of the primary and secondar instances.
*
*
* @param childComponents
* The child components of a highly available environment. For example, in a highly available SAP on AWS
* workload, the child component consists of the primary and secondar instances.
*/
public void setChildComponents(java.util.Collection childComponents) {
if (childComponents == null) {
this.childComponents = null;
return;
}
this.childComponents = new java.util.ArrayList(childComponents);
}
/**
*
* The child components of a highly available environment. For example, in a highly available SAP on AWS workload,
* the child component consists of the primary and secondar instances.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChildComponents(java.util.Collection)} or {@link #withChildComponents(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param childComponents
* The child components of a highly available environment. For example, in a highly available SAP on AWS
* workload, the child component consists of the primary and secondar instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withChildComponents(String... childComponents) {
if (this.childComponents == null) {
setChildComponents(new java.util.ArrayList(childComponents.length));
}
for (String ele : childComponents) {
this.childComponents.add(ele);
}
return this;
}
/**
*
* The child components of a highly available environment. For example, in a highly available SAP on AWS workload,
* the child component consists of the primary and secondar instances.
*
*
* @param childComponents
* The child components of a highly available environment. For example, in a highly available SAP on AWS
* workload, the child component consists of the primary and secondar instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withChildComponents(java.util.Collection childComponents) {
setChildComponents(childComponents);
return this;
}
/**
*
* The ID of the application.
*
*
* @param applicationId
* The ID of the application.
*/
public void setApplicationId(String applicationId) {
this.applicationId = applicationId;
}
/**
*
* The ID of the application.
*
*
* @return The ID of the application.
*/
public String getApplicationId() {
return this.applicationId;
}
/**
*
* The ID of the application.
*
*
* @param applicationId
* The ID of the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withApplicationId(String applicationId) {
setApplicationId(applicationId);
return this;
}
/**
*
* The type of the component.
*
*
* @param componentType
* The type of the component.
* @see ComponentType
*/
public void setComponentType(String componentType) {
this.componentType = componentType;
}
/**
*
* The type of the component.
*
*
* @return The type of the component.
* @see ComponentType
*/
public String getComponentType() {
return this.componentType;
}
/**
*
* The type of the component.
*
*
* @param componentType
* The type of the component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComponentType
*/
public Component withComponentType(String componentType) {
setComponentType(componentType);
return this;
}
/**
*
* The type of the component.
*
*
* @param componentType
* The type of the component.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComponentType
*/
public Component withComponentType(ComponentType componentType) {
this.componentType = componentType.toString();
return this;
}
/**
*
* The status of the component.
*
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call GetComponent
* to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered information.
* Verify your SAP application.
*
*
*
*
* @param status
* The status of the component.
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call
* GetComponent
to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered
* information. Verify your SAP application.
*
*
* @see ComponentStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the component.
*
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call GetComponent
* to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered information.
* Verify your SAP application.
*
*
*
*
* @return The status of the component.
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call
* GetComponent
to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered
* information. Verify your SAP application.
*
*
* @see ComponentStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the component.
*
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call GetComponent
* to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered information.
* Verify your SAP application.
*
*
*
*
* @param status
* The status of the component.
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call
* GetComponent
to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered
* information. Verify your SAP application.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComponentStatus
*/
public Component withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the component.
*
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call GetComponent
* to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered information.
* Verify your SAP application.
*
*
*
*
* @param status
* The status of the component.
*
* -
*
* ACTIVATED - this status has been deprecated.
*
*
* -
*
* STARTING - the component is in the process of being started.
*
*
* -
*
* STOPPED - the component is not running.
*
*
* -
*
* STOPPING - the component is in the process of being stopped.
*
*
* -
*
* RUNNING - the component is running.
*
*
* -
*
* RUNNING_WITH_ERROR - one or more child component(s) of the parent component is not running. Call
* GetComponent
to review the status of each child component.
*
*
* -
*
* UNDEFINED - AWS Systems Manager for SAP cannot provide the component status based on the discovered
* information. Verify your SAP application.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ComponentStatus
*/
public Component withStatus(ComponentStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The hostname of the component.
*
*
* @param sapHostname
* The hostname of the component.
*/
public void setSapHostname(String sapHostname) {
this.sapHostname = sapHostname;
}
/**
*
* The hostname of the component.
*
*
* @return The hostname of the component.
*/
public String getSapHostname() {
return this.sapHostname;
}
/**
*
* The hostname of the component.
*
*
* @param sapHostname
* The hostname of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withSapHostname(String sapHostname) {
setSapHostname(sapHostname);
return this;
}
/**
*
* The SAP feature of the component.
*
*
* @param sapFeature
* The SAP feature of the component.
*/
public void setSapFeature(String sapFeature) {
this.sapFeature = sapFeature;
}
/**
*
* The SAP feature of the component.
*
*
* @return The SAP feature of the component.
*/
public String getSapFeature() {
return this.sapFeature;
}
/**
*
* The SAP feature of the component.
*
*
* @param sapFeature
* The SAP feature of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withSapFeature(String sapFeature) {
setSapFeature(sapFeature);
return this;
}
/**
*
* The kernel version of the component.
*
*
* @param sapKernelVersion
* The kernel version of the component.
*/
public void setSapKernelVersion(String sapKernelVersion) {
this.sapKernelVersion = sapKernelVersion;
}
/**
*
* The kernel version of the component.
*
*
* @return The kernel version of the component.
*/
public String getSapKernelVersion() {
return this.sapKernelVersion;
}
/**
*
* The kernel version of the component.
*
*
* @param sapKernelVersion
* The kernel version of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withSapKernelVersion(String sapKernelVersion) {
setSapKernelVersion(sapKernelVersion);
return this;
}
/**
*
* The SAP HANA version of the component.
*
*
* @param hdbVersion
* The SAP HANA version of the component.
*/
public void setHdbVersion(String hdbVersion) {
this.hdbVersion = hdbVersion;
}
/**
*
* The SAP HANA version of the component.
*
*
* @return The SAP HANA version of the component.
*/
public String getHdbVersion() {
return this.hdbVersion;
}
/**
*
* The SAP HANA version of the component.
*
*
* @param hdbVersion
* The SAP HANA version of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withHdbVersion(String hdbVersion) {
setHdbVersion(hdbVersion);
return this;
}
/**
*
* Details of the SAP HANA system replication for the component.
*
*
* @param resilience
* Details of the SAP HANA system replication for the component.
*/
public void setResilience(Resilience resilience) {
this.resilience = resilience;
}
/**
*
* Details of the SAP HANA system replication for the component.
*
*
* @return Details of the SAP HANA system replication for the component.
*/
public Resilience getResilience() {
return this.resilience;
}
/**
*
* Details of the SAP HANA system replication for the component.
*
*
* @param resilience
* Details of the SAP HANA system replication for the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withResilience(Resilience resilience) {
setResilience(resilience);
return this;
}
/**
*
* The associated host of the component.
*
*
* @param associatedHost
* The associated host of the component.
*/
public void setAssociatedHost(AssociatedHost associatedHost) {
this.associatedHost = associatedHost;
}
/**
*
* The associated host of the component.
*
*
* @return The associated host of the component.
*/
public AssociatedHost getAssociatedHost() {
return this.associatedHost;
}
/**
*
* The associated host of the component.
*
*
* @param associatedHost
* The associated host of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withAssociatedHost(AssociatedHost associatedHost) {
setAssociatedHost(associatedHost);
return this;
}
/**
*
* The SAP HANA databases of the component.
*
*
* @return The SAP HANA databases of the component.
*/
public java.util.List getDatabases() {
return databases;
}
/**
*
* The SAP HANA databases of the component.
*
*
* @param databases
* The SAP HANA databases of the component.
*/
public void setDatabases(java.util.Collection databases) {
if (databases == null) {
this.databases = null;
return;
}
this.databases = new java.util.ArrayList(databases);
}
/**
*
* The SAP HANA databases of the component.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDatabases(java.util.Collection)} or {@link #withDatabases(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param databases
* The SAP HANA databases of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withDatabases(String... databases) {
if (this.databases == null) {
setDatabases(new java.util.ArrayList(databases.length));
}
for (String ele : databases) {
this.databases.add(ele);
}
return this;
}
/**
*
* The SAP HANA databases of the component.
*
*
* @param databases
* The SAP HANA databases of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withDatabases(java.util.Collection databases) {
setDatabases(databases);
return this;
}
/**
*
* The hosts of the component.
*
*
* @return The hosts of the component.
*/
@Deprecated
public java.util.List getHosts() {
return hosts;
}
/**
*
* The hosts of the component.
*
*
* @param hosts
* The hosts of the component.
*/
@Deprecated
public void setHosts(java.util.Collection hosts) {
if (hosts == null) {
this.hosts = null;
return;
}
this.hosts = new java.util.ArrayList(hosts);
}
/**
*
* The hosts of the component.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setHosts(java.util.Collection)} or {@link #withHosts(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param hosts
* The hosts of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public Component withHosts(Host... hosts) {
if (this.hosts == null) {
setHosts(new java.util.ArrayList(hosts.length));
}
for (Host ele : hosts) {
this.hosts.add(ele);
}
return this;
}
/**
*
* The hosts of the component.
*
*
* @param hosts
* The hosts of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public Component withHosts(java.util.Collection hosts) {
setHosts(hosts);
return this;
}
/**
*
* The primary host of the component.
*
*
* @param primaryHost
* The primary host of the component.
*/
@Deprecated
public void setPrimaryHost(String primaryHost) {
this.primaryHost = primaryHost;
}
/**
*
* The primary host of the component.
*
*
* @return The primary host of the component.
*/
@Deprecated
public String getPrimaryHost() {
return this.primaryHost;
}
/**
*
* The primary host of the component.
*
*
* @param primaryHost
* The primary host of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public Component withPrimaryHost(String primaryHost) {
setPrimaryHost(primaryHost);
return this;
}
/**
*
* The connection specifications for the database of the component.
*
*
* @param databaseConnection
* The connection specifications for the database of the component.
*/
public void setDatabaseConnection(DatabaseConnection databaseConnection) {
this.databaseConnection = databaseConnection;
}
/**
*
* The connection specifications for the database of the component.
*
*
* @return The connection specifications for the database of the component.
*/
public DatabaseConnection getDatabaseConnection() {
return this.databaseConnection;
}
/**
*
* The connection specifications for the database of the component.
*
*
* @param databaseConnection
* The connection specifications for the database of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withDatabaseConnection(DatabaseConnection databaseConnection) {
setDatabaseConnection(databaseConnection);
return this;
}
/**
*
* The time at which the component was last updated.
*
*
* @param lastUpdated
* The time at which the component was last updated.
*/
public void setLastUpdated(java.util.Date lastUpdated) {
this.lastUpdated = lastUpdated;
}
/**
*
* The time at which the component was last updated.
*
*
* @return The time at which the component was last updated.
*/
public java.util.Date getLastUpdated() {
return this.lastUpdated;
}
/**
*
* The time at which the component was last updated.
*
*
* @param lastUpdated
* The time at which the component was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withLastUpdated(java.util.Date lastUpdated) {
setLastUpdated(lastUpdated);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the component.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the component.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the component.
*
*
* @return The Amazon Resource Name (ARN) of the component.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the component.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Component withArn(String arn) {
setArn(arn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getComponentId() != null)
sb.append("ComponentId: ").append(getComponentId()).append(",");
if (getSid() != null)
sb.append("Sid: ").append(getSid()).append(",");
if (getSystemNumber() != null)
sb.append("SystemNumber: ").append(getSystemNumber()).append(",");
if (getParentComponent() != null)
sb.append("ParentComponent: ").append(getParentComponent()).append(",");
if (getChildComponents() != null)
sb.append("ChildComponents: ").append(getChildComponents()).append(",");
if (getApplicationId() != null)
sb.append("ApplicationId: ").append(getApplicationId()).append(",");
if (getComponentType() != null)
sb.append("ComponentType: ").append(getComponentType()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSapHostname() != null)
sb.append("SapHostname: ").append(getSapHostname()).append(",");
if (getSapFeature() != null)
sb.append("SapFeature: ").append(getSapFeature()).append(",");
if (getSapKernelVersion() != null)
sb.append("SapKernelVersion: ").append(getSapKernelVersion()).append(",");
if (getHdbVersion() != null)
sb.append("HdbVersion: ").append(getHdbVersion()).append(",");
if (getResilience() != null)
sb.append("Resilience: ").append(getResilience()).append(",");
if (getAssociatedHost() != null)
sb.append("AssociatedHost: ").append(getAssociatedHost()).append(",");
if (getDatabases() != null)
sb.append("Databases: ").append(getDatabases()).append(",");
if (getHosts() != null)
sb.append("Hosts: ").append(getHosts()).append(",");
if (getPrimaryHost() != null)
sb.append("PrimaryHost: ").append(getPrimaryHost()).append(",");
if (getDatabaseConnection() != null)
sb.append("DatabaseConnection: ").append(getDatabaseConnection()).append(",");
if (getLastUpdated() != null)
sb.append("LastUpdated: ").append(getLastUpdated()).append(",");
if (getArn() != null)
sb.append("Arn: ").append(getArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Component == false)
return false;
Component other = (Component) obj;
if (other.getComponentId() == null ^ this.getComponentId() == null)
return false;
if (other.getComponentId() != null && other.getComponentId().equals(this.getComponentId()) == false)
return false;
if (other.getSid() == null ^ this.getSid() == null)
return false;
if (other.getSid() != null && other.getSid().equals(this.getSid()) == false)
return false;
if (other.getSystemNumber() == null ^ this.getSystemNumber() == null)
return false;
if (other.getSystemNumber() != null && other.getSystemNumber().equals(this.getSystemNumber()) == false)
return false;
if (other.getParentComponent() == null ^ this.getParentComponent() == null)
return false;
if (other.getParentComponent() != null && other.getParentComponent().equals(this.getParentComponent()) == false)
return false;
if (other.getChildComponents() == null ^ this.getChildComponents() == null)
return false;
if (other.getChildComponents() != null && other.getChildComponents().equals(this.getChildComponents()) == false)
return false;
if (other.getApplicationId() == null ^ this.getApplicationId() == null)
return false;
if (other.getApplicationId() != null && other.getApplicationId().equals(this.getApplicationId()) == false)
return false;
if (other.getComponentType() == null ^ this.getComponentType() == null)
return false;
if (other.getComponentType() != null && other.getComponentType().equals(this.getComponentType()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSapHostname() == null ^ this.getSapHostname() == null)
return false;
if (other.getSapHostname() != null && other.getSapHostname().equals(this.getSapHostname()) == false)
return false;
if (other.getSapFeature() == null ^ this.getSapFeature() == null)
return false;
if (other.getSapFeature() != null && other.getSapFeature().equals(this.getSapFeature()) == false)
return false;
if (other.getSapKernelVersion() == null ^ this.getSapKernelVersion() == null)
return false;
if (other.getSapKernelVersion() != null && other.getSapKernelVersion().equals(this.getSapKernelVersion()) == false)
return false;
if (other.getHdbVersion() == null ^ this.getHdbVersion() == null)
return false;
if (other.getHdbVersion() != null && other.getHdbVersion().equals(this.getHdbVersion()) == false)
return false;
if (other.getResilience() == null ^ this.getResilience() == null)
return false;
if (other.getResilience() != null && other.getResilience().equals(this.getResilience()) == false)
return false;
if (other.getAssociatedHost() == null ^ this.getAssociatedHost() == null)
return false;
if (other.getAssociatedHost() != null && other.getAssociatedHost().equals(this.getAssociatedHost()) == false)
return false;
if (other.getDatabases() == null ^ this.getDatabases() == null)
return false;
if (other.getDatabases() != null && other.getDatabases().equals(this.getDatabases()) == false)
return false;
if (other.getHosts() == null ^ this.getHosts() == null)
return false;
if (other.getHosts() != null && other.getHosts().equals(this.getHosts()) == false)
return false;
if (other.getPrimaryHost() == null ^ this.getPrimaryHost() == null)
return false;
if (other.getPrimaryHost() != null && other.getPrimaryHost().equals(this.getPrimaryHost()) == false)
return false;
if (other.getDatabaseConnection() == null ^ this.getDatabaseConnection() == null)
return false;
if (other.getDatabaseConnection() != null && other.getDatabaseConnection().equals(this.getDatabaseConnection()) == false)
return false;
if (other.getLastUpdated() == null ^ this.getLastUpdated() == null)
return false;
if (other.getLastUpdated() != null && other.getLastUpdated().equals(this.getLastUpdated()) == false)
return false;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getComponentId() == null) ? 0 : getComponentId().hashCode());
hashCode = prime * hashCode + ((getSid() == null) ? 0 : getSid().hashCode());
hashCode = prime * hashCode + ((getSystemNumber() == null) ? 0 : getSystemNumber().hashCode());
hashCode = prime * hashCode + ((getParentComponent() == null) ? 0 : getParentComponent().hashCode());
hashCode = prime * hashCode + ((getChildComponents() == null) ? 0 : getChildComponents().hashCode());
hashCode = prime * hashCode + ((getApplicationId() == null) ? 0 : getApplicationId().hashCode());
hashCode = prime * hashCode + ((getComponentType() == null) ? 0 : getComponentType().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSapHostname() == null) ? 0 : getSapHostname().hashCode());
hashCode = prime * hashCode + ((getSapFeature() == null) ? 0 : getSapFeature().hashCode());
hashCode = prime * hashCode + ((getSapKernelVersion() == null) ? 0 : getSapKernelVersion().hashCode());
hashCode = prime * hashCode + ((getHdbVersion() == null) ? 0 : getHdbVersion().hashCode());
hashCode = prime * hashCode + ((getResilience() == null) ? 0 : getResilience().hashCode());
hashCode = prime * hashCode + ((getAssociatedHost() == null) ? 0 : getAssociatedHost().hashCode());
hashCode = prime * hashCode + ((getDatabases() == null) ? 0 : getDatabases().hashCode());
hashCode = prime * hashCode + ((getHosts() == null) ? 0 : getHosts().hashCode());
hashCode = prime * hashCode + ((getPrimaryHost() == null) ? 0 : getPrimaryHost().hashCode());
hashCode = prime * hashCode + ((getDatabaseConnection() == null) ? 0 : getDatabaseConnection().hashCode());
hashCode = prime * hashCode + ((getLastUpdated() == null) ? 0 : getLastUpdated().hashCode());
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
return hashCode;
}
@Override
public Component clone() {
try {
return (Component) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ssmsap.model.transform.ComponentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}