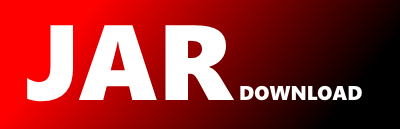
com.amazonaws.services.ssooidc.model.CreateTokenWithIAMRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ssooidc Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ssooidc.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTokenWithIAMRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique identifier string for the client or application. This value is an application ARN that has OAuth
* grants configured.
*
*/
private String clientId;
/**
*
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token Exchange.
* Specify one of the following values, depending on the grant type that you want:
*
*
* * Authorization Code - authorization_code
*
*
* * Refresh Token - refresh_token
*
*
* * JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* * Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*
*/
private String grantType;
/**
*
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to identify
* this authorization request. The code is obtained through a redirect from IAM Identity Center to a redirect URI
* persisted in the Authorization Code GrantOptions for the application.
*
*/
private String code;
/**
*
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
*
* For more information about the features and limitations of the current IAM Identity Center OIDC implementation,
* see Considerations for Using this Guide in the IAM Identity Center OIDC API
* Reference.
*
*/
private String refreshToken;
/**
*
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token (JWT)
* issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer GrantOptions for
* the application.
*
*/
private String assertion;
/**
*
* The list of scopes for which authorization is requested. The access token that is issued is limited to the scopes
* that are granted. If the value is not specified, IAM Identity Center authorizes all scopes configured for the
* application, including the following default scopes: openid
, aws
,
* sts:identity_context
.
*
*/
private java.util.List scope;
/**
*
* Used only when calling this API for the Authorization Code grant type. This value specifies the location of the
* client or application that has registered to receive the authorization code.
*
*/
private String redirectUri;
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a different
* client or application. The access token must have authorized scopes that indicate the requested application as a
* target audience.
*
*/
private String subjectToken;
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that is
* passed as the subject of the exchange. The following value is supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*/
private String subjectTokenType;
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that
* the requester can receive. The following values are supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* * Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*
*/
private String requestedTokenType;
/**
*
* Used only when calling this API for the Authorization Code grant type. This value is generated by the client and
* presented to validate the original code challenge value the client passed at authorization time.
*
*/
private String codeVerifier;
/**
*
* The unique identifier string for the client or application. This value is an application ARN that has OAuth
* grants configured.
*
*
* @param clientId
* The unique identifier string for the client or application. This value is an application ARN that has
* OAuth grants configured.
*/
public void setClientId(String clientId) {
this.clientId = clientId;
}
/**
*
* The unique identifier string for the client or application. This value is an application ARN that has OAuth
* grants configured.
*
*
* @return The unique identifier string for the client or application. This value is an application ARN that has
* OAuth grants configured.
*/
public String getClientId() {
return this.clientId;
}
/**
*
* The unique identifier string for the client or application. This value is an application ARN that has OAuth
* grants configured.
*
*
* @param clientId
* The unique identifier string for the client or application. This value is an application ARN that has
* OAuth grants configured.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withClientId(String clientId) {
setClientId(clientId);
return this;
}
/**
*
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token Exchange.
* Specify one of the following values, depending on the grant type that you want:
*
*
* * Authorization Code - authorization_code
*
*
* * Refresh Token - refresh_token
*
*
* * JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* * Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*
*
* @param grantType
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token
* Exchange. Specify one of the following values, depending on the grant type that you want:
*
* Authorization Code - authorization_code
*
*
* Refresh Token - refresh_token
*
*
* JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*/
public void setGrantType(String grantType) {
this.grantType = grantType;
}
/**
*
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token Exchange.
* Specify one of the following values, depending on the grant type that you want:
*
*
* * Authorization Code - authorization_code
*
*
* * Refresh Token - refresh_token
*
*
* * JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* * Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*
*
* @return Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token
* Exchange. Specify one of the following values, depending on the grant type that you want:
*
* Authorization Code - authorization_code
*
*
* Refresh Token - refresh_token
*
*
* JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*/
public String getGrantType() {
return this.grantType;
}
/**
*
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token Exchange.
* Specify one of the following values, depending on the grant type that you want:
*
*
* * Authorization Code - authorization_code
*
*
* * Refresh Token - refresh_token
*
*
* * JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* * Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
*
*
* @param grantType
* Supports the following OAuth grant types: Authorization Code, Refresh Token, JWT Bearer, and Token
* Exchange. Specify one of the following values, depending on the grant type that you want:
*
* Authorization Code - authorization_code
*
*
* Refresh Token - refresh_token
*
*
* JWT Bearer - urn:ietf:params:oauth:grant-type:jwt-bearer
*
*
* Token Exchange - urn:ietf:params:oauth:grant-type:token-exchange
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withGrantType(String grantType) {
setGrantType(grantType);
return this;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to identify
* this authorization request. The code is obtained through a redirect from IAM Identity Center to a redirect URI
* persisted in the Authorization Code GrantOptions for the application.
*
*
* @param code
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to
* identify this authorization request. The code is obtained through a redirect from IAM Identity Center to a
* redirect URI persisted in the Authorization Code GrantOptions for the application.
*/
public void setCode(String code) {
this.code = code;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to identify
* this authorization request. The code is obtained through a redirect from IAM Identity Center to a redirect URI
* persisted in the Authorization Code GrantOptions for the application.
*
*
* @return Used only when calling this API for the Authorization Code grant type. This short-term code is used to
* identify this authorization request. The code is obtained through a redirect from IAM Identity Center to
* a redirect URI persisted in the Authorization Code GrantOptions for the application.
*/
public String getCode() {
return this.code;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to identify
* this authorization request. The code is obtained through a redirect from IAM Identity Center to a redirect URI
* persisted in the Authorization Code GrantOptions for the application.
*
*
* @param code
* Used only when calling this API for the Authorization Code grant type. This short-term code is used to
* identify this authorization request. The code is obtained through a redirect from IAM Identity Center to a
* redirect URI persisted in the Authorization Code GrantOptions for the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withCode(String code) {
setCode(code);
return this;
}
/**
*
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
*
* For more information about the features and limitations of the current IAM Identity Center OIDC implementation,
* see Considerations for Using this Guide in the IAM Identity Center OIDC API
* Reference.
*
*
* @param refreshToken
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
* For more information about the features and limitations of the current IAM Identity Center OIDC
* implementation, see Considerations for Using this Guide in the IAM Identity Center
* OIDC API Reference.
*/
public void setRefreshToken(String refreshToken) {
this.refreshToken = refreshToken;
}
/**
*
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
*
* For more information about the features and limitations of the current IAM Identity Center OIDC implementation,
* see Considerations for Using this Guide in the IAM Identity Center OIDC API
* Reference.
*
*
* @return Used only when calling this API for the Refresh Token grant type. This token is used to refresh
* short-term tokens, such as the access token, that might expire.
*
* For more information about the features and limitations of the current IAM Identity Center OIDC
* implementation, see Considerations for Using this Guide in the IAM Identity Center
* OIDC API Reference.
*/
public String getRefreshToken() {
return this.refreshToken;
}
/**
*
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
*
* For more information about the features and limitations of the current IAM Identity Center OIDC implementation,
* see Considerations for Using this Guide in the IAM Identity Center OIDC API
* Reference.
*
*
* @param refreshToken
* Used only when calling this API for the Refresh Token grant type. This token is used to refresh short-term
* tokens, such as the access token, that might expire.
*
* For more information about the features and limitations of the current IAM Identity Center OIDC
* implementation, see Considerations for Using this Guide in the IAM Identity Center
* OIDC API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withRefreshToken(String refreshToken) {
setRefreshToken(refreshToken);
return this;
}
/**
*
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token (JWT)
* issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer GrantOptions for
* the application.
*
*
* @param assertion
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token
* (JWT) issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer
* GrantOptions for the application.
*/
public void setAssertion(String assertion) {
this.assertion = assertion;
}
/**
*
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token (JWT)
* issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer GrantOptions for
* the application.
*
*
* @return Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token
* (JWT) issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer
* GrantOptions for the application.
*/
public String getAssertion() {
return this.assertion;
}
/**
*
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token (JWT)
* issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer GrantOptions for
* the application.
*
*
* @param assertion
* Used only when calling this API for the JWT Bearer grant type. This value specifies the JSON Web Token
* (JWT) issued by a trusted token issuer. To authorize a trusted token issuer, configure the JWT Bearer
* GrantOptions for the application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withAssertion(String assertion) {
setAssertion(assertion);
return this;
}
/**
*
* The list of scopes for which authorization is requested. The access token that is issued is limited to the scopes
* that are granted. If the value is not specified, IAM Identity Center authorizes all scopes configured for the
* application, including the following default scopes: openid
, aws
,
* sts:identity_context
.
*
*
* @return The list of scopes for which authorization is requested. The access token that is issued is limited to
* the scopes that are granted. If the value is not specified, IAM Identity Center authorizes all scopes
* configured for the application, including the following default scopes: openid
,
* aws
, sts:identity_context
.
*/
public java.util.List getScope() {
return scope;
}
/**
*
* The list of scopes for which authorization is requested. The access token that is issued is limited to the scopes
* that are granted. If the value is not specified, IAM Identity Center authorizes all scopes configured for the
* application, including the following default scopes: openid
, aws
,
* sts:identity_context
.
*
*
* @param scope
* The list of scopes for which authorization is requested. The access token that is issued is limited to the
* scopes that are granted. If the value is not specified, IAM Identity Center authorizes all scopes
* configured for the application, including the following default scopes: openid
,
* aws
, sts:identity_context
.
*/
public void setScope(java.util.Collection scope) {
if (scope == null) {
this.scope = null;
return;
}
this.scope = new java.util.ArrayList(scope);
}
/**
*
* The list of scopes for which authorization is requested. The access token that is issued is limited to the scopes
* that are granted. If the value is not specified, IAM Identity Center authorizes all scopes configured for the
* application, including the following default scopes: openid
, aws
,
* sts:identity_context
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setScope(java.util.Collection)} or {@link #withScope(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param scope
* The list of scopes for which authorization is requested. The access token that is issued is limited to the
* scopes that are granted. If the value is not specified, IAM Identity Center authorizes all scopes
* configured for the application, including the following default scopes: openid
,
* aws
, sts:identity_context
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withScope(String... scope) {
if (this.scope == null) {
setScope(new java.util.ArrayList(scope.length));
}
for (String ele : scope) {
this.scope.add(ele);
}
return this;
}
/**
*
* The list of scopes for which authorization is requested. The access token that is issued is limited to the scopes
* that are granted. If the value is not specified, IAM Identity Center authorizes all scopes configured for the
* application, including the following default scopes: openid
, aws
,
* sts:identity_context
.
*
*
* @param scope
* The list of scopes for which authorization is requested. The access token that is issued is limited to the
* scopes that are granted. If the value is not specified, IAM Identity Center authorizes all scopes
* configured for the application, including the following default scopes: openid
,
* aws
, sts:identity_context
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withScope(java.util.Collection scope) {
setScope(scope);
return this;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value specifies the location of the
* client or application that has registered to receive the authorization code.
*
*
* @param redirectUri
* Used only when calling this API for the Authorization Code grant type. This value specifies the location
* of the client or application that has registered to receive the authorization code.
*/
public void setRedirectUri(String redirectUri) {
this.redirectUri = redirectUri;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value specifies the location of the
* client or application that has registered to receive the authorization code.
*
*
* @return Used only when calling this API for the Authorization Code grant type. This value specifies the location
* of the client or application that has registered to receive the authorization code.
*/
public String getRedirectUri() {
return this.redirectUri;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value specifies the location of the
* client or application that has registered to receive the authorization code.
*
*
* @param redirectUri
* Used only when calling this API for the Authorization Code grant type. This value specifies the location
* of the client or application that has registered to receive the authorization code.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withRedirectUri(String redirectUri) {
setRedirectUri(redirectUri);
return this;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a different
* client or application. The access token must have authorized scopes that indicate the requested application as a
* target audience.
*
*
* @param subjectToken
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a
* different client or application. The access token must have authorized scopes that indicate the requested
* application as a target audience.
*/
public void setSubjectToken(String subjectToken) {
this.subjectToken = subjectToken;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a different
* client or application. The access token must have authorized scopes that indicate the requested application as a
* target audience.
*
*
* @return Used only when calling this API for the Token Exchange grant type. This value specifies the subject of
* the exchange. The value of the subject token must be an access token issued by IAM Identity Center to a
* different client or application. The access token must have authorized scopes that indicate the requested
* application as a target audience.
*/
public String getSubjectToken() {
return this.subjectToken;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a different
* client or application. The access token must have authorized scopes that indicate the requested application as a
* target audience.
*
*
* @param subjectToken
* Used only when calling this API for the Token Exchange grant type. This value specifies the subject of the
* exchange. The value of the subject token must be an access token issued by IAM Identity Center to a
* different client or application. The access token must have authorized scopes that indicate the requested
* application as a target audience.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withSubjectToken(String subjectToken) {
setSubjectToken(subjectToken);
return this;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that is
* passed as the subject of the exchange. The following value is supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* @param subjectTokenType
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that is passed as the subject of the exchange. The following value is supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
*/
public void setSubjectTokenType(String subjectTokenType) {
this.subjectTokenType = subjectTokenType;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that is
* passed as the subject of the exchange. The following value is supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* @return Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that is passed as the subject of the exchange. The following value is supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
*/
public String getSubjectTokenType() {
return this.subjectTokenType;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that is
* passed as the subject of the exchange. The following value is supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* @param subjectTokenType
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that is passed as the subject of the exchange. The following value is supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withSubjectTokenType(String subjectTokenType) {
setSubjectTokenType(subjectTokenType);
return this;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that
* the requester can receive. The following values are supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* * Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*
*
* @param requestedTokenType
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that the requester can receive. The following values are supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*/
public void setRequestedTokenType(String requestedTokenType) {
this.requestedTokenType = requestedTokenType;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that
* the requester can receive. The following values are supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* * Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*
*
* @return Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that the requester can receive. The following values are supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*/
public String getRequestedTokenType() {
return this.requestedTokenType;
}
/**
*
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token that
* the requester can receive. The following values are supported:
*
*
* * Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* * Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
*
*
* @param requestedTokenType
* Used only when calling this API for the Token Exchange grant type. This value specifies the type of token
* that the requester can receive. The following values are supported:
*
* Access Token - urn:ietf:params:oauth:token-type:access_token
*
*
* Refresh Token - urn:ietf:params:oauth:token-type:refresh_token
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withRequestedTokenType(String requestedTokenType) {
setRequestedTokenType(requestedTokenType);
return this;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value is generated by the client and
* presented to validate the original code challenge value the client passed at authorization time.
*
*
* @param codeVerifier
* Used only when calling this API for the Authorization Code grant type. This value is generated by the
* client and presented to validate the original code challenge value the client passed at authorization
* time.
*/
public void setCodeVerifier(String codeVerifier) {
this.codeVerifier = codeVerifier;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value is generated by the client and
* presented to validate the original code challenge value the client passed at authorization time.
*
*
* @return Used only when calling this API for the Authorization Code grant type. This value is generated by the
* client and presented to validate the original code challenge value the client passed at authorization
* time.
*/
public String getCodeVerifier() {
return this.codeVerifier;
}
/**
*
* Used only when calling this API for the Authorization Code grant type. This value is generated by the client and
* presented to validate the original code challenge value the client passed at authorization time.
*
*
* @param codeVerifier
* Used only when calling this API for the Authorization Code grant type. This value is generated by the
* client and presented to validate the original code challenge value the client passed at authorization
* time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTokenWithIAMRequest withCodeVerifier(String codeVerifier) {
setCodeVerifier(codeVerifier);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientId() != null)
sb.append("ClientId: ").append(getClientId()).append(",");
if (getGrantType() != null)
sb.append("GrantType: ").append(getGrantType()).append(",");
if (getCode() != null)
sb.append("Code: ").append(getCode()).append(",");
if (getRefreshToken() != null)
sb.append("RefreshToken: ").append("***Sensitive Data Redacted***").append(",");
if (getAssertion() != null)
sb.append("Assertion: ").append("***Sensitive Data Redacted***").append(",");
if (getScope() != null)
sb.append("Scope: ").append(getScope()).append(",");
if (getRedirectUri() != null)
sb.append("RedirectUri: ").append(getRedirectUri()).append(",");
if (getSubjectToken() != null)
sb.append("SubjectToken: ").append("***Sensitive Data Redacted***").append(",");
if (getSubjectTokenType() != null)
sb.append("SubjectTokenType: ").append(getSubjectTokenType()).append(",");
if (getRequestedTokenType() != null)
sb.append("RequestedTokenType: ").append(getRequestedTokenType()).append(",");
if (getCodeVerifier() != null)
sb.append("CodeVerifier: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTokenWithIAMRequest == false)
return false;
CreateTokenWithIAMRequest other = (CreateTokenWithIAMRequest) obj;
if (other.getClientId() == null ^ this.getClientId() == null)
return false;
if (other.getClientId() != null && other.getClientId().equals(this.getClientId()) == false)
return false;
if (other.getGrantType() == null ^ this.getGrantType() == null)
return false;
if (other.getGrantType() != null && other.getGrantType().equals(this.getGrantType()) == false)
return false;
if (other.getCode() == null ^ this.getCode() == null)
return false;
if (other.getCode() != null && other.getCode().equals(this.getCode()) == false)
return false;
if (other.getRefreshToken() == null ^ this.getRefreshToken() == null)
return false;
if (other.getRefreshToken() != null && other.getRefreshToken().equals(this.getRefreshToken()) == false)
return false;
if (other.getAssertion() == null ^ this.getAssertion() == null)
return false;
if (other.getAssertion() != null && other.getAssertion().equals(this.getAssertion()) == false)
return false;
if (other.getScope() == null ^ this.getScope() == null)
return false;
if (other.getScope() != null && other.getScope().equals(this.getScope()) == false)
return false;
if (other.getRedirectUri() == null ^ this.getRedirectUri() == null)
return false;
if (other.getRedirectUri() != null && other.getRedirectUri().equals(this.getRedirectUri()) == false)
return false;
if (other.getSubjectToken() == null ^ this.getSubjectToken() == null)
return false;
if (other.getSubjectToken() != null && other.getSubjectToken().equals(this.getSubjectToken()) == false)
return false;
if (other.getSubjectTokenType() == null ^ this.getSubjectTokenType() == null)
return false;
if (other.getSubjectTokenType() != null && other.getSubjectTokenType().equals(this.getSubjectTokenType()) == false)
return false;
if (other.getRequestedTokenType() == null ^ this.getRequestedTokenType() == null)
return false;
if (other.getRequestedTokenType() != null && other.getRequestedTokenType().equals(this.getRequestedTokenType()) == false)
return false;
if (other.getCodeVerifier() == null ^ this.getCodeVerifier() == null)
return false;
if (other.getCodeVerifier() != null && other.getCodeVerifier().equals(this.getCodeVerifier()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientId() == null) ? 0 : getClientId().hashCode());
hashCode = prime * hashCode + ((getGrantType() == null) ? 0 : getGrantType().hashCode());
hashCode = prime * hashCode + ((getCode() == null) ? 0 : getCode().hashCode());
hashCode = prime * hashCode + ((getRefreshToken() == null) ? 0 : getRefreshToken().hashCode());
hashCode = prime * hashCode + ((getAssertion() == null) ? 0 : getAssertion().hashCode());
hashCode = prime * hashCode + ((getScope() == null) ? 0 : getScope().hashCode());
hashCode = prime * hashCode + ((getRedirectUri() == null) ? 0 : getRedirectUri().hashCode());
hashCode = prime * hashCode + ((getSubjectToken() == null) ? 0 : getSubjectToken().hashCode());
hashCode = prime * hashCode + ((getSubjectTokenType() == null) ? 0 : getSubjectTokenType().hashCode());
hashCode = prime * hashCode + ((getRequestedTokenType() == null) ? 0 : getRequestedTokenType().hashCode());
hashCode = prime * hashCode + ((getCodeVerifier() == null) ? 0 : getCodeVerifier().hashCode());
return hashCode;
}
@Override
public CreateTokenWithIAMRequest clone() {
return (CreateTokenWithIAMRequest) super.clone();
}
}