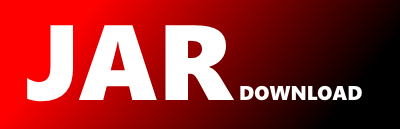
com.amazonaws.services.storagegateway.AWSStorageGatewayAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not
* use this file except in compliance with the License. A copy of the License is
* located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.storagegateway;
import com.amazonaws.services.storagegateway.model.*;
/**
* Interface for accessing AWS Storage Gateway asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* AWS Storage Gateway Service
*
* AWS Storage Gateway is the service that connects an on-premises software
* appliance with cloud-based storage to provide seamless and secure integration
* between an organization's on-premises IT environment and AWS's storage
* infrastructure. The service enables you to securely upload data to the AWS
* cloud for cost effective backup and rapid disaster recovery.
*
*
* Use the following links to get started using the AWS Storage Gateway
* Service API Reference:
*
*
* -
*
* AWS Storage Gateway Required Request Headers: Describes the required
* headers that you must send with every POST request to AWS Storage Gateway.
*
*
* -
*
* Signing Requests: AWS Storage Gateway requires that you authenticate
* every request you send; this topic describes how sign such a request.
*
*
* -
*
* Error Responses: Provides reference information about AWS Storage
* Gateway errors.
*
*
* -
*
* Operations in AWS Storage Gateway: Contains detailed descriptions of all
* AWS Storage Gateway operations, their request parameters, response elements,
* possible errors, and examples of requests and responses.
*
*
* -
*
*
* AWS Storage Gateway Regions and Endpoints: Provides a list of each of the
* s and endpoints available for use with AWS Storage Gateway.
*
*
*
*
*
* AWS Storage Gateway resource IDs are in uppercase. When you use these
* resource IDs with the Amazon EC2 API, EC2 expects resource IDs in lowercase.
* You must change your resource ID to lowercase to use it with the EC2 API. For
* example, in Storage Gateway the ID for a volume might be
* vol-1122AABB
. When you use this ID with the EC2 API, you must
* change it to vol-1122aabb
. Otherwise, the EC2 API might not
* behave as expected.
*
*
*
* IDs for Storage Gateway volumes and Amazon EBS snapshots created from gateway
* volumes are changing to a longer format. Starting in December 2016, all new
* volumes and snapshots will be created with a 17-character string. Starting in
* April 2016, you will be able to use these longer IDs so you can test your
* systems with the new format. For more information, see Longer EC2 and EBS
* Resource IDs.
*
*
* For example, a volume ARN with the longer volume ID format will look like
* this:
*
*
* arn:aws:storagegateway:us-west-2:111122223333:gateway/sgw-12A3456B/volume/vol-1122AABBCCDDEEFFG
* .
*
*
* A snapshot ID with the longer ID format will look like this:
* snap-78e226633445566ee
.
*
*
* For more information, see Announcement:
* Heads-up – Longer AWS Storage Gateway volume and snapshot IDs coming in
* 2016.
*
*
*/
public interface AWSStorageGatewayAsync extends AWSStorageGateway {
/**
*
* Activates the gateway you previously deployed on your host. For more
* information, see Activate the AWS Storage Gateway. In the activation process, you
* specify information such as the you want to use for storing snapshots,
* the time zone for scheduled snapshots the gateway snapshot schedule
* window, an activation key, and a name for your gateway. The activation
* process also associates your gateway with your account; for more
* information, see UpdateGatewayInformation.
*
*
*
* You must turn on the gateway VM before you can activate your gateway.
*
*
*
* @param activateGatewayRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ActivateGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsync.ActivateGateway
*/
java.util.concurrent.Future activateGatewayAsync(
ActivateGatewayRequest activateGatewayRequest);
/**
*
* Activates the gateway you previously deployed on your host. For more
* information, see Activate the AWS Storage Gateway. In the activation process, you
* specify information such as the you want to use for storing snapshots,
* the time zone for scheduled snapshots the gateway snapshot schedule
* window, an activation key, and a name for your gateway. The activation
* process also associates your gateway with your account; for more
* information, see UpdateGatewayInformation.
*
*
*
* You must turn on the gateway VM before you can activate your gateway.
*
*
*
* @param activateGatewayRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ActivateGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ActivateGateway
*/
java.util.concurrent.Future activateGatewayAsync(
ActivateGatewayRequest activateGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as cache for a cached-volume
* gateway. This operation is supported only for the gateway-cached volume
* architecture (see Storage Gateway Concepts).
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add cache, and one or more disk IDs that you want to
* configure as cache.
*
*
* @param addCacheRequest
* @return A Java Future containing the result of the AddCache operation
* returned by the service.
* @sample AWSStorageGatewayAsync.AddCache
*/
java.util.concurrent.Future addCacheAsync(
AddCacheRequest addCacheRequest);
/**
*
* Configures one or more gateway local disks as cache for a cached-volume
* gateway. This operation is supported only for the gateway-cached volume
* architecture (see Storage Gateway Concepts).
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add cache, and one or more disk IDs that you want to
* configure as cache.
*
*
* @param addCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddCache operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddCache
*/
java.util.concurrent.Future addCacheAsync(
AddCacheRequest addCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags to the specified resource. You use tags to add
* metadata to resources, which you can use to categorize these resources.
* For example, you can categorize resources by purpose, owner, environment,
* or team. Each tag consists of a key and a value, which you define. You
* can add tags to the following AWS Storage Gateway resources:
*
*
* -
*
* Storage gateways of all types
*
*
*
*
* -
*
* Storage Volumes
*
*
*
*
* -
*
* Virtual Tapes
*
*
*
*
* You can create a maximum of 10 tags for each resource. Virtual tapes and
* storage volumes that are recovered to a new gateway maintain their tags.
*
*
* @param addTagsToResourceRequest
* AddTagsToResourceInput
* @return A Java Future containing the result of the AddTagsToResource
* operation returned by the service.
* @sample AWSStorageGatewayAsync.AddTagsToResource
*/
java.util.concurrent.Future addTagsToResourceAsync(
AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds one or more tags to the specified resource. You use tags to add
* metadata to resources, which you can use to categorize these resources.
* For example, you can categorize resources by purpose, owner, environment,
* or team. Each tag consists of a key and a value, which you define. You
* can add tags to the following AWS Storage Gateway resources:
*
*
* -
*
* Storage gateways of all types
*
*
*
*
* -
*
* Storage Volumes
*
*
*
*
* -
*
* Virtual Tapes
*
*
*
*
* You can create a maximum of 10 tags for each resource. Virtual tapes and
* storage volumes that are recovered to a new gateway maintain their tags.
*
*
* @param addTagsToResourceRequest
* AddTagsToResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddTagsToResource
*/
java.util.concurrent.Future addTagsToResourceAsync(
AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as upload buffer for a
* specified gateway. This operation is supported for both the
* gateway-stored and gateway-cached volume architectures.
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add upload buffer, and one or more disk IDs that you
* want to configure as upload buffer.
*
*
* @param addUploadBufferRequest
* @return A Java Future containing the result of the AddUploadBuffer
* operation returned by the service.
* @sample AWSStorageGatewayAsync.AddUploadBuffer
*/
java.util.concurrent.Future addUploadBufferAsync(
AddUploadBufferRequest addUploadBufferRequest);
/**
*
* Configures one or more gateway local disks as upload buffer for a
* specified gateway. This operation is supported for both the
* gateway-stored and gateway-cached volume architectures.
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add upload buffer, and one or more disk IDs that you
* want to configure as upload buffer.
*
*
* @param addUploadBufferRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddUploadBuffer
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddUploadBuffer
*/
java.util.concurrent.Future addUploadBufferAsync(
AddUploadBufferRequest addUploadBufferRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as working storage for a
* gateway. This operation is supported only for the gateway-stored volume
* architecture. This operation is deprecated in cached-volumes API version
* 20120630. Use AddUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use
* the AddUploadBuffer operation to add upload buffer to a
* stored-volume gateway.
*
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add working storage, and one or more disk IDs that you
* want to configure as working storage.
*
*
* @param addWorkingStorageRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* @return A Java Future containing the result of the AddWorkingStorage
* operation returned by the service.
* @sample AWSStorageGatewayAsync.AddWorkingStorage
*/
java.util.concurrent.Future addWorkingStorageAsync(
AddWorkingStorageRequest addWorkingStorageRequest);
/**
*
* Configures one or more gateway local disks as working storage for a
* gateway. This operation is supported only for the gateway-stored volume
* architecture. This operation is deprecated in cached-volumes API version
* 20120630. Use AddUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use
* the AddUploadBuffer operation to add upload buffer to a
* stored-volume gateway.
*
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to
* which you want to add working storage, and one or more disk IDs that you
* want to configure as working storage.
*
*
* @param addWorkingStorageRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddWorkingStorage
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddWorkingStorage
*/
java.util.concurrent.Future addWorkingStorageAsync(
AddWorkingStorageRequest addWorkingStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels archiving of a virtual tape to the virtual tape shelf (VTS) after
* the archiving process is initiated.
*
*
* @param cancelArchivalRequest
* CancelArchivalInput
* @return A Java Future containing the result of the CancelArchival
* operation returned by the service.
* @sample AWSStorageGatewayAsync.CancelArchival
*/
java.util.concurrent.Future cancelArchivalAsync(
CancelArchivalRequest cancelArchivalRequest);
/**
*
* Cancels archiving of a virtual tape to the virtual tape shelf (VTS) after
* the archiving process is initiated.
*
*
* @param cancelArchivalRequest
* CancelArchivalInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelArchival
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CancelArchival
*/
java.util.concurrent.Future cancelArchivalAsync(
CancelArchivalRequest cancelArchivalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels retrieval of a virtual tape from the virtual tape shelf (VTS) to
* a gateway after the retrieval process is initiated. The virtual tape is
* returned to the VTS.
*
*
* @param cancelRetrievalRequest
* CancelRetrievalInput
* @return A Java Future containing the result of the CancelRetrieval
* operation returned by the service.
* @sample AWSStorageGatewayAsync.CancelRetrieval
*/
java.util.concurrent.Future cancelRetrievalAsync(
CancelRetrievalRequest cancelRetrievalRequest);
/**
*
* Cancels retrieval of a virtual tape from the virtual tape shelf (VTS) to
* a gateway after the retrieval process is initiated. The virtual tape is
* returned to the VTS.
*
*
* @param cancelRetrievalRequest
* CancelRetrievalInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelRetrieval
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CancelRetrieval
*/
java.util.concurrent.Future cancelRetrievalAsync(
CancelRetrievalRequest cancelRetrievalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a cached volume on a specified cached gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
*
* Cache storage must be allocated to the gateway before you can create a
* cached volume. Use the AddCache operation to add cache storage to
* a gateway.
*
*
*
* In the request, you must specify the gateway, size of the volume in
* bytes, the iSCSI target name, an IP address on which to expose the
* target, and a unique client token. In response, AWS Storage Gateway
* creates the volume and returns information about it such as the volume
* Amazon Resource Name (ARN), its size, and the iSCSI target ARN that
* initiators can use to connect to the volume target.
*
*
* @param createCachediSCSIVolumeRequest
* @return A Java Future containing the result of the
* CreateCachediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateCachediSCSIVolume
*/
java.util.concurrent.Future createCachediSCSIVolumeAsync(
CreateCachediSCSIVolumeRequest createCachediSCSIVolumeRequest);
/**
*
* Creates a cached volume on a specified cached gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
*
* Cache storage must be allocated to the gateway before you can create a
* cached volume. Use the AddCache operation to add cache storage to
* a gateway.
*
*
*
* In the request, you must specify the gateway, size of the volume in
* bytes, the iSCSI target name, an IP address on which to expose the
* target, and a unique client token. In response, AWS Storage Gateway
* creates the volume and returns information about it such as the volume
* Amazon Resource Name (ARN), its size, and the iSCSI target ARN that
* initiators can use to connect to the volume target.
*
*
* @param createCachediSCSIVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateCachediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateCachediSCSIVolume
*/
java.util.concurrent.Future createCachediSCSIVolumeAsync(
CreateCachediSCSIVolumeRequest createCachediSCSIVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a snapshot of a volume.
*
*
* AWS Storage Gateway provides the ability to back up point-in-time
* snapshots of your data to Amazon Simple Storage (S3) for durable off-site
* recovery, as well as import the data to an Amazon Elastic Block Store
* (EBS) volume in Amazon Elastic Compute Cloud (EC2). You can take
* snapshots of your gateway volume on a scheduled or ad-hoc basis. This API
* enables you to take ad-hoc snapshot. For more information, see Working With Snapshots in the AWS Storage Gateway Console.
*
*
* In the CreateSnapshot request you identify the volume by providing its
* Amazon Resource Name (ARN). You must also provide description for the
* snapshot. When AWS Storage Gateway takes the snapshot of specified
* volume, the snapshot and description appears in the AWS Storage Gateway
* Console. In response, AWS Storage Gateway returns you a snapshot ID. You
* can use this snapshot ID to check the snapshot progress or later use it
* when you want to create a volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more
* information, see DescribeSnapshots or DeleteSnapshot in the EC2 API reference.
*
*
*
* Volume and snapshot IDs are changing to a longer length ID format. For
* more information, see the important note on the Welcome page.
*
*
*
* @param createSnapshotRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the CreateSnapshot
* operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateSnapshot
*/
java.util.concurrent.Future createSnapshotAsync(
CreateSnapshotRequest createSnapshotRequest);
/**
*
* Initiates a snapshot of a volume.
*
*
* AWS Storage Gateway provides the ability to back up point-in-time
* snapshots of your data to Amazon Simple Storage (S3) for durable off-site
* recovery, as well as import the data to an Amazon Elastic Block Store
* (EBS) volume in Amazon Elastic Compute Cloud (EC2). You can take
* snapshots of your gateway volume on a scheduled or ad-hoc basis. This API
* enables you to take ad-hoc snapshot. For more information, see Working With Snapshots in the AWS Storage Gateway Console.
*
*
* In the CreateSnapshot request you identify the volume by providing its
* Amazon Resource Name (ARN). You must also provide description for the
* snapshot. When AWS Storage Gateway takes the snapshot of specified
* volume, the snapshot and description appears in the AWS Storage Gateway
* Console. In response, AWS Storage Gateway returns you a snapshot ID. You
* can use this snapshot ID to check the snapshot progress or later use it
* when you want to create a volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more
* information, see DescribeSnapshots or DeleteSnapshot in the EC2 API reference.
*
*
*
* Volume and snapshot IDs are changing to a longer length ID format. For
* more information, see the important note on the Welcome page.
*
*
*
* @param createSnapshotRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateSnapshot
*/
java.util.concurrent.Future createSnapshotAsync(
CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a snapshot of a gateway from a volume recovery point. This
* operation is supported only for the gateway-cached volume architecture.
*
*
* A volume recovery point is a point in time at which all data of the
* volume is consistent and from which you can create a snapshot. To get a
* list of volume recovery point for gateway-cached volumes, use
* ListVolumeRecoveryPoints.
*
*
* In the CreateSnapshotFromVolumeRecoveryPoint
request, you
* identify the volume by providing its Amazon Resource Name (ARN). You must
* also provide a description for the snapshot. When AWS Storage Gateway
* takes a snapshot of the specified volume, the snapshot and its
* description appear in the AWS Storage Gateway console. In response, AWS
* Storage Gateway returns you a snapshot ID. You can use this snapshot ID
* to check the snapshot progress or later use it when you want to create a
* volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more
* information, in Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param createSnapshotFromVolumeRecoveryPointRequest
* @return A Java Future containing the result of the
* CreateSnapshotFromVolumeRecoveryPoint operation returned by the
* service.
* @sample AWSStorageGatewayAsync.CreateSnapshotFromVolumeRecoveryPoint
*/
java.util.concurrent.Future createSnapshotFromVolumeRecoveryPointAsync(
CreateSnapshotFromVolumeRecoveryPointRequest createSnapshotFromVolumeRecoveryPointRequest);
/**
*
* Initiates a snapshot of a gateway from a volume recovery point. This
* operation is supported only for the gateway-cached volume architecture.
*
*
* A volume recovery point is a point in time at which all data of the
* volume is consistent and from which you can create a snapshot. To get a
* list of volume recovery point for gateway-cached volumes, use
* ListVolumeRecoveryPoints.
*
*
* In the CreateSnapshotFromVolumeRecoveryPoint
request, you
* identify the volume by providing its Amazon Resource Name (ARN). You must
* also provide a description for the snapshot. When AWS Storage Gateway
* takes a snapshot of the specified volume, the snapshot and its
* description appear in the AWS Storage Gateway console. In response, AWS
* Storage Gateway returns you a snapshot ID. You can use this snapshot ID
* to check the snapshot progress or later use it when you want to create a
* volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more
* information, in Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param createSnapshotFromVolumeRecoveryPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateSnapshotFromVolumeRecoveryPoint operation returned by the
* service.
* @sample
* AWSStorageGatewayAsyncHandler.CreateSnapshotFromVolumeRecoveryPoint
*/
java.util.concurrent.Future createSnapshotFromVolumeRecoveryPointAsync(
CreateSnapshotFromVolumeRecoveryPointRequest createSnapshotFromVolumeRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a volume on a specified gateway. This operation is supported only
* for the gateway-stored volume architecture.
*
*
* The size of the volume to create is inferred from the disk size. You can
* choose to preserve existing data on the disk, create volume from an
* existing snapshot, or create an empty volume. If you choose to create an
* empty gateway volume, then any existing data on the disk is erased.
*
*
* In the request you must specify the gateway and the disk information on
* which you are creating the volume. In response, AWS Storage Gateway
* creates the volume and returns volume information such as the volume
* Amazon Resource Name (ARN), its size, and the iSCSI target ARN that
* initiators can use to connect to the volume target.
*
*
* @param createStorediSCSIVolumeRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the
* CreateStorediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateStorediSCSIVolume
*/
java.util.concurrent.Future createStorediSCSIVolumeAsync(
CreateStorediSCSIVolumeRequest createStorediSCSIVolumeRequest);
/**
*
* Creates a volume on a specified gateway. This operation is supported only
* for the gateway-stored volume architecture.
*
*
* The size of the volume to create is inferred from the disk size. You can
* choose to preserve existing data on the disk, create volume from an
* existing snapshot, or create an empty volume. If you choose to create an
* empty gateway volume, then any existing data on the disk is erased.
*
*
* In the request you must specify the gateway and the disk information on
* which you are creating the volume. In response, AWS Storage Gateway
* creates the volume and returns volume information such as the volume
* Amazon Resource Name (ARN), its size, and the iSCSI target ARN that
* initiators can use to connect to the volume target.
*
*
* @param createStorediSCSIVolumeRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* CreateStorediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateStorediSCSIVolume
*/
java.util.concurrent.Future createStorediSCSIVolumeAsync(
CreateStorediSCSIVolumeRequest createStorediSCSIVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual tape by using your own barcode. You write data to the
* virtual tape and then archive the tape.
*
*
*
* Cache storage must be allocated to the gateway before you can create a
* virtual tape. Use the AddCache operation to add cache storage to a
* gateway.
*
*
*
* @param createTapeWithBarcodeRequest
* CreateTapeWithBarcodeInput
* @return A Java Future containing the result of the CreateTapeWithBarcode
* operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateTapeWithBarcode
*/
java.util.concurrent.Future createTapeWithBarcodeAsync(
CreateTapeWithBarcodeRequest createTapeWithBarcodeRequest);
/**
*
* Creates a virtual tape by using your own barcode. You write data to the
* virtual tape and then archive the tape.
*
*
*
* Cache storage must be allocated to the gateway before you can create a
* virtual tape. Use the AddCache operation to add cache storage to a
* gateway.
*
*
*
* @param createTapeWithBarcodeRequest
* CreateTapeWithBarcodeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTapeWithBarcode
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateTapeWithBarcode
*/
java.util.concurrent.Future createTapeWithBarcodeAsync(
CreateTapeWithBarcodeRequest createTapeWithBarcodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates one or more virtual tapes. You write data to the virtual tapes
* and then archive the tapes.
*
*
*
* Cache storage must be allocated to the gateway before you can create
* virtual tapes. Use the AddCache operation to add cache storage to
* a gateway.
*
*
*
* @param createTapesRequest
* CreateTapesInput
* @return A Java Future containing the result of the CreateTapes operation
* returned by the service.
* @sample AWSStorageGatewayAsync.CreateTapes
*/
java.util.concurrent.Future createTapesAsync(
CreateTapesRequest createTapesRequest);
/**
*
* Creates one or more virtual tapes. You write data to the virtual tapes
* and then archive the tapes.
*
*
*
* Cache storage must be allocated to the gateway before you can create
* virtual tapes. Use the AddCache operation to add cache storage to
* a gateway.
*
*
*
* @param createTapesRequest
* CreateTapesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTapes operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateTapes
*/
java.util.concurrent.Future createTapesAsync(
CreateTapesRequest createTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the bandwidth rate limits of a gateway. You can delete either the
* upload and download bandwidth rate limit, or you can delete both. If you
* delete only one of the limits, the other limit remains unchanged. To
* specify which gateway to work with, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param deleteBandwidthRateLimitRequest
* @return A Java Future containing the result of the
* DeleteBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteBandwidthRateLimit
*/
java.util.concurrent.Future deleteBandwidthRateLimitAsync(
DeleteBandwidthRateLimitRequest deleteBandwidthRateLimitRequest);
/**
*
* Deletes the bandwidth rate limits of a gateway. You can delete either the
* upload and download bandwidth rate limit, or you can delete both. If you
* delete only one of the limits, the other limit remains unchanged. To
* specify which gateway to work with, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param deleteBandwidthRateLimitRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeleteBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteBandwidthRateLimit
*/
java.util.concurrent.Future deleteBandwidthRateLimitAsync(
DeleteBandwidthRateLimitRequest deleteBandwidthRateLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes Challenge-Handshake Authentication Protocol (CHAP) credentials
* for a specified iSCSI target and initiator pair.
*
*
* @param deleteChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the DeleteChapCredentials
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteChapCredentials
*/
java.util.concurrent.Future deleteChapCredentialsAsync(
DeleteChapCredentialsRequest deleteChapCredentialsRequest);
/**
*
* Deletes Challenge-Handshake Authentication Protocol (CHAP) credentials
* for a specified iSCSI target and initiator pair.
*
*
* @param deleteChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChapCredentials
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteChapCredentials
*/
java.util.concurrent.Future deleteChapCredentialsAsync(
DeleteChapCredentialsRequest deleteChapCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a gateway. To specify which gateway to delete, use the Amazon
* Resource Name (ARN) of the gateway in your request. The operation deletes
* the gateway; however, it does not delete the gateway virtual machine (VM)
* from your host computer.
*
*
* After you delete a gateway, you cannot reactivate it. Completed snapshots
* of the gateway volumes are not deleted upon deleting the gateway,
* however, pending snapshots will not complete. After you delete a gateway,
* your next step is to remove it from your environment.
*
*
*
* You no longer pay software charges after the gateway is deleted; however,
* your existing Amazon EBS snapshots persist and you will continue to be
* billed for these snapshots. You can choose to remove all remaining Amazon
* EBS snapshots by canceling your Amazon EC2 subscription. If you prefer
* not to cancel your Amazon EC2 subscription, you can delete your snapshots
* using the Amazon EC2 console. For more information, see the AWS Storage Gateway Detail
* Page.
*
*
*
* @param deleteGatewayRequest
* A JSON object containing the id of the gateway to delete.
* @return A Java Future containing the result of the DeleteGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteGateway
*/
java.util.concurrent.Future deleteGatewayAsync(
DeleteGatewayRequest deleteGatewayRequest);
/**
*
* Deletes a gateway. To specify which gateway to delete, use the Amazon
* Resource Name (ARN) of the gateway in your request. The operation deletes
* the gateway; however, it does not delete the gateway virtual machine (VM)
* from your host computer.
*
*
* After you delete a gateway, you cannot reactivate it. Completed snapshots
* of the gateway volumes are not deleted upon deleting the gateway,
* however, pending snapshots will not complete. After you delete a gateway,
* your next step is to remove it from your environment.
*
*
*
* You no longer pay software charges after the gateway is deleted; however,
* your existing Amazon EBS snapshots persist and you will continue to be
* billed for these snapshots. You can choose to remove all remaining Amazon
* EBS snapshots by canceling your Amazon EC2 subscription. If you prefer
* not to cancel your Amazon EC2 subscription, you can delete your snapshots
* using the Amazon EC2 console. For more information, see the AWS Storage Gateway Detail
* Page.
*
*
*
* @param deleteGatewayRequest
* A JSON object containing the id of the gateway to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteGateway
*/
java.util.concurrent.Future deleteGatewayAsync(
DeleteGatewayRequest deleteGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a snapshot of a volume.
*
*
* You can take snapshots of your gateway volumes on a scheduled or ad hoc
* basis. This API action enables you to delete a snapshot schedule for a
* volume. For more information, see Working with Snapshots. In the DeleteSnapshotSchedule
* request, you identify the volume by providing its Amazon Resource Name
* (ARN).
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. in
* Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param deleteSnapshotScheduleRequest
* @return A Java Future containing the result of the DeleteSnapshotSchedule
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteSnapshotSchedule
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(
DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest);
/**
*
* Deletes a snapshot of a volume.
*
*
* You can take snapshots of your gateway volumes on a scheduled or ad hoc
* basis. This API action enables you to delete a snapshot schedule for a
* volume. For more information, see Working with Snapshots. In the DeleteSnapshotSchedule
* request, you identify the volume by providing its Amazon Resource Name
* (ARN).
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. in
* Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param deleteSnapshotScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshotSchedule
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteSnapshotSchedule
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(
DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified virtual tape.
*
*
* @param deleteTapeRequest
* DeleteTapeInput
* @return A Java Future containing the result of the DeleteTape operation
* returned by the service.
* @sample AWSStorageGatewayAsync.DeleteTape
*/
java.util.concurrent.Future deleteTapeAsync(
DeleteTapeRequest deleteTapeRequest);
/**
*
* Deletes the specified virtual tape.
*
*
* @param deleteTapeRequest
* DeleteTapeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTape operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteTape
*/
java.util.concurrent.Future deleteTapeAsync(
DeleteTapeRequest deleteTapeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified virtual tape from the virtual tape shelf (VTS).
*
*
* @param deleteTapeArchiveRequest
* DeleteTapeArchiveInput
* @return A Java Future containing the result of the DeleteTapeArchive
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteTapeArchive
*/
java.util.concurrent.Future deleteTapeArchiveAsync(
DeleteTapeArchiveRequest deleteTapeArchiveRequest);
/**
*
* Deletes the specified virtual tape from the virtual tape shelf (VTS).
*
*
* @param deleteTapeArchiveRequest
* DeleteTapeArchiveInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTapeArchive
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteTapeArchive
*/
java.util.concurrent.Future deleteTapeArchiveAsync(
DeleteTapeArchiveRequest deleteTapeArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified gateway volume that you previously created using
* the CreateCachediSCSIVolume or CreateStorediSCSIVolume API.
* For gateway-stored volumes, the local disk that was configured as the
* storage volume is not deleted. You can reuse the local disk to create
* another storage volume.
*
*
* Before you delete a gateway volume, make sure there are no iSCSI
* connections to the volume you are deleting. You should also make sure
* there is no snapshot in progress. You can use the Amazon Elastic Compute
* Cloud (Amazon EC2) API to query snapshots on the volume you are deleting
* and check the snapshot status. For more information, go to DescribeSnapshots in the Amazon Elastic Compute Cloud API
* Reference.
*
*
* In the request, you must provide the Amazon Resource Name (ARN) of the
* storage volume you want to delete.
*
*
* @param deleteVolumeRequest
* A JSON object containing the DeleteVolumeInput$VolumeARN to
* delete.
* @return A Java Future containing the result of the DeleteVolume operation
* returned by the service.
* @sample AWSStorageGatewayAsync.DeleteVolume
*/
java.util.concurrent.Future deleteVolumeAsync(
DeleteVolumeRequest deleteVolumeRequest);
/**
*
* Deletes the specified gateway volume that you previously created using
* the CreateCachediSCSIVolume or CreateStorediSCSIVolume API.
* For gateway-stored volumes, the local disk that was configured as the
* storage volume is not deleted. You can reuse the local disk to create
* another storage volume.
*
*
* Before you delete a gateway volume, make sure there are no iSCSI
* connections to the volume you are deleting. You should also make sure
* there is no snapshot in progress. You can use the Amazon Elastic Compute
* Cloud (Amazon EC2) API to query snapshots on the volume you are deleting
* and check the snapshot status. For more information, go to DescribeSnapshots in the Amazon Elastic Compute Cloud API
* Reference.
*
*
* In the request, you must provide the Amazon Resource Name (ARN) of the
* storage volume you want to delete.
*
*
* @param deleteVolumeRequest
* A JSON object containing the DeleteVolumeInput$VolumeARN to
* delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVolume operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteVolume
*/
java.util.concurrent.Future deleteVolumeAsync(
DeleteVolumeRequest deleteVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the bandwidth rate limits of a gateway. By default, these limits
* are not set, which means no bandwidth rate limiting is in effect.
*
*
* This operation only returns a value for a bandwidth rate limit only if
* the limit is set. If no limits are set for the gateway, then this
* operation returns only the gateway ARN in the response body. To specify
* which gateway to describe, use the Amazon Resource Name (ARN) of the
* gateway in your request.
*
*
* @param describeBandwidthRateLimitRequest
* A JSON object containing the of the gateway.
* @return A Java Future containing the result of the
* DescribeBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeBandwidthRateLimit
*/
java.util.concurrent.Future describeBandwidthRateLimitAsync(
DescribeBandwidthRateLimitRequest describeBandwidthRateLimitRequest);
/**
*
* Returns the bandwidth rate limits of a gateway. By default, these limits
* are not set, which means no bandwidth rate limiting is in effect.
*
*
* This operation only returns a value for a bandwidth rate limit only if
* the limit is set. If no limits are set for the gateway, then this
* operation returns only the gateway ARN in the response body. To specify
* which gateway to describe, use the Amazon Resource Name (ARN) of the
* gateway in your request.
*
*
* @param describeBandwidthRateLimitRequest
* A JSON object containing the of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeBandwidthRateLimit
*/
java.util.concurrent.Future describeBandwidthRateLimitAsync(
DescribeBandwidthRateLimitRequest describeBandwidthRateLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the cache of a gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
* The response includes disk IDs that are configured as cache, and it
* includes the amount of cache allocated and used.
*
*
* @param describeCacheRequest
* @return A Java Future containing the result of the DescribeCache
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeCache
*/
java.util.concurrent.Future describeCacheAsync(
DescribeCacheRequest describeCacheRequest);
/**
*
* Returns information about the cache of a gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
* The response includes disk IDs that are configured as cache, and it
* includes the amount of cache allocated and used.
*
*
* @param describeCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCache
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeCache
*/
java.util.concurrent.Future describeCacheAsync(
DescribeCacheRequest describeCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the gateway volumes specified in the request.
* This operation is supported only for the gateway-cached volume
* architecture.
*
*
* The list of gateway volumes in the request must be from one gateway. In
* the response Amazon Storage Gateway returns volume information sorted by
* volume Amazon Resource Name (ARN).
*
*
* @param describeCachediSCSIVolumesRequest
* @return A Java Future containing the result of the
* DescribeCachediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeCachediSCSIVolumes
*/
java.util.concurrent.Future describeCachediSCSIVolumesAsync(
DescribeCachediSCSIVolumesRequest describeCachediSCSIVolumesRequest);
/**
*
* Returns a description of the gateway volumes specified in the request.
* This operation is supported only for the gateway-cached volume
* architecture.
*
*
* The list of gateway volumes in the request must be from one gateway. In
* the response Amazon Storage Gateway returns volume information sorted by
* volume Amazon Resource Name (ARN).
*
*
* @param describeCachediSCSIVolumesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeCachediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeCachediSCSIVolumes
*/
java.util.concurrent.Future describeCachediSCSIVolumesAsync(
DescribeCachediSCSIVolumesRequest describeCachediSCSIVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of Challenge-Handshake Authentication Protocol (CHAP)
* credentials information for a specified iSCSI target, one for each
* target-initiator pair.
*
*
* @param describeChapCredentialsRequest
* A JSON object containing the Amazon Resource Name (ARN) of the
* iSCSI volume target.
* @return A Java Future containing the result of the
* DescribeChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeChapCredentials
*/
java.util.concurrent.Future describeChapCredentialsAsync(
DescribeChapCredentialsRequest describeChapCredentialsRequest);
/**
*
* Returns an array of Challenge-Handshake Authentication Protocol (CHAP)
* credentials information for a specified iSCSI target, one for each
* target-initiator pair.
*
*
* @param describeChapCredentialsRequest
* A JSON object containing the Amazon Resource Name (ARN) of the
* iSCSI volume target.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeChapCredentials
*/
java.util.concurrent.Future describeChapCredentialsAsync(
DescribeChapCredentialsRequest describeChapCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about a gateway such as its name, network interfaces,
* configured time zone, and the state (whether the gateway is running or
* not). To specify which gateway to describe, use the Amazon Resource Name
* (ARN) of the gateway in your request.
*
*
* @param describeGatewayInformationRequest
* A JSON object containing the id of the gateway.
* @return A Java Future containing the result of the
* DescribeGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeGatewayInformation
*/
java.util.concurrent.Future describeGatewayInformationAsync(
DescribeGatewayInformationRequest describeGatewayInformationRequest);
/**
*
* Returns metadata about a gateway such as its name, network interfaces,
* configured time zone, and the state (whether the gateway is running or
* not). To specify which gateway to describe, use the Amazon Resource Name
* (ARN) of the gateway in your request.
*
*
* @param describeGatewayInformationRequest
* A JSON object containing the id of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeGatewayInformation
*/
java.util.concurrent.Future describeGatewayInformationAsync(
DescribeGatewayInformationRequest describeGatewayInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns your gateway's weekly maintenance start time including the day
* and time of the week. Note that values are in terms of the gateway's time
* zone.
*
*
* @param describeMaintenanceStartTimeRequest
* A JSON object containing the of the gateway.
* @return A Java Future containing the result of the
* DescribeMaintenanceStartTime operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeMaintenanceStartTime
*/
java.util.concurrent.Future describeMaintenanceStartTimeAsync(
DescribeMaintenanceStartTimeRequest describeMaintenanceStartTimeRequest);
/**
*
* Returns your gateway's weekly maintenance start time including the day
* and time of the week. Note that values are in terms of the gateway's time
* zone.
*
*
* @param describeMaintenanceStartTimeRequest
* A JSON object containing the of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeMaintenanceStartTime operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeMaintenanceStartTime
*/
java.util.concurrent.Future describeMaintenanceStartTimeAsync(
DescribeMaintenanceStartTimeRequest describeMaintenanceStartTimeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the snapshot schedule for the specified gateway volume. The
* snapshot schedule information includes intervals at which snapshots are
* automatically initiated on the volume.
*
*
* @param describeSnapshotScheduleRequest
* A JSON object containing the
* DescribeSnapshotScheduleInput$VolumeARN of the volume.
* @return A Java Future containing the result of the
* DescribeSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeSnapshotSchedule
*/
java.util.concurrent.Future describeSnapshotScheduleAsync(
DescribeSnapshotScheduleRequest describeSnapshotScheduleRequest);
/**
*
* Describes the snapshot schedule for the specified gateway volume. The
* snapshot schedule information includes intervals at which snapshots are
* automatically initiated on the volume.
*
*
* @param describeSnapshotScheduleRequest
* A JSON object containing the
* DescribeSnapshotScheduleInput$VolumeARN of the volume.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeSnapshotSchedule
*/
java.util.concurrent.Future describeSnapshotScheduleAsync(
DescribeSnapshotScheduleRequest describeSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of the gateway volumes specified in the request.
* The list of gateway volumes in the request must be from one gateway. In
* the response Amazon Storage Gateway returns volume information sorted by
* volume ARNs.
*
*
* @param describeStorediSCSIVolumesRequest
* A JSON object containing a list of
* DescribeStorediSCSIVolumesInput$VolumeARNs.
* @return A Java Future containing the result of the
* DescribeStorediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeStorediSCSIVolumes
*/
java.util.concurrent.Future describeStorediSCSIVolumesAsync(
DescribeStorediSCSIVolumesRequest describeStorediSCSIVolumesRequest);
/**
*
* Returns the description of the gateway volumes specified in the request.
* The list of gateway volumes in the request must be from one gateway. In
* the response Amazon Storage Gateway returns volume information sorted by
* volume ARNs.
*
*
* @param describeStorediSCSIVolumesRequest
* A JSON object containing a list of
* DescribeStorediSCSIVolumesInput$VolumeARNs.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeStorediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeStorediSCSIVolumes
*/
java.util.concurrent.Future describeStorediSCSIVolumesAsync(
DescribeStorediSCSIVolumesRequest describeStorediSCSIVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of specified virtual tapes in the virtual tape
* shelf (VTS).
*
*
* If a specific TapeARN
is not specified, AWS Storage Gateway
* returns a description of all virtual tapes found in the VTS associated
* with your account.
*
*
* @param describeTapeArchivesRequest
* DescribeTapeArchivesInput
* @return A Java Future containing the result of the DescribeTapeArchives
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapeArchives
*/
java.util.concurrent.Future describeTapeArchivesAsync(
DescribeTapeArchivesRequest describeTapeArchivesRequest);
/**
*
* Returns a description of specified virtual tapes in the virtual tape
* shelf (VTS).
*
*
* If a specific TapeARN
is not specified, AWS Storage Gateway
* returns a description of all virtual tapes found in the VTS associated
* with your account.
*
*
* @param describeTapeArchivesRequest
* DescribeTapeArchivesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTapeArchives
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapeArchives
*/
java.util.concurrent.Future describeTapeArchivesAsync(
DescribeTapeArchivesRequest describeTapeArchivesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeTapeArchives operation.
*
* @see #describeTapeArchivesAsync(DescribeTapeArchivesRequest)
*/
java.util.concurrent.Future describeTapeArchivesAsync();
/**
* Simplified method form for invoking the DescribeTapeArchives operation
* with an AsyncHandler.
*
* @see #describeTapeArchivesAsync(DescribeTapeArchivesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeTapeArchivesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of virtual tape recovery points that are available for the
* specified gateway-VTL.
*
*
* A recovery point is a point-in-time view of a virtual tape at which all
* the data on the virtual tape is consistent. If your gateway crashes,
* virtual tapes that have recovery points can be recovered to a new
* gateway.
*
*
* @param describeTapeRecoveryPointsRequest
* DescribeTapeRecoveryPointsInput
* @return A Java Future containing the result of the
* DescribeTapeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapeRecoveryPoints
*/
java.util.concurrent.Future describeTapeRecoveryPointsAsync(
DescribeTapeRecoveryPointsRequest describeTapeRecoveryPointsRequest);
/**
*
* Returns a list of virtual tape recovery points that are available for the
* specified gateway-VTL.
*
*
* A recovery point is a point-in-time view of a virtual tape at which all
* the data on the virtual tape is consistent. If your gateway crashes,
* virtual tapes that have recovery points can be recovered to a new
* gateway.
*
*
* @param describeTapeRecoveryPointsRequest
* DescribeTapeRecoveryPointsInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTapeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapeRecoveryPoints
*/
java.util.concurrent.Future describeTapeRecoveryPointsAsync(
DescribeTapeRecoveryPointsRequest describeTapeRecoveryPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the specified Amazon Resource Name (ARN) of
* virtual tapes. If a TapeARN
is not specified, returns a
* description of all virtual tapes associated with the specified gateway.
*
*
* @param describeTapesRequest
* DescribeTapesInput
* @return A Java Future containing the result of the DescribeTapes
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapes
*/
java.util.concurrent.Future describeTapesAsync(
DescribeTapesRequest describeTapesRequest);
/**
*
* Returns a description of the specified Amazon Resource Name (ARN) of
* virtual tapes. If a TapeARN
is not specified, returns a
* description of all virtual tapes associated with the specified gateway.
*
*
* @param describeTapesRequest
* DescribeTapesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTapes
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapes
*/
java.util.concurrent.Future describeTapesAsync(
DescribeTapesRequest describeTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the upload buffer of a gateway. This operation
* is supported for both the gateway-stored and gateway-cached volume
* architectures.
*
*
* The response includes disk IDs that are configured as upload buffer
* space, and it includes the amount of upload buffer space allocated and
* used.
*
*
* @param describeUploadBufferRequest
* @return A Java Future containing the result of the DescribeUploadBuffer
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeUploadBuffer
*/
java.util.concurrent.Future describeUploadBufferAsync(
DescribeUploadBufferRequest describeUploadBufferRequest);
/**
*
* Returns information about the upload buffer of a gateway. This operation
* is supported for both the gateway-stored and gateway-cached volume
* architectures.
*
*
* The response includes disk IDs that are configured as upload buffer
* space, and it includes the amount of upload buffer space allocated and
* used.
*
*
* @param describeUploadBufferRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUploadBuffer
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeUploadBuffer
*/
java.util.concurrent.Future describeUploadBufferAsync(
DescribeUploadBufferRequest describeUploadBufferRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of virtual tape library (VTL) devices for the
* specified gateway. In the response, AWS Storage Gateway returns VTL
* device information.
*
*
* The list of VTL devices must be from one gateway.
*
*
* @param describeVTLDevicesRequest
* DescribeVTLDevicesInput
* @return A Java Future containing the result of the DescribeVTLDevices
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeVTLDevices
*/
java.util.concurrent.Future describeVTLDevicesAsync(
DescribeVTLDevicesRequest describeVTLDevicesRequest);
/**
*
* Returns a description of virtual tape library (VTL) devices for the
* specified gateway. In the response, AWS Storage Gateway returns VTL
* device information.
*
*
* The list of VTL devices must be from one gateway.
*
*
* @param describeVTLDevicesRequest
* DescribeVTLDevicesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVTLDevices
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeVTLDevices
*/
java.util.concurrent.Future describeVTLDevicesAsync(
DescribeVTLDevicesRequest describeVTLDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the working storage of a gateway. This
* operation is supported only for the gateway-stored volume architecture.
* This operation is deprecated in cached-volumes API version (20120630).
* Use DescribeUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use
* the DescribeUploadBuffer operation to add upload buffer to a
* stored-volume gateway.
*
*
*
* The response includes disk IDs that are configured as working storage,
* and it includes the amount of working storage allocated and used.
*
*
* @param describeWorkingStorageRequest
* A JSON object containing the of the gateway.
* @return A Java Future containing the result of the DescribeWorkingStorage
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeWorkingStorage
*/
java.util.concurrent.Future describeWorkingStorageAsync(
DescribeWorkingStorageRequest describeWorkingStorageRequest);
/**
*
* Returns information about the working storage of a gateway. This
* operation is supported only for the gateway-stored volume architecture.
* This operation is deprecated in cached-volumes API version (20120630).
* Use DescribeUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use
* the DescribeUploadBuffer operation to add upload buffer to a
* stored-volume gateway.
*
*
*
* The response includes disk IDs that are configured as working storage,
* and it includes the amount of working storage allocated and used.
*
*
* @param describeWorkingStorageRequest
* A JSON object containing the of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkingStorage
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeWorkingStorage
*/
java.util.concurrent.Future describeWorkingStorageAsync(
DescribeWorkingStorageRequest describeWorkingStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables a gateway when the gateway is no longer functioning. For
* example, if your gateway VM is damaged, you can disable the gateway so
* you can recover virtual tapes.
*
*
* Use this operation for a gateway-VTL that is not reachable or not
* functioning.
*
*
*
* Once a gateway is disabled it cannot be enabled.
*
*
*
* @param disableGatewayRequest
* DisableGatewayInput
* @return A Java Future containing the result of the DisableGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsync.DisableGateway
*/
java.util.concurrent.Future disableGatewayAsync(
DisableGatewayRequest disableGatewayRequest);
/**
*
* Disables a gateway when the gateway is no longer functioning. For
* example, if your gateway VM is damaged, you can disable the gateway so
* you can recover virtual tapes.
*
*
* Use this operation for a gateway-VTL that is not reachable or not
* functioning.
*
*
*
* Once a gateway is disabled it cannot be enabled.
*
*
*
* @param disableGatewayRequest
* DisableGatewayInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DisableGateway
*/
java.util.concurrent.Future disableGatewayAsync(
DisableGatewayRequest disableGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists gateways owned by an AWS account in a region specified in the
* request. The returned list is ordered by gateway Amazon Resource Name
* (ARN).
*
*
* By default, the operation returns a maximum of 100 gateways. This
* operation supports pagination that allows you to optionally reduce the
* number of gateways returned in a response.
*
*
* If you have more gateways than are returned in a response (that is, the
* response returns only a truncated list of your gateways), the response
* contains a marker that you can specify in your next request to fetch the
* next page of gateways.
*
*
* @param listGatewaysRequest
* A JSON object containing zero or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListGateways operation
* returned by the service.
* @sample AWSStorageGatewayAsync.ListGateways
*/
java.util.concurrent.Future listGatewaysAsync(
ListGatewaysRequest listGatewaysRequest);
/**
*
* Lists gateways owned by an AWS account in a region specified in the
* request. The returned list is ordered by gateway Amazon Resource Name
* (ARN).
*
*
* By default, the operation returns a maximum of 100 gateways. This
* operation supports pagination that allows you to optionally reduce the
* number of gateways returned in a response.
*
*
* If you have more gateways than are returned in a response (that is, the
* response returns only a truncated list of your gateways), the response
* contains a marker that you can specify in your next request to fetch the
* next page of gateways.
*
*
* @param listGatewaysRequest
* A JSON object containing zero or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGateways operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListGateways
*/
java.util.concurrent.Future listGatewaysAsync(
ListGatewaysRequest listGatewaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListGateways operation.
*
* @see #listGatewaysAsync(ListGatewaysRequest)
*/
java.util.concurrent.Future listGatewaysAsync();
/**
* Simplified method form for invoking the ListGateways operation with an
* AsyncHandler.
*
* @see #listGatewaysAsync(ListGatewaysRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listGatewaysAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the gateway's local disks. To specify which gateway to
* describe, you use the Amazon Resource Name (ARN) of the gateway in the
* body of the request.
*
*
* The request returns a list of all disks, specifying which are configured
* as working storage, cache storage, or stored volume or not configured at
* all. The response includes a DiskStatus
field. This field
* can have a value of present (the disk is available to use), missing (the
* disk is no longer connected to the gateway), or mismatch (the disk node
* is occupied by a disk that has incorrect metadata or the disk content is
* corrupted).
*
*
* @param listLocalDisksRequest
* A JSON object containing the of the gateway.
* @return A Java Future containing the result of the ListLocalDisks
* operation returned by the service.
* @sample AWSStorageGatewayAsync.ListLocalDisks
*/
java.util.concurrent.Future listLocalDisksAsync(
ListLocalDisksRequest listLocalDisksRequest);
/**
*
* Returns a list of the gateway's local disks. To specify which gateway to
* describe, you use the Amazon Resource Name (ARN) of the gateway in the
* body of the request.
*
*
* The request returns a list of all disks, specifying which are configured
* as working storage, cache storage, or stored volume or not configured at
* all. The response includes a DiskStatus
field. This field
* can have a value of present (the disk is available to use), missing (the
* disk is no longer connected to the gateway), or mismatch (the disk node
* is occupied by a disk that has incorrect metadata or the disk content is
* corrupted).
*
*
* @param listLocalDisksRequest
* A JSON object containing the of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLocalDisks
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListLocalDisks
*/
java.util.concurrent.Future listLocalDisksAsync(
ListLocalDisksRequest listLocalDisksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags that have been added to the specified resource.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceInput
* @return A Java Future containing the result of the ListTagsForResource
* operation returned by the service.
* @sample AWSStorageGatewayAsync.ListTagsForResource
*/
java.util.concurrent.Future listTagsForResourceAsync(
ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags that have been added to the specified resource.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListTagsForResource
*/
java.util.concurrent.Future listTagsForResourceAsync(
ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListTagsForResource operation.
*
* @see #listTagsForResourceAsync(ListTagsForResourceRequest)
*/
java.util.concurrent.Future listTagsForResourceAsync();
/**
* Simplified method form for invoking the ListTagsForResource operation
* with an AsyncHandler.
*
* @see #listTagsForResourceAsync(ListTagsForResourceRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listTagsForResourceAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists virtual tapes in your virtual tape library (VTL) and your virtual
* tape shelf (VTS). You specify the tapes to list by specifying one or more
* tape Amazon Resource Names (ARNs). If you don't specify a tape ARN, the
* operation lists all virtual tapes in both your VTL and VTS.
*
*
* This operation supports pagination. By default, the operation returns a
* maximum of up to 100 tapes. You can optionally specify the
* Limit
parameter in the body to limit the number of tapes in
* the response. If the number of tapes returned in the response is
* truncated, the response includes a Marker
element that you
* can use in your subsequent request to retrieve the next set of tapes.
*
*
* @param listTapesRequest
* A JSON object that contains one or more of the following
* fields:
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListTapes operation
* returned by the service.
* @sample AWSStorageGatewayAsync.ListTapes
*/
java.util.concurrent.Future listTapesAsync(
ListTapesRequest listTapesRequest);
/**
*
* Lists virtual tapes in your virtual tape library (VTL) and your virtual
* tape shelf (VTS). You specify the tapes to list by specifying one or more
* tape Amazon Resource Names (ARNs). If you don't specify a tape ARN, the
* operation lists all virtual tapes in both your VTL and VTS.
*
*
* This operation supports pagination. By default, the operation returns a
* maximum of up to 100 tapes. You can optionally specify the
* Limit
parameter in the body to limit the number of tapes in
* the response. If the number of tapes returned in the response is
* truncated, the response includes a Marker
element that you
* can use in your subsequent request to retrieve the next set of tapes.
*
*
* @param listTapesRequest
* A JSON object that contains one or more of the following
* fields:
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTapes operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListTapes
*/
java.util.concurrent.Future listTapesAsync(
ListTapesRequest listTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists iSCSI initiators that are connected to a volume. You can use this
* operation to determine whether a volume is being used or not.
*
*
* @param listVolumeInitiatorsRequest
* ListVolumeInitiatorsInput
* @return A Java Future containing the result of the ListVolumeInitiators
* operation returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumeInitiators
*/
java.util.concurrent.Future listVolumeInitiatorsAsync(
ListVolumeInitiatorsRequest listVolumeInitiatorsRequest);
/**
*
* Lists iSCSI initiators that are connected to a volume. You can use this
* operation to determine whether a volume is being used or not.
*
*
* @param listVolumeInitiatorsRequest
* ListVolumeInitiatorsInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVolumeInitiators
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumeInitiators
*/
java.util.concurrent.Future listVolumeInitiatorsAsync(
ListVolumeInitiatorsRequest listVolumeInitiatorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the recovery points for a specified gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
* Each gateway-cached volume has one recovery point. A volume recovery
* point is a point in time at which all data of the volume is consistent
* and from which you can create a snapshot. To create a snapshot from a
* volume recovery point use the
* CreateSnapshotFromVolumeRecoveryPoint operation.
*
*
* @param listVolumeRecoveryPointsRequest
* @return A Java Future containing the result of the
* ListVolumeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumeRecoveryPoints
*/
java.util.concurrent.Future listVolumeRecoveryPointsAsync(
ListVolumeRecoveryPointsRequest listVolumeRecoveryPointsRequest);
/**
*
* Lists the recovery points for a specified gateway. This operation is
* supported only for the gateway-cached volume architecture.
*
*
* Each gateway-cached volume has one recovery point. A volume recovery
* point is a point in time at which all data of the volume is consistent
* and from which you can create a snapshot. To create a snapshot from a
* volume recovery point use the
* CreateSnapshotFromVolumeRecoveryPoint operation.
*
*
* @param listVolumeRecoveryPointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListVolumeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumeRecoveryPoints
*/
java.util.concurrent.Future listVolumeRecoveryPointsAsync(
ListVolumeRecoveryPointsRequest listVolumeRecoveryPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the iSCSI stored volumes of a gateway. Results are sorted by volume
* ARN. The response includes only the volume ARNs. If you want additional
* volume information, use the DescribeStorediSCSIVolumes API.
*
*
* The operation supports pagination. By default, the operation returns a
* maximum of up to 100 volumes. You can optionally specify the
* Limit
field in the body to limit the number of volumes in
* the response. If the number of volumes returned in the response is
* truncated, the response includes a Marker field. You can use this Marker
* value in your subsequent request to retrieve the next set of volumes.
*
*
* @param listVolumesRequest
* A JSON object that contains one or more of the following
* fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListVolumes operation
* returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumes
*/
java.util.concurrent.Future listVolumesAsync(
ListVolumesRequest listVolumesRequest);
/**
*
* Lists the iSCSI stored volumes of a gateway. Results are sorted by volume
* ARN. The response includes only the volume ARNs. If you want additional
* volume information, use the DescribeStorediSCSIVolumes API.
*
*
* The operation supports pagination. By default, the operation returns a
* maximum of up to 100 volumes. You can optionally specify the
* Limit
field in the body to limit the number of volumes in
* the response. If the number of volumes returned in the response is
* truncated, the response includes a Marker field. You can use this Marker
* value in your subsequent request to retrieve the next set of volumes.
*
*
* @param listVolumesRequest
* A JSON object that contains one or more of the following
* fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVolumes operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumes
*/
java.util.concurrent.Future listVolumesAsync(
ListVolumesRequest listVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param removeTagsFromResourceRequest
* RemoveTagsFromResourceInput
* @return A Java Future containing the result of the RemoveTagsFromResource
* operation returned by the service.
* @sample AWSStorageGatewayAsync.RemoveTagsFromResource
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Removes one or more tags from the specified resource.
*
*
* @param removeTagsFromResourceRequest
* RemoveTagsFromResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RemoveTagsFromResource
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the RemoveTagsFromResource operation.
*
* @see #removeTagsFromResourceAsync(RemoveTagsFromResourceRequest)
*/
java.util.concurrent.Future removeTagsFromResourceAsync();
/**
* Simplified method form for invoking the RemoveTagsFromResource operation
* with an AsyncHandler.
*
* @see #removeTagsFromResourceAsync(RemoveTagsFromResourceRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resets all cache disks that have encountered a error and makes the disks
* available for reconfiguration as cache storage. If your cache disk
* encounters a error, the gateway prevents read and write operations on
* virtual tapes in the gateway. For example, an error can occur when a disk
* is corrupted or removed from the gateway. When a cache is reset, the
* gateway loses its cache storage. At this point you can reconfigure the
* disks as cache disks.
*
*
*
* If the cache disk you are resetting contains data that has not been
* uploaded to Amazon S3 yet, that data can be lost. After you reset cache
* disks, there will be no configured cache disks left in the gateway, so
* you must configure at least one new cache disk for your gateway to
* function properly.
*
*
*
* @param resetCacheRequest
* @return A Java Future containing the result of the ResetCache operation
* returned by the service.
* @sample AWSStorageGatewayAsync.ResetCache
*/
java.util.concurrent.Future resetCacheAsync(
ResetCacheRequest resetCacheRequest);
/**
*
* Resets all cache disks that have encountered a error and makes the disks
* available for reconfiguration as cache storage. If your cache disk
* encounters a error, the gateway prevents read and write operations on
* virtual tapes in the gateway. For example, an error can occur when a disk
* is corrupted or removed from the gateway. When a cache is reset, the
* gateway loses its cache storage. At this point you can reconfigure the
* disks as cache disks.
*
*
*
* If the cache disk you are resetting contains data that has not been
* uploaded to Amazon S3 yet, that data can be lost. After you reset cache
* disks, there will be no configured cache disks left in the gateway, so
* you must configure at least one new cache disk for your gateway to
* function properly.
*
*
*
* @param resetCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetCache operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ResetCache
*/
java.util.concurrent.Future resetCacheAsync(
ResetCacheRequest resetCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves an archived virtual tape from the virtual tape shelf (VTS) to a
* gateway-VTL. Virtual tapes archived in the VTS are not associated with
* any gateway. However after a tape is retrieved, it is associated with a
* gateway, even though it is also listed in the VTS.
*
*
* Once a tape is successfully retrieved to a gateway, it cannot be
* retrieved again to another gateway. You must archive the tape again
* before you can retrieve it to another gateway.
*
*
* @param retrieveTapeArchiveRequest
* RetrieveTapeArchiveInput
* @return A Java Future containing the result of the RetrieveTapeArchive
* operation returned by the service.
* @sample AWSStorageGatewayAsync.RetrieveTapeArchive
*/
java.util.concurrent.Future retrieveTapeArchiveAsync(
RetrieveTapeArchiveRequest retrieveTapeArchiveRequest);
/**
*
* Retrieves an archived virtual tape from the virtual tape shelf (VTS) to a
* gateway-VTL. Virtual tapes archived in the VTS are not associated with
* any gateway. However after a tape is retrieved, it is associated with a
* gateway, even though it is also listed in the VTS.
*
*
* Once a tape is successfully retrieved to a gateway, it cannot be
* retrieved again to another gateway. You must archive the tape again
* before you can retrieve it to another gateway.
*
*
* @param retrieveTapeArchiveRequest
* RetrieveTapeArchiveInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RetrieveTapeArchive
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RetrieveTapeArchive
*/
java.util.concurrent.Future retrieveTapeArchiveAsync(
RetrieveTapeArchiveRequest retrieveTapeArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the recovery point for the specified virtual tape.
*
*
* A recovery point is a point in time view of a virtual tape at which all
* the data on the tape is consistent. If your gateway crashes, virtual
* tapes that have recovery points can be recovered to a new gateway.
*
*
*
* The virtual tape can be retrieved to only one gateway. The retrieved tape
* is read-only. The virtual tape can be retrieved to only a gateway-VTL.
* There is no charge for retrieving recovery points.
*
*
*
* @param retrieveTapeRecoveryPointRequest
* RetrieveTapeRecoveryPointInput
* @return A Java Future containing the result of the
* RetrieveTapeRecoveryPoint operation returned by the service.
* @sample AWSStorageGatewayAsync.RetrieveTapeRecoveryPoint
*/
java.util.concurrent.Future retrieveTapeRecoveryPointAsync(
RetrieveTapeRecoveryPointRequest retrieveTapeRecoveryPointRequest);
/**
*
* Retrieves the recovery point for the specified virtual tape.
*
*
* A recovery point is a point in time view of a virtual tape at which all
* the data on the tape is consistent. If your gateway crashes, virtual
* tapes that have recovery points can be recovered to a new gateway.
*
*
*
* The virtual tape can be retrieved to only one gateway. The retrieved tape
* is read-only. The virtual tape can be retrieved to only a gateway-VTL.
* There is no charge for retrieving recovery points.
*
*
*
* @param retrieveTapeRecoveryPointRequest
* RetrieveTapeRecoveryPointInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RetrieveTapeRecoveryPoint operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RetrieveTapeRecoveryPoint
*/
java.util.concurrent.Future retrieveTapeRecoveryPointAsync(
RetrieveTapeRecoveryPointRequest retrieveTapeRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the password for your VM local console. When you log in to the local
* console for the first time, you log in to the VM with the default
* credentials. We recommend that you set a new password. You don't need to
* know the default password to set a new password.
*
*
* @param setLocalConsolePasswordRequest
* SetLocalConsolePasswordInput
* @return A Java Future containing the result of the
* SetLocalConsolePassword operation returned by the service.
* @sample AWSStorageGatewayAsync.SetLocalConsolePassword
*/
java.util.concurrent.Future setLocalConsolePasswordAsync(
SetLocalConsolePasswordRequest setLocalConsolePasswordRequest);
/**
*
* Sets the password for your VM local console. When you log in to the local
* console for the first time, you log in to the VM with the default
* credentials. We recommend that you set a new password. You don't need to
* know the default password to set a new password.
*
*
* @param setLocalConsolePasswordRequest
* SetLocalConsolePasswordInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SetLocalConsolePassword operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.SetLocalConsolePassword
*/
java.util.concurrent.Future setLocalConsolePasswordAsync(
SetLocalConsolePasswordRequest setLocalConsolePasswordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shuts down a gateway. To specify which gateway to shut down, use the
* Amazon Resource Name (ARN) of the gateway in the body of your request.
*
*
* The operation shuts down the gateway service component running in the
* storage gateway's virtual machine (VM) and not the VM.
*
*
*
* If you want to shut down the VM, it is recommended that you first shut
* down the gateway component in the VM to avoid unpredictable conditions.
*
*
*
* After the gateway is shutdown, you cannot call any other API except
* StartGateway, DescribeGatewayInformation, and
* ListGateways. For more information, see ActivateGateway.
* Your applications cannot read from or write to the gateway's storage
* volumes, and there are no snapshots taken.
*
*
*
* When you make a shutdown request, you will get a 200 OK
* success response immediately. However, it might take some time for the
* gateway to shut down. You can call the DescribeGatewayInformation
* API to check the status. For more information, see
* ActivateGateway.
*
*
*
* If do not intend to use the gateway again, you must delete the gateway
* (using DeleteGateway) to no longer pay software charges associated
* with the gateway.
*
*
* @param shutdownGatewayRequest
* A JSON object containing the of the gateway to shut down.
* @return A Java Future containing the result of the ShutdownGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsync.ShutdownGateway
*/
java.util.concurrent.Future shutdownGatewayAsync(
ShutdownGatewayRequest shutdownGatewayRequest);
/**
*
* Shuts down a gateway. To specify which gateway to shut down, use the
* Amazon Resource Name (ARN) of the gateway in the body of your request.
*
*
* The operation shuts down the gateway service component running in the
* storage gateway's virtual machine (VM) and not the VM.
*
*
*
* If you want to shut down the VM, it is recommended that you first shut
* down the gateway component in the VM to avoid unpredictable conditions.
*
*
*
* After the gateway is shutdown, you cannot call any other API except
* StartGateway, DescribeGatewayInformation, and
* ListGateways. For more information, see ActivateGateway.
* Your applications cannot read from or write to the gateway's storage
* volumes, and there are no snapshots taken.
*
*
*
* When you make a shutdown request, you will get a 200 OK
* success response immediately. However, it might take some time for the
* gateway to shut down. You can call the DescribeGatewayInformation
* API to check the status. For more information, see
* ActivateGateway.
*
*
*
* If do not intend to use the gateway again, you must delete the gateway
* (using DeleteGateway) to no longer pay software charges associated
* with the gateway.
*
*
* @param shutdownGatewayRequest
* A JSON object containing the of the gateway to shut down.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ShutdownGateway
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ShutdownGateway
*/
java.util.concurrent.Future shutdownGatewayAsync(
ShutdownGatewayRequest shutdownGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a gateway that you previously shut down (see
* ShutdownGateway). After the gateway starts, you can then make
* other API calls, your applications can read from or write to the
* gateway's storage volumes and you will be able to take snapshot backups.
*
*
*
* When you make a request, you will get a 200 OK success response
* immediately. However, it might take some time for the gateway to be
* ready. You should call DescribeGatewayInformation and check the
* status before making any additional API calls. For more information, see
* ActivateGateway.
*
*
*
* To specify which gateway to start, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param startGatewayRequest
* A JSON object containing the of the gateway to start.
* @return A Java Future containing the result of the StartGateway operation
* returned by the service.
* @sample AWSStorageGatewayAsync.StartGateway
*/
java.util.concurrent.Future startGatewayAsync(
StartGatewayRequest startGatewayRequest);
/**
*
* Starts a gateway that you previously shut down (see
* ShutdownGateway). After the gateway starts, you can then make
* other API calls, your applications can read from or write to the
* gateway's storage volumes and you will be able to take snapshot backups.
*
*
*
* When you make a request, you will get a 200 OK success response
* immediately. However, it might take some time for the gateway to be
* ready. You should call DescribeGatewayInformation and check the
* status before making any additional API calls. For more information, see
* ActivateGateway.
*
*
*
* To specify which gateway to start, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param startGatewayRequest
* A JSON object containing the of the gateway to start.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartGateway operation
* returned by the service.
* @sample AWSStorageGatewayAsyncHandler.StartGateway
*/
java.util.concurrent.Future startGatewayAsync(
StartGatewayRequest startGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the bandwidth rate limits of a gateway. You can update both the
* upload and download bandwidth rate limit or specify only one of the two.
* If you don't set a bandwidth rate limit, the existing rate limit remains.
*
*
* By default, a gateway's bandwidth rate limits are not set. If you don't
* set any limit, the gateway does not have any limitations on its bandwidth
* usage and could potentially use the maximum available bandwidth.
*
*
* To specify which gateway to update, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param updateBandwidthRateLimitRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* UpdateBandwidthRateLimitInput$AverageDownloadRateLimitInBitsPerSec
*
*
*
* -
*
*
* UpdateBandwidthRateLimitInput$AverageUploadRateLimitInBitsPerSec
*
*
*
* @return A Java Future containing the result of the
* UpdateBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateBandwidthRateLimit
*/
java.util.concurrent.Future updateBandwidthRateLimitAsync(
UpdateBandwidthRateLimitRequest updateBandwidthRateLimitRequest);
/**
*
* Updates the bandwidth rate limits of a gateway. You can update both the
* upload and download bandwidth rate limit or specify only one of the two.
* If you don't set a bandwidth rate limit, the existing rate limit remains.
*
*
* By default, a gateway's bandwidth rate limits are not set. If you don't
* set any limit, the gateway does not have any limitations on its bandwidth
* usage and could potentially use the maximum available bandwidth.
*
*
* To specify which gateway to update, use the Amazon Resource Name (ARN) of
* the gateway in your request.
*
*
* @param updateBandwidthRateLimitRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* UpdateBandwidthRateLimitInput$AverageDownloadRateLimitInBitsPerSec
*
*
*
* -
*
*
* UpdateBandwidthRateLimitInput$AverageUploadRateLimitInBitsPerSec
*
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateBandwidthRateLimit
*/
java.util.concurrent.Future updateBandwidthRateLimitAsync(
UpdateBandwidthRateLimitRequest updateBandwidthRateLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Challenge-Handshake Authentication Protocol (CHAP)
* credentials for a specified iSCSI target. By default, a gateway does not
* have CHAP enabled; however, for added security, you might use it.
*
*
*
* When you update CHAP credentials, all existing connections on the target
* are closed and initiators must reconnect with the new credentials.
*
*
*
* @param updateChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
* UpdateChapCredentialsInput$SecretToAuthenticateInitiator
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the UpdateChapCredentials
* operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateChapCredentials
*/
java.util.concurrent.Future updateChapCredentialsAsync(
UpdateChapCredentialsRequest updateChapCredentialsRequest);
/**
*
* Updates the Challenge-Handshake Authentication Protocol (CHAP)
* credentials for a specified iSCSI target. By default, a gateway does not
* have CHAP enabled; however, for added security, you might use it.
*
*
*
* When you update CHAP credentials, all existing connections on the target
* are closed and initiators must reconnect with the new credentials.
*
*
*
* @param updateChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
* UpdateChapCredentialsInput$SecretToAuthenticateInitiator
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateChapCredentials
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateChapCredentials
*/
java.util.concurrent.Future updateChapCredentialsAsync(
UpdateChapCredentialsRequest updateChapCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a gateway's metadata, which includes the gateway's name and time
* zone. To specify which gateway to update, use the Amazon Resource Name
* (ARN) of the gateway in your request.
*
*
*
* For Gateways activated after September 2, 2015, the gateway's ARN
* contains the gateway ID rather than the gateway name. However, changing
* the name of the gateway has no effect on the gateway's ARN.
*
*
*
* @param updateGatewayInformationRequest
* @return A Java Future containing the result of the
* UpdateGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateGatewayInformation
*/
java.util.concurrent.Future updateGatewayInformationAsync(
UpdateGatewayInformationRequest updateGatewayInformationRequest);
/**
*
* Updates a gateway's metadata, which includes the gateway's name and time
* zone. To specify which gateway to update, use the Amazon Resource Name
* (ARN) of the gateway in your request.
*
*
*
* For Gateways activated after September 2, 2015, the gateway's ARN
* contains the gateway ID rather than the gateway name. However, changing
* the name of the gateway has no effect on the gateway's ARN.
*
*
*
* @param updateGatewayInformationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateGatewayInformation
*/
java.util.concurrent.Future updateGatewayInformationAsync(
UpdateGatewayInformationRequest updateGatewayInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the gateway virtual machine (VM) software. The request
* immediately triggers the software update.
*
*
*
* When you make this request, you get a 200 OK
success
* response immediately. However, it might take some time for the update to
* complete. You can call DescribeGatewayInformation to verify the
* gateway is in the STATE_RUNNING
state.
*
*
*
* A software update forces a system restart of your gateway. You can
* minimize the chance of any disruption to your applications by increasing
* your iSCSI Initiators' timeouts. For more information about increasing
* iSCSI Initiator timeouts for Windows and Linux, see Customizing Your Windows iSCSI Settings and Customizing Your Linux iSCSI Settings, respectively.
*
*
*
* @param updateGatewaySoftwareNowRequest
* A JSON object containing the of the gateway to update.
* @return A Java Future containing the result of the
* UpdateGatewaySoftwareNow operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateGatewaySoftwareNow
*/
java.util.concurrent.Future updateGatewaySoftwareNowAsync(
UpdateGatewaySoftwareNowRequest updateGatewaySoftwareNowRequest);
/**
*
* Updates the gateway virtual machine (VM) software. The request
* immediately triggers the software update.
*
*
*
* When you make this request, you get a 200 OK
success
* response immediately. However, it might take some time for the update to
* complete. You can call DescribeGatewayInformation to verify the
* gateway is in the STATE_RUNNING
state.
*
*
*
* A software update forces a system restart of your gateway. You can
* minimize the chance of any disruption to your applications by increasing
* your iSCSI Initiators' timeouts. For more information about increasing
* iSCSI Initiator timeouts for Windows and Linux, see Customizing Your Windows iSCSI Settings and Customizing Your Linux iSCSI Settings, respectively.
*
*
*
* @param updateGatewaySoftwareNowRequest
* A JSON object containing the of the gateway to update.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateGatewaySoftwareNow operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateGatewaySoftwareNow
*/
java.util.concurrent.Future updateGatewaySoftwareNowAsync(
UpdateGatewaySoftwareNowRequest updateGatewaySoftwareNowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a gateway's weekly maintenance start time information, including
* day and time of the week. The maintenance time is the time in your
* gateway's time zone.
*
*
* @param updateMaintenanceStartTimeRequest
* A JSON object containing the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the
* UpdateMaintenanceStartTime operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateMaintenanceStartTime
*/
java.util.concurrent.Future updateMaintenanceStartTimeAsync(
UpdateMaintenanceStartTimeRequest updateMaintenanceStartTimeRequest);
/**
*
* Updates a gateway's weekly maintenance start time information, including
* day and time of the week. The maintenance time is the time in your
* gateway's time zone.
*
*
* @param updateMaintenanceStartTimeRequest
* A JSON object containing the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* UpdateMaintenanceStartTime operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateMaintenanceStartTime
*/
java.util.concurrent.Future updateMaintenanceStartTimeAsync(
UpdateMaintenanceStartTimeRequest updateMaintenanceStartTimeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a snapshot schedule configured for a gateway volume.
*
*
* The default snapshot schedule for volume is once every 24 hours, starting
* at the creation time of the volume. You can use this API to change the
* snapshot schedule configured for the volume.
*
*
* In the request you must identify the gateway volume whose snapshot
* schedule you want to update, and the schedule information, including when
* you want the snapshot to begin on a day and the frequency (in hours) of
* snapshots.
*
*
* @param updateSnapshotScheduleRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the UpdateSnapshotSchedule
* operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateSnapshotSchedule
*/
java.util.concurrent.Future updateSnapshotScheduleAsync(
UpdateSnapshotScheduleRequest updateSnapshotScheduleRequest);
/**
*
* Updates a snapshot schedule configured for a gateway volume.
*
*
* The default snapshot schedule for volume is once every 24 hours, starting
* at the creation time of the volume. You can use this API to change the
* snapshot schedule configured for the volume.
*
*
* In the request you must identify the gateway volume whose snapshot
* schedule you want to update, and the schedule information, including when
* you want the snapshot to begin on a day and the frequency (in hours) of
* snapshots.
*
*
* @param updateSnapshotScheduleRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSnapshotSchedule
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateSnapshotSchedule
*/
java.util.concurrent.Future updateSnapshotScheduleAsync(
UpdateSnapshotScheduleRequest updateSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the type of medium changer in a gateway-VTL. When you activate a
* gateway-VTL, you select a medium changer type for the gateway-VTL. This
* operation enables you to select a different type of medium changer after
* a gateway-VTL is activated.
*
*
* @param updateVTLDeviceTypeRequest
* @return A Java Future containing the result of the UpdateVTLDeviceType
* operation returned by the service.
* @sample AWSStorageGatewayAsync.UpdateVTLDeviceType
*/
java.util.concurrent.Future updateVTLDeviceTypeAsync(
UpdateVTLDeviceTypeRequest updateVTLDeviceTypeRequest);
/**
*
* Updates the type of medium changer in a gateway-VTL. When you activate a
* gateway-VTL, you select a medium changer type for the gateway-VTL. This
* operation enables you to select a different type of medium changer after
* a gateway-VTL is activated.
*
*
* @param updateVTLDeviceTypeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVTLDeviceType
* operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.UpdateVTLDeviceType
*/
java.util.concurrent.Future updateVTLDeviceTypeAsync(
UpdateVTLDeviceTypeRequest updateVTLDeviceTypeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}