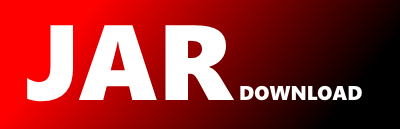
com.amazonaws.services.storagegateway.model.UpdateSMBFileShareRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.storagegateway.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* UpdateSMBFileShareInput
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateSMBFileShareRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*
*/
private String fileShareARN;
/**
*
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by Amazon
* S3. Optional.
*
*/
private Boolean kMSEncrypted;
/**
*
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value can only
* be set when KMSEncrypted is true. Optional.
*
*/
private String kMSKey;
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values are
* S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is not
* populated, the default value S3_STANDARD
is used. Optional.
*
*/
private String defaultStorageClass;
/**
*
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
*
*/
private String objectACL;
/**
*
* A value that sets the write status of a file share. This value is true if the write status is read-only, and
* otherwise false.
*
*/
private Boolean readOnly;
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true to enable MIME type guessing, and otherwise to false. The default value is true.
*
*/
private Boolean guessMIMETypeEnabled;
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays. However, the S3
* bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*/
private Boolean requesterPays;
/**
*
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to map file
* and directory permissions to the POSIX permissions.
*
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the Storage
* Gateway User Guide.
*
*/
private Boolean sMBACLEnabled;
/**
*
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList adminUserList;
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList validUserList;
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList invalidUserList;
/**
*
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*
*
* @param fileShareARN
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*/
public void setFileShareARN(String fileShareARN) {
this.fileShareARN = fileShareARN;
}
/**
*
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*
*
* @return The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*/
public String getFileShareARN() {
return this.fileShareARN;
}
/**
*
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
*
*
* @param fileShareARN
* The Amazon Resource Name (ARN) of the SMB file share that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withFileShareARN(String fileShareARN) {
setFileShareARN(fileShareARN);
return this;
}
/**
*
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by Amazon
* S3. Optional.
*
*
* @param kMSEncrypted
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by
* Amazon S3. Optional.
*/
public void setKMSEncrypted(Boolean kMSEncrypted) {
this.kMSEncrypted = kMSEncrypted;
}
/**
*
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by Amazon
* S3. Optional.
*
*
* @return True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by
* Amazon S3. Optional.
*/
public Boolean getKMSEncrypted() {
return this.kMSEncrypted;
}
/**
*
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by Amazon
* S3. Optional.
*
*
* @param kMSEncrypted
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by
* Amazon S3. Optional.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withKMSEncrypted(Boolean kMSEncrypted) {
setKMSEncrypted(kMSEncrypted);
return this;
}
/**
*
* True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by Amazon
* S3. Optional.
*
*
* @return True to use Amazon S3 server side encryption with your own AWS KMS key, or false to use a key managed by
* Amazon S3. Optional.
*/
public Boolean isKMSEncrypted() {
return this.kMSEncrypted;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value can only
* be set when KMSEncrypted is true. Optional.
*
*
* @param kMSKey
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value
* can only be set when KMSEncrypted is true. Optional.
*/
public void setKMSKey(String kMSKey) {
this.kMSKey = kMSKey;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value can only
* be set when KMSEncrypted is true. Optional.
*
*
* @return The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value
* can only be set when KMSEncrypted is true. Optional.
*/
public String getKMSKey() {
return this.kMSKey;
}
/**
*
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value can only
* be set when KMSEncrypted is true. Optional.
*
*
* @param kMSKey
* The Amazon Resource Name (ARN) of the AWS KMS key used for Amazon S3 server side encryption. This value
* can only be set when KMSEncrypted is true. Optional.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withKMSKey(String kMSKey) {
setKMSKey(kMSKey);
return this;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values are
* S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is not
* populated, the default value S3_STANDARD
is used. Optional.
*
*
* @param defaultStorageClass
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values
* are S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is
* not populated, the default value S3_STANDARD
is used. Optional.
*/
public void setDefaultStorageClass(String defaultStorageClass) {
this.defaultStorageClass = defaultStorageClass;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values are
* S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is not
* populated, the default value S3_STANDARD
is used. Optional.
*
*
* @return The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values
* are S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field
* is not populated, the default value S3_STANDARD
is used. Optional.
*/
public String getDefaultStorageClass() {
return this.defaultStorageClass;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values are
* S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is not
* populated, the default value S3_STANDARD
is used. Optional.
*
*
* @param defaultStorageClass
* The default storage class for objects put into an Amazon S3 bucket by the file gateway. Possible values
* are S3_STANDARD
, S3_STANDARD_IA
, or S3_ONEZONE_IA
. If this field is
* not populated, the default value S3_STANDARD
is used. Optional.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withDefaultStorageClass(String defaultStorageClass) {
setDefaultStorageClass(defaultStorageClass);
return this;
}
/**
*
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
*
*
* @param objectACL
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
* @see ObjectACL
*/
public void setObjectACL(String objectACL) {
this.objectACL = objectACL;
}
/**
*
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
*
*
* @return A value that sets the access control list permission for objects in the S3 bucket that a file gateway
* puts objects into. The default value is "private".
* @see ObjectACL
*/
public String getObjectACL() {
return this.objectACL;
}
/**
*
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
*
*
* @param objectACL
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectACL
*/
public UpdateSMBFileShareRequest withObjectACL(String objectACL) {
setObjectACL(objectACL);
return this;
}
/**
*
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
*
*
* @param objectACL
* A value that sets the access control list permission for objects in the S3 bucket that a file gateway puts
* objects into. The default value is "private".
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectACL
*/
public UpdateSMBFileShareRequest withObjectACL(ObjectACL objectACL) {
this.objectACL = objectACL.toString();
return this;
}
/**
*
* A value that sets the write status of a file share. This value is true if the write status is read-only, and
* otherwise false.
*
*
* @param readOnly
* A value that sets the write status of a file share. This value is true if the write status is read-only,
* and otherwise false.
*/
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
/**
*
* A value that sets the write status of a file share. This value is true if the write status is read-only, and
* otherwise false.
*
*
* @return A value that sets the write status of a file share. This value is true if the write status is read-only,
* and otherwise false.
*/
public Boolean getReadOnly() {
return this.readOnly;
}
/**
*
* A value that sets the write status of a file share. This value is true if the write status is read-only, and
* otherwise false.
*
*
* @param readOnly
* A value that sets the write status of a file share. This value is true if the write status is read-only,
* and otherwise false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withReadOnly(Boolean readOnly) {
setReadOnly(readOnly);
return this;
}
/**
*
* A value that sets the write status of a file share. This value is true if the write status is read-only, and
* otherwise false.
*
*
* @return A value that sets the write status of a file share. This value is true if the write status is read-only,
* and otherwise false.
*/
public Boolean isReadOnly() {
return this.readOnly;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true to enable MIME type guessing, and otherwise to false. The default value is true.
*
*
* @param guessMIMETypeEnabled
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true to enable MIME type guessing, and otherwise to false. The default value is true.
*/
public void setGuessMIMETypeEnabled(Boolean guessMIMETypeEnabled) {
this.guessMIMETypeEnabled = guessMIMETypeEnabled;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true to enable MIME type guessing, and otherwise to false. The default value is true.
*
*
* @return A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true to enable MIME type guessing, and otherwise to false. The default value is true.
*/
public Boolean getGuessMIMETypeEnabled() {
return this.guessMIMETypeEnabled;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true to enable MIME type guessing, and otherwise to false. The default value is true.
*
*
* @param guessMIMETypeEnabled
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true to enable MIME type guessing, and otherwise to false. The default value is true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withGuessMIMETypeEnabled(Boolean guessMIMETypeEnabled) {
setGuessMIMETypeEnabled(guessMIMETypeEnabled);
return this;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true to enable MIME type guessing, and otherwise to false. The default value is true.
*
*
* @return A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true to enable MIME type guessing, and otherwise to false. The default value is true.
*/
public Boolean isGuessMIMETypeEnabled() {
return this.guessMIMETypeEnabled;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays. However, the S3
* bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* @param requesterPays
* A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*/
public void setRequesterPays(Boolean requesterPays) {
this.requesterPays = requesterPays;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays. However, the S3
* bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* @return A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*/
public Boolean getRequesterPays() {
return this.requesterPays;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays. However, the S3
* bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* @param requesterPays
* A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withRequesterPays(Boolean requesterPays) {
setRequesterPays(requesterPays);
return this;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays. However, the S3
* bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* @return A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true, the requester pays the costs. Otherwise the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*/
public Boolean isRequesterPays() {
return this.requesterPays;
}
/**
*
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to map file
* and directory permissions to the POSIX permissions.
*
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the Storage
* Gateway User Guide.
*
*
* @param sMBACLEnabled
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to
* map file and directory permissions to the POSIX permissions.
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the
* Storage Gateway User Guide.
*/
public void setSMBACLEnabled(Boolean sMBACLEnabled) {
this.sMBACLEnabled = sMBACLEnabled;
}
/**
*
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to map file
* and directory permissions to the POSIX permissions.
*
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the Storage
* Gateway User Guide.
*
*
* @return Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to
* map file and directory permissions to the POSIX permissions.
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the
* Storage Gateway User Guide.
*/
public Boolean getSMBACLEnabled() {
return this.sMBACLEnabled;
}
/**
*
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to map file
* and directory permissions to the POSIX permissions.
*
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the Storage
* Gateway User Guide.
*
*
* @param sMBACLEnabled
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to
* map file and directory permissions to the POSIX permissions.
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the
* Storage Gateway User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withSMBACLEnabled(Boolean sMBACLEnabled) {
setSMBACLEnabled(sMBACLEnabled);
return this;
}
/**
*
* Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to map file
* and directory permissions to the POSIX permissions.
*
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the Storage
* Gateway User Guide.
*
*
* @return Set this value to "true to enable ACL (access control list) on the SMB file share. Set it to "false" to
* map file and directory permissions to the POSIX permissions.
*
* For more information, see https://docs.aws.amazon.com/storagegateway/latest/userguide/smb-acl.htmlin the
* Storage Gateway User Guide.
*/
public Boolean isSMBACLEnabled() {
return this.sMBACLEnabled;
}
/**
*
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set
* to ActiveDirectory
.
*/
public java.util.List getAdminUserList() {
if (adminUserList == null) {
adminUserList = new com.amazonaws.internal.SdkInternalList();
}
return adminUserList;
}
/**
*
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param adminUserList
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set
* to ActiveDirectory
.
*/
public void setAdminUserList(java.util.Collection adminUserList) {
if (adminUserList == null) {
this.adminUserList = null;
return;
}
this.adminUserList = new com.amazonaws.internal.SdkInternalList(adminUserList);
}
/**
*
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdminUserList(java.util.Collection)} or {@link #withAdminUserList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param adminUserList
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set
* to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withAdminUserList(String... adminUserList) {
if (this.adminUserList == null) {
setAdminUserList(new com.amazonaws.internal.SdkInternalList(adminUserList.length));
}
for (String ele : adminUserList) {
this.adminUserList.add(ele);
}
return this;
}
/**
*
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param adminUserList
* A list of users in the Active Directory that have administrator rights to the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set
* to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withAdminUserList(java.util.Collection adminUserList) {
setAdminUserList(adminUserList);
return this;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. For example @group1
. Can only be set if Authentication is
* set to ActiveDirectory
.
*/
public java.util.List getValidUserList() {
if (validUserList == null) {
validUserList = new com.amazonaws.internal.SdkInternalList();
}
return validUserList;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. For example @group1
. Can only be set if Authentication is
* set to ActiveDirectory
.
*/
public void setValidUserList(java.util.Collection validUserList) {
if (validUserList == null) {
this.validUserList = null;
return;
}
this.validUserList = new com.amazonaws.internal.SdkInternalList(validUserList);
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValidUserList(java.util.Collection)} or {@link #withValidUserList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. For example @group1
. Can only be set if Authentication is
* set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withValidUserList(String... validUserList) {
if (this.validUserList == null) {
setValidUserList(new com.amazonaws.internal.SdkInternalList(validUserList.length));
}
for (String ele : validUserList) {
this.validUserList.add(ele);
}
return this;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. For example @group1
. Can only be set if Authentication is
* set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withValidUserList(java.util.Collection validUserList) {
setValidUserList(validUserList);
return this;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. For example @group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public java.util.List getInvalidUserList() {
if (invalidUserList == null) {
invalidUserList = new com.amazonaws.internal.SdkInternalList();
}
return invalidUserList;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. For example @group1
. Can only be set if Authentication
* is set to ActiveDirectory
.
*/
public void setInvalidUserList(java.util.Collection invalidUserList) {
if (invalidUserList == null) {
this.invalidUserList = null;
return;
}
this.invalidUserList = new com.amazonaws.internal.SdkInternalList(invalidUserList);
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInvalidUserList(java.util.Collection)} or {@link #withInvalidUserList(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. For example @group1
. Can only be set if Authentication
* is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withInvalidUserList(String... invalidUserList) {
if (this.invalidUserList == null) {
setInvalidUserList(new com.amazonaws.internal.SdkInternalList(invalidUserList.length));
}
for (String ele : invalidUserList) {
this.invalidUserList.add(ele);
}
return this;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. For example @group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. For example @group1
. Can only be set if Authentication
* is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSMBFileShareRequest withInvalidUserList(java.util.Collection invalidUserList) {
setInvalidUserList(invalidUserList);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFileShareARN() != null)
sb.append("FileShareARN: ").append(getFileShareARN()).append(",");
if (getKMSEncrypted() != null)
sb.append("KMSEncrypted: ").append(getKMSEncrypted()).append(",");
if (getKMSKey() != null)
sb.append("KMSKey: ").append(getKMSKey()).append(",");
if (getDefaultStorageClass() != null)
sb.append("DefaultStorageClass: ").append(getDefaultStorageClass()).append(",");
if (getObjectACL() != null)
sb.append("ObjectACL: ").append(getObjectACL()).append(",");
if (getReadOnly() != null)
sb.append("ReadOnly: ").append(getReadOnly()).append(",");
if (getGuessMIMETypeEnabled() != null)
sb.append("GuessMIMETypeEnabled: ").append(getGuessMIMETypeEnabled()).append(",");
if (getRequesterPays() != null)
sb.append("RequesterPays: ").append(getRequesterPays()).append(",");
if (getSMBACLEnabled() != null)
sb.append("SMBACLEnabled: ").append(getSMBACLEnabled()).append(",");
if (getAdminUserList() != null)
sb.append("AdminUserList: ").append(getAdminUserList()).append(",");
if (getValidUserList() != null)
sb.append("ValidUserList: ").append(getValidUserList()).append(",");
if (getInvalidUserList() != null)
sb.append("InvalidUserList: ").append(getInvalidUserList());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateSMBFileShareRequest == false)
return false;
UpdateSMBFileShareRequest other = (UpdateSMBFileShareRequest) obj;
if (other.getFileShareARN() == null ^ this.getFileShareARN() == null)
return false;
if (other.getFileShareARN() != null && other.getFileShareARN().equals(this.getFileShareARN()) == false)
return false;
if (other.getKMSEncrypted() == null ^ this.getKMSEncrypted() == null)
return false;
if (other.getKMSEncrypted() != null && other.getKMSEncrypted().equals(this.getKMSEncrypted()) == false)
return false;
if (other.getKMSKey() == null ^ this.getKMSKey() == null)
return false;
if (other.getKMSKey() != null && other.getKMSKey().equals(this.getKMSKey()) == false)
return false;
if (other.getDefaultStorageClass() == null ^ this.getDefaultStorageClass() == null)
return false;
if (other.getDefaultStorageClass() != null && other.getDefaultStorageClass().equals(this.getDefaultStorageClass()) == false)
return false;
if (other.getObjectACL() == null ^ this.getObjectACL() == null)
return false;
if (other.getObjectACL() != null && other.getObjectACL().equals(this.getObjectACL()) == false)
return false;
if (other.getReadOnly() == null ^ this.getReadOnly() == null)
return false;
if (other.getReadOnly() != null && other.getReadOnly().equals(this.getReadOnly()) == false)
return false;
if (other.getGuessMIMETypeEnabled() == null ^ this.getGuessMIMETypeEnabled() == null)
return false;
if (other.getGuessMIMETypeEnabled() != null && other.getGuessMIMETypeEnabled().equals(this.getGuessMIMETypeEnabled()) == false)
return false;
if (other.getRequesterPays() == null ^ this.getRequesterPays() == null)
return false;
if (other.getRequesterPays() != null && other.getRequesterPays().equals(this.getRequesterPays()) == false)
return false;
if (other.getSMBACLEnabled() == null ^ this.getSMBACLEnabled() == null)
return false;
if (other.getSMBACLEnabled() != null && other.getSMBACLEnabled().equals(this.getSMBACLEnabled()) == false)
return false;
if (other.getAdminUserList() == null ^ this.getAdminUserList() == null)
return false;
if (other.getAdminUserList() != null && other.getAdminUserList().equals(this.getAdminUserList()) == false)
return false;
if (other.getValidUserList() == null ^ this.getValidUserList() == null)
return false;
if (other.getValidUserList() != null && other.getValidUserList().equals(this.getValidUserList()) == false)
return false;
if (other.getInvalidUserList() == null ^ this.getInvalidUserList() == null)
return false;
if (other.getInvalidUserList() != null && other.getInvalidUserList().equals(this.getInvalidUserList()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFileShareARN() == null) ? 0 : getFileShareARN().hashCode());
hashCode = prime * hashCode + ((getKMSEncrypted() == null) ? 0 : getKMSEncrypted().hashCode());
hashCode = prime * hashCode + ((getKMSKey() == null) ? 0 : getKMSKey().hashCode());
hashCode = prime * hashCode + ((getDefaultStorageClass() == null) ? 0 : getDefaultStorageClass().hashCode());
hashCode = prime * hashCode + ((getObjectACL() == null) ? 0 : getObjectACL().hashCode());
hashCode = prime * hashCode + ((getReadOnly() == null) ? 0 : getReadOnly().hashCode());
hashCode = prime * hashCode + ((getGuessMIMETypeEnabled() == null) ? 0 : getGuessMIMETypeEnabled().hashCode());
hashCode = prime * hashCode + ((getRequesterPays() == null) ? 0 : getRequesterPays().hashCode());
hashCode = prime * hashCode + ((getSMBACLEnabled() == null) ? 0 : getSMBACLEnabled().hashCode());
hashCode = prime * hashCode + ((getAdminUserList() == null) ? 0 : getAdminUserList().hashCode());
hashCode = prime * hashCode + ((getValidUserList() == null) ? 0 : getValidUserList().hashCode());
hashCode = prime * hashCode + ((getInvalidUserList() == null) ? 0 : getInvalidUserList().hashCode());
return hashCode;
}
@Override
public UpdateSMBFileShareRequest clone() {
return (UpdateSMBFileShareRequest) super.clone();
}
}