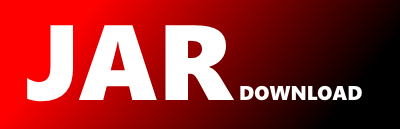
com.amazonaws.services.storagegateway.AWSStorageGatewayAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.storagegateway;
import javax.annotation.Generated;
import com.amazonaws.services.storagegateway.model.*;
/**
* Interface for accessing AWS Storage Gateway asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.storagegateway.AbstractAWSStorageGatewayAsync} instead.
*
*
* Storage Gateway Service
*
* Storage Gateway is the service that connects an on-premises software appliance with cloud-based storage to provide
* seamless and secure integration between an organization's on-premises IT environment and the Amazon Web Services
* storage infrastructure. The service enables you to securely upload data to the Amazon Web Services Cloud for cost
* effective backup and rapid disaster recovery.
*
*
* Use the following links to get started using the Storage Gateway Service API Reference:
*
*
* -
*
* Storage Gateway required request headers: Describes the required headers that you must send with every POST
* request to Storage Gateway.
*
*
* -
*
* Signing requests: Storage Gateway requires that you authenticate every request you send; this topic describes
* how sign such a request.
*
*
* -
*
*
* Error responses: Provides reference information about Storage Gateway errors.
*
*
* -
*
* Operations in Storage
* Gateway: Contains detailed descriptions of all Storage Gateway operations, their request parameters, response
* elements, possible errors, and examples of requests and responses.
*
*
* -
*
* Storage Gateway endpoints and quotas: Provides a
* list of each Amazon Web Services Region and the endpoints available for use with Storage Gateway.
*
*
*
*
*
* Storage Gateway resource IDs are in uppercase. When you use these resource IDs with the Amazon EC2 API, EC2 expects
* resource IDs in lowercase. You must change your resource ID to lowercase to use it with the EC2 API. For example, in
* Storage Gateway the ID for a volume might be vol-AA22BB012345DAF670
. When you use this ID with the EC2
* API, you must change it to vol-aa22bb012345daf670
. Otherwise, the EC2 API might not behave as expected.
*
*
*
* IDs for Storage Gateway volumes and Amazon EBS snapshots created from gateway volumes are changing to a longer
* format. Starting in December 2016, all new volumes and snapshots will be created with a 17-character string. Starting
* in April 2016, you will be able to use these longer IDs so you can test your systems with the new format. For more
* information, see Longer EC2 and EBS resource IDs.
*
*
* For example, a volume Amazon Resource Name (ARN) with the longer volume ID format looks like the following:
*
*
* arn:aws:storagegateway:us-west-2:111122223333:gateway/sgw-12A3456B/volume/vol-1122AABBCCDDEEFFG
.
*
*
* A snapshot ID with the longer ID format looks like the following: snap-78e226633445566ee
.
*
*
* For more information, see Announcement: Heads-up – Longer
* Storage Gateway volume and snapshot IDs coming in 2016.
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSStorageGatewayAsync extends AWSStorageGateway {
/**
*
* Activates the gateway you previously deployed on your host. In the activation process, you specify information
* such as the Amazon Web Services Region that you want to use for storing snapshots or tapes, the time zone for
* scheduled snapshots the gateway snapshot schedule window, an activation key, and a name for your gateway. The
* activation process also associates your gateway with your account. For more information, see
* UpdateGatewayInformation.
*
*
*
* You must turn on the gateway VM before you can activate your gateway.
*
*
*
* @param activateGatewayRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ActivateGateway operation returned by the service.
* @sample AWSStorageGatewayAsync.ActivateGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future activateGatewayAsync(ActivateGatewayRequest activateGatewayRequest);
/**
*
* Activates the gateway you previously deployed on your host. In the activation process, you specify information
* such as the Amazon Web Services Region that you want to use for storing snapshots or tapes, the time zone for
* scheduled snapshots the gateway snapshot schedule window, an activation key, and a name for your gateway. The
* activation process also associates your gateway with your account. For more information, see
* UpdateGatewayInformation.
*
*
*
* You must turn on the gateway VM before you can activate your gateway.
*
*
*
* @param activateGatewayRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ActivateGateway operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ActivateGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future activateGatewayAsync(ActivateGatewayRequest activateGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as cache for a gateway. This operation is only supported in the cached
* volume, tape, and file gateway type (see How Storage
* Gateway works (architecture).
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add cache, and one or
* more disk IDs that you want to configure as cache.
*
*
* @param addCacheRequest
* @return A Java Future containing the result of the AddCache operation returned by the service.
* @sample AWSStorageGatewayAsync.AddCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addCacheAsync(AddCacheRequest addCacheRequest);
/**
*
* Configures one or more gateway local disks as cache for a gateway. This operation is only supported in the cached
* volume, tape, and file gateway type (see How Storage
* Gateway works (architecture).
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add cache, and one or
* more disk IDs that you want to configure as cache.
*
*
* @param addCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddCache operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addCacheAsync(AddCacheRequest addCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more tags to the specified resource. You use tags to add metadata to resources, which you can use to
* categorize these resources. For example, you can categorize resources by purpose, owner, environment, or team.
* Each tag consists of a key and a value, which you define. You can add tags to the following Storage Gateway
* resources:
*
*
* -
*
* Storage gateways of all types
*
*
* -
*
* Storage volumes
*
*
* -
*
* Virtual tapes
*
*
* -
*
* NFS and SMB file shares
*
*
* -
*
* File System associations
*
*
*
*
* You can create a maximum of 50 tags for each resource. Virtual tapes and storage volumes that are recovered to a
* new gateway maintain their tags.
*
*
* @param addTagsToResourceRequest
* AddTagsToResourceInput
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSStorageGatewayAsync.AddTagsToResource
* @see AWS API Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds one or more tags to the specified resource. You use tags to add metadata to resources, which you can use to
* categorize these resources. For example, you can categorize resources by purpose, owner, environment, or team.
* Each tag consists of a key and a value, which you define. You can add tags to the following Storage Gateway
* resources:
*
*
* -
*
* Storage gateways of all types
*
*
* -
*
* Storage volumes
*
*
* -
*
* Virtual tapes
*
*
* -
*
* NFS and SMB file shares
*
*
* -
*
* File System associations
*
*
*
*
* You can create a maximum of 50 tags for each resource. Virtual tapes and storage volumes that are recovered to a
* new gateway maintain their tags.
*
*
* @param addTagsToResourceRequest
* AddTagsToResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddTagsToResource
* @see AWS API Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as upload buffer for a specified gateway. This operation is supported
* for the stored volume, cached volume, and tape gateway types.
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add upload buffer, and
* one or more disk IDs that you want to configure as upload buffer.
*
*
* @param addUploadBufferRequest
* @return A Java Future containing the result of the AddUploadBuffer operation returned by the service.
* @sample AWSStorageGatewayAsync.AddUploadBuffer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addUploadBufferAsync(AddUploadBufferRequest addUploadBufferRequest);
/**
*
* Configures one or more gateway local disks as upload buffer for a specified gateway. This operation is supported
* for the stored volume, cached volume, and tape gateway types.
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add upload buffer, and
* one or more disk IDs that you want to configure as upload buffer.
*
*
* @param addUploadBufferRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddUploadBuffer operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddUploadBuffer
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addUploadBufferAsync(AddUploadBufferRequest addUploadBufferRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Configures one or more gateway local disks as working storage for a gateway. This operation is only supported in
* the stored volume gateway type. This operation is deprecated in cached volume API version 20120630. Use
* AddUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use the AddUploadBuffer operation to
* add upload buffer to a stored volume gateway.
*
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add working storage, and
* one or more disk IDs that you want to configure as working storage.
*
*
* @param addWorkingStorageRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* @return A Java Future containing the result of the AddWorkingStorage operation returned by the service.
* @sample AWSStorageGatewayAsync.AddWorkingStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future addWorkingStorageAsync(AddWorkingStorageRequest addWorkingStorageRequest);
/**
*
* Configures one or more gateway local disks as working storage for a gateway. This operation is only supported in
* the stored volume gateway type. This operation is deprecated in cached volume API version 20120630. Use
* AddUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use the AddUploadBuffer operation to
* add upload buffer to a stored volume gateway.
*
*
*
* In the request, you specify the gateway Amazon Resource Name (ARN) to which you want to add working storage, and
* one or more disk IDs that you want to configure as working storage.
*
*
* @param addWorkingStorageRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddWorkingStorage operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AddWorkingStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future addWorkingStorageAsync(AddWorkingStorageRequest addWorkingStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns a tape to a tape pool for archiving. The tape assigned to a pool is archived in the S3 storage class that
* is associated with the pool. When you use your backup application to eject the tape, the tape is archived
* directly into the S3 storage class (S3 Glacier or S3 Glacier Deep Archive) that corresponds to the pool.
*
*
* @param assignTapePoolRequest
* @return A Java Future containing the result of the AssignTapePool operation returned by the service.
* @sample AWSStorageGatewayAsync.AssignTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignTapePoolAsync(AssignTapePoolRequest assignTapePoolRequest);
/**
*
* Assigns a tape to a tape pool for archiving. The tape assigned to a pool is archived in the S3 storage class that
* is associated with the pool. When you use your backup application to eject the tape, the tape is archived
* directly into the S3 storage class (S3 Glacier or S3 Glacier Deep Archive) that corresponds to the pool.
*
*
* @param assignTapePoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignTapePool operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AssignTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignTapePoolAsync(AssignTapePoolRequest assignTapePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associate an Amazon FSx file system with the FSx File Gateway. After the association process is complete, the
* file shares on the Amazon FSx file system are available for access through the gateway. This operation only
* supports the FSx File Gateway type.
*
*
* @param associateFileSystemRequest
* @return A Java Future containing the result of the AssociateFileSystem operation returned by the service.
* @sample AWSStorageGatewayAsync.AssociateFileSystem
* @see AWS API Documentation
*/
java.util.concurrent.Future associateFileSystemAsync(AssociateFileSystemRequest associateFileSystemRequest);
/**
*
* Associate an Amazon FSx file system with the FSx File Gateway. After the association process is complete, the
* file shares on the Amazon FSx file system are available for access through the gateway. This operation only
* supports the FSx File Gateway type.
*
*
* @param associateFileSystemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateFileSystem operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AssociateFileSystem
* @see AWS API Documentation
*/
java.util.concurrent.Future associateFileSystemAsync(AssociateFileSystemRequest associateFileSystemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Connects a volume to an iSCSI connection and then attaches the volume to the specified gateway. Detaching and
* attaching a volume enables you to recover your data from one gateway to a different gateway without creating a
* snapshot. It also makes it easier to move your volumes from an on-premises gateway to a gateway hosted on an
* Amazon EC2 instance.
*
*
* @param attachVolumeRequest
* AttachVolumeInput
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.AttachVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest);
/**
*
* Connects a volume to an iSCSI connection and then attaches the volume to the specified gateway. Detaching and
* attaching a volume enables you to recover your data from one gateway to a different gateway without creating a
* snapshot. It also makes it easier to move your volumes from an on-premises gateway to a gateway hosted on an
* Amazon EC2 instance.
*
*
* @param attachVolumeRequest
* AttachVolumeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.AttachVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels archiving of a virtual tape to the virtual tape shelf (VTS) after the archiving process is initiated.
* This operation is only supported in the tape gateway type.
*
*
* @param cancelArchivalRequest
* CancelArchivalInput
* @return A Java Future containing the result of the CancelArchival operation returned by the service.
* @sample AWSStorageGatewayAsync.CancelArchival
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelArchivalAsync(CancelArchivalRequest cancelArchivalRequest);
/**
*
* Cancels archiving of a virtual tape to the virtual tape shelf (VTS) after the archiving process is initiated.
* This operation is only supported in the tape gateway type.
*
*
* @param cancelArchivalRequest
* CancelArchivalInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelArchival operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CancelArchival
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelArchivalAsync(CancelArchivalRequest cancelArchivalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels retrieval of a virtual tape from the virtual tape shelf (VTS) to a gateway after the retrieval process is
* initiated. The virtual tape is returned to the VTS. This operation is only supported in the tape gateway type.
*
*
* @param cancelRetrievalRequest
* CancelRetrievalInput
* @return A Java Future containing the result of the CancelRetrieval operation returned by the service.
* @sample AWSStorageGatewayAsync.CancelRetrieval
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelRetrievalAsync(CancelRetrievalRequest cancelRetrievalRequest);
/**
*
* Cancels retrieval of a virtual tape from the virtual tape shelf (VTS) to a gateway after the retrieval process is
* initiated. The virtual tape is returned to the VTS. This operation is only supported in the tape gateway type.
*
*
* @param cancelRetrievalRequest
* CancelRetrievalInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelRetrieval operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CancelRetrieval
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelRetrievalAsync(CancelRetrievalRequest cancelRetrievalRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a cached volume on a specified cached volume gateway. This operation is only supported in the cached
* volume gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create a cached volume. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* In the request, you must specify the gateway, size of the volume in bytes, the iSCSI target name, an IP address
* on which to expose the target, and a unique client token. In response, the gateway creates the volume and returns
* information about it. This information includes the volume Amazon Resource Name (ARN), its size, and the iSCSI
* target ARN that initiators can use to connect to the volume target.
*
*
* Optionally, you can provide the ARN for an existing volume as the SourceVolumeARN
for this cached
* volume, which creates an exact copy of the existing volume’s latest recovery point. The
* VolumeSizeInBytes
value must be equal to or larger than the size of the copied volume, in bytes.
*
*
* @param createCachediSCSIVolumeRequest
* @return A Java Future containing the result of the CreateCachediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateCachediSCSIVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future createCachediSCSIVolumeAsync(CreateCachediSCSIVolumeRequest createCachediSCSIVolumeRequest);
/**
*
* Creates a cached volume on a specified cached volume gateway. This operation is only supported in the cached
* volume gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create a cached volume. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* In the request, you must specify the gateway, size of the volume in bytes, the iSCSI target name, an IP address
* on which to expose the target, and a unique client token. In response, the gateway creates the volume and returns
* information about it. This information includes the volume Amazon Resource Name (ARN), its size, and the iSCSI
* target ARN that initiators can use to connect to the volume target.
*
*
* Optionally, you can provide the ARN for an existing volume as the SourceVolumeARN
for this cached
* volume, which creates an exact copy of the existing volume’s latest recovery point. The
* VolumeSizeInBytes
value must be equal to or larger than the size of the copied volume, in bytes.
*
*
* @param createCachediSCSIVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCachediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateCachediSCSIVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future createCachediSCSIVolumeAsync(CreateCachediSCSIVolumeRequest createCachediSCSIVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Network File System (NFS) file share on an existing S3 File Gateway. In Storage Gateway, a file share
* is a file system mount point backed by Amazon S3 cloud storage. Storage Gateway exposes file shares using an NFS
* interface. This operation is only supported for S3 File Gateways.
*
*
*
* S3 File gateway requires Security Token Service (Amazon Web Services STS) to be activated to enable you to create
* a file share. Make sure Amazon Web Services STS is activated in the Amazon Web Services Region you are creating
* your S3 File Gateway in. If Amazon Web Services STS is not activated in the Amazon Web Services Region, activate
* it. For information about how to activate Amazon Web Services STS, see Activating and
* deactivating Amazon Web Services STS in an Amazon Web Services Region in the Identity and Access
* Management User Guide.
*
*
* S3 File Gateways do not support creating hard or symbolic links on a file share.
*
*
*
* @param createNFSFileShareRequest
* CreateNFSFileShareInput
* @return A Java Future containing the result of the CreateNFSFileShare operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateNFSFileShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createNFSFileShareAsync(CreateNFSFileShareRequest createNFSFileShareRequest);
/**
*
* Creates a Network File System (NFS) file share on an existing S3 File Gateway. In Storage Gateway, a file share
* is a file system mount point backed by Amazon S3 cloud storage. Storage Gateway exposes file shares using an NFS
* interface. This operation is only supported for S3 File Gateways.
*
*
*
* S3 File gateway requires Security Token Service (Amazon Web Services STS) to be activated to enable you to create
* a file share. Make sure Amazon Web Services STS is activated in the Amazon Web Services Region you are creating
* your S3 File Gateway in. If Amazon Web Services STS is not activated in the Amazon Web Services Region, activate
* it. For information about how to activate Amazon Web Services STS, see Activating and
* deactivating Amazon Web Services STS in an Amazon Web Services Region in the Identity and Access
* Management User Guide.
*
*
* S3 File Gateways do not support creating hard or symbolic links on a file share.
*
*
*
* @param createNFSFileShareRequest
* CreateNFSFileShareInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNFSFileShare operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateNFSFileShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createNFSFileShareAsync(CreateNFSFileShareRequest createNFSFileShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Server Message Block (SMB) file share on an existing S3 File Gateway. In Storage Gateway, a file share
* is a file system mount point backed by Amazon S3 cloud storage. Storage Gateway exposes file shares using an SMB
* interface. This operation is only supported for S3 File Gateways.
*
*
*
* S3 File Gateways require Security Token Service (Amazon Web Services STS) to be activated to enable you to create
* a file share. Make sure that Amazon Web Services STS is activated in the Amazon Web Services Region you are
* creating your S3 File Gateway in. If Amazon Web Services STS is not activated in this Amazon Web Services Region,
* activate it. For information about how to activate Amazon Web Services STS, see Activating and
* deactivating Amazon Web Services STS in an Amazon Web Services Region in the Identity and Access
* Management User Guide.
*
*
* File gateways don't support creating hard or symbolic links on a file share.
*
*
*
* @param createSMBFileShareRequest
* CreateSMBFileShareInput
* @return A Java Future containing the result of the CreateSMBFileShare operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateSMBFileShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createSMBFileShareAsync(CreateSMBFileShareRequest createSMBFileShareRequest);
/**
*
* Creates a Server Message Block (SMB) file share on an existing S3 File Gateway. In Storage Gateway, a file share
* is a file system mount point backed by Amazon S3 cloud storage. Storage Gateway exposes file shares using an SMB
* interface. This operation is only supported for S3 File Gateways.
*
*
*
* S3 File Gateways require Security Token Service (Amazon Web Services STS) to be activated to enable you to create
* a file share. Make sure that Amazon Web Services STS is activated in the Amazon Web Services Region you are
* creating your S3 File Gateway in. If Amazon Web Services STS is not activated in this Amazon Web Services Region,
* activate it. For information about how to activate Amazon Web Services STS, see Activating and
* deactivating Amazon Web Services STS in an Amazon Web Services Region in the Identity and Access
* Management User Guide.
*
*
* File gateways don't support creating hard or symbolic links on a file share.
*
*
*
* @param createSMBFileShareRequest
* CreateSMBFileShareInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSMBFileShare operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateSMBFileShare
* @see AWS API Documentation
*/
java.util.concurrent.Future createSMBFileShareAsync(CreateSMBFileShareRequest createSMBFileShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a snapshot of a volume.
*
*
* Storage Gateway provides the ability to back up point-in-time snapshots of your data to Amazon Simple Storage
* (Amazon S3) for durable off-site recovery, and also import the data to an Amazon Elastic Block Store (EBS) volume
* in Amazon Elastic Compute Cloud (EC2). You can take snapshots of your gateway volume on a scheduled or ad hoc
* basis. This API enables you to take an ad hoc snapshot. For more information, see Editing a snapshot schedule.
*
*
* In the CreateSnapshot
request, you identify the volume by providing its Amazon Resource Name (ARN).
* You must also provide description for the snapshot. When Storage Gateway takes the snapshot of specified volume,
* the snapshot and description appears in the Storage Gateway console. In response, Storage Gateway returns you a
* snapshot ID. You can use this snapshot ID to check the snapshot progress or later use it when you want to create
* a volume from a snapshot. This operation is only supported in stored and cached volume gateway type.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, see DescribeSnapshots or
* DeleteSnapshot in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* Volume and snapshot IDs are changing to a longer length ID format. For more information, see the important note
* on the Welcome page.
*
*
*
* @param createSnapshotRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Initiates a snapshot of a volume.
*
*
* Storage Gateway provides the ability to back up point-in-time snapshots of your data to Amazon Simple Storage
* (Amazon S3) for durable off-site recovery, and also import the data to an Amazon Elastic Block Store (EBS) volume
* in Amazon Elastic Compute Cloud (EC2). You can take snapshots of your gateway volume on a scheduled or ad hoc
* basis. This API enables you to take an ad hoc snapshot. For more information, see Editing a snapshot schedule.
*
*
* In the CreateSnapshot
request, you identify the volume by providing its Amazon Resource Name (ARN).
* You must also provide description for the snapshot. When Storage Gateway takes the snapshot of specified volume,
* the snapshot and description appears in the Storage Gateway console. In response, Storage Gateway returns you a
* snapshot ID. You can use this snapshot ID to check the snapshot progress or later use it when you want to create
* a volume from a snapshot. This operation is only supported in stored and cached volume gateway type.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, see DescribeSnapshots or
* DeleteSnapshot in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* Volume and snapshot IDs are changing to a longer length ID format. For more information, see the important note
* on the Welcome page.
*
*
*
* @param createSnapshotRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateSnapshot
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates a snapshot of a gateway from a volume recovery point. This operation is only supported in the cached
* volume gateway type.
*
*
* A volume recovery point is a point in time at which all data of the volume is consistent and from which you can
* create a snapshot. To get a list of volume recovery point for cached volume gateway, use
* ListVolumeRecoveryPoints.
*
*
* In the CreateSnapshotFromVolumeRecoveryPoint
request, you identify the volume by providing its
* Amazon Resource Name (ARN). You must also provide a description for the snapshot. When the gateway takes a
* snapshot of the specified volume, the snapshot and its description appear in the Storage Gateway console. In
* response, the gateway returns you a snapshot ID. You can use this snapshot ID to check the snapshot progress or
* later use it when you want to create a volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, see DescribeSnapshots or
* DeleteSnapshot in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param createSnapshotFromVolumeRecoveryPointRequest
* @return A Java Future containing the result of the CreateSnapshotFromVolumeRecoveryPoint operation returned by
* the service.
* @sample AWSStorageGatewayAsync.CreateSnapshotFromVolumeRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotFromVolumeRecoveryPointAsync(
CreateSnapshotFromVolumeRecoveryPointRequest createSnapshotFromVolumeRecoveryPointRequest);
/**
*
* Initiates a snapshot of a gateway from a volume recovery point. This operation is only supported in the cached
* volume gateway type.
*
*
* A volume recovery point is a point in time at which all data of the volume is consistent and from which you can
* create a snapshot. To get a list of volume recovery point for cached volume gateway, use
* ListVolumeRecoveryPoints.
*
*
* In the CreateSnapshotFromVolumeRecoveryPoint
request, you identify the volume by providing its
* Amazon Resource Name (ARN). You must also provide a description for the snapshot. When the gateway takes a
* snapshot of the specified volume, the snapshot and its description appear in the Storage Gateway console. In
* response, the gateway returns you a snapshot ID. You can use this snapshot ID to check the snapshot progress or
* later use it when you want to create a volume from a snapshot.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, see DescribeSnapshots or
* DeleteSnapshot in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param createSnapshotFromVolumeRecoveryPointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshotFromVolumeRecoveryPoint operation returned by
* the service.
* @sample AWSStorageGatewayAsyncHandler.CreateSnapshotFromVolumeRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future createSnapshotFromVolumeRecoveryPointAsync(
CreateSnapshotFromVolumeRecoveryPointRequest createSnapshotFromVolumeRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a volume on a specified gateway. This operation is only supported in the stored volume gateway type.
*
*
* The size of the volume to create is inferred from the disk size. You can choose to preserve existing data on the
* disk, create volume from an existing snapshot, or create an empty volume. If you choose to create an empty
* gateway volume, then any existing data on the disk is erased.
*
*
* In the request, you must specify the gateway and the disk information on which you are creating the volume. In
* response, the gateway creates the volume and returns volume information such as the volume Amazon Resource Name
* (ARN), its size, and the iSCSI target ARN that initiators can use to connect to the volume target.
*
*
* @param createStorediSCSIVolumeRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the CreateStorediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateStorediSCSIVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorediSCSIVolumeAsync(CreateStorediSCSIVolumeRequest createStorediSCSIVolumeRequest);
/**
*
* Creates a volume on a specified gateway. This operation is only supported in the stored volume gateway type.
*
*
* The size of the volume to create is inferred from the disk size. You can choose to preserve existing data on the
* disk, create volume from an existing snapshot, or create an empty volume. If you choose to create an empty
* gateway volume, then any existing data on the disk is erased.
*
*
* In the request, you must specify the gateway and the disk information on which you are creating the volume. In
* response, the gateway creates the volume and returns volume information such as the volume Amazon Resource Name
* (ARN), its size, and the iSCSI target ARN that initiators can use to connect to the volume target.
*
*
* @param createStorediSCSIVolumeRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStorediSCSIVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateStorediSCSIVolume
* @see AWS API Documentation
*/
java.util.concurrent.Future createStorediSCSIVolumeAsync(CreateStorediSCSIVolumeRequest createStorediSCSIVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom tape pool. You can use custom tape pool to enable tape retention lock on tapes that are
* archived in the custom pool.
*
*
* @param createTapePoolRequest
* @return A Java Future containing the result of the CreateTapePool operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTapePoolAsync(CreateTapePoolRequest createTapePoolRequest);
/**
*
* Creates a new custom tape pool. You can use custom tape pool to enable tape retention lock on tapes that are
* archived in the custom pool.
*
*
* @param createTapePoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTapePool operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTapePoolAsync(CreateTapePoolRequest createTapePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a virtual tape by using your own barcode. You write data to the virtual tape and then archive the tape. A
* barcode is unique and cannot be reused if it has already been used on a tape. This applies to barcodes used on
* deleted tapes. This operation is only supported in the tape gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create a virtual tape. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* @param createTapeWithBarcodeRequest
* CreateTapeWithBarcodeInput
* @return A Java Future containing the result of the CreateTapeWithBarcode operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateTapeWithBarcode
* @see AWS API Documentation
*/
java.util.concurrent.Future createTapeWithBarcodeAsync(CreateTapeWithBarcodeRequest createTapeWithBarcodeRequest);
/**
*
* Creates a virtual tape by using your own barcode. You write data to the virtual tape and then archive the tape. A
* barcode is unique and cannot be reused if it has already been used on a tape. This applies to barcodes used on
* deleted tapes. This operation is only supported in the tape gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create a virtual tape. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* @param createTapeWithBarcodeRequest
* CreateTapeWithBarcodeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTapeWithBarcode operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateTapeWithBarcode
* @see AWS API Documentation
*/
java.util.concurrent.Future createTapeWithBarcodeAsync(CreateTapeWithBarcodeRequest createTapeWithBarcodeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates one or more virtual tapes. You write data to the virtual tapes and then archive the tapes. This operation
* is only supported in the tape gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create virtual tapes. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* @param createTapesRequest
* CreateTapesInput
* @return A Java Future containing the result of the CreateTapes operation returned by the service.
* @sample AWSStorageGatewayAsync.CreateTapes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTapesAsync(CreateTapesRequest createTapesRequest);
/**
*
* Creates one or more virtual tapes. You write data to the virtual tapes and then archive the tapes. This operation
* is only supported in the tape gateway type.
*
*
*
* Cache storage must be allocated to the gateway before you can create virtual tapes. Use the AddCache
* operation to add cache storage to a gateway.
*
*
*
* @param createTapesRequest
* CreateTapesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTapes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.CreateTapes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTapesAsync(CreateTapesRequest createTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the automatic tape creation policy of a gateway. If you delete this policy, new virtual tapes must be
* created manually. Use the Amazon Resource Name (ARN) of the gateway in your request to remove the policy.
*
*
* @param deleteAutomaticTapeCreationPolicyRequest
* @return A Java Future containing the result of the DeleteAutomaticTapeCreationPolicy operation returned by the
* service.
* @sample AWSStorageGatewayAsync.DeleteAutomaticTapeCreationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAutomaticTapeCreationPolicyAsync(
DeleteAutomaticTapeCreationPolicyRequest deleteAutomaticTapeCreationPolicyRequest);
/**
*
* Deletes the automatic tape creation policy of a gateway. If you delete this policy, new virtual tapes must be
* created manually. Use the Amazon Resource Name (ARN) of the gateway in your request to remove the policy.
*
*
* @param deleteAutomaticTapeCreationPolicyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAutomaticTapeCreationPolicy operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.DeleteAutomaticTapeCreationPolicy
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAutomaticTapeCreationPolicyAsync(
DeleteAutomaticTapeCreationPolicyRequest deleteAutomaticTapeCreationPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the bandwidth rate limits of a gateway. You can delete either the upload and download bandwidth rate
* limit, or you can delete both. If you delete only one of the limits, the other limit remains unchanged. To
* specify which gateway to work with, use the Amazon Resource Name (ARN) of the gateway in your request. This
* operation is supported only for the stored volume, cached volume, and tape gateway types.
*
*
* @param deleteBandwidthRateLimitRequest
* A JSON object containing the following fields:
*
* -
*
*
* @return A Java Future containing the result of the DeleteBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteBandwidthRateLimit
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBandwidthRateLimitAsync(DeleteBandwidthRateLimitRequest deleteBandwidthRateLimitRequest);
/**
*
* Deletes the bandwidth rate limits of a gateway. You can delete either the upload and download bandwidth rate
* limit, or you can delete both. If you delete only one of the limits, the other limit remains unchanged. To
* specify which gateway to work with, use the Amazon Resource Name (ARN) of the gateway in your request. This
* operation is supported only for the stored volume, cached volume, and tape gateway types.
*
*
* @param deleteBandwidthRateLimitRequest
* A JSON object containing the following fields:
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteBandwidthRateLimit
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBandwidthRateLimitAsync(DeleteBandwidthRateLimitRequest deleteBandwidthRateLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes Challenge-Handshake Authentication Protocol (CHAP) credentials for a specified iSCSI target and initiator
* pair. This operation is supported in volume and tape gateway types.
*
*
* @param deleteChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the DeleteChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteChapCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChapCredentialsAsync(DeleteChapCredentialsRequest deleteChapCredentialsRequest);
/**
*
* Deletes Challenge-Handshake Authentication Protocol (CHAP) credentials for a specified iSCSI target and initiator
* pair. This operation is supported in volume and tape gateway types.
*
*
* @param deleteChapCredentialsRequest
* A JSON object containing one or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteChapCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChapCredentialsAsync(DeleteChapCredentialsRequest deleteChapCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a file share from an S3 File Gateway. This operation is only supported for S3 File Gateways.
*
*
* @param deleteFileShareRequest
* DeleteFileShareInput
* @return A Java Future containing the result of the DeleteFileShare operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteFileShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteFileShareAsync(DeleteFileShareRequest deleteFileShareRequest);
/**
*
* Deletes a file share from an S3 File Gateway. This operation is only supported for S3 File Gateways.
*
*
* @param deleteFileShareRequest
* DeleteFileShareInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFileShare operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteFileShare
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteFileShareAsync(DeleteFileShareRequest deleteFileShareRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a gateway. To specify which gateway to delete, use the Amazon Resource Name (ARN) of the gateway in your
* request. The operation deletes the gateway; however, it does not delete the gateway virtual machine (VM) from
* your host computer.
*
*
* After you delete a gateway, you cannot reactivate it. Completed snapshots of the gateway volumes are not deleted
* upon deleting the gateway, however, pending snapshots will not complete. After you delete a gateway, your next
* step is to remove it from your environment.
*
*
*
* You no longer pay software charges after the gateway is deleted; however, your existing Amazon EBS snapshots
* persist and you will continue to be billed for these snapshots. You can choose to remove all remaining Amazon EBS
* snapshots by canceling your Amazon EC2 subscription. If you prefer not to cancel your Amazon EC2 subscription,
* you can delete your snapshots using the Amazon EC2 console. For more information, see the Storage Gateway detail page.
*
*
*
* @param deleteGatewayRequest
* A JSON object containing the ID of the gateway to delete.
* @return A Java Future containing the result of the DeleteGateway operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGatewayAsync(DeleteGatewayRequest deleteGatewayRequest);
/**
*
* Deletes a gateway. To specify which gateway to delete, use the Amazon Resource Name (ARN) of the gateway in your
* request. The operation deletes the gateway; however, it does not delete the gateway virtual machine (VM) from
* your host computer.
*
*
* After you delete a gateway, you cannot reactivate it. Completed snapshots of the gateway volumes are not deleted
* upon deleting the gateway, however, pending snapshots will not complete. After you delete a gateway, your next
* step is to remove it from your environment.
*
*
*
* You no longer pay software charges after the gateway is deleted; however, your existing Amazon EBS snapshots
* persist and you will continue to be billed for these snapshots. You can choose to remove all remaining Amazon EBS
* snapshots by canceling your Amazon EC2 subscription. If you prefer not to cancel your Amazon EC2 subscription,
* you can delete your snapshots using the Amazon EC2 console. For more information, see the Storage Gateway detail page.
*
*
*
* @param deleteGatewayRequest
* A JSON object containing the ID of the gateway to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteGateway operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteGatewayAsync(DeleteGatewayRequest deleteGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a snapshot of a volume.
*
*
* You can take snapshots of your gateway volumes on a scheduled or ad hoc basis. This API action enables you to
* delete a snapshot schedule for a volume. For more information, see Backing up your
* volumes. In the DeleteSnapshotSchedule
request, you identify the volume by providing its Amazon
* Resource Name (ARN). This operation is only supported for cached volume gateway types.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, go to DescribeSnapshots in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param deleteSnapshotScheduleRequest
* @return A Java Future containing the result of the DeleteSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest);
/**
*
* Deletes a snapshot of a volume.
*
*
* You can take snapshots of your gateway volumes on a scheduled or ad hoc basis. This API action enables you to
* delete a snapshot schedule for a volume. For more information, see Backing up your
* volumes. In the DeleteSnapshotSchedule
request, you identify the volume by providing its Amazon
* Resource Name (ARN). This operation is only supported for cached volume gateway types.
*
*
*
* To list or delete a snapshot, you must use the Amazon EC2 API. For more information, go to DescribeSnapshots in
* the Amazon Elastic Compute Cloud API Reference.
*
*
*
* @param deleteSnapshotScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSnapshotScheduleAsync(DeleteSnapshotScheduleRequest deleteSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified virtual tape. This operation is only supported in the tape gateway type.
*
*
* @param deleteTapeRequest
* DeleteTapeInput
* @return A Java Future containing the result of the DeleteTape operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteTape
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTapeAsync(DeleteTapeRequest deleteTapeRequest);
/**
*
* Deletes the specified virtual tape. This operation is only supported in the tape gateway type.
*
*
* @param deleteTapeRequest
* DeleteTapeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTape operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteTape
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteTapeAsync(DeleteTapeRequest deleteTapeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified virtual tape from the virtual tape shelf (VTS). This operation is only supported in the
* tape gateway type.
*
*
* @param deleteTapeArchiveRequest
* DeleteTapeArchiveInput
* @return A Java Future containing the result of the DeleteTapeArchive operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteTapeArchive
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTapeArchiveAsync(DeleteTapeArchiveRequest deleteTapeArchiveRequest);
/**
*
* Deletes the specified virtual tape from the virtual tape shelf (VTS). This operation is only supported in the
* tape gateway type.
*
*
* @param deleteTapeArchiveRequest
* DeleteTapeArchiveInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTapeArchive operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteTapeArchive
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTapeArchiveAsync(DeleteTapeArchiveRequest deleteTapeArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Delete a custom tape pool. A custom tape pool can only be deleted if there are no tapes in the pool and if there
* are no automatic tape creation policies that reference the custom tape pool.
*
*
* @param deleteTapePoolRequest
* @return A Java Future containing the result of the DeleteTapePool operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTapePoolAsync(DeleteTapePoolRequest deleteTapePoolRequest);
/**
*
* Delete a custom tape pool. A custom tape pool can only be deleted if there are no tapes in the pool and if there
* are no automatic tape creation policies that reference the custom tape pool.
*
*
* @param deleteTapePoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTapePool operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteTapePool
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTapePoolAsync(DeleteTapePoolRequest deleteTapePoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified storage volume that you previously created using the CreateCachediSCSIVolume or
* CreateStorediSCSIVolume API. This operation is only supported in the cached volume and stored volume
* types. For stored volume gateways, the local disk that was configured as the storage volume is not deleted. You
* can reuse the local disk to create another storage volume.
*
*
* Before you delete a volume, make sure there are no iSCSI connections to the volume you are deleting. You should
* also make sure there is no snapshot in progress. You can use the Amazon Elastic Compute Cloud (Amazon EC2) API to
* query snapshots on the volume you are deleting and check the snapshot status. For more information, go to
* DescribeSnapshots in the Amazon Elastic Compute Cloud API Reference.
*
*
* In the request, you must provide the Amazon Resource Name (ARN) of the storage volume you want to delete.
*
*
* @param deleteVolumeRequest
* A JSON object containing the DeleteVolumeInput$VolumeARN to delete.
* @return A Java Future containing the result of the DeleteVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.DeleteVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVolumeAsync(DeleteVolumeRequest deleteVolumeRequest);
/**
*
* Deletes the specified storage volume that you previously created using the CreateCachediSCSIVolume or
* CreateStorediSCSIVolume API. This operation is only supported in the cached volume and stored volume
* types. For stored volume gateways, the local disk that was configured as the storage volume is not deleted. You
* can reuse the local disk to create another storage volume.
*
*
* Before you delete a volume, make sure there are no iSCSI connections to the volume you are deleting. You should
* also make sure there is no snapshot in progress. You can use the Amazon Elastic Compute Cloud (Amazon EC2) API to
* query snapshots on the volume you are deleting and check the snapshot status. For more information, go to
* DescribeSnapshots in the Amazon Elastic Compute Cloud API Reference.
*
*
* In the request, you must provide the Amazon Resource Name (ARN) of the storage volume you want to delete.
*
*
* @param deleteVolumeRequest
* A JSON object containing the DeleteVolumeInput$VolumeARN to delete.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DeleteVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVolumeAsync(DeleteVolumeRequest deleteVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the most recent high availability monitoring test that was performed on the host in a
* cluster. If a test isn't performed, the status and start time in the response would be null.
*
*
* @param describeAvailabilityMonitorTestRequest
* @return A Java Future containing the result of the DescribeAvailabilityMonitorTest operation returned by the
* service.
* @sample AWSStorageGatewayAsync.DescribeAvailabilityMonitorTest
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAvailabilityMonitorTestAsync(
DescribeAvailabilityMonitorTestRequest describeAvailabilityMonitorTestRequest);
/**
*
* Returns information about the most recent high availability monitoring test that was performed on the host in a
* cluster. If a test isn't performed, the status and start time in the response would be null.
*
*
* @param describeAvailabilityMonitorTestRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAvailabilityMonitorTest operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.DescribeAvailabilityMonitorTest
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAvailabilityMonitorTestAsync(
DescribeAvailabilityMonitorTestRequest describeAvailabilityMonitorTestRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the bandwidth rate limits of a gateway. By default, these limits are not set, which means no bandwidth
* rate limiting is in effect. This operation is supported only for the stored volume, cached volume, and tape
* gateway types. To describe bandwidth rate limits for S3 file gateways, use
* DescribeBandwidthRateLimitSchedule.
*
*
* This operation returns a value for a bandwidth rate limit only if the limit is set. If no limits are set for the
* gateway, then this operation returns only the gateway ARN in the response body. To specify which gateway to
* describe, use the Amazon Resource Name (ARN) of the gateway in your request.
*
*
* @param describeBandwidthRateLimitRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @return A Java Future containing the result of the DescribeBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeBandwidthRateLimit
* @see AWS API Documentation
*/
java.util.concurrent.Future describeBandwidthRateLimitAsync(
DescribeBandwidthRateLimitRequest describeBandwidthRateLimitRequest);
/**
*
* Returns the bandwidth rate limits of a gateway. By default, these limits are not set, which means no bandwidth
* rate limiting is in effect. This operation is supported only for the stored volume, cached volume, and tape
* gateway types. To describe bandwidth rate limits for S3 file gateways, use
* DescribeBandwidthRateLimitSchedule.
*
*
* This operation returns a value for a bandwidth rate limit only if the limit is set. If no limits are set for the
* gateway, then this operation returns only the gateway ARN in the response body. To specify which gateway to
* describe, use the Amazon Resource Name (ARN) of the gateway in your request.
*
*
* @param describeBandwidthRateLimitRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBandwidthRateLimit operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeBandwidthRateLimit
* @see AWS API Documentation
*/
java.util.concurrent.Future describeBandwidthRateLimitAsync(
DescribeBandwidthRateLimitRequest describeBandwidthRateLimitRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the bandwidth rate limit schedule of a gateway. By default, gateways do not have
* bandwidth rate limit schedules, which means no bandwidth rate limiting is in effect. This operation is supported
* only for volume, tape and S3 file gateways. FSx file gateways do not support bandwidth rate limits.
*
*
* This operation returns information about a gateway's bandwidth rate limit schedule. A bandwidth rate limit
* schedule consists of one or more bandwidth rate limit intervals. A bandwidth rate limit interval defines a period
* of time on one or more days of the week, during which bandwidth rate limits are specified for uploading,
* downloading, or both.
*
*
* A bandwidth rate limit interval consists of one or more days of the week, a start hour and minute, an ending hour
* and minute, and bandwidth rate limits for uploading and downloading
*
*
* If no bandwidth rate limit schedule intervals are set for the gateway, this operation returns an empty response.
* To specify which gateway to describe, use the Amazon Resource Name (ARN) of the gateway in your request.
*
*
* @param describeBandwidthRateLimitScheduleRequest
* @return A Java Future containing the result of the DescribeBandwidthRateLimitSchedule operation returned by the
* service.
* @sample AWSStorageGatewayAsync.DescribeBandwidthRateLimitSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeBandwidthRateLimitScheduleAsync(
DescribeBandwidthRateLimitScheduleRequest describeBandwidthRateLimitScheduleRequest);
/**
*
* Returns information about the bandwidth rate limit schedule of a gateway. By default, gateways do not have
* bandwidth rate limit schedules, which means no bandwidth rate limiting is in effect. This operation is supported
* only for volume, tape and S3 file gateways. FSx file gateways do not support bandwidth rate limits.
*
*
* This operation returns information about a gateway's bandwidth rate limit schedule. A bandwidth rate limit
* schedule consists of one or more bandwidth rate limit intervals. A bandwidth rate limit interval defines a period
* of time on one or more days of the week, during which bandwidth rate limits are specified for uploading,
* downloading, or both.
*
*
* A bandwidth rate limit interval consists of one or more days of the week, a start hour and minute, an ending hour
* and minute, and bandwidth rate limits for uploading and downloading
*
*
* If no bandwidth rate limit schedule intervals are set for the gateway, this operation returns an empty response.
* To specify which gateway to describe, use the Amazon Resource Name (ARN) of the gateway in your request.
*
*
* @param describeBandwidthRateLimitScheduleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeBandwidthRateLimitSchedule operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.DescribeBandwidthRateLimitSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeBandwidthRateLimitScheduleAsync(
DescribeBandwidthRateLimitScheduleRequest describeBandwidthRateLimitScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the cache of a gateway. This operation is only supported in the cached volume, tape,
* and file gateway types.
*
*
* The response includes disk IDs that are configured as cache, and it includes the amount of cache allocated and
* used.
*
*
* @param describeCacheRequest
* @return A Java Future containing the result of the DescribeCache operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeCache
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeCacheAsync(DescribeCacheRequest describeCacheRequest);
/**
*
* Returns information about the cache of a gateway. This operation is only supported in the cached volume, tape,
* and file gateway types.
*
*
* The response includes disk IDs that are configured as cache, and it includes the amount of cache allocated and
* used.
*
*
* @param describeCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCache operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeCache
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeCacheAsync(DescribeCacheRequest describeCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the gateway volumes specified in the request. This operation is only supported in the
* cached volume gateway types.
*
*
* The list of gateway volumes in the request must be from one gateway. In the response, Storage Gateway returns
* volume information sorted by volume Amazon Resource Name (ARN).
*
*
* @param describeCachediSCSIVolumesRequest
* @return A Java Future containing the result of the DescribeCachediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeCachediSCSIVolumes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCachediSCSIVolumesAsync(
DescribeCachediSCSIVolumesRequest describeCachediSCSIVolumesRequest);
/**
*
* Returns a description of the gateway volumes specified in the request. This operation is only supported in the
* cached volume gateway types.
*
*
* The list of gateway volumes in the request must be from one gateway. In the response, Storage Gateway returns
* volume information sorted by volume Amazon Resource Name (ARN).
*
*
* @param describeCachediSCSIVolumesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCachediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeCachediSCSIVolumes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCachediSCSIVolumesAsync(
DescribeCachediSCSIVolumesRequest describeCachediSCSIVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns an array of Challenge-Handshake Authentication Protocol (CHAP) credentials information for a specified
* iSCSI target, one for each target-initiator pair. This operation is supported in the volume and tape gateway
* types.
*
*
* @param describeChapCredentialsRequest
* A JSON object containing the Amazon Resource Name (ARN) of the iSCSI volume target.
* @return A Java Future containing the result of the DescribeChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeChapCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChapCredentialsAsync(DescribeChapCredentialsRequest describeChapCredentialsRequest);
/**
*
* Returns an array of Challenge-Handshake Authentication Protocol (CHAP) credentials information for a specified
* iSCSI target, one for each target-initiator pair. This operation is supported in the volume and tape gateway
* types.
*
*
* @param describeChapCredentialsRequest
* A JSON object containing the Amazon Resource Name (ARN) of the iSCSI volume target.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChapCredentials operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeChapCredentials
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChapCredentialsAsync(DescribeChapCredentialsRequest describeChapCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the file system association information. This operation is only supported for FSx File Gateways.
*
*
* @param describeFileSystemAssociationsRequest
* @return A Java Future containing the result of the DescribeFileSystemAssociations operation returned by the
* service.
* @sample AWSStorageGatewayAsync.DescribeFileSystemAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFileSystemAssociationsAsync(
DescribeFileSystemAssociationsRequest describeFileSystemAssociationsRequest);
/**
*
* Gets the file system association information. This operation is only supported for FSx File Gateways.
*
*
* @param describeFileSystemAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFileSystemAssociations operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.DescribeFileSystemAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFileSystemAssociationsAsync(
DescribeFileSystemAssociationsRequest describeFileSystemAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns metadata about a gateway such as its name, network interfaces, configured time zone, and the state
* (whether the gateway is running or not). To specify which gateway to describe, use the Amazon Resource Name (ARN)
* of the gateway in your request.
*
*
* @param describeGatewayInformationRequest
* A JSON object containing the ID of the gateway.
* @return A Java Future containing the result of the DescribeGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeGatewayInformation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGatewayInformationAsync(
DescribeGatewayInformationRequest describeGatewayInformationRequest);
/**
*
* Returns metadata about a gateway such as its name, network interfaces, configured time zone, and the state
* (whether the gateway is running or not). To specify which gateway to describe, use the Amazon Resource Name (ARN)
* of the gateway in your request.
*
*
* @param describeGatewayInformationRequest
* A JSON object containing the ID of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeGatewayInformation operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeGatewayInformation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeGatewayInformationAsync(
DescribeGatewayInformationRequest describeGatewayInformationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns your gateway's weekly maintenance start time including the day and time of the week. Note that values are
* in terms of the gateway's time zone.
*
*
* @param describeMaintenanceStartTimeRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @return A Java Future containing the result of the DescribeMaintenanceStartTime operation returned by the
* service.
* @sample AWSStorageGatewayAsync.DescribeMaintenanceStartTime
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceStartTimeAsync(
DescribeMaintenanceStartTimeRequest describeMaintenanceStartTimeRequest);
/**
*
* Returns your gateway's weekly maintenance start time including the day and time of the week. Note that values are
* in terms of the gateway's time zone.
*
*
* @param describeMaintenanceStartTimeRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMaintenanceStartTime operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.DescribeMaintenanceStartTime
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMaintenanceStartTimeAsync(
DescribeMaintenanceStartTimeRequest describeMaintenanceStartTimeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a description for one or more Network File System (NFS) file shares from an S3 File Gateway. This operation
* is only supported for S3 File Gateways.
*
*
* @param describeNFSFileSharesRequest
* DescribeNFSFileSharesInput
* @return A Java Future containing the result of the DescribeNFSFileShares operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeNFSFileShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNFSFileSharesAsync(DescribeNFSFileSharesRequest describeNFSFileSharesRequest);
/**
*
* Gets a description for one or more Network File System (NFS) file shares from an S3 File Gateway. This operation
* is only supported for S3 File Gateways.
*
*
* @param describeNFSFileSharesRequest
* DescribeNFSFileSharesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeNFSFileShares operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeNFSFileShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describeNFSFileSharesAsync(DescribeNFSFileSharesRequest describeNFSFileSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a description for one or more Server Message Block (SMB) file shares from a S3 File Gateway. This operation
* is only supported for S3 File Gateways.
*
*
* @param describeSMBFileSharesRequest
* DescribeSMBFileSharesInput
* @return A Java Future containing the result of the DescribeSMBFileShares operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeSMBFileShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSMBFileSharesAsync(DescribeSMBFileSharesRequest describeSMBFileSharesRequest);
/**
*
* Gets a description for one or more Server Message Block (SMB) file shares from a S3 File Gateway. This operation
* is only supported for S3 File Gateways.
*
*
* @param describeSMBFileSharesRequest
* DescribeSMBFileSharesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSMBFileShares operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeSMBFileShares
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSMBFileSharesAsync(DescribeSMBFileSharesRequest describeSMBFileSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a description of a Server Message Block (SMB) file share settings from a file gateway. This operation is
* only supported for file gateways.
*
*
* @param describeSMBSettingsRequest
* @return A Java Future containing the result of the DescribeSMBSettings operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeSMBSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSMBSettingsAsync(DescribeSMBSettingsRequest describeSMBSettingsRequest);
/**
*
* Gets a description of a Server Message Block (SMB) file share settings from a file gateway. This operation is
* only supported for file gateways.
*
*
* @param describeSMBSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSMBSettings operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeSMBSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSMBSettingsAsync(DescribeSMBSettingsRequest describeSMBSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the snapshot schedule for the specified gateway volume. The snapshot schedule information includes
* intervals at which snapshots are automatically initiated on the volume. This operation is only supported in the
* cached volume and stored volume types.
*
*
* @param describeSnapshotScheduleRequest
* A JSON object containing the DescribeSnapshotScheduleInput$VolumeARN of the volume.
* @return A Java Future containing the result of the DescribeSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSnapshotScheduleAsync(DescribeSnapshotScheduleRequest describeSnapshotScheduleRequest);
/**
*
* Describes the snapshot schedule for the specified gateway volume. The snapshot schedule information includes
* intervals at which snapshots are automatically initiated on the volume. This operation is only supported in the
* cached volume and stored volume types.
*
*
* @param describeSnapshotScheduleRequest
* A JSON object containing the DescribeSnapshotScheduleInput$VolumeARN of the volume.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSnapshotSchedule operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeSnapshotSchedule
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSnapshotScheduleAsync(DescribeSnapshotScheduleRequest describeSnapshotScheduleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of the gateway volumes specified in the request. The list of gateway volumes in the
* request must be from one gateway. In the response, Storage Gateway returns volume information sorted by volume
* ARNs. This operation is only supported in stored volume gateway type.
*
*
* @param describeStorediSCSIVolumesRequest
* A JSON object containing a list of DescribeStorediSCSIVolumesInput$VolumeARNs.
* @return A Java Future containing the result of the DescribeStorediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeStorediSCSIVolumes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStorediSCSIVolumesAsync(
DescribeStorediSCSIVolumesRequest describeStorediSCSIVolumesRequest);
/**
*
* Returns the description of the gateway volumes specified in the request. The list of gateway volumes in the
* request must be from one gateway. In the response, Storage Gateway returns volume information sorted by volume
* ARNs. This operation is only supported in stored volume gateway type.
*
*
* @param describeStorediSCSIVolumesRequest
* A JSON object containing a list of DescribeStorediSCSIVolumesInput$VolumeARNs.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStorediSCSIVolumes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeStorediSCSIVolumes
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStorediSCSIVolumesAsync(
DescribeStorediSCSIVolumesRequest describeStorediSCSIVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of specified virtual tapes in the virtual tape shelf (VTS). This operation is only
* supported in the tape gateway type.
*
*
* If a specific TapeARN
is not specified, Storage Gateway returns a description of all virtual tapes
* found in the VTS associated with your account.
*
*
* @param describeTapeArchivesRequest
* DescribeTapeArchivesInput
* @return A Java Future containing the result of the DescribeTapeArchives operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapeArchives
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTapeArchivesAsync(DescribeTapeArchivesRequest describeTapeArchivesRequest);
/**
*
* Returns a description of specified virtual tapes in the virtual tape shelf (VTS). This operation is only
* supported in the tape gateway type.
*
*
* If a specific TapeARN
is not specified, Storage Gateway returns a description of all virtual tapes
* found in the VTS associated with your account.
*
*
* @param describeTapeArchivesRequest
* DescribeTapeArchivesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTapeArchives operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapeArchives
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTapeArchivesAsync(DescribeTapeArchivesRequest describeTapeArchivesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeTapeArchives operation.
*
* @see #describeTapeArchivesAsync(DescribeTapeArchivesRequest)
*/
java.util.concurrent.Future describeTapeArchivesAsync();
/**
* Simplified method form for invoking the DescribeTapeArchives operation with an AsyncHandler.
*
* @see #describeTapeArchivesAsync(DescribeTapeArchivesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeTapeArchivesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of virtual tape recovery points that are available for the specified tape gateway.
*
*
* A recovery point is a point-in-time view of a virtual tape at which all the data on the virtual tape is
* consistent. If your gateway crashes, virtual tapes that have recovery points can be recovered to a new gateway.
* This operation is only supported in the tape gateway type.
*
*
* @param describeTapeRecoveryPointsRequest
* DescribeTapeRecoveryPointsInput
* @return A Java Future containing the result of the DescribeTapeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapeRecoveryPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTapeRecoveryPointsAsync(
DescribeTapeRecoveryPointsRequest describeTapeRecoveryPointsRequest);
/**
*
* Returns a list of virtual tape recovery points that are available for the specified tape gateway.
*
*
* A recovery point is a point-in-time view of a virtual tape at which all the data on the virtual tape is
* consistent. If your gateway crashes, virtual tapes that have recovery points can be recovered to a new gateway.
* This operation is only supported in the tape gateway type.
*
*
* @param describeTapeRecoveryPointsRequest
* DescribeTapeRecoveryPointsInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTapeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapeRecoveryPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTapeRecoveryPointsAsync(
DescribeTapeRecoveryPointsRequest describeTapeRecoveryPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the specified Amazon Resource Name (ARN) of virtual tapes. If a TapeARN
is
* not specified, returns a description of all virtual tapes associated with the specified gateway. This operation
* is only supported in the tape gateway type.
*
*
* @param describeTapesRequest
* DescribeTapesInput
* @return A Java Future containing the result of the DescribeTapes operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeTapes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTapesAsync(DescribeTapesRequest describeTapesRequest);
/**
*
* Returns a description of the specified Amazon Resource Name (ARN) of virtual tapes. If a TapeARN
is
* not specified, returns a description of all virtual tapes associated with the specified gateway. This operation
* is only supported in the tape gateway type.
*
*
* @param describeTapesRequest
* DescribeTapesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTapes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeTapes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTapesAsync(DescribeTapesRequest describeTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the upload buffer of a gateway. This operation is supported for the stored volume,
* cached volume, and tape gateway types.
*
*
* The response includes disk IDs that are configured as upload buffer space, and it includes the amount of upload
* buffer space allocated and used.
*
*
* @param describeUploadBufferRequest
* @return A Java Future containing the result of the DescribeUploadBuffer operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeUploadBuffer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUploadBufferAsync(DescribeUploadBufferRequest describeUploadBufferRequest);
/**
*
* Returns information about the upload buffer of a gateway. This operation is supported for the stored volume,
* cached volume, and tape gateway types.
*
*
* The response includes disk IDs that are configured as upload buffer space, and it includes the amount of upload
* buffer space allocated and used.
*
*
* @param describeUploadBufferRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeUploadBuffer operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeUploadBuffer
* @see AWS API Documentation
*/
java.util.concurrent.Future describeUploadBufferAsync(DescribeUploadBufferRequest describeUploadBufferRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of virtual tape library (VTL) devices for the specified tape gateway. In the response,
* Storage Gateway returns VTL device information.
*
*
* This operation is only supported in the tape gateway type.
*
*
* @param describeVTLDevicesRequest
* DescribeVTLDevicesInput
* @return A Java Future containing the result of the DescribeVTLDevices operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeVTLDevices
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVTLDevicesAsync(DescribeVTLDevicesRequest describeVTLDevicesRequest);
/**
*
* Returns a description of virtual tape library (VTL) devices for the specified tape gateway. In the response,
* Storage Gateway returns VTL device information.
*
*
* This operation is only supported in the tape gateway type.
*
*
* @param describeVTLDevicesRequest
* DescribeVTLDevicesInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeVTLDevices operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeVTLDevices
* @see AWS API Documentation
*/
java.util.concurrent.Future describeVTLDevicesAsync(DescribeVTLDevicesRequest describeVTLDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the working storage of a gateway. This operation is only supported in the stored
* volumes gateway type. This operation is deprecated in cached volumes API version (20120630). Use
* DescribeUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use the DescribeUploadBuffer operation to add
* upload buffer to a stored volume gateway.
*
*
*
* The response includes disk IDs that are configured as working storage, and it includes the amount of working
* storage allocated and used.
*
*
* @param describeWorkingStorageRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @return A Java Future containing the result of the DescribeWorkingStorage operation returned by the service.
* @sample AWSStorageGatewayAsync.DescribeWorkingStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkingStorageAsync(DescribeWorkingStorageRequest describeWorkingStorageRequest);
/**
*
* Returns information about the working storage of a gateway. This operation is only supported in the stored
* volumes gateway type. This operation is deprecated in cached volumes API version (20120630). Use
* DescribeUploadBuffer instead.
*
*
*
* Working storage is also referred to as upload buffer. You can also use the DescribeUploadBuffer operation to add
* upload buffer to a stored volume gateway.
*
*
*
* The response includes disk IDs that are configured as working storage, and it includes the amount of working
* storage allocated and used.
*
*
* @param describeWorkingStorageRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeWorkingStorage operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DescribeWorkingStorage
* @see AWS API Documentation
*/
java.util.concurrent.Future describeWorkingStorageAsync(DescribeWorkingStorageRequest describeWorkingStorageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disconnects a volume from an iSCSI connection and then detaches the volume from the specified gateway. Detaching
* and attaching a volume enables you to recover your data from one gateway to a different gateway without creating
* a snapshot. It also makes it easier to move your volumes from an on-premises gateway to a gateway hosted on an
* Amazon EC2 instance. This operation is only supported in the volume gateway type.
*
*
* @param detachVolumeRequest
* AttachVolumeInput
* @return A Java Future containing the result of the DetachVolume operation returned by the service.
* @sample AWSStorageGatewayAsync.DetachVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future detachVolumeAsync(DetachVolumeRequest detachVolumeRequest);
/**
*
* Disconnects a volume from an iSCSI connection and then detaches the volume from the specified gateway. Detaching
* and attaching a volume enables you to recover your data from one gateway to a different gateway without creating
* a snapshot. It also makes it easier to move your volumes from an on-premises gateway to a gateway hosted on an
* Amazon EC2 instance. This operation is only supported in the volume gateway type.
*
*
* @param detachVolumeRequest
* AttachVolumeInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetachVolume operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DetachVolume
* @see AWS
* API Documentation
*/
java.util.concurrent.Future detachVolumeAsync(DetachVolumeRequest detachVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disables a tape gateway when the gateway is no longer functioning. For example, if your gateway VM is damaged,
* you can disable the gateway so you can recover virtual tapes.
*
*
* Use this operation for a tape gateway that is not reachable or not functioning. This operation is only supported
* in the tape gateway type.
*
*
*
* After a gateway is disabled, it cannot be enabled.
*
*
*
* @param disableGatewayRequest
* DisableGatewayInput
* @return A Java Future containing the result of the DisableGateway operation returned by the service.
* @sample AWSStorageGatewayAsync.DisableGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disableGatewayAsync(DisableGatewayRequest disableGatewayRequest);
/**
*
* Disables a tape gateway when the gateway is no longer functioning. For example, if your gateway VM is damaged,
* you can disable the gateway so you can recover virtual tapes.
*
*
* Use this operation for a tape gateway that is not reachable or not functioning. This operation is only supported
* in the tape gateway type.
*
*
*
* After a gateway is disabled, it cannot be enabled.
*
*
*
* @param disableGatewayRequest
* DisableGatewayInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisableGateway operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DisableGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future disableGatewayAsync(DisableGatewayRequest disableGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates an Amazon FSx file system from the specified gateway. After the disassociation process finishes,
* the gateway can no longer access the Amazon FSx file system. This operation is only supported in the FSx File
* Gateway type.
*
*
* @param disassociateFileSystemRequest
* @return A Java Future containing the result of the DisassociateFileSystem operation returned by the service.
* @sample AWSStorageGatewayAsync.DisassociateFileSystem
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFileSystemAsync(DisassociateFileSystemRequest disassociateFileSystemRequest);
/**
*
* Disassociates an Amazon FSx file system from the specified gateway. After the disassociation process finishes,
* the gateway can no longer access the Amazon FSx file system. This operation is only supported in the FSx File
* Gateway type.
*
*
* @param disassociateFileSystemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateFileSystem operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.DisassociateFileSystem
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateFileSystemAsync(DisassociateFileSystemRequest disassociateFileSystemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a file gateway to an Active Directory domain. This operation is only supported for file gateways that
* support the SMB file protocol.
*
*
* @param joinDomainRequest
* JoinDomainInput
* @return A Java Future containing the result of the JoinDomain operation returned by the service.
* @sample AWSStorageGatewayAsync.JoinDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future joinDomainAsync(JoinDomainRequest joinDomainRequest);
/**
*
* Adds a file gateway to an Active Directory domain. This operation is only supported for file gateways that
* support the SMB file protocol.
*
*
* @param joinDomainRequest
* JoinDomainInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the JoinDomain operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.JoinDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future joinDomainAsync(JoinDomainRequest joinDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the automatic tape creation policies for a gateway. If there are no automatic tape creation policies for
* the gateway, it returns an empty list.
*
*
* This operation is only supported for tape gateways.
*
*
* @param listAutomaticTapeCreationPoliciesRequest
* @return A Java Future containing the result of the ListAutomaticTapeCreationPolicies operation returned by the
* service.
* @sample AWSStorageGatewayAsync.ListAutomaticTapeCreationPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listAutomaticTapeCreationPoliciesAsync(
ListAutomaticTapeCreationPoliciesRequest listAutomaticTapeCreationPoliciesRequest);
/**
*
* Lists the automatic tape creation policies for a gateway. If there are no automatic tape creation policies for
* the gateway, it returns an empty list.
*
*
* This operation is only supported for tape gateways.
*
*
* @param listAutomaticTapeCreationPoliciesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAutomaticTapeCreationPolicies operation returned by the
* service.
* @sample AWSStorageGatewayAsyncHandler.ListAutomaticTapeCreationPolicies
* @see AWS API Documentation
*/
java.util.concurrent.Future listAutomaticTapeCreationPoliciesAsync(
ListAutomaticTapeCreationPoliciesRequest listAutomaticTapeCreationPoliciesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of the file shares for a specific S3 File Gateway, or the list of file shares that belong to the
* calling user account. This operation is only supported for S3 File Gateways.
*
*
* @param listFileSharesRequest
* ListFileShareInput
* @return A Java Future containing the result of the ListFileShares operation returned by the service.
* @sample AWSStorageGatewayAsync.ListFileShares
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFileSharesAsync(ListFileSharesRequest listFileSharesRequest);
/**
*
* Gets a list of the file shares for a specific S3 File Gateway, or the list of file shares that belong to the
* calling user account. This operation is only supported for S3 File Gateways.
*
*
* @param listFileSharesRequest
* ListFileShareInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFileShares operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListFileShares
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listFileSharesAsync(ListFileSharesRequest listFileSharesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of FileSystemAssociationSummary
objects. Each object contains a summary of a file system
* association. This operation is only supported for FSx File Gateways.
*
*
* @param listFileSystemAssociationsRequest
* @return A Java Future containing the result of the ListFileSystemAssociations operation returned by the service.
* @sample AWSStorageGatewayAsync.ListFileSystemAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listFileSystemAssociationsAsync(
ListFileSystemAssociationsRequest listFileSystemAssociationsRequest);
/**
*
* Gets a list of FileSystemAssociationSummary
objects. Each object contains a summary of a file system
* association. This operation is only supported for FSx File Gateways.
*
*
* @param listFileSystemAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFileSystemAssociations operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListFileSystemAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listFileSystemAssociationsAsync(
ListFileSystemAssociationsRequest listFileSystemAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists gateways owned by an Amazon Web Services account in an Amazon Web Services Region specified in the request.
* The returned list is ordered by gateway Amazon Resource Name (ARN).
*
*
* By default, the operation returns a maximum of 100 gateways. This operation supports pagination that allows you
* to optionally reduce the number of gateways returned in a response.
*
*
* If you have more gateways than are returned in a response (that is, the response returns only a truncated list of
* your gateways), the response contains a marker that you can specify in your next request to fetch the next page
* of gateways.
*
*
* @param listGatewaysRequest
* A JSON object containing zero or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* @sample AWSStorageGatewayAsync.ListGateways
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listGatewaysAsync(ListGatewaysRequest listGatewaysRequest);
/**
*
* Lists gateways owned by an Amazon Web Services account in an Amazon Web Services Region specified in the request.
* The returned list is ordered by gateway Amazon Resource Name (ARN).
*
*
* By default, the operation returns a maximum of 100 gateways. This operation supports pagination that allows you
* to optionally reduce the number of gateways returned in a response.
*
*
* If you have more gateways than are returned in a response (that is, the response returns only a truncated list of
* your gateways), the response contains a marker that you can specify in your next request to fetch the next page
* of gateways.
*
*
* @param listGatewaysRequest
* A JSON object containing zero or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListGateways operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListGateways
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listGatewaysAsync(ListGatewaysRequest listGatewaysRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListGateways operation.
*
* @see #listGatewaysAsync(ListGatewaysRequest)
*/
java.util.concurrent.Future listGatewaysAsync();
/**
* Simplified method form for invoking the ListGateways operation with an AsyncHandler.
*
* @see #listGatewaysAsync(ListGatewaysRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listGatewaysAsync(com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the gateway's local disks. To specify which gateway to describe, you use the Amazon Resource
* Name (ARN) of the gateway in the body of the request.
*
*
* The request returns a list of all disks, specifying which are configured as working storage, cache storage, or
* stored volume or not configured at all. The response includes a DiskStatus
field. This field can
* have a value of present (the disk is available to use), missing (the disk is no longer connected to the gateway),
* or mismatch (the disk node is occupied by a disk that has incorrect metadata or the disk content is corrupted).
*
*
* @param listLocalDisksRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @return A Java Future containing the result of the ListLocalDisks operation returned by the service.
* @sample AWSStorageGatewayAsync.ListLocalDisks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLocalDisksAsync(ListLocalDisksRequest listLocalDisksRequest);
/**
*
* Returns a list of the gateway's local disks. To specify which gateway to describe, you use the Amazon Resource
* Name (ARN) of the gateway in the body of the request.
*
*
* The request returns a list of all disks, specifying which are configured as working storage, cache storage, or
* stored volume or not configured at all. The response includes a DiskStatus
field. This field can
* have a value of present (the disk is available to use), missing (the disk is no longer connected to the gateway),
* or mismatch (the disk node is occupied by a disk that has incorrect metadata or the disk content is corrupted).
*
*
* @param listLocalDisksRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLocalDisks operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListLocalDisks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLocalDisksAsync(ListLocalDisksRequest listLocalDisksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags that have been added to the specified resource. This operation is supported in storage gateways of
* all types.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceInput
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSStorageGatewayAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags that have been added to the specified resource. This operation is supported in storage gateways of
* all types.
*
*
* @param listTagsForResourceRequest
* ListTagsForResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListTagsForResource operation.
*
* @see #listTagsForResourceAsync(ListTagsForResourceRequest)
*/
java.util.concurrent.Future listTagsForResourceAsync();
/**
* Simplified method form for invoking the ListTagsForResource operation with an AsyncHandler.
*
* @see #listTagsForResourceAsync(ListTagsForResourceRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listTagsForResourceAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists custom tape pools. You specify custom tape pools to list by specifying one or more custom tape pool Amazon
* Resource Names (ARNs). If you don't specify a custom tape pool ARN, the operation lists all custom tape pools.
*
*
* This operation supports pagination. You can optionally specify the Limit
parameter in the body to
* limit the number of tape pools in the response. If the number of tape pools returned in the response is
* truncated, the response includes a Marker
element that you can use in your subsequent request to
* retrieve the next set of tape pools.
*
*
* @param listTapePoolsRequest
* @return A Java Future containing the result of the ListTapePools operation returned by the service.
* @sample AWSStorageGatewayAsync.ListTapePools
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTapePoolsAsync(ListTapePoolsRequest listTapePoolsRequest);
/**
*
* Lists custom tape pools. You specify custom tape pools to list by specifying one or more custom tape pool Amazon
* Resource Names (ARNs). If you don't specify a custom tape pool ARN, the operation lists all custom tape pools.
*
*
* This operation supports pagination. You can optionally specify the Limit
parameter in the body to
* limit the number of tape pools in the response. If the number of tape pools returned in the response is
* truncated, the response includes a Marker
element that you can use in your subsequent request to
* retrieve the next set of tape pools.
*
*
* @param listTapePoolsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTapePools operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListTapePools
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTapePoolsAsync(ListTapePoolsRequest listTapePoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists virtual tapes in your virtual tape library (VTL) and your virtual tape shelf (VTS). You specify the tapes
* to list by specifying one or more tape Amazon Resource Names (ARNs). If you don't specify a tape ARN, the
* operation lists all virtual tapes in both your VTL and VTS.
*
*
* This operation supports pagination. By default, the operation returns a maximum of up to 100 tapes. You can
* optionally specify the Limit
parameter in the body to limit the number of tapes in the response. If
* the number of tapes returned in the response is truncated, the response includes a Marker
element
* that you can use in your subsequent request to retrieve the next set of tapes. This operation is only supported
* in the tape gateway type.
*
*
* @param listTapesRequest
* A JSON object that contains one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListTapes operation returned by the service.
* @sample AWSStorageGatewayAsync.ListTapes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTapesAsync(ListTapesRequest listTapesRequest);
/**
*
* Lists virtual tapes in your virtual tape library (VTL) and your virtual tape shelf (VTS). You specify the tapes
* to list by specifying one or more tape Amazon Resource Names (ARNs). If you don't specify a tape ARN, the
* operation lists all virtual tapes in both your VTL and VTS.
*
*
* This operation supports pagination. By default, the operation returns a maximum of up to 100 tapes. You can
* optionally specify the Limit
parameter in the body to limit the number of tapes in the response. If
* the number of tapes returned in the response is truncated, the response includes a Marker
element
* that you can use in your subsequent request to retrieve the next set of tapes. This operation is only supported
* in the tape gateway type.
*
*
* @param listTapesRequest
* A JSON object that contains one or more of the following fields:
*
* -
*
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTapes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListTapes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTapesAsync(ListTapesRequest listTapesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists iSCSI initiators that are connected to a volume. You can use this operation to determine whether a volume
* is being used or not. This operation is only supported in the cached volume and stored volume gateway types.
*
*
* @param listVolumeInitiatorsRequest
* ListVolumeInitiatorsInput
* @return A Java Future containing the result of the ListVolumeInitiators operation returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumeInitiators
* @see AWS API Documentation
*/
java.util.concurrent.Future listVolumeInitiatorsAsync(ListVolumeInitiatorsRequest listVolumeInitiatorsRequest);
/**
*
* Lists iSCSI initiators that are connected to a volume. You can use this operation to determine whether a volume
* is being used or not. This operation is only supported in the cached volume and stored volume gateway types.
*
*
* @param listVolumeInitiatorsRequest
* ListVolumeInitiatorsInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVolumeInitiators operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumeInitiators
* @see AWS API Documentation
*/
java.util.concurrent.Future listVolumeInitiatorsAsync(ListVolumeInitiatorsRequest listVolumeInitiatorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the recovery points for a specified gateway. This operation is only supported in the cached volume gateway
* type.
*
*
* Each cache volume has one recovery point. A volume recovery point is a point in time at which all data of the
* volume is consistent and from which you can create a snapshot or clone a new cached volume from a source volume.
* To create a snapshot from a volume recovery point use the CreateSnapshotFromVolumeRecoveryPoint operation.
*
*
* @param listVolumeRecoveryPointsRequest
* @return A Java Future containing the result of the ListVolumeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumeRecoveryPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listVolumeRecoveryPointsAsync(ListVolumeRecoveryPointsRequest listVolumeRecoveryPointsRequest);
/**
*
* Lists the recovery points for a specified gateway. This operation is only supported in the cached volume gateway
* type.
*
*
* Each cache volume has one recovery point. A volume recovery point is a point in time at which all data of the
* volume is consistent and from which you can create a snapshot or clone a new cached volume from a source volume.
* To create a snapshot from a volume recovery point use the CreateSnapshotFromVolumeRecoveryPoint operation.
*
*
* @param listVolumeRecoveryPointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVolumeRecoveryPoints operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumeRecoveryPoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listVolumeRecoveryPointsAsync(ListVolumeRecoveryPointsRequest listVolumeRecoveryPointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the iSCSI stored volumes of a gateway. Results are sorted by volume ARN. The response includes only the
* volume ARNs. If you want additional volume information, use the DescribeStorediSCSIVolumes or the
* DescribeCachediSCSIVolumes API.
*
*
* The operation supports pagination. By default, the operation returns a maximum of up to 100 volumes. You can
* optionally specify the Limit
field in the body to limit the number of volumes in the response. If
* the number of volumes returned in the response is truncated, the response includes a Marker field. You can use
* this Marker value in your subsequent request to retrieve the next set of volumes. This operation is only
* supported in the cached volume and stored volume gateway types.
*
*
* @param listVolumesRequest
* A JSON object that contains one or more of the following fields:
*
* -
*
*
* -
*
*
* @return A Java Future containing the result of the ListVolumes operation returned by the service.
* @sample AWSStorageGatewayAsync.ListVolumes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVolumesAsync(ListVolumesRequest listVolumesRequest);
/**
*
* Lists the iSCSI stored volumes of a gateway. Results are sorted by volume ARN. The response includes only the
* volume ARNs. If you want additional volume information, use the DescribeStorediSCSIVolumes or the
* DescribeCachediSCSIVolumes API.
*
*
* The operation supports pagination. By default, the operation returns a maximum of up to 100 volumes. You can
* optionally specify the Limit
field in the body to limit the number of volumes in the response. If
* the number of volumes returned in the response is truncated, the response includes a Marker field. You can use
* this Marker value in your subsequent request to retrieve the next set of volumes. This operation is only
* supported in the cached volume and stored volume gateway types.
*
*
* @param listVolumesRequest
* A JSON object that contains one or more of the following fields:
*
* -
*
*
* -
*
*
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVolumes operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ListVolumes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listVolumesAsync(ListVolumesRequest listVolumesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends you notification through CloudWatch Events when all files written to your file share have been uploaded to
* S3. Amazon S3.
*
*
* Storage Gateway can send a notification through Amazon CloudWatch Events when all files written to your file
* share up to that point in time have been uploaded to Amazon S3. These files include files written to the file
* share up to the time that you make a request for notification. When the upload is done, Storage Gateway sends you
* notification through an Amazon CloudWatch Event. You can configure CloudWatch Events to send the notification
* through event targets such as Amazon SNS or Lambda function. This operation is only supported for S3 File
* Gateways.
*
*
* For more information, see Getting file upload notification in the Storage Gateway User Guide.
*
*
* @param notifyWhenUploadedRequest
* @return A Java Future containing the result of the NotifyWhenUploaded operation returned by the service.
* @sample AWSStorageGatewayAsync.NotifyWhenUploaded
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyWhenUploadedAsync(NotifyWhenUploadedRequest notifyWhenUploadedRequest);
/**
*
* Sends you notification through CloudWatch Events when all files written to your file share have been uploaded to
* S3. Amazon S3.
*
*
* Storage Gateway can send a notification through Amazon CloudWatch Events when all files written to your file
* share up to that point in time have been uploaded to Amazon S3. These files include files written to the file
* share up to the time that you make a request for notification. When the upload is done, Storage Gateway sends you
* notification through an Amazon CloudWatch Event. You can configure CloudWatch Events to send the notification
* through event targets such as Amazon SNS or Lambda function. This operation is only supported for S3 File
* Gateways.
*
*
* For more information, see Getting file upload notification in the Storage Gateway User Guide.
*
*
* @param notifyWhenUploadedRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the NotifyWhenUploaded operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.NotifyWhenUploaded
* @see AWS API Documentation
*/
java.util.concurrent.Future notifyWhenUploadedAsync(NotifyWhenUploadedRequest notifyWhenUploadedRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Refreshes the cached inventory of objects for the specified file share. This operation finds objects in the
* Amazon S3 bucket that were added, removed, or replaced since the gateway last listed the bucket's contents and
* cached the results. This operation does not import files into the S3 File Gateway cache storage. It only updates
* the cached inventory to reflect changes in the inventory of the objects in the S3 bucket. This operation is only
* supported in the S3 File Gateway types.
*
*
* You can subscribe to be notified through an Amazon CloudWatch event when your RefreshCache
operation
* completes. For more information, see Getting notified about file operations in the Storage Gateway User Guide. This operation is Only
* supported for S3 File Gateways.
*
*
* When this API is called, it only initiates the refresh operation. When the API call completes and returns a
* success code, it doesn't necessarily mean that the file refresh has completed. You should use the
* refresh-complete notification to determine that the operation has completed before you check for new files on the
* gateway file share. You can subscribe to be notified through a CloudWatch event when your
* RefreshCache
operation completes.
*
*
* Throttle limit: This API is asynchronous, so the gateway will accept no more than two refreshes at any time. We
* recommend using the refresh-complete CloudWatch event notification before issuing additional requests. For more
* information, see Getting notified about file operations in the Storage Gateway User Guide.
*
*
*
* -
*
* Wait at least 60 seconds between consecutive RefreshCache API requests.
*
*
* -
*
* RefreshCache does not evict cache entries if invoked consecutively within 60 seconds of a previous RefreshCache
* request.
*
*
* -
*
* If you invoke the RefreshCache API when two requests are already being processed, any new request will cause an
* InvalidGatewayRequestException
error because too many requests were sent to the server.
*
*
*
*
*
* The S3 bucket name does not need to be included when entering the list of folders in the FolderList parameter.
*
*
*
* For more information, see Getting notified about file operations in the Storage Gateway User Guide.
*
*
* @param refreshCacheRequest
* RefreshCacheInput
* @return A Java Future containing the result of the RefreshCache operation returned by the service.
* @sample AWSStorageGatewayAsync.RefreshCache
* @see AWS
* API Documentation
*/
java.util.concurrent.Future refreshCacheAsync(RefreshCacheRequest refreshCacheRequest);
/**
*
* Refreshes the cached inventory of objects for the specified file share. This operation finds objects in the
* Amazon S3 bucket that were added, removed, or replaced since the gateway last listed the bucket's contents and
* cached the results. This operation does not import files into the S3 File Gateway cache storage. It only updates
* the cached inventory to reflect changes in the inventory of the objects in the S3 bucket. This operation is only
* supported in the S3 File Gateway types.
*
*
* You can subscribe to be notified through an Amazon CloudWatch event when your RefreshCache
operation
* completes. For more information, see Getting notified about file operations in the Storage Gateway User Guide. This operation is Only
* supported for S3 File Gateways.
*
*
* When this API is called, it only initiates the refresh operation. When the API call completes and returns a
* success code, it doesn't necessarily mean that the file refresh has completed. You should use the
* refresh-complete notification to determine that the operation has completed before you check for new files on the
* gateway file share. You can subscribe to be notified through a CloudWatch event when your
* RefreshCache
operation completes.
*
*
* Throttle limit: This API is asynchronous, so the gateway will accept no more than two refreshes at any time. We
* recommend using the refresh-complete CloudWatch event notification before issuing additional requests. For more
* information, see Getting notified about file operations in the Storage Gateway User Guide.
*
*
*
* -
*
* Wait at least 60 seconds between consecutive RefreshCache API requests.
*
*
* -
*
* RefreshCache does not evict cache entries if invoked consecutively within 60 seconds of a previous RefreshCache
* request.
*
*
* -
*
* If you invoke the RefreshCache API when two requests are already being processed, any new request will cause an
* InvalidGatewayRequestException
error because too many requests were sent to the server.
*
*
*
*
*
* The S3 bucket name does not need to be included when entering the list of folders in the FolderList parameter.
*
*
*
* For more information, see Getting notified about file operations in the Storage Gateway User Guide.
*
*
* @param refreshCacheRequest
* RefreshCacheInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RefreshCache operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RefreshCache
* @see AWS
* API Documentation
*/
java.util.concurrent.Future refreshCacheAsync(RefreshCacheRequest refreshCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes one or more tags from the specified resource. This operation is supported in storage gateways of all
* types.
*
*
* @param removeTagsFromResourceRequest
* RemoveTagsFromResourceInput
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSStorageGatewayAsync.RemoveTagsFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Removes one or more tags from the specified resource. This operation is supported in storage gateways of all
* types.
*
*
* @param removeTagsFromResourceRequest
* RemoveTagsFromResourceInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RemoveTagsFromResource
* @see AWS API Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the RemoveTagsFromResource operation.
*
* @see #removeTagsFromResourceAsync(RemoveTagsFromResourceRequest)
*/
java.util.concurrent.Future removeTagsFromResourceAsync();
/**
* Simplified method form for invoking the RemoveTagsFromResource operation with an AsyncHandler.
*
* @see #removeTagsFromResourceAsync(RemoveTagsFromResourceRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future removeTagsFromResourceAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resets all cache disks that have encountered an error and makes the disks available for reconfiguration as cache
* storage. If your cache disk encounters an error, the gateway prevents read and write operations on virtual tapes
* in the gateway. For example, an error can occur when a disk is corrupted or removed from the gateway. When a
* cache is reset, the gateway loses its cache storage. At this point, you can reconfigure the disks as cache disks.
* This operation is only supported in the cached volume and tape types.
*
*
*
* If the cache disk you are resetting contains data that has not been uploaded to Amazon S3 yet, that data can be
* lost. After you reset cache disks, there will be no configured cache disks left in the gateway, so you must
* configure at least one new cache disk for your gateway to function properly.
*
*
*
* @param resetCacheRequest
* @return A Java Future containing the result of the ResetCache operation returned by the service.
* @sample AWSStorageGatewayAsync.ResetCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetCacheAsync(ResetCacheRequest resetCacheRequest);
/**
*
* Resets all cache disks that have encountered an error and makes the disks available for reconfiguration as cache
* storage. If your cache disk encounters an error, the gateway prevents read and write operations on virtual tapes
* in the gateway. For example, an error can occur when a disk is corrupted or removed from the gateway. When a
* cache is reset, the gateway loses its cache storage. At this point, you can reconfigure the disks as cache disks.
* This operation is only supported in the cached volume and tape types.
*
*
*
* If the cache disk you are resetting contains data that has not been uploaded to Amazon S3 yet, that data can be
* lost. After you reset cache disks, there will be no configured cache disks left in the gateway, so you must
* configure at least one new cache disk for your gateway to function properly.
*
*
*
* @param resetCacheRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetCache operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ResetCache
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetCacheAsync(ResetCacheRequest resetCacheRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves an archived virtual tape from the virtual tape shelf (VTS) to a tape gateway. Virtual tapes archived in
* the VTS are not associated with any gateway. However after a tape is retrieved, it is associated with a gateway,
* even though it is also listed in the VTS, that is, archive. This operation is only supported in the tape gateway
* type.
*
*
* Once a tape is successfully retrieved to a gateway, it cannot be retrieved again to another gateway. You must
* archive the tape again before you can retrieve it to another gateway. This operation is only supported in the
* tape gateway type.
*
*
* @param retrieveTapeArchiveRequest
* RetrieveTapeArchiveInput
* @return A Java Future containing the result of the RetrieveTapeArchive operation returned by the service.
* @sample AWSStorageGatewayAsync.RetrieveTapeArchive
* @see AWS API Documentation
*/
java.util.concurrent.Future retrieveTapeArchiveAsync(RetrieveTapeArchiveRequest retrieveTapeArchiveRequest);
/**
*
* Retrieves an archived virtual tape from the virtual tape shelf (VTS) to a tape gateway. Virtual tapes archived in
* the VTS are not associated with any gateway. However after a tape is retrieved, it is associated with a gateway,
* even though it is also listed in the VTS, that is, archive. This operation is only supported in the tape gateway
* type.
*
*
* Once a tape is successfully retrieved to a gateway, it cannot be retrieved again to another gateway. You must
* archive the tape again before you can retrieve it to another gateway. This operation is only supported in the
* tape gateway type.
*
*
* @param retrieveTapeArchiveRequest
* RetrieveTapeArchiveInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RetrieveTapeArchive operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RetrieveTapeArchive
* @see AWS API Documentation
*/
java.util.concurrent.Future retrieveTapeArchiveAsync(RetrieveTapeArchiveRequest retrieveTapeArchiveRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the recovery point for the specified virtual tape. This operation is only supported in the tape gateway
* type.
*
*
* A recovery point is a point in time view of a virtual tape at which all the data on the tape is consistent. If
* your gateway crashes, virtual tapes that have recovery points can be recovered to a new gateway.
*
*
*
* The virtual tape can be retrieved to only one gateway. The retrieved tape is read-only. The virtual tape can be
* retrieved to only a tape gateway. There is no charge for retrieving recovery points.
*
*
*
* @param retrieveTapeRecoveryPointRequest
* RetrieveTapeRecoveryPointInput
* @return A Java Future containing the result of the RetrieveTapeRecoveryPoint operation returned by the service.
* @sample AWSStorageGatewayAsync.RetrieveTapeRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future retrieveTapeRecoveryPointAsync(
RetrieveTapeRecoveryPointRequest retrieveTapeRecoveryPointRequest);
/**
*
* Retrieves the recovery point for the specified virtual tape. This operation is only supported in the tape gateway
* type.
*
*
* A recovery point is a point in time view of a virtual tape at which all the data on the tape is consistent. If
* your gateway crashes, virtual tapes that have recovery points can be recovered to a new gateway.
*
*
*
* The virtual tape can be retrieved to only one gateway. The retrieved tape is read-only. The virtual tape can be
* retrieved to only a tape gateway. There is no charge for retrieving recovery points.
*
*
*
* @param retrieveTapeRecoveryPointRequest
* RetrieveTapeRecoveryPointInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RetrieveTapeRecoveryPoint operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.RetrieveTapeRecoveryPoint
* @see AWS API Documentation
*/
java.util.concurrent.Future retrieveTapeRecoveryPointAsync(
RetrieveTapeRecoveryPointRequest retrieveTapeRecoveryPointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the password for your VM local console. When you log in to the local console for the first time, you log in
* to the VM with the default credentials. We recommend that you set a new password. You don't need to know the
* default password to set a new password.
*
*
* @param setLocalConsolePasswordRequest
* SetLocalConsolePasswordInput
* @return A Java Future containing the result of the SetLocalConsolePassword operation returned by the service.
* @sample AWSStorageGatewayAsync.SetLocalConsolePassword
* @see AWS API Documentation
*/
java.util.concurrent.Future setLocalConsolePasswordAsync(SetLocalConsolePasswordRequest setLocalConsolePasswordRequest);
/**
*
* Sets the password for your VM local console. When you log in to the local console for the first time, you log in
* to the VM with the default credentials. We recommend that you set a new password. You don't need to know the
* default password to set a new password.
*
*
* @param setLocalConsolePasswordRequest
* SetLocalConsolePasswordInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetLocalConsolePassword operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.SetLocalConsolePassword
* @see AWS API Documentation
*/
java.util.concurrent.Future setLocalConsolePasswordAsync(SetLocalConsolePasswordRequest setLocalConsolePasswordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the password for the guest user smbguest
. The smbguest
user is the user when the
* authentication method for the file share is set to GuestAccess
. This operation only supported for S3
* File Gateways
*
*
* @param setSMBGuestPasswordRequest
* SetSMBGuestPasswordInput
* @return A Java Future containing the result of the SetSMBGuestPassword operation returned by the service.
* @sample AWSStorageGatewayAsync.SetSMBGuestPassword
* @see AWS API Documentation
*/
java.util.concurrent.Future setSMBGuestPasswordAsync(SetSMBGuestPasswordRequest setSMBGuestPasswordRequest);
/**
*
* Sets the password for the guest user smbguest
. The smbguest
user is the user when the
* authentication method for the file share is set to GuestAccess
. This operation only supported for S3
* File Gateways
*
*
* @param setSMBGuestPasswordRequest
* SetSMBGuestPasswordInput
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetSMBGuestPassword operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.SetSMBGuestPassword
* @see AWS API Documentation
*/
java.util.concurrent.Future setSMBGuestPasswordAsync(SetSMBGuestPasswordRequest setSMBGuestPasswordRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shuts down a gateway. To specify which gateway to shut down, use the Amazon Resource Name (ARN) of the gateway in
* the body of your request.
*
*
* The operation shuts down the gateway service component running in the gateway's virtual machine (VM) and not the
* host VM.
*
*
*
* If you want to shut down the VM, it is recommended that you first shut down the gateway component in the VM to
* avoid unpredictable conditions.
*
*
*
* After the gateway is shutdown, you cannot call any other API except StartGateway,
* DescribeGatewayInformation, and ListGateways. For more information, see ActivateGateway.
* Your applications cannot read from or write to the gateway's storage volumes, and there are no snapshots taken.
*
*
*
* When you make a shutdown request, you will get a 200 OK
success response immediately. However, it
* might take some time for the gateway to shut down. You can call the DescribeGatewayInformation API to
* check the status. For more information, see ActivateGateway.
*
*
*
* If do not intend to use the gateway again, you must delete the gateway (using DeleteGateway) to no longer
* pay software charges associated with the gateway.
*
*
* @param shutdownGatewayRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway to shut down.
* @return A Java Future containing the result of the ShutdownGateway operation returned by the service.
* @sample AWSStorageGatewayAsync.ShutdownGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future shutdownGatewayAsync(ShutdownGatewayRequest shutdownGatewayRequest);
/**
*
* Shuts down a gateway. To specify which gateway to shut down, use the Amazon Resource Name (ARN) of the gateway in
* the body of your request.
*
*
* The operation shuts down the gateway service component running in the gateway's virtual machine (VM) and not the
* host VM.
*
*
*
* If you want to shut down the VM, it is recommended that you first shut down the gateway component in the VM to
* avoid unpredictable conditions.
*
*
*
* After the gateway is shutdown, you cannot call any other API except StartGateway,
* DescribeGatewayInformation, and ListGateways. For more information, see ActivateGateway.
* Your applications cannot read from or write to the gateway's storage volumes, and there are no snapshots taken.
*
*
*
* When you make a shutdown request, you will get a 200 OK
success response immediately. However, it
* might take some time for the gateway to shut down. You can call the DescribeGatewayInformation API to
* check the status. For more information, see ActivateGateway.
*
*
*
* If do not intend to use the gateway again, you must delete the gateway (using DeleteGateway) to no longer
* pay software charges associated with the gateway.
*
*
* @param shutdownGatewayRequest
* A JSON object containing the Amazon Resource Name (ARN) of the gateway to shut down.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ShutdownGateway operation returned by the service.
* @sample AWSStorageGatewayAsyncHandler.ShutdownGateway
* @see AWS
* API Documentation
*/
java.util.concurrent.Future shutdownGatewayAsync(ShutdownGatewayRequest shutdownGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start a test that verifies that the specified gateway is configured for High Availability monitoring in your host
* environment. This request only initiates the test and that a successful response only indicates that the test was
* started. It doesn't indicate that the test passed. For the status of the test, invoke the
*