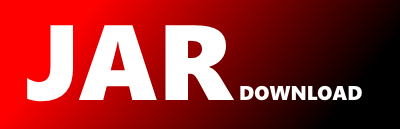
com.amazonaws.services.storagegateway.model.SMBFileShareInfo Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.storagegateway.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The Windows file permissions and ownership information assigned, by default, to native S3 objects when S3 File
* Gateway discovers them in S3 buckets. This operation is only supported for S3 File Gateways.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SMBFileShareInfo implements Serializable, Cloneable, StructuredPojo {
private String fileShareARN;
private String fileShareId;
private String fileShareStatus;
private String gatewayARN;
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*/
private Boolean kMSEncrypted;
private String kMSKey;
/**
*
* The file share path used by the SMB client to identify the mount point.
*
*/
private String path;
private String role;
private String locationARN;
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default value is
* S3_STANDARD
. Optional.
*
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
|
* S3_ONEZONE_IA
*
*/
private String defaultStorageClass;
private String objectACL;
/**
*
* A value that sets the write status of a file share. Set this value to true
to set the write status
* to read-only, otherwise set to false
.
*
*
* Valid Values: true
| false
*
*/
private Boolean readOnly;
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true
to enable MIME type guessing, otherwise set to false
. The default value is
* true
.
*
*
* Valid Values: true
| false
*
*/
private Boolean guessMIMETypeEnabled;
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*
*/
private Boolean requesterPays;
/**
*
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the SMB file
* share. If it is set to false
, it indicates that file and directory permissions are mapped to the
* POSIX permission.
*
*
* For more information, see Using Microsoft Windows ACLs to
* control access to an SMB file share in the Storage Gateway User Guide.
*
*/
private Boolean sMBACLEnabled;
/**
*
* Indicates whether AccessBasedEnumeration
is enabled.
*
*/
private Boolean accessBasedEnumeration;
/**
*
* A list of users or groups in the Active Directory that have administrator rights to the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList adminUserList;
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList validUserList;
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*/
private com.amazonaws.internal.SdkInternalList invalidUserList;
/**
*
* The Amazon Resource Name (ARN) of the storage used for audit logs.
*
*/
private String auditDestinationARN;
private String authentication;
/**
*
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines the
* case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The default value
* is ClientSpecified
.
*
*/
private String caseSensitivity;
/**
*
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The name of the file share. Optional.
*
*
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an access
* point or access point alias is used.
*
*
*/
private String fileShareName;
/**
*
* Refresh cache information for the file share.
*
*/
private CacheAttributes cacheAttributes;
/**
*
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of seconds to
* wait after the last point in time a client wrote to a file before generating an ObjectUploaded
* notification. Because clients can make many small writes to files, it's best to set this parameter for as long as
* possible to avoid generating multiple notifications for the same file in a small time period.
*
*
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only the
* timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*
*/
private String notificationPolicy;
/**
*
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*/
private String vPCEndpointDNSName;
/**
*
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*/
private String bucketRegion;
/**
*
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
*
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve access to
* files with the same name in different case.
*
*
*
* Valid Values: true
| false
*
*/
private Boolean oplocksEnabled;
/**
* @param fileShareARN
*/
public void setFileShareARN(String fileShareARN) {
this.fileShareARN = fileShareARN;
}
/**
* @return
*/
public String getFileShareARN() {
return this.fileShareARN;
}
/**
* @param fileShareARN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withFileShareARN(String fileShareARN) {
setFileShareARN(fileShareARN);
return this;
}
/**
* @param fileShareId
*/
public void setFileShareId(String fileShareId) {
this.fileShareId = fileShareId;
}
/**
* @return
*/
public String getFileShareId() {
return this.fileShareId;
}
/**
* @param fileShareId
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withFileShareId(String fileShareId) {
setFileShareId(fileShareId);
return this;
}
/**
* @param fileShareStatus
*/
public void setFileShareStatus(String fileShareStatus) {
this.fileShareStatus = fileShareStatus;
}
/**
* @return
*/
public String getFileShareStatus() {
return this.fileShareStatus;
}
/**
* @param fileShareStatus
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withFileShareStatus(String fileShareStatus) {
setFileShareStatus(fileShareStatus);
return this;
}
/**
* @param gatewayARN
*/
public void setGatewayARN(String gatewayARN) {
this.gatewayARN = gatewayARN;
}
/**
* @return
*/
public String getGatewayARN() {
return this.gatewayARN;
}
/**
* @param gatewayARN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withGatewayARN(String gatewayARN) {
setGatewayARN(gatewayARN);
return this;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @param kMSEncrypted
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public void setKMSEncrypted(Boolean kMSEncrypted) {
this.kMSEncrypted = kMSEncrypted;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @return Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public Boolean getKMSEncrypted() {
return this.kMSEncrypted;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @param kMSEncrypted
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withKMSEncrypted(Boolean kMSEncrypted) {
setKMSEncrypted(kMSEncrypted);
return this;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @return Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public Boolean isKMSEncrypted() {
return this.kMSEncrypted;
}
/**
* @param kMSKey
*/
public void setKMSKey(String kMSKey) {
this.kMSKey = kMSKey;
}
/**
* @return
*/
public String getKMSKey() {
return this.kMSKey;
}
/**
* @param kMSKey
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withKMSKey(String kMSKey) {
setKMSKey(kMSKey);
return this;
}
/**
*
* The file share path used by the SMB client to identify the mount point.
*
*
* @param path
* The file share path used by the SMB client to identify the mount point.
*/
public void setPath(String path) {
this.path = path;
}
/**
*
* The file share path used by the SMB client to identify the mount point.
*
*
* @return The file share path used by the SMB client to identify the mount point.
*/
public String getPath() {
return this.path;
}
/**
*
* The file share path used by the SMB client to identify the mount point.
*
*
* @param path
* The file share path used by the SMB client to identify the mount point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withPath(String path) {
setPath(path);
return this;
}
/**
* @param role
*/
public void setRole(String role) {
this.role = role;
}
/**
* @return
*/
public String getRole() {
return this.role;
}
/**
* @param role
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withRole(String role) {
setRole(role);
return this;
}
/**
* @param locationARN
*/
public void setLocationARN(String locationARN) {
this.locationARN = locationARN;
}
/**
* @return
*/
public String getLocationARN() {
return this.locationARN;
}
/**
* @param locationARN
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withLocationARN(String locationARN) {
setLocationARN(locationARN);
return this;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default value is
* S3_STANDARD
. Optional.
*
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
|
* S3_ONEZONE_IA
*
*
* @param defaultStorageClass
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default
* value is S3_STANDARD
. Optional.
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
* | S3_ONEZONE_IA
*/
public void setDefaultStorageClass(String defaultStorageClass) {
this.defaultStorageClass = defaultStorageClass;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default value is
* S3_STANDARD
. Optional.
*
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
|
* S3_ONEZONE_IA
*
*
* @return The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default
* value is S3_STANDARD
. Optional.
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
|
* S3_STANDARD_IA
| S3_ONEZONE_IA
*/
public String getDefaultStorageClass() {
return this.defaultStorageClass;
}
/**
*
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default value is
* S3_STANDARD
. Optional.
*
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
|
* S3_ONEZONE_IA
*
*
* @param defaultStorageClass
* The default storage class for objects put into an Amazon S3 bucket by the S3 File Gateway. The default
* value is S3_STANDARD
. Optional.
*
* Valid Values: S3_STANDARD
| S3_INTELLIGENT_TIERING
| S3_STANDARD_IA
* | S3_ONEZONE_IA
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withDefaultStorageClass(String defaultStorageClass) {
setDefaultStorageClass(defaultStorageClass);
return this;
}
/**
* @param objectACL
* @see ObjectACL
*/
public void setObjectACL(String objectACL) {
this.objectACL = objectACL;
}
/**
* @return
* @see ObjectACL
*/
public String getObjectACL() {
return this.objectACL;
}
/**
* @param objectACL
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectACL
*/
public SMBFileShareInfo withObjectACL(String objectACL) {
setObjectACL(objectACL);
return this;
}
/**
* @param objectACL
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectACL
*/
public SMBFileShareInfo withObjectACL(ObjectACL objectACL) {
this.objectACL = objectACL.toString();
return this;
}
/**
*
* A value that sets the write status of a file share. Set this value to true
to set the write status
* to read-only, otherwise set to false
.
*
*
* Valid Values: true
| false
*
*
* @param readOnly
* A value that sets the write status of a file share. Set this value to true
to set the write
* status to read-only, otherwise set to false
.
*
* Valid Values: true
| false
*/
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
/**
*
* A value that sets the write status of a file share. Set this value to true
to set the write status
* to read-only, otherwise set to false
.
*
*
* Valid Values: true
| false
*
*
* @return A value that sets the write status of a file share. Set this value to true
to set the write
* status to read-only, otherwise set to false
.
*
* Valid Values: true
| false
*/
public Boolean getReadOnly() {
return this.readOnly;
}
/**
*
* A value that sets the write status of a file share. Set this value to true
to set the write status
* to read-only, otherwise set to false
.
*
*
* Valid Values: true
| false
*
*
* @param readOnly
* A value that sets the write status of a file share. Set this value to true
to set the write
* status to read-only, otherwise set to false
.
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withReadOnly(Boolean readOnly) {
setReadOnly(readOnly);
return this;
}
/**
*
* A value that sets the write status of a file share. Set this value to true
to set the write status
* to read-only, otherwise set to false
.
*
*
* Valid Values: true
| false
*
*
* @return A value that sets the write status of a file share. Set this value to true
to set the write
* status to read-only, otherwise set to false
.
*
* Valid Values: true
| false
*/
public Boolean isReadOnly() {
return this.readOnly;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true
to enable MIME type guessing, otherwise set to false
. The default value is
* true
.
*
*
* Valid Values: true
| false
*
*
* @param guessMIMETypeEnabled
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true
to enable MIME type guessing, otherwise set to false
. The default
* value is true
.
*
* Valid Values: true
| false
*/
public void setGuessMIMETypeEnabled(Boolean guessMIMETypeEnabled) {
this.guessMIMETypeEnabled = guessMIMETypeEnabled;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true
to enable MIME type guessing, otherwise set to false
. The default value is
* true
.
*
*
* Valid Values: true
| false
*
*
* @return A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true
to enable MIME type guessing, otherwise set to false
. The default
* value is true
.
*
* Valid Values: true
| false
*/
public Boolean getGuessMIMETypeEnabled() {
return this.guessMIMETypeEnabled;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true
to enable MIME type guessing, otherwise set to false
. The default value is
* true
.
*
*
* Valid Values: true
| false
*
*
* @param guessMIMETypeEnabled
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true
to enable MIME type guessing, otherwise set to false
. The default
* value is true
.
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withGuessMIMETypeEnabled(Boolean guessMIMETypeEnabled) {
setGuessMIMETypeEnabled(guessMIMETypeEnabled);
return this;
}
/**
*
* A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this value to
* true
to enable MIME type guessing, otherwise set to false
. The default value is
* true
.
*
*
* Valid Values: true
| false
*
*
* @return A value that enables guessing of the MIME type for uploaded objects based on file extensions. Set this
* value to true
to enable MIME type guessing, otherwise set to false
. The default
* value is true
.
*
* Valid Values: true
| false
*/
public Boolean isGuessMIMETypeEnabled() {
return this.guessMIMETypeEnabled;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*
*
* @param requesterPays
* A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket
* owner pays. However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*/
public void setRequesterPays(Boolean requesterPays) {
this.requesterPays = requesterPays;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*
*
* @return A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket
* owner pays. However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*/
public Boolean getRequesterPays() {
return this.requesterPays;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*
*
* @param requesterPays
* A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket
* owner pays. However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withRequesterPays(Boolean requesterPays) {
setRequesterPays(requesterPays);
return this;
}
/**
*
* A value that sets who pays the cost of the request and the cost associated with data download from the S3 bucket.
* If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket owner pays.
* However, the S3 bucket owner always pays the cost of storing data.
*
*
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure that the
* configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*
*
* @return A value that sets who pays the cost of the request and the cost associated with data download from the S3
* bucket. If this value is set to true
, the requester pays the costs; otherwise, the S3 bucket
* owner pays. However, the S3 bucket owner always pays the cost of storing data.
*
* RequesterPays
is a configuration for the S3 bucket that backs the file share, so make sure
* that the configuration on the file share is the same as the S3 bucket configuration.
*
*
*
* Valid Values: true
| false
*/
public Boolean isRequesterPays() {
return this.requesterPays;
}
/**
*
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the SMB file
* share. If it is set to false
, it indicates that file and directory permissions are mapped to the
* POSIX permission.
*
*
* For more information, see Using Microsoft Windows ACLs to
* control access to an SMB file share in the Storage Gateway User Guide.
*
*
* @param sMBACLEnabled
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the
* SMB file share. If it is set to false
, it indicates that file and directory permissions are
* mapped to the POSIX permission.
*
* For more information, see Using Microsoft Windows
* ACLs to control access to an SMB file share in the Storage Gateway User Guide.
*/
public void setSMBACLEnabled(Boolean sMBACLEnabled) {
this.sMBACLEnabled = sMBACLEnabled;
}
/**
*
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the SMB file
* share. If it is set to false
, it indicates that file and directory permissions are mapped to the
* POSIX permission.
*
*
* For more information, see Using Microsoft Windows ACLs to
* control access to an SMB file share in the Storage Gateway User Guide.
*
*
* @return If this value is set to true
, it indicates that access control list (ACL) is enabled on the
* SMB file share. If it is set to false
, it indicates that file and directory permissions are
* mapped to the POSIX permission.
*
* For more information, see Using Microsoft Windows
* ACLs to control access to an SMB file share in the Storage Gateway User Guide.
*/
public Boolean getSMBACLEnabled() {
return this.sMBACLEnabled;
}
/**
*
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the SMB file
* share. If it is set to false
, it indicates that file and directory permissions are mapped to the
* POSIX permission.
*
*
* For more information, see Using Microsoft Windows ACLs to
* control access to an SMB file share in the Storage Gateway User Guide.
*
*
* @param sMBACLEnabled
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the
* SMB file share. If it is set to false
, it indicates that file and directory permissions are
* mapped to the POSIX permission.
*
* For more information, see Using Microsoft Windows
* ACLs to control access to an SMB file share in the Storage Gateway User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withSMBACLEnabled(Boolean sMBACLEnabled) {
setSMBACLEnabled(sMBACLEnabled);
return this;
}
/**
*
* If this value is set to true
, it indicates that access control list (ACL) is enabled on the SMB file
* share. If it is set to false
, it indicates that file and directory permissions are mapped to the
* POSIX permission.
*
*
* For more information, see Using Microsoft Windows ACLs to
* control access to an SMB file share in the Storage Gateway User Guide.
*
*
* @return If this value is set to true
, it indicates that access control list (ACL) is enabled on the
* SMB file share. If it is set to false
, it indicates that file and directory permissions are
* mapped to the POSIX permission.
*
* For more information, see Using Microsoft Windows
* ACLs to control access to an SMB file share in the Storage Gateway User Guide.
*/
public Boolean isSMBACLEnabled() {
return this.sMBACLEnabled;
}
/**
*
* Indicates whether AccessBasedEnumeration
is enabled.
*
*
* @param accessBasedEnumeration
* Indicates whether AccessBasedEnumeration
is enabled.
*/
public void setAccessBasedEnumeration(Boolean accessBasedEnumeration) {
this.accessBasedEnumeration = accessBasedEnumeration;
}
/**
*
* Indicates whether AccessBasedEnumeration
is enabled.
*
*
* @return Indicates whether AccessBasedEnumeration
is enabled.
*/
public Boolean getAccessBasedEnumeration() {
return this.accessBasedEnumeration;
}
/**
*
* Indicates whether AccessBasedEnumeration
is enabled.
*
*
* @param accessBasedEnumeration
* Indicates whether AccessBasedEnumeration
is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withAccessBasedEnumeration(Boolean accessBasedEnumeration) {
setAccessBasedEnumeration(accessBasedEnumeration);
return this;
}
/**
*
* Indicates whether AccessBasedEnumeration
is enabled.
*
*
* @return Indicates whether AccessBasedEnumeration
is enabled.
*/
public Boolean isAccessBasedEnumeration() {
return this.accessBasedEnumeration;
}
/**
*
* A list of users or groups in the Active Directory that have administrator rights to the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users or groups in the Active Directory that have administrator rights to the file share. A
* group must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public java.util.List getAdminUserList() {
if (adminUserList == null) {
adminUserList = new com.amazonaws.internal.SdkInternalList();
}
return adminUserList;
}
/**
*
* A list of users or groups in the Active Directory that have administrator rights to the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param adminUserList
* A list of users or groups in the Active Directory that have administrator rights to the file share. A
* group must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public void setAdminUserList(java.util.Collection adminUserList) {
if (adminUserList == null) {
this.adminUserList = null;
return;
}
this.adminUserList = new com.amazonaws.internal.SdkInternalList(adminUserList);
}
/**
*
* A list of users or groups in the Active Directory that have administrator rights to the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdminUserList(java.util.Collection)} or {@link #withAdminUserList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param adminUserList
* A list of users or groups in the Active Directory that have administrator rights to the file share. A
* group must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withAdminUserList(String... adminUserList) {
if (this.adminUserList == null) {
setAdminUserList(new com.amazonaws.internal.SdkInternalList(adminUserList.length));
}
for (String ele : adminUserList) {
this.adminUserList.add(ele);
}
return this;
}
/**
*
* A list of users or groups in the Active Directory that have administrator rights to the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param adminUserList
* A list of users or groups in the Active Directory that have administrator rights to the file share. A
* group must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withAdminUserList(java.util.Collection adminUserList) {
setAdminUserList(adminUserList);
return this;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public java.util.List getValidUserList() {
if (validUserList == null) {
validUserList = new com.amazonaws.internal.SdkInternalList();
}
return validUserList;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public void setValidUserList(java.util.Collection validUserList) {
if (validUserList == null) {
this.validUserList = null;
return;
}
this.validUserList = new com.amazonaws.internal.SdkInternalList(validUserList);
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValidUserList(java.util.Collection)} or {@link #withValidUserList(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withValidUserList(String... validUserList) {
if (this.validUserList == null) {
setValidUserList(new com.amazonaws.internal.SdkInternalList(validUserList.length));
}
for (String ele : validUserList) {
this.validUserList.add(ele);
}
return this;
}
/**
*
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param validUserList
* A list of users or groups in the Active Directory that are allowed to access the file share. A group must
* be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withValidUserList(java.util.Collection validUserList) {
setValidUserList(validUserList);
return this;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @return A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public java.util.List getInvalidUserList() {
if (invalidUserList == null) {
invalidUserList = new com.amazonaws.internal.SdkInternalList();
}
return invalidUserList;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
*/
public void setInvalidUserList(java.util.Collection invalidUserList) {
if (invalidUserList == null) {
this.invalidUserList = null;
return;
}
this.invalidUserList = new com.amazonaws.internal.SdkInternalList(invalidUserList);
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInvalidUserList(java.util.Collection)} or {@link #withInvalidUserList(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withInvalidUserList(String... invalidUserList) {
if (this.invalidUserList == null) {
setInvalidUserList(new com.amazonaws.internal.SdkInternalList(invalidUserList.length));
}
for (String ele : invalidUserList) {
this.invalidUserList.add(ele);
}
return this;
}
/**
*
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group must be
* prefixed with the @ character. Acceptable formats include: DOMAIN\User1
, user1
,
* @group1
, and @DOMAIN\group1
. Can only be set if Authentication is set to
* ActiveDirectory
.
*
*
* @param invalidUserList
* A list of users or groups in the Active Directory that are not allowed to access the file share. A group
* must be prefixed with the @ character. Acceptable formats include: DOMAIN\User1
,
* user1
, @group1
, and @DOMAIN\group1
. Can only be set if
* Authentication is set to ActiveDirectory
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withInvalidUserList(java.util.Collection invalidUserList) {
setInvalidUserList(invalidUserList);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the storage used for audit logs.
*
*
* @param auditDestinationARN
* The Amazon Resource Name (ARN) of the storage used for audit logs.
*/
public void setAuditDestinationARN(String auditDestinationARN) {
this.auditDestinationARN = auditDestinationARN;
}
/**
*
* The Amazon Resource Name (ARN) of the storage used for audit logs.
*
*
* @return The Amazon Resource Name (ARN) of the storage used for audit logs.
*/
public String getAuditDestinationARN() {
return this.auditDestinationARN;
}
/**
*
* The Amazon Resource Name (ARN) of the storage used for audit logs.
*
*
* @param auditDestinationARN
* The Amazon Resource Name (ARN) of the storage used for audit logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withAuditDestinationARN(String auditDestinationARN) {
setAuditDestinationARN(auditDestinationARN);
return this;
}
/**
* @param authentication
*/
public void setAuthentication(String authentication) {
this.authentication = authentication;
}
/**
* @return
*/
public String getAuthentication() {
return this.authentication;
}
/**
* @param authentication
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withAuthentication(String authentication) {
setAuthentication(authentication);
return this;
}
/**
*
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines the
* case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The default value
* is ClientSpecified
.
*
*
* @param caseSensitivity
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines
* the case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The
* default value is ClientSpecified
.
* @see CaseSensitivity
*/
public void setCaseSensitivity(String caseSensitivity) {
this.caseSensitivity = caseSensitivity;
}
/**
*
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines the
* case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The default value
* is ClientSpecified
.
*
*
* @return The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client
* determines the case sensitivity. For CaseSensitive
, the gateway determines the case
* sensitivity. The default value is ClientSpecified
.
* @see CaseSensitivity
*/
public String getCaseSensitivity() {
return this.caseSensitivity;
}
/**
*
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines the
* case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The default value
* is ClientSpecified
.
*
*
* @param caseSensitivity
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines
* the case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The
* default value is ClientSpecified
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CaseSensitivity
*/
public SMBFileShareInfo withCaseSensitivity(String caseSensitivity) {
setCaseSensitivity(caseSensitivity);
return this;
}
/**
*
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines the
* case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The default value
* is ClientSpecified
.
*
*
* @param caseSensitivity
* The case of an object name in an Amazon S3 bucket. For ClientSpecified
, the client determines
* the case sensitivity. For CaseSensitive
, the gateway determines the case sensitivity. The
* default value is ClientSpecified
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CaseSensitivity
*/
public SMBFileShareInfo withCaseSensitivity(CaseSensitivity caseSensitivity) {
this.caseSensitivity = caseSensitivity.toString();
return this;
}
/**
*
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*
*
* @return A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*
*
* @param tags
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
*
*
* @param tags
* A list of up to 50 tags assigned to the SMB file share, sorted alphabetically by key name. Each tag is a
* key-value pair. For a gateway with more than 10 tags assigned, you can view all tags using the
* ListTagsForResource
API operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The name of the file share. Optional.
*
*
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an access
* point or access point alias is used.
*
*
*
* @param fileShareName
* The name of the file share. Optional.
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an
* access point or access point alias is used.
*
*/
public void setFileShareName(String fileShareName) {
this.fileShareName = fileShareName;
}
/**
*
* The name of the file share. Optional.
*
*
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an access
* point or access point alias is used.
*
*
*
* @return The name of the file share. Optional.
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an
* access point or access point alias is used.
*
*/
public String getFileShareName() {
return this.fileShareName;
}
/**
*
* The name of the file share. Optional.
*
*
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an access
* point or access point alias is used.
*
*
*
* @param fileShareName
* The name of the file share. Optional.
*
* FileShareName
must be set if an S3 prefix name is set in LocationARN
, or if an
* access point or access point alias is used.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withFileShareName(String fileShareName) {
setFileShareName(fileShareName);
return this;
}
/**
*
* Refresh cache information for the file share.
*
*
* @param cacheAttributes
* Refresh cache information for the file share.
*/
public void setCacheAttributes(CacheAttributes cacheAttributes) {
this.cacheAttributes = cacheAttributes;
}
/**
*
* Refresh cache information for the file share.
*
*
* @return Refresh cache information for the file share.
*/
public CacheAttributes getCacheAttributes() {
return this.cacheAttributes;
}
/**
*
* Refresh cache information for the file share.
*
*
* @param cacheAttributes
* Refresh cache information for the file share.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withCacheAttributes(CacheAttributes cacheAttributes) {
setCacheAttributes(cacheAttributes);
return this;
}
/**
*
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of seconds to
* wait after the last point in time a client wrote to a file before generating an ObjectUploaded
* notification. Because clients can make many small writes to files, it's best to set this parameter for as long as
* possible to avoid generating multiple notifications for the same file in a small time period.
*
*
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only the
* timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*
*
* @param notificationPolicy
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of
* seconds to wait after the last point in time a client wrote to a file before generating an
* ObjectUploaded
notification. Because clients can make many small writes to files, it's best
* to set this parameter for as long as possible to avoid generating multiple notifications for the same file
* in a small time period.
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only
* the timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set
* to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*/
public void setNotificationPolicy(String notificationPolicy) {
this.notificationPolicy = notificationPolicy;
}
/**
*
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of seconds to
* wait after the last point in time a client wrote to a file before generating an ObjectUploaded
* notification. Because clients can make many small writes to files, it's best to set this parameter for as long as
* possible to avoid generating multiple notifications for the same file in a small time period.
*
*
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only the
* timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*
*
* @return The notification policy of the file share. SettlingTimeInSeconds
controls the number of
* seconds to wait after the last point in time a client wrote to a file before generating an
* ObjectUploaded
notification. Because clients can make many small writes to files, it's best
* to set this parameter for as long as possible to avoid generating multiple notifications for the same
* file in a small time period.
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only
* the timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set
* to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*/
public String getNotificationPolicy() {
return this.notificationPolicy;
}
/**
*
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of seconds to
* wait after the last point in time a client wrote to a file before generating an ObjectUploaded
* notification. Because clients can make many small writes to files, it's best to set this parameter for as long as
* possible to avoid generating multiple notifications for the same file in a small time period.
*
*
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only the
* timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
*
*
* @param notificationPolicy
* The notification policy of the file share. SettlingTimeInSeconds
controls the number of
* seconds to wait after the last point in time a client wrote to a file before generating an
* ObjectUploaded
notification. Because clients can make many small writes to files, it's best
* to set this parameter for as long as possible to avoid generating multiple notifications for the same file
* in a small time period.
*
* SettlingTimeInSeconds
has no effect on the timing of the object uploading to Amazon S3, only
* the timing of the notification.
*
*
*
* The following example sets NotificationPolicy
on with SettlingTimeInSeconds
set
* to 60.
*
*
* {\"Upload\": {\"SettlingTimeInSeconds\": 60}}
*
*
* The following example sets NotificationPolicy
off.
*
*
* {}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withNotificationPolicy(String notificationPolicy) {
setNotificationPolicy(notificationPolicy);
return this;
}
/**
*
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @param vPCEndpointDNSName
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
*/
public void setVPCEndpointDNSName(String vPCEndpointDNSName) {
this.vPCEndpointDNSName = vPCEndpointDNSName;
}
/**
*
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @return Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
*/
public String getVPCEndpointDNSName() {
return this.vPCEndpointDNSName;
}
/**
*
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @param vPCEndpointDNSName
* Specifies the DNS name for the VPC endpoint that the SMB file share uses to connect to Amazon S3.
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withVPCEndpointDNSName(String vPCEndpointDNSName) {
setVPCEndpointDNSName(vPCEndpointDNSName);
return this;
}
/**
*
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @param bucketRegion
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
*/
public void setBucketRegion(String bucketRegion) {
this.bucketRegion = bucketRegion;
}
/**
*
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @return Specifies the Region of the S3 bucket where the SMB file share stores files.
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
*/
public String getBucketRegion() {
return this.bucketRegion;
}
/**
*
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
*
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC access
* point, or an access point alias that points to a VPC access point.
*
*
*
* @param bucketRegion
* Specifies the Region of the S3 bucket where the SMB file share stores files.
*
* This parameter is required for SMB file shares that connect to Amazon S3 through a VPC endpoint, a VPC
* access point, or an access point alias that points to a VPC access point.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withBucketRegion(String bucketRegion) {
setBucketRegion(bucketRegion);
return this;
}
/**
*
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
*
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve access to
* files with the same name in different case.
*
*
*
* Valid Values: true
| false
*
*
* @param oplocksEnabled
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve
* access to files with the same name in different case.
*
*
*
* Valid Values: true
| false
*/
public void setOplocksEnabled(Boolean oplocksEnabled) {
this.oplocksEnabled = oplocksEnabled;
}
/**
*
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
*
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve access to
* files with the same name in different case.
*
*
*
* Valid Values: true
| false
*
*
* @return Specifies whether opportunistic locking is enabled for the SMB file share.
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve
* access to files with the same name in different case.
*
*
*
* Valid Values: true
| false
*/
public Boolean getOplocksEnabled() {
return this.oplocksEnabled;
}
/**
*
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
*
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve access to
* files with the same name in different case.
*
*
*
* Valid Values: true
| false
*
*
* @param oplocksEnabled
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve
* access to files with the same name in different case.
*
*
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SMBFileShareInfo withOplocksEnabled(Boolean oplocksEnabled) {
setOplocksEnabled(oplocksEnabled);
return this;
}
/**
*
* Specifies whether opportunistic locking is enabled for the SMB file share.
*
*
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve access to
* files with the same name in different case.
*
*
*
* Valid Values: true
| false
*
*
* @return Specifies whether opportunistic locking is enabled for the SMB file share.
*
* Enabling opportunistic locking on case-sensitive shares is not recommended for workloads that involve
* access to files with the same name in different case.
*
*
*
* Valid Values: true
| false
*/
public Boolean isOplocksEnabled() {
return this.oplocksEnabled;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFileShareARN() != null)
sb.append("FileShareARN: ").append(getFileShareARN()).append(",");
if (getFileShareId() != null)
sb.append("FileShareId: ").append(getFileShareId()).append(",");
if (getFileShareStatus() != null)
sb.append("FileShareStatus: ").append(getFileShareStatus()).append(",");
if (getGatewayARN() != null)
sb.append("GatewayARN: ").append(getGatewayARN()).append(",");
if (getKMSEncrypted() != null)
sb.append("KMSEncrypted: ").append(getKMSEncrypted()).append(",");
if (getKMSKey() != null)
sb.append("KMSKey: ").append(getKMSKey()).append(",");
if (getPath() != null)
sb.append("Path: ").append(getPath()).append(",");
if (getRole() != null)
sb.append("Role: ").append(getRole()).append(",");
if (getLocationARN() != null)
sb.append("LocationARN: ").append(getLocationARN()).append(",");
if (getDefaultStorageClass() != null)
sb.append("DefaultStorageClass: ").append(getDefaultStorageClass()).append(",");
if (getObjectACL() != null)
sb.append("ObjectACL: ").append(getObjectACL()).append(",");
if (getReadOnly() != null)
sb.append("ReadOnly: ").append(getReadOnly()).append(",");
if (getGuessMIMETypeEnabled() != null)
sb.append("GuessMIMETypeEnabled: ").append(getGuessMIMETypeEnabled()).append(",");
if (getRequesterPays() != null)
sb.append("RequesterPays: ").append(getRequesterPays()).append(",");
if (getSMBACLEnabled() != null)
sb.append("SMBACLEnabled: ").append(getSMBACLEnabled()).append(",");
if (getAccessBasedEnumeration() != null)
sb.append("AccessBasedEnumeration: ").append(getAccessBasedEnumeration()).append(",");
if (getAdminUserList() != null)
sb.append("AdminUserList: ").append(getAdminUserList()).append(",");
if (getValidUserList() != null)
sb.append("ValidUserList: ").append(getValidUserList()).append(",");
if (getInvalidUserList() != null)
sb.append("InvalidUserList: ").append(getInvalidUserList()).append(",");
if (getAuditDestinationARN() != null)
sb.append("AuditDestinationARN: ").append(getAuditDestinationARN()).append(",");
if (getAuthentication() != null)
sb.append("Authentication: ").append(getAuthentication()).append(",");
if (getCaseSensitivity() != null)
sb.append("CaseSensitivity: ").append(getCaseSensitivity()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getFileShareName() != null)
sb.append("FileShareName: ").append(getFileShareName()).append(",");
if (getCacheAttributes() != null)
sb.append("CacheAttributes: ").append(getCacheAttributes()).append(",");
if (getNotificationPolicy() != null)
sb.append("NotificationPolicy: ").append(getNotificationPolicy()).append(",");
if (getVPCEndpointDNSName() != null)
sb.append("VPCEndpointDNSName: ").append(getVPCEndpointDNSName()).append(",");
if (getBucketRegion() != null)
sb.append("BucketRegion: ").append(getBucketRegion()).append(",");
if (getOplocksEnabled() != null)
sb.append("OplocksEnabled: ").append(getOplocksEnabled());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SMBFileShareInfo == false)
return false;
SMBFileShareInfo other = (SMBFileShareInfo) obj;
if (other.getFileShareARN() == null ^ this.getFileShareARN() == null)
return false;
if (other.getFileShareARN() != null && other.getFileShareARN().equals(this.getFileShareARN()) == false)
return false;
if (other.getFileShareId() == null ^ this.getFileShareId() == null)
return false;
if (other.getFileShareId() != null && other.getFileShareId().equals(this.getFileShareId()) == false)
return false;
if (other.getFileShareStatus() == null ^ this.getFileShareStatus() == null)
return false;
if (other.getFileShareStatus() != null && other.getFileShareStatus().equals(this.getFileShareStatus()) == false)
return false;
if (other.getGatewayARN() == null ^ this.getGatewayARN() == null)
return false;
if (other.getGatewayARN() != null && other.getGatewayARN().equals(this.getGatewayARN()) == false)
return false;
if (other.getKMSEncrypted() == null ^ this.getKMSEncrypted() == null)
return false;
if (other.getKMSEncrypted() != null && other.getKMSEncrypted().equals(this.getKMSEncrypted()) == false)
return false;
if (other.getKMSKey() == null ^ this.getKMSKey() == null)
return false;
if (other.getKMSKey() != null && other.getKMSKey().equals(this.getKMSKey()) == false)
return false;
if (other.getPath() == null ^ this.getPath() == null)
return false;
if (other.getPath() != null && other.getPath().equals(this.getPath()) == false)
return false;
if (other.getRole() == null ^ this.getRole() == null)
return false;
if (other.getRole() != null && other.getRole().equals(this.getRole()) == false)
return false;
if (other.getLocationARN() == null ^ this.getLocationARN() == null)
return false;
if (other.getLocationARN() != null && other.getLocationARN().equals(this.getLocationARN()) == false)
return false;
if (other.getDefaultStorageClass() == null ^ this.getDefaultStorageClass() == null)
return false;
if (other.getDefaultStorageClass() != null && other.getDefaultStorageClass().equals(this.getDefaultStorageClass()) == false)
return false;
if (other.getObjectACL() == null ^ this.getObjectACL() == null)
return false;
if (other.getObjectACL() != null && other.getObjectACL().equals(this.getObjectACL()) == false)
return false;
if (other.getReadOnly() == null ^ this.getReadOnly() == null)
return false;
if (other.getReadOnly() != null && other.getReadOnly().equals(this.getReadOnly()) == false)
return false;
if (other.getGuessMIMETypeEnabled() == null ^ this.getGuessMIMETypeEnabled() == null)
return false;
if (other.getGuessMIMETypeEnabled() != null && other.getGuessMIMETypeEnabled().equals(this.getGuessMIMETypeEnabled()) == false)
return false;
if (other.getRequesterPays() == null ^ this.getRequesterPays() == null)
return false;
if (other.getRequesterPays() != null && other.getRequesterPays().equals(this.getRequesterPays()) == false)
return false;
if (other.getSMBACLEnabled() == null ^ this.getSMBACLEnabled() == null)
return false;
if (other.getSMBACLEnabled() != null && other.getSMBACLEnabled().equals(this.getSMBACLEnabled()) == false)
return false;
if (other.getAccessBasedEnumeration() == null ^ this.getAccessBasedEnumeration() == null)
return false;
if (other.getAccessBasedEnumeration() != null && other.getAccessBasedEnumeration().equals(this.getAccessBasedEnumeration()) == false)
return false;
if (other.getAdminUserList() == null ^ this.getAdminUserList() == null)
return false;
if (other.getAdminUserList() != null && other.getAdminUserList().equals(this.getAdminUserList()) == false)
return false;
if (other.getValidUserList() == null ^ this.getValidUserList() == null)
return false;
if (other.getValidUserList() != null && other.getValidUserList().equals(this.getValidUserList()) == false)
return false;
if (other.getInvalidUserList() == null ^ this.getInvalidUserList() == null)
return false;
if (other.getInvalidUserList() != null && other.getInvalidUserList().equals(this.getInvalidUserList()) == false)
return false;
if (other.getAuditDestinationARN() == null ^ this.getAuditDestinationARN() == null)
return false;
if (other.getAuditDestinationARN() != null && other.getAuditDestinationARN().equals(this.getAuditDestinationARN()) == false)
return false;
if (other.getAuthentication() == null ^ this.getAuthentication() == null)
return false;
if (other.getAuthentication() != null && other.getAuthentication().equals(this.getAuthentication()) == false)
return false;
if (other.getCaseSensitivity() == null ^ this.getCaseSensitivity() == null)
return false;
if (other.getCaseSensitivity() != null && other.getCaseSensitivity().equals(this.getCaseSensitivity()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getFileShareName() == null ^ this.getFileShareName() == null)
return false;
if (other.getFileShareName() != null && other.getFileShareName().equals(this.getFileShareName()) == false)
return false;
if (other.getCacheAttributes() == null ^ this.getCacheAttributes() == null)
return false;
if (other.getCacheAttributes() != null && other.getCacheAttributes().equals(this.getCacheAttributes()) == false)
return false;
if (other.getNotificationPolicy() == null ^ this.getNotificationPolicy() == null)
return false;
if (other.getNotificationPolicy() != null && other.getNotificationPolicy().equals(this.getNotificationPolicy()) == false)
return false;
if (other.getVPCEndpointDNSName() == null ^ this.getVPCEndpointDNSName() == null)
return false;
if (other.getVPCEndpointDNSName() != null && other.getVPCEndpointDNSName().equals(this.getVPCEndpointDNSName()) == false)
return false;
if (other.getBucketRegion() == null ^ this.getBucketRegion() == null)
return false;
if (other.getBucketRegion() != null && other.getBucketRegion().equals(this.getBucketRegion()) == false)
return false;
if (other.getOplocksEnabled() == null ^ this.getOplocksEnabled() == null)
return false;
if (other.getOplocksEnabled() != null && other.getOplocksEnabled().equals(this.getOplocksEnabled()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFileShareARN() == null) ? 0 : getFileShareARN().hashCode());
hashCode = prime * hashCode + ((getFileShareId() == null) ? 0 : getFileShareId().hashCode());
hashCode = prime * hashCode + ((getFileShareStatus() == null) ? 0 : getFileShareStatus().hashCode());
hashCode = prime * hashCode + ((getGatewayARN() == null) ? 0 : getGatewayARN().hashCode());
hashCode = prime * hashCode + ((getKMSEncrypted() == null) ? 0 : getKMSEncrypted().hashCode());
hashCode = prime * hashCode + ((getKMSKey() == null) ? 0 : getKMSKey().hashCode());
hashCode = prime * hashCode + ((getPath() == null) ? 0 : getPath().hashCode());
hashCode = prime * hashCode + ((getRole() == null) ? 0 : getRole().hashCode());
hashCode = prime * hashCode + ((getLocationARN() == null) ? 0 : getLocationARN().hashCode());
hashCode = prime * hashCode + ((getDefaultStorageClass() == null) ? 0 : getDefaultStorageClass().hashCode());
hashCode = prime * hashCode + ((getObjectACL() == null) ? 0 : getObjectACL().hashCode());
hashCode = prime * hashCode + ((getReadOnly() == null) ? 0 : getReadOnly().hashCode());
hashCode = prime * hashCode + ((getGuessMIMETypeEnabled() == null) ? 0 : getGuessMIMETypeEnabled().hashCode());
hashCode = prime * hashCode + ((getRequesterPays() == null) ? 0 : getRequesterPays().hashCode());
hashCode = prime * hashCode + ((getSMBACLEnabled() == null) ? 0 : getSMBACLEnabled().hashCode());
hashCode = prime * hashCode + ((getAccessBasedEnumeration() == null) ? 0 : getAccessBasedEnumeration().hashCode());
hashCode = prime * hashCode + ((getAdminUserList() == null) ? 0 : getAdminUserList().hashCode());
hashCode = prime * hashCode + ((getValidUserList() == null) ? 0 : getValidUserList().hashCode());
hashCode = prime * hashCode + ((getInvalidUserList() == null) ? 0 : getInvalidUserList().hashCode());
hashCode = prime * hashCode + ((getAuditDestinationARN() == null) ? 0 : getAuditDestinationARN().hashCode());
hashCode = prime * hashCode + ((getAuthentication() == null) ? 0 : getAuthentication().hashCode());
hashCode = prime * hashCode + ((getCaseSensitivity() == null) ? 0 : getCaseSensitivity().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getFileShareName() == null) ? 0 : getFileShareName().hashCode());
hashCode = prime * hashCode + ((getCacheAttributes() == null) ? 0 : getCacheAttributes().hashCode());
hashCode = prime * hashCode + ((getNotificationPolicy() == null) ? 0 : getNotificationPolicy().hashCode());
hashCode = prime * hashCode + ((getVPCEndpointDNSName() == null) ? 0 : getVPCEndpointDNSName().hashCode());
hashCode = prime * hashCode + ((getBucketRegion() == null) ? 0 : getBucketRegion().hashCode());
hashCode = prime * hashCode + ((getOplocksEnabled() == null) ? 0 : getOplocksEnabled().hashCode());
return hashCode;
}
@Override
public SMBFileShareInfo clone() {
try {
return (SMBFileShareInfo) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.storagegateway.model.transform.SMBFileShareInfoMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}