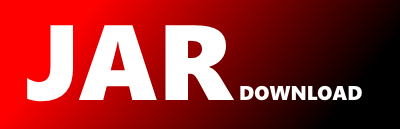
com.amazonaws.services.storagegateway.model.CachediSCSIVolume Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.storagegateway.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes an iSCSI cached volume.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CachediSCSIVolume implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the storage volume.
*
*/
private String volumeARN;
/**
*
* The unique identifier of the volume, e.g., vol-AE4B946D.
*
*/
private String volumeId;
/**
*
* One of the VolumeType enumeration values that describes the type of the volume.
*
*/
private String volumeType;
/**
*
* One of the VolumeStatus values that indicates the state of the storage volume.
*
*/
private String volumeStatus;
/**
*
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more information,
* see Moving your volumes to a different gateway.
*
*/
private String volumeAttachmentStatus;
/**
*
* The size, in bytes, of the volume capacity.
*
*/
private Long volumeSizeInBytes;
/**
*
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent of
* data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
*
*/
private Double volumeProgress;
/**
*
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g., snap-78e22663.
* Otherwise, this field is not included.
*
*/
private String sourceSnapshotId;
/**
*
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored volume.
*
*/
private VolumeiSCSIAttributes volumeiSCSIAttributes;
/**
*
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*
*/
private java.util.Date createdDate;
/**
*
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks that
* are touched, instead of the actual amount of data written. This value can be useful for sequential write patterns
* but less accurate for random write patterns. VolumeUsedInBytes
is different from the compressed size
* of the volume, which is the value that is used to calculate your bill.
*
*
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed storage
* on your volume, we recommend overwriting your files with zeros to compress the data to a negligible amount of
* actual storage.
*
*
*/
private Long volumeUsedInBytes;
private String kMSKey;
/**
*
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the target ARN.
* For example, specifying TargetName
as myvolume results in the target ARN of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as the new
* target name.
*
*/
private String targetName;
/**
*
* The Amazon Resource Name (ARN) of the storage volume.
*
*
* @param volumeARN
* The Amazon Resource Name (ARN) of the storage volume.
*/
public void setVolumeARN(String volumeARN) {
this.volumeARN = volumeARN;
}
/**
*
* The Amazon Resource Name (ARN) of the storage volume.
*
*
* @return The Amazon Resource Name (ARN) of the storage volume.
*/
public String getVolumeARN() {
return this.volumeARN;
}
/**
*
* The Amazon Resource Name (ARN) of the storage volume.
*
*
* @param volumeARN
* The Amazon Resource Name (ARN) of the storage volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeARN(String volumeARN) {
setVolumeARN(volumeARN);
return this;
}
/**
*
* The unique identifier of the volume, e.g., vol-AE4B946D.
*
*
* @param volumeId
* The unique identifier of the volume, e.g., vol-AE4B946D.
*/
public void setVolumeId(String volumeId) {
this.volumeId = volumeId;
}
/**
*
* The unique identifier of the volume, e.g., vol-AE4B946D.
*
*
* @return The unique identifier of the volume, e.g., vol-AE4B946D.
*/
public String getVolumeId() {
return this.volumeId;
}
/**
*
* The unique identifier of the volume, e.g., vol-AE4B946D.
*
*
* @param volumeId
* The unique identifier of the volume, e.g., vol-AE4B946D.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeId(String volumeId) {
setVolumeId(volumeId);
return this;
}
/**
*
* One of the VolumeType enumeration values that describes the type of the volume.
*
*
* @param volumeType
* One of the VolumeType enumeration values that describes the type of the volume.
*/
public void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
/**
*
* One of the VolumeType enumeration values that describes the type of the volume.
*
*
* @return One of the VolumeType enumeration values that describes the type of the volume.
*/
public String getVolumeType() {
return this.volumeType;
}
/**
*
* One of the VolumeType enumeration values that describes the type of the volume.
*
*
* @param volumeType
* One of the VolumeType enumeration values that describes the type of the volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeType(String volumeType) {
setVolumeType(volumeType);
return this;
}
/**
*
* One of the VolumeStatus values that indicates the state of the storage volume.
*
*
* @param volumeStatus
* One of the VolumeStatus values that indicates the state of the storage volume.
*/
public void setVolumeStatus(String volumeStatus) {
this.volumeStatus = volumeStatus;
}
/**
*
* One of the VolumeStatus values that indicates the state of the storage volume.
*
*
* @return One of the VolumeStatus values that indicates the state of the storage volume.
*/
public String getVolumeStatus() {
return this.volumeStatus;
}
/**
*
* One of the VolumeStatus values that indicates the state of the storage volume.
*
*
* @param volumeStatus
* One of the VolumeStatus values that indicates the state of the storage volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeStatus(String volumeStatus) {
setVolumeStatus(volumeStatus);
return this;
}
/**
*
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more information,
* see Moving your volumes to a different gateway.
*
*
* @param volumeAttachmentStatus
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more
* information, see Moving your volumes to a different gateway.
*/
public void setVolumeAttachmentStatus(String volumeAttachmentStatus) {
this.volumeAttachmentStatus = volumeAttachmentStatus;
}
/**
*
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more information,
* see Moving your volumes to a different gateway.
*
*
* @return A value that indicates whether a storage volume is attached to or detached from a gateway. For more
* information, see Moving your volumes to a different gateway.
*/
public String getVolumeAttachmentStatus() {
return this.volumeAttachmentStatus;
}
/**
*
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more information,
* see Moving your volumes to a different gateway.
*
*
* @param volumeAttachmentStatus
* A value that indicates whether a storage volume is attached to or detached from a gateway. For more
* information, see Moving your volumes to a different gateway.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeAttachmentStatus(String volumeAttachmentStatus) {
setVolumeAttachmentStatus(volumeAttachmentStatus);
return this;
}
/**
*
* The size, in bytes, of the volume capacity.
*
*
* @param volumeSizeInBytes
* The size, in bytes, of the volume capacity.
*/
public void setVolumeSizeInBytes(Long volumeSizeInBytes) {
this.volumeSizeInBytes = volumeSizeInBytes;
}
/**
*
* The size, in bytes, of the volume capacity.
*
*
* @return The size, in bytes, of the volume capacity.
*/
public Long getVolumeSizeInBytes() {
return this.volumeSizeInBytes;
}
/**
*
* The size, in bytes, of the volume capacity.
*
*
* @param volumeSizeInBytes
* The size, in bytes, of the volume capacity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeSizeInBytes(Long volumeSizeInBytes) {
setVolumeSizeInBytes(volumeSizeInBytes);
return this;
}
/**
*
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent of
* data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
*
*
* @param volumeProgress
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent
* of data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
*/
public void setVolumeProgress(Double volumeProgress) {
this.volumeProgress = volumeProgress;
}
/**
*
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent of
* data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
*
*
* @return Represents the percentage complete if the volume is restoring or bootstrapping that represents the
* percent of data transferred. This field does not appear in the response if the cached volume is not
* restoring or bootstrapping.
*/
public Double getVolumeProgress() {
return this.volumeProgress;
}
/**
*
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent of
* data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
*
*
* @param volumeProgress
* Represents the percentage complete if the volume is restoring or bootstrapping that represents the percent
* of data transferred. This field does not appear in the response if the cached volume is not restoring or
* bootstrapping.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeProgress(Double volumeProgress) {
setVolumeProgress(volumeProgress);
return this;
}
/**
*
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g., snap-78e22663.
* Otherwise, this field is not included.
*
*
* @param sourceSnapshotId
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g.,
* snap-78e22663. Otherwise, this field is not included.
*/
public void setSourceSnapshotId(String sourceSnapshotId) {
this.sourceSnapshotId = sourceSnapshotId;
}
/**
*
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g., snap-78e22663.
* Otherwise, this field is not included.
*
*
* @return If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g.,
* snap-78e22663. Otherwise, this field is not included.
*/
public String getSourceSnapshotId() {
return this.sourceSnapshotId;
}
/**
*
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g., snap-78e22663.
* Otherwise, this field is not included.
*
*
* @param sourceSnapshotId
* If the cached volume was created from a snapshot, this field contains the snapshot ID used, e.g.,
* snap-78e22663. Otherwise, this field is not included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withSourceSnapshotId(String sourceSnapshotId) {
setSourceSnapshotId(sourceSnapshotId);
return this;
}
/**
*
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored volume.
*
*
* @param volumeiSCSIAttributes
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored
* volume.
*/
public void setVolumeiSCSIAttributes(VolumeiSCSIAttributes volumeiSCSIAttributes) {
this.volumeiSCSIAttributes = volumeiSCSIAttributes;
}
/**
*
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored volume.
*
*
* @return An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored
* volume.
*/
public VolumeiSCSIAttributes getVolumeiSCSIAttributes() {
return this.volumeiSCSIAttributes;
}
/**
*
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored volume.
*
*
* @param volumeiSCSIAttributes
* An VolumeiSCSIAttributes object that represents a collection of iSCSI attributes for one stored
* volume.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeiSCSIAttributes(VolumeiSCSIAttributes volumeiSCSIAttributes) {
setVolumeiSCSIAttributes(volumeiSCSIAttributes);
return this;
}
/**
*
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*
*
* @param createdDate
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*
*
* @return The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
*
*
* @param createdDate
* The date the volume was created. Volumes created prior to March 28, 2017 don’t have this timestamp.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks that
* are touched, instead of the actual amount of data written. This value can be useful for sequential write patterns
* but less accurate for random write patterns. VolumeUsedInBytes
is different from the compressed size
* of the volume, which is the value that is used to calculate your bill.
*
*
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed storage
* on your volume, we recommend overwriting your files with zeros to compress the data to a negligible amount of
* actual storage.
*
*
*
* @param volumeUsedInBytes
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks
* that are touched, instead of the actual amount of data written. This value can be useful for sequential
* write patterns but less accurate for random write patterns. VolumeUsedInBytes
is different
* from the compressed size of the volume, which is the value that is used to calculate your bill.
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed
* storage on your volume, we recommend overwriting your files with zeros to compress the data to a
* negligible amount of actual storage.
*
*/
public void setVolumeUsedInBytes(Long volumeUsedInBytes) {
this.volumeUsedInBytes = volumeUsedInBytes;
}
/**
*
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks that
* are touched, instead of the actual amount of data written. This value can be useful for sequential write patterns
* but less accurate for random write patterns. VolumeUsedInBytes
is different from the compressed size
* of the volume, which is the value that is used to calculate your bill.
*
*
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed storage
* on your volume, we recommend overwriting your files with zeros to compress the data to a negligible amount of
* actual storage.
*
*
*
* @return The size of the data stored on the volume in bytes. This value is calculated based on the number of
* blocks that are touched, instead of the actual amount of data written. This value can be useful for
* sequential write patterns but less accurate for random write patterns. VolumeUsedInBytes
is
* different from the compressed size of the volume, which is the value that is used to calculate your
* bill.
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the
* volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed
* storage on your volume, we recommend overwriting your files with zeros to compress the data to a
* negligible amount of actual storage.
*
*/
public Long getVolumeUsedInBytes() {
return this.volumeUsedInBytes;
}
/**
*
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks that
* are touched, instead of the actual amount of data written. This value can be useful for sequential write patterns
* but less accurate for random write patterns. VolumeUsedInBytes
is different from the compressed size
* of the volume, which is the value that is used to calculate your bill.
*
*
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed storage
* on your volume, we recommend overwriting your files with zeros to compress the data to a negligible amount of
* actual storage.
*
*
*
* @param volumeUsedInBytes
* The size of the data stored on the volume in bytes. This value is calculated based on the number of blocks
* that are touched, instead of the actual amount of data written. This value can be useful for sequential
* write patterns but less accurate for random write patterns. VolumeUsedInBytes
is different
* from the compressed size of the volume, which is the value that is used to calculate your bill.
*
* This value is not available for volumes created prior to May 13, 2015, until you store data on the volume.
*
*
* If you use a delete tool that overwrites the data on your volume with random data, your usage will not be
* reduced. This is because the random data is not compressible. If you want to reduce the amount of billed
* storage on your volume, we recommend overwriting your files with zeros to compress the data to a
* negligible amount of actual storage.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withVolumeUsedInBytes(Long volumeUsedInBytes) {
setVolumeUsedInBytes(volumeUsedInBytes);
return this;
}
/**
* @param kMSKey
*/
public void setKMSKey(String kMSKey) {
this.kMSKey = kMSKey;
}
/**
* @return
*/
public String getKMSKey() {
return this.kMSKey;
}
/**
* @param kMSKey
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withKMSKey(String kMSKey) {
setKMSKey(kMSKey);
return this;
}
/**
*
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the target ARN.
* For example, specifying TargetName
as myvolume results in the target ARN of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as the new
* target name.
*
*
* @param targetName
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the
* target ARN. For example, specifying TargetName
as myvolume results in the target ARN
* of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as
* the new target name.
*/
public void setTargetName(String targetName) {
this.targetName = targetName;
}
/**
*
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the target ARN.
* For example, specifying TargetName
as myvolume results in the target ARN of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as the new
* target name.
*
*
* @return The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the
* target ARN. For example, specifying TargetName
as myvolume results in the target ARN
* of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as
* the new target name.
*/
public String getTargetName() {
return this.targetName;
}
/**
*
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the target ARN.
* For example, specifying TargetName
as myvolume results in the target ARN of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as the new
* target name.
*
*
* @param targetName
* The name of the iSCSI target used by an initiator to connect to a volume and used as a suffix for the
* target ARN. For example, specifying TargetName
as myvolume results in the target ARN
* of
* arn:aws:storagegateway:us-east-2:111122223333:gateway/sgw-12A3456B/target/iqn.1997-05.com.amazon:myvolume
* . The target name must be unique across all volumes on a gateway.
*
* If you don't specify a value, Storage Gateway uses the value that was previously used for this volume as
* the new target name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CachediSCSIVolume withTargetName(String targetName) {
setTargetName(targetName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVolumeARN() != null)
sb.append("VolumeARN: ").append(getVolumeARN()).append(",");
if (getVolumeId() != null)
sb.append("VolumeId: ").append(getVolumeId()).append(",");
if (getVolumeType() != null)
sb.append("VolumeType: ").append(getVolumeType()).append(",");
if (getVolumeStatus() != null)
sb.append("VolumeStatus: ").append(getVolumeStatus()).append(",");
if (getVolumeAttachmentStatus() != null)
sb.append("VolumeAttachmentStatus: ").append(getVolumeAttachmentStatus()).append(",");
if (getVolumeSizeInBytes() != null)
sb.append("VolumeSizeInBytes: ").append(getVolumeSizeInBytes()).append(",");
if (getVolumeProgress() != null)
sb.append("VolumeProgress: ").append(getVolumeProgress()).append(",");
if (getSourceSnapshotId() != null)
sb.append("SourceSnapshotId: ").append(getSourceSnapshotId()).append(",");
if (getVolumeiSCSIAttributes() != null)
sb.append("VolumeiSCSIAttributes: ").append(getVolumeiSCSIAttributes()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getVolumeUsedInBytes() != null)
sb.append("VolumeUsedInBytes: ").append(getVolumeUsedInBytes()).append(",");
if (getKMSKey() != null)
sb.append("KMSKey: ").append(getKMSKey()).append(",");
if (getTargetName() != null)
sb.append("TargetName: ").append(getTargetName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CachediSCSIVolume == false)
return false;
CachediSCSIVolume other = (CachediSCSIVolume) obj;
if (other.getVolumeARN() == null ^ this.getVolumeARN() == null)
return false;
if (other.getVolumeARN() != null && other.getVolumeARN().equals(this.getVolumeARN()) == false)
return false;
if (other.getVolumeId() == null ^ this.getVolumeId() == null)
return false;
if (other.getVolumeId() != null && other.getVolumeId().equals(this.getVolumeId()) == false)
return false;
if (other.getVolumeType() == null ^ this.getVolumeType() == null)
return false;
if (other.getVolumeType() != null && other.getVolumeType().equals(this.getVolumeType()) == false)
return false;
if (other.getVolumeStatus() == null ^ this.getVolumeStatus() == null)
return false;
if (other.getVolumeStatus() != null && other.getVolumeStatus().equals(this.getVolumeStatus()) == false)
return false;
if (other.getVolumeAttachmentStatus() == null ^ this.getVolumeAttachmentStatus() == null)
return false;
if (other.getVolumeAttachmentStatus() != null && other.getVolumeAttachmentStatus().equals(this.getVolumeAttachmentStatus()) == false)
return false;
if (other.getVolumeSizeInBytes() == null ^ this.getVolumeSizeInBytes() == null)
return false;
if (other.getVolumeSizeInBytes() != null && other.getVolumeSizeInBytes().equals(this.getVolumeSizeInBytes()) == false)
return false;
if (other.getVolumeProgress() == null ^ this.getVolumeProgress() == null)
return false;
if (other.getVolumeProgress() != null && other.getVolumeProgress().equals(this.getVolumeProgress()) == false)
return false;
if (other.getSourceSnapshotId() == null ^ this.getSourceSnapshotId() == null)
return false;
if (other.getSourceSnapshotId() != null && other.getSourceSnapshotId().equals(this.getSourceSnapshotId()) == false)
return false;
if (other.getVolumeiSCSIAttributes() == null ^ this.getVolumeiSCSIAttributes() == null)
return false;
if (other.getVolumeiSCSIAttributes() != null && other.getVolumeiSCSIAttributes().equals(this.getVolumeiSCSIAttributes()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getVolumeUsedInBytes() == null ^ this.getVolumeUsedInBytes() == null)
return false;
if (other.getVolumeUsedInBytes() != null && other.getVolumeUsedInBytes().equals(this.getVolumeUsedInBytes()) == false)
return false;
if (other.getKMSKey() == null ^ this.getKMSKey() == null)
return false;
if (other.getKMSKey() != null && other.getKMSKey().equals(this.getKMSKey()) == false)
return false;
if (other.getTargetName() == null ^ this.getTargetName() == null)
return false;
if (other.getTargetName() != null && other.getTargetName().equals(this.getTargetName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVolumeARN() == null) ? 0 : getVolumeARN().hashCode());
hashCode = prime * hashCode + ((getVolumeId() == null) ? 0 : getVolumeId().hashCode());
hashCode = prime * hashCode + ((getVolumeType() == null) ? 0 : getVolumeType().hashCode());
hashCode = prime * hashCode + ((getVolumeStatus() == null) ? 0 : getVolumeStatus().hashCode());
hashCode = prime * hashCode + ((getVolumeAttachmentStatus() == null) ? 0 : getVolumeAttachmentStatus().hashCode());
hashCode = prime * hashCode + ((getVolumeSizeInBytes() == null) ? 0 : getVolumeSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getVolumeProgress() == null) ? 0 : getVolumeProgress().hashCode());
hashCode = prime * hashCode + ((getSourceSnapshotId() == null) ? 0 : getSourceSnapshotId().hashCode());
hashCode = prime * hashCode + ((getVolumeiSCSIAttributes() == null) ? 0 : getVolumeiSCSIAttributes().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getVolumeUsedInBytes() == null) ? 0 : getVolumeUsedInBytes().hashCode());
hashCode = prime * hashCode + ((getKMSKey() == null) ? 0 : getKMSKey().hashCode());
hashCode = prime * hashCode + ((getTargetName() == null) ? 0 : getTargetName().hashCode());
return hashCode;
}
@Override
public CachediSCSIVolume clone() {
try {
return (CachediSCSIVolume) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.storagegateway.model.transform.CachediSCSIVolumeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}