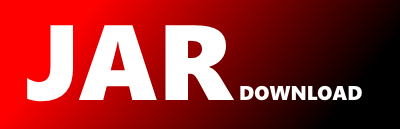
com.amazonaws.services.storagegateway.model.CreateTapeWithBarcodeRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-storagegateway Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.storagegateway.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* CreateTapeWithBarcodeInput
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTapeWithBarcodeRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use the
* ListGateways operation to return a list of gateways for your account and Amazon Web Services Region.
*
*/
private String gatewayARN;
/**
*
* The size, in bytes, of the virtual tape that you want to create.
*
*
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*
*/
private Long tapeSizeInBytes;
/**
*
* The barcode that you want to assign to the tape.
*
*
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*
*/
private String tapeBarcode;
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*/
private Boolean kMSEncrypted;
/**
*
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*
*/
private String kMSKey;
/**
*
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in the S3
* storage class that is associated with the pool. When you use your backup application to eject the tape, the tape
* is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that corresponds to the pool.
*
*/
private String poolId;
/**
*
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM) tape.
*
*/
private Boolean worm;
/**
*
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value pair.
*
*
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and the
* maximum length for a tag's value is 256.
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use the
* ListGateways operation to return a list of gateways for your account and Amazon Web Services Region.
*
*
* @param gatewayARN
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use
* the ListGateways operation to return a list of gateways for your account and Amazon Web Services
* Region.
*/
public void setGatewayARN(String gatewayARN) {
this.gatewayARN = gatewayARN;
}
/**
*
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use the
* ListGateways operation to return a list of gateways for your account and Amazon Web Services Region.
*
*
* @return The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use
* the ListGateways operation to return a list of gateways for your account and Amazon Web Services
* Region.
*/
public String getGatewayARN() {
return this.gatewayARN;
}
/**
*
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use the
* ListGateways operation to return a list of gateways for your account and Amazon Web Services Region.
*
*
* @param gatewayARN
* The unique Amazon Resource Name (ARN) that represents the gateway to associate the virtual tape with. Use
* the ListGateways operation to return a list of gateways for your account and Amazon Web Services
* Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withGatewayARN(String gatewayARN) {
setGatewayARN(gatewayARN);
return this;
}
/**
*
* The size, in bytes, of the virtual tape that you want to create.
*
*
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*
*
* @param tapeSizeInBytes
* The size, in bytes, of the virtual tape that you want to create.
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*/
public void setTapeSizeInBytes(Long tapeSizeInBytes) {
this.tapeSizeInBytes = tapeSizeInBytes;
}
/**
*
* The size, in bytes, of the virtual tape that you want to create.
*
*
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*
*
* @return The size, in bytes, of the virtual tape that you want to create.
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*/
public Long getTapeSizeInBytes() {
return this.tapeSizeInBytes;
}
/**
*
* The size, in bytes, of the virtual tape that you want to create.
*
*
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
*
*
* @param tapeSizeInBytes
* The size, in bytes, of the virtual tape that you want to create.
*
* The size must be aligned by gigabyte (1024*1024*1024 bytes).
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withTapeSizeInBytes(Long tapeSizeInBytes) {
setTapeSizeInBytes(tapeSizeInBytes);
return this;
}
/**
*
* The barcode that you want to assign to the tape.
*
*
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*
*
* @param tapeBarcode
* The barcode that you want to assign to the tape.
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*/
public void setTapeBarcode(String tapeBarcode) {
this.tapeBarcode = tapeBarcode;
}
/**
*
* The barcode that you want to assign to the tape.
*
*
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*
*
* @return The barcode that you want to assign to the tape.
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*/
public String getTapeBarcode() {
return this.tapeBarcode;
}
/**
*
* The barcode that you want to assign to the tape.
*
*
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
*
*
* @param tapeBarcode
* The barcode that you want to assign to the tape.
*
* Barcodes cannot be reused. This includes barcodes used for tapes that have been deleted.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withTapeBarcode(String tapeBarcode) {
setTapeBarcode(tapeBarcode);
return this;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @param kMSEncrypted
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public void setKMSEncrypted(Boolean kMSEncrypted) {
this.kMSEncrypted = kMSEncrypted;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @return Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public Boolean getKMSEncrypted() {
return this.kMSEncrypted;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @param kMSEncrypted
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withKMSEncrypted(Boolean kMSEncrypted) {
setKMSEncrypted(kMSEncrypted);
return this;
}
/**
*
* Set to true
to use Amazon S3 server-side encryption with your own KMS key, or false
to
* use a key managed by Amazon S3. Optional.
*
*
* Valid Values: true
| false
*
*
* @return Set to true
to use Amazon S3 server-side encryption with your own KMS key, or
* false
to use a key managed by Amazon S3. Optional.
*
* Valid Values: true
| false
*/
public Boolean isKMSEncrypted() {
return this.kMSEncrypted;
}
/**
*
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*
*
* @param kMSKey
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*/
public void setKMSKey(String kMSKey) {
this.kMSKey = kMSKey;
}
/**
*
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*
*
* @return The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*/
public String getKMSKey() {
return this.kMSKey;
}
/**
*
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
*
*
* @param kMSKey
* The Amazon Resource Name (ARN) of a symmetric customer master key (CMK) used for Amazon S3 server-side
* encryption. Storage Gateway does not support asymmetric CMKs. This value can only be set when
* KMSEncrypted
is true
. Optional.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withKMSKey(String kMSKey) {
setKMSKey(kMSKey);
return this;
}
/**
*
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in the S3
* storage class that is associated with the pool. When you use your backup application to eject the tape, the tape
* is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that corresponds to the pool.
*
*
* @param poolId
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in
* the S3 storage class that is associated with the pool. When you use your backup application to eject the
* tape, the tape is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that
* corresponds to the pool.
*/
public void setPoolId(String poolId) {
this.poolId = poolId;
}
/**
*
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in the S3
* storage class that is associated with the pool. When you use your backup application to eject the tape, the tape
* is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that corresponds to the pool.
*
*
* @return The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in
* the S3 storage class that is associated with the pool. When you use your backup application to eject the
* tape, the tape is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that
* corresponds to the pool.
*/
public String getPoolId() {
return this.poolId;
}
/**
*
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in the S3
* storage class that is associated with the pool. When you use your backup application to eject the tape, the tape
* is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that corresponds to the pool.
*
*
* @param poolId
* The ID of the pool that you want to add your tape to for archiving. The tape in this pool is archived in
* the S3 storage class that is associated with the pool. When you use your backup application to eject the
* tape, the tape is archived directly into the storage class (S3 Glacier or S3 Deep Archive) that
* corresponds to the pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withPoolId(String poolId) {
setPoolId(poolId);
return this;
}
/**
*
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM) tape.
*
*
* @param worm
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM)
* tape.
*/
public void setWorm(Boolean worm) {
this.worm = worm;
}
/**
*
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM) tape.
*
*
* @return Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many
* (WORM) tape.
*/
public Boolean getWorm() {
return this.worm;
}
/**
*
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM) tape.
*
*
* @param worm
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM)
* tape.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withWorm(Boolean worm) {
setWorm(worm);
return this;
}
/**
*
* Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many (WORM) tape.
*
*
* @return Set to TRUE
if the tape you are creating is to be configured as a write-once-read-many
* (WORM) tape.
*/
public Boolean isWorm() {
return this.worm;
}
/**
*
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value pair.
*
*
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and the
* maximum length for a tag's value is 256.
*
*
*
* @return A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a
* key-value pair.
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and
* the following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters,
* and the maximum length for a tag's value is 256.
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value pair.
*
*
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and the
* maximum length for a tag's value is 256.
*
*
*
* @param tags
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value
* pair.
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and
* the maximum length for a tag's value is 256.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value pair.
*
*
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and the
* maximum length for a tag's value is 256.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value
* pair.
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and
* the maximum length for a tag's value is 256.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value pair.
*
*
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and the
* maximum length for a tag's value is 256.
*
*
*
* @param tags
* A list of up to 50 tags that can be assigned to a virtual tape that has a barcode. Each tag is a key-value
* pair.
*
* Valid characters for key and value are letters, spaces, and numbers representable in UTF-8 format, and the
* following special characters: + - = . _ : / @. The maximum length of a tag's key is 128 characters, and
* the maximum length for a tag's value is 256.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTapeWithBarcodeRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getGatewayARN() != null)
sb.append("GatewayARN: ").append(getGatewayARN()).append(",");
if (getTapeSizeInBytes() != null)
sb.append("TapeSizeInBytes: ").append(getTapeSizeInBytes()).append(",");
if (getTapeBarcode() != null)
sb.append("TapeBarcode: ").append(getTapeBarcode()).append(",");
if (getKMSEncrypted() != null)
sb.append("KMSEncrypted: ").append(getKMSEncrypted()).append(",");
if (getKMSKey() != null)
sb.append("KMSKey: ").append(getKMSKey()).append(",");
if (getPoolId() != null)
sb.append("PoolId: ").append(getPoolId()).append(",");
if (getWorm() != null)
sb.append("Worm: ").append(getWorm()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTapeWithBarcodeRequest == false)
return false;
CreateTapeWithBarcodeRequest other = (CreateTapeWithBarcodeRequest) obj;
if (other.getGatewayARN() == null ^ this.getGatewayARN() == null)
return false;
if (other.getGatewayARN() != null && other.getGatewayARN().equals(this.getGatewayARN()) == false)
return false;
if (other.getTapeSizeInBytes() == null ^ this.getTapeSizeInBytes() == null)
return false;
if (other.getTapeSizeInBytes() != null && other.getTapeSizeInBytes().equals(this.getTapeSizeInBytes()) == false)
return false;
if (other.getTapeBarcode() == null ^ this.getTapeBarcode() == null)
return false;
if (other.getTapeBarcode() != null && other.getTapeBarcode().equals(this.getTapeBarcode()) == false)
return false;
if (other.getKMSEncrypted() == null ^ this.getKMSEncrypted() == null)
return false;
if (other.getKMSEncrypted() != null && other.getKMSEncrypted().equals(this.getKMSEncrypted()) == false)
return false;
if (other.getKMSKey() == null ^ this.getKMSKey() == null)
return false;
if (other.getKMSKey() != null && other.getKMSKey().equals(this.getKMSKey()) == false)
return false;
if (other.getPoolId() == null ^ this.getPoolId() == null)
return false;
if (other.getPoolId() != null && other.getPoolId().equals(this.getPoolId()) == false)
return false;
if (other.getWorm() == null ^ this.getWorm() == null)
return false;
if (other.getWorm() != null && other.getWorm().equals(this.getWorm()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getGatewayARN() == null) ? 0 : getGatewayARN().hashCode());
hashCode = prime * hashCode + ((getTapeSizeInBytes() == null) ? 0 : getTapeSizeInBytes().hashCode());
hashCode = prime * hashCode + ((getTapeBarcode() == null) ? 0 : getTapeBarcode().hashCode());
hashCode = prime * hashCode + ((getKMSEncrypted() == null) ? 0 : getKMSEncrypted().hashCode());
hashCode = prime * hashCode + ((getKMSKey() == null) ? 0 : getKMSKey().hashCode());
hashCode = prime * hashCode + ((getPoolId() == null) ? 0 : getPoolId().hashCode());
hashCode = prime * hashCode + ((getWorm() == null) ? 0 : getWorm().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateTapeWithBarcodeRequest clone() {
return (CreateTapeWithBarcodeRequest) super.clone();
}
}