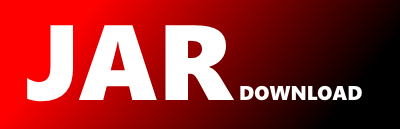
com.amazonaws.services.support.AWSSupportAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-support Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.support;
import com.amazonaws.services.support.model.*;
/**
* Interface for accessing AWS Support asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* AWS Support
*
* The AWS Support API reference is intended for programmers who need detailed
* information about the AWS Support operations and data types. This service
* enables you to manage your AWS Support cases programmatically. It uses HTTP
* methods that return results in JSON format.
*
*
* The AWS Support service also exposes a set of Trusted
* Advisor features. You can retrieve a list of checks and their
* descriptions, get check results, specify checks to refresh, and get the
* refresh status of checks.
*
*
* The following list describes the AWS Support case management operations:
*
*
* - Service names, issue categories, and available severity levels.
* The DescribeServices and DescribeSeverityLevels operations
* return AWS service names, service codes, service categories, and problem
* severity levels. You use these values when you call the CreateCase
* operation.
* - Case creation, case details, and case resolution. The
* CreateCase, DescribeCases, DescribeAttachment, and
* ResolveCase operations create AWS Support cases, retrieve information
* about cases, and resolve cases.
* - Case communication. The DescribeCommunications,
* AddCommunicationToCase, and AddAttachmentsToSet operations
* retrieve and add communications and attachments to AWS Support cases.
*
*
* The following list describes the operations available from the AWS Support
* service for Trusted Advisor:
*
*
* - DescribeTrustedAdvisorChecks returns the list of checks that run
* against your AWS resources.
* - Using the
CheckId
for a specific check returned by
* DescribeTrustedAdvisorChecks, you can call
* DescribeTrustedAdvisorCheckResult to obtain the results for the check
* you specified.
* - DescribeTrustedAdvisorCheckSummaries returns summarized results
* for one or more Trusted Advisor checks.
* - RefreshTrustedAdvisorCheck requests that Trusted Advisor rerun a
* specified check.
* - DescribeTrustedAdvisorCheckRefreshStatuses reports the refresh
* status of one or more checks.
*
*
* For authentication of requests, AWS Support uses Signature Version 4 Signing Process.
*
*
* See About
* the AWS Support API in the AWS Support User Guide for information
* about how to use this service to create and manage your support cases, and
* how to call Trusted Advisor for results of checks on your resources.
*
*/
public interface AWSSupportAsync extends AWSSupport {
/**
*
* Adds one or more attachments to an attachment set. If an
* AttachmentSetId
is not specified, a new attachment set is
* created, and the ID of the set is returned in the response. If an
* AttachmentSetId
is specified, the attachments are added to
* the specified set, if it exists.
*
*
* An attachment set is a temporary container for attachments that are to be
* added to a case or case communication. The set is available for one hour
* after it is created; the ExpiryTime
returned in the response
* indicates when the set expires. The maximum number of attachments in a
* set is 3, and the maximum size of any attachment in the set is 5 MB.
*
*
* @param addAttachmentsToSetRequest
* @return A Java Future containing the result of the AddAttachmentsToSet
* operation returned by the service.
* @sample AWSSupportAsync.AddAttachmentsToSet
*/
java.util.concurrent.Future addAttachmentsToSetAsync(
AddAttachmentsToSetRequest addAttachmentsToSetRequest);
/**
*
* Adds one or more attachments to an attachment set. If an
* AttachmentSetId
is not specified, a new attachment set is
* created, and the ID of the set is returned in the response. If an
* AttachmentSetId
is specified, the attachments are added to
* the specified set, if it exists.
*
*
* An attachment set is a temporary container for attachments that are to be
* added to a case or case communication. The set is available for one hour
* after it is created; the ExpiryTime
returned in the response
* indicates when the set expires. The maximum number of attachments in a
* set is 3, and the maximum size of any attachment in the set is 5 MB.
*
*
* @param addAttachmentsToSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddAttachmentsToSet
* operation returned by the service.
* @sample AWSSupportAsyncHandler.AddAttachmentsToSet
*/
java.util.concurrent.Future addAttachmentsToSetAsync(
AddAttachmentsToSetRequest addAttachmentsToSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds additional customer communication to an AWS Support case. You use
* the CaseId
value to identify the case to add communication
* to. You can list a set of email addresses to copy on the communication
* using the CcEmailAddresses
value. The
* CommunicationBody
value contains the text of the
* communication.
*
*
* The response indicates the success or failure of the request.
*
*
* This operation implements a subset of the features of the AWS Support
* Center.
*
*
* @param addCommunicationToCaseRequest
* To be written.
* @return A Java Future containing the result of the AddCommunicationToCase
* operation returned by the service.
* @sample AWSSupportAsync.AddCommunicationToCase
*/
java.util.concurrent.Future addCommunicationToCaseAsync(
AddCommunicationToCaseRequest addCommunicationToCaseRequest);
/**
*
* Adds additional customer communication to an AWS Support case. You use
* the CaseId
value to identify the case to add communication
* to. You can list a set of email addresses to copy on the communication
* using the CcEmailAddresses
value. The
* CommunicationBody
value contains the text of the
* communication.
*
*
* The response indicates the success or failure of the request.
*
*
* This operation implements a subset of the features of the AWS Support
* Center.
*
*
* @param addCommunicationToCaseRequest
* To be written.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddCommunicationToCase
* operation returned by the service.
* @sample AWSSupportAsyncHandler.AddCommunicationToCase
*/
java.util.concurrent.Future addCommunicationToCaseAsync(
AddCommunicationToCaseRequest addCommunicationToCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new case in the AWS Support Center. This operation is modeled
* on the behavior of the AWS Support Center Create
* Case page. Its parameters require you to specify the following
* information:
*
*
* - IssueType. The type of issue for the case. You can specify
* either "customer-service" or "technical." If you do not indicate a value,
* the default is "technical."
* - ServiceCode. The code for an AWS service. You obtain the
*
ServiceCode
by calling DescribeServices.
* - CategoryCode. The category for the service defined for the
*
ServiceCode
value. You also obtain the category code for a
* service by calling DescribeServices. Each AWS service defines its
* own set of category codes.
* - SeverityCode. A value that indicates the urgency of the case,
* which in turn determines the response time according to your service
* level agreement with AWS Support. You obtain the SeverityCode by calling
* DescribeSeverityLevels.
* - Subject. The Subject field on the AWS Support Center Create
* Case page.
* - CommunicationBody. The Description field on the AWS
* Support Center Create
* Case page.
* - AttachmentSetId. The ID of a set of attachments that has been
* created by using AddAttachmentsToSet.
* - Language. The human language in which AWS Support handles the
* case. English and Japanese are currently supported.
* - CcEmailAddresses. The AWS Support Center CC field on
* the Create
* Case page. You can list email addresses to be copied on any
* correspondence about the case. The account that opens the case is already
* identified by passing the AWS Credentials in the HTTP POST method or in a
* method or function call from one of the programming languages supported
* by an AWS SDK.
*
*
*
* To add additional communication or attachments to an existing case, use
* AddCommunicationToCase.
*
*
*
* A successful CreateCase request returns an AWS Support case
* number. Case numbers are used by the DescribeCases operation to
* retrieve existing AWS Support cases.
*
*
* @param createCaseRequest
* @return A Java Future containing the result of the CreateCase operation
* returned by the service.
* @sample AWSSupportAsync.CreateCase
*/
java.util.concurrent.Future createCaseAsync(
CreateCaseRequest createCaseRequest);
/**
*
* Creates a new case in the AWS Support Center. This operation is modeled
* on the behavior of the AWS Support Center Create
* Case page. Its parameters require you to specify the following
* information:
*
*
* - IssueType. The type of issue for the case. You can specify
* either "customer-service" or "technical." If you do not indicate a value,
* the default is "technical."
* - ServiceCode. The code for an AWS service. You obtain the
*
ServiceCode
by calling DescribeServices.
* - CategoryCode. The category for the service defined for the
*
ServiceCode
value. You also obtain the category code for a
* service by calling DescribeServices. Each AWS service defines its
* own set of category codes.
* - SeverityCode. A value that indicates the urgency of the case,
* which in turn determines the response time according to your service
* level agreement with AWS Support. You obtain the SeverityCode by calling
* DescribeSeverityLevels.
* - Subject. The Subject field on the AWS Support Center Create
* Case page.
* - CommunicationBody. The Description field on the AWS
* Support Center Create
* Case page.
* - AttachmentSetId. The ID of a set of attachments that has been
* created by using AddAttachmentsToSet.
* - Language. The human language in which AWS Support handles the
* case. English and Japanese are currently supported.
* - CcEmailAddresses. The AWS Support Center CC field on
* the Create
* Case page. You can list email addresses to be copied on any
* correspondence about the case. The account that opens the case is already
* identified by passing the AWS Credentials in the HTTP POST method or in a
* method or function call from one of the programming languages supported
* by an AWS SDK.
*
*
*
* To add additional communication or attachments to an existing case, use
* AddCommunicationToCase.
*
*
*
* A successful CreateCase request returns an AWS Support case
* number. Case numbers are used by the DescribeCases operation to
* retrieve existing AWS Support cases.
*
*
* @param createCaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCase operation
* returned by the service.
* @sample AWSSupportAsyncHandler.CreateCase
*/
java.util.concurrent.Future createCaseAsync(
CreateCaseRequest createCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the attachment that has the specified ID. Attachment IDs are
* generated by the case management system when you add an attachment to a
* case or case communication. Attachment IDs are returned in the
* AttachmentDetails objects that are returned by the
* DescribeCommunications operation.
*
*
* @param describeAttachmentRequest
* @return A Java Future containing the result of the DescribeAttachment
* operation returned by the service.
* @sample AWSSupportAsync.DescribeAttachment
*/
java.util.concurrent.Future describeAttachmentAsync(
DescribeAttachmentRequest describeAttachmentRequest);
/**
*
* Returns the attachment that has the specified ID. Attachment IDs are
* generated by the case management system when you add an attachment to a
* case or case communication. Attachment IDs are returned in the
* AttachmentDetails objects that are returned by the
* DescribeCommunications operation.
*
*
* @param describeAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAttachment
* operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeAttachment
*/
java.util.concurrent.Future describeAttachmentAsync(
DescribeAttachmentRequest describeAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of cases that you specify by passing one or more case IDs.
* In addition, you can filter the cases by date by setting values for the
* AfterTime
and BeforeTime
request parameters.
* You can set values for the IncludeResolvedCases
and
* IncludeCommunications
request parameters to control how much
* information is returned.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* The response returns the following in JSON format:
*
*
* - One or more CaseDetails data types.
* - One or more
NextToken
values, which specify where to
* paginate the returned records represented by the CaseDetails
* objects.
*
*
* @param describeCasesRequest
* @return A Java Future containing the result of the DescribeCases
* operation returned by the service.
* @sample AWSSupportAsync.DescribeCases
*/
java.util.concurrent.Future describeCasesAsync(
DescribeCasesRequest describeCasesRequest);
/**
*
* Returns a list of cases that you specify by passing one or more case IDs.
* In addition, you can filter the cases by date by setting values for the
* AfterTime
and BeforeTime
request parameters.
* You can set values for the IncludeResolvedCases
and
* IncludeCommunications
request parameters to control how much
* information is returned.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* The response returns the following in JSON format:
*
*
* - One or more CaseDetails data types.
* - One or more
NextToken
values, which specify where to
* paginate the returned records represented by the CaseDetails
* objects.
*
*
* @param describeCasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCases
* operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeCases
*/
java.util.concurrent.Future describeCasesAsync(
DescribeCasesRequest describeCasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCases operation.
*
* @see #describeCasesAsync(DescribeCasesRequest)
*/
java.util.concurrent.Future describeCasesAsync();
/**
* Simplified method form for invoking the DescribeCases operation with an
* AsyncHandler.
*
* @see #describeCasesAsync(DescribeCasesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCasesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns communications (and attachments) for one or more support cases.
* You can use the AfterTime
and BeforeTime
* parameters to filter by date. You can use the CaseId
* parameter to restrict the results to a particular case.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* You can use the MaxResults
and NextToken
* parameters to control the pagination of the result set. Set
* MaxResults
to the number of cases you want displayed on each
* page, and use NextToken
to specify the resumption of
* pagination.
*
*
* @param describeCommunicationsRequest
* @return A Java Future containing the result of the DescribeCommunications
* operation returned by the service.
* @sample AWSSupportAsync.DescribeCommunications
*/
java.util.concurrent.Future describeCommunicationsAsync(
DescribeCommunicationsRequest describeCommunicationsRequest);
/**
*
* Returns communications (and attachments) for one or more support cases.
* You can use the AfterTime
and BeforeTime
* parameters to filter by date. You can use the CaseId
* parameter to restrict the results to a particular case.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* You can use the MaxResults
and NextToken
* parameters to control the pagination of the result set. Set
* MaxResults
to the number of cases you want displayed on each
* page, and use NextToken
to specify the resumption of
* pagination.
*
*
* @param describeCommunicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCommunications
* operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeCommunications
*/
java.util.concurrent.Future describeCommunicationsAsync(
DescribeCommunicationsRequest describeCommunicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the current list of AWS services and a list of service categories
* that applies to each one. You then use service names and categories in
* your CreateCase requests. Each AWS service has its own set of
* categories.
*
*
* The service codes and category codes correspond to the values that are
* displayed in the Service and Category drop-down lists on
* the AWS Support Center Create
* Case page. The values in those fields, however, do not necessarily
* match the service codes and categories returned by the
* DescribeServices
request. Always use the service codes and
* categories obtained programmatically. This practice ensures that you
* always have the most recent set of service and category codes.
*
*
* @param describeServicesRequest
* @return A Java Future containing the result of the DescribeServices
* operation returned by the service.
* @sample AWSSupportAsync.DescribeServices
*/
java.util.concurrent.Future describeServicesAsync(
DescribeServicesRequest describeServicesRequest);
/**
*
* Returns the current list of AWS services and a list of service categories
* that applies to each one. You then use service names and categories in
* your CreateCase requests. Each AWS service has its own set of
* categories.
*
*
* The service codes and category codes correspond to the values that are
* displayed in the Service and Category drop-down lists on
* the AWS Support Center Create
* Case page. The values in those fields, however, do not necessarily
* match the service codes and categories returned by the
* DescribeServices
request. Always use the service codes and
* categories obtained programmatically. This practice ensures that you
* always have the most recent set of service and category codes.
*
*
* @param describeServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServices
* operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeServices
*/
java.util.concurrent.Future describeServicesAsync(
DescribeServicesRequest describeServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeServices operation.
*
* @see #describeServicesAsync(DescribeServicesRequest)
*/
java.util.concurrent.Future describeServicesAsync();
/**
* Simplified method form for invoking the DescribeServices operation with
* an AsyncHandler.
*
* @see #describeServicesAsync(DescribeServicesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeServicesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of severity levels that you can assign to an AWS Support
* case. The severity level for a case is also a field in the
* CaseDetails data type included in any CreateCase request.
*
*
* @param describeSeverityLevelsRequest
* @return A Java Future containing the result of the DescribeSeverityLevels
* operation returned by the service.
* @sample AWSSupportAsync.DescribeSeverityLevels
*/
java.util.concurrent.Future describeSeverityLevelsAsync(
DescribeSeverityLevelsRequest describeSeverityLevelsRequest);
/**
*
* Returns the list of severity levels that you can assign to an AWS Support
* case. The severity level for a case is also a field in the
* CaseDetails data type included in any CreateCase request.
*
*
* @param describeSeverityLevelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSeverityLevels
* operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeSeverityLevels
*/
java.util.concurrent.Future describeSeverityLevelsAsync(
DescribeSeverityLevelsRequest describeSeverityLevelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeSeverityLevels operation.
*
* @see #describeSeverityLevelsAsync(DescribeSeverityLevelsRequest)
*/
java.util.concurrent.Future describeSeverityLevelsAsync();
/**
* Simplified method form for invoking the DescribeSeverityLevels operation
* with an AsyncHandler.
*
* @see #describeSeverityLevelsAsync(DescribeSeverityLevelsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeSeverityLevelsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the refresh status of the Trusted Advisor checks that have the
* specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* @param describeTrustedAdvisorCheckRefreshStatusesRequest
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckRefreshStatuses operation returned by
* the service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckRefreshStatuses
*/
java.util.concurrent.Future describeTrustedAdvisorCheckRefreshStatusesAsync(
DescribeTrustedAdvisorCheckRefreshStatusesRequest describeTrustedAdvisorCheckRefreshStatusesRequest);
/**
*
* Returns the refresh status of the Trusted Advisor checks that have the
* specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* @param describeTrustedAdvisorCheckRefreshStatusesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckRefreshStatuses operation returned by
* the service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckRefreshStatuses
*/
java.util.concurrent.Future describeTrustedAdvisorCheckRefreshStatusesAsync(
DescribeTrustedAdvisorCheckRefreshStatusesRequest describeTrustedAdvisorCheckRefreshStatusesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the results of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckResult object, which
* contains these three objects:
*
*
* - TrustedAdvisorCategorySpecificSummary
* - TrustedAdvisorResourceDetail
* - TrustedAdvisorResourcesSummary
*
*
* In addition, the response contains these fields:
*
*
* - Status. The alert status of the check: "ok" (green), "warning"
* (yellow), "error" (red), or "not_available".
* - Timestamp. The time of the last refresh of the check.
* - CheckId. The unique identifier for the check.
*
*
* @param describeTrustedAdvisorCheckResultRequest
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckResult operation returned by the
* service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckResult
*/
java.util.concurrent.Future describeTrustedAdvisorCheckResultAsync(
DescribeTrustedAdvisorCheckResultRequest describeTrustedAdvisorCheckResultRequest);
/**
*
* Returns the results of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckResult object, which
* contains these three objects:
*
*
* - TrustedAdvisorCategorySpecificSummary
* - TrustedAdvisorResourceDetail
* - TrustedAdvisorResourcesSummary
*
*
* In addition, the response contains these fields:
*
*
* - Status. The alert status of the check: "ok" (green), "warning"
* (yellow), "error" (red), or "not_available".
* - Timestamp. The time of the last refresh of the check.
* - CheckId. The unique identifier for the check.
*
*
* @param describeTrustedAdvisorCheckResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckResult operation returned by the
* service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckResult
*/
java.util.concurrent.Future describeTrustedAdvisorCheckResultAsync(
DescribeTrustedAdvisorCheckResultRequest describeTrustedAdvisorCheckResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the summaries of the results of the Trusted Advisor checks that
* have the specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains an array of TrustedAdvisorCheckSummary
* objects.
*
*
* @param describeTrustedAdvisorCheckSummariesRequest
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckSummaries operation returned by the
* service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckSummaries
*/
java.util.concurrent.Future describeTrustedAdvisorCheckSummariesAsync(
DescribeTrustedAdvisorCheckSummariesRequest describeTrustedAdvisorCheckSummariesRequest);
/**
*
* Returns the summaries of the results of the Trusted Advisor checks that
* have the specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains an array of TrustedAdvisorCheckSummary
* objects.
*
*
* @param describeTrustedAdvisorCheckSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorCheckSummaries operation returned by the
* service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckSummaries
*/
java.util.concurrent.Future describeTrustedAdvisorCheckSummariesAsync(
DescribeTrustedAdvisorCheckSummariesRequest describeTrustedAdvisorCheckSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about all available Trusted Advisor checks, including
* name, ID, category, description, and metadata. You must specify a
* language code; English ("en") and Japanese ("ja") are currently
* supported. The response contains a TrustedAdvisorCheckDescription
* for each check.
*
*
* @param describeTrustedAdvisorChecksRequest
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorChecks operation returned by the service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorChecks
*/
java.util.concurrent.Future describeTrustedAdvisorChecksAsync(
DescribeTrustedAdvisorChecksRequest describeTrustedAdvisorChecksRequest);
/**
*
* Returns information about all available Trusted Advisor checks, including
* name, ID, category, description, and metadata. You must specify a
* language code; English ("en") and Japanese ("ja") are currently
* supported. The response contains a TrustedAdvisorCheckDescription
* for each check.
*
*
* @param describeTrustedAdvisorChecksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeTrustedAdvisorChecks operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorChecks
*/
java.util.concurrent.Future describeTrustedAdvisorChecksAsync(
DescribeTrustedAdvisorChecksRequest describeTrustedAdvisorChecksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Requests a refresh of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckRefreshStatus object,
* which contains these fields:
*
*
* - Status. The refresh status of the check: "none", "enqueued",
* "processing", "success", or "abandoned".
* - MillisUntilNextRefreshable. The amount of time, in
* milliseconds, until the check is eligible for refresh.
* - CheckId. The unique identifier for the check.
*
*
* @param refreshTrustedAdvisorCheckRequest
* @return A Java Future containing the result of the
* RefreshTrustedAdvisorCheck operation returned by the service.
* @sample AWSSupportAsync.RefreshTrustedAdvisorCheck
*/
java.util.concurrent.Future refreshTrustedAdvisorCheckAsync(
RefreshTrustedAdvisorCheckRequest refreshTrustedAdvisorCheckRequest);
/**
*
* Requests a refresh of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckRefreshStatus object,
* which contains these fields:
*
*
* - Status. The refresh status of the check: "none", "enqueued",
* "processing", "success", or "abandoned".
* - MillisUntilNextRefreshable. The amount of time, in
* milliseconds, until the check is eligible for refresh.
* - CheckId. The unique identifier for the check.
*
*
* @param refreshTrustedAdvisorCheckRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RefreshTrustedAdvisorCheck operation returned by the service.
* @sample AWSSupportAsyncHandler.RefreshTrustedAdvisorCheck
*/
java.util.concurrent.Future refreshTrustedAdvisorCheckAsync(
RefreshTrustedAdvisorCheckRequest refreshTrustedAdvisorCheckRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Takes a CaseId
and returns the initial state of the case
* along with the state of the case after the call to ResolveCase
* completed.
*
*
* @param resolveCaseRequest
* @return A Java Future containing the result of the ResolveCase operation
* returned by the service.
* @sample AWSSupportAsync.ResolveCase
*/
java.util.concurrent.Future resolveCaseAsync(
ResolveCaseRequest resolveCaseRequest);
/**
*
* Takes a CaseId
and returns the initial state of the case
* along with the state of the case after the call to ResolveCase
* completed.
*
*
* @param resolveCaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResolveCase operation
* returned by the service.
* @sample AWSSupportAsyncHandler.ResolveCase
*/
java.util.concurrent.Future resolveCaseAsync(
ResolveCaseRequest resolveCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ResolveCase operation.
*
* @see #resolveCaseAsync(ResolveCaseRequest)
*/
java.util.concurrent.Future resolveCaseAsync();
/**
* Simplified method form for invoking the ResolveCase operation with an
* AsyncHandler.
*
* @see #resolveCaseAsync(ResolveCaseRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future resolveCaseAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
}