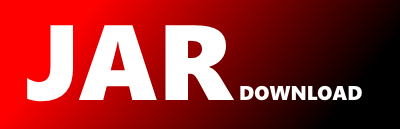
com.amazonaws.services.support.model.CreateCaseRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-support Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.support.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class CreateCaseRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The title of the AWS Support case.
*
*/
private String subject;
/**
*
* The code for the AWS service returned by the call to
* DescribeServices.
*
*/
private String serviceCode;
/**
*
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
*
*
* The availability of severity levels depends on each customer's support
* subscription. In other words, your subscription may not necessarily
* require the urgent level of response time.
*
*
*/
private String severityCode;
/**
*
* The category of problem for the AWS Support case.
*
*/
private String categoryCode;
/**
*
* The communication body text when you create an AWS Support case by
* calling CreateCase.
*
*/
private String communicationBody;
/**
*
* A list of email addresses that AWS Support copies on case correspondence.
*
*/
private com.amazonaws.internal.SdkInternalList ccEmailAddresses;
/**
*
* The ISO 639-1 code for the language in which AWS provides support. AWS
* Support currently supports English ("en") and Japanese ("ja"). Language
* parameters must be passed explicitly for operations that take them.
*
*/
private String language;
/**
*
* The type of issue for the case. You can specify either "customer-service"
* or "technical." If you do not indicate a value, the default is
* "technical."
*
*/
private String issueType;
/**
*
* The ID of a set of one or more attachments for the case. Create the set
* by using AddAttachmentsToSet.
*
*/
private String attachmentSetId;
/**
*
* The title of the AWS Support case.
*
*
* @param subject
* The title of the AWS Support case.
*/
public void setSubject(String subject) {
this.subject = subject;
}
/**
*
* The title of the AWS Support case.
*
*
* @return The title of the AWS Support case.
*/
public String getSubject() {
return this.subject;
}
/**
*
* The title of the AWS Support case.
*
*
* @param subject
* The title of the AWS Support case.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withSubject(String subject) {
setSubject(subject);
return this;
}
/**
*
* The code for the AWS service returned by the call to
* DescribeServices.
*
*
* @param serviceCode
* The code for the AWS service returned by the call to
* DescribeServices.
*/
public void setServiceCode(String serviceCode) {
this.serviceCode = serviceCode;
}
/**
*
* The code for the AWS service returned by the call to
* DescribeServices.
*
*
* @return The code for the AWS service returned by the call to
* DescribeServices.
*/
public String getServiceCode() {
return this.serviceCode;
}
/**
*
* The code for the AWS service returned by the call to
* DescribeServices.
*
*
* @param serviceCode
* The code for the AWS service returned by the call to
* DescribeServices.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withServiceCode(String serviceCode) {
setServiceCode(serviceCode);
return this;
}
/**
*
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
*
*
* The availability of severity levels depends on each customer's support
* subscription. In other words, your subscription may not necessarily
* require the urgent level of response time.
*
*
*
* @param severityCode
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
* The availability of severity levels depends on each customer's
* support subscription. In other words, your subscription may not
* necessarily require the urgent level of response time.
*
*/
public void setSeverityCode(String severityCode) {
this.severityCode = severityCode;
}
/**
*
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
*
*
* The availability of severity levels depends on each customer's support
* subscription. In other words, your subscription may not necessarily
* require the urgent level of response time.
*
*
*
* @return The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
* The availability of severity levels depends on each customer's
* support subscription. In other words, your subscription may not
* necessarily require the urgent level of response time.
*
*/
public String getSeverityCode() {
return this.severityCode;
}
/**
*
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
*
*
* The availability of severity levels depends on each customer's support
* subscription. In other words, your subscription may not necessarily
* require the urgent level of response time.
*
*
*
* @param severityCode
* The code for the severity level returned by the call to
* DescribeSeverityLevels.
*
* The availability of severity levels depends on each customer's
* support subscription. In other words, your subscription may not
* necessarily require the urgent level of response time.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withSeverityCode(String severityCode) {
setSeverityCode(severityCode);
return this;
}
/**
*
* The category of problem for the AWS Support case.
*
*
* @param categoryCode
* The category of problem for the AWS Support case.
*/
public void setCategoryCode(String categoryCode) {
this.categoryCode = categoryCode;
}
/**
*
* The category of problem for the AWS Support case.
*
*
* @return The category of problem for the AWS Support case.
*/
public String getCategoryCode() {
return this.categoryCode;
}
/**
*
* The category of problem for the AWS Support case.
*
*
* @param categoryCode
* The category of problem for the AWS Support case.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withCategoryCode(String categoryCode) {
setCategoryCode(categoryCode);
return this;
}
/**
*
* The communication body text when you create an AWS Support case by
* calling CreateCase.
*
*
* @param communicationBody
* The communication body text when you create an AWS Support case by
* calling CreateCase.
*/
public void setCommunicationBody(String communicationBody) {
this.communicationBody = communicationBody;
}
/**
*
* The communication body text when you create an AWS Support case by
* calling CreateCase.
*
*
* @return The communication body text when you create an AWS Support case
* by calling CreateCase.
*/
public String getCommunicationBody() {
return this.communicationBody;
}
/**
*
* The communication body text when you create an AWS Support case by
* calling CreateCase.
*
*
* @param communicationBody
* The communication body text when you create an AWS Support case by
* calling CreateCase.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withCommunicationBody(String communicationBody) {
setCommunicationBody(communicationBody);
return this;
}
/**
*
* A list of email addresses that AWS Support copies on case correspondence.
*
*
* @return A list of email addresses that AWS Support copies on case
* correspondence.
*/
public java.util.List getCcEmailAddresses() {
if (ccEmailAddresses == null) {
ccEmailAddresses = new com.amazonaws.internal.SdkInternalList();
}
return ccEmailAddresses;
}
/**
*
* A list of email addresses that AWS Support copies on case correspondence.
*
*
* @param ccEmailAddresses
* A list of email addresses that AWS Support copies on case
* correspondence.
*/
public void setCcEmailAddresses(
java.util.Collection ccEmailAddresses) {
if (ccEmailAddresses == null) {
this.ccEmailAddresses = null;
return;
}
this.ccEmailAddresses = new com.amazonaws.internal.SdkInternalList(
ccEmailAddresses);
}
/**
*
* A list of email addresses that AWS Support copies on case correspondence.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setCcEmailAddresses(java.util.Collection)} or
* {@link #withCcEmailAddresses(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param ccEmailAddresses
* A list of email addresses that AWS Support copies on case
* correspondence.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withCcEmailAddresses(String... ccEmailAddresses) {
if (this.ccEmailAddresses == null) {
setCcEmailAddresses(new com.amazonaws.internal.SdkInternalList(
ccEmailAddresses.length));
}
for (String ele : ccEmailAddresses) {
this.ccEmailAddresses.add(ele);
}
return this;
}
/**
*
* A list of email addresses that AWS Support copies on case correspondence.
*
*
* @param ccEmailAddresses
* A list of email addresses that AWS Support copies on case
* correspondence.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withCcEmailAddresses(
java.util.Collection ccEmailAddresses) {
setCcEmailAddresses(ccEmailAddresses);
return this;
}
/**
*
* The ISO 639-1 code for the language in which AWS provides support. AWS
* Support currently supports English ("en") and Japanese ("ja"). Language
* parameters must be passed explicitly for operations that take them.
*
*
* @param language
* The ISO 639-1 code for the language in which AWS provides support.
* AWS Support currently supports English ("en") and Japanese ("ja").
* Language parameters must be passed explicitly for operations that
* take them.
*/
public void setLanguage(String language) {
this.language = language;
}
/**
*
* The ISO 639-1 code for the language in which AWS provides support. AWS
* Support currently supports English ("en") and Japanese ("ja"). Language
* parameters must be passed explicitly for operations that take them.
*
*
* @return The ISO 639-1 code for the language in which AWS provides
* support. AWS Support currently supports English ("en") and
* Japanese ("ja"). Language parameters must be passed explicitly
* for operations that take them.
*/
public String getLanguage() {
return this.language;
}
/**
*
* The ISO 639-1 code for the language in which AWS provides support. AWS
* Support currently supports English ("en") and Japanese ("ja"). Language
* parameters must be passed explicitly for operations that take them.
*
*
* @param language
* The ISO 639-1 code for the language in which AWS provides support.
* AWS Support currently supports English ("en") and Japanese ("ja").
* Language parameters must be passed explicitly for operations that
* take them.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withLanguage(String language) {
setLanguage(language);
return this;
}
/**
*
* The type of issue for the case. You can specify either "customer-service"
* or "technical." If you do not indicate a value, the default is
* "technical."
*
*
* @param issueType
* The type of issue for the case. You can specify either
* "customer-service" or "technical." If you do not indicate a value,
* the default is "technical."
*/
public void setIssueType(String issueType) {
this.issueType = issueType;
}
/**
*
* The type of issue for the case. You can specify either "customer-service"
* or "technical." If you do not indicate a value, the default is
* "technical."
*
*
* @return The type of issue for the case. You can specify either
* "customer-service" or "technical." If you do not indicate a
* value, the default is "technical."
*/
public String getIssueType() {
return this.issueType;
}
/**
*
* The type of issue for the case. You can specify either "customer-service"
* or "technical." If you do not indicate a value, the default is
* "technical."
*
*
* @param issueType
* The type of issue for the case. You can specify either
* "customer-service" or "technical." If you do not indicate a value,
* the default is "technical."
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withIssueType(String issueType) {
setIssueType(issueType);
return this;
}
/**
*
* The ID of a set of one or more attachments for the case. Create the set
* by using AddAttachmentsToSet.
*
*
* @param attachmentSetId
* The ID of a set of one or more attachments for the case. Create
* the set by using AddAttachmentsToSet.
*/
public void setAttachmentSetId(String attachmentSetId) {
this.attachmentSetId = attachmentSetId;
}
/**
*
* The ID of a set of one or more attachments for the case. Create the set
* by using AddAttachmentsToSet.
*
*
* @return The ID of a set of one or more attachments for the case. Create
* the set by using AddAttachmentsToSet.
*/
public String getAttachmentSetId() {
return this.attachmentSetId;
}
/**
*
* The ID of a set of one or more attachments for the case. Create the set
* by using AddAttachmentsToSet.
*
*
* @param attachmentSetId
* The ID of a set of one or more attachments for the case. Create
* the set by using AddAttachmentsToSet.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public CreateCaseRequest withAttachmentSetId(String attachmentSetId) {
setAttachmentSetId(attachmentSetId);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSubject() != null)
sb.append("Subject: " + getSubject() + ",");
if (getServiceCode() != null)
sb.append("ServiceCode: " + getServiceCode() + ",");
if (getSeverityCode() != null)
sb.append("SeverityCode: " + getSeverityCode() + ",");
if (getCategoryCode() != null)
sb.append("CategoryCode: " + getCategoryCode() + ",");
if (getCommunicationBody() != null)
sb.append("CommunicationBody: " + getCommunicationBody() + ",");
if (getCcEmailAddresses() != null)
sb.append("CcEmailAddresses: " + getCcEmailAddresses() + ",");
if (getLanguage() != null)
sb.append("Language: " + getLanguage() + ",");
if (getIssueType() != null)
sb.append("IssueType: " + getIssueType() + ",");
if (getAttachmentSetId() != null)
sb.append("AttachmentSetId: " + getAttachmentSetId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCaseRequest == false)
return false;
CreateCaseRequest other = (CreateCaseRequest) obj;
if (other.getSubject() == null ^ this.getSubject() == null)
return false;
if (other.getSubject() != null
&& other.getSubject().equals(this.getSubject()) == false)
return false;
if (other.getServiceCode() == null ^ this.getServiceCode() == null)
return false;
if (other.getServiceCode() != null
&& other.getServiceCode().equals(this.getServiceCode()) == false)
return false;
if (other.getSeverityCode() == null ^ this.getSeverityCode() == null)
return false;
if (other.getSeverityCode() != null
&& other.getSeverityCode().equals(this.getSeverityCode()) == false)
return false;
if (other.getCategoryCode() == null ^ this.getCategoryCode() == null)
return false;
if (other.getCategoryCode() != null
&& other.getCategoryCode().equals(this.getCategoryCode()) == false)
return false;
if (other.getCommunicationBody() == null
^ this.getCommunicationBody() == null)
return false;
if (other.getCommunicationBody() != null
&& other.getCommunicationBody().equals(
this.getCommunicationBody()) == false)
return false;
if (other.getCcEmailAddresses() == null
^ this.getCcEmailAddresses() == null)
return false;
if (other.getCcEmailAddresses() != null
&& other.getCcEmailAddresses().equals(
this.getCcEmailAddresses()) == false)
return false;
if (other.getLanguage() == null ^ this.getLanguage() == null)
return false;
if (other.getLanguage() != null
&& other.getLanguage().equals(this.getLanguage()) == false)
return false;
if (other.getIssueType() == null ^ this.getIssueType() == null)
return false;
if (other.getIssueType() != null
&& other.getIssueType().equals(this.getIssueType()) == false)
return false;
if (other.getAttachmentSetId() == null
^ this.getAttachmentSetId() == null)
return false;
if (other.getAttachmentSetId() != null
&& other.getAttachmentSetId().equals(this.getAttachmentSetId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getSubject() == null) ? 0 : getSubject().hashCode());
hashCode = prime
* hashCode
+ ((getServiceCode() == null) ? 0 : getServiceCode().hashCode());
hashCode = prime
* hashCode
+ ((getSeverityCode() == null) ? 0 : getSeverityCode()
.hashCode());
hashCode = prime
* hashCode
+ ((getCategoryCode() == null) ? 0 : getCategoryCode()
.hashCode());
hashCode = prime
* hashCode
+ ((getCommunicationBody() == null) ? 0
: getCommunicationBody().hashCode());
hashCode = prime
* hashCode
+ ((getCcEmailAddresses() == null) ? 0 : getCcEmailAddresses()
.hashCode());
hashCode = prime * hashCode
+ ((getLanguage() == null) ? 0 : getLanguage().hashCode());
hashCode = prime * hashCode
+ ((getIssueType() == null) ? 0 : getIssueType().hashCode());
hashCode = prime
* hashCode
+ ((getAttachmentSetId() == null) ? 0 : getAttachmentSetId()
.hashCode());
return hashCode;
}
@Override
public CreateCaseRequest clone() {
return (CreateCaseRequest) super.clone();
}
}