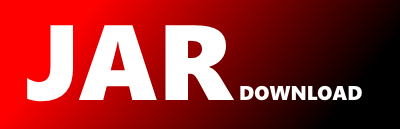
com.amazonaws.services.support.AWSSupportClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-support Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.support;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.services.support.model.*;
import com.amazonaws.services.support.model.transform.*;
/**
* Client for accessing AWS Support. All service calls made using this client
* are blocking, and will not return until the service call completes.
*
* AWS Support
*
* The AWS Support API reference is intended for programmers who need detailed
* information about the AWS Support operations and data types. This service
* enables you to manage your AWS Support cases programmatically. It uses HTTP
* methods that return results in JSON format.
*
*
* The AWS Support service also exposes a set of Trusted
* Advisor features. You can retrieve a list of checks and their
* descriptions, get check results, specify checks to refresh, and get the
* refresh status of checks.
*
*
* The following list describes the AWS Support case management operations:
*
*
* - Service names, issue categories, and available severity levels.
* The DescribeServices and DescribeSeverityLevels operations
* return AWS service names, service codes, service categories, and problem
* severity levels. You use these values when you call the CreateCase
* operation.
* - Case creation, case details, and case resolution. The
* CreateCase, DescribeCases, DescribeAttachment, and
* ResolveCase operations create AWS Support cases, retrieve information
* about cases, and resolve cases.
* - Case communication. The DescribeCommunications,
* AddCommunicationToCase, and AddAttachmentsToSet operations
* retrieve and add communications and attachments to AWS Support cases.
*
*
* The following list describes the operations available from the AWS Support
* service for Trusted Advisor:
*
*
* - DescribeTrustedAdvisorChecks returns the list of checks that run
* against your AWS resources.
* - Using the
CheckId
for a specific check returned by
* DescribeTrustedAdvisorChecks, you can call
* DescribeTrustedAdvisorCheckResult to obtain the results for the check
* you specified.
* - DescribeTrustedAdvisorCheckSummaries returns summarized results
* for one or more Trusted Advisor checks.
* - RefreshTrustedAdvisorCheck requests that Trusted Advisor rerun a
* specified check.
* - DescribeTrustedAdvisorCheckRefreshStatuses reports the refresh
* status of one or more checks.
*
*
* For authentication of requests, AWS Support uses Signature Version 4 Signing Process.
*
*
* See About
* the AWS Support API in the AWS Support User Guide for information
* about how to use this service to create and manage your support cases, and
* how to call Trusted Advisor for results of checks on your resources.
*
*/
@ThreadSafe
public class AWSSupportClient extends AmazonWebServiceClient implements
AWSSupport {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSSupport.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "support";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "support";
/**
* Client configuration factory providing ClientConfigurations tailored to
* this client
*/
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final SdkJsonProtocolFactory protocolFactory = new SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("AttachmentSetIdNotFound")
.withModeledClass(
com.amazonaws.services.support.model.AttachmentSetIdNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("AttachmentIdNotFound")
.withModeledClass(
com.amazonaws.services.support.model.AttachmentIdNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("InternalServerError")
.withModeledClass(
com.amazonaws.services.support.model.InternalServerErrorException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"DescribeAttachmentLimitExceeded")
.withModeledClass(
com.amazonaws.services.support.model.DescribeAttachmentLimitExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("AttachmentSetExpired")
.withModeledClass(
com.amazonaws.services.support.model.AttachmentSetExpiredException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("AttachmentLimitExceeded")
.withModeledClass(
com.amazonaws.services.support.model.AttachmentLimitExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("CaseIdNotFound")
.withModeledClass(
com.amazonaws.services.support.model.CaseIdNotFoundException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("CaseCreationLimitExceeded")
.withModeledClass(
com.amazonaws.services.support.model.CaseCreationLimitExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"AttachmentSetSizeLimitExceeded")
.withModeledClass(
com.amazonaws.services.support.model.AttachmentSetSizeLimitExceededException.class)));
/**
* Constructs a new client to invoke service methods on AWS Support. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSSupportClient() {
this(new DefaultAWSCredentialsProviderChain(), configFactory
.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Support. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Support (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSSupportClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWS Support using
* the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public AWSSupportClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Support using
* the specified AWS account credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Support (ex: proxy settings, retry counts, etc.).
*/
public AWSSupportClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(
awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on AWS Support using
* the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
*/
public AWSSupportClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS Support using
* the specified AWS account credentials provider and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Support (ex: proxy settings, retry counts, etc.).
*/
public AWSSupportClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWS Support using
* the specified AWS account credentials provider, client configuration
* options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS Support (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AWSSupportClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://support.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s
.addAll(chainFactory
.newRequestHandlerChain("/com/amazonaws/services/support/request.handlers"));
requestHandler2s
.addAll(chainFactory
.newRequestHandler2Chain("/com/amazonaws/services/support/request.handler2s"));
}
/**
*
* Adds one or more attachments to an attachment set. If an
* AttachmentSetId
is not specified, a new attachment set is
* created, and the ID of the set is returned in the response. If an
* AttachmentSetId
is specified, the attachments are added to
* the specified set, if it exists.
*
*
* An attachment set is a temporary container for attachments that are to be
* added to a case or case communication. The set is available for one hour
* after it is created; the ExpiryTime
returned in the response
* indicates when the set expires. The maximum number of attachments in a
* set is 3, and the maximum size of any attachment in the set is 5 MB.
*
*
* @param addAttachmentsToSetRequest
* @return Result of the AddAttachmentsToSet operation returned by the
* service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws AttachmentSetIdNotFoundException
* An attachment set with the specified ID could not be found.
* @throws AttachmentSetExpiredException
* The expiration time of the attachment set has passed. The set
* expires 1 hour after it is created.
* @throws AttachmentSetSizeLimitExceededException
* A limit for the size of an attachment set has been exceeded. The
* limits are 3 attachments and 5 MB per attachment.
* @throws AttachmentLimitExceededException
* The limit for the number of attachment sets created in a short
* period of time has been exceeded.
* @sample AWSSupport.AddAttachmentsToSet
*/
@Override
public AddAttachmentsToSetResult addAttachmentsToSet(
AddAttachmentsToSetRequest addAttachmentsToSetRequest) {
ExecutionContext executionContext = createExecutionContext(addAttachmentsToSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddAttachmentsToSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(addAttachmentsToSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new AddAttachmentsToSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds additional customer communication to an AWS Support case. You use
* the CaseId
value to identify the case to add communication
* to. You can list a set of email addresses to copy on the communication
* using the CcEmailAddresses
value. The
* CommunicationBody
value contains the text of the
* communication.
*
*
* The response indicates the success or failure of the request.
*
*
* This operation implements a subset of the features of the AWS Support
* Center.
*
*
* @param addCommunicationToCaseRequest
* To be written.
* @return Result of the AddCommunicationToCase operation returned by the
* service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws CaseIdNotFoundException
* The requested CaseId
could not be located.
* @throws AttachmentSetIdNotFoundException
* An attachment set with the specified ID could not be found.
* @throws AttachmentSetExpiredException
* The expiration time of the attachment set has passed. The set
* expires 1 hour after it is created.
* @sample AWSSupport.AddCommunicationToCase
*/
@Override
public AddCommunicationToCaseResult addCommunicationToCase(
AddCommunicationToCaseRequest addCommunicationToCaseRequest) {
ExecutionContext executionContext = createExecutionContext(addCommunicationToCaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AddCommunicationToCaseRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(addCommunicationToCaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new AddCommunicationToCaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new case in the AWS Support Center. This operation is modeled
* on the behavior of the AWS Support Center Create
* Case page. Its parameters require you to specify the following
* information:
*
*
* - IssueType. The type of issue for the case. You can specify
* either "customer-service" or "technical." If you do not indicate a value,
* the default is "technical."
* - ServiceCode. The code for an AWS service. You obtain the
*
ServiceCode
by calling DescribeServices.
* - CategoryCode. The category for the service defined for the
*
ServiceCode
value. You also obtain the category code for a
* service by calling DescribeServices. Each AWS service defines its
* own set of category codes.
* - SeverityCode. A value that indicates the urgency of the case,
* which in turn determines the response time according to your service
* level agreement with AWS Support. You obtain the SeverityCode by calling
* DescribeSeverityLevels.
* - Subject. The Subject field on the AWS Support Center Create
* Case page.
* - CommunicationBody. The Description field on the AWS
* Support Center Create
* Case page.
* - AttachmentSetId. The ID of a set of attachments that has been
* created by using AddAttachmentsToSet.
* - Language. The human language in which AWS Support handles the
* case. English and Japanese are currently supported.
* - CcEmailAddresses. The AWS Support Center CC field on
* the Create
* Case page. You can list email addresses to be copied on any
* correspondence about the case. The account that opens the case is already
* identified by passing the AWS Credentials in the HTTP POST method or in a
* method or function call from one of the programming languages supported
* by an AWS SDK.
*
*
*
* To add additional communication or attachments to an existing case, use
* AddCommunicationToCase.
*
*
*
* A successful CreateCase request returns an AWS Support case
* number. Case numbers are used by the DescribeCases operation to
* retrieve existing AWS Support cases.
*
*
* @param createCaseRequest
* @return Result of the CreateCase operation returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws CaseCreationLimitExceededException
* The case creation limit for the account has been exceeded.
* @throws AttachmentSetIdNotFoundException
* An attachment set with the specified ID could not be found.
* @throws AttachmentSetExpiredException
* The expiration time of the attachment set has passed. The set
* expires 1 hour after it is created.
* @sample AWSSupport.CreateCase
*/
@Override
public CreateCaseResult createCase(CreateCaseRequest createCaseRequest) {
ExecutionContext executionContext = createExecutionContext(createCaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateCaseRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(createCaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateCaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the attachment that has the specified ID. Attachment IDs are
* generated by the case management system when you add an attachment to a
* case or case communication. Attachment IDs are returned in the
* AttachmentDetails objects that are returned by the
* DescribeCommunications operation.
*
*
* @param describeAttachmentRequest
* @return Result of the DescribeAttachment operation returned by the
* service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws DescribeAttachmentLimitExceededException
* The limit for the number of DescribeAttachment requests in
* a short period of time has been exceeded.
* @throws AttachmentIdNotFoundException
* An attachment with the specified ID could not be found.
* @sample AWSSupport.DescribeAttachment
*/
@Override
public DescribeAttachmentResult describeAttachment(
DescribeAttachmentRequest describeAttachmentRequest) {
ExecutionContext executionContext = createExecutionContext(describeAttachmentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAttachmentRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(describeAttachmentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeAttachmentResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of cases that you specify by passing one or more case IDs.
* In addition, you can filter the cases by date by setting values for the
* AfterTime
and BeforeTime
request parameters.
* You can set values for the IncludeResolvedCases
and
* IncludeCommunications
request parameters to control how much
* information is returned.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* The response returns the following in JSON format:
*
*
* - One or more CaseDetails data types.
* - One or more
NextToken
values, which specify where to
* paginate the returned records represented by the CaseDetails
* objects.
*
*
* @param describeCasesRequest
* @return Result of the DescribeCases operation returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws CaseIdNotFoundException
* The requested CaseId
could not be located.
* @sample AWSSupport.DescribeCases
*/
@Override
public DescribeCasesResult describeCases(
DescribeCasesRequest describeCasesRequest) {
ExecutionContext executionContext = createExecutionContext(describeCasesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCasesRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(describeCasesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeCasesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeCasesResult describeCases() {
return describeCases(new DescribeCasesRequest());
}
/**
*
* Returns communications (and attachments) for one or more support cases.
* You can use the AfterTime
and BeforeTime
* parameters to filter by date. You can use the CaseId
* parameter to restrict the results to a particular case.
*
*
* Case data is available for 12 months after creation. If a case was
* created more than 12 months ago, a request for data might cause an error.
*
*
* You can use the MaxResults
and NextToken
* parameters to control the pagination of the result set. Set
* MaxResults
to the number of cases you want displayed on each
* page, and use NextToken
to specify the resumption of
* pagination.
*
*
* @param describeCommunicationsRequest
* @return Result of the DescribeCommunications operation returned by the
* service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws CaseIdNotFoundException
* The requested CaseId
could not be located.
* @sample AWSSupport.DescribeCommunications
*/
@Override
public DescribeCommunicationsResult describeCommunications(
DescribeCommunicationsRequest describeCommunicationsRequest) {
ExecutionContext executionContext = createExecutionContext(describeCommunicationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeCommunicationsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(describeCommunicationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeCommunicationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the current list of AWS services and a list of service categories
* that applies to each one. You then use service names and categories in
* your CreateCase requests. Each AWS service has its own set of
* categories.
*
*
* The service codes and category codes correspond to the values that are
* displayed in the Service and Category drop-down lists on
* the AWS Support Center Create
* Case page. The values in those fields, however, do not necessarily
* match the service codes and categories returned by the
* DescribeServices
request. Always use the service codes and
* categories obtained programmatically. This practice ensures that you
* always have the most recent set of service and category codes.
*
*
* @param describeServicesRequest
* @return Result of the DescribeServices operation returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeServices
*/
@Override
public DescribeServicesResult describeServices(
DescribeServicesRequest describeServicesRequest) {
ExecutionContext executionContext = createExecutionContext(describeServicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServicesRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(describeServicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeServicesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeServicesResult describeServices() {
return describeServices(new DescribeServicesRequest());
}
/**
*
* Returns the list of severity levels that you can assign to an AWS Support
* case. The severity level for a case is also a field in the
* CaseDetails data type included in any CreateCase request.
*
*
* @param describeSeverityLevelsRequest
* @return Result of the DescribeSeverityLevels operation returned by the
* service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeSeverityLevels
*/
@Override
public DescribeSeverityLevelsResult describeSeverityLevels(
DescribeSeverityLevelsRequest describeSeverityLevelsRequest) {
ExecutionContext executionContext = createExecutionContext(describeSeverityLevelsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSeverityLevelsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(describeSeverityLevelsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeSeverityLevelsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeSeverityLevelsResult describeSeverityLevels() {
return describeSeverityLevels(new DescribeSeverityLevelsRequest());
}
/**
*
* Returns the refresh status of the Trusted Advisor checks that have the
* specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* @param describeTrustedAdvisorCheckRefreshStatusesRequest
* @return Result of the DescribeTrustedAdvisorCheckRefreshStatuses
* operation returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeTrustedAdvisorCheckRefreshStatuses
*/
@Override
public DescribeTrustedAdvisorCheckRefreshStatusesResult describeTrustedAdvisorCheckRefreshStatuses(
DescribeTrustedAdvisorCheckRefreshStatusesRequest describeTrustedAdvisorCheckRefreshStatusesRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrustedAdvisorCheckRefreshStatusesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrustedAdvisorCheckRefreshStatusesRequestMarshaller(
protocolFactory)
.marshall(super
.beforeMarshalling(describeTrustedAdvisorCheckRefreshStatusesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeTrustedAdvisorCheckRefreshStatusesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the results of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckResult object, which
* contains these three objects:
*
*
* - TrustedAdvisorCategorySpecificSummary
* - TrustedAdvisorResourceDetail
* - TrustedAdvisorResourcesSummary
*
*
* In addition, the response contains these fields:
*
*
* - Status. The alert status of the check: "ok" (green), "warning"
* (yellow), "error" (red), or "not_available".
* - Timestamp. The time of the last refresh of the check.
* - CheckId. The unique identifier for the check.
*
*
* @param describeTrustedAdvisorCheckResultRequest
* @return Result of the DescribeTrustedAdvisorCheckResult operation
* returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeTrustedAdvisorCheckResult
*/
@Override
public DescribeTrustedAdvisorCheckResultResult describeTrustedAdvisorCheckResult(
DescribeTrustedAdvisorCheckResultRequest describeTrustedAdvisorCheckResultRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrustedAdvisorCheckResultRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrustedAdvisorCheckResultRequestMarshaller(
protocolFactory)
.marshall(super
.beforeMarshalling(describeTrustedAdvisorCheckResultRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeTrustedAdvisorCheckResultResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the summaries of the results of the Trusted Advisor checks that
* have the specified check IDs. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains an array of TrustedAdvisorCheckSummary
* objects.
*
*
* @param describeTrustedAdvisorCheckSummariesRequest
* @return Result of the DescribeTrustedAdvisorCheckSummaries operation
* returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeTrustedAdvisorCheckSummaries
*/
@Override
public DescribeTrustedAdvisorCheckSummariesResult describeTrustedAdvisorCheckSummaries(
DescribeTrustedAdvisorCheckSummariesRequest describeTrustedAdvisorCheckSummariesRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrustedAdvisorCheckSummariesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrustedAdvisorCheckSummariesRequestMarshaller(
protocolFactory)
.marshall(super
.beforeMarshalling(describeTrustedAdvisorCheckSummariesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeTrustedAdvisorCheckSummariesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about all available Trusted Advisor checks, including
* name, ID, category, description, and metadata. You must specify a
* language code; English ("en") and Japanese ("ja") are currently
* supported. The response contains a TrustedAdvisorCheckDescription
* for each check.
*
*
* @param describeTrustedAdvisorChecksRequest
* @return Result of the DescribeTrustedAdvisorChecks operation returned by
* the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.DescribeTrustedAdvisorChecks
*/
@Override
public DescribeTrustedAdvisorChecksResult describeTrustedAdvisorChecks(
DescribeTrustedAdvisorChecksRequest describeTrustedAdvisorChecksRequest) {
ExecutionContext executionContext = createExecutionContext(describeTrustedAdvisorChecksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTrustedAdvisorChecksRequestMarshaller(
protocolFactory)
.marshall(super
.beforeMarshalling(describeTrustedAdvisorChecksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DescribeTrustedAdvisorChecksResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Requests a refresh of the Trusted Advisor check that has the specified
* check ID. Check IDs can be obtained by calling
* DescribeTrustedAdvisorChecks.
*
*
* The response contains a TrustedAdvisorCheckRefreshStatus object,
* which contains these fields:
*
*
* - Status. The refresh status of the check: "none", "enqueued",
* "processing", "success", or "abandoned".
* - MillisUntilNextRefreshable. The amount of time, in
* milliseconds, until the check is eligible for refresh.
* - CheckId. The unique identifier for the check.
*
*
* @param refreshTrustedAdvisorCheckRequest
* @return Result of the RefreshTrustedAdvisorCheck operation returned by
* the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @sample AWSSupport.RefreshTrustedAdvisorCheck
*/
@Override
public RefreshTrustedAdvisorCheckResult refreshTrustedAdvisorCheck(
RefreshTrustedAdvisorCheckRequest refreshTrustedAdvisorCheckRequest) {
ExecutionContext executionContext = createExecutionContext(refreshTrustedAdvisorCheckRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RefreshTrustedAdvisorCheckRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(refreshTrustedAdvisorCheckRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new RefreshTrustedAdvisorCheckResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Takes a CaseId
and returns the initial state of the case
* along with the state of the case after the call to ResolveCase
* completed.
*
*
* @param resolveCaseRequest
* @return Result of the ResolveCase operation returned by the service.
* @throws InternalServerErrorException
* An internal server error occurred.
* @throws CaseIdNotFoundException
* The requested CaseId
could not be located.
* @sample AWSSupport.ResolveCase
*/
@Override
public ResolveCaseResult resolveCase(ResolveCaseRequest resolveCaseRequest) {
ExecutionContext executionContext = createExecutionContext(resolveCaseRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ResolveCaseRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(resolveCaseRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ResolveCaseResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ResolveCaseResult resolveCase() {
return resolveCase(new ResolveCaseRequest());
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(
AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be
* overriden at the request level.
**/
private Response invoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils
.getCredentialsProvider(request.getOriginalRequest(),
awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any
* credentials set on the client or request will be ignored for this
* operation.
**/
private Response anonymousInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack
* thereof) have been configured in the ExecutionContext beforehand.
**/
private Response doInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory
.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler,
executionContext);
}
}