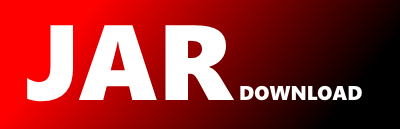
com.amazonaws.services.support.model.DescribeCasesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-support Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.support.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeCasesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*
*/
private com.amazonaws.internal.SdkInternalList caseIdList;
/**
*
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
*
*/
private String displayId;
/**
*
* The start date for a filtered date search on support case communications. Case communications are available for
* 12 months after creation.
*
*/
private String afterTime;
/**
*
* The end date for a filtered date search on support case communications. Case communications are available for 12
* months after creation.
*
*/
private String beforeTime;
/**
*
* Specifies whether to include resolved support cases in the DescribeCases
response. By default,
* resolved cases aren't included.
*
*/
private Boolean includeResolvedCases;
/**
*
* A resumption point for pagination.
*
*/
private String nextToken;
/**
*
* The maximum number of results to return before paginating.
*
*/
private Integer maxResults;
/**
*
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1 code
* for the language
parameter if you want support in that language.
*
*/
private String language;
/**
*
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*
*/
private Boolean includeCommunications;
/**
*
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*
*
* @return A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*/
public java.util.List getCaseIdList() {
if (caseIdList == null) {
caseIdList = new com.amazonaws.internal.SdkInternalList();
}
return caseIdList;
}
/**
*
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*
*
* @param caseIdList
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*/
public void setCaseIdList(java.util.Collection caseIdList) {
if (caseIdList == null) {
this.caseIdList = null;
return;
}
this.caseIdList = new com.amazonaws.internal.SdkInternalList(caseIdList);
}
/**
*
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCaseIdList(java.util.Collection)} or {@link #withCaseIdList(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param caseIdList
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withCaseIdList(String... caseIdList) {
if (this.caseIdList == null) {
setCaseIdList(new com.amazonaws.internal.SdkInternalList(caseIdList.length));
}
for (String ele : caseIdList) {
this.caseIdList.add(ele);
}
return this;
}
/**
*
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
*
*
* @param caseIdList
* A list of ID numbers of the support cases you want returned. The maximum number of cases is 100.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withCaseIdList(java.util.Collection caseIdList) {
setCaseIdList(caseIdList);
return this;
}
/**
*
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
*
*
* @param displayId
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
*/
public void setDisplayId(String displayId) {
this.displayId = displayId;
}
/**
*
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
*
*
* @return The ID displayed for a case in the Amazon Web Services Support Center user interface.
*/
public String getDisplayId() {
return this.displayId;
}
/**
*
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
*
*
* @param displayId
* The ID displayed for a case in the Amazon Web Services Support Center user interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withDisplayId(String displayId) {
setDisplayId(displayId);
return this;
}
/**
*
* The start date for a filtered date search on support case communications. Case communications are available for
* 12 months after creation.
*
*
* @param afterTime
* The start date for a filtered date search on support case communications. Case communications are
* available for 12 months after creation.
*/
public void setAfterTime(String afterTime) {
this.afterTime = afterTime;
}
/**
*
* The start date for a filtered date search on support case communications. Case communications are available for
* 12 months after creation.
*
*
* @return The start date for a filtered date search on support case communications. Case communications are
* available for 12 months after creation.
*/
public String getAfterTime() {
return this.afterTime;
}
/**
*
* The start date for a filtered date search on support case communications. Case communications are available for
* 12 months after creation.
*
*
* @param afterTime
* The start date for a filtered date search on support case communications. Case communications are
* available for 12 months after creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withAfterTime(String afterTime) {
setAfterTime(afterTime);
return this;
}
/**
*
* The end date for a filtered date search on support case communications. Case communications are available for 12
* months after creation.
*
*
* @param beforeTime
* The end date for a filtered date search on support case communications. Case communications are available
* for 12 months after creation.
*/
public void setBeforeTime(String beforeTime) {
this.beforeTime = beforeTime;
}
/**
*
* The end date for a filtered date search on support case communications. Case communications are available for 12
* months after creation.
*
*
* @return The end date for a filtered date search on support case communications. Case communications are available
* for 12 months after creation.
*/
public String getBeforeTime() {
return this.beforeTime;
}
/**
*
* The end date for a filtered date search on support case communications. Case communications are available for 12
* months after creation.
*
*
* @param beforeTime
* The end date for a filtered date search on support case communications. Case communications are available
* for 12 months after creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withBeforeTime(String beforeTime) {
setBeforeTime(beforeTime);
return this;
}
/**
*
* Specifies whether to include resolved support cases in the DescribeCases
response. By default,
* resolved cases aren't included.
*
*
* @param includeResolvedCases
* Specifies whether to include resolved support cases in the DescribeCases
response. By
* default, resolved cases aren't included.
*/
public void setIncludeResolvedCases(Boolean includeResolvedCases) {
this.includeResolvedCases = includeResolvedCases;
}
/**
*
* Specifies whether to include resolved support cases in the DescribeCases
response. By default,
* resolved cases aren't included.
*
*
* @return Specifies whether to include resolved support cases in the DescribeCases
response. By
* default, resolved cases aren't included.
*/
public Boolean getIncludeResolvedCases() {
return this.includeResolvedCases;
}
/**
*
* Specifies whether to include resolved support cases in the DescribeCases
response. By default,
* resolved cases aren't included.
*
*
* @param includeResolvedCases
* Specifies whether to include resolved support cases in the DescribeCases
response. By
* default, resolved cases aren't included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withIncludeResolvedCases(Boolean includeResolvedCases) {
setIncludeResolvedCases(includeResolvedCases);
return this;
}
/**
*
* Specifies whether to include resolved support cases in the DescribeCases
response. By default,
* resolved cases aren't included.
*
*
* @return Specifies whether to include resolved support cases in the DescribeCases
response. By
* default, resolved cases aren't included.
*/
public Boolean isIncludeResolvedCases() {
return this.includeResolvedCases;
}
/**
*
* A resumption point for pagination.
*
*
* @param nextToken
* A resumption point for pagination.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* A resumption point for pagination.
*
*
* @return A resumption point for pagination.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* A resumption point for pagination.
*
*
* @param nextToken
* A resumption point for pagination.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of results to return before paginating.
*
*
* @param maxResults
* The maximum number of results to return before paginating.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of results to return before paginating.
*
*
* @return The maximum number of results to return before paginating.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of results to return before paginating.
*
*
* @param maxResults
* The maximum number of results to return before paginating.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
*
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1 code
* for the language
parameter if you want support in that language.
*
*
* @param language
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1
* code for the language
parameter if you want support in that language.
*/
public void setLanguage(String language) {
this.language = language;
}
/**
*
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1 code
* for the language
parameter if you want support in that language.
*
*
* @return The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO
* 639-1 code for the language
parameter if you want support in that language.
*/
public String getLanguage() {
return this.language;
}
/**
*
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1 code
* for the language
parameter if you want support in that language.
*
*
* @param language
* The language in which Amazon Web Services Support handles the case. Amazon Web Services Support currently
* supports Chinese (“zh”), English ("en"), Japanese ("ja") and Korean (“ko”). You must specify the ISO 639-1
* code for the language
parameter if you want support in that language.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withLanguage(String language) {
setLanguage(language);
return this;
}
/**
*
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*
*
* @param includeCommunications
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*/
public void setIncludeCommunications(Boolean includeCommunications) {
this.includeCommunications = includeCommunications;
}
/**
*
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*
*
* @return Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*/
public Boolean getIncludeCommunications() {
return this.includeCommunications;
}
/**
*
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*
*
* @param includeCommunications
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeCasesRequest withIncludeCommunications(Boolean includeCommunications) {
setIncludeCommunications(includeCommunications);
return this;
}
/**
*
* Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*
*
* @return Specifies whether to include communications in the DescribeCases
response. By default,
* communications are included.
*/
public Boolean isIncludeCommunications() {
return this.includeCommunications;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCaseIdList() != null)
sb.append("CaseIdList: ").append(getCaseIdList()).append(",");
if (getDisplayId() != null)
sb.append("DisplayId: ").append(getDisplayId()).append(",");
if (getAfterTime() != null)
sb.append("AfterTime: ").append(getAfterTime()).append(",");
if (getBeforeTime() != null)
sb.append("BeforeTime: ").append(getBeforeTime()).append(",");
if (getIncludeResolvedCases() != null)
sb.append("IncludeResolvedCases: ").append(getIncludeResolvedCases()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults()).append(",");
if (getLanguage() != null)
sb.append("Language: ").append(getLanguage()).append(",");
if (getIncludeCommunications() != null)
sb.append("IncludeCommunications: ").append(getIncludeCommunications());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeCasesRequest == false)
return false;
DescribeCasesRequest other = (DescribeCasesRequest) obj;
if (other.getCaseIdList() == null ^ this.getCaseIdList() == null)
return false;
if (other.getCaseIdList() != null && other.getCaseIdList().equals(this.getCaseIdList()) == false)
return false;
if (other.getDisplayId() == null ^ this.getDisplayId() == null)
return false;
if (other.getDisplayId() != null && other.getDisplayId().equals(this.getDisplayId()) == false)
return false;
if (other.getAfterTime() == null ^ this.getAfterTime() == null)
return false;
if (other.getAfterTime() != null && other.getAfterTime().equals(this.getAfterTime()) == false)
return false;
if (other.getBeforeTime() == null ^ this.getBeforeTime() == null)
return false;
if (other.getBeforeTime() != null && other.getBeforeTime().equals(this.getBeforeTime()) == false)
return false;
if (other.getIncludeResolvedCases() == null ^ this.getIncludeResolvedCases() == null)
return false;
if (other.getIncludeResolvedCases() != null && other.getIncludeResolvedCases().equals(this.getIncludeResolvedCases()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
if (other.getLanguage() == null ^ this.getLanguage() == null)
return false;
if (other.getLanguage() != null && other.getLanguage().equals(this.getLanguage()) == false)
return false;
if (other.getIncludeCommunications() == null ^ this.getIncludeCommunications() == null)
return false;
if (other.getIncludeCommunications() != null && other.getIncludeCommunications().equals(this.getIncludeCommunications()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCaseIdList() == null) ? 0 : getCaseIdList().hashCode());
hashCode = prime * hashCode + ((getDisplayId() == null) ? 0 : getDisplayId().hashCode());
hashCode = prime * hashCode + ((getAfterTime() == null) ? 0 : getAfterTime().hashCode());
hashCode = prime * hashCode + ((getBeforeTime() == null) ? 0 : getBeforeTime().hashCode());
hashCode = prime * hashCode + ((getIncludeResolvedCases() == null) ? 0 : getIncludeResolvedCases().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
hashCode = prime * hashCode + ((getLanguage() == null) ? 0 : getLanguage().hashCode());
hashCode = prime * hashCode + ((getIncludeCommunications() == null) ? 0 : getIncludeCommunications().hashCode());
return hashCode;
}
@Override
public DescribeCasesRequest clone() {
return (DescribeCasesRequest) super.clone();
}
}