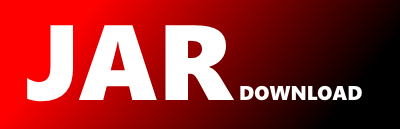
com.amazonaws.services.support.AWSSupportAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-support Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.support;
import javax.annotation.Generated;
import com.amazonaws.services.support.model.*;
/**
* Interface for accessing AWS Support asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.support.AbstractAWSSupportAsync} instead.
*
*
* Amazon Web Services Support
*
* The Amazon Web Services Support API Reference is intended for programmers who need detailed information about
* the Amazon Web Services Support operations and data types. You can use the API to manage your support cases
* programmatically. The Amazon Web Services Support API uses HTTP methods that return results in JSON format.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp, or
* Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information about
* changing your support plan, see Amazon Web Services Support.
*
*
*
*
*
* You can also use the Amazon Web Services Support API to access features for Trusted Advisor. You can return a list of checks and
* their descriptions, get check results, specify checks to refresh, and get the refresh status of checks.
*
*
* You can manage your support cases with the following Amazon Web Services Support API operations:
*
*
* -
*
* The CreateCase, DescribeCases, DescribeAttachment, and ResolveCase operations create
* Amazon Web Services Support cases, retrieve information about cases, and resolve cases.
*
*
* -
*
* The DescribeCommunications, AddCommunicationToCase, and AddAttachmentsToSet operations retrieve
* and add communications and attachments to Amazon Web Services Support cases.
*
*
* -
*
* The DescribeServices and DescribeSeverityLevels operations return Amazon Web Service names, service
* codes, service categories, and problem severity levels. You use these values when you call the CreateCase
* operation.
*
*
*
*
* You can also use the Amazon Web Services Support API to call the Trusted Advisor operations. For more information,
* see Trusted Advisor in the Amazon Web Services Support User Guide.
*
*
* For authentication of requests, Amazon Web Services Support uses Signature Version 4 Signing
* Process.
*
*
* For more information about this service and the endpoints to use, see About the Amazon Web Services
* Support API in the Amazon Web Services Support User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSSupportAsync extends AWSSupport {
/**
*
* Adds one or more attachments to an attachment set.
*
*
* An attachment set is a temporary container for attachments that you add to a case or case communication. The set
* is available for 1 hour after it's created. The expiryTime
returned in the response is when the set
* expires.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param addAttachmentsToSetRequest
* @return A Java Future containing the result of the AddAttachmentsToSet operation returned by the service.
* @sample AWSSupportAsync.AddAttachmentsToSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addAttachmentsToSetAsync(AddAttachmentsToSetRequest addAttachmentsToSetRequest);
/**
*
* Adds one or more attachments to an attachment set.
*
*
* An attachment set is a temporary container for attachments that you add to a case or case communication. The set
* is available for 1 hour after it's created. The expiryTime
returned in the response is when the set
* expires.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param addAttachmentsToSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddAttachmentsToSet operation returned by the service.
* @sample AWSSupportAsyncHandler.AddAttachmentsToSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addAttachmentsToSetAsync(AddAttachmentsToSetRequest addAttachmentsToSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds additional customer communication to an Amazon Web Services Support case. Use the caseId
* parameter to identify the case to which to add communication. You can list a set of email addresses to copy on
* the communication by using the ccEmailAddresses
parameter. The communicationBody
value
* contains the text of the communication.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param addCommunicationToCaseRequest
* @return A Java Future containing the result of the AddCommunicationToCase operation returned by the service.
* @sample AWSSupportAsync.AddCommunicationToCase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addCommunicationToCaseAsync(AddCommunicationToCaseRequest addCommunicationToCaseRequest);
/**
*
* Adds additional customer communication to an Amazon Web Services Support case. Use the caseId
* parameter to identify the case to which to add communication. You can list a set of email addresses to copy on
* the communication by using the ccEmailAddresses
parameter. The communicationBody
value
* contains the text of the communication.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param addCommunicationToCaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddCommunicationToCase operation returned by the service.
* @sample AWSSupportAsyncHandler.AddCommunicationToCase
* @see AWS
* API Documentation
*/
java.util.concurrent.Future addCommunicationToCaseAsync(AddCommunicationToCaseRequest addCommunicationToCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a case in the Amazon Web Services Support Center. This operation is similar to how you create a case in
* the Amazon Web Services Support Center Create
* Case page.
*
*
* The Amazon Web Services Support API doesn't support requesting service limit increases. You can submit a service
* limit increase in the following ways:
*
*
* -
*
* Submit a request from the Amazon Web Services Support Center Create Case page.
*
*
* -
*
* Use the Service Quotas RequestServiceQuotaIncrease operation.
*
*
*
*
* A successful CreateCase
request returns an Amazon Web Services Support case number. You can use the
* DescribeCases operation and specify the case number to get existing Amazon Web Services Support cases.
* After you create a case, use the AddCommunicationToCase operation to add additional communication or
* attachments to an existing case.
*
*
* The caseId
is separate from the displayId
that appears in the Amazon Web Services Support Center. Use the
* DescribeCases operation to get the displayId
.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param createCaseRequest
* @return A Java Future containing the result of the CreateCase operation returned by the service.
* @sample AWSSupportAsync.CreateCase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCaseAsync(CreateCaseRequest createCaseRequest);
/**
*
* Creates a case in the Amazon Web Services Support Center. This operation is similar to how you create a case in
* the Amazon Web Services Support Center Create
* Case page.
*
*
* The Amazon Web Services Support API doesn't support requesting service limit increases. You can submit a service
* limit increase in the following ways:
*
*
* -
*
* Submit a request from the Amazon Web Services Support Center Create Case page.
*
*
* -
*
* Use the Service Quotas RequestServiceQuotaIncrease operation.
*
*
*
*
* A successful CreateCase
request returns an Amazon Web Services Support case number. You can use the
* DescribeCases operation and specify the case number to get existing Amazon Web Services Support cases.
* After you create a case, use the AddCommunicationToCase operation to add additional communication or
* attachments to an existing case.
*
*
* The caseId
is separate from the displayId
that appears in the Amazon Web Services Support Center. Use the
* DescribeCases operation to get the displayId
.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param createCaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCase operation returned by the service.
* @sample AWSSupportAsyncHandler.CreateCase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCaseAsync(CreateCaseRequest createCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the attachment that has the specified ID. Attachments can include screenshots, error logs, or other files
* that describe your issue. Attachment IDs are generated by the case management system when you add an attachment
* to a case or case communication. Attachment IDs are returned in the AttachmentDetails objects that are
* returned by the DescribeCommunications operation.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeAttachmentRequest
* @return A Java Future containing the result of the DescribeAttachment operation returned by the service.
* @sample AWSSupportAsync.DescribeAttachment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAttachmentAsync(DescribeAttachmentRequest describeAttachmentRequest);
/**
*
* Returns the attachment that has the specified ID. Attachments can include screenshots, error logs, or other files
* that describe your issue. Attachment IDs are generated by the case management system when you add an attachment
* to a case or case communication. Attachment IDs are returned in the AttachmentDetails objects that are
* returned by the DescribeCommunications operation.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAttachment operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeAttachment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeAttachmentAsync(DescribeAttachmentRequest describeAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of cases that you specify by passing one or more case IDs. You can use the afterTime
* and beforeTime
parameters to filter the cases by date. You can set values for the
* includeResolvedCases
and includeCommunications
parameters to specify how much
* information to return.
*
*
* The response returns the following in JSON format:
*
*
* -
*
* One or more CaseDetails data
* types.
*
*
* -
*
* One or more nextToken
values, which specify where to paginate the returned records represented by
* the CaseDetails
objects.
*
*
*
*
* Case data is available for 12 months after creation. If a case was created more than 12 months ago, a request
* might return an error.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCasesRequest
* @return A Java Future containing the result of the DescribeCases operation returned by the service.
* @sample AWSSupportAsync.DescribeCases
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCasesAsync(DescribeCasesRequest describeCasesRequest);
/**
*
* Returns a list of cases that you specify by passing one or more case IDs. You can use the afterTime
* and beforeTime
parameters to filter the cases by date. You can set values for the
* includeResolvedCases
and includeCommunications
parameters to specify how much
* information to return.
*
*
* The response returns the following in JSON format:
*
*
* -
*
* One or more CaseDetails data
* types.
*
*
* -
*
* One or more nextToken
values, which specify where to paginate the returned records represented by
* the CaseDetails
objects.
*
*
*
*
* Case data is available for 12 months after creation. If a case was created more than 12 months ago, a request
* might return an error.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCases operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeCases
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCasesAsync(DescribeCasesRequest describeCasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeCases operation.
*
* @see #describeCasesAsync(DescribeCasesRequest)
*/
java.util.concurrent.Future describeCasesAsync();
/**
* Simplified method form for invoking the DescribeCases operation with an AsyncHandler.
*
* @see #describeCasesAsync(DescribeCasesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeCasesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns communications and attachments for one or more support cases. Use the afterTime
and
* beforeTime
parameters to filter by date. You can use the caseId
parameter to restrict
* the results to a specific case.
*
*
* Case data is available for 12 months after creation. If a case was created more than 12 months ago, a request for
* data might cause an error.
*
*
* You can use the maxResults
and nextToken
parameters to control the pagination of the
* results. Set maxResults
to the number of cases that you want to display on each page, and use
* nextToken
to specify the resumption of pagination.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCommunicationsRequest
* @return A Java Future containing the result of the DescribeCommunications operation returned by the service.
* @sample AWSSupportAsync.DescribeCommunications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeCommunicationsAsync(DescribeCommunicationsRequest describeCommunicationsRequest);
/**
*
* Returns communications and attachments for one or more support cases. Use the afterTime
and
* beforeTime
parameters to filter by date. You can use the caseId
parameter to restrict
* the results to a specific case.
*
*
* Case data is available for 12 months after creation. If a case was created more than 12 months ago, a request for
* data might cause an error.
*
*
* You can use the maxResults
and nextToken
parameters to control the pagination of the
* results. Set maxResults
to the number of cases that you want to display on each page, and use
* nextToken
to specify the resumption of pagination.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCommunicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCommunications operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeCommunications
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeCommunicationsAsync(DescribeCommunicationsRequest describeCommunicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of CreateCaseOption types along with the corresponding supported hours and language availability.
* You can specify the language
categoryCode
, issueType
and
* serviceCode
used to retrieve the CreateCaseOptions.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCreateCaseOptionsRequest
* @return A Java Future containing the result of the DescribeCreateCaseOptions operation returned by the service.
* @sample AWSSupportAsync.DescribeCreateCaseOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCreateCaseOptionsAsync(
DescribeCreateCaseOptionsRequest describeCreateCaseOptionsRequest);
/**
*
* Returns a list of CreateCaseOption types along with the corresponding supported hours and language availability.
* You can specify the language
categoryCode
, issueType
and
* serviceCode
used to retrieve the CreateCaseOptions.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeCreateCaseOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCreateCaseOptions operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeCreateCaseOptions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeCreateCaseOptionsAsync(
DescribeCreateCaseOptionsRequest describeCreateCaseOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the current list of Amazon Web Services services and a list of service categories for each service. You
* then use service names and categories in your CreateCase requests. Each Amazon Web Services service has
* its own set of categories.
*
*
* The service codes and category codes correspond to the values that appear in the Service and
* Category lists on the Amazon Web Services Support Center Create Case page. The values in those fields
* don't necessarily match the service codes and categories returned by the DescribeServices
operation.
* Always use the service codes and categories that the DescribeServices
operation returns, so that you
* have the most recent set of service and category codes.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeServicesRequest
* @return A Java Future containing the result of the DescribeServices operation returned by the service.
* @sample AWSSupportAsync.DescribeServices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeServicesAsync(DescribeServicesRequest describeServicesRequest);
/**
*
* Returns the current list of Amazon Web Services services and a list of service categories for each service. You
* then use service names and categories in your CreateCase requests. Each Amazon Web Services service has
* its own set of categories.
*
*
* The service codes and category codes correspond to the values that appear in the Service and
* Category lists on the Amazon Web Services Support Center Create Case page. The values in those fields
* don't necessarily match the service codes and categories returned by the DescribeServices
operation.
* Always use the service codes and categories that the DescribeServices
operation returns, so that you
* have the most recent set of service and category codes.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServices operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeServices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeServicesAsync(DescribeServicesRequest describeServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeServices operation.
*
* @see #describeServicesAsync(DescribeServicesRequest)
*/
java.util.concurrent.Future describeServicesAsync();
/**
* Simplified method form for invoking the DescribeServices operation with an AsyncHandler.
*
* @see #describeServicesAsync(DescribeServicesRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeServicesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the list of severity levels that you can assign to a support case. The severity level for a case is also
* a field in the CaseDetails data type that you include for a CreateCase request.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeSeverityLevelsRequest
* @return A Java Future containing the result of the DescribeSeverityLevels operation returned by the service.
* @sample AWSSupportAsync.DescribeSeverityLevels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSeverityLevelsAsync(DescribeSeverityLevelsRequest describeSeverityLevelsRequest);
/**
*
* Returns the list of severity levels that you can assign to a support case. The severity level for a case is also
* a field in the CaseDetails data type that you include for a CreateCase request.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeSeverityLevelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSeverityLevels operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeSeverityLevels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeSeverityLevelsAsync(DescribeSeverityLevelsRequest describeSeverityLevelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeSeverityLevels operation.
*
* @see #describeSeverityLevelsAsync(DescribeSeverityLevelsRequest)
*/
java.util.concurrent.Future describeSeverityLevelsAsync();
/**
* Simplified method form for invoking the DescribeSeverityLevels operation with an AsyncHandler.
*
* @see #describeSeverityLevelsAsync(DescribeSeverityLevelsRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeSeverityLevelsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of supported languages for a specified categoryCode
, issueType
and
* serviceCode
. The returned supported languages will include a ISO 639-1 code for the
* language
, and the language display name.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeSupportedLanguagesRequest
* @return A Java Future containing the result of the DescribeSupportedLanguages operation returned by the service.
* @sample AWSSupportAsync.DescribeSupportedLanguages
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSupportedLanguagesAsync(
DescribeSupportedLanguagesRequest describeSupportedLanguagesRequest);
/**
*
* Returns a list of supported languages for a specified categoryCode
, issueType
and
* serviceCode
. The returned supported languages will include a ISO 639-1 code for the
* language
, and the language display name.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param describeSupportedLanguagesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSupportedLanguages operation returned by the service.
* @sample AWSSupportAsyncHandler.DescribeSupportedLanguages
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSupportedLanguagesAsync(
DescribeSupportedLanguagesRequest describeSupportedLanguagesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the refresh status of the Trusted Advisor checks that have the specified check IDs. You can get the check
* IDs by calling the DescribeTrustedAdvisorChecks operation.
*
*
* Some checks are refreshed automatically, and you can't return their refresh statuses by using the
* DescribeTrustedAdvisorCheckRefreshStatuses
operation. If you call this operation for these checks,
* you might see an InvalidParameterValue
error.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckRefreshStatusesRequest
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckRefreshStatuses operation returned
* by the service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckRefreshStatuses
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckRefreshStatusesAsync(
DescribeTrustedAdvisorCheckRefreshStatusesRequest describeTrustedAdvisorCheckRefreshStatusesRequest);
/**
*
* Returns the refresh status of the Trusted Advisor checks that have the specified check IDs. You can get the check
* IDs by calling the DescribeTrustedAdvisorChecks operation.
*
*
* Some checks are refreshed automatically, and you can't return their refresh statuses by using the
* DescribeTrustedAdvisorCheckRefreshStatuses
operation. If you call this operation for these checks,
* you might see an InvalidParameterValue
error.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckRefreshStatusesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckRefreshStatuses operation returned
* by the service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckRefreshStatuses
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckRefreshStatusesAsync(
DescribeTrustedAdvisorCheckRefreshStatusesRequest describeTrustedAdvisorCheckRefreshStatusesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the results of the Trusted Advisor check that has the specified check ID. You can get the check IDs by
* calling the DescribeTrustedAdvisorChecks operation.
*
*
* The response contains a TrustedAdvisorCheckResult object, which contains these three objects:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* In addition, the response contains these fields:
*
*
* -
*
* status - The alert status of the check can be ok
(green), warning
(yellow),
* error
(red), or not_available
.
*
*
* -
*
* timestamp - The time of the last refresh of the check.
*
*
* -
*
* checkId - The unique identifier for the check.
*
*
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckResultRequest
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckResult operation returned by the
* service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckResult
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckResultAsync(
DescribeTrustedAdvisorCheckResultRequest describeTrustedAdvisorCheckResultRequest);
/**
*
* Returns the results of the Trusted Advisor check that has the specified check ID. You can get the check IDs by
* calling the DescribeTrustedAdvisorChecks operation.
*
*
* The response contains a TrustedAdvisorCheckResult object, which contains these three objects:
*
*
* -
*
*
* -
*
*
* -
*
*
*
*
* In addition, the response contains these fields:
*
*
* -
*
* status - The alert status of the check can be ok
(green), warning
(yellow),
* error
(red), or not_available
.
*
*
* -
*
* timestamp - The time of the last refresh of the check.
*
*
* -
*
* checkId - The unique identifier for the check.
*
*
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckResultRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckResult operation returned by the
* service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckResult
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckResultAsync(
DescribeTrustedAdvisorCheckResultRequest describeTrustedAdvisorCheckResultRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the results for the Trusted Advisor check summaries for the check IDs that you specified. You can get the
* check IDs by calling the DescribeTrustedAdvisorChecks operation.
*
*
* The response contains an array of TrustedAdvisorCheckSummary objects.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckSummariesRequest
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckSummaries operation returned by the
* service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorCheckSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckSummariesAsync(
DescribeTrustedAdvisorCheckSummariesRequest describeTrustedAdvisorCheckSummariesRequest);
/**
*
* Returns the results for the Trusted Advisor check summaries for the check IDs that you specified. You can get the
* check IDs by calling the DescribeTrustedAdvisorChecks operation.
*
*
* The response contains an array of TrustedAdvisorCheckSummary objects.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorCheckSummariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrustedAdvisorCheckSummaries operation returned by the
* service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorCheckSummaries
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorCheckSummariesAsync(
DescribeTrustedAdvisorCheckSummariesRequest describeTrustedAdvisorCheckSummariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about all available Trusted Advisor checks, including the name, ID, category, description,
* and metadata. You must specify a language code.
*
*
* The response contains a TrustedAdvisorCheckDescription object for each check. You must set the Amazon Web
* Services Region to us-east-1.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
* -
*
* The names and descriptions for Trusted Advisor checks are subject to change. We recommend that you specify the
* check ID in your code to uniquely identify a check.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorChecksRequest
* @return A Java Future containing the result of the DescribeTrustedAdvisorChecks operation returned by the
* service.
* @sample AWSSupportAsync.DescribeTrustedAdvisorChecks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorChecksAsync(
DescribeTrustedAdvisorChecksRequest describeTrustedAdvisorChecksRequest);
/**
*
* Returns information about all available Trusted Advisor checks, including the name, ID, category, description,
* and metadata. You must specify a language code.
*
*
* The response contains a TrustedAdvisorCheckDescription object for each check. You must set the Amazon Web
* Services Region to us-east-1.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
* -
*
* The names and descriptions for Trusted Advisor checks are subject to change. We recommend that you specify the
* check ID in your code to uniquely identify a check.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param describeTrustedAdvisorChecksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTrustedAdvisorChecks operation returned by the
* service.
* @sample AWSSupportAsyncHandler.DescribeTrustedAdvisorChecks
* @see AWS API Documentation
*/
java.util.concurrent.Future describeTrustedAdvisorChecksAsync(
DescribeTrustedAdvisorChecksRequest describeTrustedAdvisorChecksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Refreshes the Trusted Advisor check that you specify using the check ID. You can get the check IDs by calling the
* DescribeTrustedAdvisorChecks operation.
*
*
* Some checks are refreshed automatically. If you call the RefreshTrustedAdvisorCheck
operation to
* refresh them, you might see the InvalidParameterValue
error.
*
*
* The response contains a TrustedAdvisorCheckRefreshStatus object.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param refreshTrustedAdvisorCheckRequest
* @return A Java Future containing the result of the RefreshTrustedAdvisorCheck operation returned by the service.
* @sample AWSSupportAsync.RefreshTrustedAdvisorCheck
* @see AWS API Documentation
*/
java.util.concurrent.Future refreshTrustedAdvisorCheckAsync(
RefreshTrustedAdvisorCheckRequest refreshTrustedAdvisorCheckRequest);
/**
*
* Refreshes the Trusted Advisor check that you specify using the check ID. You can get the check IDs by calling the
* DescribeTrustedAdvisorChecks operation.
*
*
* Some checks are refreshed automatically. If you call the RefreshTrustedAdvisorCheck
operation to
* refresh them, you might see the InvalidParameterValue
error.
*
*
* The response contains a TrustedAdvisorCheckRefreshStatus object.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* To call the Trusted Advisor operations in the Amazon Web Services Support API, you must use the US East (N.
* Virginia) endpoint. Currently, the US West (Oregon) and Europe (Ireland) endpoints don't support the Trusted
* Advisor operations. For more information, see About the Amazon Web
* Services Support API in the Amazon Web Services Support User Guide.
*
*
* @param refreshTrustedAdvisorCheckRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RefreshTrustedAdvisorCheck operation returned by the service.
* @sample AWSSupportAsyncHandler.RefreshTrustedAdvisorCheck
* @see AWS API Documentation
*/
java.util.concurrent.Future refreshTrustedAdvisorCheckAsync(
RefreshTrustedAdvisorCheckRequest refreshTrustedAdvisorCheckRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resolves a support case. This operation takes a caseId
and returns the initial and final state of
* the case.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param resolveCaseRequest
* @return A Java Future containing the result of the ResolveCase operation returned by the service.
* @sample AWSSupportAsync.ResolveCase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resolveCaseAsync(ResolveCaseRequest resolveCaseRequest);
/**
*
* Resolves a support case. This operation takes a caseId
and returns the initial and final state of
* the case.
*
*
*
* -
*
* You must have a Business, Enterprise On-Ramp, or Enterprise Support plan to use the Amazon Web Services Support
* API.
*
*
* -
*
* If you call the Amazon Web Services Support API from an account that doesn't have a Business, Enterprise On-Ramp,
* or Enterprise Support plan, the SubscriptionRequiredException
error message appears. For information
* about changing your support plan, see Amazon Web Services
* Support.
*
*
*
*
*
* @param resolveCaseRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResolveCase operation returned by the service.
* @sample AWSSupportAsyncHandler.ResolveCase
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resolveCaseAsync(ResolveCaseRequest resolveCaseRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ResolveCase operation.
*
* @see #resolveCaseAsync(ResolveCaseRequest)
*/
java.util.concurrent.Future resolveCaseAsync();
/**
* Simplified method form for invoking the ResolveCase operation with an AsyncHandler.
*
* @see #resolveCaseAsync(ResolveCaseRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future resolveCaseAsync(com.amazonaws.handlers.AsyncHandler asyncHandler);
}