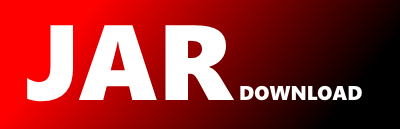
com.amazonaws.services.supportapp.AWSSupportApp Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.supportapp;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.supportapp.model.*;
/**
* Interface for accessing SupportApp.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.supportapp.AbstractAWSSupportApp} instead.
*
*
*
* Amazon Web Services Support App in Slack
*
* You can use the Amazon Web Services Support App in Slack API to manage your support cases in Slack for your Amazon
* Web Services account. After you configure your Slack workspace and channel with the Amazon Web Services Support App,
* you can perform the following tasks directly in your Slack channel:
*
*
* -
*
* Create, search, update, and resolve your support cases
*
*
* -
*
* Request service quota increases for your account
*
*
* -
*
* Invite Amazon Web Services Support agents to your channel so that you can chat directly about your support cases
*
*
*
*
* For more information about how to perform these actions in Slack, see the following documentation in the Amazon
* Web Services Support User Guide:
*
*
* -
*
*
* -
*
* Joining a live chat
* session with Amazon Web Services Support
*
*
* -
*
*
* -
*
*
*
*
* You can also use the Amazon Web Services Management Console instead of the Amazon Web Services Support App API to
* manage your Slack configurations. For more information, see Authorize a Slack workspace
* to enable the Amazon Web Services Support App.
*
*
*
* -
*
* You must have a Business or Enterprise Support plan to use the Amazon Web Services Support App API.
*
*
* -
*
* For more information about the Amazon Web Services Support App endpoints, see the Amazon Web Services
* Support App in Slack endpoints in the Amazon Web Services General Reference.
*
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSSupportApp {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "supportapp";
/**
*
* Creates a Slack channel configuration for your Amazon Web Services account.
*
*
*
* -
*
* You can add up to 5 Slack workspaces for your account.
*
*
* -
*
* You can add up to 20 Slack channels for your account.
*
*
*
*
*
* A Slack channel can have up to 100 Amazon Web Services accounts. This means that only 100 accounts can add the
* same Slack channel to the Amazon Web Services Support App. We recommend that you only add the accounts that you
* need to manage support cases for your organization. This can reduce the notifications about case updates that you
* receive in the Slack channel.
*
*
*
* We recommend that you choose a private Slack channel so that only members in that channel have read and write
* access to your support cases. Anyone in your Slack channel can create, update, or resolve support cases for your
* account. Users require an invitation to join private channels.
*
*
*
* @param createSlackChannelConfigurationRequest
* @return Result of the CreateSlackChannelConfiguration operation returned by the service.
* @throws ServiceQuotaExceededException
* Your Service Quotas request exceeds the quota for the service. For example, your Service Quotas request
* to Amazon Web Services Support App might exceed the maximum number of workspaces or channels per account,
* or the maximum number of accounts per Slack channel.
* @throws ConflictException
* Your request has a conflict. For example, you might receive this error if you try the following:
*
* -
*
* Add, update, or delete a Slack channel configuration before you add a Slack workspace to your Amazon Web
* Services account.
*
*
* -
*
* Add a Slack channel configuration that already exists in your Amazon Web Services account.
*
*
* -
*
* Delete a Slack channel configuration for a live chat channel.
*
*
* -
*
* Delete a Slack workspace from your Amazon Web Services account that has an active live chat channel.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from an Amazon Web Services account that
* doesn't belong to an organization.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from a member account, but the management
* account hasn't registered that workspace yet for the organization.
*
*
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.CreateSlackChannelConfiguration
* @see AWS API Documentation
*/
CreateSlackChannelConfigurationResult createSlackChannelConfiguration(CreateSlackChannelConfigurationRequest createSlackChannelConfigurationRequest);
/**
*
* Deletes an alias for an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support App
* page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param deleteAccountAliasRequest
* @return Result of the DeleteAccountAlias operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource is missing or doesn't exist, such as an account alias, Slack channel
* configuration, or Slack workspace configuration.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @sample AWSSupportApp.DeleteAccountAlias
* @see AWS
* API Documentation
*/
DeleteAccountAliasResult deleteAccountAlias(DeleteAccountAliasRequest deleteAccountAliasRequest);
/**
*
* Deletes a Slack channel configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack channel.
*
*
* @param deleteSlackChannelConfigurationRequest
* @return Result of the DeleteSlackChannelConfiguration operation returned by the service.
* @throws ConflictException
* Your request has a conflict. For example, you might receive this error if you try the following:
*
* -
*
* Add, update, or delete a Slack channel configuration before you add a Slack workspace to your Amazon Web
* Services account.
*
*
* -
*
* Add a Slack channel configuration that already exists in your Amazon Web Services account.
*
*
* -
*
* Delete a Slack channel configuration for a live chat channel.
*
*
* -
*
* Delete a Slack workspace from your Amazon Web Services account that has an active live chat channel.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from an Amazon Web Services account that
* doesn't belong to an organization.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from a member account, but the management
* account hasn't registered that workspace yet for the organization.
*
*
* @throws ResourceNotFoundException
* The specified resource is missing or doesn't exist, such as an account alias, Slack channel
* configuration, or Slack workspace configuration.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.DeleteSlackChannelConfiguration
* @see AWS API Documentation
*/
DeleteSlackChannelConfigurationResult deleteSlackChannelConfiguration(DeleteSlackChannelConfigurationRequest deleteSlackChannelConfigurationRequest);
/**
*
* Deletes a Slack workspace configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack workspace.
*
*
* @param deleteSlackWorkspaceConfigurationRequest
* @return Result of the DeleteSlackWorkspaceConfiguration operation returned by the service.
* @throws ConflictException
* Your request has a conflict. For example, you might receive this error if you try the following:
*
* -
*
* Add, update, or delete a Slack channel configuration before you add a Slack workspace to your Amazon Web
* Services account.
*
*
* -
*
* Add a Slack channel configuration that already exists in your Amazon Web Services account.
*
*
* -
*
* Delete a Slack channel configuration for a live chat channel.
*
*
* -
*
* Delete a Slack workspace from your Amazon Web Services account that has an active live chat channel.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from an Amazon Web Services account that
* doesn't belong to an organization.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from a member account, but the management
* account hasn't registered that workspace yet for the organization.
*
*
* @throws ResourceNotFoundException
* The specified resource is missing or doesn't exist, such as an account alias, Slack channel
* configuration, or Slack workspace configuration.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.DeleteSlackWorkspaceConfiguration
* @see AWS API Documentation
*/
DeleteSlackWorkspaceConfigurationResult deleteSlackWorkspaceConfiguration(DeleteSlackWorkspaceConfigurationRequest deleteSlackWorkspaceConfigurationRequest);
/**
*
* Retrieves the alias from an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support
* App page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param getAccountAliasRequest
* @return Result of the GetAccountAlias operation returned by the service.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @sample AWSSupportApp.GetAccountAlias
* @see AWS
* API Documentation
*/
GetAccountAliasResult getAccountAlias(GetAccountAliasRequest getAccountAliasRequest);
/**
*
* Lists the Slack channel configurations for an Amazon Web Services account.
*
*
* @param listSlackChannelConfigurationsRequest
* @return Result of the ListSlackChannelConfigurations operation returned by the service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @sample AWSSupportApp.ListSlackChannelConfigurations
* @see AWS API Documentation
*/
ListSlackChannelConfigurationsResult listSlackChannelConfigurations(ListSlackChannelConfigurationsRequest listSlackChannelConfigurationsRequest);
/**
*
* Lists the Slack workspace configurations for an Amazon Web Services account.
*
*
* @param listSlackWorkspaceConfigurationsRequest
* @return Result of the ListSlackWorkspaceConfigurations operation returned by the service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @sample AWSSupportApp.ListSlackWorkspaceConfigurations
* @see AWS API Documentation
*/
ListSlackWorkspaceConfigurationsResult listSlackWorkspaceConfigurations(ListSlackWorkspaceConfigurationsRequest listSlackWorkspaceConfigurationsRequest);
/**
*
* Creates or updates an individual alias for each Amazon Web Services account ID. The alias appears in the Amazon
* Web Services Support App page of the Amazon Web Services Support Center. The alias also appears in Slack messages
* from the Amazon Web Services Support App.
*
*
* @param putAccountAliasRequest
* @return Result of the PutAccountAlias operation returned by the service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.PutAccountAlias
* @see AWS
* API Documentation
*/
PutAccountAliasResult putAccountAlias(PutAccountAliasRequest putAccountAliasRequest);
/**
*
* Registers a Slack workspace for your Amazon Web Services account. To call this API, your account must be part of
* an organization in Organizations.
*
*
* If you're the management account and you want to register Slack workspaces for your organization, you must
* complete the following tasks:
*
*
* -
*
* Sign in to the Amazon Web Services Support Center and
* authorize the Slack workspaces where you want your organization to have access to. See Authorize a Slack
* workspace in the Amazon Web Services Support User Guide.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API to authorize each Slack workspace for the
* organization.
*
*
*
*
* After the management account authorizes the Slack workspace, member accounts can call this API to authorize the
* same Slack workspace for their individual accounts. Member accounts don't need to authorize the Slack workspace
* manually through the Amazon Web Services Support Center.
*
*
* To use the Amazon Web Services Support App, each account must then complete the following tasks:
*
*
* -
*
* Create an Identity and Access Management (IAM) role with the required permission. For more information, see Managing access to the
* Amazon Web Services Support App.
*
*
* -
*
* Configure a Slack channel to use the Amazon Web Services Support App for support cases for that account. For more
* information, see Configuring a Slack
* channel.
*
*
*
*
* @param registerSlackWorkspaceForOrganizationRequest
* @return Result of the RegisterSlackWorkspaceForOrganization operation returned by the service.
* @throws ConflictException
* Your request has a conflict. For example, you might receive this error if you try the following:
*
* -
*
* Add, update, or delete a Slack channel configuration before you add a Slack workspace to your Amazon Web
* Services account.
*
*
* -
*
* Add a Slack channel configuration that already exists in your Amazon Web Services account.
*
*
* -
*
* Delete a Slack channel configuration for a live chat channel.
*
*
* -
*
* Delete a Slack workspace from your Amazon Web Services account that has an active live chat channel.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from an Amazon Web Services account that
* doesn't belong to an organization.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from a member account, but the management
* account hasn't registered that workspace yet for the organization.
*
*
* @throws ResourceNotFoundException
* The specified resource is missing or doesn't exist, such as an account alias, Slack channel
* configuration, or Slack workspace configuration.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.RegisterSlackWorkspaceForOrganization
* @see AWS API Documentation
*/
RegisterSlackWorkspaceForOrganizationResult registerSlackWorkspaceForOrganization(
RegisterSlackWorkspaceForOrganizationRequest registerSlackWorkspaceForOrganizationRequest);
/**
*
* Updates the configuration for a Slack channel, such as case update notifications.
*
*
* @param updateSlackChannelConfigurationRequest
* @return Result of the UpdateSlackChannelConfiguration operation returned by the service.
* @throws ConflictException
* Your request has a conflict. For example, you might receive this error if you try the following:
*
* -
*
* Add, update, or delete a Slack channel configuration before you add a Slack workspace to your Amazon Web
* Services account.
*
*
* -
*
* Add a Slack channel configuration that already exists in your Amazon Web Services account.
*
*
* -
*
* Delete a Slack channel configuration for a live chat channel.
*
*
* -
*
* Delete a Slack workspace from your Amazon Web Services account that has an active live chat channel.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from an Amazon Web Services account that
* doesn't belong to an organization.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API from a member account, but the management
* account hasn't registered that workspace yet for the organization.
*
*
* @throws ResourceNotFoundException
* The specified resource is missing or doesn't exist, such as an account alias, Slack channel
* configuration, or Slack workspace configuration.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws InternalServerException
* We can’t process your request right now because of a server issue. Try again later.
* @throws ValidationException
* Your request input doesn't meet the constraints that the Amazon Web Services Support App specifies.
* @sample AWSSupportApp.UpdateSlackChannelConfiguration
* @see AWS API Documentation
*/
UpdateSlackChannelConfigurationResult updateSlackChannelConfiguration(UpdateSlackChannelConfigurationRequest updateSlackChannelConfigurationRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}