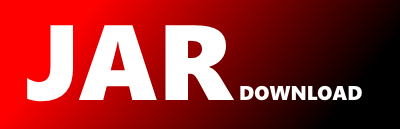
com.amazonaws.services.supportapp.AWSSupportAppAsync Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.supportapp;
import javax.annotation.Generated;
import com.amazonaws.services.supportapp.model.*;
/**
* Interface for accessing SupportApp asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.supportapp.AbstractAWSSupportAppAsync} instead.
*
*
*
* Amazon Web Services Support App in Slack
*
* You can use the Amazon Web Services Support App in Slack API to manage your support cases in Slack for your Amazon
* Web Services account. After you configure your Slack workspace and channel with the Amazon Web Services Support App,
* you can perform the following tasks directly in your Slack channel:
*
*
* -
*
* Create, search, update, and resolve your support cases
*
*
* -
*
* Request service quota increases for your account
*
*
* -
*
* Invite Amazon Web Services Support agents to your channel so that you can chat directly about your support cases
*
*
*
*
* For more information about how to perform these actions in Slack, see the following documentation in the Amazon
* Web Services Support User Guide:
*
*
* -
*
*
* -
*
* Joining a live chat
* session with Amazon Web Services Support
*
*
* -
*
*
* -
*
*
*
*
* You can also use the Amazon Web Services Management Console instead of the Amazon Web Services Support App API to
* manage your Slack configurations. For more information, see Authorize a Slack workspace
* to enable the Amazon Web Services Support App.
*
*
*
* -
*
* You must have a Business or Enterprise Support plan to use the Amazon Web Services Support App API.
*
*
* -
*
* For more information about the Amazon Web Services Support App endpoints, see the Amazon Web Services
* Support App in Slack endpoints in the Amazon Web Services General Reference.
*
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSSupportAppAsync extends AWSSupportApp {
/**
*
* Creates a Slack channel configuration for your Amazon Web Services account.
*
*
*
* -
*
* You can add up to 5 Slack workspaces for your account.
*
*
* -
*
* You can add up to 20 Slack channels for your account.
*
*
*
*
*
* A Slack channel can have up to 100 Amazon Web Services accounts. This means that only 100 accounts can add the
* same Slack channel to the Amazon Web Services Support App. We recommend that you only add the accounts that you
* need to manage support cases for your organization. This can reduce the notifications about case updates that you
* receive in the Slack channel.
*
*
*
* We recommend that you choose a private Slack channel so that only members in that channel have read and write
* access to your support cases. Anyone in your Slack channel can create, update, or resolve support cases for your
* account. Users require an invitation to join private channels.
*
*
*
* @param createSlackChannelConfigurationRequest
* @return A Java Future containing the result of the CreateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsync.CreateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlackChannelConfigurationAsync(
CreateSlackChannelConfigurationRequest createSlackChannelConfigurationRequest);
/**
*
* Creates a Slack channel configuration for your Amazon Web Services account.
*
*
*
* -
*
* You can add up to 5 Slack workspaces for your account.
*
*
* -
*
* You can add up to 20 Slack channels for your account.
*
*
*
*
*
* A Slack channel can have up to 100 Amazon Web Services accounts. This means that only 100 accounts can add the
* same Slack channel to the Amazon Web Services Support App. We recommend that you only add the accounts that you
* need to manage support cases for your organization. This can reduce the notifications about case updates that you
* receive in the Slack channel.
*
*
*
* We recommend that you choose a private Slack channel so that only members in that channel have read and write
* access to your support cases. Anyone in your Slack channel can create, update, or resolve support cases for your
* account. Users require an invitation to join private channels.
*
*
*
* @param createSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.CreateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlackChannelConfigurationAsync(
CreateSlackChannelConfigurationRequest createSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an alias for an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support App
* page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param deleteAccountAliasRequest
* @return A Java Future containing the result of the DeleteAccountAlias operation returned by the service.
* @sample AWSSupportAppAsync.DeleteAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAccountAliasAsync(DeleteAccountAliasRequest deleteAccountAliasRequest);
/**
*
* Deletes an alias for an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support App
* page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param deleteAccountAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccountAlias operation returned by the service.
* @sample AWSSupportAppAsyncHandler.DeleteAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteAccountAliasAsync(DeleteAccountAliasRequest deleteAccountAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Slack channel configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack channel.
*
*
* @param deleteSlackChannelConfigurationRequest
* @return A Java Future containing the result of the DeleteSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsync.DeleteSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackChannelConfigurationAsync(
DeleteSlackChannelConfigurationRequest deleteSlackChannelConfigurationRequest);
/**
*
* Deletes a Slack channel configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack channel.
*
*
* @param deleteSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.DeleteSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackChannelConfigurationAsync(
DeleteSlackChannelConfigurationRequest deleteSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Slack workspace configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack workspace.
*
*
* @param deleteSlackWorkspaceConfigurationRequest
* @return A Java Future containing the result of the DeleteSlackWorkspaceConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsync.DeleteSlackWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackWorkspaceConfigurationAsync(
DeleteSlackWorkspaceConfigurationRequest deleteSlackWorkspaceConfigurationRequest);
/**
*
* Deletes a Slack workspace configuration from your Amazon Web Services account. This operation doesn't delete your
* Slack workspace.
*
*
* @param deleteSlackWorkspaceConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlackWorkspaceConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.DeleteSlackWorkspaceConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackWorkspaceConfigurationAsync(
DeleteSlackWorkspaceConfigurationRequest deleteSlackWorkspaceConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the alias from an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support
* App page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param getAccountAliasRequest
* @return A Java Future containing the result of the GetAccountAlias operation returned by the service.
* @sample AWSSupportAppAsync.GetAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountAliasAsync(GetAccountAliasRequest getAccountAliasRequest);
/**
*
* Retrieves the alias from an Amazon Web Services account ID. The alias appears in the Amazon Web Services Support
* App page of the Amazon Web Services Support Center. The alias also appears in Slack messages from the Amazon Web
* Services Support App.
*
*
* @param getAccountAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountAlias operation returned by the service.
* @sample AWSSupportAppAsyncHandler.GetAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountAliasAsync(GetAccountAliasRequest getAccountAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Slack channel configurations for an Amazon Web Services account.
*
*
* @param listSlackChannelConfigurationsRequest
* @return A Java Future containing the result of the ListSlackChannelConfigurations operation returned by the
* service.
* @sample AWSSupportAppAsync.ListSlackChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSlackChannelConfigurationsAsync(
ListSlackChannelConfigurationsRequest listSlackChannelConfigurationsRequest);
/**
*
* Lists the Slack channel configurations for an Amazon Web Services account.
*
*
* @param listSlackChannelConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSlackChannelConfigurations operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.ListSlackChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSlackChannelConfigurationsAsync(
ListSlackChannelConfigurationsRequest listSlackChannelConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Slack workspace configurations for an Amazon Web Services account.
*
*
* @param listSlackWorkspaceConfigurationsRequest
* @return A Java Future containing the result of the ListSlackWorkspaceConfigurations operation returned by the
* service.
* @sample AWSSupportAppAsync.ListSlackWorkspaceConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSlackWorkspaceConfigurationsAsync(
ListSlackWorkspaceConfigurationsRequest listSlackWorkspaceConfigurationsRequest);
/**
*
* Lists the Slack workspace configurations for an Amazon Web Services account.
*
*
* @param listSlackWorkspaceConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSlackWorkspaceConfigurations operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.ListSlackWorkspaceConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listSlackWorkspaceConfigurationsAsync(
ListSlackWorkspaceConfigurationsRequest listSlackWorkspaceConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates an individual alias for each Amazon Web Services account ID. The alias appears in the Amazon
* Web Services Support App page of the Amazon Web Services Support Center. The alias also appears in Slack messages
* from the Amazon Web Services Support App.
*
*
* @param putAccountAliasRequest
* @return A Java Future containing the result of the PutAccountAlias operation returned by the service.
* @sample AWSSupportAppAsync.PutAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putAccountAliasAsync(PutAccountAliasRequest putAccountAliasRequest);
/**
*
* Creates or updates an individual alias for each Amazon Web Services account ID. The alias appears in the Amazon
* Web Services Support App page of the Amazon Web Services Support Center. The alias also appears in Slack messages
* from the Amazon Web Services Support App.
*
*
* @param putAccountAliasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAccountAlias operation returned by the service.
* @sample AWSSupportAppAsyncHandler.PutAccountAlias
* @see AWS
* API Documentation
*/
java.util.concurrent.Future putAccountAliasAsync(PutAccountAliasRequest putAccountAliasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers a Slack workspace for your Amazon Web Services account. To call this API, your account must be part of
* an organization in Organizations.
*
*
* If you're the management account and you want to register Slack workspaces for your organization, you must
* complete the following tasks:
*
*
* -
*
* Sign in to the Amazon Web Services Support Center and
* authorize the Slack workspaces where you want your organization to have access to. See Authorize a Slack
* workspace in the Amazon Web Services Support User Guide.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API to authorize each Slack workspace for the
* organization.
*
*
*
*
* After the management account authorizes the Slack workspace, member accounts can call this API to authorize the
* same Slack workspace for their individual accounts. Member accounts don't need to authorize the Slack workspace
* manually through the Amazon Web Services Support Center.
*
*
* To use the Amazon Web Services Support App, each account must then complete the following tasks:
*
*
* -
*
* Create an Identity and Access Management (IAM) role with the required permission. For more information, see Managing access to the
* Amazon Web Services Support App.
*
*
* -
*
* Configure a Slack channel to use the Amazon Web Services Support App for support cases for that account. For more
* information, see Configuring a Slack
* channel.
*
*
*
*
* @param registerSlackWorkspaceForOrganizationRequest
* @return A Java Future containing the result of the RegisterSlackWorkspaceForOrganization operation returned by
* the service.
* @sample AWSSupportAppAsync.RegisterSlackWorkspaceForOrganization
* @see AWS API Documentation
*/
java.util.concurrent.Future registerSlackWorkspaceForOrganizationAsync(
RegisterSlackWorkspaceForOrganizationRequest registerSlackWorkspaceForOrganizationRequest);
/**
*
* Registers a Slack workspace for your Amazon Web Services account. To call this API, your account must be part of
* an organization in Organizations.
*
*
* If you're the management account and you want to register Slack workspaces for your organization, you must
* complete the following tasks:
*
*
* -
*
* Sign in to the Amazon Web Services Support Center and
* authorize the Slack workspaces where you want your organization to have access to. See Authorize a Slack
* workspace in the Amazon Web Services Support User Guide.
*
*
* -
*
* Call the RegisterSlackWorkspaceForOrganization
API to authorize each Slack workspace for the
* organization.
*
*
*
*
* After the management account authorizes the Slack workspace, member accounts can call this API to authorize the
* same Slack workspace for their individual accounts. Member accounts don't need to authorize the Slack workspace
* manually through the Amazon Web Services Support Center.
*
*
* To use the Amazon Web Services Support App, each account must then complete the following tasks:
*
*
* -
*
* Create an Identity and Access Management (IAM) role with the required permission. For more information, see Managing access to the
* Amazon Web Services Support App.
*
*
* -
*
* Configure a Slack channel to use the Amazon Web Services Support App for support cases for that account. For more
* information, see Configuring a Slack
* channel.
*
*
*
*
* @param registerSlackWorkspaceForOrganizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterSlackWorkspaceForOrganization operation returned by
* the service.
* @sample AWSSupportAppAsyncHandler.RegisterSlackWorkspaceForOrganization
* @see AWS API Documentation
*/
java.util.concurrent.Future registerSlackWorkspaceForOrganizationAsync(
RegisterSlackWorkspaceForOrganizationRequest registerSlackWorkspaceForOrganizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the configuration for a Slack channel, such as case update notifications.
*
*
* @param updateSlackChannelConfigurationRequest
* @return A Java Future containing the result of the UpdateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsync.UpdateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSlackChannelConfigurationAsync(
UpdateSlackChannelConfigurationRequest updateSlackChannelConfigurationRequest);
/**
*
* Updates the configuration for a Slack channel, such as case update notifications.
*
*
* @param updateSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSSupportAppAsyncHandler.UpdateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSlackChannelConfigurationAsync(
UpdateSlackChannelConfigurationRequest updateSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}