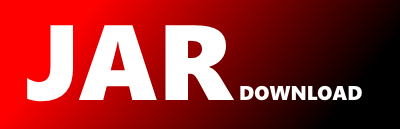
com.amazonaws.services.supportapp.model.UpdateSlackChannelConfigurationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-supportapp Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.supportapp.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateSlackChannelConfigurationRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*
*/
private String channelId;
/**
*
* The Slack channel name that you want to update.
*
*/
private String channelName;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web Services.
* For more information, see Managing access to the
* Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*
*/
private String channelRoleArn;
/**
*
* Whether you want to get notified when a support case has a new correspondence.
*
*/
private Boolean notifyOnAddCorrespondenceToCase;
/**
*
* The case severity for a support case that you want to receive notifications.
*
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the current
* values by default.
*
*
*/
private String notifyOnCaseSeverity;
/**
*
* Whether you want to get notified when a support case is created or reopened.
*
*/
private Boolean notifyOnCreateOrReopenCase;
/**
*
* Whether you want to get notified when a support case is resolved.
*
*/
private Boolean notifyOnResolveCase;
/**
*
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*
*/
private String teamId;
/**
*
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*
*
* @param channelId
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*/
public void setChannelId(String channelId) {
this.channelId = channelId;
}
/**
*
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*
*
* @return The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*/
public String getChannelId() {
return this.channelId;
}
/**
*
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
*
*
* @param channelId
* The channel ID in Slack. This ID identifies a channel within a Slack workspace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withChannelId(String channelId) {
setChannelId(channelId);
return this;
}
/**
*
* The Slack channel name that you want to update.
*
*
* @param channelName
* The Slack channel name that you want to update.
*/
public void setChannelName(String channelName) {
this.channelName = channelName;
}
/**
*
* The Slack channel name that you want to update.
*
*
* @return The Slack channel name that you want to update.
*/
public String getChannelName() {
return this.channelName;
}
/**
*
* The Slack channel name that you want to update.
*
*
* @param channelName
* The Slack channel name that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withChannelName(String channelName) {
setChannelName(channelName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web Services.
* For more information, see Managing access to the
* Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*
*
* @param channelRoleArn
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web
* Services. For more information, see Managing access to
* the Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*/
public void setChannelRoleArn(String channelRoleArn) {
this.channelRoleArn = channelRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web Services.
* For more information, see Managing access to the
* Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web
* Services. For more information, see Managing access to
* the Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*/
public String getChannelRoleArn() {
return this.channelRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web Services.
* For more information, see Managing access to the
* Amazon Web Services Support App in the Amazon Web Services Support User Guide.
*
*
* @param channelRoleArn
* The Amazon Resource Name (ARN) of an IAM role that you want to use to perform operations on Amazon Web
* Services. For more information, see Managing access to
* the Amazon Web Services Support App in the Amazon Web Services Support User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withChannelRoleArn(String channelRoleArn) {
setChannelRoleArn(channelRoleArn);
return this;
}
/**
*
* Whether you want to get notified when a support case has a new correspondence.
*
*
* @param notifyOnAddCorrespondenceToCase
* Whether you want to get notified when a support case has a new correspondence.
*/
public void setNotifyOnAddCorrespondenceToCase(Boolean notifyOnAddCorrespondenceToCase) {
this.notifyOnAddCorrespondenceToCase = notifyOnAddCorrespondenceToCase;
}
/**
*
* Whether you want to get notified when a support case has a new correspondence.
*
*
* @return Whether you want to get notified when a support case has a new correspondence.
*/
public Boolean getNotifyOnAddCorrespondenceToCase() {
return this.notifyOnAddCorrespondenceToCase;
}
/**
*
* Whether you want to get notified when a support case has a new correspondence.
*
*
* @param notifyOnAddCorrespondenceToCase
* Whether you want to get notified when a support case has a new correspondence.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withNotifyOnAddCorrespondenceToCase(Boolean notifyOnAddCorrespondenceToCase) {
setNotifyOnAddCorrespondenceToCase(notifyOnAddCorrespondenceToCase);
return this;
}
/**
*
* Whether you want to get notified when a support case has a new correspondence.
*
*
* @return Whether you want to get notified when a support case has a new correspondence.
*/
public Boolean isNotifyOnAddCorrespondenceToCase() {
return this.notifyOnAddCorrespondenceToCase;
}
/**
*
* The case severity for a support case that you want to receive notifications.
*
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the current
* values by default.
*
*
*
* @param notifyOnCaseSeverity
* The case severity for a support case that you want to receive notifications.
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the
* current values by default.
*
* @see NotificationSeverityLevel
*/
public void setNotifyOnCaseSeverity(String notifyOnCaseSeverity) {
this.notifyOnCaseSeverity = notifyOnCaseSeverity;
}
/**
*
* The case severity for a support case that you want to receive notifications.
*
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the current
* values by default.
*
*
*
* @return The case severity for a support case that you want to receive notifications.
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must
* be false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the
* current values by default.
*
* @see NotificationSeverityLevel
*/
public String getNotifyOnCaseSeverity() {
return this.notifyOnCaseSeverity;
}
/**
*
* The case severity for a support case that you want to receive notifications.
*
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the current
* values by default.
*
*
*
* @param notifyOnCaseSeverity
* The case severity for a support case that you want to receive notifications.
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the
* current values by default.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotificationSeverityLevel
*/
public UpdateSlackChannelConfigurationRequest withNotifyOnCaseSeverity(String notifyOnCaseSeverity) {
setNotifyOnCaseSeverity(notifyOnCaseSeverity);
return this;
}
/**
*
* The case severity for a support case that you want to receive notifications.
*
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the current
* values by default.
*
*
*
* @param notifyOnCaseSeverity
* The case severity for a support case that you want to receive notifications.
*
* If you specify high
or all
, at least one of the following parameters must be
* true
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
* If you specify none
, any of the following parameters that you specify in your request must be
* false
:
*
*
* -
*
* notifyOnAddCorrespondenceToCase
*
*
* -
*
* notifyOnCreateOrReopenCase
*
*
* -
*
* notifyOnResolveCase
*
*
*
*
*
* If you don't specify these parameters in your request, the Amazon Web Services Support App uses the
* current values by default.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotificationSeverityLevel
*/
public UpdateSlackChannelConfigurationRequest withNotifyOnCaseSeverity(NotificationSeverityLevel notifyOnCaseSeverity) {
this.notifyOnCaseSeverity = notifyOnCaseSeverity.toString();
return this;
}
/**
*
* Whether you want to get notified when a support case is created or reopened.
*
*
* @param notifyOnCreateOrReopenCase
* Whether you want to get notified when a support case is created or reopened.
*/
public void setNotifyOnCreateOrReopenCase(Boolean notifyOnCreateOrReopenCase) {
this.notifyOnCreateOrReopenCase = notifyOnCreateOrReopenCase;
}
/**
*
* Whether you want to get notified when a support case is created or reopened.
*
*
* @return Whether you want to get notified when a support case is created or reopened.
*/
public Boolean getNotifyOnCreateOrReopenCase() {
return this.notifyOnCreateOrReopenCase;
}
/**
*
* Whether you want to get notified when a support case is created or reopened.
*
*
* @param notifyOnCreateOrReopenCase
* Whether you want to get notified when a support case is created or reopened.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withNotifyOnCreateOrReopenCase(Boolean notifyOnCreateOrReopenCase) {
setNotifyOnCreateOrReopenCase(notifyOnCreateOrReopenCase);
return this;
}
/**
*
* Whether you want to get notified when a support case is created or reopened.
*
*
* @return Whether you want to get notified when a support case is created or reopened.
*/
public Boolean isNotifyOnCreateOrReopenCase() {
return this.notifyOnCreateOrReopenCase;
}
/**
*
* Whether you want to get notified when a support case is resolved.
*
*
* @param notifyOnResolveCase
* Whether you want to get notified when a support case is resolved.
*/
public void setNotifyOnResolveCase(Boolean notifyOnResolveCase) {
this.notifyOnResolveCase = notifyOnResolveCase;
}
/**
*
* Whether you want to get notified when a support case is resolved.
*
*
* @return Whether you want to get notified when a support case is resolved.
*/
public Boolean getNotifyOnResolveCase() {
return this.notifyOnResolveCase;
}
/**
*
* Whether you want to get notified when a support case is resolved.
*
*
* @param notifyOnResolveCase
* Whether you want to get notified when a support case is resolved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withNotifyOnResolveCase(Boolean notifyOnResolveCase) {
setNotifyOnResolveCase(notifyOnResolveCase);
return this;
}
/**
*
* Whether you want to get notified when a support case is resolved.
*
*
* @return Whether you want to get notified when a support case is resolved.
*/
public Boolean isNotifyOnResolveCase() {
return this.notifyOnResolveCase;
}
/**
*
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*
*
* @param teamId
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*/
public void setTeamId(String teamId) {
this.teamId = teamId;
}
/**
*
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*
*
* @return The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*/
public String getTeamId() {
return this.teamId;
}
/**
*
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
*
*
* @param teamId
* The team ID in Slack. This ID uniquely identifies a Slack workspace, such as T012ABCDEFG
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateSlackChannelConfigurationRequest withTeamId(String teamId) {
setTeamId(teamId);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getChannelId() != null)
sb.append("ChannelId: ").append(getChannelId()).append(",");
if (getChannelName() != null)
sb.append("ChannelName: ").append(getChannelName()).append(",");
if (getChannelRoleArn() != null)
sb.append("ChannelRoleArn: ").append(getChannelRoleArn()).append(",");
if (getNotifyOnAddCorrespondenceToCase() != null)
sb.append("NotifyOnAddCorrespondenceToCase: ").append(getNotifyOnAddCorrespondenceToCase()).append(",");
if (getNotifyOnCaseSeverity() != null)
sb.append("NotifyOnCaseSeverity: ").append(getNotifyOnCaseSeverity()).append(",");
if (getNotifyOnCreateOrReopenCase() != null)
sb.append("NotifyOnCreateOrReopenCase: ").append(getNotifyOnCreateOrReopenCase()).append(",");
if (getNotifyOnResolveCase() != null)
sb.append("NotifyOnResolveCase: ").append(getNotifyOnResolveCase()).append(",");
if (getTeamId() != null)
sb.append("TeamId: ").append(getTeamId());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateSlackChannelConfigurationRequest == false)
return false;
UpdateSlackChannelConfigurationRequest other = (UpdateSlackChannelConfigurationRequest) obj;
if (other.getChannelId() == null ^ this.getChannelId() == null)
return false;
if (other.getChannelId() != null && other.getChannelId().equals(this.getChannelId()) == false)
return false;
if (other.getChannelName() == null ^ this.getChannelName() == null)
return false;
if (other.getChannelName() != null && other.getChannelName().equals(this.getChannelName()) == false)
return false;
if (other.getChannelRoleArn() == null ^ this.getChannelRoleArn() == null)
return false;
if (other.getChannelRoleArn() != null && other.getChannelRoleArn().equals(this.getChannelRoleArn()) == false)
return false;
if (other.getNotifyOnAddCorrespondenceToCase() == null ^ this.getNotifyOnAddCorrespondenceToCase() == null)
return false;
if (other.getNotifyOnAddCorrespondenceToCase() != null
&& other.getNotifyOnAddCorrespondenceToCase().equals(this.getNotifyOnAddCorrespondenceToCase()) == false)
return false;
if (other.getNotifyOnCaseSeverity() == null ^ this.getNotifyOnCaseSeverity() == null)
return false;
if (other.getNotifyOnCaseSeverity() != null && other.getNotifyOnCaseSeverity().equals(this.getNotifyOnCaseSeverity()) == false)
return false;
if (other.getNotifyOnCreateOrReopenCase() == null ^ this.getNotifyOnCreateOrReopenCase() == null)
return false;
if (other.getNotifyOnCreateOrReopenCase() != null && other.getNotifyOnCreateOrReopenCase().equals(this.getNotifyOnCreateOrReopenCase()) == false)
return false;
if (other.getNotifyOnResolveCase() == null ^ this.getNotifyOnResolveCase() == null)
return false;
if (other.getNotifyOnResolveCase() != null && other.getNotifyOnResolveCase().equals(this.getNotifyOnResolveCase()) == false)
return false;
if (other.getTeamId() == null ^ this.getTeamId() == null)
return false;
if (other.getTeamId() != null && other.getTeamId().equals(this.getTeamId()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getChannelId() == null) ? 0 : getChannelId().hashCode());
hashCode = prime * hashCode + ((getChannelName() == null) ? 0 : getChannelName().hashCode());
hashCode = prime * hashCode + ((getChannelRoleArn() == null) ? 0 : getChannelRoleArn().hashCode());
hashCode = prime * hashCode + ((getNotifyOnAddCorrespondenceToCase() == null) ? 0 : getNotifyOnAddCorrespondenceToCase().hashCode());
hashCode = prime * hashCode + ((getNotifyOnCaseSeverity() == null) ? 0 : getNotifyOnCaseSeverity().hashCode());
hashCode = prime * hashCode + ((getNotifyOnCreateOrReopenCase() == null) ? 0 : getNotifyOnCreateOrReopenCase().hashCode());
hashCode = prime * hashCode + ((getNotifyOnResolveCase() == null) ? 0 : getNotifyOnResolveCase().hashCode());
hashCode = prime * hashCode + ((getTeamId() == null) ? 0 : getTeamId().hashCode());
return hashCode;
}
@Override
public UpdateSlackChannelConfigurationRequest clone() {
return (UpdateSlackChannelConfigurationRequest) super.clone();
}
}