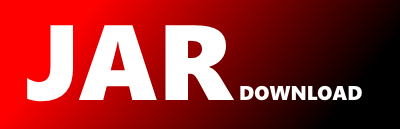
com.amazonaws.services.synthetics.model.CreateCanaryRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-synthetics Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.synthetics.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateCanaryRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries in your
* account.
*
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of the
* canary ARN, and the ARN is included in outbound calls over the internet. For more information, see Security
* Considerations for Synthetics Canaries.
*
*/
private String name;
/**
*
* A structure that includes the entry point from which the canary should start running your script. If the script
* is stored in an S3 bucket, the bucket name, key, and version are also included.
*
*/
private CanaryCodeInput code;
/**
*
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts include
* the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*
*/
private String artifactS3Location;
/**
*
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the following
* permissions:
*
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*
*/
private String executionRoleArn;
/**
*
* A structure that contains information about how often the canary is to run and when these test runs are to stop.
*
*/
private CanaryScheduleInput schedule;
/**
*
* A structure that contains the configuration for individual canary runs, such as timeout value and environment
* variables.
*
*
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this field.
*
*
*/
private CanaryRunConfigInput runConfig;
/**
*
* The number of days to retain data about successful runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*/
private Integer successRetentionPeriodInDays;
/**
*
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*/
private Integer failureRetentionPeriodInDays;
/**
*
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more information
* about runtime versions, see
* Canary Runtime Versions.
*
*/
private String runtimeVersion;
/**
*
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and security
* groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*
*/
private VpcConfigInput vpcConfig;
/**
*
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a canary.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only the resources that have certain tag values.
*
*/
private java.util.Map tags;
/**
*
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest settings for
* artifacts that the canary uploads to Amazon S3.
*
*/
private ArtifactConfigInput artifactConfig;
/**
*
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries in your
* account.
*
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of the
* canary ARN, and the ARN is included in outbound calls over the internet. For more information, see Security
* Considerations for Synthetics Canaries.
*
*
* @param name
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries
* in your account.
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of
* the canary ARN, and the ARN is included in outbound calls over the internet. For more information, see
* Security Considerations for Synthetics Canaries.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries in your
* account.
*
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of the
* canary ARN, and the ARN is included in outbound calls over the internet. For more information, see Security
* Considerations for Synthetics Canaries.
*
*
* @return The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries
* in your account.
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of
* the canary ARN, and the ARN is included in outbound calls over the internet. For more information, see
* Security Considerations for Synthetics Canaries.
*/
public String getName() {
return this.name;
}
/**
*
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries in your
* account.
*
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of the
* canary ARN, and the ARN is included in outbound calls over the internet. For more information, see Security
* Considerations for Synthetics Canaries.
*
*
* @param name
* The name for this canary. Be sure to give it a descriptive name that distinguishes it from other canaries
* in your account.
*
* Do not include secrets or proprietary information in your canary names. The canary name makes up part of
* the canary ARN, and the ARN is included in outbound calls over the internet. For more information, see
* Security Considerations for Synthetics Canaries.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withName(String name) {
setName(name);
return this;
}
/**
*
* A structure that includes the entry point from which the canary should start running your script. If the script
* is stored in an S3 bucket, the bucket name, key, and version are also included.
*
*
* @param code
* A structure that includes the entry point from which the canary should start running your script. If the
* script is stored in an S3 bucket, the bucket name, key, and version are also included.
*/
public void setCode(CanaryCodeInput code) {
this.code = code;
}
/**
*
* A structure that includes the entry point from which the canary should start running your script. If the script
* is stored in an S3 bucket, the bucket name, key, and version are also included.
*
*
* @return A structure that includes the entry point from which the canary should start running your script. If the
* script is stored in an S3 bucket, the bucket name, key, and version are also included.
*/
public CanaryCodeInput getCode() {
return this.code;
}
/**
*
* A structure that includes the entry point from which the canary should start running your script. If the script
* is stored in an S3 bucket, the bucket name, key, and version are also included.
*
*
* @param code
* A structure that includes the entry point from which the canary should start running your script. If the
* script is stored in an S3 bucket, the bucket name, key, and version are also included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withCode(CanaryCodeInput code) {
setCode(code);
return this;
}
/**
*
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts include
* the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*
*
* @param artifactS3Location
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts
* include the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*/
public void setArtifactS3Location(String artifactS3Location) {
this.artifactS3Location = artifactS3Location;
}
/**
*
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts include
* the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*
*
* @return The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts
* include the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*/
public String getArtifactS3Location() {
return this.artifactS3Location;
}
/**
*
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts include
* the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
*
*
* @param artifactS3Location
* The location in Amazon S3 where Synthetics stores artifacts from the test runs of this canary. Artifacts
* include the log file, screenshots, and HAR files. The name of the S3 bucket can't include a period (.).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withArtifactS3Location(String artifactS3Location) {
setArtifactS3Location(artifactS3Location);
return this;
}
/**
*
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the following
* permissions:
*
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*
*
* @param executionRoleArn
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the
* following permissions:
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the following
* permissions:
*
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*
*
* @return The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the
* following permissions:
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the following
* permissions:
*
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
*
*
* @param executionRoleArn
* The ARN of the IAM role to be used to run the canary. This role must already exist, and must include
* lambda.amazonaws.com
as a principal in the trust policy. The role must also have the
* following permissions:
*
* -
*
* s3:PutObject
*
*
* -
*
* s3:GetBucketLocation
*
*
* -
*
* s3:ListAllMyBuckets
*
*
* -
*
* cloudwatch:PutMetricData
*
*
* -
*
* logs:CreateLogGroup
*
*
* -
*
* logs:CreateLogStream
*
*
* -
*
* logs:PutLogEvents
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* A structure that contains information about how often the canary is to run and when these test runs are to stop.
*
*
* @param schedule
* A structure that contains information about how often the canary is to run and when these test runs are to
* stop.
*/
public void setSchedule(CanaryScheduleInput schedule) {
this.schedule = schedule;
}
/**
*
* A structure that contains information about how often the canary is to run and when these test runs are to stop.
*
*
* @return A structure that contains information about how often the canary is to run and when these test runs are
* to stop.
*/
public CanaryScheduleInput getSchedule() {
return this.schedule;
}
/**
*
* A structure that contains information about how often the canary is to run and when these test runs are to stop.
*
*
* @param schedule
* A structure that contains information about how often the canary is to run and when these test runs are to
* stop.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withSchedule(CanaryScheduleInput schedule) {
setSchedule(schedule);
return this;
}
/**
*
* A structure that contains the configuration for individual canary runs, such as timeout value and environment
* variables.
*
*
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this field.
*
*
*
* @param runConfig
* A structure that contains the configuration for individual canary runs, such as timeout value and
* environment variables.
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this
* field.
*
*/
public void setRunConfig(CanaryRunConfigInput runConfig) {
this.runConfig = runConfig;
}
/**
*
* A structure that contains the configuration for individual canary runs, such as timeout value and environment
* variables.
*
*
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this field.
*
*
*
* @return A structure that contains the configuration for individual canary runs, such as timeout value and
* environment variables.
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this
* field.
*
*/
public CanaryRunConfigInput getRunConfig() {
return this.runConfig;
}
/**
*
* A structure that contains the configuration for individual canary runs, such as timeout value and environment
* variables.
*
*
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this field.
*
*
*
* @param runConfig
* A structure that contains the configuration for individual canary runs, such as timeout value and
* environment variables.
*
* The environment variables keys and values are not encrypted. Do not store sensitive information in this
* field.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withRunConfig(CanaryRunConfigInput runConfig) {
setRunConfig(runConfig);
return this;
}
/**
*
* The number of days to retain data about successful runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @param successRetentionPeriodInDays
* The number of days to retain data about successful runs of this canary. If you omit this field, the
* default of 31 days is used. The valid range is 1 to 455 days.
*/
public void setSuccessRetentionPeriodInDays(Integer successRetentionPeriodInDays) {
this.successRetentionPeriodInDays = successRetentionPeriodInDays;
}
/**
*
* The number of days to retain data about successful runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @return The number of days to retain data about successful runs of this canary. If you omit this field, the
* default of 31 days is used. The valid range is 1 to 455 days.
*/
public Integer getSuccessRetentionPeriodInDays() {
return this.successRetentionPeriodInDays;
}
/**
*
* The number of days to retain data about successful runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @param successRetentionPeriodInDays
* The number of days to retain data about successful runs of this canary. If you omit this field, the
* default of 31 days is used. The valid range is 1 to 455 days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withSuccessRetentionPeriodInDays(Integer successRetentionPeriodInDays) {
setSuccessRetentionPeriodInDays(successRetentionPeriodInDays);
return this;
}
/**
*
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @param failureRetentionPeriodInDays
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of
* 31 days is used. The valid range is 1 to 455 days.
*/
public void setFailureRetentionPeriodInDays(Integer failureRetentionPeriodInDays) {
this.failureRetentionPeriodInDays = failureRetentionPeriodInDays;
}
/**
*
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @return The number of days to retain data about failed runs of this canary. If you omit this field, the default
* of 31 days is used. The valid range is 1 to 455 days.
*/
public Integer getFailureRetentionPeriodInDays() {
return this.failureRetentionPeriodInDays;
}
/**
*
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of 31
* days is used. The valid range is 1 to 455 days.
*
*
* @param failureRetentionPeriodInDays
* The number of days to retain data about failed runs of this canary. If you omit this field, the default of
* 31 days is used. The valid range is 1 to 455 days.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withFailureRetentionPeriodInDays(Integer failureRetentionPeriodInDays) {
setFailureRetentionPeriodInDays(failureRetentionPeriodInDays);
return this;
}
/**
*
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more information
* about runtime versions, see
* Canary Runtime Versions.
*
*
* @param runtimeVersion
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more
* information about runtime versions, see Canary Runtime Versions.
*/
public void setRuntimeVersion(String runtimeVersion) {
this.runtimeVersion = runtimeVersion;
}
/**
*
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more information
* about runtime versions, see
* Canary Runtime Versions.
*
*
* @return Specifies the runtime version to use for the canary. For a list of valid runtime versions and more
* information about runtime versions, see Canary Runtime Versions.
*/
public String getRuntimeVersion() {
return this.runtimeVersion;
}
/**
*
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more information
* about runtime versions, see
* Canary Runtime Versions.
*
*
* @param runtimeVersion
* Specifies the runtime version to use for the canary. For a list of valid runtime versions and more
* information about runtime versions, see Canary Runtime Versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withRuntimeVersion(String runtimeVersion) {
setRuntimeVersion(runtimeVersion);
return this;
}
/**
*
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and security
* groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*
*
* @param vpcConfig
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and
* security groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*/
public void setVpcConfig(VpcConfigInput vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and security
* groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*
*
* @return If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and
* security groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*/
public VpcConfigInput getVpcConfig() {
return this.vpcConfig;
}
/**
*
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and security
* groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
*
*
* @param vpcConfig
* If this canary is to test an endpoint in a VPC, this structure contains information about the subnet and
* security groups of the VPC endpoint. For more information, see
* Running a Canary in a VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withVpcConfig(VpcConfigInput vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a canary.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only the resources that have certain tag values.
*
*
* @return A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a
* canary.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user
* permissions, by granting a user permission to access or change only the resources that have certain tag
* values.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a canary.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only the resources that have certain tag values.
*
*
* @param tags
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a
* canary.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions,
* by granting a user permission to access or change only the resources that have certain tag values.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a canary.
*
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions, by
* granting a user permission to access or change only the resources that have certain tag values.
*
*
* @param tags
* A list of key-value pairs to associate with the canary. You can associate as many as 50 tags with a
* canary.
*
* Tags can help you organize and categorize your resources. You can also use them to scope user permissions,
* by granting a user permission to access or change only the resources that have certain tag values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateCanaryRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest settings for
* artifacts that the canary uploads to Amazon S3.
*
*
* @param artifactConfig
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest
* settings for artifacts that the canary uploads to Amazon S3.
*/
public void setArtifactConfig(ArtifactConfigInput artifactConfig) {
this.artifactConfig = artifactConfig;
}
/**
*
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest settings for
* artifacts that the canary uploads to Amazon S3.
*
*
* @return A structure that contains the configuration for canary artifacts, including the encryption-at-rest
* settings for artifacts that the canary uploads to Amazon S3.
*/
public ArtifactConfigInput getArtifactConfig() {
return this.artifactConfig;
}
/**
*
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest settings for
* artifacts that the canary uploads to Amazon S3.
*
*
* @param artifactConfig
* A structure that contains the configuration for canary artifacts, including the encryption-at-rest
* settings for artifacts that the canary uploads to Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCanaryRequest withArtifactConfig(ArtifactConfigInput artifactConfig) {
setArtifactConfig(artifactConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getCode() != null)
sb.append("Code: ").append(getCode()).append(",");
if (getArtifactS3Location() != null)
sb.append("ArtifactS3Location: ").append(getArtifactS3Location()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getSchedule() != null)
sb.append("Schedule: ").append(getSchedule()).append(",");
if (getRunConfig() != null)
sb.append("RunConfig: ").append(getRunConfig()).append(",");
if (getSuccessRetentionPeriodInDays() != null)
sb.append("SuccessRetentionPeriodInDays: ").append(getSuccessRetentionPeriodInDays()).append(",");
if (getFailureRetentionPeriodInDays() != null)
sb.append("FailureRetentionPeriodInDays: ").append(getFailureRetentionPeriodInDays()).append(",");
if (getRuntimeVersion() != null)
sb.append("RuntimeVersion: ").append(getRuntimeVersion()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getArtifactConfig() != null)
sb.append("ArtifactConfig: ").append(getArtifactConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCanaryRequest == false)
return false;
CreateCanaryRequest other = (CreateCanaryRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getCode() == null ^ this.getCode() == null)
return false;
if (other.getCode() != null && other.getCode().equals(this.getCode()) == false)
return false;
if (other.getArtifactS3Location() == null ^ this.getArtifactS3Location() == null)
return false;
if (other.getArtifactS3Location() != null && other.getArtifactS3Location().equals(this.getArtifactS3Location()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getSchedule() == null ^ this.getSchedule() == null)
return false;
if (other.getSchedule() != null && other.getSchedule().equals(this.getSchedule()) == false)
return false;
if (other.getRunConfig() == null ^ this.getRunConfig() == null)
return false;
if (other.getRunConfig() != null && other.getRunConfig().equals(this.getRunConfig()) == false)
return false;
if (other.getSuccessRetentionPeriodInDays() == null ^ this.getSuccessRetentionPeriodInDays() == null)
return false;
if (other.getSuccessRetentionPeriodInDays() != null && other.getSuccessRetentionPeriodInDays().equals(this.getSuccessRetentionPeriodInDays()) == false)
return false;
if (other.getFailureRetentionPeriodInDays() == null ^ this.getFailureRetentionPeriodInDays() == null)
return false;
if (other.getFailureRetentionPeriodInDays() != null && other.getFailureRetentionPeriodInDays().equals(this.getFailureRetentionPeriodInDays()) == false)
return false;
if (other.getRuntimeVersion() == null ^ this.getRuntimeVersion() == null)
return false;
if (other.getRuntimeVersion() != null && other.getRuntimeVersion().equals(this.getRuntimeVersion()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getArtifactConfig() == null ^ this.getArtifactConfig() == null)
return false;
if (other.getArtifactConfig() != null && other.getArtifactConfig().equals(this.getArtifactConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getCode() == null) ? 0 : getCode().hashCode());
hashCode = prime * hashCode + ((getArtifactS3Location() == null) ? 0 : getArtifactS3Location().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getSchedule() == null) ? 0 : getSchedule().hashCode());
hashCode = prime * hashCode + ((getRunConfig() == null) ? 0 : getRunConfig().hashCode());
hashCode = prime * hashCode + ((getSuccessRetentionPeriodInDays() == null) ? 0 : getSuccessRetentionPeriodInDays().hashCode());
hashCode = prime * hashCode + ((getFailureRetentionPeriodInDays() == null) ? 0 : getFailureRetentionPeriodInDays().hashCode());
hashCode = prime * hashCode + ((getRuntimeVersion() == null) ? 0 : getRuntimeVersion().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getArtifactConfig() == null) ? 0 : getArtifactConfig().hashCode());
return hashCode;
}
@Override
public CreateCanaryRequest clone() {
return (CreateCanaryRequest) super.clone();
}
}