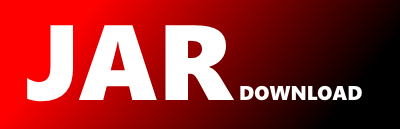
com.amazonaws.services.taxsettings.model.AdditionalInfoRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-taxsettings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.taxsettings.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Additional tax information associated with your tax registration number (TRN). Depending on the TRN for a specific
* country, you might need to specify this information when you set your TRN.
*
*
* You can only specify one of the following parameters and the value can't be empty.
*
*
*
* The parameter that you specify must match the country for the TRN, if available. For example, if you set a TRN in
* Canada for specific provinces, you must also specify the canadaAdditionalInfo
parameter.
*
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AdditionalInfoRequest implements Serializable, Cloneable, StructuredPojo {
/**
*
* Additional tax information associated with your TRN in Canada.
*
*/
private CanadaAdditionalInfo canadaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Estonia.
*
*/
private EstoniaAdditionalInfo estoniaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Georgia.
*
*/
private GeorgiaAdditionalInfo georgiaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Israel.
*
*/
private IsraelAdditionalInfo israelAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Italy.
*
*/
private ItalyAdditionalInfo italyAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Kenya.
*
*/
private KenyaAdditionalInfo kenyaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Malaysia.
*
*/
private MalaysiaAdditionalInfo malaysiaAdditionalInfo;
/**
*
* Additional tax information associated with your TRN in Poland.
*
*/
private PolandAdditionalInfo polandAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Romania.
*
*/
private RomaniaAdditionalInfo romaniaAdditionalInfo;
/**
*
* Additional tax information associated with your TRN in Saudi Arabia.
*
*/
private SaudiArabiaAdditionalInfo saudiArabiaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in South Korea.
*
*/
private SouthKoreaAdditionalInfo southKoreaAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Spain.
*
*/
private SpainAdditionalInfo spainAdditionalInfo;
/**
*
* Additional tax information to specify for a TRN in Turkey.
*
*/
private TurkeyAdditionalInfo turkeyAdditionalInfo;
/**
*
* Additional tax information associated with your TRN in Ukraine.
*
*/
private UkraineAdditionalInfo ukraineAdditionalInfo;
/**
*
* Additional tax information associated with your TRN in Canada.
*
*
* @param canadaAdditionalInfo
* Additional tax information associated with your TRN in Canada.
*/
public void setCanadaAdditionalInfo(CanadaAdditionalInfo canadaAdditionalInfo) {
this.canadaAdditionalInfo = canadaAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Canada.
*
*
* @return Additional tax information associated with your TRN in Canada.
*/
public CanadaAdditionalInfo getCanadaAdditionalInfo() {
return this.canadaAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Canada.
*
*
* @param canadaAdditionalInfo
* Additional tax information associated with your TRN in Canada.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withCanadaAdditionalInfo(CanadaAdditionalInfo canadaAdditionalInfo) {
setCanadaAdditionalInfo(canadaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Estonia.
*
*
* @param estoniaAdditionalInfo
* Additional tax information to specify for a TRN in Estonia.
*/
public void setEstoniaAdditionalInfo(EstoniaAdditionalInfo estoniaAdditionalInfo) {
this.estoniaAdditionalInfo = estoniaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Estonia.
*
*
* @return Additional tax information to specify for a TRN in Estonia.
*/
public EstoniaAdditionalInfo getEstoniaAdditionalInfo() {
return this.estoniaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Estonia.
*
*
* @param estoniaAdditionalInfo
* Additional tax information to specify for a TRN in Estonia.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withEstoniaAdditionalInfo(EstoniaAdditionalInfo estoniaAdditionalInfo) {
setEstoniaAdditionalInfo(estoniaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Georgia.
*
*
* @param georgiaAdditionalInfo
* Additional tax information to specify for a TRN in Georgia.
*/
public void setGeorgiaAdditionalInfo(GeorgiaAdditionalInfo georgiaAdditionalInfo) {
this.georgiaAdditionalInfo = georgiaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Georgia.
*
*
* @return Additional tax information to specify for a TRN in Georgia.
*/
public GeorgiaAdditionalInfo getGeorgiaAdditionalInfo() {
return this.georgiaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Georgia.
*
*
* @param georgiaAdditionalInfo
* Additional tax information to specify for a TRN in Georgia.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withGeorgiaAdditionalInfo(GeorgiaAdditionalInfo georgiaAdditionalInfo) {
setGeorgiaAdditionalInfo(georgiaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Israel.
*
*
* @param israelAdditionalInfo
* Additional tax information to specify for a TRN in Israel.
*/
public void setIsraelAdditionalInfo(IsraelAdditionalInfo israelAdditionalInfo) {
this.israelAdditionalInfo = israelAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Israel.
*
*
* @return Additional tax information to specify for a TRN in Israel.
*/
public IsraelAdditionalInfo getIsraelAdditionalInfo() {
return this.israelAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Israel.
*
*
* @param israelAdditionalInfo
* Additional tax information to specify for a TRN in Israel.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withIsraelAdditionalInfo(IsraelAdditionalInfo israelAdditionalInfo) {
setIsraelAdditionalInfo(israelAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Italy.
*
*
* @param italyAdditionalInfo
* Additional tax information to specify for a TRN in Italy.
*/
public void setItalyAdditionalInfo(ItalyAdditionalInfo italyAdditionalInfo) {
this.italyAdditionalInfo = italyAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Italy.
*
*
* @return Additional tax information to specify for a TRN in Italy.
*/
public ItalyAdditionalInfo getItalyAdditionalInfo() {
return this.italyAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Italy.
*
*
* @param italyAdditionalInfo
* Additional tax information to specify for a TRN in Italy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withItalyAdditionalInfo(ItalyAdditionalInfo italyAdditionalInfo) {
setItalyAdditionalInfo(italyAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Kenya.
*
*
* @param kenyaAdditionalInfo
* Additional tax information to specify for a TRN in Kenya.
*/
public void setKenyaAdditionalInfo(KenyaAdditionalInfo kenyaAdditionalInfo) {
this.kenyaAdditionalInfo = kenyaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Kenya.
*
*
* @return Additional tax information to specify for a TRN in Kenya.
*/
public KenyaAdditionalInfo getKenyaAdditionalInfo() {
return this.kenyaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Kenya.
*
*
* @param kenyaAdditionalInfo
* Additional tax information to specify for a TRN in Kenya.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withKenyaAdditionalInfo(KenyaAdditionalInfo kenyaAdditionalInfo) {
setKenyaAdditionalInfo(kenyaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Malaysia.
*
*
* @param malaysiaAdditionalInfo
* Additional tax information to specify for a TRN in Malaysia.
*/
public void setMalaysiaAdditionalInfo(MalaysiaAdditionalInfo malaysiaAdditionalInfo) {
this.malaysiaAdditionalInfo = malaysiaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Malaysia.
*
*
* @return Additional tax information to specify for a TRN in Malaysia.
*/
public MalaysiaAdditionalInfo getMalaysiaAdditionalInfo() {
return this.malaysiaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Malaysia.
*
*
* @param malaysiaAdditionalInfo
* Additional tax information to specify for a TRN in Malaysia.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withMalaysiaAdditionalInfo(MalaysiaAdditionalInfo malaysiaAdditionalInfo) {
setMalaysiaAdditionalInfo(malaysiaAdditionalInfo);
return this;
}
/**
*
* Additional tax information associated with your TRN in Poland.
*
*
* @param polandAdditionalInfo
* Additional tax information associated with your TRN in Poland.
*/
public void setPolandAdditionalInfo(PolandAdditionalInfo polandAdditionalInfo) {
this.polandAdditionalInfo = polandAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Poland.
*
*
* @return Additional tax information associated with your TRN in Poland.
*/
public PolandAdditionalInfo getPolandAdditionalInfo() {
return this.polandAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Poland.
*
*
* @param polandAdditionalInfo
* Additional tax information associated with your TRN in Poland.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withPolandAdditionalInfo(PolandAdditionalInfo polandAdditionalInfo) {
setPolandAdditionalInfo(polandAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Romania.
*
*
* @param romaniaAdditionalInfo
* Additional tax information to specify for a TRN in Romania.
*/
public void setRomaniaAdditionalInfo(RomaniaAdditionalInfo romaniaAdditionalInfo) {
this.romaniaAdditionalInfo = romaniaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Romania.
*
*
* @return Additional tax information to specify for a TRN in Romania.
*/
public RomaniaAdditionalInfo getRomaniaAdditionalInfo() {
return this.romaniaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Romania.
*
*
* @param romaniaAdditionalInfo
* Additional tax information to specify for a TRN in Romania.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withRomaniaAdditionalInfo(RomaniaAdditionalInfo romaniaAdditionalInfo) {
setRomaniaAdditionalInfo(romaniaAdditionalInfo);
return this;
}
/**
*
* Additional tax information associated with your TRN in Saudi Arabia.
*
*
* @param saudiArabiaAdditionalInfo
* Additional tax information associated with your TRN in Saudi Arabia.
*/
public void setSaudiArabiaAdditionalInfo(SaudiArabiaAdditionalInfo saudiArabiaAdditionalInfo) {
this.saudiArabiaAdditionalInfo = saudiArabiaAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Saudi Arabia.
*
*
* @return Additional tax information associated with your TRN in Saudi Arabia.
*/
public SaudiArabiaAdditionalInfo getSaudiArabiaAdditionalInfo() {
return this.saudiArabiaAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Saudi Arabia.
*
*
* @param saudiArabiaAdditionalInfo
* Additional tax information associated with your TRN in Saudi Arabia.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withSaudiArabiaAdditionalInfo(SaudiArabiaAdditionalInfo saudiArabiaAdditionalInfo) {
setSaudiArabiaAdditionalInfo(saudiArabiaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in South Korea.
*
*
* @param southKoreaAdditionalInfo
* Additional tax information to specify for a TRN in South Korea.
*/
public void setSouthKoreaAdditionalInfo(SouthKoreaAdditionalInfo southKoreaAdditionalInfo) {
this.southKoreaAdditionalInfo = southKoreaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in South Korea.
*
*
* @return Additional tax information to specify for a TRN in South Korea.
*/
public SouthKoreaAdditionalInfo getSouthKoreaAdditionalInfo() {
return this.southKoreaAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in South Korea.
*
*
* @param southKoreaAdditionalInfo
* Additional tax information to specify for a TRN in South Korea.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withSouthKoreaAdditionalInfo(SouthKoreaAdditionalInfo southKoreaAdditionalInfo) {
setSouthKoreaAdditionalInfo(southKoreaAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Spain.
*
*
* @param spainAdditionalInfo
* Additional tax information to specify for a TRN in Spain.
*/
public void setSpainAdditionalInfo(SpainAdditionalInfo spainAdditionalInfo) {
this.spainAdditionalInfo = spainAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Spain.
*
*
* @return Additional tax information to specify for a TRN in Spain.
*/
public SpainAdditionalInfo getSpainAdditionalInfo() {
return this.spainAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Spain.
*
*
* @param spainAdditionalInfo
* Additional tax information to specify for a TRN in Spain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withSpainAdditionalInfo(SpainAdditionalInfo spainAdditionalInfo) {
setSpainAdditionalInfo(spainAdditionalInfo);
return this;
}
/**
*
* Additional tax information to specify for a TRN in Turkey.
*
*
* @param turkeyAdditionalInfo
* Additional tax information to specify for a TRN in Turkey.
*/
public void setTurkeyAdditionalInfo(TurkeyAdditionalInfo turkeyAdditionalInfo) {
this.turkeyAdditionalInfo = turkeyAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Turkey.
*
*
* @return Additional tax information to specify for a TRN in Turkey.
*/
public TurkeyAdditionalInfo getTurkeyAdditionalInfo() {
return this.turkeyAdditionalInfo;
}
/**
*
* Additional tax information to specify for a TRN in Turkey.
*
*
* @param turkeyAdditionalInfo
* Additional tax information to specify for a TRN in Turkey.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withTurkeyAdditionalInfo(TurkeyAdditionalInfo turkeyAdditionalInfo) {
setTurkeyAdditionalInfo(turkeyAdditionalInfo);
return this;
}
/**
*
* Additional tax information associated with your TRN in Ukraine.
*
*
* @param ukraineAdditionalInfo
* Additional tax information associated with your TRN in Ukraine.
*/
public void setUkraineAdditionalInfo(UkraineAdditionalInfo ukraineAdditionalInfo) {
this.ukraineAdditionalInfo = ukraineAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Ukraine.
*
*
* @return Additional tax information associated with your TRN in Ukraine.
*/
public UkraineAdditionalInfo getUkraineAdditionalInfo() {
return this.ukraineAdditionalInfo;
}
/**
*
* Additional tax information associated with your TRN in Ukraine.
*
*
* @param ukraineAdditionalInfo
* Additional tax information associated with your TRN in Ukraine.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AdditionalInfoRequest withUkraineAdditionalInfo(UkraineAdditionalInfo ukraineAdditionalInfo) {
setUkraineAdditionalInfo(ukraineAdditionalInfo);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCanadaAdditionalInfo() != null)
sb.append("CanadaAdditionalInfo: ").append(getCanadaAdditionalInfo()).append(",");
if (getEstoniaAdditionalInfo() != null)
sb.append("EstoniaAdditionalInfo: ").append(getEstoniaAdditionalInfo()).append(",");
if (getGeorgiaAdditionalInfo() != null)
sb.append("GeorgiaAdditionalInfo: ").append(getGeorgiaAdditionalInfo()).append(",");
if (getIsraelAdditionalInfo() != null)
sb.append("IsraelAdditionalInfo: ").append(getIsraelAdditionalInfo()).append(",");
if (getItalyAdditionalInfo() != null)
sb.append("ItalyAdditionalInfo: ").append(getItalyAdditionalInfo()).append(",");
if (getKenyaAdditionalInfo() != null)
sb.append("KenyaAdditionalInfo: ").append(getKenyaAdditionalInfo()).append(",");
if (getMalaysiaAdditionalInfo() != null)
sb.append("MalaysiaAdditionalInfo: ").append(getMalaysiaAdditionalInfo()).append(",");
if (getPolandAdditionalInfo() != null)
sb.append("PolandAdditionalInfo: ").append(getPolandAdditionalInfo()).append(",");
if (getRomaniaAdditionalInfo() != null)
sb.append("RomaniaAdditionalInfo: ").append(getRomaniaAdditionalInfo()).append(",");
if (getSaudiArabiaAdditionalInfo() != null)
sb.append("SaudiArabiaAdditionalInfo: ").append(getSaudiArabiaAdditionalInfo()).append(",");
if (getSouthKoreaAdditionalInfo() != null)
sb.append("SouthKoreaAdditionalInfo: ").append(getSouthKoreaAdditionalInfo()).append(",");
if (getSpainAdditionalInfo() != null)
sb.append("SpainAdditionalInfo: ").append(getSpainAdditionalInfo()).append(",");
if (getTurkeyAdditionalInfo() != null)
sb.append("TurkeyAdditionalInfo: ").append(getTurkeyAdditionalInfo()).append(",");
if (getUkraineAdditionalInfo() != null)
sb.append("UkraineAdditionalInfo: ").append(getUkraineAdditionalInfo());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AdditionalInfoRequest == false)
return false;
AdditionalInfoRequest other = (AdditionalInfoRequest) obj;
if (other.getCanadaAdditionalInfo() == null ^ this.getCanadaAdditionalInfo() == null)
return false;
if (other.getCanadaAdditionalInfo() != null && other.getCanadaAdditionalInfo().equals(this.getCanadaAdditionalInfo()) == false)
return false;
if (other.getEstoniaAdditionalInfo() == null ^ this.getEstoniaAdditionalInfo() == null)
return false;
if (other.getEstoniaAdditionalInfo() != null && other.getEstoniaAdditionalInfo().equals(this.getEstoniaAdditionalInfo()) == false)
return false;
if (other.getGeorgiaAdditionalInfo() == null ^ this.getGeorgiaAdditionalInfo() == null)
return false;
if (other.getGeorgiaAdditionalInfo() != null && other.getGeorgiaAdditionalInfo().equals(this.getGeorgiaAdditionalInfo()) == false)
return false;
if (other.getIsraelAdditionalInfo() == null ^ this.getIsraelAdditionalInfo() == null)
return false;
if (other.getIsraelAdditionalInfo() != null && other.getIsraelAdditionalInfo().equals(this.getIsraelAdditionalInfo()) == false)
return false;
if (other.getItalyAdditionalInfo() == null ^ this.getItalyAdditionalInfo() == null)
return false;
if (other.getItalyAdditionalInfo() != null && other.getItalyAdditionalInfo().equals(this.getItalyAdditionalInfo()) == false)
return false;
if (other.getKenyaAdditionalInfo() == null ^ this.getKenyaAdditionalInfo() == null)
return false;
if (other.getKenyaAdditionalInfo() != null && other.getKenyaAdditionalInfo().equals(this.getKenyaAdditionalInfo()) == false)
return false;
if (other.getMalaysiaAdditionalInfo() == null ^ this.getMalaysiaAdditionalInfo() == null)
return false;
if (other.getMalaysiaAdditionalInfo() != null && other.getMalaysiaAdditionalInfo().equals(this.getMalaysiaAdditionalInfo()) == false)
return false;
if (other.getPolandAdditionalInfo() == null ^ this.getPolandAdditionalInfo() == null)
return false;
if (other.getPolandAdditionalInfo() != null && other.getPolandAdditionalInfo().equals(this.getPolandAdditionalInfo()) == false)
return false;
if (other.getRomaniaAdditionalInfo() == null ^ this.getRomaniaAdditionalInfo() == null)
return false;
if (other.getRomaniaAdditionalInfo() != null && other.getRomaniaAdditionalInfo().equals(this.getRomaniaAdditionalInfo()) == false)
return false;
if (other.getSaudiArabiaAdditionalInfo() == null ^ this.getSaudiArabiaAdditionalInfo() == null)
return false;
if (other.getSaudiArabiaAdditionalInfo() != null && other.getSaudiArabiaAdditionalInfo().equals(this.getSaudiArabiaAdditionalInfo()) == false)
return false;
if (other.getSouthKoreaAdditionalInfo() == null ^ this.getSouthKoreaAdditionalInfo() == null)
return false;
if (other.getSouthKoreaAdditionalInfo() != null && other.getSouthKoreaAdditionalInfo().equals(this.getSouthKoreaAdditionalInfo()) == false)
return false;
if (other.getSpainAdditionalInfo() == null ^ this.getSpainAdditionalInfo() == null)
return false;
if (other.getSpainAdditionalInfo() != null && other.getSpainAdditionalInfo().equals(this.getSpainAdditionalInfo()) == false)
return false;
if (other.getTurkeyAdditionalInfo() == null ^ this.getTurkeyAdditionalInfo() == null)
return false;
if (other.getTurkeyAdditionalInfo() != null && other.getTurkeyAdditionalInfo().equals(this.getTurkeyAdditionalInfo()) == false)
return false;
if (other.getUkraineAdditionalInfo() == null ^ this.getUkraineAdditionalInfo() == null)
return false;
if (other.getUkraineAdditionalInfo() != null && other.getUkraineAdditionalInfo().equals(this.getUkraineAdditionalInfo()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCanadaAdditionalInfo() == null) ? 0 : getCanadaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getEstoniaAdditionalInfo() == null) ? 0 : getEstoniaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getGeorgiaAdditionalInfo() == null) ? 0 : getGeorgiaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getIsraelAdditionalInfo() == null) ? 0 : getIsraelAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getItalyAdditionalInfo() == null) ? 0 : getItalyAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getKenyaAdditionalInfo() == null) ? 0 : getKenyaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getMalaysiaAdditionalInfo() == null) ? 0 : getMalaysiaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getPolandAdditionalInfo() == null) ? 0 : getPolandAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getRomaniaAdditionalInfo() == null) ? 0 : getRomaniaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getSaudiArabiaAdditionalInfo() == null) ? 0 : getSaudiArabiaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getSouthKoreaAdditionalInfo() == null) ? 0 : getSouthKoreaAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getSpainAdditionalInfo() == null) ? 0 : getSpainAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getTurkeyAdditionalInfo() == null) ? 0 : getTurkeyAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getUkraineAdditionalInfo() == null) ? 0 : getUkraineAdditionalInfo().hashCode());
return hashCode;
}
@Override
public AdditionalInfoRequest clone() {
try {
return (AdditionalInfoRequest) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.taxsettings.model.transform.AdditionalInfoRequestMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}