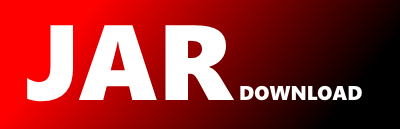
com.amazonaws.services.tnb.AWSTnb Maven / Gradle / Ivy
Show all versions of aws-java-sdk-tnb Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.tnb;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.tnb.model.*;
/**
* Interface for accessing AWS Telco Network Builder.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.tnb.AbstractAWSTnb} instead.
*
*
*
* Amazon Web Services Telco Network Builder (TNB) is a network automation service that helps you deploy and manage
* telecom networks. AWS TNB helps you with the lifecycle management of your telecommunication network functions
* throughout planning, deployment, and post-deployment activities.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSTnb {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "tnb";
/**
*
* Cancels a network operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param cancelSolNetworkOperationRequest
* @return Result of the CancelSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.CancelSolNetworkOperation
* @see AWS
* API Documentation
*/
CancelSolNetworkOperationResult cancelSolNetworkOperation(CancelSolNetworkOperationRequest cancelSolNetworkOperationRequest);
/**
*
* Creates a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network. For more information, see Function packages in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Creating a function package is the first step for creating a network in AWS TNB. This request creates an empty
* container with an ID. The next step is to upload the actual CSAR zip file into that empty container. To upload
* function package content, see PutSolFunctionPackageContent.
*
*
* @param createSolFunctionPackageRequest
* @return Result of the CreateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.CreateSolFunctionPackage
* @see AWS
* API Documentation
*/
CreateSolFunctionPackageResult createSolFunctionPackage(CreateSolFunctionPackageRequest createSolFunctionPackageRequest);
/**
*
* Creates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed. Creating a network instance is the
* third step after creating a network package. For more information about network instances, Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* Once you create a network instance, you can instantiate it. To instantiate a network, see InstantiateSolNetworkInstance.
*
*
* @param createSolNetworkInstanceRequest
* @return Result of the CreateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.CreateSolNetworkInstance
* @see AWS
* API Documentation
*/
CreateSolNetworkInstanceResult createSolNetworkInstance(CreateSolNetworkInstanceRequest createSolNetworkInstanceRequest);
/**
*
* Creates a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on. For more information, see Network instances in the Amazon
* Web Services Telco Network Builder User Guide.
*
*
* A network package consists of a network service descriptor (NSD) file (required) and any additional files
* (optional), such as scripts specific to your needs. For example, if you have multiple function packages in your
* network package, you can use the NSD to define which network functions should run in certain VPCs, subnets, or
* EKS clusters.
*
*
* This request creates an empty network package container with an ID. Once you create a network package, you can
* upload the network package content using PutSolNetworkPackageContent.
*
*
* @param createSolNetworkPackageRequest
* @return Result of the CreateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.CreateSolNetworkPackage
* @see AWS
* API Documentation
*/
CreateSolNetworkPackageResult createSolNetworkPackage(CreateSolNetworkPackageRequest createSolNetworkPackageRequest);
/**
*
* Deletes a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* To delete a function package, the package must be in a disabled state. To disable a function package, see UpdateSolFunctionPackage
* .
*
*
* @param deleteSolFunctionPackageRequest
* @return Result of the DeleteSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.DeleteSolFunctionPackage
* @see AWS
* API Documentation
*/
DeleteSolFunctionPackageResult deleteSolFunctionPackage(DeleteSolFunctionPackageRequest deleteSolFunctionPackageRequest);
/**
*
* Deletes a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* To delete a network instance, the instance must be in a stopped or terminated state. To terminate a network
* instance, see
* TerminateSolNetworkInstance.
*
*
* @param deleteSolNetworkInstanceRequest
* @return Result of the DeleteSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.DeleteSolNetworkInstance
* @see AWS
* API Documentation
*/
DeleteSolNetworkInstanceResult deleteSolNetworkInstance(DeleteSolNetworkInstanceRequest deleteSolNetworkInstanceRequest);
/**
*
* Deletes network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* To delete a network package, the package must be in a disable state. To disable a network package, see UpdateSolNetworkPackage.
*
*
* @param deleteSolNetworkPackageRequest
* @return Result of the DeleteSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.DeleteSolNetworkPackage
* @see AWS
* API Documentation
*/
DeleteSolNetworkPackageResult deleteSolNetworkPackage(DeleteSolNetworkPackageRequest deleteSolNetworkPackageRequest);
/**
*
* Gets the details of a network function instance, including the instantation state and metadata from the function
* package descriptor in the network function package.
*
*
* A network function instance is a function in a function package .
*
*
* @param getSolFunctionInstanceRequest
* @return Result of the GetSolFunctionInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolFunctionInstance
* @see AWS API
* Documentation
*/
GetSolFunctionInstanceResult getSolFunctionInstance(GetSolFunctionInstanceRequest getSolFunctionInstanceRequest);
/**
*
* Gets the details of an individual function package, such as the operational state and whether the package is in
* use.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network..
*
*
* @param getSolFunctionPackageRequest
* @return Result of the GetSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolFunctionPackage
* @see AWS API
* Documentation
*/
GetSolFunctionPackageResult getSolFunctionPackage(GetSolFunctionPackageRequest getSolFunctionPackageRequest);
/**
*
* Gets the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param getSolFunctionPackageContentRequest
* @return Result of the GetSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolFunctionPackageContent
* @see AWS API Documentation
*/
GetSolFunctionPackageContentResult getSolFunctionPackageContent(GetSolFunctionPackageContentRequest getSolFunctionPackageContentRequest);
/**
*
* Gets a function package descriptor in a function package.
*
*
* A function package descriptor is a .yaml file in a function package that uses the TOSCA standard to describe how
* the network function in the function package should run on your network.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param getSolFunctionPackageDescriptorRequest
* @return Result of the GetSolFunctionPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolFunctionPackageDescriptor
* @see AWS API Documentation
*/
GetSolFunctionPackageDescriptorResult getSolFunctionPackageDescriptor(GetSolFunctionPackageDescriptorRequest getSolFunctionPackageDescriptorRequest);
/**
*
* Gets the details of the network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* @param getSolNetworkInstanceRequest
* @return Result of the GetSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolNetworkInstance
* @see AWS API
* Documentation
*/
GetSolNetworkInstanceResult getSolNetworkInstance(GetSolNetworkInstanceRequest getSolNetworkInstanceRequest);
/**
*
* Gets the details of a network operation, including the tasks involved in the network operation and the status of
* the tasks.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param getSolNetworkOperationRequest
* @return Result of the GetSolNetworkOperation operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolNetworkOperation
* @see AWS API
* Documentation
*/
GetSolNetworkOperationResult getSolNetworkOperation(GetSolNetworkOperationRequest getSolNetworkOperationRequest);
/**
*
* Gets the details of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param getSolNetworkPackageRequest
* @return Result of the GetSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolNetworkPackage
* @see AWS API
* Documentation
*/
GetSolNetworkPackageResult getSolNetworkPackage(GetSolNetworkPackageRequest getSolNetworkPackageRequest);
/**
*
* Gets the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param getSolNetworkPackageContentRequest
* @return Result of the GetSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolNetworkPackageContent
* @see AWS API Documentation
*/
GetSolNetworkPackageContentResult getSolNetworkPackageContent(GetSolNetworkPackageContentRequest getSolNetworkPackageContentRequest);
/**
*
* Gets the content of the network service descriptor.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
* @param getSolNetworkPackageDescriptorRequest
* @return Result of the GetSolNetworkPackageDescriptor operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.GetSolNetworkPackageDescriptor
* @see AWS API Documentation
*/
GetSolNetworkPackageDescriptorResult getSolNetworkPackageDescriptor(GetSolNetworkPackageDescriptorRequest getSolNetworkPackageDescriptorRequest);
/**
*
* Instantiates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* Before you can instantiate a network instance, you have to create a network instance. For more information, see
*
* CreateSolNetworkInstance.
*
*
* @param instantiateSolNetworkInstanceRequest
* @return Result of the InstantiateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.InstantiateSolNetworkInstance
* @see AWS API Documentation
*/
InstantiateSolNetworkInstanceResult instantiateSolNetworkInstance(InstantiateSolNetworkInstanceRequest instantiateSolNetworkInstanceRequest);
/**
*
* Lists network function instances.
*
*
* A network function instance is a function in a function package .
*
*
* @param listSolFunctionInstancesRequest
* @return Result of the ListSolFunctionInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListSolFunctionInstances
* @see AWS
* API Documentation
*/
ListSolFunctionInstancesResult listSolFunctionInstances(ListSolFunctionInstancesRequest listSolFunctionInstancesRequest);
/**
*
* Lists information about function packages.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param listSolFunctionPackagesRequest
* @return Result of the ListSolFunctionPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListSolFunctionPackages
* @see AWS
* API Documentation
*/
ListSolFunctionPackagesResult listSolFunctionPackages(ListSolFunctionPackagesRequest listSolFunctionPackagesRequest);
/**
*
* Lists your network instances.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* @param listSolNetworkInstancesRequest
* @return Result of the ListSolNetworkInstances operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListSolNetworkInstances
* @see AWS
* API Documentation
*/
ListSolNetworkInstancesResult listSolNetworkInstances(ListSolNetworkInstancesRequest listSolNetworkInstancesRequest);
/**
*
* Lists details for a network operation, including when the operation started and the status of the operation.
*
*
* A network operation is any operation that is done to your network, such as network instance instantiation or
* termination.
*
*
* @param listSolNetworkOperationsRequest
* @return Result of the ListSolNetworkOperations operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListSolNetworkOperations
* @see AWS
* API Documentation
*/
ListSolNetworkOperationsResult listSolNetworkOperations(ListSolNetworkOperationsRequest listSolNetworkOperationsRequest);
/**
*
* Lists network packages.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param listSolNetworkPackagesRequest
* @return Result of the ListSolNetworkPackages operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListSolNetworkPackages
* @see AWS API
* Documentation
*/
ListSolNetworkPackagesResult listSolNetworkPackages(ListSolNetworkPackagesRequest listSolNetworkPackagesRequest);
/**
*
* Lists tags for AWS TNB resources.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Uploads the contents of a function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param putSolFunctionPackageContentRequest
* @return Result of the PutSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.PutSolFunctionPackageContent
* @see AWS API Documentation
*/
PutSolFunctionPackageContentResult putSolFunctionPackageContent(PutSolFunctionPackageContentRequest putSolFunctionPackageContentRequest);
/**
*
* Uploads the contents of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param putSolNetworkPackageContentRequest
* @return Result of the PutSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.PutSolNetworkPackageContent
* @see AWS API Documentation
*/
PutSolNetworkPackageContentResult putSolNetworkPackageContent(PutSolNetworkPackageContentRequest putSolNetworkPackageContentRequest);
/**
*
* Tags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Terminates a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* You must terminate a network instance before you can delete it.
*
*
* @param terminateSolNetworkInstanceRequest
* @return Result of the TerminateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.TerminateSolNetworkInstance
* @see AWS API Documentation
*/
TerminateSolNetworkInstanceResult terminateSolNetworkInstance(TerminateSolNetworkInstanceRequest terminateSolNetworkInstanceRequest);
/**
*
* Untags an AWS TNB resource.
*
*
* A tag is a label that you assign to an Amazon Web Services resource. Each tag consists of a key and an optional
* value. You can use tags to search and filter your resources or track your Amazon Web Services costs.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the operational state of function package.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param updateSolFunctionPackageRequest
* @return Result of the UpdateSolFunctionPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.UpdateSolFunctionPackage
* @see AWS
* API Documentation
*/
UpdateSolFunctionPackageResult updateSolFunctionPackage(UpdateSolFunctionPackageRequest updateSolFunctionPackageRequest);
/**
*
* Update a network instance.
*
*
* A network instance is a single network created in Amazon Web Services TNB that can be deployed and on which
* life-cycle operations (like terminate, update, and delete) can be performed.
*
*
* @param updateSolNetworkInstanceRequest
* @return Result of the UpdateSolNetworkInstance operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ServiceQuotaExceededException
* Service quotas have been exceeded.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.UpdateSolNetworkInstance
* @see AWS
* API Documentation
*/
UpdateSolNetworkInstanceResult updateSolNetworkInstance(UpdateSolNetworkInstanceRequest updateSolNetworkInstanceRequest);
/**
*
* Updates the operational state of a network package.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* A network service descriptor is a .yaml file in a network package that uses the TOSCA standard to describe the
* network functions you want to deploy and the Amazon Web Services infrastructure you want to deploy the network
* functions on.
*
*
* @param updateSolNetworkPackageRequest
* @return Result of the UpdateSolNetworkPackage operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.UpdateSolNetworkPackage
* @see AWS
* API Documentation
*/
UpdateSolNetworkPackageResult updateSolNetworkPackage(UpdateSolNetworkPackageRequest updateSolNetworkPackageRequest);
/**
*
* Validates function package content. This can be used as a dry run before uploading function package content with
*
* PutSolFunctionPackageContent.
*
*
* A function package is a .zip file in CSAR (Cloud Service Archive) format that contains a network function (an
* ETSI standard telecommunication application) and function package descriptor that uses the TOSCA standard to
* describe how the network functions should run on your network.
*
*
* @param validateSolFunctionPackageContentRequest
* @return Result of the ValidateSolFunctionPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ValidateSolFunctionPackageContent
* @see AWS API Documentation
*/
ValidateSolFunctionPackageContentResult validateSolFunctionPackageContent(ValidateSolFunctionPackageContentRequest validateSolFunctionPackageContentRequest);
/**
*
* Validates network package content. This can be used as a dry run before uploading network package content with
* PutSolNetworkPackageContent.
*
*
* A network package is a .zip file in CSAR (Cloud Service Archive) format defines the function packages you want to
* deploy and the Amazon Web Services infrastructure you want to deploy them on.
*
*
* @param validateSolNetworkPackageContentRequest
* @return Result of the ValidateSolNetworkPackageContent operation returned by the service.
* @throws InternalServerException
* Unexpected error occurred. Problem on the server.
* @throws ThrottlingException
* Exception caused by throttling.
* @throws ValidationException
* Unable to process the request because the client provided input failed to satisfy request constraints.
* @throws ResourceNotFoundException
* Request references a resource that doesn't exist.
* @throws AccessDeniedException
* Insufficient permissions to make request.
* @sample AWSTnb.ValidateSolNetworkPackageContent
* @see AWS API Documentation
*/
ValidateSolNetworkPackageContentResult validateSolNetworkPackageContent(ValidateSolNetworkPackageContentRequest validateSolNetworkPackageContentRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}