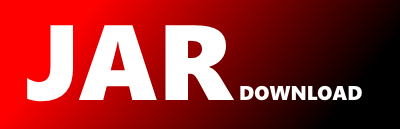
com.amazonaws.services.transcribe.AmazonTranscribeAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe;
import javax.annotation.Generated;
import com.amazonaws.services.transcribe.model.*;
/**
* Interface for accessing Amazon Transcribe Service asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.transcribe.AbstractAmazonTranscribeAsync} instead.
*
*
*
* Amazon Transcribe offers three main types of batch transcription: Standard, Medical, and Call
* Analytics.
*
*
* -
*
* Standard transcriptions are the most common option. Refer to for details.
*
*
* -
*
* Medical transcriptions are tailored to medical professionals and incorporate medical terms. A common use case
* for this service is transcribing doctor-patient dialogue into after-visit notes. Refer to for details.
*
*
* -
*
* Call Analytics transcriptions are designed for use with call center audio on two different channels; if you're
* looking for insight into customer service calls, use this option. Refer to for details.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonTranscribeAsync extends AmazonTranscribe {
/**
*
* Creates a new Call Analytics category.
*
*
* All categories are automatically applied to your Call Analytics transcriptions. Note that in order to apply
* categories to your transcriptions, you must create them before submitting your transcription request, as
* categories cannot be applied retroactively.
*
*
* When creating a new category, you can use the InputType
parameter to label the category as a
* POST_CALL
or a REAL_TIME
category. POST_CALL
categories can only be
* applied to post-call transcriptions and REAL_TIME
categories can only be applied to real-time
* transcriptions. If you do not include InputType
, your category is created as a
* POST_CALL
category by default.
*
*
* Call Analytics categories are composed of rules. For each category, you must create between 1 and 20 rules. Rules
* can include these parameters: , , , and .
*
*
* To update an existing category, see .
*
*
* To learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* @param createCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the CreateCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsync.CreateCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future createCallAnalyticsCategoryAsync(
CreateCallAnalyticsCategoryRequest createCallAnalyticsCategoryRequest);
/**
*
* Creates a new Call Analytics category.
*
*
* All categories are automatically applied to your Call Analytics transcriptions. Note that in order to apply
* categories to your transcriptions, you must create them before submitting your transcription request, as
* categories cannot be applied retroactively.
*
*
* When creating a new category, you can use the InputType
parameter to label the category as a
* POST_CALL
or a REAL_TIME
category. POST_CALL
categories can only be
* applied to post-call transcriptions and REAL_TIME
categories can only be applied to real-time
* transcriptions. If you do not include InputType
, your category is created as a
* POST_CALL
category by default.
*
*
* Call Analytics categories are composed of rules. For each category, you must create between 1 and 20 rules. Rules
* can include these parameters: , , , and .
*
*
* To update an existing category, see .
*
*
* To learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* @param createCallAnalyticsCategoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.CreateCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future createCallAnalyticsCategoryAsync(
CreateCallAnalyticsCategoryRequest createCallAnalyticsCategoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom language model.
*
*
* When creating a new custom language model, you must specify:
*
*
* -
*
* If you want a Wideband (audio sample rates over 16,000 Hz) or Narrowband (audio sample rates under 16,000 Hz)
* base model
*
*
* -
*
* The location of your training and tuning files (this must be an Amazon S3 URI)
*
*
* -
*
* The language of your model
*
*
* -
*
* A unique name for your model
*
*
*
*
* @param createLanguageModelRequest
* @return A Java Future containing the result of the CreateLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsync.CreateLanguageModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLanguageModelAsync(CreateLanguageModelRequest createLanguageModelRequest);
/**
*
* Creates a new custom language model.
*
*
* When creating a new custom language model, you must specify:
*
*
* -
*
* If you want a Wideband (audio sample rates over 16,000 Hz) or Narrowband (audio sample rates under 16,000 Hz)
* base model
*
*
* -
*
* The location of your training and tuning files (this must be an Amazon S3 URI)
*
*
* -
*
* The language of your model
*
*
* -
*
* A unique name for your model
*
*
*
*
* @param createLanguageModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.CreateLanguageModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createLanguageModelAsync(CreateLanguageModelRequest createLanguageModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom medical vocabulary.
*
*
* Before creating a new custom medical vocabulary, you must first upload a text file that contains your vocabulary
* table into an Amazon S3 bucket. Note that this differs from , where you can include a list of terms within your
* request using the Phrases
flag; CreateMedicalVocabulary
does not support the
* Phrases
flag and only accepts vocabularies in table format.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createMedicalVocabularyRequest
* @return A Java Future containing the result of the CreateMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.CreateMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future createMedicalVocabularyAsync(CreateMedicalVocabularyRequest createMedicalVocabularyRequest);
/**
*
* Creates a new custom medical vocabulary.
*
*
* Before creating a new custom medical vocabulary, you must first upload a text file that contains your vocabulary
* table into an Amazon S3 bucket. Note that this differs from , where you can include a list of terms within your
* request using the Phrases
flag; CreateMedicalVocabulary
does not support the
* Phrases
flag and only accepts vocabularies in table format.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createMedicalVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.CreateMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future createMedicalVocabularyAsync(CreateMedicalVocabularyRequest createMedicalVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom vocabulary.
*
*
* When creating a new custom vocabulary, you can either upload a text file that contains your new entries, phrases,
* and terms into an Amazon S3 bucket and include the URI in your request. Or you can include a list of terms
* directly in your request using the Phrases
flag.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createVocabularyRequest
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.CreateVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVocabularyAsync(CreateVocabularyRequest createVocabularyRequest);
/**
*
* Creates a new custom vocabulary.
*
*
* When creating a new custom vocabulary, you can either upload a text file that contains your new entries, phrases,
* and terms into an Amazon S3 bucket and include the URI in your request. Or you can include a list of terms
* directly in your request using the Phrases
flag.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Custom vocabularies.
*
*
* @param createVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.CreateVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createVocabularyAsync(CreateVocabularyRequest createVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new custom vocabulary filter.
*
*
* You can use custom vocabulary filters to mask, delete, or flag specific words from your transcript. Custom
* vocabulary filters are commonly used to mask profanity in transcripts.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Vocabulary filtering.
*
*
* @param createVocabularyFilterRequest
* @return A Java Future containing the result of the CreateVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsync.CreateVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future createVocabularyFilterAsync(CreateVocabularyFilterRequest createVocabularyFilterRequest);
/**
*
* Creates a new custom vocabulary filter.
*
*
* You can use custom vocabulary filters to mask, delete, or flag specific words from your transcript. Custom
* vocabulary filters are commonly used to mask profanity in transcripts.
*
*
* Each language has a character set that contains all allowed characters for that specific language. If you use
* unsupported characters, your custom vocabulary filter request fails. Refer to Character Sets for Custom Vocabularies
* to get the character set for your language.
*
*
* For more information, see Vocabulary filtering.
*
*
* @param createVocabularyFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.CreateVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future createVocabularyFilterAsync(CreateVocabularyFilterRequest createVocabularyFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Call Analytics category. To use this operation, specify the name of the category you want to delete
* using CategoryName
. Category names are case sensitive.
*
*
* @param deleteCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCallAnalyticsCategoryAsync(
DeleteCallAnalyticsCategoryRequest deleteCallAnalyticsCategoryRequest);
/**
*
* Deletes a Call Analytics category. To use this operation, specify the name of the category you want to delete
* using CategoryName
. Category names are case sensitive.
*
*
* @param deleteCallAnalyticsCategoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCallAnalyticsCategoryAsync(
DeleteCallAnalyticsCategoryRequest deleteCallAnalyticsCategoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Call Analytics job. To use this operation, specify the name of the job you want to delete using
* CallAnalyticsJobName
. Job names are case sensitive.
*
*
* @param deleteCallAnalyticsJobRequest
* @return A Java Future containing the result of the DeleteCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteCallAnalyticsJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCallAnalyticsJobAsync(DeleteCallAnalyticsJobRequest deleteCallAnalyticsJobRequest);
/**
*
* Deletes a Call Analytics job. To use this operation, specify the name of the job you want to delete using
* CallAnalyticsJobName
. Job names are case sensitive.
*
*
* @param deleteCallAnalyticsJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteCallAnalyticsJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCallAnalyticsJobAsync(DeleteCallAnalyticsJobRequest deleteCallAnalyticsJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom language model. To use this operation, specify the name of the language model you want to delete
* using ModelName
. custom language model names are case sensitive.
*
*
* @param deleteLanguageModelRequest
* @return A Java Future containing the result of the DeleteLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteLanguageModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLanguageModelAsync(DeleteLanguageModelRequest deleteLanguageModelRequest);
/**
*
* Deletes a custom language model. To use this operation, specify the name of the language model you want to delete
* using ModelName
. custom language model names are case sensitive.
*
*
* @param deleteLanguageModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteLanguageModel
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteLanguageModelAsync(DeleteLanguageModelRequest deleteLanguageModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a Medical Scribe job. To use this operation, specify the name of the job you want to delete using
* MedicalScribeJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalScribeJobRequest
* @return A Java Future containing the result of the DeleteMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteMedicalScribeJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalScribeJobAsync(DeleteMedicalScribeJobRequest deleteMedicalScribeJobRequest);
/**
*
* Deletes a Medical Scribe job. To use this operation, specify the name of the job you want to delete using
* MedicalScribeJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalScribeJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteMedicalScribeJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalScribeJobAsync(DeleteMedicalScribeJobRequest deleteMedicalScribeJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a medical transcription job. To use this operation, specify the name of the job you want to delete using
* MedicalTranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteMedicalTranscriptionJob operation returned by the
* service.
* @sample AmazonTranscribeAsync.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalTranscriptionJobAsync(
DeleteMedicalTranscriptionJobRequest deleteMedicalTranscriptionJobRequest);
/**
*
* Deletes a medical transcription job. To use this operation, specify the name of the job you want to delete using
* MedicalTranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteMedicalTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMedicalTranscriptionJob operation returned by the
* service.
* @sample AmazonTranscribeAsyncHandler.DeleteMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalTranscriptionJobAsync(
DeleteMedicalTranscriptionJobRequest deleteMedicalTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom medical vocabulary. To use this operation, specify the name of the custom vocabulary you want to
* delete using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteMedicalVocabularyRequest
* @return A Java Future containing the result of the DeleteMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalVocabularyAsync(DeleteMedicalVocabularyRequest deleteMedicalVocabularyRequest);
/**
*
* Deletes a custom medical vocabulary. To use this operation, specify the name of the custom vocabulary you want to
* delete using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteMedicalVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMedicalVocabularyAsync(DeleteMedicalVocabularyRequest deleteMedicalVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a transcription job. To use this operation, specify the name of the job you want to delete using
* TranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteTranscriptionJobRequest
* @return A Java Future containing the result of the DeleteTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTranscriptionJobAsync(DeleteTranscriptionJobRequest deleteTranscriptionJobRequest);
/**
*
* Deletes a transcription job. To use this operation, specify the name of the job you want to delete using
* TranscriptionJobName
. Job names are case sensitive.
*
*
* @param deleteTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteTranscriptionJobAsync(DeleteTranscriptionJobRequest deleteTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom vocabulary. To use this operation, specify the name of the custom vocabulary you want to delete
* using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteVocabularyRequest
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVocabularyAsync(DeleteVocabularyRequest deleteVocabularyRequest);
/**
*
* Deletes a custom vocabulary. To use this operation, specify the name of the custom vocabulary you want to delete
* using VocabularyName
. Custom vocabulary names are case sensitive.
*
*
* @param deleteVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteVocabularyAsync(DeleteVocabularyRequest deleteVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a custom vocabulary filter. To use this operation, specify the name of the custom vocabulary filter you
* want to delete using VocabularyFilterName
. Custom vocabulary filter names are case sensitive.
*
*
* @param deleteVocabularyFilterRequest
* @return A Java Future containing the result of the DeleteVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsync.DeleteVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVocabularyFilterAsync(DeleteVocabularyFilterRequest deleteVocabularyFilterRequest);
/**
*
* Deletes a custom vocabulary filter. To use this operation, specify the name of the custom vocabulary filter you
* want to delete using VocabularyFilterName
. Custom vocabulary filter names are case sensitive.
*
*
* @param deleteVocabularyFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DeleteVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteVocabularyFilterAsync(DeleteVocabularyFilterRequest deleteVocabularyFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified custom language model.
*
*
* This operation also shows if the base language model that you used to create your custom language model has been
* updated. If Amazon Transcribe has updated the base model, you can create a new custom language model using the
* updated base model.
*
*
* If you tried to create a new custom language model and the request wasn't successful, you can use
* DescribeLanguageModel
to help identify the reason for this failure.
*
*
* @param describeLanguageModelRequest
* @return A Java Future containing the result of the DescribeLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsync.DescribeLanguageModel
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLanguageModelAsync(DescribeLanguageModelRequest describeLanguageModelRequest);
/**
*
* Provides information about the specified custom language model.
*
*
* This operation also shows if the base language model that you used to create your custom language model has been
* updated. If Amazon Transcribe has updated the base model, you can create a new custom language model using the
* updated base model.
*
*
* If you tried to create a new custom language model and the request wasn't successful, you can use
* DescribeLanguageModel
to help identify the reason for this failure.
*
*
* @param describeLanguageModelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeLanguageModel operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.DescribeLanguageModel
* @see AWS API Documentation
*/
java.util.concurrent.Future describeLanguageModelAsync(DescribeLanguageModelRequest describeLanguageModelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified Call Analytics category.
*
*
* To get a list of your Call Analytics categories, use the operation.
*
*
* @param getCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the GetCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsync.GetCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future getCallAnalyticsCategoryAsync(GetCallAnalyticsCategoryRequest getCallAnalyticsCategoryRequest);
/**
*
* Provides information about the specified Call Analytics category.
*
*
* To get a list of your Call Analytics categories, use the operation.
*
*
* @param getCallAnalyticsCategoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future getCallAnalyticsCategoryAsync(GetCallAnalyticsCategoryRequest getCallAnalyticsCategoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified Call Analytics job.
*
*
* To view the job's status, refer to CallAnalyticsJobStatus
. If the status is COMPLETED
,
* the job is finished. You can find your completed transcript at the URI specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled personally identifiable information (PII) redaction, the redacted transcript appears at the
* location specified in RedactedTranscriptFileUri
.
*
*
* If you chose to redact the audio in your media file, you can find your redacted media file at the location
* specified in RedactedMediaFileUri
.
*
*
* To get a list of your Call Analytics jobs, use the operation.
*
*
* @param getCallAnalyticsJobRequest
* @return A Java Future containing the result of the GetCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsync.GetCallAnalyticsJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCallAnalyticsJobAsync(GetCallAnalyticsJobRequest getCallAnalyticsJobRequest);
/**
*
* Provides information about the specified Call Analytics job.
*
*
* To view the job's status, refer to CallAnalyticsJobStatus
. If the status is COMPLETED
,
* the job is finished. You can find your completed transcript at the URI specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled personally identifiable information (PII) redaction, the redacted transcript appears at the
* location specified in RedactedTranscriptFileUri
.
*
*
* If you chose to redact the audio in your media file, you can find your redacted media file at the location
* specified in RedactedMediaFileUri
.
*
*
* To get a list of your Call Analytics jobs, use the operation.
*
*
* @param getCallAnalyticsJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetCallAnalyticsJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getCallAnalyticsJobAsync(GetCallAnalyticsJobRequest getCallAnalyticsJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified Medical Scribe job.
*
*
* To view the status of the specified medical transcription job, check the MedicalScribeJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in MedicalScribeOutput
. If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* To get a list of your Medical Scribe jobs, use the operation.
*
*
* @param getMedicalScribeJobRequest
* @return A Java Future containing the result of the GetMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsync.GetMedicalScribeJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMedicalScribeJobAsync(GetMedicalScribeJobRequest getMedicalScribeJobRequest);
/**
*
* Provides information about the specified Medical Scribe job.
*
*
* To view the status of the specified medical transcription job, check the MedicalScribeJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in MedicalScribeOutput
. If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* To get a list of your Medical Scribe jobs, use the operation.
*
*
* @param getMedicalScribeJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetMedicalScribeJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMedicalScribeJobAsync(GetMedicalScribeJobRequest getMedicalScribeJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified medical transcription job.
*
*
* To view the status of the specified medical transcription job, check the TranscriptionJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in TranscriptFileUri
. If the status is FAILED
, FailureReason
* provides details on why your transcription job failed.
*
*
* To get a list of your medical transcription jobs, use the operation.
*
*
* @param getMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the GetMedicalTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsync.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getMedicalTranscriptionJobAsync(
GetMedicalTranscriptionJobRequest getMedicalTranscriptionJobRequest);
/**
*
* Provides information about the specified medical transcription job.
*
*
* To view the status of the specified medical transcription job, check the TranscriptionJobStatus
* field. If the status is COMPLETED
, the job is finished. You can find the results at the location
* specified in TranscriptFileUri
. If the status is FAILED
, FailureReason
* provides details on why your transcription job failed.
*
*
* To get a list of your medical transcription jobs, use the operation.
*
*
* @param getMedicalTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMedicalTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future getMedicalTranscriptionJobAsync(
GetMedicalTranscriptionJobRequest getMedicalTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified custom medical vocabulary.
*
*
* To view the status of the specified custom medical vocabulary, check the VocabularyState
field. If
* the status is READY
, your custom vocabulary is available to use. If the status is
* FAILED
, FailureReason
provides details on why your vocabulary failed.
*
*
* To get a list of your custom medical vocabularies, use the operation.
*
*
* @param getMedicalVocabularyRequest
* @return A Java Future containing the result of the GetMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.GetMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future getMedicalVocabularyAsync(GetMedicalVocabularyRequest getMedicalVocabularyRequest);
/**
*
* Provides information about the specified custom medical vocabulary.
*
*
* To view the status of the specified custom medical vocabulary, check the VocabularyState
field. If
* the status is READY
, your custom vocabulary is available to use. If the status is
* FAILED
, FailureReason
provides details on why your vocabulary failed.
*
*
* To get a list of your custom medical vocabularies, use the operation.
*
*
* @param getMedicalVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future getMedicalVocabularyAsync(GetMedicalVocabularyRequest getMedicalVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified transcription job.
*
*
* To view the status of the specified transcription job, check the TranscriptionJobStatus
field. If
* the status is COMPLETED
, the job is finished. You can find the results at the location specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in
* RedactedTranscriptFileUri
.
*
*
* To get a list of your transcription jobs, use the operation.
*
*
* @param getTranscriptionJobRequest
* @return A Java Future containing the result of the GetTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsync.GetTranscriptionJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTranscriptionJobAsync(GetTranscriptionJobRequest getTranscriptionJobRequest);
/**
*
* Provides information about the specified transcription job.
*
*
* To view the status of the specified transcription job, check the TranscriptionJobStatus
field. If
* the status is COMPLETED
, the job is finished. You can find the results at the location specified in
* TranscriptFileUri
. If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
*
*
* If you enabled content redaction, the redacted transcript can be found at the location specified in
* RedactedTranscriptFileUri
.
*
*
* To get a list of your transcription jobs, use the operation.
*
*
* @param getTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetTranscriptionJob
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getTranscriptionJobAsync(GetTranscriptionJobRequest getTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified custom vocabulary.
*
*
* To view the status of the specified custom vocabulary, check the VocabularyState
field. If the
* status is READY
, your custom vocabulary is available to use. If the status is FAILED
,
* FailureReason
provides details on why your custom vocabulary failed.
*
*
* To get a list of your custom vocabularies, use the operation.
*
*
* @param getVocabularyRequest
* @return A Java Future containing the result of the GetVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.GetVocabulary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getVocabularyAsync(GetVocabularyRequest getVocabularyRequest);
/**
*
* Provides information about the specified custom vocabulary.
*
*
* To view the status of the specified custom vocabulary, check the VocabularyState
field. If the
* status is READY
, your custom vocabulary is available to use. If the status is FAILED
,
* FailureReason
provides details on why your custom vocabulary failed.
*
*
* To get a list of your custom vocabularies, use the operation.
*
*
* @param getVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetVocabulary
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getVocabularyAsync(GetVocabularyRequest getVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information about the specified custom vocabulary filter.
*
*
* To get a list of your custom vocabulary filters, use the operation.
*
*
* @param getVocabularyFilterRequest
* @return A Java Future containing the result of the GetVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsync.GetVocabularyFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getVocabularyFilterAsync(GetVocabularyFilterRequest getVocabularyFilterRequest);
/**
*
* Provides information about the specified custom vocabulary filter.
*
*
* To get a list of your custom vocabulary filters, use the operation.
*
*
* @param getVocabularyFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.GetVocabularyFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getVocabularyFilterAsync(GetVocabularyFilterRequest getVocabularyFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of Call Analytics categories, including all rules that make up each category.
*
*
* To get detailed information about a specific Call Analytics category, use the operation.
*
*
* @param listCallAnalyticsCategoriesRequest
* @return A Java Future containing the result of the ListCallAnalyticsCategories operation returned by the service.
* @sample AmazonTranscribeAsync.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future listCallAnalyticsCategoriesAsync(
ListCallAnalyticsCategoriesRequest listCallAnalyticsCategoriesRequest);
/**
*
* Provides a list of Call Analytics categories, including all rules that make up each category.
*
*
* To get detailed information about a specific Call Analytics category, use the operation.
*
*
* @param listCallAnalyticsCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCallAnalyticsCategories operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListCallAnalyticsCategories
* @see AWS API Documentation
*/
java.util.concurrent.Future listCallAnalyticsCategoriesAsync(
ListCallAnalyticsCategoriesRequest listCallAnalyticsCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of Call Analytics jobs that match the specified criteria. If no criteria are specified, all Call
* Analytics jobs are returned.
*
*
* To get detailed information about a specific Call Analytics job, use the operation.
*
*
* @param listCallAnalyticsJobsRequest
* @return A Java Future containing the result of the ListCallAnalyticsJobs operation returned by the service.
* @sample AmazonTranscribeAsync.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listCallAnalyticsJobsAsync(ListCallAnalyticsJobsRequest listCallAnalyticsJobsRequest);
/**
*
* Provides a list of Call Analytics jobs that match the specified criteria. If no criteria are specified, all Call
* Analytics jobs are returned.
*
*
* To get detailed information about a specific Call Analytics job, use the operation.
*
*
* @param listCallAnalyticsJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCallAnalyticsJobs operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListCallAnalyticsJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listCallAnalyticsJobsAsync(ListCallAnalyticsJobsRequest listCallAnalyticsJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of custom language models that match the specified criteria. If no criteria are specified, all
* custom language models are returned.
*
*
* To get detailed information about a specific custom language model, use the operation.
*
*
* @param listLanguageModelsRequest
* @return A Java Future containing the result of the ListLanguageModels operation returned by the service.
* @sample AmazonTranscribeAsync.ListLanguageModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLanguageModelsAsync(ListLanguageModelsRequest listLanguageModelsRequest);
/**
*
* Provides a list of custom language models that match the specified criteria. If no criteria are specified, all
* custom language models are returned.
*
*
* To get detailed information about a specific custom language model, use the operation.
*
*
* @param listLanguageModelsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListLanguageModels operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListLanguageModels
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listLanguageModelsAsync(ListLanguageModelsRequest listLanguageModelsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of Medical Scribe jobs that match the specified criteria. If no criteria are specified, all
* Medical Scribe jobs are returned.
*
*
* To get detailed information about a specific Medical Scribe job, use the operation.
*
*
* @param listMedicalScribeJobsRequest
* @return A Java Future containing the result of the ListMedicalScribeJobs operation returned by the service.
* @sample AmazonTranscribeAsync.ListMedicalScribeJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalScribeJobsAsync(ListMedicalScribeJobsRequest listMedicalScribeJobsRequest);
/**
*
* Provides a list of Medical Scribe jobs that match the specified criteria. If no criteria are specified, all
* Medical Scribe jobs are returned.
*
*
* To get detailed information about a specific Medical Scribe job, use the operation.
*
*
* @param listMedicalScribeJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMedicalScribeJobs operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListMedicalScribeJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalScribeJobsAsync(ListMedicalScribeJobsRequest listMedicalScribeJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of medical transcription jobs that match the specified criteria. If no criteria are specified,
* all medical transcription jobs are returned.
*
*
* To get detailed information about a specific medical transcription job, use the operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @return A Java Future containing the result of the ListMedicalTranscriptionJobs operation returned by the
* service.
* @sample AmazonTranscribeAsync.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalTranscriptionJobsAsync(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest);
/**
*
* Provides a list of medical transcription jobs that match the specified criteria. If no criteria are specified,
* all medical transcription jobs are returned.
*
*
* To get detailed information about a specific medical transcription job, use the operation.
*
*
* @param listMedicalTranscriptionJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMedicalTranscriptionJobs operation returned by the
* service.
* @sample AmazonTranscribeAsyncHandler.ListMedicalTranscriptionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalTranscriptionJobsAsync(
ListMedicalTranscriptionJobsRequest listMedicalTranscriptionJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of custom medical vocabularies that match the specified criteria. If no criteria are specified,
* all custom medical vocabularies are returned.
*
*
* To get detailed information about a specific custom medical vocabulary, use the operation.
*
*
* @param listMedicalVocabulariesRequest
* @return A Java Future containing the result of the ListMedicalVocabularies operation returned by the service.
* @sample AmazonTranscribeAsync.ListMedicalVocabularies
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalVocabulariesAsync(ListMedicalVocabulariesRequest listMedicalVocabulariesRequest);
/**
*
* Provides a list of custom medical vocabularies that match the specified criteria. If no criteria are specified,
* all custom medical vocabularies are returned.
*
*
* To get detailed information about a specific custom medical vocabulary, use the operation.
*
*
* @param listMedicalVocabulariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMedicalVocabularies operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListMedicalVocabularies
* @see AWS API Documentation
*/
java.util.concurrent.Future listMedicalVocabulariesAsync(ListMedicalVocabulariesRequest listMedicalVocabulariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags associated with the specified transcription job, vocabulary, model, or resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonTranscribeAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags associated with the specified transcription job, vocabulary, model, or resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
* @param listTranscriptionJobsRequest
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* @sample AmazonTranscribeAsync.ListTranscriptionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listTranscriptionJobsAsync(ListTranscriptionJobsRequest listTranscriptionJobsRequest);
/**
*
* Provides a list of transcription jobs that match the specified criteria. If no criteria are specified, all
* transcription jobs are returned.
*
*
* To get detailed information about a specific transcription job, use the operation.
*
*
* @param listTranscriptionJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTranscriptionJobs operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListTranscriptionJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listTranscriptionJobsAsync(ListTranscriptionJobsRequest listTranscriptionJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
* @param listVocabulariesRequest
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* @sample AmazonTranscribeAsync.ListVocabularies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVocabulariesAsync(ListVocabulariesRequest listVocabulariesRequest);
/**
*
* Provides a list of custom vocabularies that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary, use the operation.
*
*
* @param listVocabulariesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVocabularies operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListVocabularies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listVocabulariesAsync(ListVocabulariesRequest listVocabulariesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of custom vocabulary filters that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary filter, use the operation.
*
*
* @param listVocabularyFiltersRequest
* @return A Java Future containing the result of the ListVocabularyFilters operation returned by the service.
* @sample AmazonTranscribeAsync.ListVocabularyFilters
* @see AWS API Documentation
*/
java.util.concurrent.Future listVocabularyFiltersAsync(ListVocabularyFiltersRequest listVocabularyFiltersRequest);
/**
*
* Provides a list of custom vocabulary filters that match the specified criteria. If no criteria are specified, all
* custom vocabularies are returned.
*
*
* To get detailed information about a specific custom vocabulary filter, use the operation.
*
*
* @param listVocabularyFiltersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListVocabularyFilters operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.ListVocabularyFilters
* @see AWS API Documentation
*/
java.util.concurrent.Future listVocabularyFiltersAsync(ListVocabularyFiltersRequest listVocabularyFiltersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Transcribes the audio from a customer service call and applies any additional Request Parameters you choose to
* include in your request.
*
*
* In addition to many standard transcription features, Call Analytics provides you with call characteristics, call
* summarization, speaker sentiment, and optional redaction of your text transcript and your audio file. You can
* also apply custom categories to flag specified conditions. To learn more about these features and insights, refer
* to Analyzing call center audio
* with Call Analytics.
*
*
* If you want to apply categories to your Call Analytics job, you must create them before submitting your job
* request. Categories cannot be retroactively applied to a job. To create a new category, use the operation. To
* learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* To make a StartCallAnalyticsJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* Note that job queuing is enabled by default for Call Analytics jobs.
*
*
* You must include the following parameters in your StartCallAnalyticsJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* CallAnalyticsJobName
: A custom name that you create for your transcription job that's unique within
* your Amazon Web Services account.
*
*
* -
*
* DataAccessRoleArn
: The Amazon Resource Name (ARN) of an IAM role that has permissions to access the
* Amazon S3 bucket that contains your input files.
*
*
* -
*
* Media
(MediaFileUri
or RedactedMediaFileUri
): The Amazon S3 location of
* your media file.
*
*
*
*
*
* With Call Analytics, you can redact the audio contained in your media file by including
* RedactedMediaFileUri
, instead of MediaFileUri
, to specify the location of your input
* audio. If you choose to redact your audio, you can find your redacted media at the location specified in the
* RedactedMediaFileUri
field of your response.
*
*
*
* @param startCallAnalyticsJobRequest
* @return A Java Future containing the result of the StartCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsync.StartCallAnalyticsJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startCallAnalyticsJobAsync(StartCallAnalyticsJobRequest startCallAnalyticsJobRequest);
/**
*
* Transcribes the audio from a customer service call and applies any additional Request Parameters you choose to
* include in your request.
*
*
* In addition to many standard transcription features, Call Analytics provides you with call characteristics, call
* summarization, speaker sentiment, and optional redaction of your text transcript and your audio file. You can
* also apply custom categories to flag specified conditions. To learn more about these features and insights, refer
* to Analyzing call center audio
* with Call Analytics.
*
*
* If you want to apply categories to your Call Analytics job, you must create them before submitting your job
* request. Categories cannot be retroactively applied to a job. To create a new category, use the operation. To
* learn more about Call Analytics categories, see Creating categories for
* post-call transcriptions and Creating categories for
* real-time transcriptions.
*
*
* To make a StartCallAnalyticsJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* Note that job queuing is enabled by default for Call Analytics jobs.
*
*
* You must include the following parameters in your StartCallAnalyticsJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* CallAnalyticsJobName
: A custom name that you create for your transcription job that's unique within
* your Amazon Web Services account.
*
*
* -
*
* DataAccessRoleArn
: The Amazon Resource Name (ARN) of an IAM role that has permissions to access the
* Amazon S3 bucket that contains your input files.
*
*
* -
*
* Media
(MediaFileUri
or RedactedMediaFileUri
): The Amazon S3 location of
* your media file.
*
*
*
*
*
* With Call Analytics, you can redact the audio contained in your media file by including
* RedactedMediaFileUri
, instead of MediaFileUri
, to specify the location of your input
* audio. If you choose to redact your audio, you can find your redacted media at the location specified in the
* RedactedMediaFileUri
field of your response.
*
*
*
* @param startCallAnalyticsJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartCallAnalyticsJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.StartCallAnalyticsJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startCallAnalyticsJobAsync(StartCallAnalyticsJobRequest startCallAnalyticsJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Transcribes patient-clinician conversations and generates clinical notes.
*
*
* Amazon Web Services HealthScribe automatically provides rich conversation transcripts, identifies speaker roles,
* classifies dialogues, extracts medical terms, and generates preliminary clinical notes. To learn more about these
* features, refer to Amazon Web
* Services HealthScribe.
*
*
* To make a StartMedicalScribeJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* DataAccessRoleArn
: The ARN of an IAM role with the these minimum permissions: read permission on
* input file Amazon S3 bucket specified in Media
, write permission on the Amazon S3 bucket specified
* in OutputBucketName
, and full permissions on the KMS key specified in
* OutputEncryptionKMSKeyId
(if set). The role should also allow transcribe.amazonaws.com
* to assume it.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* MedicalScribeJobName
: A custom name you create for your MedicalScribe job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your output files stored.
*
*
* -
*
* Settings
: A MedicalScribeSettings
obect that must set exactly one of
* ShowSpeakerLabels
or ChannelIdentification
to true. If ShowSpeakerLabels
* is true, MaxSpeakerLabels
must also be set.
*
*
* -
*
* ChannelDefinitions
: A MedicalScribeChannelDefinitions
array should be set if and only
* if the ChannelIdentification
value of Settings
is set to true.
*
*
*
*
* @param startMedicalScribeJobRequest
* @return A Java Future containing the result of the StartMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsync.StartMedicalScribeJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMedicalScribeJobAsync(StartMedicalScribeJobRequest startMedicalScribeJobRequest);
/**
*
* Transcribes patient-clinician conversations and generates clinical notes.
*
*
* Amazon Web Services HealthScribe automatically provides rich conversation transcripts, identifies speaker roles,
* classifies dialogues, extracts medical terms, and generates preliminary clinical notes. To learn more about these
* features, refer to Amazon Web
* Services HealthScribe.
*
*
* To make a StartMedicalScribeJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* DataAccessRoleArn
: The ARN of an IAM role with the these minimum permissions: read permission on
* input file Amazon S3 bucket specified in Media
, write permission on the Amazon S3 bucket specified
* in OutputBucketName
, and full permissions on the KMS key specified in
* OutputEncryptionKMSKeyId
(if set). The role should also allow transcribe.amazonaws.com
* to assume it.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* MedicalScribeJobName
: A custom name you create for your MedicalScribe job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your output files stored.
*
*
* -
*
* Settings
: A MedicalScribeSettings
obect that must set exactly one of
* ShowSpeakerLabels
or ChannelIdentification
to true. If ShowSpeakerLabels
* is true, MaxSpeakerLabels
must also be set.
*
*
* -
*
* ChannelDefinitions
: A MedicalScribeChannelDefinitions
array should be set if and only
* if the ChannelIdentification
value of Settings
is set to true.
*
*
*
*
* @param startMedicalScribeJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMedicalScribeJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.StartMedicalScribeJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMedicalScribeJobAsync(StartMedicalScribeJobRequest startMedicalScribeJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Transcribes the audio from a medical dictation or conversation and applies any additional Request Parameters you
* choose to include in your request.
*
*
* In addition to many standard transcription features, Amazon Transcribe Medical provides you with a robust medical
* vocabulary and, optionally, content identification, which adds flags to personal health information (PHI). To
* learn more about these features, refer to How Amazon Transcribe Medical
* works.
*
*
* To make a StartMedicalTranscriptionJob
request, you must first upload your media file into an Amazon
* S3 bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* MedicalTranscriptionJobName
: A custom name you create for your transcription job that is unique
* within your Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* LanguageCode
: This must be en-US
.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your transcript stored. If you want your
* output stored in a sub-folder of this bucket, you must also include OutputKey
.
*
*
* -
*
* Specialty
: This must be PRIMARYCARE
.
*
*
* -
*
* Type
: Choose whether your audio is a conversation or a dictation.
*
*
*
*
* @param startMedicalTranscriptionJobRequest
* @return A Java Future containing the result of the StartMedicalTranscriptionJob operation returned by the
* service.
* @sample AmazonTranscribeAsync.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMedicalTranscriptionJobAsync(
StartMedicalTranscriptionJobRequest startMedicalTranscriptionJobRequest);
/**
*
* Transcribes the audio from a medical dictation or conversation and applies any additional Request Parameters you
* choose to include in your request.
*
*
* In addition to many standard transcription features, Amazon Transcribe Medical provides you with a robust medical
* vocabulary and, optionally, content identification, which adds flags to personal health information (PHI). To
* learn more about these features, refer to How Amazon Transcribe Medical
* works.
*
*
* To make a StartMedicalTranscriptionJob
request, you must first upload your media file into an Amazon
* S3 bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartMedicalTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* MedicalTranscriptionJobName
: A custom name you create for your transcription job that is unique
* within your Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* LanguageCode
: This must be en-US
.
*
*
* -
*
* OutputBucketName
: The Amazon S3 bucket where you want your transcript stored. If you want your
* output stored in a sub-folder of this bucket, you must also include OutputKey
.
*
*
* -
*
* Specialty
: This must be PRIMARYCARE
.
*
*
* -
*
* Type
: Choose whether your audio is a conversation or a dictation.
*
*
*
*
* @param startMedicalTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMedicalTranscriptionJob operation returned by the
* service.
* @sample AmazonTranscribeAsyncHandler.StartMedicalTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startMedicalTranscriptionJobAsync(
StartMedicalTranscriptionJobRequest startMedicalTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Transcribes the audio from a media file and applies any additional Request Parameters you choose to include in
* your request.
*
*
* To make a StartTranscriptionJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* TranscriptionJobName
: A custom name you create for your transcription job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* One of LanguageCode
, IdentifyLanguage
, or IdentifyMultipleLanguages
: If
* you know the language of your media file, specify it using the LanguageCode
parameter; you can find
* all valid language codes in the Supported languages table.
* If you do not know the languages spoken in your media, use either IdentifyLanguage
or
* IdentifyMultipleLanguages
and let Amazon Transcribe identify the languages for you.
*
*
*
*
* @param startTranscriptionJobRequest
* @return A Java Future containing the result of the StartTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsync.StartTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startTranscriptionJobAsync(StartTranscriptionJobRequest startTranscriptionJobRequest);
/**
*
* Transcribes the audio from a media file and applies any additional Request Parameters you choose to include in
* your request.
*
*
* To make a StartTranscriptionJob
request, you must first upload your media file into an Amazon S3
* bucket; you can then specify the Amazon S3 location of the file using the Media
parameter.
*
*
* You must include the following parameters in your StartTranscriptionJob
request:
*
*
* -
*
* region
: The Amazon Web Services Region where you are making your request. For a list of Amazon Web
* Services Regions supported with Amazon Transcribe, refer to Amazon Transcribe endpoints and quotas.
*
*
* -
*
* TranscriptionJobName
: A custom name you create for your transcription job that is unique within your
* Amazon Web Services account.
*
*
* -
*
* Media
(MediaFileUri
): The Amazon S3 location of your media file.
*
*
* -
*
* One of LanguageCode
, IdentifyLanguage
, or IdentifyMultipleLanguages
: If
* you know the language of your media file, specify it using the LanguageCode
parameter; you can find
* all valid language codes in the Supported languages table.
* If you do not know the languages spoken in your media, use either IdentifyLanguage
or
* IdentifyMultipleLanguages
and let Amazon Transcribe identify the languages for you.
*
*
*
*
* @param startTranscriptionJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartTranscriptionJob operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.StartTranscriptionJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startTranscriptionJobAsync(StartTranscriptionJobRequest startTranscriptionJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the specified resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonTranscribeAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the specified resource.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified Amazon Transcribe resource.
*
*
* If you include UntagResource
in your request, you must also include ResourceArn
and
* TagKeys
.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonTranscribeAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from the specified Amazon Transcribe resource.
*
*
* If you include UntagResource
in your request, you must also include ResourceArn
and
* TagKeys
.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified Call Analytics category with new rules. Note that the
* UpdateCallAnalyticsCategory
operation overwrites all existing rules contained in the specified
* category. You cannot append additional rules onto an existing category.
*
*
* To create a new category, see .
*
*
* @param updateCallAnalyticsCategoryRequest
* @return A Java Future containing the result of the UpdateCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsync.UpdateCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCallAnalyticsCategoryAsync(
UpdateCallAnalyticsCategoryRequest updateCallAnalyticsCategoryRequest);
/**
*
* Updates the specified Call Analytics category with new rules. Note that the
* UpdateCallAnalyticsCategory
operation overwrites all existing rules contained in the specified
* category. You cannot append additional rules onto an existing category.
*
*
* To create a new category, see .
*
*
* @param updateCallAnalyticsCategoryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCallAnalyticsCategory operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.UpdateCallAnalyticsCategory
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCallAnalyticsCategoryAsync(
UpdateCallAnalyticsCategoryRequest updateCallAnalyticsCategoryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing custom medical vocabulary with new values. This operation overwrites all existing information
* with your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateMedicalVocabularyRequest
* @return A Java Future containing the result of the UpdateMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMedicalVocabularyAsync(UpdateMedicalVocabularyRequest updateMedicalVocabularyRequest);
/**
*
* Updates an existing custom medical vocabulary with new values. This operation overwrites all existing information
* with your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateMedicalVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMedicalVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.UpdateMedicalVocabulary
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMedicalVocabularyAsync(UpdateMedicalVocabularyRequest updateMedicalVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing custom vocabulary with new values. This operation overwrites all existing information with
* your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateVocabularyRequest
* @return A Java Future containing the result of the UpdateVocabulary operation returned by the service.
* @sample AmazonTranscribeAsync.UpdateVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVocabularyAsync(UpdateVocabularyRequest updateVocabularyRequest);
/**
*
* Updates an existing custom vocabulary with new values. This operation overwrites all existing information with
* your new values; you cannot append new terms onto an existing custom vocabulary.
*
*
* @param updateVocabularyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVocabulary operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.UpdateVocabulary
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateVocabularyAsync(UpdateVocabularyRequest updateVocabularyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing custom vocabulary filter with a new list of words. The new list you provide overwrites all
* previous entries; you cannot append new terms onto an existing custom vocabulary filter.
*
*
* @param updateVocabularyFilterRequest
* @return A Java Future containing the result of the UpdateVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsync.UpdateVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVocabularyFilterAsync(UpdateVocabularyFilterRequest updateVocabularyFilterRequest);
/**
*
* Updates an existing custom vocabulary filter with a new list of words. The new list you provide overwrites all
* previous entries; you cannot append new terms onto an existing custom vocabulary filter.
*
*
* @param updateVocabularyFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateVocabularyFilter operation returned by the service.
* @sample AmazonTranscribeAsyncHandler.UpdateVocabularyFilter
* @see AWS API Documentation
*/
java.util.concurrent.Future updateVocabularyFilterAsync(UpdateVocabularyFilterRequest updateVocabularyFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}