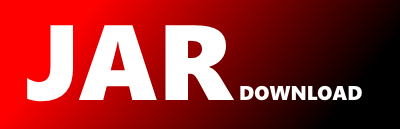
com.amazonaws.services.transcribe.model.GetMedicalVocabularyResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetMedicalVocabularyResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the custom medical vocabulary you requested information about.
*
*/
private String vocabularyName;
/**
*
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the only
* language supported with Amazon Transcribe Medical.
*
*/
private String languageCode;
/**
*
* The processing state of your custom medical vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartMedicalTranscriptionJob
request.
*
*/
private String vocabularyState;
/**
*
* The date and time the specified custom medical vocabulary was last modified.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* If VocabularyState
is FAILED
, FailureReason
contains information about why
* the custom medical vocabulary request failed. See also: Common Errors.
*
*/
private String failureReason;
/**
*
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or download
* the custom vocabulary.
*
*/
private String downloadUri;
/**
*
* The name of the custom medical vocabulary you requested information about.
*
*
* @param vocabularyName
* The name of the custom medical vocabulary you requested information about.
*/
public void setVocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
}
/**
*
* The name of the custom medical vocabulary you requested information about.
*
*
* @return The name of the custom medical vocabulary you requested information about.
*/
public String getVocabularyName() {
return this.vocabularyName;
}
/**
*
* The name of the custom medical vocabulary you requested information about.
*
*
* @param vocabularyName
* The name of the custom medical vocabulary you requested information about.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMedicalVocabularyResult withVocabularyName(String vocabularyName) {
setVocabularyName(vocabularyName);
return this;
}
/**
*
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the only
* language supported with Amazon Transcribe Medical.
*
*
* @param languageCode
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the
* only language supported with Amazon Transcribe Medical.
* @see LanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
*
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the only
* language supported with Amazon Transcribe Medical.
*
*
* @return The language code you selected for your custom medical vocabulary. US English (en-US
) is the
* only language supported with Amazon Transcribe Medical.
* @see LanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
*
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the only
* language supported with Amazon Transcribe Medical.
*
*
* @param languageCode
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the
* only language supported with Amazon Transcribe Medical.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public GetMedicalVocabularyResult withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
*
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the only
* language supported with Amazon Transcribe Medical.
*
*
* @param languageCode
* The language code you selected for your custom medical vocabulary. US English (en-US
) is the
* only language supported with Amazon Transcribe Medical.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public GetMedicalVocabularyResult withLanguageCode(LanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
*
* The processing state of your custom medical vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartMedicalTranscriptionJob
request.
*
*
* @param vocabularyState
* The processing state of your custom medical vocabulary. If the state is READY
, you can use
* the custom vocabulary in a StartMedicalTranscriptionJob
request.
* @see VocabularyState
*/
public void setVocabularyState(String vocabularyState) {
this.vocabularyState = vocabularyState;
}
/**
*
* The processing state of your custom medical vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartMedicalTranscriptionJob
request.
*
*
* @return The processing state of your custom medical vocabulary. If the state is READY
, you can use
* the custom vocabulary in a StartMedicalTranscriptionJob
request.
* @see VocabularyState
*/
public String getVocabularyState() {
return this.vocabularyState;
}
/**
*
* The processing state of your custom medical vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartMedicalTranscriptionJob
request.
*
*
* @param vocabularyState
* The processing state of your custom medical vocabulary. If the state is READY
, you can use
* the custom vocabulary in a StartMedicalTranscriptionJob
request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see VocabularyState
*/
public GetMedicalVocabularyResult withVocabularyState(String vocabularyState) {
setVocabularyState(vocabularyState);
return this;
}
/**
*
* The processing state of your custom medical vocabulary. If the state is READY
, you can use the
* custom vocabulary in a StartMedicalTranscriptionJob
request.
*
*
* @param vocabularyState
* The processing state of your custom medical vocabulary. If the state is READY
, you can use
* the custom vocabulary in a StartMedicalTranscriptionJob
request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see VocabularyState
*/
public GetMedicalVocabularyResult withVocabularyState(VocabularyState vocabularyState) {
this.vocabularyState = vocabularyState.toString();
return this;
}
/**
*
* The date and time the specified custom medical vocabulary was last modified.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @param lastModifiedTime
* The date and time the specified custom medical vocabulary was last modified.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The date and time the specified custom medical vocabulary was last modified.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified custom medical vocabulary was last modified.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The date and time the specified custom medical vocabulary was last modified.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
*
*
* @param lastModifiedTime
* The date and time the specified custom medical vocabulary was last modified.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMedicalVocabularyResult withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* If VocabularyState
is FAILED
, FailureReason
contains information about why
* the custom medical vocabulary request failed. See also: Common Errors.
*
*
* @param failureReason
* If VocabularyState
is FAILED
, FailureReason
contains information
* about why the custom medical vocabulary request failed. See also: Common Errors.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* If VocabularyState
is FAILED
, FailureReason
contains information about why
* the custom medical vocabulary request failed. See also: Common Errors.
*
*
* @return If VocabularyState
is FAILED
, FailureReason
contains information
* about why the custom medical vocabulary request failed. See also: Common Errors.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* If VocabularyState
is FAILED
, FailureReason
contains information about why
* the custom medical vocabulary request failed. See also: Common Errors.
*
*
* @param failureReason
* If VocabularyState
is FAILED
, FailureReason
contains information
* about why the custom medical vocabulary request failed. See also: Common Errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMedicalVocabularyResult withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or download
* the custom vocabulary.
*
*
* @param downloadUri
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or
* download the custom vocabulary.
*/
public void setDownloadUri(String downloadUri) {
this.downloadUri = downloadUri;
}
/**
*
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or download
* the custom vocabulary.
*
*
* @return The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or
* download the custom vocabulary.
*/
public String getDownloadUri() {
return this.downloadUri;
}
/**
*
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or download
* the custom vocabulary.
*
*
* @param downloadUri
* The Amazon S3 location where the specified custom medical vocabulary is stored; use this URI to view or
* download the custom vocabulary.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetMedicalVocabularyResult withDownloadUri(String downloadUri) {
setDownloadUri(downloadUri);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVocabularyName() != null)
sb.append("VocabularyName: ").append(getVocabularyName()).append(",");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getVocabularyState() != null)
sb.append("VocabularyState: ").append(getVocabularyState()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getDownloadUri() != null)
sb.append("DownloadUri: ").append(getDownloadUri());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetMedicalVocabularyResult == false)
return false;
GetMedicalVocabularyResult other = (GetMedicalVocabularyResult) obj;
if (other.getVocabularyName() == null ^ this.getVocabularyName() == null)
return false;
if (other.getVocabularyName() != null && other.getVocabularyName().equals(this.getVocabularyName()) == false)
return false;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getVocabularyState() == null ^ this.getVocabularyState() == null)
return false;
if (other.getVocabularyState() != null && other.getVocabularyState().equals(this.getVocabularyState()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getDownloadUri() == null ^ this.getDownloadUri() == null)
return false;
if (other.getDownloadUri() != null && other.getDownloadUri().equals(this.getDownloadUri()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVocabularyName() == null) ? 0 : getVocabularyName().hashCode());
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getVocabularyState() == null) ? 0 : getVocabularyState().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getDownloadUri() == null) ? 0 : getDownloadUri().hashCode());
return hashCode;
}
@Override
public GetMedicalVocabularyResult clone() {
try {
return (GetMedicalVocabularyResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}