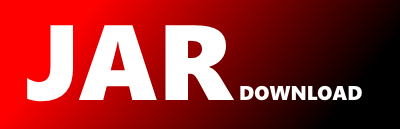
com.amazonaws.services.transcribe.model.StartMedicalScribeJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartMedicalScribeJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique name, chosen by you, for your Medical Scribe job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*/
private String medicalScribeJobName;
private Media media;
/**
*
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*
*/
private String outputBucketName;
/**
*
* The KMS key you want to use to encrypt your Medical Scribe output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified KMS key.
*
*/
private String outputEncryptionKMSKeyId;
/**
*
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added layer
* of security for your data. For more information, see KMS encryption
* context and Asymmetric
* keys in KMS.
*
*/
private java.util.Map kMSEncryptionContext;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*/
private String dataAccessRoleArn;
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*/
private MedicalScribeSettings settings;
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*/
private java.util.List channelDefinitions;
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*/
private java.util.List tags;
/**
*
* A unique name, chosen by you, for your Medical Scribe job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @param medicalScribeJobName
* A unique name, chosen by you, for your Medical Scribe job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
*/
public void setMedicalScribeJobName(String medicalScribeJobName) {
this.medicalScribeJobName = medicalScribeJobName;
}
/**
*
* A unique name, chosen by you, for your Medical Scribe job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @return A unique name, chosen by you, for your Medical Scribe job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
*/
public String getMedicalScribeJobName() {
return this.medicalScribeJobName;
}
/**
*
* A unique name, chosen by you, for your Medical Scribe job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @param medicalScribeJobName
* A unique name, chosen by you, for your Medical Scribe job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withMedicalScribeJobName(String medicalScribeJobName) {
setMedicalScribeJobName(medicalScribeJobName);
return this;
}
/**
* @param media
*/
public void setMedia(Media media) {
this.media = media;
}
/**
* @return
*/
public Media getMedia() {
return this.media;
}
/**
* @param media
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withMedia(Media media) {
setMedia(media);
return this;
}
/**
*
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*
*
* @param outputBucketName
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*/
public void setOutputBucketName(String outputBucketName) {
this.outputBucketName = outputBucketName;
}
/**
*
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*
*
* @return The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*/
public String getOutputBucketName() {
return this.outputBucketName;
}
/**
*
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
*
*
* @param outputBucketName
* The name of the Amazon S3 bucket where you want your Medical Scribe output stored. Do not include the
* S3://
prefix of the specified bucket.
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified location. You can change Amazon S3 permissions using the Amazon Web Services Management Console. See also Permissions Required for IAM User Roles.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withOutputBucketName(String outputBucketName) {
setOutputBucketName(outputBucketName);
return this;
}
/**
*
* The KMS key you want to use to encrypt your Medical Scribe output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified KMS key.
*
*
* @param outputEncryptionKMSKeyId
* The KMS key you want to use to encrypt your Medical Scribe output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified KMS key.
*/
public void setOutputEncryptionKMSKeyId(String outputEncryptionKMSKeyId) {
this.outputEncryptionKMSKeyId = outputEncryptionKMSKeyId;
}
/**
*
* The KMS key you want to use to encrypt your Medical Scribe output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified KMS key.
*
*
* @return The KMS key you want to use to encrypt your Medical Scribe output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key
* (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified KMS key.
*/
public String getOutputEncryptionKMSKeyId() {
return this.outputEncryptionKMSKeyId;
}
/**
*
* The KMS key you want to use to encrypt your Medical Scribe output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission to use
* the specified KMS key.
*
*
* @param outputEncryptionKMSKeyId
* The KMS key you want to use to encrypt your Medical Scribe output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* Note that the role specified in the DataAccessRoleArn
request parameter must have permission
* to use the specified KMS key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withOutputEncryptionKMSKeyId(String outputEncryptionKMSKeyId) {
setOutputEncryptionKMSKeyId(outputEncryptionKMSKeyId);
return this;
}
/**
*
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added layer
* of security for your data. For more information, see KMS encryption
* context and Asymmetric
* keys in KMS.
*
*
* @return A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added
* layer of security for your data. For more information, see KMS encryption
* context and Asymmetric keys in
* KMS.
*/
public java.util.Map getKMSEncryptionContext() {
return kMSEncryptionContext;
}
/**
*
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added layer
* of security for your data. For more information, see KMS encryption
* context and Asymmetric
* keys in KMS.
*
*
* @param kMSEncryptionContext
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added
* layer of security for your data. For more information, see KMS encryption
* context and Asymmetric keys in
* KMS.
*/
public void setKMSEncryptionContext(java.util.Map kMSEncryptionContext) {
this.kMSEncryptionContext = kMSEncryptionContext;
}
/**
*
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added layer
* of security for your data. For more information, see KMS encryption
* context and Asymmetric
* keys in KMS.
*
*
* @param kMSEncryptionContext
* A map of plain text, non-secret key:value pairs, known as encryption context pairs, that provide an added
* layer of security for your data. For more information, see KMS encryption
* context and Asymmetric keys in
* KMS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withKMSEncryptionContext(java.util.Map kMSEncryptionContext) {
setKMSEncryptionContext(kMSEncryptionContext);
return this;
}
/**
* Add a single KMSEncryptionContext entry
*
* @see StartMedicalScribeJobRequest#withKMSEncryptionContext
* @returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest addKMSEncryptionContextEntry(String key, String value) {
if (null == this.kMSEncryptionContext) {
this.kMSEncryptionContext = new java.util.HashMap();
}
if (this.kMSEncryptionContext.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.kMSEncryptionContext.put(key, value);
return this;
}
/**
* Removes all the entries added into KMSEncryptionContext.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest clearKMSEncryptionContextEntries() {
this.kMSEncryptionContext = null;
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public String getDataAccessRoleArn() {
return this.dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withDataAccessRoleArn(String dataAccessRoleArn) {
setDataAccessRoleArn(dataAccessRoleArn);
return this;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @param settings
* Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
*/
public void setSettings(MedicalScribeSettings settings) {
this.settings = settings;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @return Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
*/
public MedicalScribeSettings getSettings() {
return this.settings;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @param settings
* Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withSettings(MedicalScribeSettings settings) {
setSettings(settings);
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @return Makes it possible to specify which speaker is on which channel. For example, if the clinician is the
* first participant to speak, you would set ChannelId
of the first
* ChannelDefinition
in the list to 0
(to indicate the first channel) and
* ParticipantRole
to CLINICIAN
(to indicate that it's the clinician speaking).
* Then you would set the ChannelId
of the second ChannelDefinition
in the list to
* 1
(to indicate the second channel) and ParticipantRole
to PATIENT
* (to indicate that it's the patient speaking).
*/
public java.util.List getChannelDefinitions() {
return channelDefinitions;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
*/
public void setChannelDefinitions(java.util.Collection channelDefinitions) {
if (channelDefinitions == null) {
this.channelDefinitions = null;
return;
}
this.channelDefinitions = new java.util.ArrayList(channelDefinitions);
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChannelDefinitions(java.util.Collection)} or {@link #withChannelDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withChannelDefinitions(MedicalScribeChannelDefinition... channelDefinitions) {
if (this.channelDefinitions == null) {
setChannelDefinitions(new java.util.ArrayList(channelDefinitions.length));
}
for (MedicalScribeChannelDefinition ele : channelDefinitions) {
this.channelDefinitions.add(ele);
}
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withChannelDefinitions(java.util.Collection channelDefinitions) {
setChannelDefinitions(channelDefinitions);
return this;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @return Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartMedicalScribeJobRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMedicalScribeJobName() != null)
sb.append("MedicalScribeJobName: ").append(getMedicalScribeJobName()).append(",");
if (getMedia() != null)
sb.append("Media: ").append(getMedia()).append(",");
if (getOutputBucketName() != null)
sb.append("OutputBucketName: ").append(getOutputBucketName()).append(",");
if (getOutputEncryptionKMSKeyId() != null)
sb.append("OutputEncryptionKMSKeyId: ").append(getOutputEncryptionKMSKeyId()).append(",");
if (getKMSEncryptionContext() != null)
sb.append("KMSEncryptionContext: ").append(getKMSEncryptionContext()).append(",");
if (getDataAccessRoleArn() != null)
sb.append("DataAccessRoleArn: ").append(getDataAccessRoleArn()).append(",");
if (getSettings() != null)
sb.append("Settings: ").append(getSettings()).append(",");
if (getChannelDefinitions() != null)
sb.append("ChannelDefinitions: ").append(getChannelDefinitions()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartMedicalScribeJobRequest == false)
return false;
StartMedicalScribeJobRequest other = (StartMedicalScribeJobRequest) obj;
if (other.getMedicalScribeJobName() == null ^ this.getMedicalScribeJobName() == null)
return false;
if (other.getMedicalScribeJobName() != null && other.getMedicalScribeJobName().equals(this.getMedicalScribeJobName()) == false)
return false;
if (other.getMedia() == null ^ this.getMedia() == null)
return false;
if (other.getMedia() != null && other.getMedia().equals(this.getMedia()) == false)
return false;
if (other.getOutputBucketName() == null ^ this.getOutputBucketName() == null)
return false;
if (other.getOutputBucketName() != null && other.getOutputBucketName().equals(this.getOutputBucketName()) == false)
return false;
if (other.getOutputEncryptionKMSKeyId() == null ^ this.getOutputEncryptionKMSKeyId() == null)
return false;
if (other.getOutputEncryptionKMSKeyId() != null && other.getOutputEncryptionKMSKeyId().equals(this.getOutputEncryptionKMSKeyId()) == false)
return false;
if (other.getKMSEncryptionContext() == null ^ this.getKMSEncryptionContext() == null)
return false;
if (other.getKMSEncryptionContext() != null && other.getKMSEncryptionContext().equals(this.getKMSEncryptionContext()) == false)
return false;
if (other.getDataAccessRoleArn() == null ^ this.getDataAccessRoleArn() == null)
return false;
if (other.getDataAccessRoleArn() != null && other.getDataAccessRoleArn().equals(this.getDataAccessRoleArn()) == false)
return false;
if (other.getSettings() == null ^ this.getSettings() == null)
return false;
if (other.getSettings() != null && other.getSettings().equals(this.getSettings()) == false)
return false;
if (other.getChannelDefinitions() == null ^ this.getChannelDefinitions() == null)
return false;
if (other.getChannelDefinitions() != null && other.getChannelDefinitions().equals(this.getChannelDefinitions()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMedicalScribeJobName() == null) ? 0 : getMedicalScribeJobName().hashCode());
hashCode = prime * hashCode + ((getMedia() == null) ? 0 : getMedia().hashCode());
hashCode = prime * hashCode + ((getOutputBucketName() == null) ? 0 : getOutputBucketName().hashCode());
hashCode = prime * hashCode + ((getOutputEncryptionKMSKeyId() == null) ? 0 : getOutputEncryptionKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getKMSEncryptionContext() == null) ? 0 : getKMSEncryptionContext().hashCode());
hashCode = prime * hashCode + ((getDataAccessRoleArn() == null) ? 0 : getDataAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getSettings() == null) ? 0 : getSettings().hashCode());
hashCode = prime * hashCode + ((getChannelDefinitions() == null) ? 0 : getChannelDefinitions().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public StartMedicalScribeJobRequest clone() {
return (StartMedicalScribeJobRequest) super.clone();
}
}