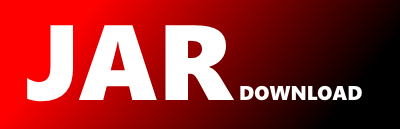
com.amazonaws.services.transcribe.model.SubtitlesOutput Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information about your subtitle file, including format, start index, and Amazon S3 location.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SubtitlesOutput implements Serializable, Cloneable, StructuredPojo {
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*/
private java.util.List formats;
/**
*
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file. Your
* subtitle file is stored in the same location as your transcript. If you specified both WebVTT and SubRip subtitle
* formats, two URIs are provided.
*
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that bucket.
* If you also included OutputKey
in your request, your output is located in the path you specified in
* your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file is
* stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary URI you can
* use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a GetTranscriptionJob
or
* ListTranscriptionJob
request.
*
*
*/
private java.util.List subtitleFileUris;
/**
*
* Provides the start index value for your subtitle files. If you did not specify a value in your request, the
* default value of 0
is used.
*
*/
private Integer outputStartIndex;
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*
* @return Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and
* SubRip (srt
) formats, both formats are shown.
* @see SubtitleFormat
*/
public java.util.List getFormats() {
return formats;
}
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*
* @param formats
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and
* SubRip (srt
) formats, both formats are shown.
* @see SubtitleFormat
*/
public void setFormats(java.util.Collection formats) {
if (formats == null) {
this.formats = null;
return;
}
this.formats = new java.util.ArrayList(formats);
}
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFormats(java.util.Collection)} or {@link #withFormats(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param formats
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and
* SubRip (srt
) formats, both formats are shown.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SubtitleFormat
*/
public SubtitlesOutput withFormats(String... formats) {
if (this.formats == null) {
setFormats(new java.util.ArrayList(formats.length));
}
for (String ele : formats) {
this.formats.add(ele);
}
return this;
}
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*
* @param formats
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and
* SubRip (srt
) formats, both formats are shown.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SubtitleFormat
*/
public SubtitlesOutput withFormats(java.util.Collection formats) {
setFormats(formats);
return this;
}
/**
*
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and SubRip (
* srt
) formats, both formats are shown.
*
*
* @param formats
* Provides the format of your subtitle files. If your request included both WebVTT (vtt
) and
* SubRip (srt
) formats, both formats are shown.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SubtitleFormat
*/
public SubtitlesOutput withFormats(SubtitleFormat... formats) {
java.util.ArrayList formatsCopy = new java.util.ArrayList(formats.length);
for (SubtitleFormat value : formats) {
formatsCopy.add(value.toString());
}
if (getFormats() == null) {
setFormats(formatsCopy);
} else {
getFormats().addAll(formatsCopy);
}
return this;
}
/**
*
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file. Your
* subtitle file is stored in the same location as your transcript. If you specified both WebVTT and SubRip subtitle
* formats, two URIs are provided.
*
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that bucket.
* If you also included OutputKey
in your request, your output is located in the path you specified in
* your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file is
* stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary URI you can
* use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a GetTranscriptionJob
or
* ListTranscriptionJob
request.
*
*
*
* @return The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file.
* Your subtitle file is stored in the same location as your transcript. If you specified both WebVTT and
* SubRip subtitle formats, two URIs are provided.
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that
* bucket. If you also included OutputKey
in your request, your output is located in the path
* you specified in your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file
* is stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary
* URI you can use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a
* GetTranscriptionJob
or ListTranscriptionJob
request.
*
*/
public java.util.List getSubtitleFileUris() {
return subtitleFileUris;
}
/**
*
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file. Your
* subtitle file is stored in the same location as your transcript. If you specified both WebVTT and SubRip subtitle
* formats, two URIs are provided.
*
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that bucket.
* If you also included OutputKey
in your request, your output is located in the path you specified in
* your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file is
* stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary URI you can
* use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a GetTranscriptionJob
or
* ListTranscriptionJob
request.
*
*
*
* @param subtitleFileUris
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file.
* Your subtitle file is stored in the same location as your transcript. If you specified both WebVTT and
* SubRip subtitle formats, two URIs are provided.
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that
* bucket. If you also included OutputKey
in your request, your output is located in the path
* you specified in your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file
* is stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary
* URI you can use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a
* GetTranscriptionJob
or ListTranscriptionJob
request.
*
*/
public void setSubtitleFileUris(java.util.Collection subtitleFileUris) {
if (subtitleFileUris == null) {
this.subtitleFileUris = null;
return;
}
this.subtitleFileUris = new java.util.ArrayList(subtitleFileUris);
}
/**
*
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file. Your
* subtitle file is stored in the same location as your transcript. If you specified both WebVTT and SubRip subtitle
* formats, two URIs are provided.
*
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that bucket.
* If you also included OutputKey
in your request, your output is located in the path you specified in
* your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file is
* stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary URI you can
* use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a GetTranscriptionJob
or
* ListTranscriptionJob
request.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubtitleFileUris(java.util.Collection)} or {@link #withSubtitleFileUris(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param subtitleFileUris
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file.
* Your subtitle file is stored in the same location as your transcript. If you specified both WebVTT and
* SubRip subtitle formats, two URIs are provided.
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that
* bucket. If you also included OutputKey
in your request, your output is located in the path
* you specified in your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file
* is stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary
* URI you can use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a
* GetTranscriptionJob
or ListTranscriptionJob
request.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtitlesOutput withSubtitleFileUris(String... subtitleFileUris) {
if (this.subtitleFileUris == null) {
setSubtitleFileUris(new java.util.ArrayList(subtitleFileUris.length));
}
for (String ele : subtitleFileUris) {
this.subtitleFileUris.add(ele);
}
return this;
}
/**
*
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file. Your
* subtitle file is stored in the same location as your transcript. If you specified both WebVTT and SubRip subtitle
* formats, two URIs are provided.
*
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that bucket.
* If you also included OutputKey
in your request, your output is located in the path you specified in
* your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file is
* stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary URI you can
* use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a GetTranscriptionJob
or
* ListTranscriptionJob
request.
*
*
*
* @param subtitleFileUris
* The Amazon S3 location of your transcript. You can use this URI to access or download your subtitle file.
* Your subtitle file is stored in the same location as your transcript. If you specified both WebVTT and
* SubRip subtitle formats, two URIs are provided.
*
* If you included OutputBucketName
in your transcription job request, this is the URI of that
* bucket. If you also included OutputKey
in your request, your output is located in the path
* you specified in your request.
*
*
* If you didn't include OutputBucketName
in your transcription job request, your subtitle file
* is stored in a service-managed bucket, and TranscriptFileUri
provides you with a temporary
* URI you can use for secure access to your subtitle file.
*
*
*
* Temporary URIs for service-managed Amazon S3 buckets are only valid for 15 minutes. If you get an
* AccesDenied
error, you can get a new temporary URI by running a
* GetTranscriptionJob
or ListTranscriptionJob
request.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtitlesOutput withSubtitleFileUris(java.util.Collection subtitleFileUris) {
setSubtitleFileUris(subtitleFileUris);
return this;
}
/**
*
* Provides the start index value for your subtitle files. If you did not specify a value in your request, the
* default value of 0
is used.
*
*
* @param outputStartIndex
* Provides the start index value for your subtitle files. If you did not specify a value in your request,
* the default value of 0
is used.
*/
public void setOutputStartIndex(Integer outputStartIndex) {
this.outputStartIndex = outputStartIndex;
}
/**
*
* Provides the start index value for your subtitle files. If you did not specify a value in your request, the
* default value of 0
is used.
*
*
* @return Provides the start index value for your subtitle files. If you did not specify a value in your request,
* the default value of 0
is used.
*/
public Integer getOutputStartIndex() {
return this.outputStartIndex;
}
/**
*
* Provides the start index value for your subtitle files. If you did not specify a value in your request, the
* default value of 0
is used.
*
*
* @param outputStartIndex
* Provides the start index value for your subtitle files. If you did not specify a value in your request,
* the default value of 0
is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SubtitlesOutput withOutputStartIndex(Integer outputStartIndex) {
setOutputStartIndex(outputStartIndex);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFormats() != null)
sb.append("Formats: ").append(getFormats()).append(",");
if (getSubtitleFileUris() != null)
sb.append("SubtitleFileUris: ").append(getSubtitleFileUris()).append(",");
if (getOutputStartIndex() != null)
sb.append("OutputStartIndex: ").append(getOutputStartIndex());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SubtitlesOutput == false)
return false;
SubtitlesOutput other = (SubtitlesOutput) obj;
if (other.getFormats() == null ^ this.getFormats() == null)
return false;
if (other.getFormats() != null && other.getFormats().equals(this.getFormats()) == false)
return false;
if (other.getSubtitleFileUris() == null ^ this.getSubtitleFileUris() == null)
return false;
if (other.getSubtitleFileUris() != null && other.getSubtitleFileUris().equals(this.getSubtitleFileUris()) == false)
return false;
if (other.getOutputStartIndex() == null ^ this.getOutputStartIndex() == null)
return false;
if (other.getOutputStartIndex() != null && other.getOutputStartIndex().equals(this.getOutputStartIndex()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFormats() == null) ? 0 : getFormats().hashCode());
hashCode = prime * hashCode + ((getSubtitleFileUris() == null) ? 0 : getSubtitleFileUris().hashCode());
hashCode = prime * hashCode + ((getOutputStartIndex() == null) ? 0 : getOutputStartIndex().hashCode());
return hashCode;
}
@Override
public SubtitlesOutput clone() {
try {
return (SubtitlesOutput) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.transcribe.model.transform.SubtitlesOutputMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}