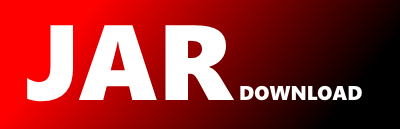
com.amazonaws.services.transcribe.model.RelativeTimeRange Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A time range, in percentage, between two points in your media file.
*
*
* You can use StartPercentage
and EndPercentage
to search a custom segment. For example,
* setting StartPercentage
to 10 and EndPercentage
to 50 only searches for your specified
* criteria in the audio contained between the 10 percent mark and the 50 percent mark of your media file.
*
*
* You can use also First
to search from the start of the media file until the time that you specify. Or
* use Last
to search from the time that you specify until the end of the media file. For example, setting
* First
to 10 only searches for your specified criteria in the audio contained in the first 10 percent of
* the media file.
*
*
* If you prefer to use milliseconds instead of percentage, see .
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RelativeTimeRange implements Serializable, Cloneable, StructuredPojo {
/**
*
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file.
* If you include StartPercentage
in your request, you must also include EndPercentage
.
*
*/
private Integer startPercentage;
/**
*
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If
* you include EndPercentage
in your request, you must also include StartPercentage
.
*
*/
private Integer endPercentage;
/**
*
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*/
private Integer first;
/**
*
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*/
private Integer last;
/**
*
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file.
* If you include StartPercentage
in your request, you must also include EndPercentage
.
*
*
* @param startPercentage
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media
* file. If you include StartPercentage
in your request, you must also include
* EndPercentage
.
*/
public void setStartPercentage(Integer startPercentage) {
this.startPercentage = startPercentage;
}
/**
*
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file.
* If you include StartPercentage
in your request, you must also include EndPercentage
.
*
*
* @return The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media
* file. If you include StartPercentage
in your request, you must also include
* EndPercentage
.
*/
public Integer getStartPercentage() {
return this.startPercentage;
}
/**
*
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media file.
* If you include StartPercentage
in your request, you must also include EndPercentage
.
*
*
* @param startPercentage
* The time, in percentage, when Amazon Transcribe starts searching for the specified criteria in your media
* file. If you include StartPercentage
in your request, you must also include
* EndPercentage
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RelativeTimeRange withStartPercentage(Integer startPercentage) {
setStartPercentage(startPercentage);
return this;
}
/**
*
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If
* you include EndPercentage
in your request, you must also include StartPercentage
.
*
*
* @param endPercentage
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media
* file. If you include EndPercentage
in your request, you must also include
* StartPercentage
.
*/
public void setEndPercentage(Integer endPercentage) {
this.endPercentage = endPercentage;
}
/**
*
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If
* you include EndPercentage
in your request, you must also include StartPercentage
.
*
*
* @return The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media
* file. If you include EndPercentage
in your request, you must also include
* StartPercentage
.
*/
public Integer getEndPercentage() {
return this.endPercentage;
}
/**
*
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media file. If
* you include EndPercentage
in your request, you must also include StartPercentage
.
*
*
* @param endPercentage
* The time, in percentage, when Amazon Transcribe stops searching for the specified criteria in your media
* file. If you include EndPercentage
in your request, you must also include
* StartPercentage
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RelativeTimeRange withEndPercentage(Integer endPercentage) {
setEndPercentage(endPercentage);
return this;
}
/**
*
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @param first
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe
* searches for your specified criteria in this time segment.
*/
public void setFirst(Integer first) {
this.first = first;
}
/**
*
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @return The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe
* searches for your specified criteria in this time segment.
*/
public Integer getFirst() {
return this.first;
}
/**
*
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @param first
* The time, in percentage, from the start of your media file until the specified value. Amazon Transcribe
* searches for your specified criteria in this time segment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RelativeTimeRange withFirst(Integer first) {
setFirst(first);
return this;
}
/**
*
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @param last
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe
* searches for your specified criteria in this time segment.
*/
public void setLast(Integer last) {
this.last = last;
}
/**
*
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @return The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe
* searches for your specified criteria in this time segment.
*/
public Integer getLast() {
return this.last;
}
/**
*
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe searches
* for your specified criteria in this time segment.
*
*
* @param last
* The time, in percentage, from the specified value until the end of your media file. Amazon Transcribe
* searches for your specified criteria in this time segment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RelativeTimeRange withLast(Integer last) {
setLast(last);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStartPercentage() != null)
sb.append("StartPercentage: ").append(getStartPercentage()).append(",");
if (getEndPercentage() != null)
sb.append("EndPercentage: ").append(getEndPercentage()).append(",");
if (getFirst() != null)
sb.append("First: ").append(getFirst()).append(",");
if (getLast() != null)
sb.append("Last: ").append(getLast());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RelativeTimeRange == false)
return false;
RelativeTimeRange other = (RelativeTimeRange) obj;
if (other.getStartPercentage() == null ^ this.getStartPercentage() == null)
return false;
if (other.getStartPercentage() != null && other.getStartPercentage().equals(this.getStartPercentage()) == false)
return false;
if (other.getEndPercentage() == null ^ this.getEndPercentage() == null)
return false;
if (other.getEndPercentage() != null && other.getEndPercentage().equals(this.getEndPercentage()) == false)
return false;
if (other.getFirst() == null ^ this.getFirst() == null)
return false;
if (other.getFirst() != null && other.getFirst().equals(this.getFirst()) == false)
return false;
if (other.getLast() == null ^ this.getLast() == null)
return false;
if (other.getLast() != null && other.getLast().equals(this.getLast()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStartPercentage() == null) ? 0 : getStartPercentage().hashCode());
hashCode = prime * hashCode + ((getEndPercentage() == null) ? 0 : getEndPercentage().hashCode());
hashCode = prime * hashCode + ((getFirst() == null) ? 0 : getFirst().hashCode());
hashCode = prime * hashCode + ((getLast() == null) ? 0 : getLast().hashCode());
return hashCode;
}
@Override
public RelativeTimeRange clone() {
try {
return (RelativeTimeRange) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.transcribe.model.transform.RelativeTimeRangeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}