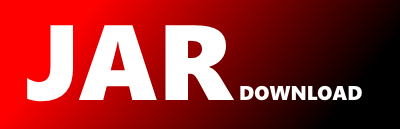
com.amazonaws.services.transcribe.model.StartCallAnalyticsJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartCallAnalyticsJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique name, chosen by you, for your Call Analytics job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*/
private String callAnalyticsJobName;
/**
*
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*
*/
private Media media;
/**
*
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of the
* following formats to specify the output location:
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches the name
* you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
parameter. If
* you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon S3
* bucket and you are provided with a URI to access your transcript.
*
*/
private String outputLocation;
/**
*
* The KMS key you want to use to encrypt your Call Analytics output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*
*/
private String outputEncryptionKMSKeyId;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files. If the role that you specify doesn’t have the appropriate permissions to access the specified
* Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*/
private String dataAccessRoleArn;
/**
*
* Specify additional optional settings in your request, including content redaction; allows you to apply custom
* language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*
*/
private CallAnalyticsJobSettings settings;
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*/
private java.util.List channelDefinitions;
/**
*
* A unique name, chosen by you, for your Call Analytics job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @param callAnalyticsJobName
* A unique name, chosen by you, for your Call Analytics job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
*/
public void setCallAnalyticsJobName(String callAnalyticsJobName) {
this.callAnalyticsJobName = callAnalyticsJobName;
}
/**
*
* A unique name, chosen by you, for your Call Analytics job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @return A unique name, chosen by you, for your Call Analytics job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
*/
public String getCallAnalyticsJobName() {
return this.callAnalyticsJobName;
}
/**
*
* A unique name, chosen by you, for your Call Analytics job.
*
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services account. If
* you try to create a new job with the same name as an existing job, you get a ConflictException
* error.
*
*
* @param callAnalyticsJobName
* A unique name, chosen by you, for your Call Analytics job.
*
* This name is case sensitive, cannot contain spaces, and must be unique within an Amazon Web Services
* account. If you try to create a new job with the same name as an existing job, you get a
* ConflictException
error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withCallAnalyticsJobName(String callAnalyticsJobName) {
setCallAnalyticsJobName(callAnalyticsJobName);
return this;
}
/**
*
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*
*
* @param media
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*/
public void setMedia(Media media) {
this.media = media;
}
/**
*
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*
*
* @return Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*/
public Media getMedia() {
return this.media;
}
/**
*
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
*
*
* @param media
* Describes the Amazon S3 location of the media file you want to use in your Call Analytics request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withMedia(Media media) {
setMedia(media);
return this;
}
/**
*
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of the
* following formats to specify the output location:
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches the name
* you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
parameter. If
* you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon S3
* bucket and you are provided with a URI to access your transcript.
*
*
* @param outputLocation
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of
* the following formats to specify the output location:
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches
* the name you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
* parameter. If you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for
* server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon
* S3 bucket and you are provided with a URI to access your transcript.
*/
public void setOutputLocation(String outputLocation) {
this.outputLocation = outputLocation;
}
/**
*
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of the
* following formats to specify the output location:
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches the name
* you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
parameter. If
* you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon S3
* bucket and you are provided with a URI to access your transcript.
*
*
* @return The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of
* the following formats to specify the output location:
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches
* the name you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
* parameter. If you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for
* server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon
* S3 bucket and you are provided with a URI to access your transcript.
*/
public String getOutputLocation() {
return this.outputLocation;
}
/**
*
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of the
* following formats to specify the output location:
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches the name
* you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
parameter. If
* you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon S3
* bucket and you are provided with a URI to access your transcript.
*
*
* @param outputLocation
* The Amazon S3 location where you want your Call Analytics transcription output stored. You can use any of
* the following formats to specify the output location:
*
* -
*
* s3://DOC-EXAMPLE-BUCKET
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/
*
*
* -
*
* s3://DOC-EXAMPLE-BUCKET/my-output-folder/my-call-analytics-job.json
*
*
*
*
* Unless you specify a file name (option 3), the name of your output file has a default value that matches
* the name you specified for your transcription job using the CallAnalyticsJobName
parameter.
*
*
* You can specify a KMS key to encrypt your output using the OutputEncryptionKMSKeyId
* parameter. If you do not specify a KMS key, Amazon Transcribe uses the default Amazon S3 key for
* server-side encryption.
*
*
* If you do not specify OutputLocation
, your transcript is placed in a service-managed Amazon
* S3 bucket and you are provided with a URI to access your transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withOutputLocation(String outputLocation) {
setOutputLocation(outputLocation);
return this;
}
/**
*
* The KMS key you want to use to encrypt your Call Analytics output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*
*
* @param outputEncryptionKMSKeyId
* The KMS key you want to use to encrypt your Call Analytics output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*/
public void setOutputEncryptionKMSKeyId(String outputEncryptionKMSKeyId) {
this.outputEncryptionKMSKeyId = outputEncryptionKMSKeyId;
}
/**
*
* The KMS key you want to use to encrypt your Call Analytics output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*
*
* @return The KMS key you want to use to encrypt your Call Analytics output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key
* (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*/
public String getOutputEncryptionKMSKeyId() {
return this.outputEncryptionKMSKeyId;
}
/**
*
* The KMS key you want to use to encrypt your Call Analytics output.
*
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in one of
* four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web Services
* account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example, arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
*
*
* @param outputEncryptionKMSKeyId
* The KMS key you want to use to encrypt your Call Analytics output.
*
* If using a key located in the current Amazon Web Services account, you can specify your KMS key in
* one of four ways:
*
*
* -
*
* Use the KMS key ID itself. For example, 1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use an alias for the KMS key ID. For example, alias/ExampleAlias
.
*
*
* -
*
* Use the Amazon Resource Name (ARN) for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If using a key located in a different Amazon Web Services account than the current Amazon Web
* Services account, you can specify your KMS key in one of two ways:
*
*
* -
*
* Use the ARN for the KMS key ID. For example,
* arn:aws:kms:region:account-ID:key/1234abcd-12ab-34cd-56ef-1234567890ab
.
*
*
* -
*
* Use the ARN for the KMS key alias. For example,
* arn:aws:kms:region:account-ID:alias/ExampleAlias
.
*
*
*
*
* If you do not specify an encryption key, your output is encrypted with the default Amazon S3 key (SSE-S3).
*
*
* If you specify a KMS key to encrypt your output, you must also specify an output location using the
* OutputLocation
parameter.
*
*
* Note that the role making the request must have permission to use the specified KMS key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withOutputEncryptionKMSKeyId(String outputEncryptionKMSKeyId) {
setOutputEncryptionKMSKeyId(outputEncryptionKMSKeyId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files. If the role that you specify doesn’t have the appropriate permissions to access the specified
* Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files. If the role that you specify doesn’t have the appropriate permissions to access
* the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files. If the role that you specify doesn’t have the appropriate permissions to access the specified
* Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files. If the role that you specify doesn’t have the appropriate permissions to
* access the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public String getDataAccessRoleArn() {
return this.dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files. If the role that you specify doesn’t have the appropriate permissions to access the specified
* Amazon S3 location, your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files. If the role that you specify doesn’t have the appropriate permissions to access
* the specified Amazon S3 location, your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withDataAccessRoleArn(String dataAccessRoleArn) {
setDataAccessRoleArn(dataAccessRoleArn);
return this;
}
/**
*
* Specify additional optional settings in your request, including content redaction; allows you to apply custom
* language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*
*
* @param settings
* Specify additional optional settings in your request, including content redaction; allows you to apply
* custom language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*/
public void setSettings(CallAnalyticsJobSettings settings) {
this.settings = settings;
}
/**
*
* Specify additional optional settings in your request, including content redaction; allows you to apply custom
* language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*
*
* @return Specify additional optional settings in your request, including content redaction; allows you to apply
* custom language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*/
public CallAnalyticsJobSettings getSettings() {
return this.settings;
}
/**
*
* Specify additional optional settings in your request, including content redaction; allows you to apply custom
* language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
*
*
* @param settings
* Specify additional optional settings in your request, including content redaction; allows you to apply
* custom language models, vocabulary filters, and custom vocabularies to your Call Analytics job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withSettings(CallAnalyticsJobSettings settings) {
setSettings(settings);
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*
* @return Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first
* channel) and ParticipantRole
to AGENT
(to indicate that it's the agent
* speaking).
*/
public java.util.List getChannelDefinitions() {
return channelDefinitions;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first
* channel) and ParticipantRole
to AGENT
(to indicate that it's the agent
* speaking).
*/
public void setChannelDefinitions(java.util.Collection channelDefinitions) {
if (channelDefinitions == null) {
this.channelDefinitions = null;
return;
}
this.channelDefinitions = new java.util.ArrayList(channelDefinitions);
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChannelDefinitions(java.util.Collection)} or {@link #withChannelDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first
* channel) and ParticipantRole
to AGENT
(to indicate that it's the agent
* speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withChannelDefinitions(ChannelDefinition... channelDefinitions) {
if (this.channelDefinitions == null) {
setChannelDefinitions(new java.util.ArrayList(channelDefinitions.length));
}
for (ChannelDefinition ele : channelDefinitions) {
this.channelDefinitions.add(ele);
}
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first channel) and
* ParticipantRole
to AGENT
(to indicate that it's the agent speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if your agent is the first
* participant to speak, you would set ChannelId
to 0
(to indicate the first
* channel) and ParticipantRole
to AGENT
(to indicate that it's the agent
* speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartCallAnalyticsJobRequest withChannelDefinitions(java.util.Collection channelDefinitions) {
setChannelDefinitions(channelDefinitions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCallAnalyticsJobName() != null)
sb.append("CallAnalyticsJobName: ").append(getCallAnalyticsJobName()).append(",");
if (getMedia() != null)
sb.append("Media: ").append(getMedia()).append(",");
if (getOutputLocation() != null)
sb.append("OutputLocation: ").append(getOutputLocation()).append(",");
if (getOutputEncryptionKMSKeyId() != null)
sb.append("OutputEncryptionKMSKeyId: ").append(getOutputEncryptionKMSKeyId()).append(",");
if (getDataAccessRoleArn() != null)
sb.append("DataAccessRoleArn: ").append(getDataAccessRoleArn()).append(",");
if (getSettings() != null)
sb.append("Settings: ").append(getSettings()).append(",");
if (getChannelDefinitions() != null)
sb.append("ChannelDefinitions: ").append(getChannelDefinitions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartCallAnalyticsJobRequest == false)
return false;
StartCallAnalyticsJobRequest other = (StartCallAnalyticsJobRequest) obj;
if (other.getCallAnalyticsJobName() == null ^ this.getCallAnalyticsJobName() == null)
return false;
if (other.getCallAnalyticsJobName() != null && other.getCallAnalyticsJobName().equals(this.getCallAnalyticsJobName()) == false)
return false;
if (other.getMedia() == null ^ this.getMedia() == null)
return false;
if (other.getMedia() != null && other.getMedia().equals(this.getMedia()) == false)
return false;
if (other.getOutputLocation() == null ^ this.getOutputLocation() == null)
return false;
if (other.getOutputLocation() != null && other.getOutputLocation().equals(this.getOutputLocation()) == false)
return false;
if (other.getOutputEncryptionKMSKeyId() == null ^ this.getOutputEncryptionKMSKeyId() == null)
return false;
if (other.getOutputEncryptionKMSKeyId() != null && other.getOutputEncryptionKMSKeyId().equals(this.getOutputEncryptionKMSKeyId()) == false)
return false;
if (other.getDataAccessRoleArn() == null ^ this.getDataAccessRoleArn() == null)
return false;
if (other.getDataAccessRoleArn() != null && other.getDataAccessRoleArn().equals(this.getDataAccessRoleArn()) == false)
return false;
if (other.getSettings() == null ^ this.getSettings() == null)
return false;
if (other.getSettings() != null && other.getSettings().equals(this.getSettings()) == false)
return false;
if (other.getChannelDefinitions() == null ^ this.getChannelDefinitions() == null)
return false;
if (other.getChannelDefinitions() != null && other.getChannelDefinitions().equals(this.getChannelDefinitions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCallAnalyticsJobName() == null) ? 0 : getCallAnalyticsJobName().hashCode());
hashCode = prime * hashCode + ((getMedia() == null) ? 0 : getMedia().hashCode());
hashCode = prime * hashCode + ((getOutputLocation() == null) ? 0 : getOutputLocation().hashCode());
hashCode = prime * hashCode + ((getOutputEncryptionKMSKeyId() == null) ? 0 : getOutputEncryptionKMSKeyId().hashCode());
hashCode = prime * hashCode + ((getDataAccessRoleArn() == null) ? 0 : getDataAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getSettings() == null) ? 0 : getSettings().hashCode());
hashCode = prime * hashCode + ((getChannelDefinitions() == null) ? 0 : getChannelDefinitions().hashCode());
return hashCode;
}
@Override
public StartCallAnalyticsJobRequest clone() {
return (StartCallAnalyticsJobRequest) super.clone();
}
}