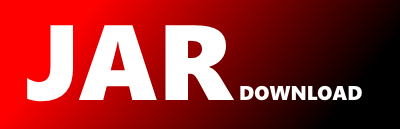
com.amazonaws.services.transcribe.model.CallAnalyticsJobSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides detailed information about a specific Call Analytics job.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CallAnalyticsJobSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*/
private String callAnalyticsJobName;
/**
*
* The date and time the specified Call Analytics job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*/
private java.util.Date creationTime;
/**
*
* The date and time your Call Analytics job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*/
private java.util.Date startTime;
/**
*
* The date and time the specified Call Analytics job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at 12:33 PM
* UTC-7 on May 4, 2022.
*
*/
private java.util.Date completionTime;
/**
*
* The language code used to create your Call Analytics transcription.
*
*/
private String languageCode;
/**
*
* Provides the status of your Call Analytics job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details on why
* your transcription job failed.
*
*/
private String callAnalyticsJobStatus;
/**
*
* Provides detailed information about a call analytics job, including information about skipped analytics features.
*
*/
private CallAnalyticsJobDetails callAnalyticsJobDetails;
/**
*
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains information
* about why the Call Analytics job failed. See also: Common Errors.
*
*/
private String failureReason;
/**
*
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @param callAnalyticsJobName
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
*/
public void setCallAnalyticsJobName(String callAnalyticsJobName) {
this.callAnalyticsJobName = callAnalyticsJobName;
}
/**
*
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @return The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
*/
public String getCallAnalyticsJobName() {
return this.callAnalyticsJobName;
}
/**
*
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @param callAnalyticsJobName
* The name of the Call Analytics job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withCallAnalyticsJobName(String callAnalyticsJobName) {
setCallAnalyticsJobName(callAnalyticsJobName);
return this;
}
/**
*
* The date and time the specified Call Analytics job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param creationTime
* The date and time the specified Call Analytics job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The date and time the specified Call Analytics job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified Call Analytics job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The date and time the specified Call Analytics job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param creationTime
* The date and time the specified Call Analytics job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The date and time your Call Analytics job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param startTime
* The date and time your Call Analytics job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time your Call Analytics job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @return The date and time your Call Analytics job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time your Call Analytics job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param startTime
* The date and time your Call Analytics job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a transcription job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The date and time the specified Call Analytics job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at 12:33 PM
* UTC-7 on May 4, 2022.
*
*
* @param completionTime
* The date and time the specified Call Analytics job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at
* 12:33 PM UTC-7 on May 4, 2022.
*/
public void setCompletionTime(java.util.Date completionTime) {
this.completionTime = completionTime;
}
/**
*
* The date and time the specified Call Analytics job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at 12:33 PM
* UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified Call Analytics job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at
* 12:33 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getCompletionTime() {
return this.completionTime;
}
/**
*
* The date and time the specified Call Analytics job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at 12:33 PM
* UTC-7 on May 4, 2022.
*
*
* @param completionTime
* The date and time the specified Call Analytics job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:33:13.922000-07:00
represents a transcription job that started processing at
* 12:33 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withCompletionTime(java.util.Date completionTime) {
setCompletionTime(completionTime);
return this;
}
/**
*
* The language code used to create your Call Analytics transcription.
*
*
* @param languageCode
* The language code used to create your Call Analytics transcription.
* @see LanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
*
* The language code used to create your Call Analytics transcription.
*
*
* @return The language code used to create your Call Analytics transcription.
* @see LanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
*
* The language code used to create your Call Analytics transcription.
*
*
* @param languageCode
* The language code used to create your Call Analytics transcription.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public CallAnalyticsJobSummary withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
*
* The language code used to create your Call Analytics transcription.
*
*
* @param languageCode
* The language code used to create your Call Analytics transcription.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LanguageCode
*/
public CallAnalyticsJobSummary withLanguageCode(LanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
*
* Provides the status of your Call Analytics job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details on why
* your transcription job failed.
*
*
* @param callAnalyticsJobStatus
* Provides the status of your Call Analytics job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
* @see CallAnalyticsJobStatus
*/
public void setCallAnalyticsJobStatus(String callAnalyticsJobStatus) {
this.callAnalyticsJobStatus = callAnalyticsJobStatus;
}
/**
*
* Provides the status of your Call Analytics job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details on why
* your transcription job failed.
*
*
* @return Provides the status of your Call Analytics job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
* @see CallAnalyticsJobStatus
*/
public String getCallAnalyticsJobStatus() {
return this.callAnalyticsJobStatus;
}
/**
*
* Provides the status of your Call Analytics job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details on why
* your transcription job failed.
*
*
* @param callAnalyticsJobStatus
* Provides the status of your Call Analytics job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CallAnalyticsJobStatus
*/
public CallAnalyticsJobSummary withCallAnalyticsJobStatus(String callAnalyticsJobStatus) {
setCallAnalyticsJobStatus(callAnalyticsJobStatus);
return this;
}
/**
*
* Provides the status of your Call Analytics job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details on why
* your transcription job failed.
*
*
* @param callAnalyticsJobStatus
* Provides the status of your Call Analytics job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in TranscriptFileUri
(or RedactedTranscriptFileUri
, if you requested
* transcript redaction). If the status is FAILED
, FailureReason
provides details
* on why your transcription job failed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CallAnalyticsJobStatus
*/
public CallAnalyticsJobSummary withCallAnalyticsJobStatus(CallAnalyticsJobStatus callAnalyticsJobStatus) {
this.callAnalyticsJobStatus = callAnalyticsJobStatus.toString();
return this;
}
/**
*
* Provides detailed information about a call analytics job, including information about skipped analytics features.
*
*
* @param callAnalyticsJobDetails
* Provides detailed information about a call analytics job, including information about skipped analytics
* features.
*/
public void setCallAnalyticsJobDetails(CallAnalyticsJobDetails callAnalyticsJobDetails) {
this.callAnalyticsJobDetails = callAnalyticsJobDetails;
}
/**
*
* Provides detailed information about a call analytics job, including information about skipped analytics features.
*
*
* @return Provides detailed information about a call analytics job, including information about skipped analytics
* features.
*/
public CallAnalyticsJobDetails getCallAnalyticsJobDetails() {
return this.callAnalyticsJobDetails;
}
/**
*
* Provides detailed information about a call analytics job, including information about skipped analytics features.
*
*
* @param callAnalyticsJobDetails
* Provides detailed information about a call analytics job, including information about skipped analytics
* features.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withCallAnalyticsJobDetails(CallAnalyticsJobDetails callAnalyticsJobDetails) {
setCallAnalyticsJobDetails(callAnalyticsJobDetails);
return this;
}
/**
*
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains information
* about why the Call Analytics job failed. See also: Common Errors.
*
*
* @param failureReason
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains
* information about why the Call Analytics job failed. See also: Common Errors.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains information
* about why the Call Analytics job failed. See also: Common Errors.
*
*
* @return If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains
* information about why the Call Analytics job failed. See also: Common Errors.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains information
* about why the Call Analytics job failed. See also: Common Errors.
*
*
* @param failureReason
* If CallAnalyticsJobStatus
is FAILED
, FailureReason
contains
* information about why the Call Analytics job failed. See also: Common Errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CallAnalyticsJobSummary withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCallAnalyticsJobName() != null)
sb.append("CallAnalyticsJobName: ").append(getCallAnalyticsJobName()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getCompletionTime() != null)
sb.append("CompletionTime: ").append(getCompletionTime()).append(",");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getCallAnalyticsJobStatus() != null)
sb.append("CallAnalyticsJobStatus: ").append(getCallAnalyticsJobStatus()).append(",");
if (getCallAnalyticsJobDetails() != null)
sb.append("CallAnalyticsJobDetails: ").append(getCallAnalyticsJobDetails()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CallAnalyticsJobSummary == false)
return false;
CallAnalyticsJobSummary other = (CallAnalyticsJobSummary) obj;
if (other.getCallAnalyticsJobName() == null ^ this.getCallAnalyticsJobName() == null)
return false;
if (other.getCallAnalyticsJobName() != null && other.getCallAnalyticsJobName().equals(this.getCallAnalyticsJobName()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getCompletionTime() == null ^ this.getCompletionTime() == null)
return false;
if (other.getCompletionTime() != null && other.getCompletionTime().equals(this.getCompletionTime()) == false)
return false;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getCallAnalyticsJobStatus() == null ^ this.getCallAnalyticsJobStatus() == null)
return false;
if (other.getCallAnalyticsJobStatus() != null && other.getCallAnalyticsJobStatus().equals(this.getCallAnalyticsJobStatus()) == false)
return false;
if (other.getCallAnalyticsJobDetails() == null ^ this.getCallAnalyticsJobDetails() == null)
return false;
if (other.getCallAnalyticsJobDetails() != null && other.getCallAnalyticsJobDetails().equals(this.getCallAnalyticsJobDetails()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCallAnalyticsJobName() == null) ? 0 : getCallAnalyticsJobName().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getCompletionTime() == null) ? 0 : getCompletionTime().hashCode());
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getCallAnalyticsJobStatus() == null) ? 0 : getCallAnalyticsJobStatus().hashCode());
hashCode = prime * hashCode + ((getCallAnalyticsJobDetails() == null) ? 0 : getCallAnalyticsJobDetails().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
return hashCode;
}
@Override
public CallAnalyticsJobSummary clone() {
try {
return (CallAnalyticsJobSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.transcribe.model.transform.CallAnalyticsJobSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}