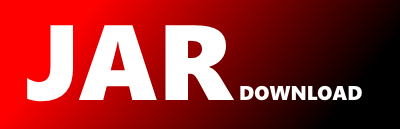
com.amazonaws.services.transcribe.model.MedicalScribeJob Maven / Gradle / Ivy
Show all versions of aws-java-sdk-transcribe Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.transcribe.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides detailed information about a Medical Scribe job.
*
*
* To view the status of the specified Medical Scribe job, check the MedicalScribeJobStatus
field. If the
* status is COMPLETED
, the job is finished and you can find the results at the locations specified in
* MedicalScribeOutput
. If the status is FAILED
, FailureReason
provides details
* on why your Medical Scribe job failed.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class MedicalScribeJob implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*/
private String medicalScribeJobName;
/**
*
* Provides the status of the specified Medical Scribe job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*/
private String medicalScribeJobStatus;
/**
*
* The language code used to create your Medical Scribe job. US English (en-US
) is the only supported
* language for Medical Scribe jobs.
*
*/
private String languageCode;
private Media media;
/**
*
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon S3 URI
* for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the Transcript.
*
*/
private MedicalScribeOutput medicalScribeOutput;
/**
*
* The date and time your Medical Scribe job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*/
private java.util.Date startTime;
/**
*
* The date and time the specified Medical Scribe job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*/
private java.util.Date creationTime;
/**
*
* The date and time the specified Medical Scribe job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at 12:32
* PM UTC-7 on May 4, 2022.
*
*/
private java.util.Date completionTime;
/**
*
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains information
* about why the transcription job failed. See also: Common Errors.
*
*/
private String failureReason;
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*/
private MedicalScribeSettings settings;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*/
private String dataAccessRoleArn;
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*/
private java.util.List channelDefinitions;
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*/
private java.util.List tags;
/**
*
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @param medicalScribeJobName
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
*/
public void setMedicalScribeJobName(String medicalScribeJobName) {
this.medicalScribeJobName = medicalScribeJobName;
}
/**
*
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @return The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
*/
public String getMedicalScribeJobName() {
return this.medicalScribeJobName;
}
/**
*
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web Services
* account.
*
*
* @param medicalScribeJobName
* The name of the Medical Scribe job. Job names are case sensitive and must be unique within an Amazon Web
* Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withMedicalScribeJobName(String medicalScribeJobName) {
setMedicalScribeJobName(medicalScribeJobName);
return this;
}
/**
*
* Provides the status of the specified Medical Scribe job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* @param medicalScribeJobStatus
* Provides the status of the specified Medical Scribe job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
,
* FailureReason
provides details on why your Medical Scribe job failed.
* @see MedicalScribeJobStatus
*/
public void setMedicalScribeJobStatus(String medicalScribeJobStatus) {
this.medicalScribeJobStatus = medicalScribeJobStatus;
}
/**
*
* Provides the status of the specified Medical Scribe job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* @return Provides the status of the specified Medical Scribe job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
,
* FailureReason
provides details on why your Medical Scribe job failed.
* @see MedicalScribeJobStatus
*/
public String getMedicalScribeJobStatus() {
return this.medicalScribeJobStatus;
}
/**
*
* Provides the status of the specified Medical Scribe job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* @param medicalScribeJobStatus
* Provides the status of the specified Medical Scribe job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
,
* FailureReason
provides details on why your Medical Scribe job failed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalScribeJobStatus
*/
public MedicalScribeJob withMedicalScribeJobStatus(String medicalScribeJobStatus) {
setMedicalScribeJobStatus(medicalScribeJobStatus);
return this;
}
/**
*
* Provides the status of the specified Medical Scribe job.
*
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
, FailureReason
* provides details on why your Medical Scribe job failed.
*
*
* @param medicalScribeJobStatus
* Provides the status of the specified Medical Scribe job.
*
* If the status is COMPLETED
, the job is finished and you can find the results at the location
* specified in MedicalScribeOutput
If the status is FAILED
,
* FailureReason
provides details on why your Medical Scribe job failed.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalScribeJobStatus
*/
public MedicalScribeJob withMedicalScribeJobStatus(MedicalScribeJobStatus medicalScribeJobStatus) {
this.medicalScribeJobStatus = medicalScribeJobStatus.toString();
return this;
}
/**
*
* The language code used to create your Medical Scribe job. US English (en-US
) is the only supported
* language for Medical Scribe jobs.
*
*
* @param languageCode
* The language code used to create your Medical Scribe job. US English (en-US
) is the only
* supported language for Medical Scribe jobs.
* @see MedicalScribeLanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
*
* The language code used to create your Medical Scribe job. US English (en-US
) is the only supported
* language for Medical Scribe jobs.
*
*
* @return The language code used to create your Medical Scribe job. US English (en-US
) is the only
* supported language for Medical Scribe jobs.
* @see MedicalScribeLanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
*
* The language code used to create your Medical Scribe job. US English (en-US
) is the only supported
* language for Medical Scribe jobs.
*
*
* @param languageCode
* The language code used to create your Medical Scribe job. US English (en-US
) is the only
* supported language for Medical Scribe jobs.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalScribeLanguageCode
*/
public MedicalScribeJob withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
*
* The language code used to create your Medical Scribe job. US English (en-US
) is the only supported
* language for Medical Scribe jobs.
*
*
* @param languageCode
* The language code used to create your Medical Scribe job. US English (en-US
) is the only
* supported language for Medical Scribe jobs.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MedicalScribeLanguageCode
*/
public MedicalScribeJob withLanguageCode(MedicalScribeLanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
* @param media
*/
public void setMedia(Media media) {
this.media = media;
}
/**
* @return
*/
public Media getMedia() {
return this.media;
}
/**
* @param media
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withMedia(Media media) {
setMedia(media);
return this;
}
/**
*
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon S3 URI
* for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the Transcript.
*
*
* @param medicalScribeOutput
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon
* S3 URI for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the
* Transcript.
*/
public void setMedicalScribeOutput(MedicalScribeOutput medicalScribeOutput) {
this.medicalScribeOutput = medicalScribeOutput;
}
/**
*
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon S3 URI
* for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the Transcript.
*
*
* @return The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon
* S3 URI for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the
* Transcript.
*/
public MedicalScribeOutput getMedicalScribeOutput() {
return this.medicalScribeOutput;
}
/**
*
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon S3 URI
* for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the Transcript.
*
*
* @param medicalScribeOutput
* The location of the output of your Medical Scribe job. ClinicalDocumentUri
holds the Amazon
* S3 URI for the Clinical Document and TranscriptFileUri
holds the Amazon S3 URI for the
* Transcript.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withMedicalScribeOutput(MedicalScribeOutput medicalScribeOutput) {
setMedicalScribeOutput(medicalScribeOutput);
return this;
}
/**
*
* The date and time your Medical Scribe job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param startTime
* The date and time your Medical Scribe job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The date and time your Medical Scribe job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @return The date and time your Medical Scribe job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The date and time your Medical Scribe job began processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param startTime
* The date and time your Medical Scribe job began processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.789000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The date and time the specified Medical Scribe job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param creationTime
* The date and time the specified Medical Scribe job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The date and time the specified Medical Scribe job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified Medical Scribe job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The date and time the specified Medical Scribe job request was made.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at 12:32 PM
* UTC-7 on May 4, 2022.
*
*
* @param creationTime
* The date and time the specified Medical Scribe job request was made.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that started processing at
* 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The date and time the specified Medical Scribe job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at 12:32
* PM UTC-7 on May 4, 2022.
*
*
* @param completionTime
* The date and time the specified Medical Scribe job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public void setCompletionTime(java.util.Date completionTime) {
this.completionTime = completionTime;
}
/**
*
* The date and time the specified Medical Scribe job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at 12:32
* PM UTC-7 on May 4, 2022.
*
*
* @return The date and time the specified Medical Scribe job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at
* 12:32 PM UTC-7 on May 4, 2022.
*/
public java.util.Date getCompletionTime() {
return this.completionTime;
}
/**
*
* The date and time the specified Medical Scribe job finished processing.
*
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at 12:32
* PM UTC-7 on May 4, 2022.
*
*
* @param completionTime
* The date and time the specified Medical Scribe job finished processing.
*
* Timestamps are in the format YYYY-MM-DD'T'HH:MM:SS.SSSSSS-UTC
. For example,
* 2022-05-04T12:32:58.761000-07:00
represents a Medical Scribe job that finished processing at
* 12:32 PM UTC-7 on May 4, 2022.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withCompletionTime(java.util.Date completionTime) {
setCompletionTime(completionTime);
return this;
}
/**
*
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains information
* about why the transcription job failed. See also: Common Errors.
*
*
* @param failureReason
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains
* information about why the transcription job failed. See also: Common Errors.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains information
* about why the transcription job failed. See also: Common Errors.
*
*
* @return If MedicalScribeJobStatus
is FAILED
, FailureReason
contains
* information about why the transcription job failed. See also: Common Errors.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains information
* about why the transcription job failed. See also: Common Errors.
*
*
* @param failureReason
* If MedicalScribeJobStatus
is FAILED
, FailureReason
contains
* information about why the transcription job failed. See also: Common Errors.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @param settings
* Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
*/
public void setSettings(MedicalScribeSettings settings) {
this.settings = settings;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @return Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
*/
public MedicalScribeSettings getSettings() {
return this.settings;
}
/**
*
* Makes it possible to control how your Medical Scribe job is processed using a MedicalScribeSettings
* object. Specify ChannelIdentification
if ChannelDefinitions
are set. Enabled
* ShowSpeakerLabels
if ChannelIdentification
and ChannelDefinitions
are not
* set. One and only one of ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use Settings
* to specify a vocabulary or vocabulary filter or both using VocabularyName
,
* VocabularyFilterName
. VocabularyFilterMethod
must be specified if
* VocabularyFilterName
is set.
*
*
* @param settings
* Makes it possible to control how your Medical Scribe job is processed using a
* MedicalScribeSettings
object. Specify ChannelIdentification
if
* ChannelDefinitions
are set. Enabled ShowSpeakerLabels
if
* ChannelIdentification
and ChannelDefinitions
are not set. One and only one of
* ChannelIdentification
and ShowSpeakerLabels
must be set. If
* ShowSpeakerLabels
is set, MaxSpeakerLabels
must also be set. Use
* Settings
to specify a vocabulary or vocabulary filter or both using
* VocabularyName
, VocabularyFilterName
. VocabularyFilterMethod
must
* be specified if VocabularyFilterName
is set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withSettings(MedicalScribeSettings settings) {
setSettings(settings);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
*/
public String getDataAccessRoleArn() {
return this.dataAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that contains
* your input files, write to the output bucket, and use your KMS key if supplied. If the role that you specify
* doesn’t have the appropriate permissions your request fails.
*
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For example:
* arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM ARNs.
*
*
* @param dataAccessRoleArn
* The Amazon Resource Name (ARN) of an IAM role that has permissions to access the Amazon S3 bucket that
* contains your input files, write to the output bucket, and use your KMS key if supplied. If the role that
* you specify doesn’t have the appropriate permissions your request fails.
*
* IAM role ARNs have the format arn:partition:iam::account:role/role-name-with-path
. For
* example: arn:aws:iam::111122223333:role/Admin
.
*
*
* For more information, see IAM
* ARNs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withDataAccessRoleArn(String dataAccessRoleArn) {
setDataAccessRoleArn(dataAccessRoleArn);
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @return Makes it possible to specify which speaker is on which channel. For example, if the clinician is the
* first participant to speak, you would set ChannelId
of the first
* ChannelDefinition
in the list to 0
(to indicate the first channel) and
* ParticipantRole
to CLINICIAN
(to indicate that it's the clinician speaking).
* Then you would set the ChannelId
of the second ChannelDefinition
in the list to
* 1
(to indicate the second channel) and ParticipantRole
to PATIENT
* (to indicate that it's the patient speaking).
*/
public java.util.List getChannelDefinitions() {
return channelDefinitions;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
*/
public void setChannelDefinitions(java.util.Collection channelDefinitions) {
if (channelDefinitions == null) {
this.channelDefinitions = null;
return;
}
this.channelDefinitions = new java.util.ArrayList(channelDefinitions);
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setChannelDefinitions(java.util.Collection)} or {@link #withChannelDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withChannelDefinitions(MedicalScribeChannelDefinition... channelDefinitions) {
if (this.channelDefinitions == null) {
setChannelDefinitions(new java.util.ArrayList(channelDefinitions.length));
}
for (MedicalScribeChannelDefinition ele : channelDefinitions) {
this.channelDefinitions.add(ele);
}
return this;
}
/**
*
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in the
* list to 0
(to indicate the first channel) and ParticipantRole
to CLINICIAN
* (to indicate that it's the clinician speaking). Then you would set the ChannelId
of the second
* ChannelDefinition
in the list to 1
(to indicate the second channel) and
* ParticipantRole
to PATIENT
(to indicate that it's the patient speaking).
*
*
* @param channelDefinitions
* Makes it possible to specify which speaker is on which channel. For example, if the clinician is the first
* participant to speak, you would set ChannelId
of the first ChannelDefinition
in
* the list to 0
(to indicate the first channel) and ParticipantRole
to
* CLINICIAN
(to indicate that it's the clinician speaking). Then you would set the
* ChannelId
of the second ChannelDefinition
in the list to 1
(to
* indicate the second channel) and ParticipantRole
to PATIENT
(to indicate that
* it's the patient speaking).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withChannelDefinitions(java.util.Collection channelDefinitions) {
setChannelDefinitions(channelDefinitions);
return this;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @return Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
*
*
* @param tags
* Adds one or more custom tags, each in the form of a key:value pair, to the Medica Scribe job.
*
* To learn more about using tags with Amazon Transcribe, refer to Tagging resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public MedicalScribeJob withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMedicalScribeJobName() != null)
sb.append("MedicalScribeJobName: ").append(getMedicalScribeJobName()).append(",");
if (getMedicalScribeJobStatus() != null)
sb.append("MedicalScribeJobStatus: ").append(getMedicalScribeJobStatus()).append(",");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getMedia() != null)
sb.append("Media: ").append(getMedia()).append(",");
if (getMedicalScribeOutput() != null)
sb.append("MedicalScribeOutput: ").append(getMedicalScribeOutput()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getCompletionTime() != null)
sb.append("CompletionTime: ").append(getCompletionTime()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getSettings() != null)
sb.append("Settings: ").append(getSettings()).append(",");
if (getDataAccessRoleArn() != null)
sb.append("DataAccessRoleArn: ").append(getDataAccessRoleArn()).append(",");
if (getChannelDefinitions() != null)
sb.append("ChannelDefinitions: ").append(getChannelDefinitions()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof MedicalScribeJob == false)
return false;
MedicalScribeJob other = (MedicalScribeJob) obj;
if (other.getMedicalScribeJobName() == null ^ this.getMedicalScribeJobName() == null)
return false;
if (other.getMedicalScribeJobName() != null && other.getMedicalScribeJobName().equals(this.getMedicalScribeJobName()) == false)
return false;
if (other.getMedicalScribeJobStatus() == null ^ this.getMedicalScribeJobStatus() == null)
return false;
if (other.getMedicalScribeJobStatus() != null && other.getMedicalScribeJobStatus().equals(this.getMedicalScribeJobStatus()) == false)
return false;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getMedia() == null ^ this.getMedia() == null)
return false;
if (other.getMedia() != null && other.getMedia().equals(this.getMedia()) == false)
return false;
if (other.getMedicalScribeOutput() == null ^ this.getMedicalScribeOutput() == null)
return false;
if (other.getMedicalScribeOutput() != null && other.getMedicalScribeOutput().equals(this.getMedicalScribeOutput()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getCompletionTime() == null ^ this.getCompletionTime() == null)
return false;
if (other.getCompletionTime() != null && other.getCompletionTime().equals(this.getCompletionTime()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getSettings() == null ^ this.getSettings() == null)
return false;
if (other.getSettings() != null && other.getSettings().equals(this.getSettings()) == false)
return false;
if (other.getDataAccessRoleArn() == null ^ this.getDataAccessRoleArn() == null)
return false;
if (other.getDataAccessRoleArn() != null && other.getDataAccessRoleArn().equals(this.getDataAccessRoleArn()) == false)
return false;
if (other.getChannelDefinitions() == null ^ this.getChannelDefinitions() == null)
return false;
if (other.getChannelDefinitions() != null && other.getChannelDefinitions().equals(this.getChannelDefinitions()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMedicalScribeJobName() == null) ? 0 : getMedicalScribeJobName().hashCode());
hashCode = prime * hashCode + ((getMedicalScribeJobStatus() == null) ? 0 : getMedicalScribeJobStatus().hashCode());
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getMedia() == null) ? 0 : getMedia().hashCode());
hashCode = prime * hashCode + ((getMedicalScribeOutput() == null) ? 0 : getMedicalScribeOutput().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getCompletionTime() == null) ? 0 : getCompletionTime().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getSettings() == null) ? 0 : getSettings().hashCode());
hashCode = prime * hashCode + ((getDataAccessRoleArn() == null) ? 0 : getDataAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getChannelDefinitions() == null) ? 0 : getChannelDefinitions().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public MedicalScribeJob clone() {
try {
return (MedicalScribeJob) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.transcribe.model.transform.MedicalScribeJobMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}