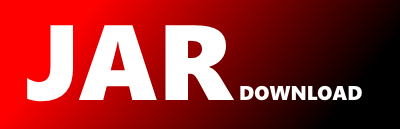
com.amazonaws.services.translate.AmazonTranslateAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-translate Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.translate;
import javax.annotation.Generated;
import com.amazonaws.services.translate.model.*;
/**
* Interface for accessing Amazon Translate asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.translate.AbstractAmazonTranslateAsync} instead.
*
*
*
* Provides translation between one source language and another of the same set of languages.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonTranslateAsync extends AmazonTranslate {
/**
*
* A synchronous action that deletes a custom terminology.
*
*
* @param deleteTerminologyRequest
* @return A Java Future containing the result of the DeleteTerminology operation returned by the service.
* @sample AmazonTranslateAsync.DeleteTerminology
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTerminologyAsync(DeleteTerminologyRequest deleteTerminologyRequest);
/**
*
* A synchronous action that deletes a custom terminology.
*
*
* @param deleteTerminologyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteTerminology operation returned by the service.
* @sample AmazonTranslateAsyncHandler.DeleteTerminology
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteTerminologyAsync(DeleteTerminologyRequest deleteTerminologyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves a custom terminology.
*
*
* @param getTerminologyRequest
* @return A Java Future containing the result of the GetTerminology operation returned by the service.
* @sample AmazonTranslateAsync.GetTerminology
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTerminologyAsync(GetTerminologyRequest getTerminologyRequest);
/**
*
* Retrieves a custom terminology.
*
*
* @param getTerminologyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTerminology operation returned by the service.
* @sample AmazonTranslateAsyncHandler.GetTerminology
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTerminologyAsync(GetTerminologyRequest getTerminologyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates or updates a custom terminology, depending on whether or not one already exists for the given terminology
* name. Importing a terminology with the same name as an existing one will merge the terminologies based on the
* chosen merge strategy. Currently, the only supported merge strategy is OVERWRITE, and so the imported terminology
* will overwrite an existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology take up to 10 minutes to fully
* propagate and be available for use in a translation due to cache policies with the DataPlane service that
* performs the translations.
*
*
* @param importTerminologyRequest
* @return A Java Future containing the result of the ImportTerminology operation returned by the service.
* @sample AmazonTranslateAsync.ImportTerminology
* @see AWS
* API Documentation
*/
java.util.concurrent.Future importTerminologyAsync(ImportTerminologyRequest importTerminologyRequest);
/**
*
* Creates or updates a custom terminology, depending on whether or not one already exists for the given terminology
* name. Importing a terminology with the same name as an existing one will merge the terminologies based on the
* chosen merge strategy. Currently, the only supported merge strategy is OVERWRITE, and so the imported terminology
* will overwrite an existing terminology of the same name.
*
*
* If you import a terminology that overwrites an existing one, the new terminology take up to 10 minutes to fully
* propagate and be available for use in a translation due to cache policies with the DataPlane service that
* performs the translations.
*
*
* @param importTerminologyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportTerminology operation returned by the service.
* @sample AmazonTranslateAsyncHandler.ImportTerminology
* @see AWS
* API Documentation
*/
java.util.concurrent.Future importTerminologyAsync(ImportTerminologyRequest importTerminologyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @param listTerminologiesRequest
* @return A Java Future containing the result of the ListTerminologies operation returned by the service.
* @sample AmazonTranslateAsync.ListTerminologies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTerminologiesAsync(ListTerminologiesRequest listTerminologiesRequest);
/**
*
* Provides a list of custom terminologies associated with your account.
*
*
* @param listTerminologiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTerminologies operation returned by the service.
* @sample AmazonTranslateAsyncHandler.ListTerminologies
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTerminologiesAsync(ListTerminologiesRequest listTerminologiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Translates input text from the source language to the target language. It is not necessary to use English (en) as
* either the source or the target language but not all language combinations are supported by Amazon Translate. For
* more information, see Supported Language
* Pairs.
*
*
* -
*
* Arabic (ar)
*
*
* -
*
* Chinese (Simplified) (zh)
*
*
* -
*
* Chinese (Traditional) (zh-TW)
*
*
* -
*
* Czech (cs)
*
*
* -
*
* Danish (da)
*
*
* -
*
* Dutch (nl)
*
*
* -
*
* English (en)
*
*
* -
*
* Finnish (fi)
*
*
* -
*
* French (fr)
*
*
* -
*
* German (de)
*
*
* -
*
* Hebrew (he)
*
*
* -
*
* Indonesian (id)
*
*
* -
*
* Italian (it)
*
*
* -
*
* Japanese (ja)
*
*
* -
*
* Korean (ko)
*
*
* -
*
* Polish (pl)
*
*
* -
*
* Portuguese (pt)
*
*
* -
*
* Russian (ru)
*
*
* -
*
* Spanish (es)
*
*
* -
*
* Swedish (sv)
*
*
* -
*
* Turkish (tr)
*
*
*
*
* To have Amazon Translate determine the source language of your text, you can specify auto
in the
* SourceLanguageCode
field. If you specify auto
, Amazon Translate will call Amazon
* Comprehend to determine the source language.
*
*
* @param translateTextRequest
* @return A Java Future containing the result of the TranslateText operation returned by the service.
* @sample AmazonTranslateAsync.TranslateText
* @see AWS API
* Documentation
*/
java.util.concurrent.Future translateTextAsync(TranslateTextRequest translateTextRequest);
/**
*
* Translates input text from the source language to the target language. It is not necessary to use English (en) as
* either the source or the target language but not all language combinations are supported by Amazon Translate. For
* more information, see Supported Language
* Pairs.
*
*
* -
*
* Arabic (ar)
*
*
* -
*
* Chinese (Simplified) (zh)
*
*
* -
*
* Chinese (Traditional) (zh-TW)
*
*
* -
*
* Czech (cs)
*
*
* -
*
* Danish (da)
*
*
* -
*
* Dutch (nl)
*
*
* -
*
* English (en)
*
*
* -
*
* Finnish (fi)
*
*
* -
*
* French (fr)
*
*
* -
*
* German (de)
*
*
* -
*
* Hebrew (he)
*
*
* -
*
* Indonesian (id)
*
*
* -
*
* Italian (it)
*
*
* -
*
* Japanese (ja)
*
*
* -
*
* Korean (ko)
*
*
* -
*
* Polish (pl)
*
*
* -
*
* Portuguese (pt)
*
*
* -
*
* Russian (ru)
*
*
* -
*
* Spanish (es)
*
*
* -
*
* Swedish (sv)
*
*
* -
*
* Turkish (tr)
*
*
*
*
* To have Amazon Translate determine the source language of your text, you can specify auto
in the
* SourceLanguageCode
field. If you specify auto
, Amazon Translate will call Amazon
* Comprehend to determine the source language.
*
*
* @param translateTextRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TranslateText operation returned by the service.
* @sample AmazonTranslateAsyncHandler.TranslateText
* @see AWS API
* Documentation
*/
java.util.concurrent.Future translateTextAsync(TranslateTextRequest translateTextRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}