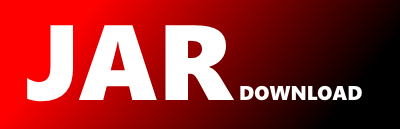
com.amazonaws.services.trustedadvisor.model.UpdateOrganizationRecommendationLifecycleRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-trustedadvisor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.trustedadvisor.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateOrganizationRecommendationLifecycleRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The new lifecycle stage
*
*/
private String lifecycleStage;
/**
*
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*
*/
private String organizationRecommendationIdentifier;
/**
*
* Reason for the lifecycle stage change
*
*/
private String updateReason;
/**
*
* Reason code for the lifecycle state change
*
*/
private String updateReasonCode;
/**
*
* The new lifecycle stage
*
*
* @param lifecycleStage
* The new lifecycle stage
* @see UpdateRecommendationLifecycleStage
*/
public void setLifecycleStage(String lifecycleStage) {
this.lifecycleStage = lifecycleStage;
}
/**
*
* The new lifecycle stage
*
*
* @return The new lifecycle stage
* @see UpdateRecommendationLifecycleStage
*/
public String getLifecycleStage() {
return this.lifecycleStage;
}
/**
*
* The new lifecycle stage
*
*
* @param lifecycleStage
* The new lifecycle stage
* @return Returns a reference to this object so that method calls can be chained together.
* @see UpdateRecommendationLifecycleStage
*/
public UpdateOrganizationRecommendationLifecycleRequest withLifecycleStage(String lifecycleStage) {
setLifecycleStage(lifecycleStage);
return this;
}
/**
*
* The new lifecycle stage
*
*
* @param lifecycleStage
* The new lifecycle stage
* @return Returns a reference to this object so that method calls can be chained together.
* @see UpdateRecommendationLifecycleStage
*/
public UpdateOrganizationRecommendationLifecycleRequest withLifecycleStage(UpdateRecommendationLifecycleStage lifecycleStage) {
this.lifecycleStage = lifecycleStage.toString();
return this;
}
/**
*
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*
*
* @param organizationRecommendationIdentifier
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*/
public void setOrganizationRecommendationIdentifier(String organizationRecommendationIdentifier) {
this.organizationRecommendationIdentifier = organizationRecommendationIdentifier;
}
/**
*
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*
*
* @return The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*/
public String getOrganizationRecommendationIdentifier() {
return this.organizationRecommendationIdentifier;
}
/**
*
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
*
*
* @param organizationRecommendationIdentifier
* The Recommendation identifier for AWS Trusted Advisor Priority recommendations
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateOrganizationRecommendationLifecycleRequest withOrganizationRecommendationIdentifier(String organizationRecommendationIdentifier) {
setOrganizationRecommendationIdentifier(organizationRecommendationIdentifier);
return this;
}
/**
*
* Reason for the lifecycle stage change
*
*
* @param updateReason
* Reason for the lifecycle stage change
*/
public void setUpdateReason(String updateReason) {
this.updateReason = updateReason;
}
/**
*
* Reason for the lifecycle stage change
*
*
* @return Reason for the lifecycle stage change
*/
public String getUpdateReason() {
return this.updateReason;
}
/**
*
* Reason for the lifecycle stage change
*
*
* @param updateReason
* Reason for the lifecycle stage change
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateOrganizationRecommendationLifecycleRequest withUpdateReason(String updateReason) {
setUpdateReason(updateReason);
return this;
}
/**
*
* Reason code for the lifecycle state change
*
*
* @param updateReasonCode
* Reason code for the lifecycle state change
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public void setUpdateReasonCode(String updateReasonCode) {
this.updateReasonCode = updateReasonCode;
}
/**
*
* Reason code for the lifecycle state change
*
*
* @return Reason code for the lifecycle state change
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public String getUpdateReasonCode() {
return this.updateReasonCode;
}
/**
*
* Reason code for the lifecycle state change
*
*
* @param updateReasonCode
* Reason code for the lifecycle state change
* @return Returns a reference to this object so that method calls can be chained together.
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public UpdateOrganizationRecommendationLifecycleRequest withUpdateReasonCode(String updateReasonCode) {
setUpdateReasonCode(updateReasonCode);
return this;
}
/**
*
* Reason code for the lifecycle state change
*
*
* @param updateReasonCode
* Reason code for the lifecycle state change
* @return Returns a reference to this object so that method calls can be chained together.
* @see UpdateRecommendationLifecycleStageReasonCode
*/
public UpdateOrganizationRecommendationLifecycleRequest withUpdateReasonCode(UpdateRecommendationLifecycleStageReasonCode updateReasonCode) {
this.updateReasonCode = updateReasonCode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLifecycleStage() != null)
sb.append("LifecycleStage: ").append(getLifecycleStage()).append(",");
if (getOrganizationRecommendationIdentifier() != null)
sb.append("OrganizationRecommendationIdentifier: ").append(getOrganizationRecommendationIdentifier()).append(",");
if (getUpdateReason() != null)
sb.append("UpdateReason: ").append("***Sensitive Data Redacted***").append(",");
if (getUpdateReasonCode() != null)
sb.append("UpdateReasonCode: ").append(getUpdateReasonCode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateOrganizationRecommendationLifecycleRequest == false)
return false;
UpdateOrganizationRecommendationLifecycleRequest other = (UpdateOrganizationRecommendationLifecycleRequest) obj;
if (other.getLifecycleStage() == null ^ this.getLifecycleStage() == null)
return false;
if (other.getLifecycleStage() != null && other.getLifecycleStage().equals(this.getLifecycleStage()) == false)
return false;
if (other.getOrganizationRecommendationIdentifier() == null ^ this.getOrganizationRecommendationIdentifier() == null)
return false;
if (other.getOrganizationRecommendationIdentifier() != null
&& other.getOrganizationRecommendationIdentifier().equals(this.getOrganizationRecommendationIdentifier()) == false)
return false;
if (other.getUpdateReason() == null ^ this.getUpdateReason() == null)
return false;
if (other.getUpdateReason() != null && other.getUpdateReason().equals(this.getUpdateReason()) == false)
return false;
if (other.getUpdateReasonCode() == null ^ this.getUpdateReasonCode() == null)
return false;
if (other.getUpdateReasonCode() != null && other.getUpdateReasonCode().equals(this.getUpdateReasonCode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLifecycleStage() == null) ? 0 : getLifecycleStage().hashCode());
hashCode = prime * hashCode + ((getOrganizationRecommendationIdentifier() == null) ? 0 : getOrganizationRecommendationIdentifier().hashCode());
hashCode = prime * hashCode + ((getUpdateReason() == null) ? 0 : getUpdateReason().hashCode());
hashCode = prime * hashCode + ((getUpdateReasonCode() == null) ? 0 : getUpdateReasonCode().hashCode());
return hashCode;
}
@Override
public UpdateOrganizationRecommendationLifecycleRequest clone() {
return (UpdateOrganizationRecommendationLifecycleRequest) super.clone();
}
}