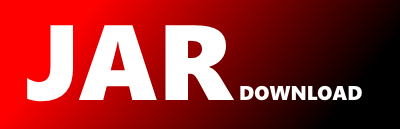
com.amazonaws.services.verifiedpermissions.AmazonVerifiedPermissions Maven / Gradle / Ivy
Show all versions of aws-java-sdk-verifiedpermissions Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.verifiedpermissions;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.verifiedpermissions.model.*;
/**
* Interface for accessing Amazon Verified Permissions.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.verifiedpermissions.AbstractAmazonVerifiedPermissions} instead.
*
*
*
* Amazon Verified Permissions is a permissions management service from Amazon Web Services. You can use Verified
* Permissions to manage permissions for your application, and authorize user access based on those permissions. Using
* Verified Permissions, application developers can grant access based on information about the users, resources, and
* requested actions. You can also evaluate additional information like group membership, attributes of the resources,
* and session context, such as time of request and IP addresses. Verified Permissions manages these permissions by
* letting you create and store authorization policies for your applications, such as consumer-facing web sites and
* enterprise business systems.
*
*
* Verified Permissions uses Cedar as the policy language to express your permission requirements. Cedar supports both
* role-based access control (RBAC) and attribute-based access control (ABAC) authorization models.
*
*
* For more information about configuring, administering, and using Amazon Verified Permissions in your applications,
* see the Amazon Verified Permissions User
* Guide.
*
*
* For more information about the Cedar policy language, see the Cedar Policy
* Language Guide.
*
*
*
* When you write Cedar policies that reference principals, resources and actions, you can define the unique identifiers
* used for each of those elements. We strongly recommend that you follow these best practices:
*
*
* -
*
* Use values like universally unique identifiers (UUIDs) for all principal and resource identifiers.
*
*
* For example, if user jane
leaves the company, and you later let someone else use the name
* jane
, then that new user automatically gets access to everything granted by policies that still
* reference User::"jane"
. Cedar can’t distinguish between the new user and the old. This applies to both
* principal and resource identifiers. Always use identifiers that are guaranteed unique and never reused to ensure that
* you don’t unintentionally grant access because of the presence of an old identifier in a policy.
*
*
* Where you use a UUID for an entity, we recommend that you follow it with the // comment specifier and the ‘friendly’
* name of your entity. This helps to make your policies easier to understand. For example: principal ==
* User::"a1b2c3d4-e5f6-a1b2-c3d4-EXAMPLE11111", // alice
*
*
* -
*
* Do not include personally identifying, confidential, or sensitive information as part of the unique identifier for
* your principals or resources. These identifiers are included in log entries shared in CloudTrail trails.
*
*
*
*
*
* Several operations return structures that appear similar, but have different purposes. As new functionality is added
* to the product, the structure used in a parameter of one operation might need to change in a way that wouldn't make
* sense for the same parameter in a different operation. To help you understand the purpose of each, the following
* naming convention is used for the structures:
*
*
* -
*
* Parameter type structures that end in Detail
are used in Get
operations.
*
*
* -
*
* Parameter type structures that end in Item
are used in List
operations.
*
*
* -
*
* Parameter type structures that use neither suffix are used in the mutating (create and update) operations.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonVerifiedPermissions {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "verifiedpermissions";
/**
*
* Makes a series of decisions about multiple authorization requests for one principal or resource. Each request
* contains the equivalent content of an IsAuthorized
request: principal, action, resource, and
* context. Either the principal
or the resource
parameter must be identical across all
* requests. For example, Verified Permissions won't evaluate a pair of requests where bob
views
* photo1
and alice
views photo2
. Authorization of bob
to view
* photo1
and photo2
, or bob
and alice
to view
* photo1
, are valid batches.
*
*
* The request is evaluated against all policies in the specified policy store that match the entities that you
* declare. The result of the decisions is a series of Allow
or Deny
responses, along with
* the IDs of the policies that produced each decision.
*
*
* The entities
of a BatchIsAuthorized
API request can contain up to 100 principals and up
* to 100 resources. The requests
of a BatchIsAuthorized
API request can contain up to 30
* requests.
*
*
*
* The BatchIsAuthorized
operation doesn't have its own IAM permission. To authorize this operation for
* Amazon Web Services principals, include the permission verifiedpermissions:IsAuthorized
in their IAM
* policies.
*
*
*
* @param batchIsAuthorizedRequest
* @return Result of the BatchIsAuthorized operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.BatchIsAuthorized
* @see AWS API Documentation
*/
BatchIsAuthorizedResult batchIsAuthorized(BatchIsAuthorizedRequest batchIsAuthorizedRequest);
/**
*
* Makes a series of decisions about multiple authorization requests for one token. The principal in this request
* comes from an external identity source in the form of an identity or access token, formatted as a JSON web token (JWT). The information in the parameters can
* also define additional context that Verified Permissions can include in the evaluations.
*
*
* The request is evaluated against all policies in the specified policy store that match the entities that you
* provide in the entities declaration and in the token. The result of the decisions is a series of
* Allow
or Deny
responses, along with the IDs of the policies that produced each
* decision.
*
*
* The entities
of a BatchIsAuthorizedWithToken
API request can contain up to 100
* resources and up to 99 user groups. The requests
of a BatchIsAuthorizedWithToken
API
* request can contain up to 30 requests.
*
*
*
* The BatchIsAuthorizedWithToken
operation doesn't have its own IAM permission. To authorize this
* operation for Amazon Web Services principals, include the permission
* verifiedpermissions:IsAuthorizedWithToken
in their IAM policies.
*
*
*
* @param batchIsAuthorizedWithTokenRequest
* @return Result of the BatchIsAuthorizedWithToken operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.BatchIsAuthorizedWithToken
* @see AWS API Documentation
*/
BatchIsAuthorizedWithTokenResult batchIsAuthorizedWithToken(BatchIsAuthorizedWithTokenRequest batchIsAuthorizedWithTokenRequest);
/**
*
* Adds an identity source to a policy store–an Amazon Cognito user pool or OpenID Connect (OIDC) identity provider
* (IdP).
*
*
* After you create an identity source, you can use the identities provided by the IdP as proxies for the principal
* in authorization queries that use the IsAuthorizedWithToken or BatchIsAuthorizedWithToken API operations. These identities take the form of tokens that contain claims
* about the user, such as IDs, attributes and group memberships. Identity sources provide identity (ID) tokens and
* access tokens. Verified Permissions derives information about your user and session from token claims. Access
* tokens provide action context
to your policies, and ID tokens provide principal
* Attributes
.
*
*
*
* Tokens from an identity source user continue to be usable until they expire. Token revocation and resource
* deletion have no effect on the validity of a token in your policy store
*
*
*
* To reference a user from this identity source in your Cedar policies, refer to the following syntax examples.
*
*
* -
*
* Amazon Cognito user pool: Namespace::[Entity type]::[User pool ID]|[user principal attribute]
, for
* example MyCorp::User::us-east-1_EXAMPLE|a1b2c3d4-5678-90ab-cdef-EXAMPLE11111
.
*
*
* -
*
* OpenID Connect (OIDC) provider:
* Namespace::[Entity type]::[principalIdClaim]|[user principal attribute]
, for example
* MyCorp::User::MyOIDCProvider|a1b2c3d4-5678-90ab-cdef-EXAMPLE22222
.
*
*
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param createIdentitySourceRequest
* @return Result of the CreateIdentitySource operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.CreateIdentitySource
* @see AWS API Documentation
*/
CreateIdentitySourceResult createIdentitySource(CreateIdentitySourceRequest createIdentitySourceRequest);
/**
*
* Creates a Cedar policy and saves it in the specified policy store. You can create either a static policy or a
* policy linked to a policy template.
*
*
* -
*
* To create a static policy, provide the Cedar policy text in the StaticPolicy
section of the
* PolicyDefinition
.
*
*
* -
*
* To create a policy that is dynamically linked to a policy template, specify the policy template ID and the
* principal and resource to associate with this policy in the templateLinked
section of the
* PolicyDefinition
. If the policy template is ever updated, any policies linked to the policy template
* automatically use the updated template.
*
*
*
*
*
* Creating a policy causes it to be validated against the schema in the policy store. If the policy doesn't pass
* validation, the operation fails and the policy isn't stored.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param createPolicyRequest
* @return Result of the CreatePolicy operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.CreatePolicy
* @see AWS API Documentation
*/
CreatePolicyResult createPolicy(CreatePolicyRequest createPolicyRequest);
/**
*
* Creates a policy store. A policy store is a container for policy resources.
*
*
*
* Although Cedar supports multiple
* namespaces, Verified Permissions currently supports only one namespace per policy store.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param createPolicyStoreRequest
* @return Result of the CreatePolicyStore operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.CreatePolicyStore
* @see AWS API Documentation
*/
CreatePolicyStoreResult createPolicyStore(CreatePolicyStoreRequest createPolicyStoreRequest);
/**
*
* Creates a policy template. A template can use placeholders for the principal and resource. A template must be
* instantiated into a policy by associating it with specific principals and resources to use for the placeholders.
* That instantiated policy can then be considered in authorization decisions. The instantiated policy works
* identically to any other policy, except that it is dynamically linked to the template. If the template changes,
* then any policies that are linked to that template are immediately updated as well.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param createPolicyTemplateRequest
* @return Result of the CreatePolicyTemplate operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.CreatePolicyTemplate
* @see AWS API Documentation
*/
CreatePolicyTemplateResult createPolicyTemplate(CreatePolicyTemplateRequest createPolicyTemplateRequest);
/**
*
* Deletes an identity source that references an identity provider (IdP) such as Amazon Cognito. After you delete
* the identity source, you can no longer use tokens for identities from that identity source to represent
* principals in authorization queries made using IsAuthorizedWithToken. operations.
*
*
* @param deleteIdentitySourceRequest
* @return Result of the DeleteIdentitySource operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.DeleteIdentitySource
* @see AWS API Documentation
*/
DeleteIdentitySourceResult deleteIdentitySource(DeleteIdentitySourceRequest deleteIdentitySourceRequest);
/**
*
* Deletes the specified policy from the policy store.
*
*
* This operation is idempotent; if you specify a policy that doesn't exist, the request response returns a
* successful HTTP 200
status code.
*
*
* @param deletePolicyRequest
* @return Result of the DeletePolicy operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.DeletePolicy
* @see AWS API Documentation
*/
DeletePolicyResult deletePolicy(DeletePolicyRequest deletePolicyRequest);
/**
*
* Deletes the specified policy store.
*
*
* This operation is idempotent. If you specify a policy store that does not exist, the request response will still
* return a successful HTTP 200 status code.
*
*
* @param deletePolicyStoreRequest
* @return Result of the DeletePolicyStore operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.DeletePolicyStore
* @see AWS API Documentation
*/
DeletePolicyStoreResult deletePolicyStore(DeletePolicyStoreRequest deletePolicyStoreRequest);
/**
*
* Deletes the specified policy template from the policy store.
*
*
*
* This operation also deletes any policies that were created from the specified policy template. Those policies are
* immediately removed from all future API responses, and are asynchronously deleted from the policy store.
*
*
*
* @param deletePolicyTemplateRequest
* @return Result of the DeletePolicyTemplate operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.DeletePolicyTemplate
* @see AWS API Documentation
*/
DeletePolicyTemplateResult deletePolicyTemplate(DeletePolicyTemplateRequest deletePolicyTemplateRequest);
/**
*
* Retrieves the details about the specified identity source.
*
*
* @param getIdentitySourceRequest
* @return Result of the GetIdentitySource operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.GetIdentitySource
* @see AWS API Documentation
*/
GetIdentitySourceResult getIdentitySource(GetIdentitySourceRequest getIdentitySourceRequest);
/**
*
* Retrieves information about the specified policy.
*
*
* @param getPolicyRequest
* @return Result of the GetPolicy operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.GetPolicy
* @see AWS
* API Documentation
*/
GetPolicyResult getPolicy(GetPolicyRequest getPolicyRequest);
/**
*
* Retrieves details about a policy store.
*
*
* @param getPolicyStoreRequest
* @return Result of the GetPolicyStore operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.GetPolicyStore
* @see AWS API Documentation
*/
GetPolicyStoreResult getPolicyStore(GetPolicyStoreRequest getPolicyStoreRequest);
/**
*
* Retrieve the details for the specified policy template in the specified policy store.
*
*
* @param getPolicyTemplateRequest
* @return Result of the GetPolicyTemplate operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.GetPolicyTemplate
* @see AWS API Documentation
*/
GetPolicyTemplateResult getPolicyTemplate(GetPolicyTemplateRequest getPolicyTemplateRequest);
/**
*
* Retrieve the details for the specified schema in the specified policy store.
*
*
* @param getSchemaRequest
* @return Result of the GetSchema operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.GetSchema
* @see AWS
* API Documentation
*/
GetSchemaResult getSchema(GetSchemaRequest getSchemaRequest);
/**
*
* Makes an authorization decision about a service request described in the parameters. The information in the
* parameters can also define additional context that Verified Permissions can include in the evaluation. The
* request is evaluated against all matching policies in the specified policy store. The result of the decision is
* either Allow
or Deny
, along with a list of the policies that resulted in the decision.
*
*
* @param isAuthorizedRequest
* @return Result of the IsAuthorized operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.IsAuthorized
* @see AWS API Documentation
*/
IsAuthorizedResult isAuthorized(IsAuthorizedRequest isAuthorizedRequest);
/**
*
* Makes an authorization decision about a service request described in the parameters. The principal in this
* request comes from an external identity source in the form of an identity token formatted as a JSON web token (JWT). The information in the parameters can
* also define additional context that Verified Permissions can include in the evaluation. The request is evaluated
* against all matching policies in the specified policy store. The result of the decision is either
* Allow
or Deny
, along with a list of the policies that resulted in the decision.
*
*
* At this time, Verified Permissions accepts tokens from only Amazon Cognito.
*
*
* Verified Permissions validates each token that is specified in a request by checking its expiration date and its
* signature.
*
*
*
* Tokens from an identity source user continue to be usable until they expire. Token revocation and resource
* deletion have no effect on the validity of a token in your policy store
*
*
*
* @param isAuthorizedWithTokenRequest
* @return Result of the IsAuthorizedWithToken operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.IsAuthorizedWithToken
* @see AWS API Documentation
*/
IsAuthorizedWithTokenResult isAuthorizedWithToken(IsAuthorizedWithTokenRequest isAuthorizedWithTokenRequest);
/**
*
* Returns a paginated list of all of the identity sources defined in the specified policy store.
*
*
* @param listIdentitySourcesRequest
* @return Result of the ListIdentitySources operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.ListIdentitySources
* @see AWS API Documentation
*/
ListIdentitySourcesResult listIdentitySources(ListIdentitySourcesRequest listIdentitySourcesRequest);
/**
*
* Returns a paginated list of all policies stored in the specified policy store.
*
*
* @param listPoliciesRequest
* @return Result of the ListPolicies operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.ListPolicies
* @see AWS API Documentation
*/
ListPoliciesResult listPolicies(ListPoliciesRequest listPoliciesRequest);
/**
*
* Returns a paginated list of all policy stores in the calling Amazon Web Services account.
*
*
* @param listPolicyStoresRequest
* @return Result of the ListPolicyStores operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.ListPolicyStores
* @see AWS API Documentation
*/
ListPolicyStoresResult listPolicyStores(ListPolicyStoresRequest listPolicyStoresRequest);
/**
*
* Returns a paginated list of all policy templates in the specified policy store.
*
*
* @param listPolicyTemplatesRequest
* @return Result of the ListPolicyTemplates operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.ListPolicyTemplates
* @see AWS API Documentation
*/
ListPolicyTemplatesResult listPolicyTemplates(ListPolicyTemplatesRequest listPolicyTemplatesRequest);
/**
*
* Creates or updates the policy schema in the specified policy store. The schema is used to validate any Cedar
* policies and policy templates submitted to the policy store. Any changes to the schema validate only policies and
* templates submitted after the schema change. Existing policies and templates are not re-evaluated against the
* changed schema. If you later update a policy, then it is evaluated against the new schema at that time.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param putSchemaRequest
* @return Result of the PutSchema operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.PutSchema
* @see AWS
* API Documentation
*/
PutSchemaResult putSchema(PutSchemaRequest putSchemaRequest);
/**
*
* Updates the specified identity source to use a new identity provider (IdP), or to change the mapping of
* identities from the IdP to a different principal entity type.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param updateIdentitySourceRequest
* @return Result of the UpdateIdentitySource operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.UpdateIdentitySource
* @see AWS API Documentation
*/
UpdateIdentitySourceResult updateIdentitySource(UpdateIdentitySourceRequest updateIdentitySourceRequest);
/**
*
* Modifies a Cedar static policy in the specified policy store. You can change only certain elements of the UpdatePolicyDefinition parameter. You can directly update only static policies. To change a template-linked
* policy, you must update the template instead, using UpdatePolicyTemplate.
*
*
*
* -
*
* If policy validation is enabled in the policy store, then updating a static policy causes Verified Permissions to
* validate the policy against the schema in the policy store. If the updated static policy doesn't pass validation,
* the operation fails and the update isn't stored.
*
*
* -
*
* When you edit a static policy, you can change only certain elements of a static policy:
*
*
* -
*
* The action referenced by the policy.
*
*
* -
*
* A condition clause, such as when and unless.
*
*
*
*
* You can't change these elements of a static policy:
*
*
* -
*
* Changing a policy from a static policy to a template-linked policy.
*
*
* -
*
* Changing the effect of a static policy from permit or forbid.
*
*
* -
*
* The principal referenced by a static policy.
*
*
* -
*
* The resource referenced by a static policy.
*
*
*
*
* -
*
* To update a template-linked policy, you must update the template instead.
*
*
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param updatePolicyRequest
* @return Result of the UpdatePolicy operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ServiceQuotaExceededException
* The request failed because it would cause a service quota to be exceeded.
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.UpdatePolicy
* @see AWS API Documentation
*/
UpdatePolicyResult updatePolicy(UpdatePolicyRequest updatePolicyRequest);
/**
*
* Modifies the validation setting for a policy store.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param updatePolicyStoreRequest
* @return Result of the UpdatePolicyStore operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.UpdatePolicyStore
* @see AWS API Documentation
*/
UpdatePolicyStoreResult updatePolicyStore(UpdatePolicyStoreRequest updatePolicyStoreRequest);
/**
*
* Updates the specified policy template. You can update only the description and the some elements of the policyBody.
*
*
*
* Changes you make to the policy template content are immediately (within the constraints of eventual consistency)
* reflected in authorization decisions that involve all template-linked policies instantiated from this template.
*
*
*
* Verified Permissions is eventually consistent
* . It can take a few seconds for a new or changed element to propagate through the service and be visible in
* the results of other Verified Permissions operations.
*
*
*
* @param updatePolicyTemplateRequest
* @return Result of the UpdatePolicyTemplate operation returned by the service.
* @throws ValidationException
* The request failed because one or more input parameters don't satisfy their constraint requirements. The
* output is provided as a list of fields and a reason for each field that isn't valid.
*
* The possible reasons include the following:
*
*
* -
*
* UnrecognizedEntityType
*
*
* The policy includes an entity type that isn't found in the schema.
*
*
* -
*
* UnrecognizedActionId
*
*
* The policy includes an action id that isn't found in the schema.
*
*
* -
*
* InvalidActionApplication
*
*
* The policy includes an action that, according to the schema, doesn't support the specified principal and
* resource.
*
*
* -
*
* UnexpectedType
*
*
* The policy included an operand that isn't a valid type for the specified operation.
*
*
* -
*
* IncompatibleTypes
*
*
* The types of elements included in a set
, or the types of expressions used in an
* if...then...else
clause aren't compatible in this context.
*
*
* -
*
* MissingAttribute
*
*
* The policy attempts to access a record or entity attribute that isn't specified in the schema. Test for
* the existence of the attribute first before attempting to access its value. For more information, see the
* has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* UnsafeOptionalAttributeAccess
*
*
* The policy attempts to access a record or entity attribute that is optional and isn't guaranteed to be
* present. Test for the existence of the attribute first before attempting to access its value. For more
* information, see the has
* (presence of attribute test) operator in the Cedar Policy Language Guide.
*
*
* -
*
* ImpossiblePolicy
*
*
* Cedar has determined that a policy condition always evaluates to false. If the policy is always false, it
* can never apply to any query, and so it can never affect an authorization decision.
*
*
* -
*
* WrongNumberArguments
*
*
* The policy references an extension type with the wrong number of arguments.
*
*
* -
*
* FunctionArgumentValidationError
*
*
* Cedar couldn't parse the argument passed to an extension type. For example, a string that is to be parsed
* as an IPv4 address can contain only digits and the period character.
*
*
* @throws ConflictException
* The request failed because another request to modify a resource occurred at the same.
* @throws AccessDeniedException
* You don't have sufficient access to perform this action.
* @throws ResourceNotFoundException
* The request failed because it references a resource that doesn't exist.
* @throws ThrottlingException
* The request failed because it exceeded a throttling quota.
* @throws InternalServerException
* The request failed because of an internal error. Try your request again later
* @sample AmazonVerifiedPermissions.UpdatePolicyTemplate
* @see AWS API Documentation
*/
UpdatePolicyTemplateResult updatePolicyTemplate(UpdatePolicyTemplateRequest updatePolicyTemplateRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}