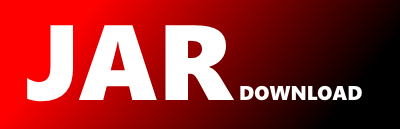
com.amazonaws.services.verifiedpermissions.model.AttributeValue Maven / Gradle / Ivy
Show all versions of aws-java-sdk-verifiedpermissions Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.verifiedpermissions.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The value of an attribute.
*
*
* Contains information about the runtime context for a request for which an authorization decision is made.
*
*
* This data type is used as a member of the ContextDefinition structure which is uses as a request parameter for the IsAuthorized, BatchIsAuthorized
* , and IsAuthorizedWithToken operations.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AttributeValue implements Serializable, Cloneable, StructuredPojo {
/**
*
* An attribute value of Boolean
* type.
*
*
* Example: {"boolean": true}
*
*/
private Boolean booleanValue;
/**
*
* An attribute value of type EntityIdentifier.
*
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*
*/
private EntityIdentifier entityIdentifier;
/**
*
* An attribute value of Long type.
*
*
* Example: {"long": 0}
*
*/
private Long longValue;
/**
*
* An attribute value of String
* type.
*
*
* Example: {"string": "abc"}
*
*/
private String string;
/**
*
* An attribute value of Set type.
*
*
* Example: {"set": [ {} ] }
*
*/
private java.util.List set;
/**
*
* An attribute value of Record
* type.
*
*
* Example: {"record": { "keyName": {} } }
*
*/
private java.util.Map record;
/**
*
* An attribute value of Boolean
* type.
*
*
* Example: {"boolean": true}
*
*
* @param booleanValue
* An attribute value of Boolean type.
*
* Example: {"boolean": true}
*/
public void setBoolean(Boolean booleanValue) {
this.booleanValue = booleanValue;
}
/**
*
* An attribute value of Boolean
* type.
*
*
* Example: {"boolean": true}
*
*
* @return An attribute value of Boolean type.
*
* Example: {"boolean": true}
*/
public Boolean getBoolean() {
return this.booleanValue;
}
/**
*
* An attribute value of Boolean
* type.
*
*
* Example: {"boolean": true}
*
*
* @param booleanValue
* An attribute value of Boolean type.
*
* Example: {"boolean": true}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withBoolean(Boolean booleanValue) {
setBoolean(booleanValue);
return this;
}
/**
*
* An attribute value of Boolean
* type.
*
*
* Example: {"boolean": true}
*
*
* @return An attribute value of Boolean type.
*
* Example: {"boolean": true}
*/
public Boolean isBoolean() {
return this.booleanValue;
}
/**
*
* An attribute value of type EntityIdentifier.
*
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*
*
* @param entityIdentifier
* An attribute value of type EntityIdentifier.
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*/
public void setEntityIdentifier(EntityIdentifier entityIdentifier) {
this.entityIdentifier = entityIdentifier;
}
/**
*
* An attribute value of type EntityIdentifier.
*
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*
*
* @return An attribute value of type EntityIdentifier.
*
* Example:
* "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*/
public EntityIdentifier getEntityIdentifier() {
return this.entityIdentifier;
}
/**
*
* An attribute value of type EntityIdentifier.
*
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
*
*
* @param entityIdentifier
* An attribute value of type EntityIdentifier.
*
* Example: "entityIdentifier": { "entityId": "<id>", "entityType": "<entity type>"}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withEntityIdentifier(EntityIdentifier entityIdentifier) {
setEntityIdentifier(entityIdentifier);
return this;
}
/**
*
* An attribute value of Long type.
*
*
* Example: {"long": 0}
*
*
* @param longValue
* An attribute value of Long
* type.
*
* Example: {"long": 0}
*/
public void setLong(Long longValue) {
this.longValue = longValue;
}
/**
*
* An attribute value of Long type.
*
*
* Example: {"long": 0}
*
*
* @return An attribute value of Long
* type.
*
* Example: {"long": 0}
*/
public Long getLong() {
return this.longValue;
}
/**
*
* An attribute value of Long type.
*
*
* Example: {"long": 0}
*
*
* @param longValue
* An attribute value of Long
* type.
*
* Example: {"long": 0}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withLong(Long longValue) {
setLong(longValue);
return this;
}
/**
*
* An attribute value of String
* type.
*
*
* Example: {"string": "abc"}
*
*
* @param string
* An attribute value of String type.
*
* Example: {"string": "abc"}
*/
public void setString(String string) {
this.string = string;
}
/**
*
* An attribute value of String
* type.
*
*
* Example: {"string": "abc"}
*
*
* @return An attribute value of String type.
*
* Example: {"string": "abc"}
*/
public String getString() {
return this.string;
}
/**
*
* An attribute value of String
* type.
*
*
* Example: {"string": "abc"}
*
*
* @param string
* An attribute value of String type.
*
* Example: {"string": "abc"}
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withString(String string) {
setString(string);
return this;
}
/**
*
* An attribute value of Set type.
*
*
* Example: {"set": [ {} ] }
*
*
* @return An attribute value of Set
* type.
*
* Example: {"set": [ {} ] }
*/
public java.util.List getSet() {
return set;
}
/**
*
* An attribute value of Set type.
*
*
* Example: {"set": [ {} ] }
*
*
* @param set
* An attribute value of Set
* type.
*
* Example: {"set": [ {} ] }
*/
public void setSet(java.util.Collection set) {
if (set == null) {
this.set = null;
return;
}
this.set = new java.util.ArrayList(set);
}
/**
*
* An attribute value of Set type.
*
*
* Example: {"set": [ {} ] }
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSet(java.util.Collection)} or {@link #withSet(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param set
* An attribute value of Set
* type.
*
* Example: {"set": [ {} ] }
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withSet(AttributeValue... set) {
if (this.set == null) {
setSet(new java.util.ArrayList(set.length));
}
for (AttributeValue ele : set) {
this.set.add(ele);
}
return this;
}
/**
*
* An attribute value of Set type.
*
*
* Example: {"set": [ {} ] }
*
*
* @param set
* An attribute value of Set
* type.
*
* Example: {"set": [ {} ] }
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withSet(java.util.Collection set) {
setSet(set);
return this;
}
/**
*
* An attribute value of Record
* type.
*
*
* Example: {"record": { "keyName": {} } }
*
*
* @return An attribute value of Record type.
*
* Example: {"record": { "keyName": {} } }
*/
public java.util.Map getRecord() {
return record;
}
/**
*
* An attribute value of Record
* type.
*
*
* Example: {"record": { "keyName": {} } }
*
*
* @param record
* An attribute value of Record type.
*
* Example: {"record": { "keyName": {} } }
*/
public void setRecord(java.util.Map record) {
this.record = record;
}
/**
*
* An attribute value of Record
* type.
*
*
* Example: {"record": { "keyName": {} } }
*
*
* @param record
* An attribute value of Record type.
*
* Example: {"record": { "keyName": {} } }
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue withRecord(java.util.Map record) {
setRecord(record);
return this;
}
/**
* Add a single Record entry
*
* @see AttributeValue#withRecord
* @returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue addRecordEntry(String key, AttributeValue value) {
if (null == this.record) {
this.record = new java.util.HashMap();
}
if (this.record.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.record.put(key, value);
return this;
}
/**
* Removes all the entries added into Record.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AttributeValue clearRecordEntries() {
this.record = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBoolean() != null)
sb.append("Boolean: ").append("***Sensitive Data Redacted***").append(",");
if (getEntityIdentifier() != null)
sb.append("EntityIdentifier: ").append(getEntityIdentifier()).append(",");
if (getLong() != null)
sb.append("Long: ").append("***Sensitive Data Redacted***").append(",");
if (getString() != null)
sb.append("String: ").append("***Sensitive Data Redacted***").append(",");
if (getSet() != null)
sb.append("Set: ").append(getSet()).append(",");
if (getRecord() != null)
sb.append("Record: ").append(getRecord());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AttributeValue == false)
return false;
AttributeValue other = (AttributeValue) obj;
if (other.getBoolean() == null ^ this.getBoolean() == null)
return false;
if (other.getBoolean() != null && other.getBoolean().equals(this.getBoolean()) == false)
return false;
if (other.getEntityIdentifier() == null ^ this.getEntityIdentifier() == null)
return false;
if (other.getEntityIdentifier() != null && other.getEntityIdentifier().equals(this.getEntityIdentifier()) == false)
return false;
if (other.getLong() == null ^ this.getLong() == null)
return false;
if (other.getLong() != null && other.getLong().equals(this.getLong()) == false)
return false;
if (other.getString() == null ^ this.getString() == null)
return false;
if (other.getString() != null && other.getString().equals(this.getString()) == false)
return false;
if (other.getSet() == null ^ this.getSet() == null)
return false;
if (other.getSet() != null && other.getSet().equals(this.getSet()) == false)
return false;
if (other.getRecord() == null ^ this.getRecord() == null)
return false;
if (other.getRecord() != null && other.getRecord().equals(this.getRecord()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBoolean() == null) ? 0 : getBoolean().hashCode());
hashCode = prime * hashCode + ((getEntityIdentifier() == null) ? 0 : getEntityIdentifier().hashCode());
hashCode = prime * hashCode + ((getLong() == null) ? 0 : getLong().hashCode());
hashCode = prime * hashCode + ((getString() == null) ? 0 : getString().hashCode());
hashCode = prime * hashCode + ((getSet() == null) ? 0 : getSet().hashCode());
hashCode = prime * hashCode + ((getRecord() == null) ? 0 : getRecord().hashCode());
return hashCode;
}
@Override
public AttributeValue clone() {
try {
return (AttributeValue) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.verifiedpermissions.model.transform.AttributeValueMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}