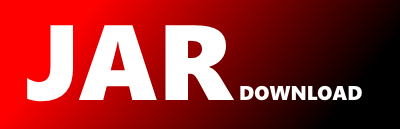
com.amazonaws.services.verifiedpermissions.model.OpenIdConnectConfigurationItem Maven / Gradle / Ivy
Show all versions of aws-java-sdk-verifiedpermissions Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.verifiedpermissions.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains configuration details of an OpenID Connect (OIDC) identity provider, or identity source, that Verified
* Permissions can use to generate entities from authenticated identities. It specifies the issuer URL, token type that
* you want to use, and policy store entity details.
*
*
* This data type is part of a ConfigurationItem structure, which is a parameter to ListIdentitySources.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OpenIdConnectConfigurationItem implements Serializable, Cloneable, StructuredPojo {
/**
*
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*
*/
private String issuer;
/**
*
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For example, if
* you set an entityIdPrefix
of MyOIDCProvider
, you can reference principals in your
* policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*
*/
private String entityIdPrefix;
/**
*
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type that you
* want to map it to. For example, this object can map the contents of a groups
claim to
* MyCorp::UserGroup
.
*
*/
private OpenIdConnectGroupConfigurationItem groupConfiguration;
/**
*
* The token type that you want to process from your OIDC identity provider. Your policy store can process either
* identity (ID) or access tokens from a given OIDC identity source.
*
*/
private OpenIdConnectTokenSelectionItem tokenSelection;
/**
*
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*
*
* @param issuer
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*/
public void setIssuer(String issuer) {
this.issuer = issuer;
}
/**
*
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*
*
* @return The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*/
public String getIssuer() {
return this.issuer;
}
/**
*
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
*
*
* @param issuer
* The issuer URL of an OIDC identity provider. This URL must have an OIDC discovery endpoint at the path
* .well-known/openid-configuration
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpenIdConnectConfigurationItem withIssuer(String issuer) {
setIssuer(issuer);
return this;
}
/**
*
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For example, if
* you set an entityIdPrefix
of MyOIDCProvider
, you can reference principals in your
* policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*
*
* @param entityIdPrefix
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For
* example, if you set an entityIdPrefix
of MyOIDCProvider
, you can reference
* principals in your policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*/
public void setEntityIdPrefix(String entityIdPrefix) {
this.entityIdPrefix = entityIdPrefix;
}
/**
*
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For example, if
* you set an entityIdPrefix
of MyOIDCProvider
, you can reference principals in your
* policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*
*
* @return A descriptive string that you want to prefix to user entities from your OIDC identity provider. For
* example, if you set an entityIdPrefix
of MyOIDCProvider
, you can reference
* principals in your policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*/
public String getEntityIdPrefix() {
return this.entityIdPrefix;
}
/**
*
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For example, if
* you set an entityIdPrefix
of MyOIDCProvider
, you can reference principals in your
* policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
*
*
* @param entityIdPrefix
* A descriptive string that you want to prefix to user entities from your OIDC identity provider. For
* example, if you set an entityIdPrefix
of MyOIDCProvider
, you can reference
* principals in your policies in the format MyCorp::User::MyOIDCProvider|Carlos
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpenIdConnectConfigurationItem withEntityIdPrefix(String entityIdPrefix) {
setEntityIdPrefix(entityIdPrefix);
return this;
}
/**
*
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type that you
* want to map it to. For example, this object can map the contents of a groups
claim to
* MyCorp::UserGroup
.
*
*
* @param groupConfiguration
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type
* that you want to map it to. For example, this object can map the contents of a groups
claim
* to MyCorp::UserGroup
.
*/
public void setGroupConfiguration(OpenIdConnectGroupConfigurationItem groupConfiguration) {
this.groupConfiguration = groupConfiguration;
}
/**
*
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type that you
* want to map it to. For example, this object can map the contents of a groups
claim to
* MyCorp::UserGroup
.
*
*
* @return The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type
* that you want to map it to. For example, this object can map the contents of a groups
claim
* to MyCorp::UserGroup
.
*/
public OpenIdConnectGroupConfigurationItem getGroupConfiguration() {
return this.groupConfiguration;
}
/**
*
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type that you
* want to map it to. For example, this object can map the contents of a groups
claim to
* MyCorp::UserGroup
.
*
*
* @param groupConfiguration
* The claim in OIDC identity provider tokens that indicates a user's group membership, and the entity type
* that you want to map it to. For example, this object can map the contents of a groups
claim
* to MyCorp::UserGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpenIdConnectConfigurationItem withGroupConfiguration(OpenIdConnectGroupConfigurationItem groupConfiguration) {
setGroupConfiguration(groupConfiguration);
return this;
}
/**
*
* The token type that you want to process from your OIDC identity provider. Your policy store can process either
* identity (ID) or access tokens from a given OIDC identity source.
*
*
* @param tokenSelection
* The token type that you want to process from your OIDC identity provider. Your policy store can process
* either identity (ID) or access tokens from a given OIDC identity source.
*/
public void setTokenSelection(OpenIdConnectTokenSelectionItem tokenSelection) {
this.tokenSelection = tokenSelection;
}
/**
*
* The token type that you want to process from your OIDC identity provider. Your policy store can process either
* identity (ID) or access tokens from a given OIDC identity source.
*
*
* @return The token type that you want to process from your OIDC identity provider. Your policy store can process
* either identity (ID) or access tokens from a given OIDC identity source.
*/
public OpenIdConnectTokenSelectionItem getTokenSelection() {
return this.tokenSelection;
}
/**
*
* The token type that you want to process from your OIDC identity provider. Your policy store can process either
* identity (ID) or access tokens from a given OIDC identity source.
*
*
* @param tokenSelection
* The token type that you want to process from your OIDC identity provider. Your policy store can process
* either identity (ID) or access tokens from a given OIDC identity source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OpenIdConnectConfigurationItem withTokenSelection(OpenIdConnectTokenSelectionItem tokenSelection) {
setTokenSelection(tokenSelection);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIssuer() != null)
sb.append("Issuer: ").append(getIssuer()).append(",");
if (getEntityIdPrefix() != null)
sb.append("EntityIdPrefix: ").append("***Sensitive Data Redacted***").append(",");
if (getGroupConfiguration() != null)
sb.append("GroupConfiguration: ").append(getGroupConfiguration()).append(",");
if (getTokenSelection() != null)
sb.append("TokenSelection: ").append(getTokenSelection());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OpenIdConnectConfigurationItem == false)
return false;
OpenIdConnectConfigurationItem other = (OpenIdConnectConfigurationItem) obj;
if (other.getIssuer() == null ^ this.getIssuer() == null)
return false;
if (other.getIssuer() != null && other.getIssuer().equals(this.getIssuer()) == false)
return false;
if (other.getEntityIdPrefix() == null ^ this.getEntityIdPrefix() == null)
return false;
if (other.getEntityIdPrefix() != null && other.getEntityIdPrefix().equals(this.getEntityIdPrefix()) == false)
return false;
if (other.getGroupConfiguration() == null ^ this.getGroupConfiguration() == null)
return false;
if (other.getGroupConfiguration() != null && other.getGroupConfiguration().equals(this.getGroupConfiguration()) == false)
return false;
if (other.getTokenSelection() == null ^ this.getTokenSelection() == null)
return false;
if (other.getTokenSelection() != null && other.getTokenSelection().equals(this.getTokenSelection()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIssuer() == null) ? 0 : getIssuer().hashCode());
hashCode = prime * hashCode + ((getEntityIdPrefix() == null) ? 0 : getEntityIdPrefix().hashCode());
hashCode = prime * hashCode + ((getGroupConfiguration() == null) ? 0 : getGroupConfiguration().hashCode());
hashCode = prime * hashCode + ((getTokenSelection() == null) ? 0 : getTokenSelection().hashCode());
return hashCode;
}
@Override
public OpenIdConnectConfigurationItem clone() {
try {
return (OpenIdConnectConfigurationItem) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.verifiedpermissions.model.transform.OpenIdConnectConfigurationItemMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}