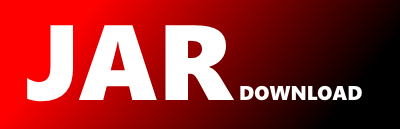
com.amazonaws.services.voiceid.AmazonVoiceIDAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-voiceid Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.voiceid;
import javax.annotation.Generated;
import com.amazonaws.services.voiceid.model.*;
/**
* Interface for accessing Amazon Voice ID asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.voiceid.AbstractAmazonVoiceIDAsync} instead.
*
*
*
* Amazon Connect Voice ID provides real-time caller authentication and fraud screening. This guide describes the APIs
* used for this service.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonVoiceIDAsync extends AmazonVoiceID {
/**
*
* Creates a domain that contains all Amazon Connect Voice ID data, such as speakers, fraudsters, customer audio,
* and voiceprints.
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonVoiceIDAsync.CreateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest);
/**
*
* Creates a domain that contains all Amazon Connect Voice ID data, such as speakers, fraudsters, customer audio,
* and voiceprints.
*
*
* @param createDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.CreateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDomainAsync(CreateDomainRequest createDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified domain from Voice ID.
*
*
* @param deleteDomainRequest
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonVoiceIDAsync.DeleteDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes the specified domain from Voice ID.
*
*
* @param deleteDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DeleteDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDomainAsync(DeleteDomainRequest deleteDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified fraudster from Voice ID.
*
*
* @param deleteFraudsterRequest
* @return A Java Future containing the result of the DeleteFraudster operation returned by the service.
* @sample AmazonVoiceIDAsync.DeleteFraudster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFraudsterAsync(DeleteFraudsterRequest deleteFraudsterRequest);
/**
*
* Deletes the specified fraudster from Voice ID.
*
*
* @param deleteFraudsterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFraudster operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DeleteFraudster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteFraudsterAsync(DeleteFraudsterRequest deleteFraudsterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified speaker from Voice ID.
*
*
* @param deleteSpeakerRequest
* @return A Java Future containing the result of the DeleteSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsync.DeleteSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSpeakerAsync(DeleteSpeakerRequest deleteSpeakerRequest);
/**
*
* Deletes the specified speaker from Voice ID.
*
*
* @param deleteSpeakerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DeleteSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteSpeakerAsync(DeleteSpeakerRequest deleteSpeakerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified domain.
*
*
* @param describeDomainRequest
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* @sample AmazonVoiceIDAsync.DescribeDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDomainAsync(DescribeDomainRequest describeDomainRequest);
/**
*
* Describes the specified domain.
*
*
* @param describeDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DescribeDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDomainAsync(DescribeDomainRequest describeDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified fraudster.
*
*
* @param describeFraudsterRequest
* @return A Java Future containing the result of the DescribeFraudster operation returned by the service.
* @sample AmazonVoiceIDAsync.DescribeFraudster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFraudsterAsync(DescribeFraudsterRequest describeFraudsterRequest);
/**
*
* Describes the specified fraudster.
*
*
* @param describeFraudsterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFraudster operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DescribeFraudster
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeFraudsterAsync(DescribeFraudsterRequest describeFraudsterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified fraudster registration job.
*
*
* @param describeFraudsterRegistrationJobRequest
* @return A Java Future containing the result of the DescribeFraudsterRegistrationJob operation returned by the
* service.
* @sample AmazonVoiceIDAsync.DescribeFraudsterRegistrationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFraudsterRegistrationJobAsync(
DescribeFraudsterRegistrationJobRequest describeFraudsterRegistrationJobRequest);
/**
*
* Describes the specified fraudster registration job.
*
*
* @param describeFraudsterRegistrationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFraudsterRegistrationJob operation returned by the
* service.
* @sample AmazonVoiceIDAsyncHandler.DescribeFraudsterRegistrationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFraudsterRegistrationJobAsync(
DescribeFraudsterRegistrationJobRequest describeFraudsterRegistrationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified speaker.
*
*
* @param describeSpeakerRequest
* @return A Java Future containing the result of the DescribeSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsync.DescribeSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSpeakerAsync(DescribeSpeakerRequest describeSpeakerRequest);
/**
*
* Describes the specified speaker.
*
*
* @param describeSpeakerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.DescribeSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSpeakerAsync(DescribeSpeakerRequest describeSpeakerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified speaker enrollment job.
*
*
* @param describeSpeakerEnrollmentJobRequest
* @return A Java Future containing the result of the DescribeSpeakerEnrollmentJob operation returned by the
* service.
* @sample AmazonVoiceIDAsync.DescribeSpeakerEnrollmentJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSpeakerEnrollmentJobAsync(
DescribeSpeakerEnrollmentJobRequest describeSpeakerEnrollmentJobRequest);
/**
*
* Describes the specified speaker enrollment job.
*
*
* @param describeSpeakerEnrollmentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSpeakerEnrollmentJob operation returned by the
* service.
* @sample AmazonVoiceIDAsyncHandler.DescribeSpeakerEnrollmentJob
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSpeakerEnrollmentJobAsync(
DescribeSpeakerEnrollmentJobRequest describeSpeakerEnrollmentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Evaluates a specified session based on audio data accumulated during a streaming Amazon Connect Voice ID call.
*
*
* @param evaluateSessionRequest
* @return A Java Future containing the result of the EvaluateSession operation returned by the service.
* @sample AmazonVoiceIDAsync.EvaluateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future evaluateSessionAsync(EvaluateSessionRequest evaluateSessionRequest);
/**
*
* Evaluates a specified session based on audio data accumulated during a streaming Amazon Connect Voice ID call.
*
*
* @param evaluateSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EvaluateSession operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.EvaluateSession
* @see AWS API
* Documentation
*/
java.util.concurrent.Future evaluateSessionAsync(EvaluateSessionRequest evaluateSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the domains in the Amazon Web Services account.
*
*
* @param listDomainsRequest
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonVoiceIDAsync.ListDomains
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest);
/**
*
* Lists all the domains in the Amazon Web Services account.
*
*
* @param listDomainsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListDomains operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.ListDomains
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listDomainsAsync(ListDomainsRequest listDomainsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the fraudster registration jobs in the domain with the given JobStatus
. If
* JobStatus
is not provided, this lists all fraudster registration jobs in the given domain.
*
*
* @param listFraudsterRegistrationJobsRequest
* @return A Java Future containing the result of the ListFraudsterRegistrationJobs operation returned by the
* service.
* @sample AmazonVoiceIDAsync.ListFraudsterRegistrationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listFraudsterRegistrationJobsAsync(
ListFraudsterRegistrationJobsRequest listFraudsterRegistrationJobsRequest);
/**
*
* Lists all the fraudster registration jobs in the domain with the given JobStatus
. If
* JobStatus
is not provided, this lists all fraudster registration jobs in the given domain.
*
*
* @param listFraudsterRegistrationJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListFraudsterRegistrationJobs operation returned by the
* service.
* @sample AmazonVoiceIDAsyncHandler.ListFraudsterRegistrationJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listFraudsterRegistrationJobsAsync(
ListFraudsterRegistrationJobsRequest listFraudsterRegistrationJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the speaker enrollment jobs in the domain with the specified JobStatus
. If
* JobStatus
is not provided, this lists all jobs with all possible speaker enrollment job statuses.
*
*
* @param listSpeakerEnrollmentJobsRequest
* @return A Java Future containing the result of the ListSpeakerEnrollmentJobs operation returned by the service.
* @sample AmazonVoiceIDAsync.ListSpeakerEnrollmentJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listSpeakerEnrollmentJobsAsync(
ListSpeakerEnrollmentJobsRequest listSpeakerEnrollmentJobsRequest);
/**
*
* Lists all the speaker enrollment jobs in the domain with the specified JobStatus
. If
* JobStatus
is not provided, this lists all jobs with all possible speaker enrollment job statuses.
*
*
* @param listSpeakerEnrollmentJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSpeakerEnrollmentJobs operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.ListSpeakerEnrollmentJobs
* @see AWS API Documentation
*/
java.util.concurrent.Future listSpeakerEnrollmentJobsAsync(
ListSpeakerEnrollmentJobsRequest listSpeakerEnrollmentJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all speakers in a specified domain.
*
*
* @param listSpeakersRequest
* @return A Java Future containing the result of the ListSpeakers operation returned by the service.
* @sample AmazonVoiceIDAsync.ListSpeakers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSpeakersAsync(ListSpeakersRequest listSpeakersRequest);
/**
*
* Lists all speakers in a specified domain.
*
*
* @param listSpeakersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListSpeakers operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.ListSpeakers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listSpeakersAsync(ListSpeakersRequest listSpeakersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags associated with a specified Voice ID resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonVoiceIDAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags associated with a specified Voice ID resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Opts out a speaker from Voice ID. A speaker can be opted out regardless of whether or not they already exist in
* Voice ID. If they don't yet exist, a new speaker is created in an opted out state. If they already exist, their
* existing status is overridden and they are opted out. Enrollment and evaluation authentication requests are
* rejected for opted out speakers, and opted out speakers have no voice embeddings stored in Voice ID.
*
*
* @param optOutSpeakerRequest
* @return A Java Future containing the result of the OptOutSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsync.OptOutSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future optOutSpeakerAsync(OptOutSpeakerRequest optOutSpeakerRequest);
/**
*
* Opts out a speaker from Voice ID. A speaker can be opted out regardless of whether or not they already exist in
* Voice ID. If they don't yet exist, a new speaker is created in an opted out state. If they already exist, their
* existing status is overridden and they are opted out. Enrollment and evaluation authentication requests are
* rejected for opted out speakers, and opted out speakers have no voice embeddings stored in Voice ID.
*
*
* @param optOutSpeakerRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the OptOutSpeaker operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.OptOutSpeaker
* @see AWS API
* Documentation
*/
java.util.concurrent.Future optOutSpeakerAsync(OptOutSpeakerRequest optOutSpeakerRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a new batch fraudster registration job using provided details.
*
*
* @param startFraudsterRegistrationJobRequest
* @return A Java Future containing the result of the StartFraudsterRegistrationJob operation returned by the
* service.
* @sample AmazonVoiceIDAsync.StartFraudsterRegistrationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startFraudsterRegistrationJobAsync(
StartFraudsterRegistrationJobRequest startFraudsterRegistrationJobRequest);
/**
*
* Starts a new batch fraudster registration job using provided details.
*
*
* @param startFraudsterRegistrationJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartFraudsterRegistrationJob operation returned by the
* service.
* @sample AmazonVoiceIDAsyncHandler.StartFraudsterRegistrationJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startFraudsterRegistrationJobAsync(
StartFraudsterRegistrationJobRequest startFraudsterRegistrationJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a new batch speaker enrollment job using specified details.
*
*
* @param startSpeakerEnrollmentJobRequest
* @return A Java Future containing the result of the StartSpeakerEnrollmentJob operation returned by the service.
* @sample AmazonVoiceIDAsync.StartSpeakerEnrollmentJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startSpeakerEnrollmentJobAsync(
StartSpeakerEnrollmentJobRequest startSpeakerEnrollmentJobRequest);
/**
*
* Starts a new batch speaker enrollment job using specified details.
*
*
* @param startSpeakerEnrollmentJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSpeakerEnrollmentJob operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.StartSpeakerEnrollmentJob
* @see AWS API Documentation
*/
java.util.concurrent.Future startSpeakerEnrollmentJobAsync(
StartSpeakerEnrollmentJobRequest startSpeakerEnrollmentJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tags a Voice ID resource with the provided list of tags.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonVoiceIDAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Tags a Voice ID resource with the provided list of tags.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes specified tags from a specified Amazon Connect Voice ID resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonVoiceIDAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes specified tags from a specified Amazon Connect Voice ID resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the specified domain. This API has clobber behavior, and clears and replaces all attributes. If an
* optional field, such as 'Description' is not provided, it is removed from the domain.
*
*
* @param updateDomainRequest
* @return A Java Future containing the result of the UpdateDomain operation returned by the service.
* @sample AmazonVoiceIDAsync.UpdateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDomainAsync(UpdateDomainRequest updateDomainRequest);
/**
*
* Updates the specified domain. This API has clobber behavior, and clears and replaces all attributes. If an
* optional field, such as 'Description' is not provided, it is removed from the domain.
*
*
* @param updateDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateDomain operation returned by the service.
* @sample AmazonVoiceIDAsyncHandler.UpdateDomain
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateDomainAsync(UpdateDomainRequest updateDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}