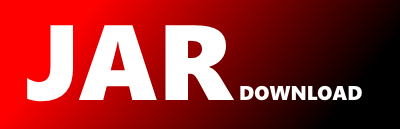
com.amazonaws.services.voiceid.model.EvaluateSessionResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-voiceid Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.voiceid.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EvaluateSessionResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* Details resulting from the authentication process, such as authentication decision and authentication score.
*
*/
private AuthenticationResult authenticationResult;
/**
*
* The identifier of the domain that contains the session.
*
*/
private String domainId;
/**
*
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*
*/
private FraudDetectionResult fraudDetectionResult;
/**
*
* The service-generated identifier of the session.
*
*/
private String sessionId;
/**
*
* The client-provided name of the session.
*
*/
private String sessionName;
/**
*
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In this
* situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can mean that
* the client should call the API again later, after Voice ID has enough audio to produce a result. If the decision
* remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is ENDED
, it means
* that the previously streamed session did not have enough speech to perform evaluation, and a new streaming
* session is needed to try again.
*
*/
private String streamingStatus;
/**
*
* Details resulting from the authentication process, such as authentication decision and authentication score.
*
*
* @param authenticationResult
* Details resulting from the authentication process, such as authentication decision and authentication
* score.
*/
public void setAuthenticationResult(AuthenticationResult authenticationResult) {
this.authenticationResult = authenticationResult;
}
/**
*
* Details resulting from the authentication process, such as authentication decision and authentication score.
*
*
* @return Details resulting from the authentication process, such as authentication decision and authentication
* score.
*/
public AuthenticationResult getAuthenticationResult() {
return this.authenticationResult;
}
/**
*
* Details resulting from the authentication process, such as authentication decision and authentication score.
*
*
* @param authenticationResult
* Details resulting from the authentication process, such as authentication decision and authentication
* score.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EvaluateSessionResult withAuthenticationResult(AuthenticationResult authenticationResult) {
setAuthenticationResult(authenticationResult);
return this;
}
/**
*
* The identifier of the domain that contains the session.
*
*
* @param domainId
* The identifier of the domain that contains the session.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The identifier of the domain that contains the session.
*
*
* @return The identifier of the domain that contains the session.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The identifier of the domain that contains the session.
*
*
* @param domainId
* The identifier of the domain that contains the session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EvaluateSessionResult withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*
*
* @param fraudDetectionResult
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*/
public void setFraudDetectionResult(FraudDetectionResult fraudDetectionResult) {
this.fraudDetectionResult = fraudDetectionResult;
}
/**
*
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*
*
* @return Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*/
public FraudDetectionResult getFraudDetectionResult() {
return this.fraudDetectionResult;
}
/**
*
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
*
*
* @param fraudDetectionResult
* Details resulting from the fraud detection process, such as fraud detection decision and risk score.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EvaluateSessionResult withFraudDetectionResult(FraudDetectionResult fraudDetectionResult) {
setFraudDetectionResult(fraudDetectionResult);
return this;
}
/**
*
* The service-generated identifier of the session.
*
*
* @param sessionId
* The service-generated identifier of the session.
*/
public void setSessionId(String sessionId) {
this.sessionId = sessionId;
}
/**
*
* The service-generated identifier of the session.
*
*
* @return The service-generated identifier of the session.
*/
public String getSessionId() {
return this.sessionId;
}
/**
*
* The service-generated identifier of the session.
*
*
* @param sessionId
* The service-generated identifier of the session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EvaluateSessionResult withSessionId(String sessionId) {
setSessionId(sessionId);
return this;
}
/**
*
* The client-provided name of the session.
*
*
* @param sessionName
* The client-provided name of the session.
*/
public void setSessionName(String sessionName) {
this.sessionName = sessionName;
}
/**
*
* The client-provided name of the session.
*
*
* @return The client-provided name of the session.
*/
public String getSessionName() {
return this.sessionName;
}
/**
*
* The client-provided name of the session.
*
*
* @param sessionName
* The client-provided name of the session.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EvaluateSessionResult withSessionName(String sessionName) {
setSessionName(sessionName);
return this;
}
/**
*
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In this
* situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can mean that
* the client should call the API again later, after Voice ID has enough audio to produce a result. If the decision
* remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is ENDED
, it means
* that the previously streamed session did not have enough speech to perform evaluation, and a new streaming
* session is needed to try again.
*
*
* @param streamingStatus
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In
* this situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can
* mean that the client should call the API again later, after Voice ID has enough audio to produce a result.
* If the decision remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is
* ENDED
, it means that the previously streamed session did not have enough speech to perform
* evaluation, and a new streaming session is needed to try again.
* @see StreamingStatus
*/
public void setStreamingStatus(String streamingStatus) {
this.streamingStatus = streamingStatus;
}
/**
*
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In this
* situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can mean that
* the client should call the API again later, after Voice ID has enough audio to produce a result. If the decision
* remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is ENDED
, it means
* that the previously streamed session did not have enough speech to perform evaluation, and a new streaming
* session is needed to try again.
*
*
* @return The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In
* this situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can
* mean that the client should call the API again later, after Voice ID has enough audio to produce a
* result. If the decision remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is
* ENDED
, it means that the previously streamed session did not have enough speech to perform
* evaluation, and a new streaming session is needed to try again.
* @see StreamingStatus
*/
public String getStreamingStatus() {
return this.streamingStatus;
}
/**
*
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In this
* situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can mean that
* the client should call the API again later, after Voice ID has enough audio to produce a result. If the decision
* remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is ENDED
, it means
* that the previously streamed session did not have enough speech to perform evaluation, and a new streaming
* session is needed to try again.
*
*
* @param streamingStatus
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In
* this situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can
* mean that the client should call the API again later, after Voice ID has enough audio to produce a result.
* If the decision remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is
* ENDED
, it means that the previously streamed session did not have enough speech to perform
* evaluation, and a new streaming session is needed to try again.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StreamingStatus
*/
public EvaluateSessionResult withStreamingStatus(String streamingStatus) {
setStreamingStatus(streamingStatus);
return this;
}
/**
*
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In this
* situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can mean that
* the client should call the API again later, after Voice ID has enough audio to produce a result. If the decision
* remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is ENDED
, it means
* that the previously streamed session did not have enough speech to perform evaluation, and a new streaming
* session is needed to try again.
*
*
* @param streamingStatus
* The current status of audio streaming for this session. This field is useful to infer next steps when the
* Authentication or Fraud Detection results are empty or the decision is NOT_ENOUGH_SPEECH
. In
* this situation, if the StreamingStatus
is ONGOING/PENDING_CONFIGURATION
, it can
* mean that the client should call the API again later, after Voice ID has enough audio to produce a result.
* If the decision remains NOT_ENOUGH_SPEECH
even after StreamingStatus
is
* ENDED
, it means that the previously streamed session did not have enough speech to perform
* evaluation, and a new streaming session is needed to try again.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StreamingStatus
*/
public EvaluateSessionResult withStreamingStatus(StreamingStatus streamingStatus) {
this.streamingStatus = streamingStatus.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAuthenticationResult() != null)
sb.append("AuthenticationResult: ").append(getAuthenticationResult()).append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getFraudDetectionResult() != null)
sb.append("FraudDetectionResult: ").append(getFraudDetectionResult()).append(",");
if (getSessionId() != null)
sb.append("SessionId: ").append(getSessionId()).append(",");
if (getSessionName() != null)
sb.append("SessionName: ").append(getSessionName()).append(",");
if (getStreamingStatus() != null)
sb.append("StreamingStatus: ").append(getStreamingStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EvaluateSessionResult == false)
return false;
EvaluateSessionResult other = (EvaluateSessionResult) obj;
if (other.getAuthenticationResult() == null ^ this.getAuthenticationResult() == null)
return false;
if (other.getAuthenticationResult() != null && other.getAuthenticationResult().equals(this.getAuthenticationResult()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getFraudDetectionResult() == null ^ this.getFraudDetectionResult() == null)
return false;
if (other.getFraudDetectionResult() != null && other.getFraudDetectionResult().equals(this.getFraudDetectionResult()) == false)
return false;
if (other.getSessionId() == null ^ this.getSessionId() == null)
return false;
if (other.getSessionId() != null && other.getSessionId().equals(this.getSessionId()) == false)
return false;
if (other.getSessionName() == null ^ this.getSessionName() == null)
return false;
if (other.getSessionName() != null && other.getSessionName().equals(this.getSessionName()) == false)
return false;
if (other.getStreamingStatus() == null ^ this.getStreamingStatus() == null)
return false;
if (other.getStreamingStatus() != null && other.getStreamingStatus().equals(this.getStreamingStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAuthenticationResult() == null) ? 0 : getAuthenticationResult().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getFraudDetectionResult() == null) ? 0 : getFraudDetectionResult().hashCode());
hashCode = prime * hashCode + ((getSessionId() == null) ? 0 : getSessionId().hashCode());
hashCode = prime * hashCode + ((getSessionName() == null) ? 0 : getSessionName().hashCode());
hashCode = prime * hashCode + ((getStreamingStatus() == null) ? 0 : getStreamingStatus().hashCode());
return hashCode;
}
@Override
public EvaluateSessionResult clone() {
try {
return (EvaluateSessionResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}