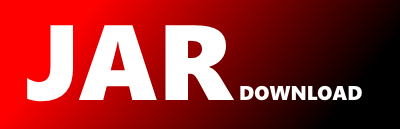
com.amazonaws.services.voiceid.AmazonVoiceID Maven / Gradle / Ivy
Show all versions of aws-java-sdk-voiceid Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.voiceid;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.voiceid.model.*;
/**
* Interface for accessing Amazon Voice ID.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.voiceid.AbstractAmazonVoiceID} instead.
*
*
*
* Amazon Connect Voice ID provides real-time caller authentication and fraud risk detection, which make voice
* interactions in contact centers more secure and efficient.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonVoiceID {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "voiceid";
/**
*
* Associates the fraudsters with the watchlist specified in the same domain.
*
*
* @param associateFraudsterRequest
* @return Result of the AssociateFraudster operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.AssociateFraudster
* @see AWS
* API Documentation
*/
AssociateFraudsterResult associateFraudster(AssociateFraudsterRequest associateFraudsterRequest);
/**
*
* Creates a domain that contains all Amazon Connect Voice ID data, such as speakers, fraudsters, customer audio,
* and voiceprints. Every domain is created with a default watchlist that fraudsters can be a part of.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.CreateDomain
* @see AWS API
* Documentation
*/
CreateDomainResult createDomain(CreateDomainRequest createDomainRequest);
/**
*
* Creates a watchlist that fraudsters can be a part of.
*
*
* @param createWatchlistRequest
* @return Result of the CreateWatchlist operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.CreateWatchlist
* @see AWS API
* Documentation
*/
CreateWatchlistResult createWatchlist(CreateWatchlistRequest createWatchlistRequest);
/**
*
* Deletes the specified domain from Voice ID.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteDomain
* @see AWS API
* Documentation
*/
DeleteDomainResult deleteDomain(DeleteDomainRequest deleteDomainRequest);
/**
*
* Deletes the specified fraudster from Voice ID. This action disassociates the fraudster from any watchlists it is
* a part of.
*
*
* @param deleteFraudsterRequest
* @return Result of the DeleteFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteFraudster
* @see AWS API
* Documentation
*/
DeleteFraudsterResult deleteFraudster(DeleteFraudsterRequest deleteFraudsterRequest);
/**
*
* Deletes the specified speaker from Voice ID.
*
*
* @param deleteSpeakerRequest
* @return Result of the DeleteSpeaker operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteSpeaker
* @see AWS API
* Documentation
*/
DeleteSpeakerResult deleteSpeaker(DeleteSpeakerRequest deleteSpeakerRequest);
/**
*
* Deletes the specified watchlist from Voice ID. This API throws an exception when there are fraudsters in the
* watchlist that you are trying to delete. You must delete the fraudsters, and then delete the watchlist. Every
* domain has a default watchlist which cannot be deleted.
*
*
* @param deleteWatchlistRequest
* @return Result of the DeleteWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteWatchlist
* @see AWS API
* Documentation
*/
DeleteWatchlistResult deleteWatchlist(DeleteWatchlistRequest deleteWatchlistRequest);
/**
*
* Describes the specified domain.
*
*
* @param describeDomainRequest
* @return Result of the DescribeDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeDomain
* @see AWS API
* Documentation
*/
DescribeDomainResult describeDomain(DescribeDomainRequest describeDomainRequest);
/**
*
* Describes the specified fraudster.
*
*
* @param describeFraudsterRequest
* @return Result of the DescribeFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeFraudster
* @see AWS API
* Documentation
*/
DescribeFraudsterResult describeFraudster(DescribeFraudsterRequest describeFraudsterRequest);
/**
*
* Describes the specified fraudster registration job.
*
*
* @param describeFraudsterRegistrationJobRequest
* @return Result of the DescribeFraudsterRegistrationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeFraudsterRegistrationJob
* @see AWS API Documentation
*/
DescribeFraudsterRegistrationJobResult describeFraudsterRegistrationJob(DescribeFraudsterRegistrationJobRequest describeFraudsterRegistrationJobRequest);
/**
*
* Describes the specified speaker.
*
*
* @param describeSpeakerRequest
* @return Result of the DescribeSpeaker operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeSpeaker
* @see AWS API
* Documentation
*/
DescribeSpeakerResult describeSpeaker(DescribeSpeakerRequest describeSpeakerRequest);
/**
*
* Describes the specified speaker enrollment job.
*
*
* @param describeSpeakerEnrollmentJobRequest
* @return Result of the DescribeSpeakerEnrollmentJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeSpeakerEnrollmentJob
* @see AWS API Documentation
*/
DescribeSpeakerEnrollmentJobResult describeSpeakerEnrollmentJob(DescribeSpeakerEnrollmentJobRequest describeSpeakerEnrollmentJobRequest);
/**
*
* Describes the specified watchlist.
*
*
* @param describeWatchlistRequest
* @return Result of the DescribeWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeWatchlist
* @see AWS API
* Documentation
*/
DescribeWatchlistResult describeWatchlist(DescribeWatchlistRequest describeWatchlistRequest);
/**
*
* Disassociates the fraudsters from the watchlist specified. Voice ID always expects a fraudster to be a part of at
* least one watchlist. If you try to disassociate a fraudster from its only watchlist, a
* ValidationException
is thrown.
*
*
* @param disassociateFraudsterRequest
* @return Result of the DisassociateFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DisassociateFraudster
* @see AWS
* API Documentation
*/
DisassociateFraudsterResult disassociateFraudster(DisassociateFraudsterRequest disassociateFraudsterRequest);
/**
*
* Evaluates a specified session based on audio data accumulated during a streaming Amazon Connect Voice ID call.
*
*
* @param evaluateSessionRequest
* @return Result of the EvaluateSession operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.EvaluateSession
* @see AWS API
* Documentation
*/
EvaluateSessionResult evaluateSession(EvaluateSessionRequest evaluateSessionRequest);
/**
*
* Lists all the domains in the Amazon Web Services account.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListDomains
* @see AWS API
* Documentation
*/
ListDomainsResult listDomains(ListDomainsRequest listDomainsRequest);
/**
*
* Lists all the fraudster registration jobs in the domain with the given JobStatus
. If
* JobStatus
is not provided, this lists all fraudster registration jobs in the given domain.
*
*
* @param listFraudsterRegistrationJobsRequest
* @return Result of the ListFraudsterRegistrationJobs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListFraudsterRegistrationJobs
* @see AWS API Documentation
*/
ListFraudsterRegistrationJobsResult listFraudsterRegistrationJobs(ListFraudsterRegistrationJobsRequest listFraudsterRegistrationJobsRequest);
/**
*
* Lists all fraudsters in a specified watchlist or domain.
*
*
* @param listFraudstersRequest
* @return Result of the ListFraudsters operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListFraudsters
* @see AWS API
* Documentation
*/
ListFraudstersResult listFraudsters(ListFraudstersRequest listFraudstersRequest);
/**
*
* Lists all the speaker enrollment jobs in the domain with the specified JobStatus
. If
* JobStatus
is not provided, this lists all jobs with all possible speaker enrollment job statuses.
*
*
* @param listSpeakerEnrollmentJobsRequest
* @return Result of the ListSpeakerEnrollmentJobs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListSpeakerEnrollmentJobs
* @see AWS API Documentation
*/
ListSpeakerEnrollmentJobsResult listSpeakerEnrollmentJobs(ListSpeakerEnrollmentJobsRequest listSpeakerEnrollmentJobsRequest);
/**
*
* Lists all speakers in a specified domain.
*
*
* @param listSpeakersRequest
* @return Result of the ListSpeakers operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListSpeakers
* @see AWS API
* Documentation
*/
ListSpeakersResult listSpeakers(ListSpeakersRequest listSpeakersRequest);
/**
*
* Lists all tags associated with a specified Voice ID resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListTagsForResource
* @see AWS
* API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all watchlists in a specified domain.
*
*
* @param listWatchlistsRequest
* @return Result of the ListWatchlists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListWatchlists
* @see AWS API
* Documentation
*/
ListWatchlistsResult listWatchlists(ListWatchlistsRequest listWatchlistsRequest);
/**
*
* Opts out a speaker from Voice ID. A speaker can be opted out regardless of whether or not they already exist in
* Voice ID. If they don't yet exist, a new speaker is created in an opted out state. If they already exist, their
* existing status is overridden and they are opted out. Enrollment and evaluation authentication requests are
* rejected for opted out speakers, and opted out speakers have no voice embeddings stored in Voice ID.
*
*
* @param optOutSpeakerRequest
* @return Result of the OptOutSpeaker operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.OptOutSpeaker
* @see AWS API
* Documentation
*/
OptOutSpeakerResult optOutSpeaker(OptOutSpeakerRequest optOutSpeakerRequest);
/**
*
* Starts a new batch fraudster registration job using provided details.
*
*
* @param startFraudsterRegistrationJobRequest
* @return Result of the StartFraudsterRegistrationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.StartFraudsterRegistrationJob
* @see AWS API Documentation
*/
StartFraudsterRegistrationJobResult startFraudsterRegistrationJob(StartFraudsterRegistrationJobRequest startFraudsterRegistrationJobRequest);
/**
*
* Starts a new batch speaker enrollment job using specified details.
*
*
* @param startSpeakerEnrollmentJobRequest
* @return Result of the StartSpeakerEnrollmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.StartSpeakerEnrollmentJob
* @see AWS API Documentation
*/
StartSpeakerEnrollmentJobResult startSpeakerEnrollmentJob(StartSpeakerEnrollmentJobRequest startSpeakerEnrollmentJobRequest);
/**
*
* Tags a Voice ID resource with the provided list of tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes specified tags from a specified Amazon Connect Voice ID resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the specified domain. This API has clobber behavior, and clears and replaces all attributes. If an
* optional field, such as 'Description' is not provided, it is removed from the domain.
*
*
* @param updateDomainRequest
* @return Result of the UpdateDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UpdateDomain
* @see AWS API
* Documentation
*/
UpdateDomainResult updateDomain(UpdateDomainRequest updateDomainRequest);
/**
*
* Updates the specified watchlist. Every domain has a default watchlist which cannot be updated.
*
*
* @param updateWatchlistRequest
* @return Result of the UpdateWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UpdateWatchlist
* @see AWS API
* Documentation
*/
UpdateWatchlistResult updateWatchlist(UpdateWatchlistRequest updateWatchlistRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}