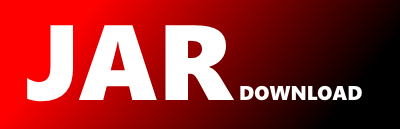
com.amazonaws.services.voiceid.AmazonVoiceIDClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-voiceid Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.voiceid;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.voiceid.AmazonVoiceIDClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.voiceid.model.*;
import com.amazonaws.services.voiceid.model.transform.*;
/**
* Client for accessing Amazon Voice ID. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
*
* Amazon Connect Voice ID provides real-time caller authentication and fraud risk detection, which make voice
* interactions in contact centers more secure and efficient.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonVoiceIDClient extends AmazonWebServiceClient implements AmazonVoiceID {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonVoiceID.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "voiceid";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.0")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceQuotaExceededException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.ServiceQuotaExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServerException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.InternalServerExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ValidationException").withExceptionUnmarshaller(
com.amazonaws.services.voiceid.model.transform.ValidationExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.voiceid.model.AmazonVoiceIDException.class));
public static AmazonVoiceIDClientBuilder builder() {
return AmazonVoiceIDClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Voice ID using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonVoiceIDClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Voice ID using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonVoiceIDClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("voiceid.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/voiceid/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/voiceid/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates the fraudsters with the watchlist specified in the same domain.
*
*
* @param associateFraudsterRequest
* @return Result of the AssociateFraudster operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.AssociateFraudster
* @see AWS
* API Documentation
*/
@Override
public AssociateFraudsterResult associateFraudster(AssociateFraudsterRequest request) {
request = beforeClientExecution(request);
return executeAssociateFraudster(request);
}
@SdkInternalApi
final AssociateFraudsterResult executeAssociateFraudster(AssociateFraudsterRequest associateFraudsterRequest) {
ExecutionContext executionContext = createExecutionContext(associateFraudsterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateFraudsterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(associateFraudsterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateFraudster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new AssociateFraudsterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a domain that contains all Amazon Connect Voice ID data, such as speakers, fraudsters, customer audio,
* and voiceprints. Every domain is created with a default watchlist that fraudsters can be a part of.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.CreateDomain
* @see AWS API
* Documentation
*/
@Override
public CreateDomainResult createDomain(CreateDomainRequest request) {
request = beforeClientExecution(request);
return executeCreateDomain(request);
}
@SdkInternalApi
final CreateDomainResult executeCreateDomain(CreateDomainRequest createDomainRequest) {
ExecutionContext executionContext = createExecutionContext(createDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a watchlist that fraudsters can be a part of.
*
*
* @param createWatchlistRequest
* @return Result of the CreateWatchlist operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.CreateWatchlist
* @see AWS API
* Documentation
*/
@Override
public CreateWatchlistResult createWatchlist(CreateWatchlistRequest request) {
request = beforeClientExecution(request);
return executeCreateWatchlist(request);
}
@SdkInternalApi
final CreateWatchlistResult executeCreateWatchlist(CreateWatchlistRequest createWatchlistRequest) {
ExecutionContext executionContext = createExecutionContext(createWatchlistRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWatchlistRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createWatchlistRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateWatchlist");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateWatchlistResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified domain from Voice ID.
*
*
* @param deleteDomainRequest
* @return Result of the DeleteDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteDomain
* @see AWS API
* Documentation
*/
@Override
public DeleteDomainResult deleteDomain(DeleteDomainRequest request) {
request = beforeClientExecution(request);
return executeDeleteDomain(request);
}
@SdkInternalApi
final DeleteDomainResult executeDeleteDomain(DeleteDomainRequest deleteDomainRequest) {
ExecutionContext executionContext = createExecutionContext(deleteDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified fraudster from Voice ID. This action disassociates the fraudster from any watchlists it is
* a part of.
*
*
* @param deleteFraudsterRequest
* @return Result of the DeleteFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteFraudster
* @see AWS API
* Documentation
*/
@Override
public DeleteFraudsterResult deleteFraudster(DeleteFraudsterRequest request) {
request = beforeClientExecution(request);
return executeDeleteFraudster(request);
}
@SdkInternalApi
final DeleteFraudsterResult executeDeleteFraudster(DeleteFraudsterRequest deleteFraudsterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteFraudsterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteFraudsterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteFraudsterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteFraudster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteFraudsterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified speaker from Voice ID.
*
*
* @param deleteSpeakerRequest
* @return Result of the DeleteSpeaker operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteSpeaker
* @see AWS API
* Documentation
*/
@Override
public DeleteSpeakerResult deleteSpeaker(DeleteSpeakerRequest request) {
request = beforeClientExecution(request);
return executeDeleteSpeaker(request);
}
@SdkInternalApi
final DeleteSpeakerResult executeDeleteSpeaker(DeleteSpeakerRequest deleteSpeakerRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSpeakerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSpeakerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSpeakerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSpeaker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSpeakerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified watchlist from Voice ID. This API throws an exception when there are fraudsters in the
* watchlist that you are trying to delete. You must delete the fraudsters, and then delete the watchlist. Every
* domain has a default watchlist which cannot be deleted.
*
*
* @param deleteWatchlistRequest
* @return Result of the DeleteWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DeleteWatchlist
* @see AWS API
* Documentation
*/
@Override
public DeleteWatchlistResult deleteWatchlist(DeleteWatchlistRequest request) {
request = beforeClientExecution(request);
return executeDeleteWatchlist(request);
}
@SdkInternalApi
final DeleteWatchlistResult executeDeleteWatchlist(DeleteWatchlistRequest deleteWatchlistRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWatchlistRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWatchlistRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteWatchlistRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteWatchlist");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteWatchlistResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified domain.
*
*
* @param describeDomainRequest
* @return Result of the DescribeDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeDomain
* @see AWS API
* Documentation
*/
@Override
public DescribeDomainResult describeDomain(DescribeDomainRequest request) {
request = beforeClientExecution(request);
return executeDescribeDomain(request);
}
@SdkInternalApi
final DescribeDomainResult executeDescribeDomain(DescribeDomainRequest describeDomainRequest) {
ExecutionContext executionContext = createExecutionContext(describeDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified fraudster.
*
*
* @param describeFraudsterRequest
* @return Result of the DescribeFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeFraudster
* @see AWS API
* Documentation
*/
@Override
public DescribeFraudsterResult describeFraudster(DescribeFraudsterRequest request) {
request = beforeClientExecution(request);
return executeDescribeFraudster(request);
}
@SdkInternalApi
final DescribeFraudsterResult executeDescribeFraudster(DescribeFraudsterRequest describeFraudsterRequest) {
ExecutionContext executionContext = createExecutionContext(describeFraudsterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFraudsterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeFraudsterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFraudster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeFraudsterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified fraudster registration job.
*
*
* @param describeFraudsterRegistrationJobRequest
* @return Result of the DescribeFraudsterRegistrationJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeFraudsterRegistrationJob
* @see AWS API Documentation
*/
@Override
public DescribeFraudsterRegistrationJobResult describeFraudsterRegistrationJob(DescribeFraudsterRegistrationJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeFraudsterRegistrationJob(request);
}
@SdkInternalApi
final DescribeFraudsterRegistrationJobResult executeDescribeFraudsterRegistrationJob(
DescribeFraudsterRegistrationJobRequest describeFraudsterRegistrationJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeFraudsterRegistrationJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeFraudsterRegistrationJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeFraudsterRegistrationJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeFraudsterRegistrationJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeFraudsterRegistrationJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified speaker.
*
*
* @param describeSpeakerRequest
* @return Result of the DescribeSpeaker operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeSpeaker
* @see AWS API
* Documentation
*/
@Override
public DescribeSpeakerResult describeSpeaker(DescribeSpeakerRequest request) {
request = beforeClientExecution(request);
return executeDescribeSpeaker(request);
}
@SdkInternalApi
final DescribeSpeakerResult executeDescribeSpeaker(DescribeSpeakerRequest describeSpeakerRequest) {
ExecutionContext executionContext = createExecutionContext(describeSpeakerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSpeakerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSpeakerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSpeaker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSpeakerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified speaker enrollment job.
*
*
* @param describeSpeakerEnrollmentJobRequest
* @return Result of the DescribeSpeakerEnrollmentJob operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeSpeakerEnrollmentJob
* @see AWS API Documentation
*/
@Override
public DescribeSpeakerEnrollmentJobResult describeSpeakerEnrollmentJob(DescribeSpeakerEnrollmentJobRequest request) {
request = beforeClientExecution(request);
return executeDescribeSpeakerEnrollmentJob(request);
}
@SdkInternalApi
final DescribeSpeakerEnrollmentJobResult executeDescribeSpeakerEnrollmentJob(DescribeSpeakerEnrollmentJobRequest describeSpeakerEnrollmentJobRequest) {
ExecutionContext executionContext = createExecutionContext(describeSpeakerEnrollmentJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSpeakerEnrollmentJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeSpeakerEnrollmentJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSpeakerEnrollmentJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeSpeakerEnrollmentJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified watchlist.
*
*
* @param describeWatchlistRequest
* @return Result of the DescribeWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DescribeWatchlist
* @see AWS API
* Documentation
*/
@Override
public DescribeWatchlistResult describeWatchlist(DescribeWatchlistRequest request) {
request = beforeClientExecution(request);
return executeDescribeWatchlist(request);
}
@SdkInternalApi
final DescribeWatchlistResult executeDescribeWatchlist(DescribeWatchlistRequest describeWatchlistRequest) {
ExecutionContext executionContext = createExecutionContext(describeWatchlistRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeWatchlistRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeWatchlistRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeWatchlist");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeWatchlistResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the fraudsters from the watchlist specified. Voice ID always expects a fraudster to be a part of at
* least one watchlist. If you try to disassociate a fraudster from its only watchlist, a
* ValidationException
is thrown.
*
*
* @param disassociateFraudsterRequest
* @return Result of the DisassociateFraudster operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.DisassociateFraudster
* @see AWS
* API Documentation
*/
@Override
public DisassociateFraudsterResult disassociateFraudster(DisassociateFraudsterRequest request) {
request = beforeClientExecution(request);
return executeDisassociateFraudster(request);
}
@SdkInternalApi
final DisassociateFraudsterResult executeDisassociateFraudster(DisassociateFraudsterRequest disassociateFraudsterRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateFraudsterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateFraudsterRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(disassociateFraudsterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateFraudster");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateFraudsterResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Evaluates a specified session based on audio data accumulated during a streaming Amazon Connect Voice ID call.
*
*
* @param evaluateSessionRequest
* @return Result of the EvaluateSession operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.EvaluateSession
* @see AWS API
* Documentation
*/
@Override
public EvaluateSessionResult evaluateSession(EvaluateSessionRequest request) {
request = beforeClientExecution(request);
return executeEvaluateSession(request);
}
@SdkInternalApi
final EvaluateSessionResult executeEvaluateSession(EvaluateSessionRequest evaluateSessionRequest) {
ExecutionContext executionContext = createExecutionContext(evaluateSessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EvaluateSessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(evaluateSessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EvaluateSession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new EvaluateSessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the domains in the Amazon Web Services account.
*
*
* @param listDomainsRequest
* @return Result of the ListDomains operation returned by the service.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListDomains
* @see AWS API
* Documentation
*/
@Override
public ListDomainsResult listDomains(ListDomainsRequest request) {
request = beforeClientExecution(request);
return executeListDomains(request);
}
@SdkInternalApi
final ListDomainsResult executeListDomains(ListDomainsRequest listDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(listDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListDomainsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listDomainsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListDomains");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListDomainsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the fraudster registration jobs in the domain with the given JobStatus
. If
* JobStatus
is not provided, this lists all fraudster registration jobs in the given domain.
*
*
* @param listFraudsterRegistrationJobsRequest
* @return Result of the ListFraudsterRegistrationJobs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListFraudsterRegistrationJobs
* @see AWS API Documentation
*/
@Override
public ListFraudsterRegistrationJobsResult listFraudsterRegistrationJobs(ListFraudsterRegistrationJobsRequest request) {
request = beforeClientExecution(request);
return executeListFraudsterRegistrationJobs(request);
}
@SdkInternalApi
final ListFraudsterRegistrationJobsResult executeListFraudsterRegistrationJobs(ListFraudsterRegistrationJobsRequest listFraudsterRegistrationJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listFraudsterRegistrationJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFraudsterRegistrationJobsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listFraudsterRegistrationJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFraudsterRegistrationJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListFraudsterRegistrationJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all fraudsters in a specified watchlist or domain.
*
*
* @param listFraudstersRequest
* @return Result of the ListFraudsters operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListFraudsters
* @see AWS API
* Documentation
*/
@Override
public ListFraudstersResult listFraudsters(ListFraudstersRequest request) {
request = beforeClientExecution(request);
return executeListFraudsters(request);
}
@SdkInternalApi
final ListFraudstersResult executeListFraudsters(ListFraudstersRequest listFraudstersRequest) {
ExecutionContext executionContext = createExecutionContext(listFraudstersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListFraudstersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listFraudstersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListFraudsters");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListFraudstersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all the speaker enrollment jobs in the domain with the specified JobStatus
. If
* JobStatus
is not provided, this lists all jobs with all possible speaker enrollment job statuses.
*
*
* @param listSpeakerEnrollmentJobsRequest
* @return Result of the ListSpeakerEnrollmentJobs operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListSpeakerEnrollmentJobs
* @see AWS API Documentation
*/
@Override
public ListSpeakerEnrollmentJobsResult listSpeakerEnrollmentJobs(ListSpeakerEnrollmentJobsRequest request) {
request = beforeClientExecution(request);
return executeListSpeakerEnrollmentJobs(request);
}
@SdkInternalApi
final ListSpeakerEnrollmentJobsResult executeListSpeakerEnrollmentJobs(ListSpeakerEnrollmentJobsRequest listSpeakerEnrollmentJobsRequest) {
ExecutionContext executionContext = createExecutionContext(listSpeakerEnrollmentJobsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSpeakerEnrollmentJobsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSpeakerEnrollmentJobsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSpeakerEnrollmentJobs");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSpeakerEnrollmentJobsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all speakers in a specified domain.
*
*
* @param listSpeakersRequest
* @return Result of the ListSpeakers operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListSpeakers
* @see AWS API
* Documentation
*/
@Override
public ListSpeakersResult listSpeakers(ListSpeakersRequest request) {
request = beforeClientExecution(request);
return executeListSpeakers(request);
}
@SdkInternalApi
final ListSpeakersResult executeListSpeakers(ListSpeakersRequest listSpeakersRequest) {
ExecutionContext executionContext = createExecutionContext(listSpeakersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSpeakersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSpeakersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSpeakers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSpeakersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all tags associated with a specified Voice ID resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all watchlists in a specified domain.
*
*
* @param listWatchlistsRequest
* @return Result of the ListWatchlists operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.ListWatchlists
* @see AWS API
* Documentation
*/
@Override
public ListWatchlistsResult listWatchlists(ListWatchlistsRequest request) {
request = beforeClientExecution(request);
return executeListWatchlists(request);
}
@SdkInternalApi
final ListWatchlistsResult executeListWatchlists(ListWatchlistsRequest listWatchlistsRequest) {
ExecutionContext executionContext = createExecutionContext(listWatchlistsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWatchlistsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listWatchlistsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListWatchlists");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListWatchlistsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Opts out a speaker from Voice ID. A speaker can be opted out regardless of whether or not they already exist in
* Voice ID. If they don't yet exist, a new speaker is created in an opted out state. If they already exist, their
* existing status is overridden and they are opted out. Enrollment and evaluation authentication requests are
* rejected for opted out speakers, and opted out speakers have no voice embeddings stored in Voice ID.
*
*
* @param optOutSpeakerRequest
* @return Result of the OptOutSpeaker operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.OptOutSpeaker
* @see AWS API
* Documentation
*/
@Override
public OptOutSpeakerResult optOutSpeaker(OptOutSpeakerRequest request) {
request = beforeClientExecution(request);
return executeOptOutSpeaker(request);
}
@SdkInternalApi
final OptOutSpeakerResult executeOptOutSpeaker(OptOutSpeakerRequest optOutSpeakerRequest) {
ExecutionContext executionContext = createExecutionContext(optOutSpeakerRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new OptOutSpeakerRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(optOutSpeakerRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "OptOutSpeaker");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new OptOutSpeakerResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a new batch fraudster registration job using provided details.
*
*
* @param startFraudsterRegistrationJobRequest
* @return Result of the StartFraudsterRegistrationJob operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.StartFraudsterRegistrationJob
* @see AWS API Documentation
*/
@Override
public StartFraudsterRegistrationJobResult startFraudsterRegistrationJob(StartFraudsterRegistrationJobRequest request) {
request = beforeClientExecution(request);
return executeStartFraudsterRegistrationJob(request);
}
@SdkInternalApi
final StartFraudsterRegistrationJobResult executeStartFraudsterRegistrationJob(StartFraudsterRegistrationJobRequest startFraudsterRegistrationJobRequest) {
ExecutionContext executionContext = createExecutionContext(startFraudsterRegistrationJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartFraudsterRegistrationJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startFraudsterRegistrationJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartFraudsterRegistrationJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartFraudsterRegistrationJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a new batch speaker enrollment job using specified details.
*
*
* @param startSpeakerEnrollmentJobRequest
* @return Result of the StartSpeakerEnrollmentJob operation returned by the service.
* @throws ServiceQuotaExceededException
* The request exceeded the service quota. Refer to Voice ID Service Quotas and try your request again.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.StartSpeakerEnrollmentJob
* @see AWS API Documentation
*/
@Override
public StartSpeakerEnrollmentJobResult startSpeakerEnrollmentJob(StartSpeakerEnrollmentJobRequest request) {
request = beforeClientExecution(request);
return executeStartSpeakerEnrollmentJob(request);
}
@SdkInternalApi
final StartSpeakerEnrollmentJobResult executeStartSpeakerEnrollmentJob(StartSpeakerEnrollmentJobRequest startSpeakerEnrollmentJobRequest) {
ExecutionContext executionContext = createExecutionContext(startSpeakerEnrollmentJobRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSpeakerEnrollmentJobRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startSpeakerEnrollmentJobRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSpeakerEnrollmentJob");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartSpeakerEnrollmentJobResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tags a Voice ID resource with the provided list of tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes specified tags from a specified Amazon Connect Voice ID resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UntagResource
* @see AWS API
* Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified domain. This API has clobber behavior, and clears and replaces all attributes. If an
* optional field, such as 'Description' is not provided, it is removed from the domain.
*
*
* @param updateDomainRequest
* @return Result of the UpdateDomain operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UpdateDomain
* @see AWS API
* Documentation
*/
@Override
public UpdateDomainResult updateDomain(UpdateDomainRequest request) {
request = beforeClientExecution(request);
return executeUpdateDomain(request);
}
@SdkInternalApi
final UpdateDomainResult executeUpdateDomain(UpdateDomainRequest updateDomainRequest) {
ExecutionContext executionContext = createExecutionContext(updateDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the specified watchlist. Every domain has a default watchlist which cannot be updated.
*
*
* @param updateWatchlistRequest
* @return Result of the UpdateWatchlist operation returned by the service.
* @throws ResourceNotFoundException
* The specified resource cannot be found. Check the ResourceType
and error message for more
* details.
* @throws ValidationException
* The request failed one or more validations; check the error message for more details.
* @throws ConflictException
* The request failed due to a conflict. Check the ConflictType
and error message for more
* details.
* @throws InternalServerException
* The request failed due to an unknown error on the server side.
* @throws ThrottlingException
* The request was denied due to request throttling. Please slow down your request rate. Refer to Amazon Connect Voice ID Service API throttling quotas and try your request again.
* @throws AccessDeniedException
* You do not have sufficient permissions to perform this action. Check the error message and try again.
* @sample AmazonVoiceID.UpdateWatchlist
* @see AWS API
* Documentation
*/
@Override
public UpdateWatchlistResult updateWatchlist(UpdateWatchlistRequest request) {
request = beforeClientExecution(request);
return executeUpdateWatchlist(request);
}
@SdkInternalApi
final UpdateWatchlistResult executeUpdateWatchlist(UpdateWatchlistRequest updateWatchlistRequest) {
ExecutionContext executionContext = createExecutionContext(updateWatchlistRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWatchlistRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateWatchlistRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Voice ID");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateWatchlist");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateWatchlistResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}