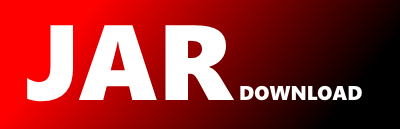
com.amazonaws.services.voiceid.model.SpeakerEnrollmentJob Maven / Gradle / Ivy
Show all versions of aws-java-sdk-voiceid Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.voiceid.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains all the information about a speaker enrollment job.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SpeakerEnrollmentJob implements Serializable, Cloneable, StructuredPojo {
/**
*
* A timestamp of when the speaker enrollment job was created.
*
*/
private java.util.Date createdAt;
/**
*
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to read the
* input manifest file and write the job output file.
*
*/
private String dataAccessRoleArn;
/**
*
* The identifier of the domain that contains the speaker enrollment job.
*
*/
private String domainId;
/**
*
* A timestamp of when the speaker enrollment job ended.
*
*/
private java.util.Date endedAt;
/**
*
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and the
* FraudDetectionConfig
to use.
*
*/
private EnrollmentConfig enrollmentConfig;
/**
*
* Contains details that are populated when an entire batch job fails. In cases of individual registration job
* failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual registration
* requests that failed.
*
*/
private FailureDetails failureDetails;
/**
*
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*
*/
private InputDataConfig inputDataConfig;
/**
*
* The service-generated identifier for the speaker enrollment job.
*
*/
private String jobId;
/**
*
* The client-provided name for the speaker enrollment job.
*
*/
private String jobName;
/**
*
* Provides details on job progress. This field shows the completed percentage of registration requests listed in
* the input file.
*
*/
private JobProgress jobProgress;
/**
*
* The current status of the speaker enrollment job.
*
*/
private String jobStatus;
/**
*
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
*
*/
private OutputDataConfig outputDataConfig;
/**
*
* A timestamp of when the speaker enrollment job was created.
*
*
* @param createdAt
* A timestamp of when the speaker enrollment job was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* A timestamp of when the speaker enrollment job was created.
*
*
* @return A timestamp of when the speaker enrollment job was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* A timestamp of when the speaker enrollment job was created.
*
*
* @param createdAt
* A timestamp of when the speaker enrollment job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to read the
* input manifest file and write the job output file.
*
*
* @param dataAccessRoleArn
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to
* read the input manifest file and write the job output file.
*/
public void setDataAccessRoleArn(String dataAccessRoleArn) {
this.dataAccessRoleArn = dataAccessRoleArn;
}
/**
*
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to read the
* input manifest file and write the job output file.
*
*
* @return The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to
* read the input manifest file and write the job output file.
*/
public String getDataAccessRoleArn() {
return this.dataAccessRoleArn;
}
/**
*
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to read the
* input manifest file and write the job output file.
*
*
* @param dataAccessRoleArn
* The IAM role Amazon Resource Name (ARN) that grants Voice ID permissions to access customer's buckets to
* read the input manifest file and write the job output file.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withDataAccessRoleArn(String dataAccessRoleArn) {
setDataAccessRoleArn(dataAccessRoleArn);
return this;
}
/**
*
* The identifier of the domain that contains the speaker enrollment job.
*
*
* @param domainId
* The identifier of the domain that contains the speaker enrollment job.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The identifier of the domain that contains the speaker enrollment job.
*
*
* @return The identifier of the domain that contains the speaker enrollment job.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The identifier of the domain that contains the speaker enrollment job.
*
*
* @param domainId
* The identifier of the domain that contains the speaker enrollment job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* A timestamp of when the speaker enrollment job ended.
*
*
* @param endedAt
* A timestamp of when the speaker enrollment job ended.
*/
public void setEndedAt(java.util.Date endedAt) {
this.endedAt = endedAt;
}
/**
*
* A timestamp of when the speaker enrollment job ended.
*
*
* @return A timestamp of when the speaker enrollment job ended.
*/
public java.util.Date getEndedAt() {
return this.endedAt;
}
/**
*
* A timestamp of when the speaker enrollment job ended.
*
*
* @param endedAt
* A timestamp of when the speaker enrollment job ended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withEndedAt(java.util.Date endedAt) {
setEndedAt(endedAt);
return this;
}
/**
*
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and the
* FraudDetectionConfig
to use.
*
*
* @param enrollmentConfig
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and
* the FraudDetectionConfig
to use.
*/
public void setEnrollmentConfig(EnrollmentConfig enrollmentConfig) {
this.enrollmentConfig = enrollmentConfig;
}
/**
*
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and the
* FraudDetectionConfig
to use.
*
*
* @return The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and
* the FraudDetectionConfig
to use.
*/
public EnrollmentConfig getEnrollmentConfig() {
return this.enrollmentConfig;
}
/**
*
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and the
* FraudDetectionConfig
to use.
*
*
* @param enrollmentConfig
* The configuration that defines the action to take when the speaker is already enrolled in Voice ID, and
* the FraudDetectionConfig
to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withEnrollmentConfig(EnrollmentConfig enrollmentConfig) {
setEnrollmentConfig(enrollmentConfig);
return this;
}
/**
*
* Contains details that are populated when an entire batch job fails. In cases of individual registration job
* failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual registration
* requests that failed.
*
*
* @param failureDetails
* Contains details that are populated when an entire batch job fails. In cases of individual registration
* job failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual
* registration requests that failed.
*/
public void setFailureDetails(FailureDetails failureDetails) {
this.failureDetails = failureDetails;
}
/**
*
* Contains details that are populated when an entire batch job fails. In cases of individual registration job
* failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual registration
* requests that failed.
*
*
* @return Contains details that are populated when an entire batch job fails. In cases of individual registration
* job failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual
* registration requests that failed.
*/
public FailureDetails getFailureDetails() {
return this.failureDetails;
}
/**
*
* Contains details that are populated when an entire batch job fails. In cases of individual registration job
* failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual registration
* requests that failed.
*
*
* @param failureDetails
* Contains details that are populated when an entire batch job fails. In cases of individual registration
* job failures, the batch job as a whole doesn't fail; it is completed with a JobStatus
of
* COMPLETED_WITH_ERRORS
. You can use the job output file to identify the individual
* registration requests that failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withFailureDetails(FailureDetails failureDetails) {
setFailureDetails(failureDetails);
return this;
}
/**
*
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*
*
* @param inputDataConfig
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*/
public void setInputDataConfig(InputDataConfig inputDataConfig) {
this.inputDataConfig = inputDataConfig;
}
/**
*
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*
*
* @return The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*/
public InputDataConfig getInputDataConfig() {
return this.inputDataConfig;
}
/**
*
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
*
*
* @param inputDataConfig
* The input data config containing an S3 URI for the input manifest file that contains the list of speaker
* enrollment job requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withInputDataConfig(InputDataConfig inputDataConfig) {
setInputDataConfig(inputDataConfig);
return this;
}
/**
*
* The service-generated identifier for the speaker enrollment job.
*
*
* @param jobId
* The service-generated identifier for the speaker enrollment job.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
*
* The service-generated identifier for the speaker enrollment job.
*
*
* @return The service-generated identifier for the speaker enrollment job.
*/
public String getJobId() {
return this.jobId;
}
/**
*
* The service-generated identifier for the speaker enrollment job.
*
*
* @param jobId
* The service-generated identifier for the speaker enrollment job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withJobId(String jobId) {
setJobId(jobId);
return this;
}
/**
*
* The client-provided name for the speaker enrollment job.
*
*
* @param jobName
* The client-provided name for the speaker enrollment job.
*/
public void setJobName(String jobName) {
this.jobName = jobName;
}
/**
*
* The client-provided name for the speaker enrollment job.
*
*
* @return The client-provided name for the speaker enrollment job.
*/
public String getJobName() {
return this.jobName;
}
/**
*
* The client-provided name for the speaker enrollment job.
*
*
* @param jobName
* The client-provided name for the speaker enrollment job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withJobName(String jobName) {
setJobName(jobName);
return this;
}
/**
*
* Provides details on job progress. This field shows the completed percentage of registration requests listed in
* the input file.
*
*
* @param jobProgress
* Provides details on job progress. This field shows the completed percentage of registration requests
* listed in the input file.
*/
public void setJobProgress(JobProgress jobProgress) {
this.jobProgress = jobProgress;
}
/**
*
* Provides details on job progress. This field shows the completed percentage of registration requests listed in
* the input file.
*
*
* @return Provides details on job progress. This field shows the completed percentage of registration requests
* listed in the input file.
*/
public JobProgress getJobProgress() {
return this.jobProgress;
}
/**
*
* Provides details on job progress. This field shows the completed percentage of registration requests listed in
* the input file.
*
*
* @param jobProgress
* Provides details on job progress. This field shows the completed percentage of registration requests
* listed in the input file.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withJobProgress(JobProgress jobProgress) {
setJobProgress(jobProgress);
return this;
}
/**
*
* The current status of the speaker enrollment job.
*
*
* @param jobStatus
* The current status of the speaker enrollment job.
* @see SpeakerEnrollmentJobStatus
*/
public void setJobStatus(String jobStatus) {
this.jobStatus = jobStatus;
}
/**
*
* The current status of the speaker enrollment job.
*
*
* @return The current status of the speaker enrollment job.
* @see SpeakerEnrollmentJobStatus
*/
public String getJobStatus() {
return this.jobStatus;
}
/**
*
* The current status of the speaker enrollment job.
*
*
* @param jobStatus
* The current status of the speaker enrollment job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SpeakerEnrollmentJobStatus
*/
public SpeakerEnrollmentJob withJobStatus(String jobStatus) {
setJobStatus(jobStatus);
return this;
}
/**
*
* The current status of the speaker enrollment job.
*
*
* @param jobStatus
* The current status of the speaker enrollment job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SpeakerEnrollmentJobStatus
*/
public SpeakerEnrollmentJob withJobStatus(SpeakerEnrollmentJobStatus jobStatus) {
this.jobStatus = jobStatus.toString();
return this;
}
/**
*
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
*
*
* @param outputDataConfig
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
*/
public void setOutputDataConfig(OutputDataConfig outputDataConfig) {
this.outputDataConfig = outputDataConfig;
}
/**
*
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
*
*
* @return The output data config containing the S3 location where Voice ID writes the job output file; you must
* also include a KMS key ID to encrypt the file.
*/
public OutputDataConfig getOutputDataConfig() {
return this.outputDataConfig;
}
/**
*
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
*
*
* @param outputDataConfig
* The output data config containing the S3 location where Voice ID writes the job output file; you must also
* include a KMS key ID to encrypt the file.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SpeakerEnrollmentJob withOutputDataConfig(OutputDataConfig outputDataConfig) {
setOutputDataConfig(outputDataConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDataAccessRoleArn() != null)
sb.append("DataAccessRoleArn: ").append(getDataAccessRoleArn()).append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getEndedAt() != null)
sb.append("EndedAt: ").append(getEndedAt()).append(",");
if (getEnrollmentConfig() != null)
sb.append("EnrollmentConfig: ").append(getEnrollmentConfig()).append(",");
if (getFailureDetails() != null)
sb.append("FailureDetails: ").append(getFailureDetails()).append(",");
if (getInputDataConfig() != null)
sb.append("InputDataConfig: ").append(getInputDataConfig()).append(",");
if (getJobId() != null)
sb.append("JobId: ").append(getJobId()).append(",");
if (getJobName() != null)
sb.append("JobName: ").append("***Sensitive Data Redacted***").append(",");
if (getJobProgress() != null)
sb.append("JobProgress: ").append(getJobProgress()).append(",");
if (getJobStatus() != null)
sb.append("JobStatus: ").append(getJobStatus()).append(",");
if (getOutputDataConfig() != null)
sb.append("OutputDataConfig: ").append(getOutputDataConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SpeakerEnrollmentJob == false)
return false;
SpeakerEnrollmentJob other = (SpeakerEnrollmentJob) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDataAccessRoleArn() == null ^ this.getDataAccessRoleArn() == null)
return false;
if (other.getDataAccessRoleArn() != null && other.getDataAccessRoleArn().equals(this.getDataAccessRoleArn()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getEndedAt() == null ^ this.getEndedAt() == null)
return false;
if (other.getEndedAt() != null && other.getEndedAt().equals(this.getEndedAt()) == false)
return false;
if (other.getEnrollmentConfig() == null ^ this.getEnrollmentConfig() == null)
return false;
if (other.getEnrollmentConfig() != null && other.getEnrollmentConfig().equals(this.getEnrollmentConfig()) == false)
return false;
if (other.getFailureDetails() == null ^ this.getFailureDetails() == null)
return false;
if (other.getFailureDetails() != null && other.getFailureDetails().equals(this.getFailureDetails()) == false)
return false;
if (other.getInputDataConfig() == null ^ this.getInputDataConfig() == null)
return false;
if (other.getInputDataConfig() != null && other.getInputDataConfig().equals(this.getInputDataConfig()) == false)
return false;
if (other.getJobId() == null ^ this.getJobId() == null)
return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false)
return false;
if (other.getJobName() == null ^ this.getJobName() == null)
return false;
if (other.getJobName() != null && other.getJobName().equals(this.getJobName()) == false)
return false;
if (other.getJobProgress() == null ^ this.getJobProgress() == null)
return false;
if (other.getJobProgress() != null && other.getJobProgress().equals(this.getJobProgress()) == false)
return false;
if (other.getJobStatus() == null ^ this.getJobStatus() == null)
return false;
if (other.getJobStatus() != null && other.getJobStatus().equals(this.getJobStatus()) == false)
return false;
if (other.getOutputDataConfig() == null ^ this.getOutputDataConfig() == null)
return false;
if (other.getOutputDataConfig() != null && other.getOutputDataConfig().equals(this.getOutputDataConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDataAccessRoleArn() == null) ? 0 : getDataAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getEndedAt() == null) ? 0 : getEndedAt().hashCode());
hashCode = prime * hashCode + ((getEnrollmentConfig() == null) ? 0 : getEnrollmentConfig().hashCode());
hashCode = prime * hashCode + ((getFailureDetails() == null) ? 0 : getFailureDetails().hashCode());
hashCode = prime * hashCode + ((getInputDataConfig() == null) ? 0 : getInputDataConfig().hashCode());
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode + ((getJobName() == null) ? 0 : getJobName().hashCode());
hashCode = prime * hashCode + ((getJobProgress() == null) ? 0 : getJobProgress().hashCode());
hashCode = prime * hashCode + ((getJobStatus() == null) ? 0 : getJobStatus().hashCode());
hashCode = prime * hashCode + ((getOutputDataConfig() == null) ? 0 : getOutputDataConfig().hashCode());
return hashCode;
}
@Override
public SpeakerEnrollmentJob clone() {
try {
return (SpeakerEnrollmentJob) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.voiceid.model.transform.SpeakerEnrollmentJobMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}