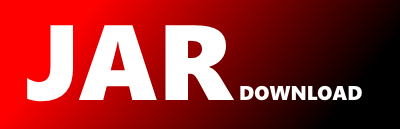
com.amazonaws.services.waf.AWSWAFClient Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.waf;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.services.waf.model.*;
import com.amazonaws.services.waf.model.transform.*;
/**
* Client for accessing WAF. All service calls made using this client are
* blocking, and will not return until the service call completes.
*
*
* This is the AWS WAF API Reference. This guide is for developers who
* need detailed information about the AWS WAF API actions, data types, and
* errors. For detailed information about AWS WAF features and an overview of
* how to use the AWS WAF API, see the AWS WAF
* Developer Guide.
*
*/
@ThreadSafe
public class AWSWAFClient extends AmazonWebServiceClient implements AWSWAF {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSWAF.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "waf";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "waf";
/**
* Client configuration factory providing ClientConfigurations tailored to
* this client
*/
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final SdkJsonProtocolFactory protocolFactory = new SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFInternalErrorException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFInternalErrorException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFReferencedItemException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFReferencedItemException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFInvalidAccountException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFInvalidAccountException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFStaleDataException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFStaleDataException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"WAFNonexistentContainerException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFNonexistentContainerException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFLimitsExceededException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFLimitsExceededException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"WAFInvalidParameterException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFInvalidParameterException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFNonEmptyEntityException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFNonEmptyEntityException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode("WAFDisallowedNameException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFDisallowedNameException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"WAFInvalidOperationException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFInvalidOperationException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata()
.withErrorCode(
"WAFNonexistentItemException")
.withModeledClass(
com.amazonaws.services.waf.model.WAFNonexistentItemException.class)));
/**
* Constructs a new client to invoke service methods on WAF. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSWAFClient() {
this(new DefaultAWSCredentialsProviderChain(), configFactory
.getConfig());
}
/**
* Constructs a new client to invoke service methods on WAF. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to WAF (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AWSWAFClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on WAF using the
* specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public AWSWAFClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on WAF using the
* specified AWS account credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to WAF (ex: proxy settings, retry counts, etc.).
*/
public AWSWAFClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(
awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on WAF using the
* specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
*/
public AWSWAFClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on WAF using the
* specified AWS account credentials provider and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to WAF (ex: proxy settings, retry counts, etc.).
*/
public AWSWAFClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on WAF using the
* specified AWS account credentials provider, client configuration options,
* and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to WAF (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AWSWAFClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://waf.amazonaws.com/");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s
.addAll(chainFactory
.newRequestHandlerChain("/com/amazonaws/services/waf/request.handlers"));
requestHandler2s
.addAll(chainFactory
.newRequestHandler2Chain("/com/amazonaws/services/waf/request.handler2s"));
}
/**
*
* Creates a ByteMatchSet
. You then use
* UpdateByteMatchSet to identify the part of a web request that you
* want AWS WAF to inspect, such as the values of the
* User-Agent
header or the query string. For example, you can
* create a ByteMatchSet
that matches any requests with
* User-Agent
headers that contain the string
* BadBot
. You can then configure AWS WAF to reject those
* requests.
*
*
* To create and configure a ByteMatchSet
, perform the
* following steps:
*
*
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* CreateByteMatchSet
request.
* - Submit a
CreateByteMatchSet
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateByteMatchSet
request.
* - Submit an UpdateByteMatchSet request to specify the part of
* the request that you want AWS WAF to inspect (for example, the header or
* the URI) and the value that you want AWS WAF to watch for.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createByteMatchSetRequest
* @return Result of the CreateByteMatchSet operation returned by the
* service.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateByteMatchSet
*/
@Override
public CreateByteMatchSetResult createByteMatchSet(
CreateByteMatchSetRequest createByteMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(createByteMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateByteMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(createByteMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateByteMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an IPSet, which you use to specify which web requests you
* want to allow or block based on the IP addresses that the requests
* originate from. For example, if you're receiving a lot of requests from
* one or more individual IP addresses or one or more ranges of IP addresses
* and you want to block the requests, you can create an IPSet
* that contains those IP addresses and then configure AWS WAF to block the
* requests.
*
*
* To create and configure an IPSet
, perform the following
* steps:
*
*
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a CreateIPSet
* request.
* - Submit a
CreateIPSet
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateIPSet request.
* - Submit an
UpdateIPSet
request to specify the IP
* addresses that you want AWS WAF to watch for.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createIPSetRequest
* @return Result of the CreateIPSet operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateIPSet
*/
@Override
public CreateIPSetResult createIPSet(CreateIPSetRequest createIPSetRequest) {
ExecutionContext executionContext = createExecutionContext(createIPSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateIPSetRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(createIPSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateIPSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a Rule
, which contains the IPSet
* objects, ByteMatchSet
objects, and other predicates that
* identify the requests that you want to block. If you add more than one
* predicate to a Rule
, a request must match all of the
* specifications to be allowed or blocked. For example, suppose you add the
* following to a Rule
:
*
*
* - An
IPSet
that matches the IP address
* 192.0.2.44/32
* - A
ByteMatchSet
that matches BadBot
in the
* User-Agent
header
*
*
* You then add the Rule
to a WebACL
and specify
* that you want to blocks requests that satisfy the Rule
. For
* a request to be blocked, it must come from the IP address 192.0.2.44
* and the User-Agent
header in the request must contain
* the value BadBot
.
*
*
* To create and configure a Rule
, perform the following steps:
*
*
* - Create and update the predicates that you want to include in the
*
Rule
. For more information, see CreateByteMatchSet,
* CreateIPSet, and CreateSqlInjectionMatchSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a CreateRule
* request.
* - Submit a
CreateRule
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an UpdateRule
* request.
* - Submit an
UpdateRule
request to specify the predicates
* that you want to include in the Rule
.
* - Create and update a
WebACL
that contains the
* Rule
. For more information, see CreateWebACL.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createRuleRequest
* @return Result of the CreateRule operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateRule
*/
@Override
public CreateRuleResult createRule(CreateRuleRequest createRuleRequest) {
ExecutionContext executionContext = createExecutionContext(createRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRuleRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(createRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SizeConstraintSet
. You then use
* UpdateSizeConstraintSet to identify the part of a web request that
* you want AWS WAF to check for length, such as the length of the
* User-Agent
header or the length of the query string. For
* example, you can create a SizeConstraintSet
that matches any
* requests that have a query string that is longer than 100 bytes. You can
* then configure AWS WAF to reject those requests.
*
*
* To create and configure a SizeConstraintSet
, perform the
* following steps:
*
*
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* CreateSizeConstraintSet
request.
* - Submit a
CreateSizeConstraintSet
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateSizeConstraintSet
request.
* - Submit an UpdateSizeConstraintSet request to specify the part
* of the request that you want AWS WAF to inspect (for example, the header
* or the URI) and the value that you want AWS WAF to watch for.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createSizeConstraintSetRequest
* @return Result of the CreateSizeConstraintSet operation returned by the
* service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateSizeConstraintSet
*/
@Override
public CreateSizeConstraintSetResult createSizeConstraintSet(
CreateSizeConstraintSetRequest createSizeConstraintSetRequest) {
ExecutionContext executionContext = createExecutionContext(createSizeConstraintSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSizeConstraintSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(createSizeConstraintSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateSizeConstraintSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SqlInjectionMatchSet, which you use to allow, block, or
* count requests that contain snippets of SQL code in a specified part of
* web requests. AWS WAF searches for character sequences that are likely to
* be malicious strings.
*
*
* To create and configure a SqlInjectionMatchSet
, perform the
* following steps:
*
*
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* CreateSqlInjectionMatchSet
request.
* - Submit a
CreateSqlInjectionMatchSet
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateSqlInjectionMatchSet request.
* - Submit an UpdateSqlInjectionMatchSet request to specify the
* parts of web requests in which you want to allow, block, or count
* malicious SQL code.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createSqlInjectionMatchSetRequest
* A request to create a SqlInjectionMatchSet.
* @return Result of the CreateSqlInjectionMatchSet operation returned by
* the service.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateSqlInjectionMatchSet
*/
@Override
public CreateSqlInjectionMatchSetResult createSqlInjectionMatchSet(
CreateSqlInjectionMatchSetRequest createSqlInjectionMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(createSqlInjectionMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSqlInjectionMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(createSqlInjectionMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateSqlInjectionMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a WebACL
, which contains the Rules
that
* identify the CloudFront web requests that you want to allow, block, or
* count. AWS WAF evaluates Rules
in order based on the value
* of Priority
for each Rule
.
*
*
* You also specify a default action, either ALLOW
or
* BLOCK
. If a web request doesn't match any of the
* Rules
in a WebACL
, AWS WAF responds to the
* request with the default action.
*
*
* To create and configure a WebACL
, perform the following
* steps:
*
*
* - Create and update the
ByteMatchSet
objects and other
* predicates that you want to include in Rules
. For more
* information, see CreateByteMatchSet, UpdateByteMatchSet,
* CreateIPSet, UpdateIPSet,
* CreateSqlInjectionMatchSet, and UpdateSqlInjectionMatchSet.
*
* - Create and update the
Rules
that you want to include in
* the WebACL
. For more information, see CreateRule and
* UpdateRule.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a CreateWebACL
* request.
* - Submit a
CreateWebACL
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateWebACL request.
* - Submit an UpdateWebACL request to specify the
*
Rules
that you want to include in the WebACL
,
* to specify the default action, and to associate the WebACL
* with a CloudFront distribution.
*
*
* For more information about how to use the AWS WAF API, see the AWS WAF
* Developer Guide.
*
*
* @param createWebACLRequest
* @return Result of the CreateWebACL operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateWebACL
*/
@Override
public CreateWebACLResult createWebACL(
CreateWebACLRequest createWebACLRequest) {
ExecutionContext executionContext = createExecutionContext(createWebACLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateWebACLRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(createWebACLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateWebACLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an XssMatchSet, which you use to allow, block, or count
* requests that contain cross-site scripting attacks in the specified part
* of web requests. AWS WAF searches for character sequences that are likely
* to be malicious strings.
*
*
* To create and configure an XssMatchSet
, perform the
* following steps:
*
*
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* CreateXssMatchSet
request.
* - Submit a
CreateXssMatchSet
request.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateXssMatchSet request.
* - Submit an UpdateXssMatchSet request to specify the parts of
* web requests in which you want to allow, block, or count cross-site
* scripting attacks.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param createXssMatchSetRequest
* A request to create an XssMatchSet.
* @return Result of the CreateXssMatchSet operation returned by the
* service.
* @throws WAFDisallowedNameException
* The name specified is invalid.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.CreateXssMatchSet
*/
@Override
public CreateXssMatchSetResult createXssMatchSet(
CreateXssMatchSetRequest createXssMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(createXssMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateXssMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(createXssMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new CreateXssMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a ByteMatchSet. You can't delete a
* ByteMatchSet
if it's still used in any Rules
or
* if it still includes any ByteMatchTuple objects (any filters).
*
*
* If you just want to remove a ByteMatchSet
from a
* Rule
, use UpdateRule.
*
*
* To permanently delete a ByteMatchSet
, perform the following
* steps:
*
*
* - Update the
ByteMatchSet
to remove filters, if any. For
* more information, see UpdateByteMatchSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* DeleteByteMatchSet
request.
* - Submit a
DeleteByteMatchSet
request.
*
*
* @param deleteByteMatchSetRequest
* @return Result of the DeleteByteMatchSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteByteMatchSet
*/
@Override
public DeleteByteMatchSetResult deleteByteMatchSet(
DeleteByteMatchSetRequest deleteByteMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteByteMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteByteMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(deleteByteMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteByteMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes an IPSet. You can't delete an
* IPSet
if it's still used in any Rules
or if it
* still includes any IP addresses.
*
*
* If you just want to remove an IPSet
from a Rule
* , use UpdateRule.
*
*
* To permanently delete an IPSet
from AWS WAF, perform the
* following steps:
*
*
* - Update the
IPSet
to remove IP address ranges, if any.
* For more information, see UpdateIPSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a DeleteIPSet
* request.
* - Submit a
DeleteIPSet
request.
*
*
* @param deleteIPSetRequest
* @return Result of the DeleteIPSet operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteIPSet
*/
@Override
public DeleteIPSetResult deleteIPSet(DeleteIPSetRequest deleteIPSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteIPSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteIPSetRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(deleteIPSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteIPSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a Rule. You can't delete a Rule
* if it's still used in any WebACL
objects or if it still
* includes any predicates, such as ByteMatchSet
objects.
*
*
* If you just want to remove a Rule
from a WebACL
* , use UpdateWebACL.
*
*
* To permanently delete a Rule
from AWS WAF, perform the
* following steps:
*
*
* - Update the
Rule
to remove predicates, if any. For more
* information, see UpdateRule.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a DeleteRule
* request.
* - Submit a
DeleteRule
request.
*
*
* @param deleteRuleRequest
* @return Result of the DeleteRule operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteRule
*/
@Override
public DeleteRuleResult deleteRule(DeleteRuleRequest deleteRuleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRuleRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(deleteRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a SizeConstraintSet. You can't delete a
* SizeConstraintSet
if it's still used in any
* Rules
or if it still includes any SizeConstraint
* objects (any filters).
*
*
* If you just want to remove a SizeConstraintSet
from a
* Rule
, use UpdateRule.
*
*
* To permanently delete a SizeConstraintSet
, perform the
* following steps:
*
*
* - Update the
SizeConstraintSet
to remove filters, if any.
* For more information, see UpdateSizeConstraintSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* DeleteSizeConstraintSet
request.
* - Submit a
DeleteSizeConstraintSet
request.
*
*
* @param deleteSizeConstraintSetRequest
* @return Result of the DeleteSizeConstraintSet operation returned by the
* service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteSizeConstraintSet
*/
@Override
public DeleteSizeConstraintSetResult deleteSizeConstraintSet(
DeleteSizeConstraintSetRequest deleteSizeConstraintSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSizeConstraintSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSizeConstraintSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(deleteSizeConstraintSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteSizeConstraintSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a SqlInjectionMatchSet. You can't delete a
* SqlInjectionMatchSet
if it's still used in any
* Rules
or if it still contains any
* SqlInjectionMatchTuple objects.
*
*
* If you just want to remove a SqlInjectionMatchSet
from a
* Rule
, use UpdateRule.
*
*
* To permanently delete a SqlInjectionMatchSet
from AWS WAF,
* perform the following steps:
*
*
* - Update the
SqlInjectionMatchSet
to remove filters, if
* any. For more information, see UpdateSqlInjectionMatchSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* DeleteSqlInjectionMatchSet
request.
* - Submit a
DeleteSqlInjectionMatchSet
request.
*
*
* @param deleteSqlInjectionMatchSetRequest
* A request to delete a SqlInjectionMatchSet from AWS WAF.
* @return Result of the DeleteSqlInjectionMatchSet operation returned by
* the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteSqlInjectionMatchSet
*/
@Override
public DeleteSqlInjectionMatchSetResult deleteSqlInjectionMatchSet(
DeleteSqlInjectionMatchSetRequest deleteSqlInjectionMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSqlInjectionMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSqlInjectionMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(deleteSqlInjectionMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteSqlInjectionMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes a WebACL. You can't delete a
* WebACL
if it still contains any Rules
.
*
*
* To delete a WebACL
, perform the following steps:
*
*
* - Update the
WebACL
to remove Rules
, if any.
* For more information, see UpdateWebACL.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a DeleteWebACL
* request.
* - Submit a
DeleteWebACL
request.
*
*
* @param deleteWebACLRequest
* @return Result of the DeleteWebACL operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteWebACL
*/
@Override
public DeleteWebACLResult deleteWebACL(
DeleteWebACLRequest deleteWebACLRequest) {
ExecutionContext executionContext = createExecutionContext(deleteWebACLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteWebACLRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(deleteWebACLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteWebACLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently deletes an XssMatchSet. You can't delete an
* XssMatchSet
if it's still used in any Rules
or
* if it still contains any XssMatchTuple objects.
*
*
* If you just want to remove an XssMatchSet
from a
* Rule
, use UpdateRule.
*
*
* To permanently delete an XssMatchSet
from AWS WAF, perform
* the following steps:
*
*
* - Update the
XssMatchSet
to remove filters, if any. For
* more information, see UpdateXssMatchSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of a
* DeleteXssMatchSet
request.
* - Submit a
DeleteXssMatchSet
request.
*
*
* @param deleteXssMatchSetRequest
* A request to delete an XssMatchSet from AWS WAF.
* @return Result of the DeleteXssMatchSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFNonEmptyEntityException
* The operation failed because you tried to delete an object that
* isn't empty. For example:
*
* - You tried to delete a
WebACL
that still contains
* one or more Rule
objects.
* - You tried to delete a
Rule
that still contains
* one or more ByteMatchSet
objects or other
* predicates.
* - You tried to delete a
ByteMatchSet
that contains
* one or more ByteMatchTuple
objects.
* - You tried to delete an
IPSet
that references one
* or more IP addresses.
* @sample AWSWAF.DeleteXssMatchSet
*/
@Override
public DeleteXssMatchSetResult deleteXssMatchSet(
DeleteXssMatchSetRequest deleteXssMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteXssMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteXssMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(deleteXssMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new DeleteXssMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the ByteMatchSet specified by ByteMatchSetId
.
*
*
* @param getByteMatchSetRequest
* @return Result of the GetByteMatchSet operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetByteMatchSet
*/
@Override
public GetByteMatchSetResult getByteMatchSet(
GetByteMatchSetRequest getByteMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(getByteMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetByteMatchSetRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(getByteMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetByteMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* When you want to create, update, or delete AWS WAF objects, get a change
* token and include the change token in the create, update, or delete
* request. Change tokens ensure that your application doesn't submit
* conflicting requests to AWS WAF.
*
*
* Each create, update, or delete request must use a unique change token. If
* your application submits a GetChangeToken
request and then
* submits a second GetChangeToken
request before submitting a
* create, update, or delete request, the second GetChangeToken
* request returns the same value as the first GetChangeToken
* request.
*
*
* When you use a change token in a create, update, or delete request, the
* status of the change token changes to PENDING
, which
* indicates that AWS WAF is propagating the change to all AWS WAF servers.
* Use GetChangeTokenStatus
to determine the status of your
* change token.
*
*
* @param getChangeTokenRequest
* @return Result of the GetChangeToken operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @sample AWSWAF.GetChangeToken
*/
@Override
public GetChangeTokenResult getChangeToken(
GetChangeTokenRequest getChangeTokenRequest) {
ExecutionContext executionContext = createExecutionContext(getChangeTokenRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetChangeTokenRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(getChangeTokenRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetChangeTokenResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the status of a ChangeToken
that you got by calling
* GetChangeToken. ChangeTokenStatus
is one of the
* following values:
*
*
* PROVISIONED
: You requested the change token by calling
* GetChangeToken
, but you haven't used it yet in a call to
* create, update, or delete an AWS WAF object.
* PENDING
: AWS WAF is propagating the create, update, or
* delete request to all AWS WAF servers.
* IN_SYNC
: Propagation is complete.
*
*
* @param getChangeTokenStatusRequest
* @return Result of the GetChangeTokenStatus operation returned by the
* service.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @sample AWSWAF.GetChangeTokenStatus
*/
@Override
public GetChangeTokenStatusResult getChangeTokenStatus(
GetChangeTokenStatusRequest getChangeTokenStatusRequest) {
ExecutionContext executionContext = createExecutionContext(getChangeTokenStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetChangeTokenStatusRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getChangeTokenStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetChangeTokenStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the IPSet that is specified by IPSetId
.
*
*
* @param getIPSetRequest
* @return Result of the GetIPSet operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetIPSet
*/
@Override
public GetIPSetResult getIPSet(GetIPSetRequest getIPSetRequest) {
ExecutionContext executionContext = createExecutionContext(getIPSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetIPSetRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getIPSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetIPSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the Rule that is specified by the RuleId
that
* you included in the GetRule
request.
*
*
* @param getRuleRequest
* @return Result of the GetRule operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetRule
*/
@Override
public GetRuleResult getRule(GetRuleRequest getRuleRequest) {
ExecutionContext executionContext = createExecutionContext(getRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRuleRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets detailed information about a specified number of requests--a
* sample--that AWS WAF randomly selects from among the first 5,000 requests
* that your AWS resource received during a time range that you choose. You
* can specify a sample size of up to 100 requests, and you can specify any
* time range in the previous three hours.
*
*
* GetSampledRequests
returns a time range, which is usually
* the time range that you specified. However, if your resource (such as a
* CloudFront distribution) received 5,000 requests before the specified
* time range elapsed, GetSampledRequests
returns an updated
* time range. This new time range indicates the actual period during which
* AWS WAF selected the requests in the sample.
*
*
* @param getSampledRequestsRequest
* @return Result of the GetSampledRequests operation returned by the
* service.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @sample AWSWAF.GetSampledRequests
*/
@Override
public GetSampledRequestsResult getSampledRequests(
GetSampledRequestsRequest getSampledRequestsRequest) {
ExecutionContext executionContext = createExecutionContext(getSampledRequestsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSampledRequestsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getSampledRequestsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetSampledRequestsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the SizeConstraintSet specified by
* SizeConstraintSetId
.
*
*
* @param getSizeConstraintSetRequest
* @return Result of the GetSizeConstraintSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetSizeConstraintSet
*/
@Override
public GetSizeConstraintSetResult getSizeConstraintSet(
GetSizeConstraintSetRequest getSizeConstraintSetRequest) {
ExecutionContext executionContext = createExecutionContext(getSizeConstraintSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSizeConstraintSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getSizeConstraintSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetSizeConstraintSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the SqlInjectionMatchSet that is specified by
* SqlInjectionMatchSetId
.
*
*
* @param getSqlInjectionMatchSetRequest
* A request to get a SqlInjectionMatchSet.
* @return Result of the GetSqlInjectionMatchSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetSqlInjectionMatchSet
*/
@Override
public GetSqlInjectionMatchSetResult getSqlInjectionMatchSet(
GetSqlInjectionMatchSetRequest getSqlInjectionMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(getSqlInjectionMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSqlInjectionMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(getSqlInjectionMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetSqlInjectionMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the WebACL that is specified by WebACLId
.
*
*
* @param getWebACLRequest
* @return Result of the GetWebACL operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetWebACL
*/
@Override
public GetWebACLResult getWebACL(GetWebACLRequest getWebACLRequest) {
ExecutionContext executionContext = createExecutionContext(getWebACLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetWebACLRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(getWebACLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetWebACLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the XssMatchSet that is specified by
* XssMatchSetId
.
*
*
* @param getXssMatchSetRequest
* A request to get an XssMatchSet.
* @return Result of the GetXssMatchSet operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @sample AWSWAF.GetXssMatchSet
*/
@Override
public GetXssMatchSetResult getXssMatchSet(
GetXssMatchSetRequest getXssMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(getXssMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetXssMatchSetRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(getXssMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new GetXssMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of ByteMatchSetSummary objects.
*
*
* @param listByteMatchSetsRequest
* @return Result of the ListByteMatchSets operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListByteMatchSets
*/
@Override
public ListByteMatchSetsResult listByteMatchSets(
ListByteMatchSetsRequest listByteMatchSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listByteMatchSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListByteMatchSetsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(listByteMatchSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListByteMatchSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of IPSetSummary objects in the response.
*
*
* @param listIPSetsRequest
* @return Result of the ListIPSets operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListIPSets
*/
@Override
public ListIPSetsResult listIPSets(ListIPSetsRequest listIPSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listIPSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListIPSetsRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(listIPSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListIPSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of RuleSummary objects.
*
*
* @param listRulesRequest
* @return Result of the ListRules operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListRules
*/
@Override
public ListRulesResult listRules(ListRulesRequest listRulesRequest) {
ExecutionContext executionContext = createExecutionContext(listRulesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListRulesRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(listRulesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListRulesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of SizeConstraintSetSummary objects.
*
*
* @param listSizeConstraintSetsRequest
* @return Result of the ListSizeConstraintSets operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListSizeConstraintSets
*/
@Override
public ListSizeConstraintSetsResult listSizeConstraintSets(
ListSizeConstraintSetsRequest listSizeConstraintSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listSizeConstraintSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSizeConstraintSetsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(listSizeConstraintSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListSizeConstraintSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of SqlInjectionMatchSet objects.
*
*
* @param listSqlInjectionMatchSetsRequest
* A request to list the SqlInjectionMatchSet objects created
* by the current AWS account.
* @return Result of the ListSqlInjectionMatchSets operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListSqlInjectionMatchSets
*/
@Override
public ListSqlInjectionMatchSetsResult listSqlInjectionMatchSets(
ListSqlInjectionMatchSetsRequest listSqlInjectionMatchSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listSqlInjectionMatchSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSqlInjectionMatchSetsRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(listSqlInjectionMatchSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListSqlInjectionMatchSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of WebACLSummary objects in the response.
*
*
* @param listWebACLsRequest
* @return Result of the ListWebACLs operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListWebACLs
*/
@Override
public ListWebACLsResult listWebACLs(ListWebACLsRequest listWebACLsRequest) {
ExecutionContext executionContext = createExecutionContext(listWebACLsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListWebACLsRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(listWebACLsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListWebACLsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns an array of XssMatchSet objects.
*
*
* @param listXssMatchSetsRequest
* A request to list the XssMatchSet objects created by the
* current AWS account.
* @return Result of the ListXssMatchSets operation returned by the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @sample AWSWAF.ListXssMatchSets
*/
@Override
public ListXssMatchSetsResult listXssMatchSets(
ListXssMatchSetsRequest listXssMatchSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listXssMatchSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListXssMatchSetsRequestMarshaller(protocolFactory)
.marshall(super
.beforeMarshalling(listXssMatchSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new ListXssMatchSetsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes ByteMatchTuple objects (filters) in a
* ByteMatchSet. For each ByteMatchTuple
object, you
* specify the following values:
*
*
* - Whether to insert or delete the object from the array. If you want to
* change a
ByteMatchSetUpdate
object, you delete the existing
* object and add a new one.
* - The part of a web request that you want AWS WAF to inspect, such as a
* query string or the value of the
User-Agent
header.
* - The bytes (typically a string that corresponds with ASCII characters)
* that you want AWS WAF to look for. For more information, including how
* you specify the values for the AWS WAF API and the AWS CLI or SDKs, see
*
TargetString
in the ByteMatchTuple data type.
* - Where to look, such as at the beginning or the end of a query string.
*
* - Whether to perform any conversions on the request, such as converting
* it to lowercase, before inspecting it for the specified string.
*
*
* For example, you can add a ByteMatchSetUpdate
object that
* matches web requests in which User-Agent
headers contain the
* string BadBot
. You can then configure AWS WAF to block those
* requests.
*
*
* To create and configure a ByteMatchSet
, perform the
* following steps:
*
*
* - Create a
ByteMatchSet.
For more information, see
* CreateByteMatchSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of an
* UpdateByteMatchSet
request.
* - Submit an
UpdateByteMatchSet
request to specify the part
* of the request that you want AWS WAF to inspect (for example, the header
* or the URI) and the value that you want AWS WAF to watch for.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateByteMatchSetRequest
* @return Result of the UpdateByteMatchSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateByteMatchSet
*/
@Override
public UpdateByteMatchSetResult updateByteMatchSet(
UpdateByteMatchSetRequest updateByteMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateByteMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateByteMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateByteMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateByteMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes IPSetDescriptor objects in an
* IPSet
. For each IPSetDescriptor
object, you
* specify the following values:
*
*
* - Whether to insert or delete the object from the array. If you want to
* change an
IPSetDescriptor
object, you delete the existing
* object and add a new one.
* - The IP address version,
IPv4
.
* - The IP address in CIDR notation, for example,
*
192.0.2.0/24
(for the range of IP addresses from
* 192.0.2.0
to 192.0.2.255
) or
* 192.0.2.44/32
(for the individual IP address
* 192.0.2.44
).
*
*
* AWS WAF supports /8, /16, /24, and /32 IP address ranges. For more
* information about CIDR notation, see the Wikipedia entry Classless Inter-Domain Routing.
*
*
* You use an IPSet
to specify which web requests you want to
* allow or block based on the IP addresses that the requests originated
* from. For example, if you're receiving a lot of requests from one or a
* small number of IP addresses and you want to block the requests, you can
* create an IPSet
that specifies those IP addresses, and then
* configure AWS WAF to block the requests.
*
*
* To create and configure an IPSet
, perform the following
* steps:
*
*
* - Submit a CreateIPSet request.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of an UpdateIPSet request.
* - Submit an
UpdateIPSet
request to specify the IP
* addresses that you want AWS WAF to watch for.
*
*
* When you update an IPSet
, you specify the IP addresses that
* you want to add and/or the IP addresses that you want to delete. If you
* want to change an IP address, you delete the existing IP address and add
* the new one.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateIPSetRequest
* @return Result of the UpdateIPSet operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateIPSet
*/
@Override
public UpdateIPSetResult updateIPSet(UpdateIPSetRequest updateIPSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateIPSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateIPSetRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(updateIPSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateIPSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes Predicate objects in a Rule
. Each
* Predicate
object identifies a predicate, such as a
* ByteMatchSet or an IPSet, that specifies the web requests
* that you want to allow, block, or count. If you add more than one
* predicate to a Rule
, a request must match all of the
* specifications to be allowed, blocked, or counted. For example, suppose
* you add the following to a Rule
:
*
*
* - A
ByteMatchSet
that matches the value
* BadBot
in the User-Agent
header
* - An
IPSet
that matches the IP address
* 192.0.2.44
*
*
* You then add the Rule
to a WebACL
and specify
* that you want to block requests that satisfy the Rule
. For a
* request to be blocked, the User-Agent
header in the request
* must contain the value BadBot
and the request must
* originate from the IP address 192.0.2.44.
*
*
* To create and configure a Rule
, perform the following steps:
*
*
* - Create and update the predicates that you want to include in the
*
Rule
.
* - Create the
Rule
. See CreateRule.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an UpdateRule
* request.
* - Submit an
UpdateRule
request to add predicates to the
* Rule
.
* - Create and update a
WebACL
that contains the
* Rule
. See CreateWebACL.
*
*
* If you want to replace one ByteMatchSet
or
* IPSet
with another, you delete the existing one and add the
* new one.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateRuleRequest
* @return Result of the UpdateRule operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateRule
*/
@Override
public UpdateRuleResult updateRule(UpdateRuleRequest updateRuleRequest) {
ExecutionContext executionContext = createExecutionContext(updateRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateRuleRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(updateRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes SizeConstraint objects (filters) in a
* SizeConstraintSet. For each SizeConstraint
object,
* you specify the following values:
*
*
* - Whether to insert or delete the object from the array. If you want to
* change a
SizeConstraintSetUpdate
object, you delete the
* existing object and add a new one.
* - The part of a web request that you want AWS WAF to evaluate, such as
* the length of a query string or the length of the
User-Agent
* header.
* - Whether to perform any transformations on the request, such as
* converting it to lowercase, before checking its length. Note that
* transformations of the request body are not supported because the AWS
* resource forwards only the first
8192
bytes of your request
* to AWS WAF.
* - A
ComparisonOperator
used for evaluating the selected
* part of the request against the specified Size
, such as
* equals, greater than, less than, and so on.
* - The length, in bytes, that you want AWS WAF to watch for in selected
* part of the request. The length is computed after applying the
* transformation.
*
*
* For example, you can add a SizeConstraintSetUpdate
object
* that matches web requests in which the length of the
* User-Agent
header is greater than 100 bytes. You can then
* configure AWS WAF to block those requests.
*
*
* To create and configure a SizeConstraintSet
, perform the
* following steps:
*
*
* - Create a
SizeConstraintSet.
For more information, see
* CreateSizeConstraintSet.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of an
* UpdateSizeConstraintSet
request.
* - Submit an
UpdateSizeConstraintSet
request to specify the
* part of the request that you want AWS WAF to inspect (for example, the
* header or the URI) and the value that you want AWS WAF to watch for.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateSizeConstraintSetRequest
* @return Result of the UpdateSizeConstraintSet operation returned by the
* service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateSizeConstraintSet
*/
@Override
public UpdateSizeConstraintSetResult updateSizeConstraintSet(
UpdateSizeConstraintSetRequest updateSizeConstraintSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateSizeConstraintSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSizeConstraintSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateSizeConstraintSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateSizeConstraintSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes SqlInjectionMatchTuple objects (filters) in a
* SqlInjectionMatchSet. For each SqlInjectionMatchTuple
* object, you specify the following values:
*
*
* Action
: Whether to insert the object into or delete the
* object from the array. To change a SqlInjectionMatchTuple
,
* you delete the existing object and add a new one.
* FieldToMatch
: The part of web requests that you want AWS
* WAF to inspect and, if you want AWS WAF to inspect a header, the name of
* the header.
* TextTransformation
: Which text transformation, if any,
* to perform on the web request before inspecting the request for snippets
* of malicious SQL code.
*
*
* You use SqlInjectionMatchSet
objects to specify which
* CloudFront requests you want to allow, block, or count. For example, if
* you're receiving requests that contain snippets of SQL code in the query
* string and you want to block the requests, you can create a
* SqlInjectionMatchSet
with the applicable settings, and then
* configure AWS WAF to block the requests.
*
*
* To create and configure a SqlInjectionMatchSet
, perform the
* following steps:
*
*
* - Submit a CreateSqlInjectionMatchSet request.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of an UpdateIPSet request.
* - Submit an
UpdateSqlInjectionMatchSet
request to specify
* the parts of web requests that you want AWS WAF to inspect for snippets
* of SQL code.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateSqlInjectionMatchSetRequest
* A request to update a SqlInjectionMatchSet.
* @return Result of the UpdateSqlInjectionMatchSet operation returned by
* the service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateSqlInjectionMatchSet
*/
@Override
public UpdateSqlInjectionMatchSetResult updateSqlInjectionMatchSet(
UpdateSqlInjectionMatchSetRequest updateSqlInjectionMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateSqlInjectionMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSqlInjectionMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateSqlInjectionMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateSqlInjectionMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes ActivatedRule objects in a WebACL
.
* Each Rule
identifies web requests that you want to allow,
* block, or count. When you update a WebACL
, you specify the
* following values:
*
*
* - A default action for the
WebACL
, either
* ALLOW
or BLOCK
. AWS WAF performs the default
* action if a request doesn't match the criteria in any of the
* Rules
in a WebACL
.
* - The
Rules
that you want to add and/or delete. If you
* want to replace one Rule
with another, you delete the
* existing Rule
and add the new one.
* - For each
Rule
, whether you want AWS WAF to allow
* requests, block requests, or count requests that match the conditions in
* the Rule
.
* - The order in which you want AWS WAF to evaluate the
*
Rules
in a WebACL
. If you add more than one
* Rule
to a WebACL
, AWS WAF evaluates each
* request against the Rules
in order based on the value of
* Priority
. (The Rule
that has the lowest value
* for Priority
is evaluated first.) When a web request matches
* all of the predicates (such as ByteMatchSets
and
* IPSets
) in a Rule
, AWS WAF immediately takes
* the corresponding action, allow or block, and doesn't evaluate the
* request against the remaining Rules
in the
* WebACL
, if any.
* - The CloudFront distribution that you want to associate with the
*
WebACL
.
*
*
* To create and configure a WebACL
, perform the following
* steps:
*
*
* - Create and update the predicates that you want to include in
*
Rules
. For more information, see CreateByteMatchSet,
* UpdateByteMatchSet, CreateIPSet, UpdateIPSet,
* CreateSqlInjectionMatchSet, and UpdateSqlInjectionMatchSet.
*
* - Create and update the
Rules
that you want to include in
* the WebACL
. For more information, see CreateRule and
* UpdateRule.
* - Create a
WebACL
. See CreateWebACL.
* - Use
GetChangeToken
to get the change token that you
* provide in the ChangeToken
parameter of an
* UpdateWebACL request.
* - Submit an
UpdateWebACL
request to specify the
* Rules
that you want to include in the WebACL
,
* to specify the default action, and to associate the WebACL
* with a CloudFront distribution.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateWebACLRequest
* @return Result of the UpdateWebACL operation returned by the service.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFReferencedItemException
* The operation failed because you tried to delete an object that
* is still in use. For example:
*
* - You tried to delete a
ByteMatchSet
that is still
* referenced by a Rule
.
* - You tried to delete a
Rule
that is still
* referenced by a WebACL
.
*
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateWebACL
*/
@Override
public UpdateWebACLResult updateWebACL(
UpdateWebACLRequest updateWebACLRequest) {
ExecutionContext executionContext = createExecutionContext(updateWebACLRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateWebACLRequestMarshaller(protocolFactory)
.marshall(super.beforeMarshalling(updateWebACLRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateWebACLResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Inserts or deletes XssMatchTuple objects (filters) in an
* XssMatchSet. For each XssMatchTuple
object, you
* specify the following values:
*
*
* Action
: Whether to insert the object into or delete the
* object from the array. To change a XssMatchTuple
, you delete
* the existing object and add a new one.
* FieldToMatch
: The part of web requests that you want AWS
* WAF to inspect and, if you want AWS WAF to inspect a header, the name of
* the header.
* TextTransformation
: Which text transformation, if any,
* to perform on the web request before inspecting the request for
* cross-site scripting attacks.
*
*
* You use XssMatchSet
objects to specify which CloudFront
* requests you want to allow, block, or count. For example, if you're
* receiving requests that contain cross-site scripting attacks in the
* request body and you want to block the requests, you can create an
* XssMatchSet
with the applicable settings, and then configure
* AWS WAF to block the requests.
*
*
* To create and configure an XssMatchSet
, perform the
* following steps:
*
*
* - Submit a CreateXssMatchSet request.
* - Use GetChangeToken to get the change token that you provide in
* the
ChangeToken
parameter of an UpdateIPSet request.
* - Submit an
UpdateXssMatchSet
request to specify the parts
* of web requests that you want AWS WAF to inspect for cross-site scripting
* attacks.
*
*
* For more information about how to use the AWS WAF API to allow or block
* HTTP requests, see the AWS WAF
* Developer Guide.
*
*
* @param updateXssMatchSetRequest
* A request to update an XssMatchSet.
* @return Result of the UpdateXssMatchSet operation returned by the
* service.
* @throws WAFInternalErrorException
* The operation failed because of a system problem, even though the
* request was valid. Retry your request.
* @throws WAFInvalidAccountException
* The operation failed because you tried to create, update, or
* delete an object by using an invalid account identifier.
* @throws WAFInvalidOperationException
* The operation failed because there was nothing to do. For
* example:
*
* - You tried to remove a
Rule
from a
* WebACL
, but the Rule
isn't in the
* specified WebACL
.
* - You tried to remove an IP address from an
IPSet
,
* but the IP address isn't in the specified IPSet
.
* - You tried to remove a
ByteMatchTuple
from a
* ByteMatchSet
, but the ByteMatchTuple
* isn't in the specified WebACL
.
* - You tried to add a
Rule
to a WebACL
* , but the Rule
already exists in the specified
* WebACL
.
* - You tried to add an IP address to an
IPSet
, but
* the IP address already exists in the specified IPSet
* .
* - You tried to add a
ByteMatchTuple
to a
* ByteMatchSet
, but the ByteMatchTuple
* already exists in the specified WebACL
.
* @throws WAFInvalidParameterException
* The operation failed because AWS WAF didn't recognize a parameter
* in the request. For example:
*
* - You specified an invalid parameter name.
* - You specified an invalid value.
* - You tried to update an object (
ByteMatchSet
,
* IPSet
, Rule
, or WebACL
)
* using an action other than INSERT
or
* DELETE
.
* - You tried to create a
WebACL
with a
* DefaultAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
WebACL
with a
* WafAction
Type
other than
* ALLOW
, BLOCK
, or COUNT
.
* - You tried to update a
ByteMatchSet
with a
* FieldToMatch
Type
other than HEADER,
* QUERY_STRING, or URI.
* - You tried to update a
ByteMatchSet
with a
* Field
of HEADER
but no value for
* Data
.
* @throws WAFNonexistentContainerException
* The operation failed because you tried to add an object to or
* delete an object from another object that doesn't exist. For
* example:
*
* - You tried to add a
Rule
to or delete a
* Rule
from a WebACL
that doesn't exist.
* - You tried to add a
ByteMatchSet
to or delete a
* ByteMatchSet
from a Rule
that doesn't
* exist.
* - You tried to add an IP address to or delete an IP address
* from an
IPSet
that doesn't exist.
* - You tried to add a
ByteMatchTuple
to or delete a
* ByteMatchTuple
from a ByteMatchSet
that
* doesn't exist.
* @throws WAFNonexistentItemException
* The operation failed because the referenced object doesn't exist.
* @throws WAFStaleDataException
* The operation failed because you tried to create, update, or
* delete an object by using a change token that has already been
* used.
* @throws WAFLimitsExceededException
* The operation exceeds a resource limit, for example, the maximum
* number of WebACL
objects that you can create for an
* AWS account. For more information, see Limits in the AWS WAF Developer Guide.
* @sample AWSWAF.UpdateXssMatchSet
*/
@Override
public UpdateXssMatchSetResult updateXssMatchSet(
UpdateXssMatchSetRequest updateXssMatchSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateXssMatchSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateXssMatchSetRequestMarshaller(
protocolFactory).marshall(super
.beforeMarshalling(updateXssMatchSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true)
.withHasStreamingSuccessResponse(false),
new UpdateXssMatchSetResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(
AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be
* overriden at the request level.
**/
private Response invoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils
.getCredentialsProvider(request.getOriginalRequest(),
awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any
* credentials set on the client or request will be ignored for this
* operation.
**/
private Response anonymousInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack
* thereof) have been configured in the ExecutionContext beforehand.
**/
private Response doInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory
.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler,
executionContext);
}
}