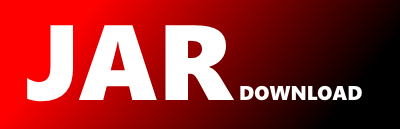
com.amazonaws.services.waf.model.ByteMatchSet Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.waf.model;
import java.io.Serializable;
/**
*
* In a GetByteMatchSet request, ByteMatchSet
is a complex
* type that contains the ByteMatchSetId
and Name
of a
* ByteMatchSet
, and the values that you specified when you updated
* the ByteMatchSet
.
*
*
* A complex type that contains ByteMatchTuple
objects, which
* specify the parts of web requests that you want AWS WAF to inspect and the
* values that you want AWS WAF to search for. If a ByteMatchSet
* contains more than one ByteMatchTuple
object, a request needs to
* match the settings in only one ByteMatchTuple
to be considered a
* match.
*
*/
public class ByteMatchSet implements Serializable, Cloneable {
/**
*
* The ByteMatchSetId
for a ByteMatchSet
. You use
* ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert a
* ByteMatchSet
into a Rule
or delete one from a
* Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see DeleteByteMatchSet).
*
*
* ByteMatchSetId
is returned by CreateByteMatchSet and
* by ListByteMatchSets.
*
*/
private String byteMatchSetId;
/**
*
* A friendly name or description of the ByteMatchSet. You can't
* change Name
after you create a ByteMatchSet
.
*
*/
private String name;
/**
*
* Specifies the bytes (typically a string that corresponds with ASCII
* characters) that you want AWS WAF to search for in web requests, the
* location in requests that you want AWS WAF to search, and other settings.
*
*/
private java.util.List byteMatchTuples;
/**
*
* The ByteMatchSetId
for a ByteMatchSet
. You use
* ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert a
* ByteMatchSet
into a Rule
or delete one from a
* Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see DeleteByteMatchSet).
*
*
* ByteMatchSetId
is returned by CreateByteMatchSet and
* by ListByteMatchSets.
*
*
* @param byteMatchSetId
* The ByteMatchSetId
for a ByteMatchSet
.
* You use ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert
* a ByteMatchSet
into a Rule
or delete one
* from a Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see
* DeleteByteMatchSet).
*
* ByteMatchSetId
is returned by
* CreateByteMatchSet and by ListByteMatchSets.
*/
public void setByteMatchSetId(String byteMatchSetId) {
this.byteMatchSetId = byteMatchSetId;
}
/**
*
* The ByteMatchSetId
for a ByteMatchSet
. You use
* ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert a
* ByteMatchSet
into a Rule
or delete one from a
* Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see DeleteByteMatchSet).
*
*
* ByteMatchSetId
is returned by CreateByteMatchSet and
* by ListByteMatchSets.
*
*
* @return The ByteMatchSetId
for a ByteMatchSet
.
* You use ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert
* a ByteMatchSet
into a Rule
or delete
* one from a Rule
(see UpdateRule), and delete
* a ByteMatchSet
from AWS WAF (see
* DeleteByteMatchSet).
*
* ByteMatchSetId
is returned by
* CreateByteMatchSet and by ListByteMatchSets.
*/
public String getByteMatchSetId() {
return this.byteMatchSetId;
}
/**
*
* The ByteMatchSetId
for a ByteMatchSet
. You use
* ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert a
* ByteMatchSet
into a Rule
or delete one from a
* Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see DeleteByteMatchSet).
*
*
* ByteMatchSetId
is returned by CreateByteMatchSet and
* by ListByteMatchSets.
*
*
* @param byteMatchSetId
* The ByteMatchSetId
for a ByteMatchSet
.
* You use ByteMatchSetId
to get information about a
* ByteMatchSet
(see GetByteMatchSet), update a
* ByteMatchSet
(see UpdateByteMatchSet), insert
* a ByteMatchSet
into a Rule
or delete one
* from a Rule
(see UpdateRule), and delete a
* ByteMatchSet
from AWS WAF (see
* DeleteByteMatchSet).
*
* ByteMatchSetId
is returned by
* CreateByteMatchSet and by ListByteMatchSets.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchSet withByteMatchSetId(String byteMatchSetId) {
setByteMatchSetId(byteMatchSetId);
return this;
}
/**
*
* A friendly name or description of the ByteMatchSet. You can't
* change Name
after you create a ByteMatchSet
.
*
*
* @param name
* A friendly name or description of the ByteMatchSet. You
* can't change Name
after you create a
* ByteMatchSet
.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A friendly name or description of the ByteMatchSet. You can't
* change Name
after you create a ByteMatchSet
.
*
*
* @return A friendly name or description of the ByteMatchSet. You
* can't change Name
after you create a
* ByteMatchSet
.
*/
public String getName() {
return this.name;
}
/**
*
* A friendly name or description of the ByteMatchSet. You can't
* change Name
after you create a ByteMatchSet
.
*
*
* @param name
* A friendly name or description of the ByteMatchSet. You
* can't change Name
after you create a
* ByteMatchSet
.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchSet withName(String name) {
setName(name);
return this;
}
/**
*
* Specifies the bytes (typically a string that corresponds with ASCII
* characters) that you want AWS WAF to search for in web requests, the
* location in requests that you want AWS WAF to search, and other settings.
*
*
* @return Specifies the bytes (typically a string that corresponds with
* ASCII characters) that you want AWS WAF to search for in web
* requests, the location in requests that you want AWS WAF to
* search, and other settings.
*/
public java.util.List getByteMatchTuples() {
return byteMatchTuples;
}
/**
*
* Specifies the bytes (typically a string that corresponds with ASCII
* characters) that you want AWS WAF to search for in web requests, the
* location in requests that you want AWS WAF to search, and other settings.
*
*
* @param byteMatchTuples
* Specifies the bytes (typically a string that corresponds with
* ASCII characters) that you want AWS WAF to search for in web
* requests, the location in requests that you want AWS WAF to
* search, and other settings.
*/
public void setByteMatchTuples(
java.util.Collection byteMatchTuples) {
if (byteMatchTuples == null) {
this.byteMatchTuples = null;
return;
}
this.byteMatchTuples = new java.util.ArrayList(
byteMatchTuples);
}
/**
*
* Specifies the bytes (typically a string that corresponds with ASCII
* characters) that you want AWS WAF to search for in web requests, the
* location in requests that you want AWS WAF to search, and other settings.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setByteMatchTuples(java.util.Collection)} or
* {@link #withByteMatchTuples(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param byteMatchTuples
* Specifies the bytes (typically a string that corresponds with
* ASCII characters) that you want AWS WAF to search for in web
* requests, the location in requests that you want AWS WAF to
* search, and other settings.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchSet withByteMatchTuples(ByteMatchTuple... byteMatchTuples) {
if (this.byteMatchTuples == null) {
setByteMatchTuples(new java.util.ArrayList(
byteMatchTuples.length));
}
for (ByteMatchTuple ele : byteMatchTuples) {
this.byteMatchTuples.add(ele);
}
return this;
}
/**
*
* Specifies the bytes (typically a string that corresponds with ASCII
* characters) that you want AWS WAF to search for in web requests, the
* location in requests that you want AWS WAF to search, and other settings.
*
*
* @param byteMatchTuples
* Specifies the bytes (typically a string that corresponds with
* ASCII characters) that you want AWS WAF to search for in web
* requests, the location in requests that you want AWS WAF to
* search, and other settings.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchSet withByteMatchTuples(
java.util.Collection byteMatchTuples) {
setByteMatchTuples(byteMatchTuples);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getByteMatchSetId() != null)
sb.append("ByteMatchSetId: " + getByteMatchSetId() + ",");
if (getName() != null)
sb.append("Name: " + getName() + ",");
if (getByteMatchTuples() != null)
sb.append("ByteMatchTuples: " + getByteMatchTuples());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ByteMatchSet == false)
return false;
ByteMatchSet other = (ByteMatchSet) obj;
if (other.getByteMatchSetId() == null
^ this.getByteMatchSetId() == null)
return false;
if (other.getByteMatchSetId() != null
&& other.getByteMatchSetId().equals(this.getByteMatchSetId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null
&& other.getName().equals(this.getName()) == false)
return false;
if (other.getByteMatchTuples() == null
^ this.getByteMatchTuples() == null)
return false;
if (other.getByteMatchTuples() != null
&& other.getByteMatchTuples().equals(this.getByteMatchTuples()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getByteMatchSetId() == null) ? 0 : getByteMatchSetId()
.hashCode());
hashCode = prime * hashCode
+ ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime
* hashCode
+ ((getByteMatchTuples() == null) ? 0 : getByteMatchTuples()
.hashCode());
return hashCode;
}
@Override
public ByteMatchSet clone() {
try {
return (ByteMatchSet) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}