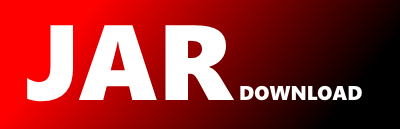
com.amazonaws.services.waf.model.SizeConstraint Maven / Gradle / Ivy
Show all versions of aws-java-sdk-waf Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.waf.model;
import java.io.Serializable;
/**
*
* Specifies a constraint on the size of a part of the web request. AWS WAF uses
* the Size
, ComparisonOperator
, and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*/
public class SizeConstraint implements Serializable, Cloneable {
private FieldToMatch fieldToMatch;
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*/
private String textTransformation;
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*/
private String comparisonOperator;
/**
*
* The size in bytes that you want AWS WAF to compare against the size of
* the specified FieldToMatch
. AWS WAF uses this in combination
* with ComparisonOperator
and FieldToMatch
to
* build an expression in the form of "Size
* ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
, the /
* in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
*
*/
private Long size;
/**
* @param fieldToMatch
*/
public void setFieldToMatch(FieldToMatch fieldToMatch) {
this.fieldToMatch = fieldToMatch;
}
/**
* @return
*/
public FieldToMatch getFieldToMatch() {
return this.fieldToMatch;
}
/**
* @param fieldToMatch
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public SizeConstraint withFieldToMatch(FieldToMatch fieldToMatch) {
setFieldToMatch(fieldToMatch);
return this;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on FieldToMatch
before inspecting a request for a
* match.
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only
* the first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system command line command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
* @see TextTransformation
*/
public void setTextTransformation(String textTransformation) {
this.textTransformation = textTransformation;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* @return Text transformations eliminate some of the unusual formatting
* that attackers use in web requests in an effort to bypass AWS
* WAF. If you specify a transformation, AWS WAF performs the
* transformation on FieldToMatch
before inspecting a
* request for a match.
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only
* the first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system command line command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the
* following operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than"
* symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
* @see TextTransformation
*/
public String getTextTransformation() {
return this.textTransformation;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on FieldToMatch
before inspecting a request for a
* match.
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only
* the first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system command line command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see TextTransformation
*/
public SizeConstraint withTextTransformation(String textTransformation) {
setTextTransformation(textTransformation);
return this;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on FieldToMatch
before inspecting a request for a
* match.
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only
* the first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system command line command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
* @see TextTransformation
*/
public void setTextTransformation(TextTransformation textTransformation) {
this.textTransformation = textTransformation.toString();
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* FieldToMatch
before inspecting a request for a match.
*
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only the
* first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* command line command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on FieldToMatch
before inspecting a request for a
* match.
*
* Note that if you choose BODY
for the value of
* Type
, you must choose NONE
for
* TextTransformation
because CloudFront forwards only
* the first 8192 bytes for inspection.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system command line command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see TextTransformation
*/
public SizeConstraint withTextTransformation(
TextTransformation textTransformation) {
setTextTransformation(textTransformation);
return this;
}
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*
* @param comparisonOperator
* The type of comparison you want AWS WAF to perform. AWS WAF uses
* this in combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* EQ: Used to test if the Size
is equal to the
* size of the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to
* the size of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or
* equal to the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less
* than the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than
* or equal to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly
* greater than the size of the FieldToMatch
* @see ComparisonOperator
*/
public void setComparisonOperator(String comparisonOperator) {
this.comparisonOperator = comparisonOperator;
}
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*
* @return The type of comparison you want AWS WAF to perform. AWS WAF uses
* this in combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes
* of FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* EQ: Used to test if the Size
is equal to the
* size of the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to
* the size of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or
* equal to the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less
* than the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than
* or equal to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly
* greater than the size of the FieldToMatch
* @see ComparisonOperator
*/
public String getComparisonOperator() {
return this.comparisonOperator;
}
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*
* @param comparisonOperator
* The type of comparison you want AWS WAF to perform. AWS WAF uses
* this in combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* EQ: Used to test if the Size
is equal to the
* size of the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to
* the size of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or
* equal to the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less
* than the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than
* or equal to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly
* greater than the size of the FieldToMatch
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ComparisonOperator
*/
public SizeConstraint withComparisonOperator(String comparisonOperator) {
setComparisonOperator(comparisonOperator);
return this;
}
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*
* @param comparisonOperator
* The type of comparison you want AWS WAF to perform. AWS WAF uses
* this in combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* EQ: Used to test if the Size
is equal to the
* size of the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to
* the size of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or
* equal to the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less
* than the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than
* or equal to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly
* greater than the size of the FieldToMatch
* @see ComparisonOperator
*/
public void setComparisonOperator(ComparisonOperator comparisonOperator) {
this.comparisonOperator = comparisonOperator.toString();
}
/**
*
* The type of comparison you want AWS WAF to perform. AWS WAF uses this in
* combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* EQ: Used to test if the Size
is equal to the size of
* the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to the size
* of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or equal to
* the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less than
* the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than or equal
* to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly greater than
* the size of the FieldToMatch
*
*
* @param comparisonOperator
* The type of comparison you want AWS WAF to perform. AWS WAF uses
* this in combination with the provided Size
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* EQ: Used to test if the Size
is equal to the
* size of the FieldToMatch
*
*
* NE: Used to test if the Size
is not equal to
* the size of the FieldToMatch
*
*
* LE: Used to test if the Size
is less than or
* equal to the size of the FieldToMatch
*
*
* LT: Used to test if the Size
is strictly less
* than the size of the FieldToMatch
*
*
* GE: Used to test if the Size
is greater than
* or equal to the size of the FieldToMatch
*
*
* GT: Used to test if the Size
is strictly
* greater than the size of the FieldToMatch
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see ComparisonOperator
*/
public SizeConstraint withComparisonOperator(
ComparisonOperator comparisonOperator) {
setComparisonOperator(comparisonOperator);
return this;
}
/**
*
* The size in bytes that you want AWS WAF to compare against the size of
* the specified FieldToMatch
. AWS WAF uses this in combination
* with ComparisonOperator
and FieldToMatch
to
* build an expression in the form of "Size
* ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
, the /
* in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
*
*
* @param size
* The size in bytes that you want AWS WAF to compare against the
* size of the specified FieldToMatch
. AWS WAF uses this
* in combination with ComparisonOperator
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
* , the / in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
*/
public void setSize(Long size) {
this.size = size;
}
/**
*
* The size in bytes that you want AWS WAF to compare against the size of
* the specified FieldToMatch
. AWS WAF uses this in combination
* with ComparisonOperator
and FieldToMatch
to
* build an expression in the form of "Size
* ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
, the /
* in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
*
*
* @return The size in bytes that you want AWS WAF to compare against the
* size of the specified FieldToMatch
. AWS WAF uses
* this in combination with ComparisonOperator
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes
* of FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of
* Type
, the / in the URI counts as one character. For
* example, the URI /logo.jpg
is nine characters long.
*/
public Long getSize() {
return this.size;
}
/**
*
* The size in bytes that you want AWS WAF to compare against the size of
* the specified FieldToMatch
. AWS WAF uses this in combination
* with ComparisonOperator
and FieldToMatch
to
* build an expression in the form of "Size
* ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
, the /
* in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
*
*
* @param size
* The size in bytes that you want AWS WAF to compare against the
* size of the specified FieldToMatch
. AWS WAF uses this
* in combination with ComparisonOperator
and
* FieldToMatch
to build an expression in the form of "
* Size
ComparisonOperator
size in bytes of
* FieldToMatch
". If that expression is true, the
* SizeConstraint
is considered to match.
*
* Valid values for size are 0 - 21474836480 bytes (0 - 20 GB).
*
*
* If you specify URI
for the value of Type
* , the / in the URI counts as one character. For example, the URI
* /logo.jpg
is nine characters long.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public SizeConstraint withSize(Long size) {
setSize(size);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFieldToMatch() != null)
sb.append("FieldToMatch: " + getFieldToMatch() + ",");
if (getTextTransformation() != null)
sb.append("TextTransformation: " + getTextTransformation() + ",");
if (getComparisonOperator() != null)
sb.append("ComparisonOperator: " + getComparisonOperator() + ",");
if (getSize() != null)
sb.append("Size: " + getSize());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SizeConstraint == false)
return false;
SizeConstraint other = (SizeConstraint) obj;
if (other.getFieldToMatch() == null ^ this.getFieldToMatch() == null)
return false;
if (other.getFieldToMatch() != null
&& other.getFieldToMatch().equals(this.getFieldToMatch()) == false)
return false;
if (other.getTextTransformation() == null
^ this.getTextTransformation() == null)
return false;
if (other.getTextTransformation() != null
&& other.getTextTransformation().equals(
this.getTextTransformation()) == false)
return false;
if (other.getComparisonOperator() == null
^ this.getComparisonOperator() == null)
return false;
if (other.getComparisonOperator() != null
&& other.getComparisonOperator().equals(
this.getComparisonOperator()) == false)
return false;
if (other.getSize() == null ^ this.getSize() == null)
return false;
if (other.getSize() != null
&& other.getSize().equals(this.getSize()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getFieldToMatch() == null) ? 0 : getFieldToMatch()
.hashCode());
hashCode = prime
* hashCode
+ ((getTextTransformation() == null) ? 0
: getTextTransformation().hashCode());
hashCode = prime
* hashCode
+ ((getComparisonOperator() == null) ? 0
: getComparisonOperator().hashCode());
hashCode = prime * hashCode
+ ((getSize() == null) ? 0 : getSize().hashCode());
return hashCode;
}
@Override
public SizeConstraint clone() {
try {
return (SizeConstraint) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}