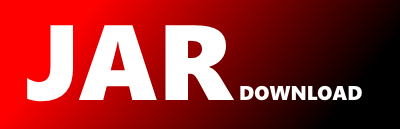
com.amazonaws.services.waf.model.ByteMatchTuple Maven / Gradle / Ivy
Show all versions of aws-java-sdk-waf Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.waf.model;
import java.io.Serializable;
/**
*
* The bytes (typically a string that corresponds with ASCII characters) that
* you want AWS WAF to search for in web requests, the location in requests that
* you want AWS WAF to search, and other settings.
*
*/
public class ByteMatchTuple implements Serializable, Cloneable {
/**
*
* The part of a web request that you want AWS WAF to search, such as a
* specified header or a query string. For more information, see
* FieldToMatch.
*
*/
private FieldToMatch fieldToMatch;
/**
*
* The value that you want AWS WAF to search for. AWS WAF searches for the
* specified string in the part of web requests that you specified in
* FieldToMatch
. The maximum length of the value is 50 bytes.
*
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search for in
* the request header that you specified in FieldToMatch, for
* example, the value of the User-Agent
or Referer
* header.
* METHOD
: The HTTP method, which indicates the type of
* operation specified in the request. CloudFront supports the following
* methods: DELETE
, GET
, HEAD
,
* OPTIONS
, PATCH
, POST
, and
* PUT
.
* QUERY_STRING
: The value that you want AWS WAF to search
* for in the query string, which is the part of a URL that appears after a
* ?
character.
* URI
: The value that you want AWS WAF to search for in
* the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any additional
* data that you want to send to your web server as the HTTP request body,
* such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the
* request body are forwarded to AWS WAF for inspection. To allow or block
* requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
*
* If TargetString
includes alphabetic characters A-Z and a-z,
* note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length of the
* value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
, you
* base64-encode BadBot
using MIME base64 encoding and include
* the resulting value, QmFkQm90
, in the value of
* TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK automatically
* base64 encodes the value.
*
*/
private java.nio.ByteBuffer targetString;
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*/
private String textTransformation;
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*/
private String positionalConstraint;
/**
*
* The part of a web request that you want AWS WAF to search, such as a
* specified header or a query string. For more information, see
* FieldToMatch.
*
*
* @param fieldToMatch
* The part of a web request that you want AWS WAF to search, such as
* a specified header or a query string. For more information, see
* FieldToMatch.
*/
public void setFieldToMatch(FieldToMatch fieldToMatch) {
this.fieldToMatch = fieldToMatch;
}
/**
*
* The part of a web request that you want AWS WAF to search, such as a
* specified header or a query string. For more information, see
* FieldToMatch.
*
*
* @return The part of a web request that you want AWS WAF to search, such
* as a specified header or a query string. For more information,
* see FieldToMatch.
*/
public FieldToMatch getFieldToMatch() {
return this.fieldToMatch;
}
/**
*
* The part of a web request that you want AWS WAF to search, such as a
* specified header or a query string. For more information, see
* FieldToMatch.
*
*
* @param fieldToMatch
* The part of a web request that you want AWS WAF to search, such as
* a specified header or a query string. For more information, see
* FieldToMatch.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchTuple withFieldToMatch(FieldToMatch fieldToMatch) {
setFieldToMatch(fieldToMatch);
return this;
}
/**
*
* The value that you want AWS WAF to search for. AWS WAF searches for the
* specified string in the part of web requests that you specified in
* FieldToMatch
. The maximum length of the value is 50 bytes.
*
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search for in
* the request header that you specified in FieldToMatch, for
* example, the value of the User-Agent
or Referer
* header.
* METHOD
: The HTTP method, which indicates the type of
* operation specified in the request. CloudFront supports the following
* methods: DELETE
, GET
, HEAD
,
* OPTIONS
, PATCH
, POST
, and
* PUT
.
* QUERY_STRING
: The value that you want AWS WAF to search
* for in the query string, which is the part of a URL that appears after a
* ?
character.
* URI
: The value that you want AWS WAF to search for in
* the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any additional
* data that you want to send to your web server as the HTTP request body,
* such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the
* request body are forwarded to AWS WAF for inspection. To allow or block
* requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
*
* If TargetString
includes alphabetic characters A-Z and a-z,
* note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length of the
* value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
, you
* base64-encode BadBot
using MIME base64 encoding and include
* the resulting value, QmFkQm90
, in the value of
* TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK automatically
* base64 encodes the value.
*
*
* AWS SDK for Java performs a Base64 encoding on this field before sending
* this request to AWS service by default. Users of the SDK should not
* perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the
* content or position of the byte buffer will be seen by all objects that
* have a reference to this object. It is recommended to call
* ByteBuffer.duplicate() or ByteBuffer.asReadOnlyBuffer() before using or
* reading from the buffer. This behavior will be changed in a future major
* version of the SDK.
*
*
* @param targetString
* The value that you want AWS WAF to search for. AWS WAF searches
* for the specified string in the part of web requests that you
* specified in FieldToMatch
. The maximum length of the
* value is 50 bytes.
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search
* for in the request header that you specified in
* FieldToMatch, for example, the value of the
* User-Agent
or Referer
header.
* METHOD
: The HTTP method, which indicates the type
* of operation specified in the request. CloudFront supports the
* following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
,
* POST
, and PUT
.
* QUERY_STRING
: The value that you want AWS WAF to
* search for in the query string, which is the part of a URL that
* appears after a ?
character.
* URI
: The value that you want AWS WAF to search
* for in the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any
* additional data that you want to send to your web server as the
* HTTP request body, such as data from a form. The request body
* immediately follows the request headers. Note that only the first
* 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length
* of the body, you can create a size constraint set. For more
* information, see CreateSizeConstraintSet.
*
*
* If TargetString
includes alphabetic characters A-Z
* and a-z, note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length
* of the value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
,
* you base64-encode BadBot
using MIME base64 encoding
* and include the resulting value, QmFkQm90
, in the
* value of TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK
* automatically base64 encodes the value.
*/
public void setTargetString(java.nio.ByteBuffer targetString) {
this.targetString = targetString;
}
/**
*
* The value that you want AWS WAF to search for. AWS WAF searches for the
* specified string in the part of web requests that you specified in
* FieldToMatch
. The maximum length of the value is 50 bytes.
*
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search for in
* the request header that you specified in FieldToMatch, for
* example, the value of the User-Agent
or Referer
* header.
* METHOD
: The HTTP method, which indicates the type of
* operation specified in the request. CloudFront supports the following
* methods: DELETE
, GET
, HEAD
,
* OPTIONS
, PATCH
, POST
, and
* PUT
.
* QUERY_STRING
: The value that you want AWS WAF to search
* for in the query string, which is the part of a URL that appears after a
* ?
character.
* URI
: The value that you want AWS WAF to search for in
* the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any additional
* data that you want to send to your web server as the HTTP request body,
* such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the
* request body are forwarded to AWS WAF for inspection. To allow or block
* requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
*
* If TargetString
includes alphabetic characters A-Z and a-z,
* note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length of the
* value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
, you
* base64-encode BadBot
using MIME base64 encoding and include
* the resulting value, QmFkQm90
, in the value of
* TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK automatically
* base64 encodes the value.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods
* changes their {@code position}. We recommend using
* {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view
* of the buffer with an independent {@code position}, and calling
* {@code get} methods on this rather than directly on the returned
* {@code ByteBuffer}. Doing so will ensure that anyone else using the
* {@code ByteBuffer} will not be affected by changes to the {@code position}
* .
*
*
* @return The value that you want AWS WAF to search for. AWS WAF searches
* for the specified string in the part of web requests that you
* specified in FieldToMatch
. The maximum length of the
* value is 50 bytes.
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to
* search for in the request header that you specified in
* FieldToMatch, for example, the value of the
* User-Agent
or Referer
header.
* METHOD
: The HTTP method, which indicates the
* type of operation specified in the request. CloudFront supports
* the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
,
* POST
, and PUT
.
* QUERY_STRING
: The value that you want AWS WAF to
* search for in the query string, which is the part of a URL that
* appears after a ?
character.
* URI
: The value that you want AWS WAF to search
* for in the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any
* additional data that you want to send to your web server as the
* HTTP request body, such as data from a form. The request body
* immediately follows the request headers. Note that only the first
* 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the
* length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* If TargetString
includes alphabetic characters A-Z
* and a-z, note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length
* of the value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
,
* you base64-encode BadBot
using MIME base64 encoding
* and include the resulting value, QmFkQm90
, in the
* value of TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK
* automatically base64 encodes the value.
*/
public java.nio.ByteBuffer getTargetString() {
return this.targetString;
}
/**
*
* The value that you want AWS WAF to search for. AWS WAF searches for the
* specified string in the part of web requests that you specified in
* FieldToMatch
. The maximum length of the value is 50 bytes.
*
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search for in
* the request header that you specified in FieldToMatch, for
* example, the value of the User-Agent
or Referer
* header.
* METHOD
: The HTTP method, which indicates the type of
* operation specified in the request. CloudFront supports the following
* methods: DELETE
, GET
, HEAD
,
* OPTIONS
, PATCH
, POST
, and
* PUT
.
* QUERY_STRING
: The value that you want AWS WAF to search
* for in the query string, which is the part of a URL that appears after a
* ?
character.
* URI
: The value that you want AWS WAF to search for in
* the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any additional
* data that you want to send to your web server as the HTTP request body,
* such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the
* request body are forwarded to AWS WAF for inspection. To allow or block
* requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
*
* If TargetString
includes alphabetic characters A-Z and a-z,
* note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length of the
* value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
, you
* base64-encode BadBot
using MIME base64 encoding and include
* the resulting value, QmFkQm90
, in the value of
* TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK automatically
* base64 encodes the value.
*
*
* @param targetString
* The value that you want AWS WAF to search for. AWS WAF searches
* for the specified string in the part of web requests that you
* specified in FieldToMatch
. The maximum length of the
* value is 50 bytes.
*
* Valid values depend on the values that you specified for
* FieldToMatch
:
*
*
* HEADER
: The value that you want AWS WAF to search
* for in the request header that you specified in
* FieldToMatch, for example, the value of the
* User-Agent
or Referer
header.
* METHOD
: The HTTP method, which indicates the type
* of operation specified in the request. CloudFront supports the
* following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
,
* POST
, and PUT
.
* QUERY_STRING
: The value that you want AWS WAF to
* search for in the query string, which is the part of a URL that
* appears after a ?
character.
* URI
: The value that you want AWS WAF to search
* for in the part of a URL that identifies a resource, for example,
* /images/daily-ad.jpg
.
* BODY
: The part of a request that contains any
* additional data that you want to send to your web server as the
* HTTP request body, such as data from a form. The request body
* immediately follows the request headers. Note that only the first
* 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length
* of the body, you can create a size constraint set. For more
* information, see CreateSizeConstraintSet.
*
*
* If TargetString
includes alphabetic characters A-Z
* and a-z, note that the value is case sensitive.
*
*
* If you're using the AWS WAF API
*
*
* Specify a base64-encoded version of the value. The maximum length
* of the value before you base64-encode it is 50 bytes.
*
*
* For example, suppose the value of Type
is
* HEADER
and the value of Data
is
* User-Agent
. If you want to search the
* User-Agent
header for the value BadBot
,
* you base64-encode BadBot
using MIME base64 encoding
* and include the resulting value, QmFkQm90
, in the
* value of TargetString
.
*
*
* If you're using the AWS CLI or one of the AWS SDKs
*
*
* The value that you want AWS WAF to search for. The SDK
* automatically base64 encodes the value.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ByteMatchTuple withTargetString(java.nio.ByteBuffer targetString) {
setTargetString(targetString);
return this;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on TargetString
before inspecting a request for a
* match.
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system commandline command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
* @see TextTransformation
*/
public void setTextTransformation(String textTransformation) {
this.textTransformation = textTransformation;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* @return Text transformations eliminate some of the unusual formatting
* that attackers use in web requests in an effort to bypass AWS
* WAF. If you specify a transformation, AWS WAF performs the
* transformation on TargetString
before inspecting a
* request for a match.
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system commandline command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the
* following operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than"
* symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
* @see TextTransformation
*/
public String getTextTransformation() {
return this.textTransformation;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on TargetString
before inspecting a request for a
* match.
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system commandline command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see TextTransformation
*/
public ByteMatchTuple withTextTransformation(String textTransformation) {
setTextTransformation(textTransformation);
return this;
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on TargetString
before inspecting a request for a
* match.
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system commandline command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
* @see TextTransformation
*/
public void setTextTransformation(TextTransformation textTransformation) {
this.textTransformation = textTransformation.toString();
}
/**
*
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If you
* specify a transformation, AWS WAF performs the transformation on
* TargetString
before inspecting a request for a match.
*
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating system
* commandline command and using unusual formatting to disguise some or all
* of the command, use this option to perform the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces with one
* space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking space,
* decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal format,
*
(ampersand)#xhhhh;
, with the corresponding characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
*
*
* @param textTransformation
* Text transformations eliminate some of the unusual formatting that
* attackers use in web requests in an effort to bypass AWS WAF. If
* you specify a transformation, AWS WAF performs the transformation
* on TargetString
before inspecting a request for a
* match.
*
* CMD_LINE
*
*
* When you're concerned that attackers are injecting an operating
* system commandline command and using unusual formatting to
* disguise some or all of the command, use this option to perform
* the following transformations:
*
*
* - Delete the following characters: \ " ' ^
* - Delete spaces before the following characters: / (
* - Replace the following characters with a space: , ;
* - Replace multiple spaces with one space
* - Convert uppercase letters (A-Z) to lowercase (a-z)
*
*
* COMPRESS_WHITE_SPACE
*
*
* Use this option to replace the following characters with a space
* character (decimal 32):
*
*
* - \f, formfeed, decimal 12
* - \t, tab, decimal 9
* - \n, newline, decimal 10
* - \r, carriage return, decimal 13
* - \v, vertical tab, decimal 11
* - non-breaking space, decimal 160
*
*
* COMPRESS_WHITE_SPACE
also replaces multiple spaces
* with one space.
*
*
* HTML_ENTITY_DECODE
*
*
* Use this option to replace HTML-encoded characters with unencoded
* characters. HTML_ENTITY_DECODE
performs the following
* operations:
*
*
* - Replaces
(ampersand)quot;
with "
* - Replaces
(ampersand)nbsp;
with a non-breaking
* space, decimal 160
* - Replaces
(ampersand)lt;
with a "less than" symbol
*
* - Replaces
(ampersand)gt;
with >
* - Replaces characters that are represented in hexadecimal
* format,
(ampersand)#xhhhh;
, with the corresponding
* characters
* - Replaces characters that are represented in decimal format,
*
(ampersand)#nnnn;
, with the corresponding characters
*
*
* LOWERCASE
*
*
* Use this option to convert uppercase letters (A-Z) to lowercase
* (a-z).
*
*
* URL_DECODE
*
*
* Use this option to decode a URL-encoded value.
*
*
* NONE
*
*
* Specify NONE
if you don't want to perform any text
* transformations.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see TextTransformation
*/
public ByteMatchTuple withTextTransformation(
TextTransformation textTransformation) {
setTextTransformation(textTransformation);
return this;
}
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*
* @param positionalConstraint
* Within the portion of a web request that you want to search (for
* example, in the query string, if any), specify where you want AWS
* WAF to search. Valid values include the following:
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must
* contain only alphanumeric characters or underscore (A-Z, a-z, 0-9,
* or _). In addition, TargetString
must be a word,
* which means one of the following:
*
*
* TargetString
exactly matches the value of the
* specified part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified
* part of the web request and is followed by a character other than
* an alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part
* of the web request and is preceded by a character other than an
* alphanumeric character or underscore (_), for example,
* ;BadBot
.
* TargetString
is in the middle of the specified
* part of the web request and is preceded and followed by characters
* other than alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly
* match the value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the
* beginning of the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of
* the specified part of the web request.
* @see PositionalConstraint
*/
public void setPositionalConstraint(String positionalConstraint) {
this.positionalConstraint = positionalConstraint;
}
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*
* @return Within the portion of a web request that you want to search (for
* example, in the query string, if any), specify where you want AWS
* WAF to search. Valid values include the following:
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must
* contain only alphanumeric characters or underscore (A-Z, a-z,
* 0-9, or _). In addition, TargetString
must be a
* word, which means one of the following:
*
*
* TargetString
exactly matches the value of the
* specified part of the web request, such as the value of a header.
*
* TargetString
is at the beginning of the
* specified part of the web request and is followed by a character
* other than an alphanumeric character or underscore (_), for
* example, BadBot;
.
* TargetString
is at the end of the specified part
* of the web request and is preceded by a character other than an
* alphanumeric character or underscore (_), for example,
* ;BadBot
.
* TargetString
is in the middle of the specified
* part of the web request and is preceded and followed by
* characters other than alphanumeric characters or underscore (_),
* for example, -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly
* match the value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the
* beginning of the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of
* the specified part of the web request.
* @see PositionalConstraint
*/
public String getPositionalConstraint() {
return this.positionalConstraint;
}
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*
* @param positionalConstraint
* Within the portion of a web request that you want to search (for
* example, in the query string, if any), specify where you want AWS
* WAF to search. Valid values include the following:
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must
* contain only alphanumeric characters or underscore (A-Z, a-z, 0-9,
* or _). In addition, TargetString
must be a word,
* which means one of the following:
*
*
* TargetString
exactly matches the value of the
* specified part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified
* part of the web request and is followed by a character other than
* an alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part
* of the web request and is preceded by a character other than an
* alphanumeric character or underscore (_), for example,
* ;BadBot
.
* TargetString
is in the middle of the specified
* part of the web request and is preceded and followed by characters
* other than alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly
* match the value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the
* beginning of the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of
* the specified part of the web request.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see PositionalConstraint
*/
public ByteMatchTuple withPositionalConstraint(String positionalConstraint) {
setPositionalConstraint(positionalConstraint);
return this;
}
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*
* @param positionalConstraint
* Within the portion of a web request that you want to search (for
* example, in the query string, if any), specify where you want AWS
* WAF to search. Valid values include the following:
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must
* contain only alphanumeric characters or underscore (A-Z, a-z, 0-9,
* or _). In addition, TargetString
must be a word,
* which means one of the following:
*
*
* TargetString
exactly matches the value of the
* specified part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified
* part of the web request and is followed by a character other than
* an alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part
* of the web request and is preceded by a character other than an
* alphanumeric character or underscore (_), for example,
* ;BadBot
.
* TargetString
is in the middle of the specified
* part of the web request and is preceded and followed by characters
* other than alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly
* match the value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the
* beginning of the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of
* the specified part of the web request.
* @see PositionalConstraint
*/
public void setPositionalConstraint(
PositionalConstraint positionalConstraint) {
this.positionalConstraint = positionalConstraint.toString();
}
/**
*
* Within the portion of a web request that you want to search (for example,
* in the query string, if any), specify where you want AWS WAF to search.
* Valid values include the following:
*
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must contain
* only alphanumeric characters or underscore (A-Z, a-z, 0-9, or _). In
* addition, TargetString
must be a word, which means one of
* the following:
*
*
* TargetString
exactly matches the value of the specified
* part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified part
* of the web request and is followed by a character other than an
* alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part of the
* web request and is preceded by a character other than an alphanumeric
* character or underscore (_), for example, ;BadBot
.
* TargetString
is in the middle of the specified part of
* the web request and is preceded and followed by characters other than
* alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly match the
* value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the beginning of
* the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of the
* specified part of the web request.
*
*
* @param positionalConstraint
* Within the portion of a web request that you want to search (for
* example, in the query string, if any), specify where you want AWS
* WAF to search. Valid values include the following:
*
* CONTAINS
*
*
* The specified part of the web request must include the value of
* TargetString
, but the location doesn't matter.
*
*
* CONTAINS_WORD
*
*
* The specified part of the web request must include the value of
* TargetString
, and TargetString
must
* contain only alphanumeric characters or underscore (A-Z, a-z, 0-9,
* or _). In addition, TargetString
must be a word,
* which means one of the following:
*
*
* TargetString
exactly matches the value of the
* specified part of the web request, such as the value of a header.
* TargetString
is at the beginning of the specified
* part of the web request and is followed by a character other than
* an alphanumeric character or underscore (_), for example,
* BadBot;
.
* TargetString
is at the end of the specified part
* of the web request and is preceded by a character other than an
* alphanumeric character or underscore (_), for example,
* ;BadBot
.
* TargetString
is in the middle of the specified
* part of the web request and is preceded and followed by characters
* other than alphanumeric characters or underscore (_), for example,
* -BadBot;
.
*
*
* EXACTLY
*
*
* The value of the specified part of the web request must exactly
* match the value of TargetString
.
*
*
* STARTS_WITH
*
*
* The value of TargetString
must appear at the
* beginning of the specified part of the web request.
*
*
* ENDS_WITH
*
*
* The value of TargetString
must appear at the end of
* the specified part of the web request.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see PositionalConstraint
*/
public ByteMatchTuple withPositionalConstraint(
PositionalConstraint positionalConstraint) {
setPositionalConstraint(positionalConstraint);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFieldToMatch() != null)
sb.append("FieldToMatch: " + getFieldToMatch() + ",");
if (getTargetString() != null)
sb.append("TargetString: " + getTargetString() + ",");
if (getTextTransformation() != null)
sb.append("TextTransformation: " + getTextTransformation() + ",");
if (getPositionalConstraint() != null)
sb.append("PositionalConstraint: " + getPositionalConstraint());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ByteMatchTuple == false)
return false;
ByteMatchTuple other = (ByteMatchTuple) obj;
if (other.getFieldToMatch() == null ^ this.getFieldToMatch() == null)
return false;
if (other.getFieldToMatch() != null
&& other.getFieldToMatch().equals(this.getFieldToMatch()) == false)
return false;
if (other.getTargetString() == null ^ this.getTargetString() == null)
return false;
if (other.getTargetString() != null
&& other.getTargetString().equals(this.getTargetString()) == false)
return false;
if (other.getTextTransformation() == null
^ this.getTextTransformation() == null)
return false;
if (other.getTextTransformation() != null
&& other.getTextTransformation().equals(
this.getTextTransformation()) == false)
return false;
if (other.getPositionalConstraint() == null
^ this.getPositionalConstraint() == null)
return false;
if (other.getPositionalConstraint() != null
&& other.getPositionalConstraint().equals(
this.getPositionalConstraint()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime
* hashCode
+ ((getFieldToMatch() == null) ? 0 : getFieldToMatch()
.hashCode());
hashCode = prime
* hashCode
+ ((getTargetString() == null) ? 0 : getTargetString()
.hashCode());
hashCode = prime
* hashCode
+ ((getTextTransformation() == null) ? 0
: getTextTransformation().hashCode());
hashCode = prime
* hashCode
+ ((getPositionalConstraint() == null) ? 0
: getPositionalConstraint().hashCode());
return hashCode;
}
@Override
public ByteMatchTuple clone() {
try {
return (ByteMatchTuple) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}