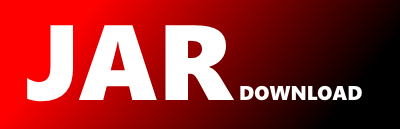
com.amazonaws.services.waf.model.FieldToMatch Maven / Gradle / Ivy
Show all versions of aws-java-sdk-waf Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.waf.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
*
* This is AWS WAF Classic documentation. For more information, see AWS WAF Classic in the
* developer guide.
*
*
* For the latest version of AWS WAF, use the AWS WAFV2 API and see the AWS WAF Developer Guide. With the
* latest version, AWS WAF has a single set of endpoints for regional and global use.
*
*
*
* Specifies where in a web request to look for TargetString
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class FieldToMatch implements Serializable, Cloneable, StructuredPojo {
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*/
private String type;
/**
*
* When the value of Type
is HEADER
, enter the name of the header that you want AWS WAF to
* search, for example, User-Agent
or Referer
. The name of the header is not case
* sensitive.
*
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that you
* want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter name is not
* case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*
*/
private String data;
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*
* @param type
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request
* that you can search include the following:
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the
* header in Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the
* origin to perform. Amazon CloudFront supports the following methods: DELETE
, GET
, HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your
* web server as the HTTP request body, such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as
* UserName or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30
* characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you
* specify in TargetString
.
*
*
* @see MatchFieldType
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*
* @return The part of the web request that you want AWS WAF to search for a specified string. Parts of a request
* that you can search include the following:
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the
* header in Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking
* the origin to perform. Amazon CloudFront supports the following methods: DELETE
,
* GET
, HEAD
, OPTIONS
, PATCH
, POST
, and
* PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your
* web server as the HTTP request body, such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the request body are forwarded to
* AWS WAF for inspection. To allow or block requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as
* UserName or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30
* characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a
* single parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern
* that you specify in TargetString
.
*
*
* @see MatchFieldType
*/
public String getType() {
return this.type;
}
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*
* @param type
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request
* that you can search include the following:
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the
* header in Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the
* origin to perform. Amazon CloudFront supports the following methods: DELETE
, GET
, HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your
* web server as the HTTP request body, such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as
* UserName or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30
* characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you
* specify in TargetString
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MatchFieldType
*/
public FieldToMatch withType(String type) {
setType(type);
return this;
}
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*
* @param type
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request
* that you can search include the following:
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the
* header in Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the
* origin to perform. Amazon CloudFront supports the following methods: DELETE
, GET
, HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your
* web server as the HTTP request body, such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as
* UserName or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30
* characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you
* specify in TargetString
.
*
*
* @see MatchFieldType
*/
public void setType(MatchFieldType type) {
withType(type);
}
/**
*
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request that you
* can search include the following:
*
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the header in
* Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the origin
* to perform. Amazon CloudFront supports the following methods: DELETE
, GET
,
* HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your web
* server as the HTTP request body, such as data from a form. The request body immediately follows the request
* headers. Note that only the first 8192
bytes of the request body are forwarded to AWS WAF for
* inspection. To allow or block requests based on the length of the body, you can create a size constraint set. For
* more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as UserName
* or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30 characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you specify
* in TargetString
.
*
*
*
*
* @param type
* The part of the web request that you want AWS WAF to search for a specified string. Parts of a request
* that you can search include the following:
*
* -
*
* HEADER
: A specified request header, for example, the value of the User-Agent
or
* Referer
header. If you choose HEADER
for the type, specify the name of the
* header in Data
.
*
*
* -
*
* METHOD
: The HTTP method, which indicated the type of operation that the request is asking the
* origin to perform. Amazon CloudFront supports the following methods: DELETE
, GET
, HEAD
, OPTIONS
, PATCH
, POST
, and PUT
.
*
*
* -
*
* QUERY_STRING
: A query string, which is the part of a URL that appears after a ?
* character, if any.
*
*
* -
*
* URI
: The part of a web request that identifies a resource, for example,
* /images/daily-ad.jpg
.
*
*
* -
*
* BODY
: The part of a request that contains any additional data that you want to send to your
* web server as the HTTP request body, such as data from a form. The request body immediately follows the
* request headers. Note that only the first 8192
bytes of the request body are forwarded to AWS
* WAF for inspection. To allow or block requests based on the length of the body, you can create a size
* constraint set. For more information, see CreateSizeConstraintSet.
*
*
* -
*
* SINGLE_QUERY_ARG
: The parameter in the query string that you will inspect, such as
* UserName or SalesRegion. The maximum length for SINGLE_QUERY_ARG
is 30
* characters.
*
*
* -
*
* ALL_QUERY_ARGS
: Similar to SINGLE_QUERY_ARG
, but rather than inspecting a single
* parameter, AWS WAF will inspect all parameters within the query for the value or regex pattern that you
* specify in TargetString
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see MatchFieldType
*/
public FieldToMatch withType(MatchFieldType type) {
this.type = type.toString();
return this;
}
/**
*
* When the value of Type
is HEADER
, enter the name of the header that you want AWS WAF to
* search, for example, User-Agent
or Referer
. The name of the header is not case
* sensitive.
*
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that you
* want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter name is not
* case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*
*
* @param data
* When the value of Type
is HEADER
, enter the name of the header that you want AWS
* WAF to search, for example, User-Agent
or Referer
. The name of the header is not
* case sensitive.
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that
* you want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter
* name is not case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*/
public void setData(String data) {
this.data = data;
}
/**
*
* When the value of Type
is HEADER
, enter the name of the header that you want AWS WAF to
* search, for example, User-Agent
or Referer
. The name of the header is not case
* sensitive.
*
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that you
* want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter name is not
* case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*
*
* @return When the value of Type
is HEADER
, enter the name of the header that you want
* AWS WAF to search, for example, User-Agent
or Referer
. The name of the header
* is not case sensitive.
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter
* that you want AWS WAF to search, for example, UserName
or SalesRegion
. The
* parameter name is not case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*/
public String getData() {
return this.data;
}
/**
*
* When the value of Type
is HEADER
, enter the name of the header that you want AWS WAF to
* search, for example, User-Agent
or Referer
. The name of the header is not case
* sensitive.
*
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that you
* want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter name is not
* case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
*
*
* @param data
* When the value of Type
is HEADER
, enter the name of the header that you want AWS
* WAF to search, for example, User-Agent
or Referer
. The name of the header is not
* case sensitive.
*
* When the value of Type
is SINGLE_QUERY_ARG
, enter the name of the parameter that
* you want AWS WAF to search, for example, UserName
or SalesRegion
. The parameter
* name is not case sensitive.
*
*
* If the value of Type
is any other value, omit Data
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public FieldToMatch withData(String data) {
setData(data);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getType() != null)
sb.append("Type: ").append(getType()).append(",");
if (getData() != null)
sb.append("Data: ").append(getData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof FieldToMatch == false)
return false;
FieldToMatch other = (FieldToMatch) obj;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null && other.getType().equals(this.getType()) == false)
return false;
if (other.getData() == null ^ this.getData() == null)
return false;
if (other.getData() != null && other.getData().equals(this.getData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime * hashCode + ((getData() == null) ? 0 : getData().hashCode());
return hashCode;
}
@Override
public FieldToMatch clone() {
try {
return (FieldToMatch) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.waf.model.waf_regional.transform.FieldToMatchMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}