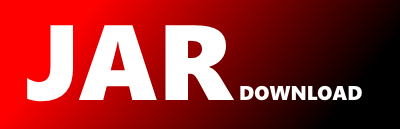
com.amazonaws.services.waf.model.GeoMatchConstraintValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-waf Show documentation
Show all versions of aws-java-sdk-waf Show documentation
The AWS Java SDK for AWS WAF Service module holds the client classes that are used for communicating with AWS WAF Service
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.waf.model;
import javax.annotation.Generated;
/**
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public enum GeoMatchConstraintValue {
AF("AF"),
AX("AX"),
AL("AL"),
DZ("DZ"),
AS("AS"),
AD("AD"),
AO("AO"),
AI("AI"),
AQ("AQ"),
AG("AG"),
AR("AR"),
AM("AM"),
AW("AW"),
AU("AU"),
AT("AT"),
AZ("AZ"),
BS("BS"),
BH("BH"),
BD("BD"),
BB("BB"),
BY("BY"),
BE("BE"),
BZ("BZ"),
BJ("BJ"),
BM("BM"),
BT("BT"),
BO("BO"),
BQ("BQ"),
BA("BA"),
BW("BW"),
BV("BV"),
BR("BR"),
IO("IO"),
BN("BN"),
BG("BG"),
BF("BF"),
BI("BI"),
KH("KH"),
CM("CM"),
CA("CA"),
CV("CV"),
KY("KY"),
CF("CF"),
TD("TD"),
CL("CL"),
CN("CN"),
CX("CX"),
CC("CC"),
CO("CO"),
KM("KM"),
CG("CG"),
CD("CD"),
CK("CK"),
CR("CR"),
CI("CI"),
HR("HR"),
CU("CU"),
CW("CW"),
CY("CY"),
CZ("CZ"),
DK("DK"),
DJ("DJ"),
DM("DM"),
DO("DO"),
EC("EC"),
EG("EG"),
SV("SV"),
GQ("GQ"),
ER("ER"),
EE("EE"),
ET("ET"),
FK("FK"),
FO("FO"),
FJ("FJ"),
FI("FI"),
FR("FR"),
GF("GF"),
PF("PF"),
TF("TF"),
GA("GA"),
GM("GM"),
GE("GE"),
DE("DE"),
GH("GH"),
GI("GI"),
GR("GR"),
GL("GL"),
GD("GD"),
GP("GP"),
GU("GU"),
GT("GT"),
GG("GG"),
GN("GN"),
GW("GW"),
GY("GY"),
HT("HT"),
HM("HM"),
VA("VA"),
HN("HN"),
HK("HK"),
HU("HU"),
IS("IS"),
IN("IN"),
ID("ID"),
IR("IR"),
IQ("IQ"),
IE("IE"),
IM("IM"),
IL("IL"),
IT("IT"),
JM("JM"),
JP("JP"),
JE("JE"),
JO("JO"),
KZ("KZ"),
KE("KE"),
KI("KI"),
KP("KP"),
KR("KR"),
KW("KW"),
KG("KG"),
LA("LA"),
LV("LV"),
LB("LB"),
LS("LS"),
LR("LR"),
LY("LY"),
LI("LI"),
LT("LT"),
LU("LU"),
MO("MO"),
MK("MK"),
MG("MG"),
MW("MW"),
MY("MY"),
MV("MV"),
ML("ML"),
MT("MT"),
MH("MH"),
MQ("MQ"),
MR("MR"),
MU("MU"),
YT("YT"),
MX("MX"),
FM("FM"),
MD("MD"),
MC("MC"),
MN("MN"),
ME("ME"),
MS("MS"),
MA("MA"),
MZ("MZ"),
MM("MM"),
NA("NA"),
NR("NR"),
NP("NP"),
NL("NL"),
NC("NC"),
NZ("NZ"),
NI("NI"),
NE("NE"),
NG("NG"),
NU("NU"),
NF("NF"),
MP("MP"),
NO("NO"),
OM("OM"),
PK("PK"),
PW("PW"),
PS("PS"),
PA("PA"),
PG("PG"),
PY("PY"),
PE("PE"),
PH("PH"),
PN("PN"),
PL("PL"),
PT("PT"),
PR("PR"),
QA("QA"),
RE("RE"),
RO("RO"),
RU("RU"),
RW("RW"),
BL("BL"),
SH("SH"),
KN("KN"),
LC("LC"),
MF("MF"),
PM("PM"),
VC("VC"),
WS("WS"),
SM("SM"),
ST("ST"),
SA("SA"),
SN("SN"),
RS("RS"),
SC("SC"),
SL("SL"),
SG("SG"),
SX("SX"),
SK("SK"),
SI("SI"),
SB("SB"),
SO("SO"),
ZA("ZA"),
GS("GS"),
SS("SS"),
ES("ES"),
LK("LK"),
SD("SD"),
SR("SR"),
SJ("SJ"),
SZ("SZ"),
SE("SE"),
CH("CH"),
SY("SY"),
TW("TW"),
TJ("TJ"),
TZ("TZ"),
TH("TH"),
TL("TL"),
TG("TG"),
TK("TK"),
TO("TO"),
TT("TT"),
TN("TN"),
TR("TR"),
TM("TM"),
TC("TC"),
TV("TV"),
UG("UG"),
UA("UA"),
AE("AE"),
GB("GB"),
US("US"),
UM("UM"),
UY("UY"),
UZ("UZ"),
VU("VU"),
VE("VE"),
VN("VN"),
VG("VG"),
VI("VI"),
WF("WF"),
EH("EH"),
YE("YE"),
ZM("ZM"),
ZW("ZW");
private String value;
private GeoMatchConstraintValue(String value) {
this.value = value;
}
@Override
public String toString() {
return this.value;
}
/**
* Use this in place of valueOf.
*
* @param value
* real value
* @return GeoMatchConstraintValue corresponding to the value
*
* @throws IllegalArgumentException
* If the specified value does not map to one of the known values in this enum.
*/
public static GeoMatchConstraintValue fromValue(String value) {
if (value == null || "".equals(value)) {
throw new IllegalArgumentException("Value cannot be null or empty!");
}
for (GeoMatchConstraintValue enumEntry : GeoMatchConstraintValue.values()) {
if (enumEntry.toString().equals(value)) {
return enumEntry;
}
}
throw new IllegalArgumentException("Cannot create enum from " + value + " value!");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy